Quicksort from C to MIPS - How to pass parameters and maintaining variables for stack frame?
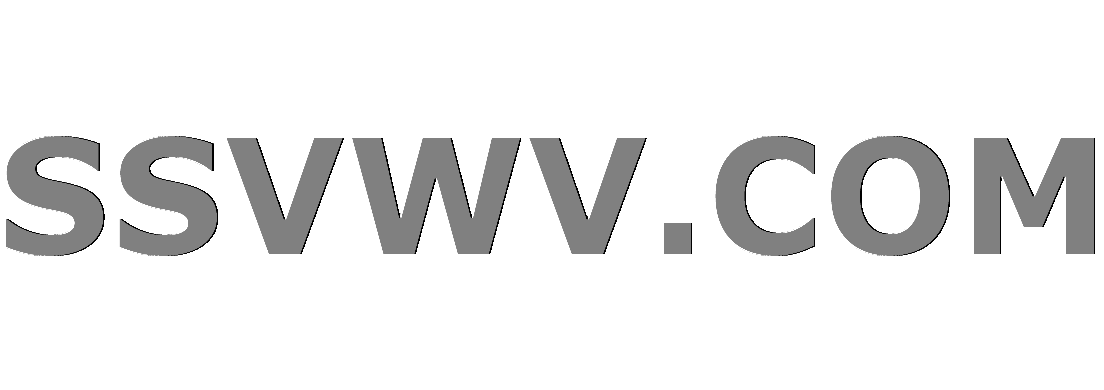
Multi tool use
I am creating a Quicksort algorithm on an array of integers. I am using this C algorithm and translating it into MIPS. However, MIPS and recursion is very tough indeed.
I am unsure how to send parameters into the recursive call QS. I recently discovered that I can change my $s registers for each frame in the call stack, by moving the stack pointer 4 bytes. This will allow me to change the $s registers for each stack frame such that I don't need a million variables for each QS frame.
My problem is that I don't really understand how and when to set and get these $sx values during recursion.
c recursion parameters mips quicksort
add a comment |
I am creating a Quicksort algorithm on an array of integers. I am using this C algorithm and translating it into MIPS. However, MIPS and recursion is very tough indeed.
I am unsure how to send parameters into the recursive call QS. I recently discovered that I can change my $s registers for each frame in the call stack, by moving the stack pointer 4 bytes. This will allow me to change the $s registers for each stack frame such that I don't need a million variables for each QS frame.
My problem is that I don't really understand how and when to set and get these $sx values during recursion.
c recursion parameters mips quicksort
Why don't you just compile your C code with a MIPS cross-compiler, as virtually everyone else does?
– Kit.
Nov 24 '18 at 22:19
this C algorithm and translating it into MIPS
What C algorithm? Usually a compiler translates C code into MIPS.
– Kamil Cuk
Nov 24 '18 at 22:37
add a comment |
I am creating a Quicksort algorithm on an array of integers. I am using this C algorithm and translating it into MIPS. However, MIPS and recursion is very tough indeed.
I am unsure how to send parameters into the recursive call QS. I recently discovered that I can change my $s registers for each frame in the call stack, by moving the stack pointer 4 bytes. This will allow me to change the $s registers for each stack frame such that I don't need a million variables for each QS frame.
My problem is that I don't really understand how and when to set and get these $sx values during recursion.
c recursion parameters mips quicksort
I am creating a Quicksort algorithm on an array of integers. I am using this C algorithm and translating it into MIPS. However, MIPS and recursion is very tough indeed.
I am unsure how to send parameters into the recursive call QS. I recently discovered that I can change my $s registers for each frame in the call stack, by moving the stack pointer 4 bytes. This will allow me to change the $s registers for each stack frame such that I don't need a million variables for each QS frame.
