Check something exists in array before executing
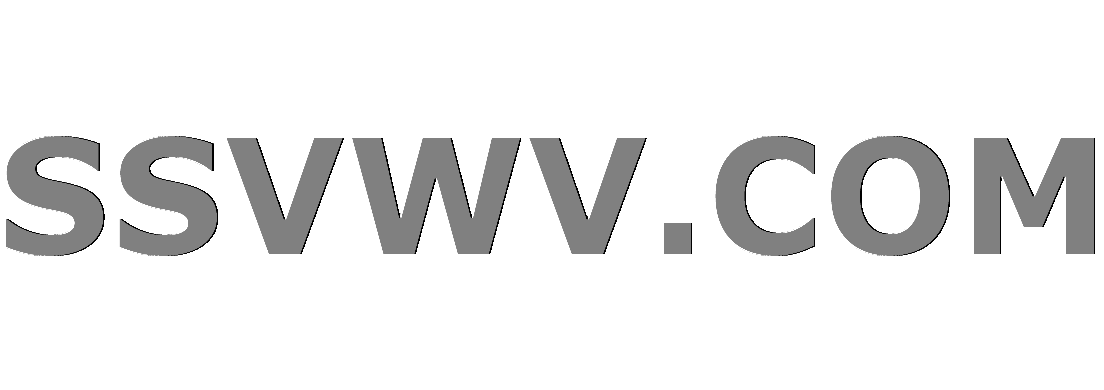
Multi tool use
I have a array below that is constantly changing. It is being using in a node.js app. I have everything working good that updates elements of the array but if I restart node and the browser is still running, the webpage still sends data and causes it to crash, so I need to check if array exists before it tries to do something.
My array is like this, called users :-
[ { username: 'a',
active: true,
lat: '52.4099584',
lng: '-1.5310848' } ]
So I need to check username 'a' exists. I have tried
users.map(obj => obj.username).indexOf(value) >= 0;
but doesnt work?
Any suggestions?
Thanks
javascript jquery
|
show 1 more comment
I have a array below that is constantly changing. It is being using in a node.js app. I have everything working good that updates elements of the array but if I restart node and the browser is still running, the webpage still sends data and causes it to crash, so I need to check if array exists before it tries to do something.
My array is like this, called users :-
[ { username: 'a',
active: true,
lat: '52.4099584',
lng: '-1.5310848' } ]
So I need to check username 'a' exists. I have tried
users.map(obj => obj.username).indexOf(value) >= 0;
but doesnt work?
Any suggestions?
Thanks
javascript jquery
3
users.some( obj => obj.username === 'a' )
?
– David Thomas
Nov 24 '18 at 21:48
It was on here somewhere, supposed to give a true or false if exists
– larry chambers
Nov 24 '18 at 21:49
1
return users.find(user => user.username === 'a'); or as Thomas wrote. return users.some(user => user.username);
– Adam Orlov
Nov 24 '18 at 21:52
1
Seems to work fine
– trincot
Nov 24 '18 at 21:56
Wierd, that works great in jsfiddle but I get this error when added to my script result = users.map(obj => obj.username).indexOf(value) >= 0; ^ ReferenceError: value is not defined
– larry chambers
Nov 24 '18 at 22:10
|
show 1 more comment
I have a array below that is constantly changing. It is being using in a node.js app. I have everything working good that updates elements of the array but if I restart node and the browser is still running, the webpage still sends data and causes it to crash, so I need to check if array exists before it tries to do something.
My array is like this, called users :-
[ { username: 'a',
active: true,
lat: '52.4099584',
lng: '-1.5310848' } ]
So I need to check username 'a' exists. I have tried
users.map(obj => obj.username).indexOf(value) >= 0;
but doesnt work?
Any suggestions?
Thanks
javascript jquery
I have a array below that is constantly changing. It is being using in a node.js app. I have everything working good that updates elements of the array but if I restart node and the browser is still running, the webpage still sends data and causes it to crash, so I need to check if array exists before it tries to do something.
My array is like this, called users :-
[ { username: 'a',
active: true,
lat: '52.4099584',
lng: '-1.5310848' } ]
So I need to check username 'a' exists. I have tried
users.map(obj => obj.username).indexOf(value) >= 0;
but doesnt work?
Any suggestions?
Thanks
javascript jquery
javascript jquery
asked Nov 24 '18 at 21:45
larry chamberslarry chambers
949
949
3
users.some( obj => obj.username === 'a' )
?
