Instantiate and use an object with only the textual class name in Java
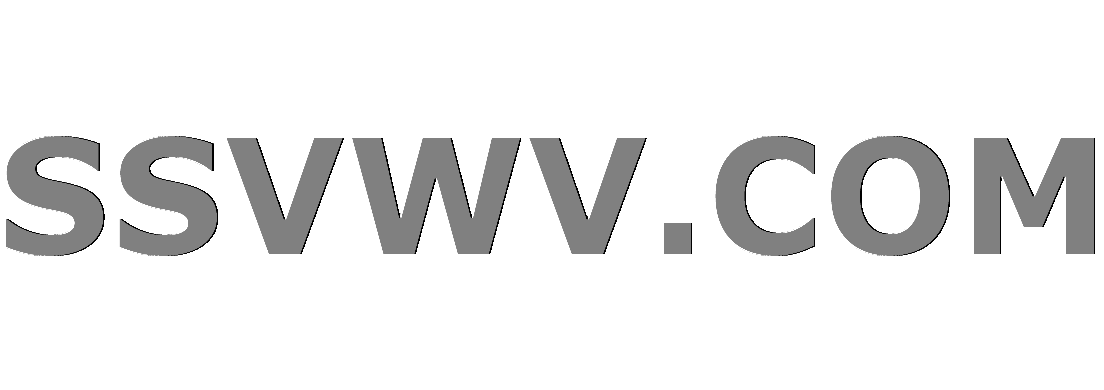
Multi tool use
I have several classes in the same package in Java. I want to instantiate objects of these classes from an array that has the class names as strings.
Here is an example of a class I would like to use, they all have the same structure.
class Class1 {
public String firstMethod(){
String data = {"NEW_ITEM"};
return data;
}
}
Here is the class I am attemtempting to instantiate them from.
class Main {
static {
String classes = {"Class1","Class2"};
for (String cls : classes) {
try {
Object o = Class.forName(cls).newInstance();
o.firstMethod();
} catch(ClassNotFoundException | IllegalAccessException | InstantiationException ex) {
System.out.println(ex.toString());
}
}
My problem is that when I try to call firstMethod() using the object o, I am getting this error.
exit status 1
Main.java:19: error: cannot find symbol
o.firstMethod();
^
symbol: method firstMethod()
location: variable o of type Object
1 error
I suspect that it is because it is of type Object and not type Class1. I have seen solutions where you typecast the object to the object of the class that you need. However when you typcast, you need to use the name of the class, which is exactly what I am trying to avoid. I need to use the class name as a string.
Does anyone know of a solution where I can call methods with the objects that are created?
java reflection
add a comment |
I have several classes in the same package in Java. I want to instantiate objects of these classes from an array that has the class names as strings.
Here is an example of a class I would like to use, they all have the same structure.
class Class1 {
public String firstMethod(){
String data = {"NEW_ITEM"};
return data;
}
}
Here is the class I am attemtempting to instantiate them from.
class Main {
static {
String classes = {"Class1","Class2"};
for (String cls : classes) {
try {
Object o = Class.forName(cls).newInstance();
o.firstMethod();
} catch(ClassNotFoundException | IllegalAccessException | InstantiationException ex) {
System.out.println(ex.toString());
}
}
My problem is that when I try to call firstMethod() using the object o, I am getting this error.
exit status 1
Main.java:19: error: cannot find symbol
o.firstMethod();
^
symbol: method firstMethod()
location: variable o of type Object
1 error
I suspect that it is because it is of type Object and not type Class1. I have seen solutions where you typecast the object to the object of the class that you need. However when you typcast, you need to use the name of the class, which is exactly what I am trying to avoid. I need to use the class name as a string.
Does anyone know of a solution where I can call methods with the objects that are created?
java reflection
1
continue using reflection likeMethod.invoke()
- usegetMethod
orgetDeclaredMethod
of the class to get the method, callinvoke
on it, passing the created instance as parameter - e.g. stackoverflow.com/q/160970/85421
– Carlos Heuberger
Nov 23 '18 at 21:53
1
I assume that these classes have something in common, generally speaking they implement some kind of interface. In this case you can simply cast to the interface and call that method. If this is not possible, you have to invoke the methods via reflection as well.
