Only one usage of each socket address (protocol/network address/port) is normally permitted?
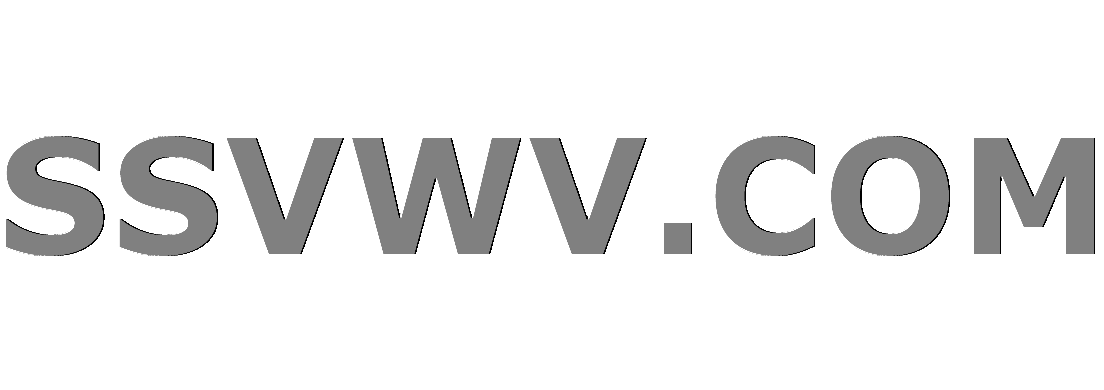
Multi tool use
I've been looking for a serious solution on google and i only get "Regisrty solutions" kind of stuff which i don't think even relate to my problem.
For some reason i get this Error, while i'm only starting the TcpListner once, and when+if fails i stop the server.
I really don't get it.
Here is my code:
class Program
{
private static string ServerName = "";
private static string UserName = "";
private static string Password = "";
private static string dbConnectionSring = "";
private static X509Certificate adminCertificate;
private static byte readBuffer = new byte[4096];
static void Main(string args)
{
Console.WriteLine("Please grant SQL Server access to the Admin Server:n");
Console.Write("Server Name: ");
ServerName = Console.ReadLine();
Console.Write("nUser Name: ");
UserName = Console.ReadLine();
Console.Write("nPassword: ");
Password = PasswordMasker.Mask(Password);
dbConnectionSring = SQLServerAccess.CreateConnection(ServerName, UserName, Password);
adminCertificate = Certificate.GenerateOrImportCertificate("AdminCert.pfx", "randomPassword");
try
{
Console.WriteLine("Initializing server on the WildCard address on port 443...");
TcpListener listener = new TcpListener(IPAddress.Any, 443);
try
{
Console.WriteLine("Starting to listen at {0}: 443...", IPAddress.Any);
//the backlog is set to the maximum integer value, but the underlying network stack will reset this value to its internal maximum value
listener.Start(int.MaxValue);
Console.WriteLine("Listening... Waiting for a client to connect...");
int ConnectionCount = 0;
while (true)
{
try
{
listener.BeginAcceptTcpClient(new AsyncCallback(AcceptCallback), listener);
ConnectionCount++;
Console.WriteLine(
" Accepted connection #" + ConnectionCount.ToString());
}
catch (SocketException err)
{
Console.WriteLine("Accept failed: {0}", err.Message);
}
}
}
catch (Exception ex)
{
Console.WriteLine("Listening failed to start.");
listener.Stop();
Console.WriteLine(ex.Message);
}
}
catch (Exception ex)
{
Console.WriteLine("Initialiazing server Failed.");
Console.WriteLine(ex.Message);
}
}
I will really appreciate your help!
c# sockets tcp tcplistener socketexception
add a comment |
I've been looking for a serious solution on google and i only get "Regisrty solutions" kind of stuff which i don't think even relate to my problem.
For some reason i get this Error, while i'm only starting the TcpListner once, and when+if fails i stop the server.
I really don't get it.