– David Thomas
Nov 24 '18 at 21:48
It was on here somewhere, supposed to give a true or false if exists
– larry chambers
Nov 24 '18 at 21:49
1
return users.find(user => user.username === 'a'); or as Thomas wrote. return users.some(user => user.username);
– Adam Orlov
Nov 24 '18 at 21:52
1
Seems to work fine
– trincot
Nov 24 '18 at 21:56
Wierd, that works great in jsfiddle but I get this error when added to my script result = users.map(obj => obj.username).indexOf(value) >= 0; ^ ReferenceError: value is not defined
– larry chambers
Nov 24 '18 at 22:10
|
show 1 more comment
3
users.some( obj => obj.username === 'a' )
?
– David Thomas
Nov 24 '18 at 21:48
It was on here somewhere, supposed to give a true or false if exists
– larry chambers
Nov 24 '18 at 21:49
1
return users.find(user => user.username === 'a'); or as Thomas wrote. return users.some(user => user.username);
– Adam Orlov
Nov 24 '18 at 21:52
1
Seems to work fine
– trincot
Nov 24 '18 at 21:56
Wierd, that works great in jsfiddle but I get this error when added to my script result = users.map(obj => obj.username).indexOf(value) >= 0; ^ ReferenceError: value is not defined
– larry chambers
Nov 24 '18 at 22:10
3
3
users.some( obj => obj.username === 'a' )
?– David Thomas
Nov 24 '18 at 21:48
users.some( obj => obj.username === 'a' )
?– David Thomas
Nov 24 '18 at 21:48
It was on here somewhere, supposed to give a true or false if exists
– larry chambers
Nov 24 '18 at 21:49
It was on here somewhere, supposed to give a true or false if exists
– larry chambers
Nov 24 '18 at 21:49
1
1
return users.find(user => user.username === 'a'); or as Thomas wrote. return users.some(user => user.username);
– Adam Orlov
Nov 24 '18 at 21:52
return users.find(user => user.username === 'a'); or as Thomas wrote. return users.some(user => user.username);
– Adam Orlov
Nov 24 '18 at 21:52
1
1
Seems to work fine
– trincot
Nov 24 '18 at 21:56
Seems to work fine
– trincot
Nov 24 '18 at 21:56
Wierd, that works great in jsfiddle but I get this error when added to my script result = users.map(obj => obj.username).indexOf(value) >= 0; ^ ReferenceError: value is not defined
– larry chambers
Nov 24 '18 at 22:10
Wierd, that works great in jsfiddle but I get this error when added to my script result = users.map(obj => obj.username).indexOf(value) >= 0; ^ ReferenceError: value is not defined
– larry chambers
Nov 24 '18 at 22:10
|
show 1 more comment
1 Answer
1
active
oldest
votes
If you're looking to remve the users that don't have a username, then you could do something like this.
const data = [{
username: 'a',
active: true,
lat: '52.4099584',
lng: '-1.5310848' },
{
username: undefined,
active: true,
lat: '52.4099584',
lng: '-1.5310848' }];
console.log(data.filter(({username})=> username && username !== null));
Otherwise, you should just use something like every
const data = [ {
username: undefined,
active: true,
lat: '52.4099584',
lng: '-1.5310848' },
{
username: 'a',
active: true,
lat: '52.4099584',
lng: '-1.5310848' }];
console.log(data.every(({username})=> username && username !== null));
Or findIndex
const data = [ {
username: undefined,
active: true,
lat: '52.4099584',
lng: '-1.5310848' },
{
username: 'a',
active: true,
lat: '52.4099584',
lng: '-1.5310848' }];
console.log(data.findIndex(({username})=> !username || username === null) > -1);
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53462640%2fcheck-something-exists-in-array-before-executing%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
If you're looking to remve the users that don't have a username, then you could do something like this.
const data = [{
username: 'a',
active: true,
lat: '52.4099584',
lng: '-1.5310848' },
{
username: undefined,
active: true,
lat: '52.4099584',
lng: '-1.5310848' }];
console.log(data.filter(({username})=> username && username !== null));
Otherwise, you should just use something like every
const data = [ {
username: undefined,
active: true,
lat: '52.4099584',
lng: '-1.5310848' },
{
username: 'a',
active: true,
lat: '52.4099584',
lng: '-1.5310848' }];
console.log(data.every(({username})=> username && username !== null));
Or findIndex
const data = [ {
username: undefined,
active: true,
lat: '52.4099584',
lng: '-1.5310848' },
{
username: 'a',
active: true,
lat: '52.4099584',
lng: '-1.5310848' }];
console.log(data.findIndex(({username})=> !username || username === null) > -1);
add a comment |
If you're looking to remve the users that don't have a username, then you could do something like this.
