How to override rescue_from in application controller for one specific instance
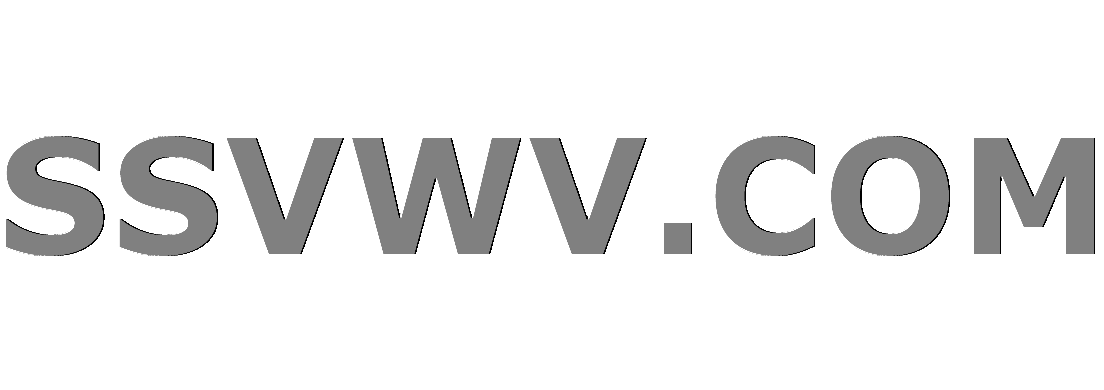
Multi tool use
I have this in my application_controller.rb
:
rescue_from Pundit::NotAuthorizedError, with: :user_not_authorized
def user_not_authorized
redirect_back fallback_location: root_url,
warning: 'Not authorized'
end
but for one method, I need
def slug_available
authorize Page
rescue Pundit::NotAuthorizedError
render status: :unauthorized
else
render json: { available: Page.where(slug: params[:slug]).empty? }
end
However, the rescue_from
is overriding the explicit rescue
in slug_available
, and I am getting a 302 Found instead of a 401 Unauthorized.
I would have thought an explicit rescue
would have taken priority. How can I make this happen?
ruby-on-rails ruby
add a comment |
I have this in my application_controller.rb
:
rescue_from Pundit::NotAuthorizedError, with: :user_not_authorized
def user_not_authorized
redirect_back fallback_location: root_url,
warning: 'Not authorized'
end
but for one method, I need
def slug_available
authorize Page
rescue Pundit::NotAuthorizedError
render status: :unauthorized
else
render json: { available: Page.where(slug: params[:slug]).empty? }
end
However, the rescue_from
is overriding the explicit rescue
in slug_available
, and I am getting a 302 Found instead of a 401 Unauthorized.
I would have thought an explicit rescue
would have taken priority. How can I make this happen?
ruby-on-rails ruby
add a comment |
I have this in my application_controller.rb
:
rescue_from Pundit::NotAuthorizedError, with: :user_not_authorized
def user_not_authorized
redirect_back fallback_location: root_url,
warning: 'Not authorized'
end
but for one method, I need
def slug_available
authorize Page
rescue Pundit::NotAuthorizedError
render status: :unauthorized
else
render json: { available: Page.where(slug: params[:slug]).empty? }
end
However, the rescue_from
is overriding the explicit rescue
in slug_available
, and I am getting a 302 Found instead of a 401 Unauthorized.
I would have thought an explicit rescue
would have taken priority. How can I make this happen?
ruby-on-rails ruby
I have this in my application_controller.rb
:
rescue_from Pundit::NotAuthorizedError, with: :user_not_authorized
def user_not_authorized
redirect_back fallback_location: root_url,
warning: 'Not authorized'
end
but for one method, I need
def slug_available
authorize Page
rescue Pundit::NotAuthorizedError
render status: :unauthorized
else
render json: { available: Page.where(slug: params[:slug]).empty? }
end
However, the rescue_from
is overriding the explicit rescue
in slug_available
, and I am getting a 302 Found instead of a 401 Unauthorized.