Here is my code:
class Program
{
private static string ServerName = "";
private static string UserName = "";
private static string Password = "";
private static string dbConnectionSring = "";
private static X509Certificate adminCertificate;
private static byte readBuffer = new byte[4096];
static void Main(string args)
{
Console.WriteLine("Please grant SQL Server access to the Admin Server:n");
Console.Write("Server Name: ");
ServerName = Console.ReadLine();
Console.Write("nUser Name: ");
UserName = Console.ReadLine();
Console.Write("nPassword: ");
Password = PasswordMasker.Mask(Password);
dbConnectionSring = SQLServerAccess.CreateConnection(ServerName, UserName, Password);
adminCertificate = Certificate.GenerateOrImportCertificate("AdminCert.pfx", "randomPassword");
try
{
Console.WriteLine("Initializing server on the WildCard address on port 443...");
TcpListener listener = new TcpListener(IPAddress.Any, 443);
try
{
Console.WriteLine("Starting to listen at {0}: 443...", IPAddress.Any);
//the backlog is set to the maximum integer value, but the underlying network stack will reset this value to its internal maximum value
listener.Start(int.MaxValue);
Console.WriteLine("Listening... Waiting for a client to connect...");
int ConnectionCount = 0;
while (true)
{
try
{
listener.BeginAcceptTcpClient(new AsyncCallback(AcceptCallback), listener);
ConnectionCount++;
Console.WriteLine(
" Accepted connection #" + ConnectionCount.ToString());
}
catch (SocketException err)
{
Console.WriteLine("Accept failed: {0}", err.Message);
}
}
}
catch (Exception ex)
{
Console.WriteLine("Listening failed to start.");
listener.Stop();
Console.WriteLine(ex.Message);
}
}
catch (Exception ex)
{
Console.WriteLine("Initialiazing server Failed.");
Console.WriteLine(ex.Message);
}
}
I will really appreciate your help!
c# sockets tcp tcplistener socketexception
3
The problem is another program is already listening on that port...
– Gusman
Jan 24 '17 at 18:24
1
You might want to try either a different port or binding to a specific local IP address rather than all of them.
– David Schwartz
Jan 24 '17 at 18:25
@DavidSchwartz how can i know which ports are free to use?
– WeinForce
Jan 24 '17 at 18:30
1
Are you this machine's administrator? If so, you should know what services it's running and what ports they use. If not, you should talk to the person who administers the services running on this machine. You can start with commands likenetstat -tan
to take inventory if needed.
– David Schwartz
Jan 24 '17 at 18:33
1
Port 443 is the default port for HTTPS, so you may have an http server running on the machine.
– Gusman
Jan 24 '17 at 18:40
add a comment |
I've been looking for a serious solution on google and i only get "Regisrty solutions" kind of stuff which i don't think even relate to my problem.
For some reason i get this Error, while i'm only starting the TcpListner once, and when+if fails i stop the server.
I really don't get it.
Here is my code:
class Program
{
private static string ServerName = "";
private static string UserName = "";
private static string Password = "";
private static string dbConnectionSring = "";
private static X509Certificate adminCertificate;
private static byte readBuffer = new byte[4096];
static void Main(string args)
{
Console.WriteLine("Please grant SQL Server access to the Admin Server:n");
Console.Write("Server Name: ");
ServerName = Console.ReadLine();
Console.Write("nUser Name: ");
UserName = Console.ReadLine();
Console.Write("nPassword: ");
Password = PasswordMasker.Mask(Password);
dbConnectionSring = SQLServerAccess.CreateConnection(ServerName, UserName, Password);
adminCertificate = Certificate.GenerateOrImportCertificate("AdminCert.pfx", "randomPassword");
try
{
Console.WriteLine("Initializing server on the WildCard address on port 443...");
TcpListener listener = new TcpListener(IPAddress.Any, 443);
try
{
Console.WriteLine("Starting to listen at {0}: 443...", IPAddress.Any);
//the backlog is set to the maximum integer value, but the underlying network stack will reset this value to its internal maximum value
listener.Start(int.MaxValue);
Console.WriteLine("Listening... Waiting for a client to connect...");
int ConnectionCount = 0;
while (true)
{
try
{
listener.BeginAcceptTcpClient(new AsyncCallback(AcceptCallback), listener);
ConnectionCount++;
Console.WriteLine(
" Accepted connection #" + ConnectionCount.ToString());
}
catch (SocketException err)
{
Console.WriteLine("Accept failed: {0}", err.Message);
}
}
}
catch (Exception ex)
{
Console.WriteLine("Listening failed to start.");
listener.Stop();
Console.WriteLine(ex.Message);
}
}
catch (Exception ex)
{
Console.WriteLine("Initialiazing server Failed.");
Console.WriteLine(ex.Message);
}
}
I will really appreciate your help!
c# sockets tcp tcplistener socketexception
I've been looking for a serious solution on google and i only get "Regisrty solutions" kind of stuff which i don't think even relate to my problem.
For some reason i get this Error, while i'm only starting the TcpListner once, and when+if fails i stop the server.