– Glains
Nov 23 '18 at 21:53
Carlos Heuberger, thanks for helping a noob. Method method = o.getClass().getDeclaredMethod("firstMethod"); method.invoke(o); I got it working with this.
– J.Dor
Nov 23 '18 at 22:01
in case the method is private you can use the method.setAccessible(true);
– nmorenor
Nov 23 '18 at 23:39
add a comment |
I have several classes in the same package in Java. I want to instantiate objects of these classes from an array that has the class names as strings.
Here is an example of a class I would like to use, they all have the same structure.
class Class1 {
public String firstMethod(){
String data = {"NEW_ITEM"};
return data;
}
}
Here is the class I am attemtempting to instantiate them from.
class Main {
static {
String classes = {"Class1","Class2"};
for (String cls : classes) {
try {
Object o = Class.forName(cls).newInstance();
o.firstMethod();
} catch(ClassNotFoundException | IllegalAccessException | InstantiationException ex) {
System.out.println(ex.toString());
}
}
My problem is that when I try to call firstMethod() using the object o, I am getting this error.
exit status 1
Main.java:19: error: cannot find symbol
o.firstMethod();
^
symbol: method firstMethod()
location: variable o of type Object
1 error
I suspect that it is because it is of type Object and not type Class1. I have seen solutions where you typecast the object to the object of the class that you need. However when you typcast, you need to use the name of the class, which is exactly what I am trying to avoid. I need to use the class name as a string.
Does anyone know of a solution where I can call methods with the objects that are created?
java reflection
I have several classes in the same package in Java. I want to instantiate objects of these classes from an array that has the class names as strings.
Here is an example of a class I would like to use, they all have the same structure.
class Class1 {
public String firstMethod(){
String data = {"NEW_ITEM"};
return data;
}
}
Here is the class I am attemtempting to instantiate them from.
class Main {
static {
String classes = {"Class1","Class2"};
for (String cls : classes) {
try {
Object o = Class.forName(cls).newInstance();
o.firstMethod();
} catch(ClassNotFoundException | IllegalAccessException | InstantiationException ex) {
System.out.println(ex.toString());
}
}
My problem is that when I try to call firstMethod() using the object o, I am getting this error.
exit status 1
Main.java:19: error: cannot find symbol
o.firstMethod();
^
symbol: method firstMethod()
location: variable o of type Object
1 error
I suspect that it is because it is of type Object and not type Class1. I have seen solutions where you typecast the object to the object of the class that you need. However when you typcast, you need to use the name of the class, which is exactly what I am trying to avoid. I need to use the class name as a string.
Does anyone know of a solution where I can call methods with the objects that are created?
java reflection
java reflection
asked Nov 23 '18 at 21:50
J.DorJ.Dor
12
12
1
continue using reflection likeMethod.invoke()
- usegetMethod
orgetDeclaredMethod
of the class to get the method, callinvoke
on it, passing the created instance as parameter - e.g. stackoverflow.com/q/160970/85421
– Carlos Heuberger
Nov 23 '18 at 21:53
1
I assume that these classes have something in common, generally speaking they implement some kind of interface. In this case you can simply cast to the interface and call that method. If this is not possible, you have to invoke the methods via reflection as well.
– Glains
Nov 23 '18 at 21:53
Carlos Heuberger, thanks for helping a noob. Method method = o.getClass().getDeclaredMethod("firstMethod"); method.invoke(o); I got it working with this.
– J.Dor
Nov 23 '18 at 22:01
in case the method is private you can use the method.setAccessible(true);
– nmorenor
Nov 23 '18 at 23:39
add a comment |
1
continue using reflection likeMethod.invoke()
- usegetMethod
orgetDeclaredMethod
of the class to get the method, callinvoke
on it, passing the created instance as parameter - e.g. stackoverflow.com/q/160970/85421
– Carlos Heuberger
Nov 23 '18 at 21:53
1
I assume that these classes have something in common, generally speaking they implement some kind of interface. In this case you can simply cast to the interface and call that method. If this is not possible, you have to invoke the methods via reflection as well.