My problem is that I don't really understand how and when to set and get these $sx values during recursion.
c recursion parameters mips quicksort
c recursion parameters mips quicksort
edited Nov 27 '18 at 4:09
MontgommeryJR
asked Nov 24 '18 at 21:33
MontgommeryJRMontgommeryJR
10510
10510
Why don't you just compile your C code with a MIPS cross-compiler, as virtually everyone else does?
– Kit.
Nov 24 '18 at 22:19
this C algorithm and translating it into MIPS
What C algorithm? Usually a compiler translates C code into MIPS.
– Kamil Cuk
Nov 24 '18 at 22:37
add a comment |
Why don't you just compile your C code with a MIPS cross-compiler, as virtually everyone else does?
– Kit.
Nov 24 '18 at 22:19
this C algorithm and translating it into MIPS
What C algorithm? Usually a compiler translates C code into MIPS.
– Kamil Cuk
Nov 24 '18 at 22:37
Why don't you just compile your C code with a MIPS cross-compiler, as virtually everyone else does?
– Kit.
Nov 24 '18 at 22:19
Why don't you just compile your C code with a MIPS cross-compiler, as virtually everyone else does?
– Kit.
Nov 24 '18 at 22:19
this C algorithm and translating it into MIPS
What C algorithm? Usually a compiler translates C code into MIPS.– Kamil Cuk
Nov 24 '18 at 22:37
this C algorithm and translating it into MIPS
What C algorithm? Usually a compiler translates C code into MIPS.– Kamil Cuk
Nov 24 '18 at 22:37
add a comment |
1 Answer
1
active
oldest
votes
Recursion is implemented by moving the stack pointer register ($sp).
First of all, let's understand the point of moving the stack pointer:
When you use recursion in a high level language, what it does, basically, is to "save" the state of the current function call in the "stack memory".
To achieve this, you will have to:
- Save the current state of your program (all the variables/registers
you are using within the scope of the "function"), in the stack memory; - Call the function "recursively" (which might modify all the registers you were using);
- When the function finishes, you have to restore the previous state and "free" the space you allocated.
But besides that, we have to save the value of $ra, to keep track of where we're supposed to go when the upper function ends.
Here's a simple example of a program that calculates factorial(n) recursively:
.text
main:
# Calls Fact with Input ($a0) N = 10
li $a0, 10
jal fact
# prints the Output ($v0) Factorial(N)
move $a0, $v0
li $v0, 1
syscall
# exit
li $v0, 10
syscall
# Input: $a0 - N
# Output: $v0 - Factorial(N)
fact:
# Fact(0) = 1
beq $a0, 0, r_one
# Fact(N) = N * Fact(N-1) use recursion
# allocate 8 bytes in the stack for storing N, and $ra
addi $sp, $sp, -8
# stores N in the first, and $ra in the last position
sw $a0, 4($sp)
sw $ra, 0($sp)
# call Fact(N-1)
addi $a0, $a0, -1
jal fact
# Restore the values of N and $ra
lw $a0, 4($sp)
lw $ra, 0($sp)
# Free the 8 bytes used
addi $sp, $sp, 8
# Set the return value to be N * Fact(N-1) and return
mul $v0, $a0, $v0
jr $ra
# return 1;
r_one:
li $v0, 1
jr $ra
This is what you should keep in mind when implementing your code, basically.
Just pay attention to:
- The stack pointer is decremented;
- How many bytes you need to allocate. In this example I use 2 32-bits integers, 8 bytes in total. It will deppend on how many variables you need to store, and their size.
- How to access them with lw and sw, using the correct index. Also, be aware of memory alignment;
- This does not only apply for recursion. You can use the stack memory to call another function that uses registers that are being used (basically the same thing as recursion, except that you don't need to save $ra). And also store an array, a struct, etc.
Edit:
Some considerations:
- The right place to do that is where your code calls the function (allocate and save), and after this call (restore and free).
- Understand your code to know which variables need to be saved (might be used).