I really don't get it.
Here is my code:
class Program
{
private static string ServerName = "";
private static string UserName = "";
private static string Password = "";
private static string dbConnectionSring = "";
private static X509Certificate adminCertificate;
private static byte readBuffer = new byte[4096];
static void Main(string args)
{
Console.WriteLine("Please grant SQL Server access to the Admin Server:n");
Console.Write("Server Name: ");
ServerName = Console.ReadLine();
Console.Write("nUser Name: ");
UserName = Console.ReadLine();
Console.Write("nPassword: ");
Password = PasswordMasker.Mask(Password);
dbConnectionSring = SQLServerAccess.CreateConnection(ServerName, UserName, Password);
adminCertificate = Certificate.GenerateOrImportCertificate("AdminCert.pfx", "randomPassword");
try
{
Console.WriteLine("Initializing server on the WildCard address on port 443...");
TcpListener listener = new TcpListener(IPAddress.Any, 443);
try
{
Console.WriteLine("Starting to listen at {0}: 443...", IPAddress.Any);
//the backlog is set to the maximum integer value, but the underlying network stack will reset this value to its internal maximum value
listener.Start(int.MaxValue);
Console.WriteLine("Listening... Waiting for a client to connect...");
int ConnectionCount = 0;
while (true)
{
try
{
listener.BeginAcceptTcpClient(new AsyncCallback(AcceptCallback), listener);
ConnectionCount++;
Console.WriteLine(
" Accepted connection #" + ConnectionCount.ToString());
}
catch (SocketException err)
{
Console.WriteLine("Accept failed: {0}", err.Message);
}
}
}
catch (Exception ex)
{
Console.WriteLine("Listening failed to start.");
listener.Stop();
Console.WriteLine(ex.Message);
}
}
catch (Exception ex)
{
Console.WriteLine("Initialiazing server Failed.");
Console.WriteLine(ex.Message);
}
}
I will really appreciate your help!
c# sockets tcp tcplistener socketexception
c# sockets tcp tcplistener socketexception
asked Jan 24 '17 at 18:22


WeinForce
1631212
1631212
3
The problem is another program is already listening on that port...
– Gusman
Jan 24 '17 at 18:24
1
You might want to try either a different port or binding to a specific local IP address rather than all of them.
– David Schwartz
Jan 24 '17 at 18:25
@DavidSchwartz how can i know which ports are free to use?
– WeinForce
Jan 24 '17 at 18:30
1
Are you this machine's administrator? If so, you should know what services it's running and what ports they use. If not, you should talk to the person who administers the services running on this machine. You can start with commands likenetstat -tan
to take inventory if needed.
– David Schwartz
Jan 24 '17 at 18:33
1
Port 443 is the default port for HTTPS, so you may have an http server running on the machine.
– Gusman
Jan 24 '17 at 18:40
add a comment |
3
The problem is another program is already listening on that port...
– Gusman
Jan 24 '17 at 18:24
1
You might want to try either a different port or binding to a specific local IP address rather than all of them.
– David Schwartz
Jan 24 '17 at 18:25
@DavidSchwartz how can i know which ports are free to use?
– WeinForce
Jan 24 '17 at 18:30
1
Are you this machine's administrator? If so, you should know what services it's running and what ports they use. If not, you should talk to the person who administers the services running on this machine. You can start with commands likenetstat -tan
to take inventory if needed.
– David Schwartz
Jan 24 '17 at 18:33
1
Port 443 is the default port for HTTPS, so you may have an http server running on the machine.
– Gusman
Jan 24 '17 at 18:40
3
3
The problem is another program is already listening on that port...
– Gusman
Jan 24 '17 at 18:24
The problem is another program is already listening on that port...
– Gusman
Jan 24 '17 at 18:24
1
1
You might want to try either a different port or binding to a specific local IP address rather than all of them.
– David Schwartz
Jan 24 '17 at 18:25
You might want to try either a different port or binding to a specific local IP address rather than all of them.
– David Schwartz
Jan 24 '17 at 18:25
@DavidSchwartz how can i know which ports are free to use?
– WeinForce
Jan 24 '17 at 18:30
@DavidSchwartz how can i know which ports are free to use?
