C Typedef Enum members in header file not defined in source file [closed]
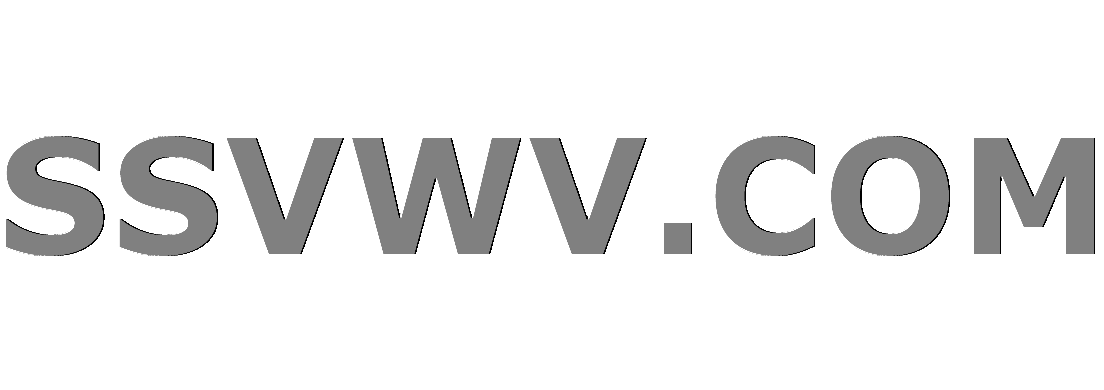
Multi tool use
probably a very basic question but it confuses me a lot.
Application
I am writing a driver for an I2C hardware interface. The idea is to have a module that does the actual hardware access (i2c_driver.c/.h) and a module describing the devices (i2c_devices.c/.h), that makes use of the i2c_driver.
The driver is meant to be used with a single function call that a structure describing the transaction is passed to. So for example, accessing a device would look like this:
I2C_Transaction_TypeDef trans;
trans.Instance = I2C1;
trans.Operation = I2C_OPERATION_WRITE;
trans.DeviceAddress = HDC1080_I2C_ADDR;
trans.RegAddress = HDC1080_TEMP_ADDR;
trans.Count = 2;
trans.pData = (uint8_t *)txbuf;
I2C_do_transaction(&trans);
The modules are used like this:
main.c -> i2c_devices.h -> i2c_drivers.h -> (low level MCU stuff)
i2c_devices.c i2c_drivers.c
Problem
The I2C_Transaction_TypeDef description for the transaction shown above contains a member "Operation" tha can be any option of fixed set of options. These options are stored in the i2c_driver.h as
typedef enum {
I2C_OPERATION_WRITE,
I2C_OPERATION_READ,
I2C_OPERATION_READ_ADDRESS
} I2C_Operations;
and therefore the I2C_Transaction_TypeDef member Operation is of type I2C_Operations to clearly state that one of these options/members shall be used.
This works fine for usage in the i2c_driver.c (includes i2c_driver.h), for something like this:
trans->Operation = I2C_OPERATION_WRITE;
What is the exact same line as in the first code snippet. However, in the first code snippet, I end up with an error.
identifier "I2C_OPERATION_WRITE" is undefined
If I change the i2c_devices.c to include the i2c_driver.h directly instead of indirectly through the the i2c_driver.h it works.
NOT WORKING:
i2c_devices.c -> i2c_devices.h -> i2c_drivers.h
WORKING:
i2c_devices.c -> i2c_devices.h
-> i2c_drivers.h
Please help me understand why.
c enums typedef member
closed as off-topic by Swordfish, Lundin, Gerhardh, Rishikesh Raje, M Oehm Nov 21 '18 at 12:02
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "This question was caused by a problem that can no longer be reproduced or a simple typographical error. While similar questions may be on-topic here, this one was resolved in a manner unlikely to help future readers. This can often be avoided by identifying and closely inspecting the shortest program necessary to reproduce the problem before posting." – Lundin, Gerhardh, Rishikesh Raje, M Oehm
If this question can be reworded to fit the rules in the help center, please edit the question.
|
show 1 more comment
probably a very basic question but it confuses me a lot.
Application
I am writing a driver for an I2C hardware interface. The idea is to have a module that does the actual hardware access (i2c_driver.c/.h) and a module describing the devices (i2c_devices.c/.h), that makes use of the i2c_driver.