Very helpful! I am having trouble understanding the relation between the $ra and the $s0 registers. For instance if my $sp is -4 and I want to store my variables in that particular frame in $s0:addi $sp, $sp, -4
sw $s3, 0($sp)
sw $s2, 0($sp)
sw $s1, 0($sp)
sw $s4, 0($sp)
sw $s5, 0($sp)
then how would I send those variables into the next frame when$sp
is -8
– MontgommeryJR
Nov 25 '18 at 20:10
There are different usages for MIPS registers, as described here. I hope this clarifies your doubt. In your example, you are trying to save the value of 5 registers (all 4 bytes, I assume). The wrong thing on your example is: You are not allocating enough space (which would be a total of 20 bytes), and you are storing them all in the same memory address. Here's the correction:addi $sp, $sp, -20 sw $s3, 0($sp) sw $s2, 4($sp) sw $s1, 8($sp) sw $s4, 12($sp) sw $s5, 16($sp)
– Wilson Neto
Nov 25 '18 at 22:08
Thank you for the clarification. Although I still cannot seem to implement it the way everyone is suggesting online. I feel like I didn't set/reset these $sx variables to their appropriate frame in the right spot of my code. Im really unsure here. Can you have a look at what I have? I have added my full code
– MontgommeryJR
Nov 26 '18 at 2:29
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53462557%2fquicksort-from-c-to-mips-how-to-pass-parameters-and-maintaining-variables-for%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Recursion is implemented by moving the stack pointer register ($sp).
First of all, let's understand the point of moving the stack pointer:
When you use recursion in a high level language, what it does, basically, is to "save" the state of the current function call in the "stack memory".
To achieve this, you will have to:
- Save the current state of your program (all the variables/registers
you are using within the scope of the "function"), in the stack memory; - Call the function "recursively" (which might modify all the registers you were using);
- When the function finishes, you have to restore the previous state and "free" the space you allocated.
But besides that, we have to save the value of $ra, to keep track of where we're supposed to go when the upper function ends.
Here's a simple example of a program that calculates factorial(n) recursively:
.text
main:
# Calls Fact with Input ($a0) N = 10
li $a0, 10
jal fact
# prints the Output ($v0) Factorial(N)
move $a0, $v0
li $v0, 1
syscall
# exit
li $v0, 10
syscall
# Input: $a0 - N
# Output: $v0 - Factorial(N)
fact:
# Fact(0) = 1
beq $a0, 0, r_one
# Fact(N) = N * Fact(N-1) use recursion
# allocate 8 bytes in the stack for storing N, and $ra
addi $sp, $sp, -8
# stores N in the first, and $ra in the last position
sw $a0, 4($sp)
sw $ra, 0($sp)
# call Fact(N-1)
addi $a0, $a0, -1
jal fact
# Restore the values of N and $ra
lw $a0, 4($sp)
lw $ra, 0($sp)
# Free the 8 bytes used
addi $sp, $sp, 8
# Set the return value to be N * Fact(N-1) and return
mul $v0, $a0, $v0
jr $ra
# return 1;
r_one:
li $v0, 1
jr $ra
This is what you should keep in mind when implementing your code, basically.
Just pay attention to:
- The stack pointer is decremented;
- How many bytes you need to allocate. In this example I use 2 32-bits integers, 8 bytes in total. It will deppend on how many variables you need to store, and their size.
- How to access them with lw and sw, using the correct index. Also, be aware of memory alignment;
- This does not only apply for recursion. You can use the stack memory to call another function that uses registers that are being used (basically the same thing as recursion, except that you don't need to save $ra). And also store an array, a struct, etc.
Edit:
Some considerations:
- The right place to do that is where your code calls the function (allocate and save), and after this call (restore and free).
- Understand your code to know which variables need to be saved (might be used).
