Uncaught Error: Call to a member function fetchAll()
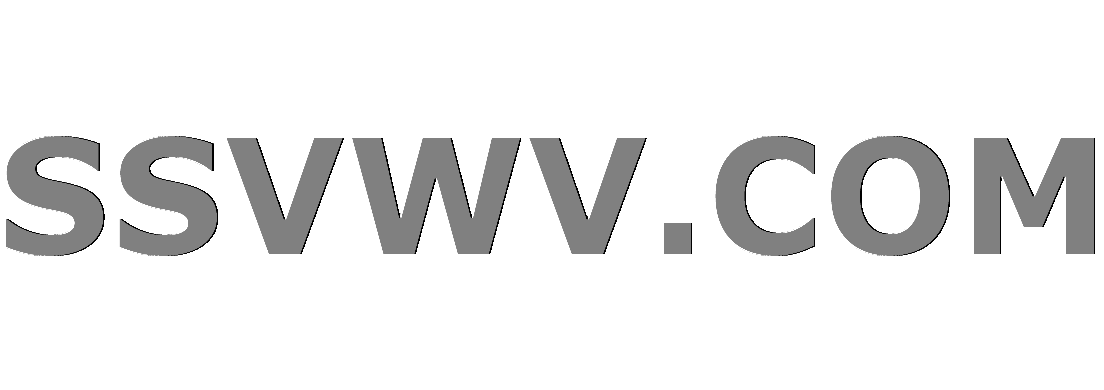
Multi tool use
Guys i am trying to do a webservice for uni project. Everything seems ok untill i run the code and get this error Uncaught Error: Call to a member function fetchAll() on null . This is the code can anyone please tell me what is wrong with it?
<?php
header("Content-type: application/json");
$conn = new PDO("mysql:host=localhost;dbname=user;", "user", "pass");
$loc = $_GET["location"];
$type = $_GET["type"];
if(isset($_GET["location"]) && isset($_GET["type"]))
{
$result = $conn->query("Select * from resit_accommodation where location='$loc' and type='$type'")
}
else if (isset($_GET["location"]))
{
$result = $conn->query("Select * from resit_accommodation where location='$loc'");
}
else if (isset($_GET["type"]))
{
$result = $conn->query("Select * from resit_accommodation where location='$type'");
}
$rows = $result->fetchAll(PDO::FETCH_ASSOC);
echo json_encode($rows);
?>
php mysql pdo
add a comment |
Guys i am trying to do a webservice for uni project. Everything seems ok untill i run the code and get this error Uncaught Error: Call to a member function fetchAll() on null . This is the code can anyone please tell me what is wrong with it?
<?php
header("Content-type: application/json");
$conn = new PDO("mysql:host=localhost;dbname=user;", "user", "pass");
$loc = $_GET["location"];
$type = $_GET["type"];
if(isset($_GET["location"]) && isset($_GET["type"]))
{
$result = $conn->query("Select * from resit_accommodation where location='$loc' and type='$type'")
}
else if (isset($_GET["location"]))
{
$result = $conn->query("Select * from resit_accommodation where location='$loc'");
}
else if (isset($_GET["type"]))
{
$result = $conn->query("Select * from resit_accommodation where location='$type'");
}
$rows = $result->fetchAll(PDO::FETCH_ASSOC);
echo json_encode($rows);
?>
php mysql pdo
2
If none of theif
conditions are true, you never set$result
.
– Barmar
Mar 29 '18 at 20:48
2
You also are open to SQL injections. You should parameterize the parameters you are passing in.
– chris85
Mar 29 '18 at 20:51
WARNING: When using PDO you should be using prepared statements with placeholder values and supply any user data as separate arguments. In this code you have potentially severe SQL injection bugs. Never use string interpolation or concatenation and instead use prepared statements and never put$_POST
,$_GET
or any user data directly in your query. Refer to PHP The Right Way for general guidance and advice.
– tadman
Mar 29 '18 at 21:13
add a comment |
Guys i am trying to do a webservice for uni project. Everything seems ok untill i run the code and get this error Uncaught Error: Call to a member function fetchAll() on null . This is the code can anyone please tell me what is wrong with it?
<?php
header("Content-type: application/json");
$conn = new PDO("mysql:host=localhost;dbname=user;", "user", "pass");
$loc = $_GET["location"];
$type = $_GET["type"];
if(isset($_GET["location"]) && isset($_GET["type"]))
{
$result = $conn->query("Select * from resit_accommodation where location='$loc' and type='$type'")
}
else if (isset($_GET["location"]))
{
$result = $conn->query("Select * from resit_accommodation where location='$loc'");
}
else if (isset($_GET["type"]))
{
$result = $conn->query("Select * from resit_accommodation where location='$type'");
}
$rows = $result->fetchAll(PDO::FETCH_ASSOC);
echo json_encode($rows);
?>
php mysql pdo
Guys i am trying to do a webservice for uni project. Everything seems ok untill i run the code and get this error Uncaught Error: Call to a member function fetchAll() on null . This is the code can anyone please tell me what is wrong with it?