The driver is meant to be used with a single function call that a structure describing the transaction is passed to. So for example, accessing a device would look like this:
I2C_Transaction_TypeDef trans;
trans.Instance = I2C1;
trans.Operation = I2C_OPERATION_WRITE;
trans.DeviceAddress = HDC1080_I2C_ADDR;
trans.RegAddress = HDC1080_TEMP_ADDR;
trans.Count = 2;
trans.pData = (uint8_t *)txbuf;
I2C_do_transaction(&trans);
The modules are used like this:
main.c -> i2c_devices.h -> i2c_drivers.h -> (low level MCU stuff)
i2c_devices.c i2c_drivers.c
Problem
The I2C_Transaction_TypeDef description for the transaction shown above contains a member "Operation" tha can be any option of fixed set of options. These options are stored in the i2c_driver.h as
typedef enum {
I2C_OPERATION_WRITE,
I2C_OPERATION_READ,
I2C_OPERATION_READ_ADDRESS
} I2C_Operations;
and therefore the I2C_Transaction_TypeDef member Operation is of type I2C_Operations to clearly state that one of these options/members shall be used.
This works fine for usage in the i2c_driver.c (includes i2c_driver.h), for something like this:
trans->Operation = I2C_OPERATION_WRITE;
What is the exact same line as in the first code snippet. However, in the first code snippet, I end up with an error.
identifier "I2C_OPERATION_WRITE" is undefined
If I change the i2c_devices.c to include the i2c_driver.h directly instead of indirectly through the the i2c_driver.h it works.
NOT WORKING:
i2c_devices.c -> i2c_devices.h -> i2c_drivers.h
WORKING:
i2c_devices.c -> i2c_devices.h
-> i2c_drivers.h
Please help me understand why.
c enums typedef member
closed as off-topic by Swordfish, Lundin, Gerhardh, Rishikesh Raje, M Oehm Nov 21 '18 at 12:02
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "This question was caused by a problem that can no longer be reproduced or a simple typographical error. While similar questions may be on-topic here, this one was resolved in a manner unlikely to help future readers. This can often be avoided by identifying and closely inspecting the shortest program necessary to reproduce the problem before posting." – Lundin, Gerhardh, Rishikesh Raje, M Oehm
If this question can be reworded to fit the rules in the help center, please edit the question.
3
Without a MCVE we can only guess. I would assume your include guards in the headers are wrong.
– Gerhardh
Nov 21 '18 at 11:10
You could try to only run the preprocessor and check what is going on.
– Gerhardh
Nov 21 '18 at 11:10
Check headers are included, I assume Operation is declared as type I2C_Operations?
– SPlatten
Nov 21 '18 at 11:13
Please post all the.c
files with relevant#include
statements. My magic glass tells me you have circular includes and include guards are declared when you re-include i2c_drivers.h
– Kamil Cuk
Nov 21 '18 at 11:14
1
Then I'm voting to close this as "cannot be reproduced".
– Lundin
Nov 21 '18 at 11:30
|
show 1 more comment
probably a very basic question but it confuses me a lot.
Application
I am writing a driver for an I2C hardware interface. The idea is to have a module that does the actual hardware access (i2c_driver.c/.h) and a module describing the devices (i2c_devices.c/.h), that makes use of the i2c_driver.
The driver is meant to be used with a single function call that a structure describing the transaction is passed to. So for example, accessing a device would look like this:
I2C_Transaction_TypeDef trans;
trans.Instance = I2C1;
trans.Operation = I2C_OPERATION_WRITE;
trans.DeviceAddress = HDC1080_I2C_ADDR;
trans.RegAddress = HDC1080_TEMP_ADDR;
trans.Count = 2;
trans.pData = (uint8_t *)txbuf;
I2C_do_transaction(&trans);
The modules are used like this:
main.c -> i2c_devices.h -> i2c_drivers.h -> (low level MCU stuff)
i2c_devices.c i2c_drivers.c
Problem
The I2C_Transaction_TypeDef description for the transaction shown above contains a member "Operation" tha can be any option of fixed set of options. These options are stored in the i2c_driver.h as
typedef enum {
I2C_OPERATION_WRITE,
I2C_OPERATION_READ,
I2C_OPERATION_READ_ADDRESS
} I2C_Operations;
and therefore the I2C_Transaction_TypeDef member Operation is of type I2C_Operations to clearly state that one of these options/members shall be used.
