How to set up Message in Snackbar
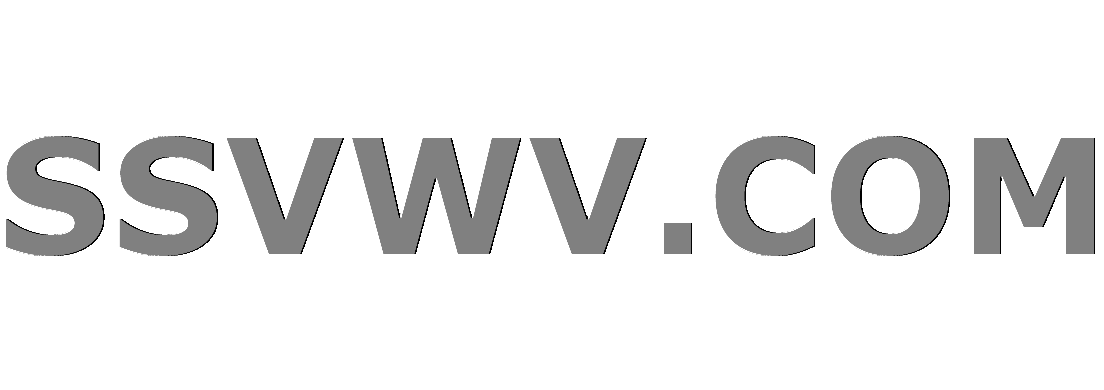
Multi tool use
I have a problem with Material Design Snackbar configuration. What I'm trying to achieve is to fire up Snackbar whenever there is a message about some functionality, for example: when I delete entry than message fire up and inform the user that everything was all right. It works as component and it appears as bootstrap alert, but I want it to be in snackbar.
Part of Category
component:
delete(category: Category): void {
this.categories = this.categories.filter(c => c !== category);
this.categoryService.deleteCategory(category).subscribe();
}
Part of Category
service:
/** DELETE: delete the Category from the server */
deleteCategory (category: Category | number): Observable<Category> {
const CategoryId = typeof category === 'number' ? category :
category.id;
const urlCategoryId = `${this.urlCategory}/${CategoryId}`;
return this.http.delete<Category>(urlCategoryId, httpOptions).pipe(
tap(_ => this.log(`Category has been deleted`)),
catchError(this.handleError<Category>('deleteCategory'))
);
}
/**
* Handle Http operation that failed.
* Let the app continue.
* @param operation - name of the operation that failed
* @param result - optional value to return as the observable result
*/
private handleError<T>(operation = 'operation', result?: T) {
return (error: any): Observable<T> => {
// TODO: send the error to remote logging infrastructure
console.error(error); // log to console instead
// // TODO: better job of transforming error for user consumption
// this.log(`${operation} failed: ${error.message}`);
// Let the app keep running by returning an empty result.
return of(result as T);
};
}
private log(message: string) {
this.messageService.add(`${message}`);
}
Message
component:
import { Component, OnInit } from '@angular/core';
import {MessageService} from '../../services/message
/message.service';
@Component({
selector: 'app-messages',
templateUrl: './messages.component.html',
styleUrls: ['./messages.component.scss']
})
export class MessagesComponent implements OnInit {
constructor(public messageService: MessageService) { }
ngOnInit(): void {
}
}
Message
service:
import {Injectable} from '@angular/core';
import {MatSnackBar} from '@angular/material';
@Injectable({
providedIn: 'root'
})
export class MessageService {
messages: string = ;
constructor(private snackBar: MatSnackBar) { }
clear() {
this.messages = ;
}
add(message: string) {
this.snackBar.open(message, 'Close', {
duration: 5000, horizontalPosition: 'right', verticalPosition:
'bottom', panelClass: 'snackbar-style'});
}
}
in assets/custom.scss
.snackbar-style {
background-color: 'color of your choice' !important;
color: 'color of your choice' !important;
}
Message
html:
<div *ngIf="messageService.messages.length" class="alert alert-info
alert-with-icon" data-notify="container">
<button type="button" aria-hidden="true" class="close"
(click)="messageService.clear()">
<i class="now-ui-icons ui-1_simple-remove"></i>
</button>
<span data-notify="icon" class="now-ui-icons ui-1_bell-53"></span>
<span data-notify="message">{{ messageService.messages }}</span>
</div>
Complete solution.
Thanks everyone (especially JB Nizet) for help!
angular material-design angular7
|
show 2 more comments
I have a problem with Material Design Snackbar configuration. What I'm trying to achieve is to fire up Snackbar whenever there is a message about some functionality, for example: when I delete entry than message fire up and inform the user that everything was all right. It works as component and it appears as bootstrap alert, but I want it to be in snackbar.
