how to quite a Looper of a worker thread
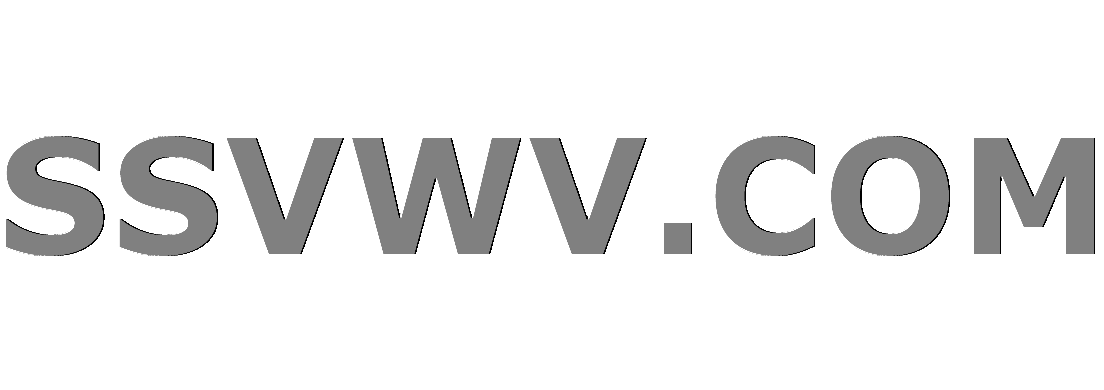
Multi tool use
I wrote the below code to better understand the Handler and the Looper. I would liek to know how can I quit the Looper on occurence of a specific condition
for example, when a counter reaches a specific limit.
In the below code, I want to call .quit() on the looper of the Thread T when "what" is equal to 10.
According to the code i wrote below, even when the contents of "what" exceeds 10, the "handleMessage" method is getting called...i expected that when .quit()
is called, the "handleMessage" will no longer be called.
please let me know how can I properly quit a Looper.
app.gradle
public class ActMain extends AppCompatActivity {
private static final String TAG = ActMain.class.getSimpleName();
private Handler mHandler = null;
private Button mBtnValues = null;
private int i = -1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.layout_act_main);
this.mBtnValues = findViewById(R.id.btnValues);
this.mBtnValues.setOnClickListener(x-> {
Message msg = new Message();
Bundle bundle = new Bundle();
bundle.putString("what", "" + i++);
Log.d(TAG, "i: " + i);
msg.setData(bundle);
mHandler.sendMessage(msg);
});
new T().start();
}
private class T extends Thread {
private String str = "";
public T() {}
@Override
public void run() {
super.run();
Log.d(TAG, "run method started");
Looper.prepare();
Log.d(TAG, "beginning of the looped section");
final String cnt = {""};
mHandler = new Handler() {
@Override
public void handleMessage(Message msg) {
super.handleMessage(msg);
String what= msg.getData().getString("what");
str += what;
Log.d(TAG, "new str: " + str);
}
};
Log.d(TAG, "end of the looped section");
if (i == 10) {
Looper.myLooper().quit();
}
Looper.loop();
}
}
}

add a comment |
I wrote the below code to better understand the Handler and the Looper. I would liek to know how can I quit the Looper on occurence of a specific condition
for example, when a counter reaches a specific limit.
In the below code, I want to call .quit() on the looper of the Thread T when "what" is equal to 10.
According to the code i wrote below, even when the contents of "what" exceeds 10, the "handleMessage" method is getting called...i expected that when .quit()
is called, the "handleMessage" will no longer be called.
please let me know how can I properly quit a Looper.