This works fine for usage in the i2c_driver.c (includes i2c_driver.h), for something like this:
trans->Operation = I2C_OPERATION_WRITE;
What is the exact same line as in the first code snippet. However, in the first code snippet, I end up with an error.
identifier "I2C_OPERATION_WRITE" is undefined
If I change the i2c_devices.c to include the i2c_driver.h directly instead of indirectly through the the i2c_driver.h it works.
NOT WORKING:
i2c_devices.c -> i2c_devices.h -> i2c_drivers.h
WORKING:
i2c_devices.c -> i2c_devices.h
-> i2c_drivers.h
Please help me understand why.
c enums typedef member
probably a very basic question but it confuses me a lot.
Application
I am writing a driver for an I2C hardware interface. The idea is to have a module that does the actual hardware access (i2c_driver.c/.h) and a module describing the devices (i2c_devices.c/.h), that makes use of the i2c_driver.
The driver is meant to be used with a single function call that a structure describing the transaction is passed to. So for example, accessing a device would look like this:
I2C_Transaction_TypeDef trans;
trans.Instance = I2C1;
trans.Operation = I2C_OPERATION_WRITE;
trans.DeviceAddress = HDC1080_I2C_ADDR;
trans.RegAddress = HDC1080_TEMP_ADDR;
trans.Count = 2;
trans.pData = (uint8_t *)txbuf;
I2C_do_transaction(&trans);
The modules are used like this:
main.c -> i2c_devices.h -> i2c_drivers.h -> (low level MCU stuff)
i2c_devices.c i2c_drivers.c
Problem
The I2C_Transaction_TypeDef description for the transaction shown above contains a member "Operation" tha can be any option of fixed set of options. These options are stored in the i2c_driver.h as
typedef enum {
I2C_OPERATION_WRITE,
I2C_OPERATION_READ,
I2C_OPERATION_READ_ADDRESS
} I2C_Operations;
and therefore the I2C_Transaction_TypeDef member Operation is of type I2C_Operations to clearly state that one of these options/members shall be used.
This works fine for usage in the i2c_driver.c (includes i2c_driver.h), for something like this:
trans->Operation = I2C_OPERATION_WRITE;
What is the exact same line as in the first code snippet. However, in the first code snippet, I end up with an error.
identifier "I2C_OPERATION_WRITE" is undefined
If I change the i2c_devices.c to include the i2c_driver.h directly instead of indirectly through the the i2c_driver.h it works.
NOT WORKING:
i2c_devices.c -> i2c_devices.h -> i2c_drivers.h
WORKING:
i2c_devices.c -> i2c_devices.h
-> i2c_drivers.h
Please help me understand why.
c enums typedef member
c enums typedef member
asked Nov 21 '18 at 11:08
L. Heinrichs
1107
1107
closed as off-topic by Swordfish, Lundin, Gerhardh, Rishikesh Raje, M Oehm Nov 21 '18 at 12:02
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "This question was caused by a problem that can no longer be reproduced or a simple typographical error. While similar questions may be on-topic here, this one was resolved in a manner unlikely to help future readers. This can often be avoided by identifying and closely inspecting the shortest program necessary to reproduce the problem before posting." – Lundin, Gerhardh, Rishikesh Raje, M Oehm
If this question can be reworded to fit the rules in the help center, please edit the question.
closed as off-topic by Swordfish, Lundin, Gerhardh, Rishikesh Raje, M Oehm Nov 21 '18 at 12:02
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "This question was caused by a problem that can no longer be reproduced or a simple typographical error. While similar questions may be on-topic here, this one was resolved in a manner unlikely to help future readers. This can often be avoided by identifying and closely inspecting the shortest program necessary to reproduce the problem before posting." – Lundin, Gerhardh, Rishikesh Raje, M Oehm
If this question can be reworded to fit the rules in the help center, please edit the question.
3
Without a MCVE we can only guess. I would assume your include guards in the headers are wrong.
– Gerhardh
Nov 21 '18 at 11:10
You could try to only run the preprocessor and check what is going on.
– Gerhardh
Nov 21 '18 at 11:10
Check headers are included, I assume Operation is declared as type I2C_Operations?