Part of Category
component:
delete(category: Category): void {
this.categories = this.categories.filter(c => c !== category);
this.categoryService.deleteCategory(category).subscribe();
}
Part of Category
service:
/** DELETE: delete the Category from the server */
deleteCategory (category: Category | number): Observable<Category> {
const CategoryId = typeof category === 'number' ? category :
category.id;
const urlCategoryId = `${this.urlCategory}/${CategoryId}`;
return this.http.delete<Category>(urlCategoryId, httpOptions).pipe(
tap(_ => this.log(`Category has been deleted`)),
catchError(this.handleError<Category>('deleteCategory'))
);
}
/**
* Handle Http operation that failed.
* Let the app continue.
* @param operation - name of the operation that failed
* @param result - optional value to return as the observable result
*/
private handleError<T>(operation = 'operation', result?: T) {
return (error: any): Observable<T> => {
// TODO: send the error to remote logging infrastructure
console.error(error); // log to console instead
// // TODO: better job of transforming error for user consumption
// this.log(`${operation} failed: ${error.message}`);
// Let the app keep running by returning an empty result.
return of(result as T);
};
}
private log(message: string) {
this.messageService.add(`${message}`);
}
Message
component:
import { Component, OnInit } from '@angular/core';
import {MessageService} from '../../services/message
/message.service';
@Component({
selector: 'app-messages',
templateUrl: './messages.component.html',
styleUrls: ['./messages.component.scss']
})
export class MessagesComponent implements OnInit {
constructor(public messageService: MessageService) { }
ngOnInit(): void {
}
}
Message
service:
import {Injectable} from '@angular/core';
import {MatSnackBar} from '@angular/material';
@Injectable({
providedIn: 'root'
})
export class MessageService {
messages: string = ;
constructor(private snackBar: MatSnackBar) { }
clear() {
this.messages = ;
}
add(message: string) {
this.snackBar.open(message, 'Close', {
duration: 5000, horizontalPosition: 'right', verticalPosition:
'bottom', panelClass: 'snackbar-style'});
}
}
in assets/custom.scss
.snackbar-style {
background-color: 'color of your choice' !important;
color: 'color of your choice' !important;
}
Message
html:
<div *ngIf="messageService.messages.length" class="alert alert-info
alert-with-icon" data-notify="container">
<button type="button" aria-hidden="true" class="close"
(click)="messageService.clear()">
<i class="now-ui-icons ui-1_simple-remove"></i>
</button>
<span data-notify="icon" class="now-ui-icons ui-1_bell-53"></span>
<span data-notify="message">{{ messageService.messages }}</span>
</div>
Complete solution.
Thanks everyone (especially JB Nizet) for help!
angular material-design angular7
Replace the body of your add(message: string) method by snackBar.open(message)?
– JB Nizet
Nov 22 '18 at 19:32
Don't describe your code. Post it. Have you read the documentation and examples at material.angular.io/components/snack-bar/overview?
– JB Nizet
Nov 22 '18 at 19:51
Post all the relevant code, properly formatted as code, in your question. Not as a comment.
– JB Nizet
Nov 22 '18 at 19:58
Why are you trying to add messages to an array? If you’re going to use material snack bar, use it according to their docs. You’re making this too hard on yourself.
– Ben Steward
Nov 22 '18 at 20:00
ReplacesnackBar
bythis.snackBar
. If you read the compiler error messages, it would tell it: "did you mean this.snackBar". Error messages are helpful. Read them.
– JB Nizet
Nov 22 '18 at 20:11
|
show 2 more comments
I have a problem with Material Design Snackbar configuration. What I'm trying to achieve is to fire up Snackbar whenever there is a message about some functionality, for example: when I delete entry than message fire up and inform the user that everything was all right. It works as component and it appears as bootstrap alert, but I want it to be in snackbar.
Part of Category
component:
delete(category: Category): void {
this.categories = this.categories.filter(c => c !== category);
this.categoryService.deleteCategory(category).subscribe();
}
Part of Category
service:
/** DELETE: delete the Category from the server */
deleteCategory (category: Category | number): Observable<Category> {
const CategoryId = typeof category === 'number' ? category :
category.id;
const urlCategoryId = `${this.urlCategory}/${CategoryId}`;
return this.http.delete<Category>(urlCategoryId, httpOptions).pipe(
tap(_ => this.log(`Category has been deleted`)),
catchError(this.handleError<Category>('deleteCategory'))
);
}
/**
* Handle Http operation that failed.
* Let the app continue.