app.gradle
public class ActMain extends AppCompatActivity {
private static final String TAG = ActMain.class.getSimpleName();
private Handler mHandler = null;
private Button mBtnValues = null;
private int i = -1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.layout_act_main);
this.mBtnValues = findViewById(R.id.btnValues);
this.mBtnValues.setOnClickListener(x-> {
Message msg = new Message();
Bundle bundle = new Bundle();
bundle.putString("what", "" + i++);
Log.d(TAG, "i: " + i);
msg.setData(bundle);
mHandler.sendMessage(msg);
});
new T().start();
}
private class T extends Thread {
private String str = "";
public T() {}
@Override
public void run() {
super.run();
Log.d(TAG, "run method started");
Looper.prepare();
Log.d(TAG, "beginning of the looped section");
final String cnt = {""};
mHandler = new Handler() {
@Override
public void handleMessage(Message msg) {
super.handleMessage(msg);
String what= msg.getData().getString("what");
str += what;
Log.d(TAG, "new str: " + str);
}
};
Log.d(TAG, "end of the looped section");
if (i == 10) {
Looper.myLooper().quit();
}
Looper.loop();
}
}
}

You can access the Looper associated with your thread throughLooper.myLooper().quit();
instead ofgetMainLooper().quit();
– Mark Keen
Nov 24 '18 at 23:58
@MarkKeen i tried to use Looper.myLooper.quite() as you suggested...but still i can click the button more than 10 times and still the handler object inside the run method can keep prints the value no matter the value of i is....i modified the question..would you please have a look and help me quite the looper when the value of i == 10??
– LetsamrIt
Nov 25 '18 at 11:53
you should make theint i
volatile, meaning that it's value is never cached and always referenced to the same variable.
– Mark Keen
Nov 25 '18 at 14:05
add a comment |
I wrote the below code to better understand the Handler and the Looper. I would liek to know how can I quit the Looper on occurence of a specific condition
for example, when a counter reaches a specific limit.
In the below code, I want to call .quit() on the looper of the Thread T when "what" is equal to 10.
According to the code i wrote below, even when the contents of "what" exceeds 10, the "handleMessage" method is getting called...i expected that when .quit()
is called, the "handleMessage" will no longer be called.
please let me know how can I properly quit a Looper.
app.gradle
public class ActMain extends AppCompatActivity {
private static final String TAG = ActMain.class.getSimpleName();
private Handler mHandler = null;
private Button mBtnValues = null;
private int i = -1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.layout_act_main);
this.mBtnValues = findViewById(R.id.btnValues);
this.mBtnValues.setOnClickListener(x-> {
Message msg = new Message();
Bundle bundle = new Bundle();
bundle.putString("what", "" + i++);
Log.d(TAG, "i: " + i);
msg.setData(bundle);
mHandler.sendMessage(msg);
});
new T().start();
}
private class T extends Thread {
private String str = "";
public T() {}
@Override
public void run() {
super.run();
Log.d(TAG, "run method started");
Looper.prepare();
Log.d(TAG, "beginning of the looped section");
final String cnt = {""};
mHandler = new Handler() {
@Override
public void handleMessage(Message msg) {
super.handleMessage(msg);
String what= msg.getData().getString("what");
str += what;
Log.d(TAG, "new str: " + str);
}
};
Log.d(TAG, "end of the looped section");
if (i == 10) {
Looper.myLooper().quit();
}
Looper.loop();
}
}
}

I wrote the below code to better understand the Handler and the Looper. I would liek to know how can I quit the Looper on occurence of a specific condition
for example, when a counter reaches a specific limit.
In the below code, I want to call .quit() on the looper of the Thread T when "what" is equal to 10.
According to the code i wrote below, even when the contents of "what" exceeds 10, the "handleMessage" method is getting called...i expected that when .quit()
is called, the "handleMessage" will no longer be called.
please let me know how can I properly quit a Looper.