<?php
header("Content-type: application/json");
$conn = new PDO("mysql:host=localhost;dbname=user;", "user", "pass");
$loc = $_GET["location"];
$type = $_GET["type"];
if(isset($_GET["location"]) && isset($_GET["type"]))
{
$result = $conn->query("Select * from resit_accommodation where location='$loc' and type='$type'")
}
else if (isset($_GET["location"]))
{
$result = $conn->query("Select * from resit_accommodation where location='$loc'");
}
else if (isset($_GET["type"]))
{
$result = $conn->query("Select * from resit_accommodation where location='$type'");
}
$rows = $result->fetchAll(PDO::FETCH_ASSOC);
echo json_encode($rows);
?>
php mysql pdo
php mysql pdo
edited Mar 29 '18 at 21:12
tadman
152k18173207
152k18173207
asked Mar 29 '18 at 20:47
Gabriel GanchevGabriel Ganchev
1
1
2
If none of theif
conditions are true, you never set$result
.
– Barmar
Mar 29 '18 at 20:48
2
You also are open to SQL injections. You should parameterize the parameters you are passing in.
– chris85
Mar 29 '18 at 20:51
WARNING: When using PDO you should be using prepared statements with placeholder values and supply any user data as separate arguments. In this code you have potentially severe SQL injection bugs. Never use string interpolation or concatenation and instead use prepared statements and never put$_POST
,$_GET
or any user data directly in your query. Refer to PHP The Right Way for general guidance and advice.
– tadman
Mar 29 '18 at 21:13
add a comment |
2
If none of theif
conditions are true, you never set$result
.
– Barmar
Mar 29 '18 at 20:48
2
You also are open to SQL injections. You should parameterize the parameters you are passing in.
– chris85
Mar 29 '18 at 20:51
WARNING: When using PDO you should be using prepared statements with placeholder values and supply any user data as separate arguments. In this code you have potentially severe SQL injection bugs. Never use string interpolation or concatenation and instead use prepared statements and never put$_POST
,$_GET
or any user data directly in your query. Refer to PHP The Right Way for general guidance and advice.
– tadman
Mar 29 '18 at 21:13
2
2
If none of the
if
conditions are true, you never set $result
.– Barmar
Mar 29 '18 at 20:48
If none of the
if
conditions are true, you never set $result
.– Barmar
Mar 29 '18 at 20:48
2
2
You also are open to SQL injections. You should parameterize the parameters you are passing in.
– chris85
Mar 29 '18 at 20:51
You also are open to SQL injections. You should parameterize the parameters you are passing in.
– chris85
Mar 29 '18 at 20:51
WARNING: When using PDO you should be using prepared statements with placeholder values and supply any user data as separate arguments. In this code you have potentially severe SQL injection bugs. Never use string interpolation or concatenation and instead use prepared statements and never put
$_POST
, $_GET
or any user data directly in your query. Refer to PHP The Right Way for general guidance and advice.– tadman
Mar 29 '18 at 21:13
WARNING: When using PDO you should be using prepared statements with placeholder values and supply any user data as separate arguments. In this code you have potentially severe SQL injection bugs. Never use string interpolation or concatenation and instead use prepared statements and never put
$_POST
, $_GET
or any user data directly in your query. Refer to PHP The Right Way for general guidance and advice.– tadman
Mar 29 '18 at 21:13
add a comment |
1 Answer
1
active
oldest
votes
If neither of the $_GET
parameters is set, you never set $result
to anything, so you'll get an error if you try to use it.
You should also use a prepared statement rather than substituting variables, to prevent SQL injection.
<?php
header("Content-type: application/json");
$conn = new PDO("mysql:host=localhost;dbname=user;", "user", "pass");
$stmt = null;
if(isset($_GET["location"]) && isset($_GET["type"]))
{
$stmt = $conn->prepare("Select * from resit_accommodation where location= :loc and type= :type");
$stmt->execute(['loc' => $_GET['location'], 'type' => $_GET['type']]);
}
elseif (isset($_GET["location"]))
{
$stmt = $conn->prepare("Select * from resit_accommodation where location= :loc");
$stmt->execute(['loc' => $_GET['location']]);
}
elseif (isset($_GET["type"]))
{
$stmt = $conn->prepare("Select * from resit_accommodation where location= :type");
$stmt->execute(['type' => $_GET['type']]);
}
if ($stmt) {
$rows = $stmt->fetchAll(PDO::FETCH_ASSOC);
} else {
$rows = ;
}
echo json_encode($rows);
?>
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f49564767%2funcaught-error-call-to-a-member-function-fetchall%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
If neither of the $_GET
parameters is set, you never set $result
to anything, so you'll get an error if you try to use it.
You should also use a prepared statement rather than substituting variables, to prevent SQL injection.