* @param operation - name of the operation that failed
* @param result - optional value to return as the observable result
*/
private handleError<T>(operation = 'operation', result?: T) {
return (error: any): Observable<T> => {
// TODO: send the error to remote logging infrastructure
console.error(error); // log to console instead
// // TODO: better job of transforming error for user consumption
// this.log(`${operation} failed: ${error.message}`);
// Let the app keep running by returning an empty result.
return of(result as T);
};
}
private log(message: string) {
this.messageService.add(`${message}`);
}
Message
component:
import { Component, OnInit } from '@angular/core';
import {MessageService} from '../../services/message
/message.service';
@Component({
selector: 'app-messages',
templateUrl: './messages.component.html',
styleUrls: ['./messages.component.scss']
})
export class MessagesComponent implements OnInit {
constructor(public messageService: MessageService) { }
ngOnInit(): void {
}
}
Message
service:
import {Injectable} from '@angular/core';
import {MatSnackBar} from '@angular/material';
@Injectable({
providedIn: 'root'
})
export class MessageService {
messages: string = ;
constructor(private snackBar: MatSnackBar) { }
clear() {
this.messages = ;
}
add(message: string) {
this.snackBar.open(message, 'Close', {
duration: 5000, horizontalPosition: 'right', verticalPosition:
'bottom', panelClass: 'snackbar-style'});
}
}
in assets/custom.scss
.snackbar-style {
background-color: 'color of your choice' !important;
color: 'color of your choice' !important;
}
Message
html:
<div *ngIf="messageService.messages.length" class="alert alert-info
alert-with-icon" data-notify="container">
<button type="button" aria-hidden="true" class="close"
(click)="messageService.clear()">
<i class="now-ui-icons ui-1_simple-remove"></i>
</button>
<span data-notify="icon" class="now-ui-icons ui-1_bell-53"></span>
<span data-notify="message">{{ messageService.messages }}</span>
</div>
Complete solution.
Thanks everyone (especially JB Nizet) for help!
angular material-design angular7
I have a problem with Material Design Snackbar configuration. What I'm trying to achieve is to fire up Snackbar whenever there is a message about some functionality, for example: when I delete entry than message fire up and inform the user that everything was all right. It works as component and it appears as bootstrap alert, but I want it to be in snackbar.
Part of Category
component:
delete(category: Category): void {
this.categories = this.categories.filter(c => c !== category);
this.categoryService.deleteCategory(category).subscribe();
}
Part of Category
service:
/** DELETE: delete the Category from the server */
deleteCategory (category: Category | number): Observable<Category> {
const CategoryId = typeof category === 'number' ? category :
category.id;
const urlCategoryId = `${this.urlCategory}/${CategoryId}`;
return this.http.delete<Category>(urlCategoryId, httpOptions).pipe(
tap(_ => this.log(`Category has been deleted`)),
catchError(this.handleError<Category>('deleteCategory'))
);
}
/**
* Handle Http operation that failed.
* Let the app continue.
* @param operation - name of the operation that failed
* @param result - optional value to return as the observable result
*/
private handleError<T>(operation = 'operation', result?: T) {
return (error: any): Observable<T> => {
// TODO: send the error to remote logging infrastructure
console.error(error); // log to console instead
// // TODO: better job of transforming error for user consumption
// this.log(`${operation} failed: ${error.message}`);
// Let the app keep running by returning an empty result.
return of(result as T);
};
}
private log(message: string) {
this.messageService.add(`${message}`);
}
Message
component:
import { Component, OnInit } from '@angular/core';
import {MessageService} from '../../services/message
/message.service';
@Component({
selector: 'app-messages',
templateUrl: './messages.component.html',
styleUrls: ['./messages.component.scss']
})
export class MessagesComponent implements OnInit {
constructor(public messageService: MessageService) { }
ngOnInit(): void {
}
}
Message
service:
import {Injectable} from '@angular/core';
import {MatSnackBar} from '@angular/material';
@Injectable({
providedIn: 'root'
})
export class MessageService {
messages: string = ;
constructor(private snackBar: MatSnackBar) { }
clear() {
this.messages = ;
}
add(message: string) {
this.snackBar.open(message, 'Close', {
duration: 5000, horizontalPosition: 'right', verticalPosition:
'bottom', panelClass: 'snackbar-style'});
}
}
in assets/custom.scss
.snackbar-style {
background-color: 'color of your choice' !important;
color: 'color of your choice' !important;
}
Message
html:
<div *ngIf="messageService.messages.length" class="alert alert-info
alert-with-icon" data-notify="container">
<button type="button" aria-hidden="true" class="close"
(click)="messageService.clear()">
<i class="now-ui-icons ui-1_simple-remove"></i>
</button>
<span data-notify="icon" class="now-ui-icons ui-1_bell-53"></span>
<span data-notify="message">{{ messageService.messages }}</span>
</div>
Complete solution.