app.gradle
public class ActMain extends AppCompatActivity {
private static final String TAG = ActMain.class.getSimpleName();
private Handler mHandler = null;
private Button mBtnValues = null;
private int i = -1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.layout_act_main);
this.mBtnValues = findViewById(R.id.btnValues);
this.mBtnValues.setOnClickListener(x-> {
Message msg = new Message();
Bundle bundle = new Bundle();
bundle.putString("what", "" + i++);
Log.d(TAG, "i: " + i);
msg.setData(bundle);
mHandler.sendMessage(msg);
});
new T().start();
}
private class T extends Thread {
private String str = "";
public T() {}
@Override
public void run() {
super.run();
Log.d(TAG, "run method started");
Looper.prepare();
Log.d(TAG, "beginning of the looped section");
final String cnt = {""};
mHandler = new Handler() {
@Override
public void handleMessage(Message msg) {
super.handleMessage(msg);
String what= msg.getData().getString("what");
str += what;
Log.d(TAG, "new str: " + str);
}
};
Log.d(TAG, "end of the looped section");
if (i == 10) {
Looper.myLooper().quit();
}
Looper.loop();
}
}
}


edited Nov 25 '18 at 11:50
LetsamrIt
asked Nov 24 '18 at 21:32
LetsamrItLetsamrIt
1,24032141
1,24032141
You can access the Looper associated with your thread throughLooper.myLooper().quit();
instead ofgetMainLooper().quit();
– Mark Keen
Nov 24 '18 at 23:58
@MarkKeen i tried to use Looper.myLooper.quite() as you suggested...but still i can click the button more than 10 times and still the handler object inside the run method can keep prints the value no matter the value of i is....i modified the question..would you please have a look and help me quite the looper when the value of i == 10??
– LetsamrIt
Nov 25 '18 at 11:53
you should make theint i
volatile, meaning that it's value is never cached and always referenced to the same variable.
– Mark Keen
Nov 25 '18 at 14:05
add a comment |
You can access the Looper associated with your thread throughLooper.myLooper().quit();
instead ofgetMainLooper().quit();
– Mark Keen
Nov 24 '18 at 23:58
@MarkKeen i tried to use Looper.myLooper.quite() as you suggested...but still i can click the button more than 10 times and still the handler object inside the run method can keep prints the value no matter the value of i is....i modified the question..would you please have a look and help me quite the looper when the value of i == 10??
– LetsamrIt
Nov 25 '18 at 11:53
you should make theint i
volatile, meaning that it's value is never cached and always referenced to the same variable.
– Mark Keen
Nov 25 '18 at 14:05
You can access the Looper associated with your thread through
Looper.myLooper().quit();
instead of getMainLooper().quit();
– Mark Keen
Nov 24 '18 at 23:58
You can access the Looper associated with your thread through
Looper.myLooper().quit();
instead of getMainLooper().quit();
– Mark Keen
Nov 24 '18 at 23:58
@MarkKeen i tried to use Looper.myLooper.quite() as you suggested...but still i can click the button more than 10 times and still the handler object inside the run method can keep prints the value no matter the value of i is....i modified the question..would you please have a look and help me quite the looper when the value of i == 10??
– LetsamrIt
Nov 25 '18 at 11:53
@MarkKeen i tried to use Looper.myLooper.quite() as you suggested...but still i can click the button more than 10 times and still the handler object inside the run method can keep prints the value no matter the value of i is....i modified the question..would you please have a look and help me quite the looper when the value of i == 10??
– LetsamrIt
Nov 25 '18 at 11:53
you should make the
int i
volatile, meaning that it's value is never cached and always referenced to the same variable.– Mark Keen
Nov 25 '18 at 14:05
you should make the
int i
volatile, meaning that it's value is never cached and always referenced to the same variable.– Mark Keen
Nov 25 '18 at 14:05
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53462554%2fhow-to-quite-a-looper-of-a-worker-thread%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53462554%2fhow-to-quite-a-looper-of-a-worker-thread%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
9keMyzebUFQChr
You can access the Looper associated with your thread through
Looper.myLooper().quit();
instead ofgetMainLooper().quit();
– Mark Keen
Nov 24 '18 at 23:58
@MarkKeen i tried to use Looper.myLooper.quite() as you suggested...but still i can click the button more than 10 times and still the handler object inside the run method can keep prints the value no matter the value of i is....i modified the question..would you please have a look and help me quite the looper when the value of i == 10??
– LetsamrIt
Nov 25 '18 at 11:53
you should make the
int i
volatile, meaning that it's value is never cached and always referenced to the same variable.– Mark Keen
Nov 25 '18 at 14:05