– SPlatten
Nov 21 '18 at 11:13
Please post all the.c
files with relevant#include
statements. My magic glass tells me you have circular includes and include guards are declared when you re-include i2c_drivers.h
– Kamil Cuk
Nov 21 '18 at 11:14
1
Then I'm voting to close this as "cannot be reproduced".
– Lundin
Nov 21 '18 at 11:30
|
show 1 more comment
3
Without a MCVE we can only guess. I would assume your include guards in the headers are wrong.
– Gerhardh
Nov 21 '18 at 11:10
You could try to only run the preprocessor and check what is going on.
– Gerhardh
Nov 21 '18 at 11:10
Check headers are included, I assume Operation is declared as type I2C_Operations?
– SPlatten
Nov 21 '18 at 11:13
Please post all the.c
files with relevant#include
statements. My magic glass tells me you have circular includes and include guards are declared when you re-include i2c_drivers.h
– Kamil Cuk
Nov 21 '18 at 11:14
1
Then I'm voting to close this as "cannot be reproduced".
– Lundin
Nov 21 '18 at 11:30
3
3
Without a MCVE we can only guess. I would assume your include guards in the headers are wrong.
– Gerhardh
Nov 21 '18 at 11:10
Without a MCVE we can only guess. I would assume your include guards in the headers are wrong.
– Gerhardh
Nov 21 '18 at 11:10
You could try to only run the preprocessor and check what is going on.
– Gerhardh
Nov 21 '18 at 11:10
You could try to only run the preprocessor and check what is going on.
– Gerhardh
Nov 21 '18 at 11:10
Check headers are included, I assume Operation is declared as type I2C_Operations?
– SPlatten
Nov 21 '18 at 11:13
Check headers are included, I assume Operation is declared as type I2C_Operations?
– SPlatten
Nov 21 '18 at 11:13
Please post all the
.c
files with relevant #include
statements. My magic glass tells me you have circular includes and include guards are declared when you re-include i2c_drivers.h– Kamil Cuk
Nov 21 '18 at 11:14
Please post all the
.c
files with relevant #include
statements. My magic glass tells me you have circular includes and include guards are declared when you re-include i2c_drivers.h– Kamil Cuk
Nov 21 '18 at 11:14
1
1
Then I'm voting to close this as "cannot be reproduced".
– Lundin
Nov 21 '18 at 11:30
Then I'm voting to close this as "cannot be reproduced".
– Lundin
Nov 21 '18 at 11:30
|
show 1 more comment
1 Answer
1
active
oldest
votes
As some of you pointed out in the comments below the question (good spot guys!), I had a copy/paste error: When I split the previous module into devices and driver, i did not adjust the include guards in the header files, so they were identical.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
As some of you pointed out in the comments below the question (good spot guys!), I had a copy/paste error: When I split the previous module into devices and driver, i did not adjust the include guards in the header files, so they were identical.
add a comment |
As some of you pointed out in the comments below the question (good spot guys!), I had a copy/paste error: When I split the previous module into devices and driver, i did not adjust the include guards in the header files, so they were identical.
add a comment |
As some of you pointed out in the comments below the question (good spot guys!), I had a copy/paste error: When I split the previous module into devices and driver, i did not adjust the include guards in the header files, so they were identical.
As some of you pointed out in the comments below the question (good spot guys!), I had a copy/paste error: When I split the previous module into devices and driver, i did not adjust the include guards in the header files, so they were identical.
answered Nov 21 '18 at 11:23
L. Heinrichs
1107
1107
add a comment |
add a comment |
DKGRVLAMSUIBy,rtWP0R0EKGJ2FXjVcYRby,bGH,pKDG,7,9rJMR zAfhi8y
3
Without a MCVE we can only guess. I would assume your include guards in the headers are wrong.
– Gerhardh
Nov 21 '18 at 11:10
You could try to only run the preprocessor and check what is going on.
– Gerhardh
Nov 21 '18 at 11:10
Check headers are included, I assume Operation is declared as type I2C_Operations?
– SPlatten
Nov 21 '18 at 11:13
Please post all the
.c
files with relevant#include
statements. My magic glass tells me you have circular includes and include guards are declared when you re-include i2c_drivers.h– Kamil Cuk
Nov 21 '18 at 11:14
1
Then I'm voting to close this as "cannot be reproduced".
– Lundin
Nov 21 '18 at 11:30