Thanks everyone (especially JB Nizet) for help!
angular material-design angular7
angular material-design angular7
edited Nov 23 '18 at 9:40
Matis
asked Nov 22 '18 at 19:26


MatisMatis
62
62
Replace the body of your add(message: string) method by snackBar.open(message)?
– JB Nizet
Nov 22 '18 at 19:32
Don't describe your code. Post it. Have you read the documentation and examples at material.angular.io/components/snack-bar/overview?
– JB Nizet
Nov 22 '18 at 19:51
Post all the relevant code, properly formatted as code, in your question. Not as a comment.
– JB Nizet
Nov 22 '18 at 19:58
Why are you trying to add messages to an array? If you’re going to use material snack bar, use it according to their docs. You’re making this too hard on yourself.
– Ben Steward
Nov 22 '18 at 20:00
ReplacesnackBar
bythis.snackBar
. If you read the compiler error messages, it would tell it: "did you mean this.snackBar". Error messages are helpful. Read them.
– JB Nizet
Nov 22 '18 at 20:11
|
show 2 more comments
Replace the body of your add(message: string) method by snackBar.open(message)?
– JB Nizet
Nov 22 '18 at 19:32
Don't describe your code. Post it. Have you read the documentation and examples at material.angular.io/components/snack-bar/overview?
– JB Nizet
Nov 22 '18 at 19:51
Post all the relevant code, properly formatted as code, in your question. Not as a comment.
– JB Nizet
Nov 22 '18 at 19:58
Why are you trying to add messages to an array? If you’re going to use material snack bar, use it according to their docs. You’re making this too hard on yourself.
– Ben Steward
Nov 22 '18 at 20:00
ReplacesnackBar
bythis.snackBar
. If you read the compiler error messages, it would tell it: "did you mean this.snackBar". Error messages are helpful. Read them.
– JB Nizet
Nov 22 '18 at 20:11
Replace the body of your add(message: string) method by snackBar.open(message)?
– JB Nizet
Nov 22 '18 at 19:32
Replace the body of your add(message: string) method by snackBar.open(message)?
– JB Nizet
Nov 22 '18 at 19:32
Don't describe your code. Post it. Have you read the documentation and examples at material.angular.io/components/snack-bar/overview?
– JB Nizet
Nov 22 '18 at 19:51
Don't describe your code. Post it. Have you read the documentation and examples at material.angular.io/components/snack-bar/overview?
– JB Nizet
Nov 22 '18 at 19:51
Post all the relevant code, properly formatted as code, in your question. Not as a comment.
– JB Nizet
Nov 22 '18 at 19:58
Post all the relevant code, properly formatted as code, in your question. Not as a comment.
– JB Nizet
Nov 22 '18 at 19:58
Why are you trying to add messages to an array? If you’re going to use material snack bar, use it according to their docs. You’re making this too hard on yourself.
– Ben Steward
Nov 22 '18 at 20:00
Why are you trying to add messages to an array? If you’re going to use material snack bar, use it according to their docs. You’re making this too hard on yourself.
– Ben Steward
Nov 22 '18 at 20:00
Replace
snackBar
by this.snackBar
. If you read the compiler error messages, it would tell it: "did you mean this.snackBar". Error messages are helpful. Read them.– JB Nizet
Nov 22 '18 at 20:11
Replace
snackBar
by this.snackBar
. If you read the compiler error messages, it would tell it: "did you mean this.snackBar". Error messages are helpful. Read them.– JB Nizet
Nov 22 '18 at 20:11
|
show 2 more comments
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53437069%2fhow-to-set-up-message-in-snackbar%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53437069%2fhow-to-set-up-message-in-snackbar%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
KmuihDu8RX4t69TRu CxFUFkO6EEGQHJuPx,INi,P,PE2Kk0,yVz2,HwyhkCsTOqhAbOVr,WnpkFGm,BiGn8a E9
Replace the body of your add(message: string) method by snackBar.open(message)?
– JB Nizet
Nov 22 '18 at 19:32
Don't describe your code. Post it. Have you read the documentation and examples at material.angular.io/components/snack-bar/overview?
– JB Nizet
Nov 22 '18 at 19:51
Post all the relevant code, properly formatted as code, in your question. Not as a comment.
– JB Nizet
Nov 22 '18 at 19:58
Why are you trying to add messages to an array? If you’re going to use material snack bar, use it according to their docs. You’re making this too hard on yourself.
– Ben Steward
Nov 22 '18 at 20:00
Replace
snackBar
bythis.snackBar
. If you read the compiler error messages, it would tell it: "did you mean this.snackBar". Error messages are helpful. Read them.– JB Nizet
Nov 22 '18 at 20:11