Very helpful! I am having trouble understanding the relation between the $ra and the $s0 registers. For instance if my $sp is -4 and I want to store my variables in that particular frame in $s0:addi $sp, $sp, -4
sw $s3, 0($sp)
sw $s2, 0($sp)
sw $s1, 0($sp)
sw $s4, 0($sp)
sw $s5, 0($sp)
then how would I send those variables into the next frame when$sp
is -8
– MontgommeryJR
Nov 25 '18 at 20:10
There are different usages for MIPS registers, as described here. I hope this clarifies your doubt. In your example, you are trying to save the value of 5 registers (all 4 bytes, I assume). The wrong thing on your example is: You are not allocating enough space (which would be a total of 20 bytes), and you are storing them all in the same memory address. Here's the correction:addi $sp, $sp, -20 sw $s3, 0($sp) sw $s2, 4($sp) sw $s1, 8($sp) sw $s4, 12($sp) sw $s5, 16($sp)
– Wilson Neto
Nov 25 '18 at 22:08
Thank you for the clarification. Although I still cannot seem to implement it the way everyone is suggesting online. I feel like I didn't set/reset these $sx variables to their appropriate frame in the right spot of my code. Im really unsure here. Can you have a look at what I have? I have added my full code
– MontgommeryJR
Nov 26 '18 at 2:29
add a comment |
Recursion is implemented by moving the stack pointer register ($sp).
First of all, let's understand the point of moving the stack pointer:
When you use recursion in a high level language, what it does, basically, is to "save" the state of the current function call in the "stack memory".
To achieve this, you will have to:
- Save the current state of your program (all the variables/registers
you are using within the scope of the "function"), in the stack memory; - Call the function "recursively" (which might modify all the registers you were using);
- When the function finishes, you have to restore the previous state and "free" the space you allocated.
But besides that, we have to save the value of $ra, to keep track of where we're supposed to go when the upper function ends.
Here's a simple example of a program that calculates factorial(n) recursively:
.text
main:
# Calls Fact with Input ($a0) N = 10
li $a0, 10
jal fact
# prints the Output ($v0) Factorial(N)
move $a0, $v0
li $v0, 1
syscall
# exit
li $v0, 10
syscall
# Input: $a0 - N
# Output: $v0 - Factorial(N)
fact:
# Fact(0) = 1
beq $a0, 0, r_one
# Fact(N) = N * Fact(N-1) use recursion
# allocate 8 bytes in the stack for storing N, and $ra
addi $sp, $sp, -8
# stores N in the first, and $ra in the last position
sw $a0, 4($sp)
sw $ra, 0($sp)
# call Fact(N-1)
addi $a0, $a0, -1
jal fact
# Restore the values of N and $ra
lw $a0, 4($sp)
lw $ra, 0($sp)
# Free the 8 bytes used
addi $sp, $sp, 8
# Set the return value to be N * Fact(N-1) and return
mul $v0, $a0, $v0
jr $ra
# return 1;
r_one:
li $v0, 1
jr $ra
This is what you should keep in mind when implementing your code, basically.
Just pay attention to:
- The stack pointer is decremented;
- How many bytes you need to allocate. In this example I use 2 32-bits integers, 8 bytes in total. It will deppend on how many variables you need to store, and their size.
- How to access them with lw and sw, using the correct index. Also, be aware of memory alignment;
- This does not only apply for recursion. You can use the stack memory to call another function that uses registers that are being used (basically the same thing as recursion, except that you don't need to save $ra). And also store an array, a struct, etc.
Edit:
Some considerations:
- The right place to do that is where your code calls the function (allocate and save), and after this call (restore and free).
- Understand your code to know which variables need to be saved (might be used).