<?php
header("Content-type: application/json");
$conn = new PDO("mysql:host=localhost;dbname=user;", "user", "pass");
$stmt = null;
if(isset($_GET["location"]) && isset($_GET["type"]))
{
$stmt = $conn->prepare("Select * from resit_accommodation where location= :loc and type= :type");
$stmt->execute(['loc' => $_GET['location'], 'type' => $_GET['type']]);
}
elseif (isset($_GET["location"]))
{
$stmt = $conn->prepare("Select * from resit_accommodation where location= :loc");
$stmt->execute(['loc' => $_GET['location']]);
}
elseif (isset($_GET["type"]))
{
$stmt = $conn->prepare("Select * from resit_accommodation where location= :type");
$stmt->execute(['type' => $_GET['type']]);
}
if ($stmt) {
$rows = $stmt->fetchAll(PDO::FETCH_ASSOC);
} else {
$rows = ;
}
echo json_encode($rows);
?>
add a comment |
If neither of the $_GET
parameters is set, you never set $result
to anything, so you'll get an error if you try to use it.
You should also use a prepared statement rather than substituting variables, to prevent SQL injection.
<?php
header("Content-type: application/json");
$conn = new PDO("mysql:host=localhost;dbname=user;", "user", "pass");
$stmt = null;
if(isset($_GET["location"]) && isset($_GET["type"]))
{
$stmt = $conn->prepare("Select * from resit_accommodation where location= :loc and type= :type");
$stmt->execute(['loc' => $_GET['location'], 'type' => $_GET['type']]);
}
elseif (isset($_GET["location"]))
{
$stmt = $conn->prepare("Select * from resit_accommodation where location= :loc");
$stmt->execute(['loc' => $_GET['location']]);
}
elseif (isset($_GET["type"]))
{
$stmt = $conn->prepare("Select * from resit_accommodation where location= :type");
$stmt->execute(['type' => $_GET['type']]);
}
if ($stmt) {
$rows = $stmt->fetchAll(PDO::FETCH_ASSOC);
} else {
$rows = ;
}
echo json_encode($rows);
?>
add a comment |
If neither of the $_GET
parameters is set, you never set $result
to anything, so you'll get an error if you try to use it.
You should also use a prepared statement rather than substituting variables, to prevent SQL injection.
<?php
header("Content-type: application/json");
$conn = new PDO("mysql:host=localhost;dbname=user;", "user", "pass");
$stmt = null;
if(isset($_GET["location"]) && isset($_GET["type"]))
{
$stmt = $conn->prepare("Select * from resit_accommodation where location= :loc and type= :type");
$stmt->execute(['loc' => $_GET['location'], 'type' => $_GET['type']]);
}
elseif (isset($_GET["location"]))
{
$stmt = $conn->prepare("Select * from resit_accommodation where location= :loc");
$stmt->execute(['loc' => $_GET['location']]);
}
elseif (isset($_GET["type"]))
{
$stmt = $conn->prepare("Select * from resit_accommodation where location= :type");
$stmt->execute(['type' => $_GET['type']]);
}
if ($stmt) {
$rows = $stmt->fetchAll(PDO::FETCH_ASSOC);
} else {
$rows = ;
}
echo json_encode($rows);
?>
If neither of the $_GET
parameters is set, you never set $result
to anything, so you'll get an error if you try to use it.
You should also use a prepared statement rather than substituting variables, to prevent SQL injection.
<?php
header("Content-type: application/json");
$conn = new PDO("mysql:host=localhost;dbname=user;", "user", "pass");
$stmt = null;
if(isset($_GET["location"]) && isset($_GET["type"]))
{
$stmt = $conn->prepare("Select * from resit_accommodation where location= :loc and type= :type");
$stmt->execute(['loc' => $_GET['location'], 'type' => $_GET['type']]);
}
elseif (isset($_GET["location"]))
{
$stmt = $conn->prepare("Select * from resit_accommodation where location= :loc");
$stmt->execute(['loc' => $_GET['location']]);
}
elseif (isset($_GET["type"]))
{
$stmt = $conn->prepare("Select * from resit_accommodation where location= :type");
$stmt->execute(['type' => $_GET['type']]);
}
if ($stmt) {
$rows = $stmt->fetchAll(PDO::FETCH_ASSOC);
} else {
$rows = ;
}
echo json_encode($rows);
?>
answered Mar 29 '18 at 20:54
BarmarBarmar
420k35244344
420k35244344
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f49564767%2funcaught-error-call-to-a-member-function-fetchall%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
iFB0 Tb0TUFwtOc7Iqa g,bgHEaa0Ba2SPKz,9guZ4goKVd BwUDXIMnb
2
If none of the
if
conditions are true, you never set$result
.– Barmar
Mar 29 '18 at 20:48
2
You also are open to SQL injections. You should parameterize the parameters you are passing in.
– chris85
Mar 29 '18 at 20:51
WARNING: When using PDO you should be using prepared statements with placeholder values and supply any user data as separate arguments. In this code you have potentially severe SQL injection bugs. Never use string interpolation or concatenation and instead use prepared statements and never put
$_POST
,$_GET
or any user data directly in your query. Refer to PHP The Right Way for general guidance and advice.– tadman
Mar 29 '18 at 21:13