– Glains
Nov 23 '18 at 21:53
Carlos Heuberger, thanks for helping a noob. Method method = o.getClass().getDeclaredMethod("firstMethod"); method.invoke(o); I got it working with this.
– J.Dor
Nov 23 '18 at 22:01
in case the method is private you can use the method.setAccessible(true);
– nmorenor
Nov 23 '18 at 23:39
1
1
continue using reflection like
Method.invoke()
- use getMethod
or getDeclaredMethod
of the class to get the method, call invoke
on it, passing the created instance as parameter - e.g. stackoverflow.com/q/160970/85421– Carlos Heuberger
Nov 23 '18 at 21:53
continue using reflection like
Method.invoke()
- use getMethod
or getDeclaredMethod
of the class to get the method, call invoke
on it, passing the created instance as parameter - e.g. stackoverflow.com/q/160970/85421– Carlos Heuberger
Nov 23 '18 at 21:53
1
1
I assume that these classes have something in common, generally speaking they implement some kind of interface. In this case you can simply cast to the interface and call that method. If this is not possible, you have to invoke the methods via reflection as well.
– Glains
Nov 23 '18 at 21:53
I assume that these classes have something in common, generally speaking they implement some kind of interface. In this case you can simply cast to the interface and call that method. If this is not possible, you have to invoke the methods via reflection as well.
– Glains
Nov 23 '18 at 21:53
Carlos Heuberger, thanks for helping a noob. Method method = o.getClass().getDeclaredMethod("firstMethod"); method.invoke(o); I got it working with this.
– J.Dor
Nov 23 '18 at 22:01
Carlos Heuberger, thanks for helping a noob. Method method = o.getClass().getDeclaredMethod("firstMethod"); method.invoke(o); I got it working with this.
– J.Dor
Nov 23 '18 at 22:01
in case the method is private you can use the method.setAccessible(true);
– nmorenor
Nov 23 '18 at 23:39
in case the method is private you can use the method.setAccessible(true);
– nmorenor
Nov 23 '18 at 23:39
add a comment |
1 Answer
1
active
oldest
votes
You can't call your method the way in your code because you have an object which does not know the type Class1. You need to cast it explicitly like
((Class1)o).firstMethod()
which I don't think this is what you want.
Or, you can iterate through object methods and invoke it dynamically like below:
String classes = {"com.yourpackage.Class1", "com.yourpackage.Class2"};
for (String cls : classes) {
try {
Object o = Class.forName(cls).newInstance();
for(Method m : o.getClass().getMethods()) {
System.out.println(m.getName());
if ("firstMethod".equals(m.getName())) {
String data = (String)m.invoke(o, null); // here are the parameters
for(String d : data){
System.out.println(d);
}
}
}
} catch (ClassNotFoundException | IllegalAccessException | InstantiationException ex) {
System.out.println(ex.toString());
} catch (InvocationTargetException e) {
e.printStackTrace();
}
}
The output is :
NEW_ITEM
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53453277%2finstantiate-and-use-an-object-with-only-the-textual-class-name-in-java%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can't call your method the way in your code because you have an object which does not know the type Class1. You need to cast it explicitly like
((Class1)o).firstMethod()
which I don't think this is what you want.
Or, you can iterate through object methods and invoke it dynamically like below:
String classes = {"com.yourpackage.Class1", "com.yourpackage.Class2"};
for (String cls : classes) {
try {
Object o = Class.forName(cls).newInstance();
for(Method m : o.getClass().getMethods()) {
System.out.println(m.getName());
if ("firstMethod".equals(m.getName())) {
String data = (String)m.invoke(o, null); // here are the parameters
for(String d : data){
System.out.println(d);
}
}
}
} catch (ClassNotFoundException | IllegalAccessException | InstantiationException ex) {
System.out.println(ex.toString());
} catch (InvocationTargetException e) {
e.printStackTrace();
}
}
The output is :
NEW_ITEM
add a comment |
You can't call your method the way in your code because you have an object which does not know the type Class1. You need to cast it explicitly like
((Class1)o).firstMethod()
which I don't think this is what you want.