– WeinForce
Jan 24 '17 at 18:30
1
1
Are you this machine's administrator? If so, you should know what services it's running and what ports they use. If not, you should talk to the person who administers the services running on this machine. You can start with commands like
netstat -tan
to take inventory if needed.– David Schwartz
Jan 24 '17 at 18:33
Are you this machine's administrator? If so, you should know what services it's running and what ports they use. If not, you should talk to the person who administers the services running on this machine. You can start with commands like
netstat -tan
to take inventory if needed.– David Schwartz
Jan 24 '17 at 18:33
1
1
Port 443 is the default port for HTTPS, so you may have an http server running on the machine.
– Gusman
Jan 24 '17 at 18:40
Port 443 is the default port for HTTPS, so you may have an http server running on the machine.
– Gusman
Jan 24 '17 at 18:40
add a comment |
2 Answers
2
active
oldest
votes
- I opened CMD and typed in : netstat -a
- I took a look in the Local Address column.
- I took a look at the port portion.
- I saw that the port in my program is already active( in use ) in another program.
I changed my port in my program to something else.
It Worked!
Big thanks to: @DavidSchwartz, @Gusman
add a comment |
- Open cmd
- Type netstat –ano
- List of process with their ports will be opened
- Search ‘process ID’ of the port you are unable to use (in my case port 11020)
- Open task Manager and Stop that process
- Now your port is ready to use :)
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f41836209%2fonly-one-usage-of-each-socket-address-protocol-network-address-port-is-normall%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
- I opened CMD and typed in : netstat -a
- I took a look in the Local Address column.
- I took a look at the port portion.
- I saw that the port in my program is already active( in use ) in another program.
I changed my port in my program to something else.
It Worked!
Big thanks to: @DavidSchwartz, @Gusman
add a comment |
- I opened CMD and typed in : netstat -a
- I took a look in the Local Address column.
- I took a look at the port portion.
- I saw that the port in my program is already active( in use ) in another program.
I changed my port in my program to something else.
It Worked!
Big thanks to: @DavidSchwartz, @Gusman
add a comment |
- I opened CMD and typed in : netstat -a
- I took a look in the Local Address column.
- I took a look at the port portion.
- I saw that the port in my program is already active( in use ) in another program.
I changed my port in my program to something else.
It Worked!
Big thanks to: @DavidSchwartz, @Gusman
- I opened CMD and typed in : netstat -a
- I took a look in the Local Address column.
- I took a look at the port portion.
- I saw that the port in my program is already active( in use ) in another program.
I changed my port in my program to something else.
It Worked!
Big thanks to: @DavidSchwartz, @Gusman
answered Jan 24 '17 at 18:44


WeinForce
1631212
1631212
add a comment |
add a comment |
- Open cmd
- Type netstat –ano
- List of process with their ports will be opened
- Search ‘process ID’ of the port you are unable to use (in my case port 11020)
- Open task Manager and Stop that process
- Now your port is ready to use :)
add a comment |
- Open cmd
- Type netstat –ano
- List of process with their ports will be opened
- Search ‘process ID’ of the port you are unable to use (in my case port 11020)
- Open task Manager and Stop that process
- Now your port is ready to use :)
add a comment |
- Open cmd
- Type netstat –ano
- List of process with their ports will be opened
- Search ‘process ID’ of the port you are unable to use (in my case port 11020)
- Open task Manager and Stop that process
- Now your port is ready to use :)
- Open cmd
- Type netstat –ano
- List of process with their ports will be opened
- Search ‘process ID’ of the port you are unable to use (in my case port 11020)
- Open task Manager and Stop that process
- Now your port is ready to use :)
answered Dec 13 '18 at 15:09


harpreet singh
1
1
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f41836209%2fonly-one-usage-of-each-socket-address-protocol-network-address-port-is-normall%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
8wIpu,kb5QZbx VmTYm,Whu50M7jrEWM33aSXl9 VRvXrlZ p1,IyR,fGyaM0U9ipCxgTIyk Dpu4yRKLluGLhW
3
The problem is another program is already listening on that port...
– Gusman
Jan 24 '17 at 18:24
1
You might want to try either a different port or binding to a specific local IP address rather than all of them.
– David Schwartz
Jan 24 '17 at 18:25
@DavidSchwartz how can i know which ports are free to use?
– WeinForce
Jan 24 '17 at 18:30
1
Are you this machine's administrator? If so, you should know what services it's running and what ports they use. If not, you should talk to the person who administers the services running on this machine. You can start with commands like
netstat -tan
to take inventory if needed.– David Schwartz
Jan 24 '17 at 18:33
1
Port 443 is the default port for HTTPS, so you may have an http server running on the machine.
– Gusman
Jan 24 '17 at 18:40