Very helpful! I am having trouble understanding the relation between the $ra and the $s0 registers. For instance if my $sp is -4 and I want to store my variables in that particular frame in $s0:addi $sp, $sp, -4
sw $s3, 0($sp)
sw $s2, 0($sp)
sw $s1, 0($sp)
sw $s4, 0($sp)
sw $s5, 0($sp)
then how would I send those variables into the next frame when$sp
is -8
– MontgommeryJR
Nov 25 '18 at 20:10
There are different usages for MIPS registers, as described here. I hope this clarifies your doubt. In your example, you are trying to save the value of 5 registers (all 4 bytes, I assume). The wrong thing on your example is: You are not allocating enough space (which would be a total of 20 bytes), and you are storing them all in the same memory address. Here's the correction:addi $sp, $sp, -20 sw $s3, 0($sp) sw $s2, 4($sp) sw $s1, 8($sp) sw $s4, 12($sp) sw $s5, 16($sp)
– Wilson Neto
Nov 25 '18 at 22:08
Thank you for the clarification. Although I still cannot seem to implement it the way everyone is suggesting online. I feel like I didn't set/reset these $sx variables to their appropriate frame in the right spot of my code. Im really unsure here. Can you have a look at what I have? I have added my full code
– MontgommeryJR
Nov 26 '18 at 2:29
add a comment |
Recursion is implemented by moving the stack pointer register ($sp).
First of all, let's understand the point of moving the stack pointer:
When you use recursion in a high level language, what it does, basically, is to "save" the state of the current function call in the "stack memory".
To achieve this, you will have to:
- Save the current state of your program (all the variables/registers
you are using within the scope of the "function"), in the stack memory; - Call the function "recursively" (which might modify all the registers you were using);
- When the function finishes, you have to restore the previous state and "free" the space you allocated.
But besides that, we have to save the value of $ra, to keep track of where we're supposed to go when the upper function ends.
Here's a simple example of a program that calculates factorial(n) recursively:
.text
main:
# Calls Fact with Input ($a0) N = 10
li $a0, 10
jal fact
# prints the Output ($v0) Factorial(N)
move $a0, $v0
li $v0, 1
syscall
# exit
li $v0, 10
syscall
# Input: $a0 - N
# Output: $v0 - Factorial(N)
fact:
# Fact(0) = 1
beq $a0, 0, r_one
# Fact(N) = N * Fact(N-1) use recursion
# allocate 8 bytes in the stack for storing N, and $ra
addi $sp, $sp, -8
# stores N in the first, and $ra in the last position
sw $a0, 4($sp)
sw $ra, 0($sp)
# call Fact(N-1)
addi $a0, $a0, -1
jal fact
# Restore the values of N and $ra
lw $a0, 4($sp)
lw $ra, 0($sp)
# Free the 8 bytes used
addi $sp, $sp, 8
# Set the return value to be N * Fact(N-1) and return
mul $v0, $a0, $v0
jr $ra
# return 1;
r_one:
li $v0, 1
jr $ra
This is what you should keep in mind when implementing your code, basically.
Just pay attention to:
- The stack pointer is decremented;
- How many bytes you need to allocate. In this example I use 2 32-bits integers, 8 bytes in total. It will deppend on how many variables you need to store, and their size.
- How to access them with lw and sw, using the correct index. Also, be aware of memory alignment;
- This does not only apply for recursion. You can use the stack memory to call another function that uses registers that are being used (basically the same thing as recursion, except that you don't need to save $ra). And also store an array, a struct, etc.
Edit:
Some considerations:
- The right place to do that is where your code calls the function (allocate and save), and after this call (restore and free).
- Understand your code to know which variables need to be saved (might be used).
Recursion is implemented by moving the stack pointer register ($sp).
First of all, let's understand the point of moving the stack pointer:
When you use recursion in a high level language, what it does, basically, is to "save" the state of the current function call in the "stack memory".
To achieve this, you will have to:
- Save the current state of your program (all the variables/registers
you are using within the scope of the "function"), in the stack memory; - Call the function "recursively" (which might modify all the registers you were using);
- When the function finishes, you have to restore the previous state and "free" the space you allocated.
But besides that, we have to save the value of $ra, to keep track of where we're supposed to go when the upper function ends.