const data = [{
username: 'a',
active: true,
lat: '52.4099584',
lng: '-1.5310848' },
{
username: undefined,
active: true,
lat: '52.4099584',
lng: '-1.5310848' }];
console.log(data.filter(({username})=> username && username !== null));
Otherwise, you should just use something like every
const data = [ {
username: undefined,
active: true,
lat: '52.4099584',
lng: '-1.5310848' },
{
username: 'a',
active: true,
lat: '52.4099584',
lng: '-1.5310848' }];
console.log(data.every(({username})=> username && username !== null));
Or findIndex
const data = [ {
username: undefined,
active: true,
lat: '52.4099584',
lng: '-1.5310848' },
{
username: 'a',
active: true,
lat: '52.4099584',
lng: '-1.5310848' }];
console.log(data.findIndex(({username})=> !username || username === null) > -1);
add a comment |
If you're looking to remve the users that don't have a username, then you could do something like this.
const data = [{
username: 'a',
active: true,
lat: '52.4099584',
lng: '-1.5310848' },
{
username: undefined,
active: true,
lat: '52.4099584',
lng: '-1.5310848' }];
console.log(data.filter(({username})=> username && username !== null));
Otherwise, you should just use something like every
const data = [ {
username: undefined,
active: true,
lat: '52.4099584',
lng: '-1.5310848' },
{
username: 'a',
active: true,
lat: '52.4099584',
lng: '-1.5310848' }];
console.log(data.every(({username})=> username && username !== null));
Or findIndex
const data = [ {
username: undefined,
active: true,
lat: '52.4099584',
lng: '-1.5310848' },
{
username: 'a',
active: true,
lat: '52.4099584',
lng: '-1.5310848' }];
console.log(data.findIndex(({username})=> !username || username === null) > -1);
If you're looking to remve the users that don't have a username, then you could do something like this.
const data = [{
username: 'a',
active: true,
lat: '52.4099584',
lng: '-1.5310848' },
{
username: undefined,
active: true,
lat: '52.4099584',
lng: '-1.5310848' }];
console.log(data.filter(({username})=> username && username !== null));
Otherwise, you should just use something like every
const data = [ {
username: undefined,
active: true,
lat: '52.4099584',
lng: '-1.5310848' },
{
username: 'a',
active: true,
lat: '52.4099584',
lng: '-1.5310848' }];
console.log(data.every(({username})=> username && username !== null));
Or findIndex
const data = [ {
username: undefined,
active: true,
lat: '52.4099584',
lng: '-1.5310848' },
{
username: 'a',
active: true,
lat: '52.4099584',
lng: '-1.5310848' }];
console.log(data.findIndex(({username})=> !username || username === null) > -1);
const data = [{
username: 'a',
active: true,
lat: '52.4099584',
lng: '-1.5310848' },
{
username: undefined,
active: true,
lat: '52.4099584',
lng: '-1.5310848' }];
console.log(data.filter(({username})=> username && username !== null));
const data = [{
username: 'a',
active: true,
lat: '52.4099584',
lng: '-1.5310848' },
{
username: undefined,
active: true,
lat: '52.4099584',
lng: '-1.5310848' }];
console.log(data.filter(({username})=> username && username !== null));
const data = [ {
username: undefined,
active: true,
lat: '52.4099584',
lng: '-1.5310848' },
{
username: 'a',
active: true,
lat: '52.4099584',
lng: '-1.5310848' }];
console.log(data.every(({username})=> username && username !== null));
const data = [ {
username: undefined,
active: true,
lat: '52.4099584',
lng: '-1.5310848' },
{
username: 'a',
active: true,
lat: '52.4099584',
lng: '-1.5310848' }];
console.log(data.every(({username})=> username && username !== null));
const data = [ {
username: undefined,
active: true,
lat: '52.4099584',
lng: '-1.5310848' },
{
username: 'a',
active: true,
lat: '52.4099584',
lng: '-1.5310848' }];
console.log(data.findIndex(({username})=> !username || username === null) > -1);
const data = [ {
username: undefined,
active: true,
lat: '52.4099584',
lng: '-1.5310848' },
{
username: 'a',
active: true,
lat: '52.4099584',
lng: '-1.5310848' }];
console.log(data.findIndex(({username})=> !username || username === null) > -1);
answered Nov 24 '18 at 21:53


kemicofakemicofa
10.6k43983
10.6k43983
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53462640%2fcheck-something-exists-in-array-before-executing%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
xK,UYyAW u7DuChvafp
3
users.some( obj => obj.username === 'a' )
?– David Thomas
Nov 24 '18 at 21:48
It was on here somewhere, supposed to give a true or false if exists
– larry chambers
Nov 24 '18 at 21:49
1
return users.find(user => user.username === 'a'); or as Thomas wrote. return users.some(user => user.username);
– Adam Orlov
Nov 24 '18 at 21:52
1
Seems to work fine
– trincot
Nov 24 '18 at 21:56
Wierd, that works great in jsfiddle but I get this error when added to my script result = users.map(obj => obj.username).indexOf(value) >= 0; ^ ReferenceError: value is not defined
– larry chambers
Nov 24 '18 at 22:10