Or, you can iterate through object methods and invoke it dynamically like below:
String classes = {"com.yourpackage.Class1", "com.yourpackage.Class2"};
for (String cls : classes) {
try {
Object o = Class.forName(cls).newInstance();
for(Method m : o.getClass().getMethods()) {
System.out.println(m.getName());
if ("firstMethod".equals(m.getName())) {
String data = (String)m.invoke(o, null); // here are the parameters
for(String d : data){
System.out.println(d);
}
}
}
} catch (ClassNotFoundException | IllegalAccessException | InstantiationException ex) {
System.out.println(ex.toString());
} catch (InvocationTargetException e) {
e.printStackTrace();
}
}
The output is :
NEW_ITEM
add a comment |
You can't call your method the way in your code because you have an object which does not know the type Class1. You need to cast it explicitly like
((Class1)o).firstMethod()
which I don't think this is what you want.
Or, you can iterate through object methods and invoke it dynamically like below:
String classes = {"com.yourpackage.Class1", "com.yourpackage.Class2"};
for (String cls : classes) {
try {
Object o = Class.forName(cls).newInstance();
for(Method m : o.getClass().getMethods()) {
System.out.println(m.getName());
if ("firstMethod".equals(m.getName())) {
String data = (String)m.invoke(o, null); // here are the parameters
for(String d : data){
System.out.println(d);
}
}
}
} catch (ClassNotFoundException | IllegalAccessException | InstantiationException ex) {
System.out.println(ex.toString());
} catch (InvocationTargetException e) {
e.printStackTrace();
}
}
The output is :
NEW_ITEM
You can't call your method the way in your code because you have an object which does not know the type Class1. You need to cast it explicitly like
((Class1)o).firstMethod()
which I don't think this is what you want.
Or, you can iterate through object methods and invoke it dynamically like below:
String classes = {"com.yourpackage.Class1", "com.yourpackage.Class2"};
for (String cls : classes) {
try {
Object o = Class.forName(cls).newInstance();
for(Method m : o.getClass().getMethods()) {
System.out.println(m.getName());
if ("firstMethod".equals(m.getName())) {
String data = (String)m.invoke(o, null); // here are the parameters
for(String d : data){
System.out.println(d);
}
}
}
} catch (ClassNotFoundException | IllegalAccessException | InstantiationException ex) {
System.out.println(ex.toString());
} catch (InvocationTargetException e) {
e.printStackTrace();
}
}
The output is :
NEW_ITEM
answered Nov 25 '18 at 10:36
Emre SavcıEmre Savcı
2,0911819
2,0911819
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53453277%2finstantiate-and-use-an-object-with-only-the-textual-class-name-in-java%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
vbOEgD69ZFz a4T66Wr0fiUTYex0uG,m pTY9hj
1
continue using reflection like
Method.invoke()
- usegetMethod
orgetDeclaredMethod
of the class to get the method, callinvoke
on it, passing the created instance as parameter - e.g. stackoverflow.com/q/160970/85421– Carlos Heuberger
Nov 23 '18 at 21:53
1
I assume that these classes have something in common, generally speaking they implement some kind of interface. In this case you can simply cast to the interface and call that method. If this is not possible, you have to invoke the methods via reflection as well.
– Glains
Nov 23 '18 at 21:53
Carlos Heuberger, thanks for helping a noob. Method method = o.getClass().getDeclaredMethod("firstMethod"); method.invoke(o); I got it working with this.
– J.Dor
Nov 23 '18 at 22:01
in case the method is private you can use the method.setAccessible(true);
– nmorenor
Nov 23 '18 at 23:39