Here's a simple example of a program that calculates factorial(n) recursively:
.text
main:
# Calls Fact with Input ($a0) N = 10
li $a0, 10
jal fact
# prints the Output ($v0) Factorial(N)
move $a0, $v0
li $v0, 1
syscall
# exit
li $v0, 10
syscall
# Input: $a0 - N
# Output: $v0 - Factorial(N)
fact:
# Fact(0) = 1
beq $a0, 0, r_one
# Fact(N) = N * Fact(N-1) use recursion
# allocate 8 bytes in the stack for storing N, and $ra
addi $sp, $sp, -8
# stores N in the first, and $ra in the last position
sw $a0, 4($sp)
sw $ra, 0($sp)
# call Fact(N-1)
addi $a0, $a0, -1
jal fact
# Restore the values of N and $ra
lw $a0, 4($sp)
lw $ra, 0($sp)
# Free the 8 bytes used
addi $sp, $sp, 8
# Set the return value to be N * Fact(N-1) and return
mul $v0, $a0, $v0
jr $ra
# return 1;
r_one:
li $v0, 1
jr $ra
This is what you should keep in mind when implementing your code, basically.
Just pay attention to:
- The stack pointer is decremented;
- How many bytes you need to allocate. In this example I use 2 32-bits integers, 8 bytes in total. It will deppend on how many variables you need to store, and their size.
- How to access them with lw and sw, using the correct index. Also, be aware of memory alignment;
- This does not only apply for recursion. You can use the stack memory to call another function that uses registers that are being used (basically the same thing as recursion, except that you don't need to save $ra). And also store an array, a struct, etc.
Edit:
Some considerations:
- The right place to do that is where your code calls the function (allocate and save), and after this call (restore and free).
- Understand your code to know which variables need to be saved (might be used).
edited Nov 27 '18 at 20:01
answered Nov 24 '18 at 23:58


Wilson NetoWilson Neto
265
265
Very helpful! I am having trouble understanding the relation between the $ra and the $s0 registers. For instance if my $sp is -4 and I want to store my variables in that particular frame in $s0:addi $sp, $sp, -4
sw $s3, 0($sp)
sw $s2, 0($sp)
sw $s1, 0($sp)
sw $s4, 0($sp)
sw $s5, 0($sp)
then how would I send those variables into the next frame when$sp
is -8
– MontgommeryJR
Nov 25 '18 at 20:10
There are different usages for MIPS registers, as described here. I hope this clarifies your doubt. In your example, you are trying to save the value of 5 registers (all 4 bytes, I assume). The wrong thing on your example is: You are not allocating enough space (which would be a total of 20 bytes), and you are storing them all in the same memory address. Here's the correction:addi $sp, $sp, -20 sw $s3, 0($sp) sw $s2, 4($sp) sw $s1, 8($sp) sw $s4, 12($sp) sw $s5, 16($sp)
– Wilson Neto
Nov 25 '18 at 22:08
Thank you for the clarification. Although I still cannot seem to implement it the way everyone is suggesting online. I feel like I didn't set/reset these $sx variables to their appropriate frame in the right spot of my code. Im really unsure here. Can you have a look at what I have? I have added my full code
– MontgommeryJR
Nov 26 '18 at 2:29
add a comment |
Very helpful! I am having trouble understanding the relation between the $ra and the $s0 registers. For instance if my $sp is -4 and I want to store my variables in that particular frame in $s0:addi $sp, $sp, -4
sw $s3, 0($sp)
sw $s2, 0($sp)
sw $s1, 0($sp)
sw $s4, 0($sp)
sw $s5, 0($sp)
then how would I send those variables into the next frame when$sp
is -8
– MontgommeryJR
Nov 25 '18 at 20:10