I would have thought an explicit rescue
would have taken priority. How can I make this happen?
ruby-on-rails ruby
ruby-on-rails ruby
asked Nov 23 '18 at 12:27
John YJohn Y
663516
663516
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
You can overwrite the not_authorized
declaration method in your controller and check the action_name
.
protected
def not_authorized
if action_name == 'slug_available'
render status: :unauthorized
else
super
end
end
then, you do not need to authorize your slug_avaiable
action method
def slug_available
render json: { available: Page.where(slug: params[:slug]).empty? }
end
The ApplicationController
base method needs to be a protected method.
add a comment |
It turned out that replacing
render status: :unauthorized
with
render plain: 'Not authorized.', status: :unauthorized
fixed the problem. It looks like Rails was trying to render the current page as HTML, with a 401 status, until I was explicit about what to render.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53446725%2fhow-to-override-rescue-from-in-application-controller-for-one-specific-instance%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can overwrite the not_authorized
declaration method in your controller and check the action_name
.
protected
def not_authorized
if action_name == 'slug_available'
render status: :unauthorized
else
super
end
end
then, you do not need to authorize your slug_avaiable
action method
def slug_available
render json: { available: Page.where(slug: params[:slug]).empty? }
end
The ApplicationController
base method needs to be a protected method.
add a comment |
You can overwrite the not_authorized
declaration method in your controller and check the action_name
.
protected
def not_authorized
if action_name == 'slug_available'
render status: :unauthorized
else
super
end
end
then, you do not need to authorize your slug_avaiable
action method
def slug_available
render json: { available: Page.where(slug: params[:slug]).empty? }
end
The ApplicationController
base method needs to be a protected method.
add a comment |
You can overwrite the not_authorized
declaration method in your controller and check the action_name
.
protected
def not_authorized
if action_name == 'slug_available'
render status: :unauthorized
else
super
end
end
then, you do not need to authorize your slug_avaiable
action method
def slug_available
render json: { available: Page.where(slug: params[:slug]).empty? }
end
The ApplicationController
base method needs to be a protected method.
You can overwrite the not_authorized
declaration method in your controller and check the action_name
.
protected
def not_authorized
if action_name == 'slug_available'
render status: :unauthorized
else
super
end
end
then, you do not need to authorize your slug_avaiable
action method
def slug_available
render json: { available: Page.where(slug: params[:slug]).empty? }
end
The ApplicationController
base method needs to be a protected method.
edited Nov 23 '18 at 13:55
answered Nov 23 '18 at 13:49


vzamanillovzamanillo
8,42212348
8,42212348
add a comment |
add a comment |
It turned out that replacing
render status: :unauthorized
with
render plain: 'Not authorized.', status: :unauthorized
fixed the problem. It looks like Rails was trying to render the current page as HTML, with a 401 status, until I was explicit about what to render.
add a comment |
It turned out that replacing
render status: :unauthorized
with
render plain: 'Not authorized.', status: :unauthorized
fixed the problem. It looks like Rails was trying to render the current page as HTML, with a 401 status, until I was explicit about what to render.
add a comment |
It turned out that replacing
render status: :unauthorized
with
render plain: 'Not authorized.', status: :unauthorized
fixed the problem. It looks like Rails was trying to render the current page as HTML, with a 401 status, until I was explicit about what to render.
It turned out that replacing
render status: :unauthorized
with
render plain: 'Not authorized.', status: :unauthorized
fixed the problem. It looks like Rails was trying to render the current page as HTML, with a 401 status, until I was explicit about what to render.
answered Nov 23 '18 at 16:04
John YJohn Y
663516
663516
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53446725%2fhow-to-override-rescue-from-in-application-controller-for-one-specific-instance%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
tAEa99ILPwDX KrkySEvjALYd2azqE,ZZ VZELb8xEB mmue 1bYOqMVIYtz4gAzcmScV,HKg93pi pIx,MGCUgBnta1,uYwV2HOe5o