There are different usages for MIPS registers, as described here. I hope this clarifies your doubt. In your example, you are trying to save the value of 5 registers (all 4 bytes, I assume). The wrong thing on your example is: You are not allocating enough space (which would be a total of 20 bytes), and you are storing them all in the same memory address. Here's the correction:addi $sp, $sp, -20 sw $s3, 0($sp) sw $s2, 4($sp) sw $s1, 8($sp) sw $s4, 12($sp) sw $s5, 16($sp)
– Wilson Neto
Nov 25 '18 at 22:08
Thank you for the clarification. Although I still cannot seem to implement it the way everyone is suggesting online. I feel like I didn't set/reset these $sx variables to their appropriate frame in the right spot of my code. Im really unsure here. Can you have a look at what I have? I have added my full code
– MontgommeryJR
Nov 26 '18 at 2:29
Very helpful! I am having trouble understanding the relation between the $ra and the $s0 registers. For instance if my $sp is -4 and I want to store my variables in that particular frame in $s0:
addi $sp, $sp, -4
sw $s3, 0($sp)
sw $s2, 0($sp)
sw $s1, 0($sp)
sw $s4, 0($sp)
sw $s5, 0($sp)
then how would I send those variables into the next frame when $sp
is -8– MontgommeryJR
Nov 25 '18 at 20:10
Very helpful! I am having trouble understanding the relation between the $ra and the $s0 registers. For instance if my $sp is -4 and I want to store my variables in that particular frame in $s0:
addi $sp, $sp, -4
sw $s3, 0($sp)
sw $s2, 0($sp)
sw $s1, 0($sp)
sw $s4, 0($sp)
sw $s5, 0($sp)
then how would I send those variables into the next frame when $sp
is -8– MontgommeryJR
Nov 25 '18 at 20:10
There are different usages for MIPS registers, as described here. I hope this clarifies your doubt. In your example, you are trying to save the value of 5 registers (all 4 bytes, I assume). The wrong thing on your example is: You are not allocating enough space (which would be a total of 20 bytes), and you are storing them all in the same memory address. Here's the correction:
addi $sp, $sp, -20 sw $s3, 0($sp) sw $s2, 4($sp) sw $s1, 8($sp) sw $s4, 12($sp) sw $s5, 16($sp)
– Wilson Neto
Nov 25 '18 at 22:08
There are different usages for MIPS registers, as described here. I hope this clarifies your doubt. In your example, you are trying to save the value of 5 registers (all 4 bytes, I assume). The wrong thing on your example is: You are not allocating enough space (which would be a total of 20 bytes), and you are storing them all in the same memory address. Here's the correction:
addi $sp, $sp, -20 sw $s3, 0($sp) sw $s2, 4($sp) sw $s1, 8($sp) sw $s4, 12($sp) sw $s5, 16($sp)
– Wilson Neto
Nov 25 '18 at 22:08
Thank you for the clarification. Although I still cannot seem to implement it the way everyone is suggesting online. I feel like I didn't set/reset these $sx variables to their appropriate frame in the right spot of my code. Im really unsure here. Can you have a look at what I have? I have added my full code
– MontgommeryJR
Nov 26 '18 at 2:29
Thank you for the clarification. Although I still cannot seem to implement it the way everyone is suggesting online. I feel like I didn't set/reset these $sx variables to their appropriate frame in the right spot of my code. Im really unsure here. Can you have a look at what I have? I have added my full code
– MontgommeryJR
Nov 26 '18 at 2:29
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53462557%2fquicksort-from-c-to-mips-how-to-pass-parameters-and-maintaining-variables-for%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
fWKHoiG9XE6Z1dJoSwM40 ZOzr336k9y81r,A,dPqAthQY77 0JMTTeadYMIS GRwE a
Why don't you just compile your C code with a MIPS cross-compiler, as virtually everyone else does?
– Kit.
Nov 24 '18 at 22:19
this C algorithm and translating it into MIPS
What C algorithm? Usually a compiler translates C code into MIPS.– Kamil Cuk
Nov 24 '18 at 22:37