Using an all semaphore program instead of mutex and cond_t
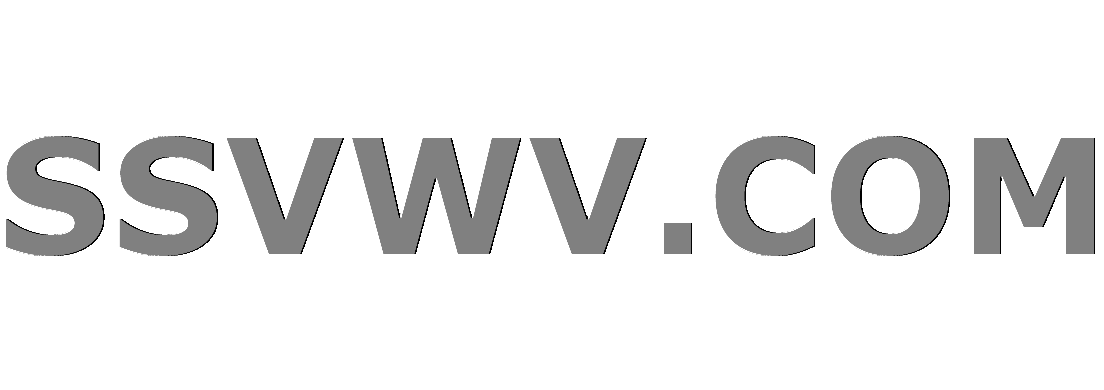
Multi tool use
I am a beginner at C and I was just studying more in a book I picked up. I got to mutex locks and conditional variables and stuff like that. I saw an exercise in the book that said to switch a program that had mutex locks and conditional to a program that used semaphores instead to achieve the same results. I'm currently stuck because when I run the code, I believe I have the situation of being "deadlocked" and nothing happens after. I'm not sure what's wrong but any help would be appreciated. This is what I have now.
int main(){
pthread_t pid;
pthread_t cid;
sem_init(&empty, 1, BUFSIZE);
sem_init(&full, 1, BUFSIZE);
randData = (unsigned int) time(NULL);
printf("Creating threadsnn");
pthread_create(&pid, NULL, produce, ids);
pthread_create(&cid, NULL, consume, ids);
pthread_join(pid, NULL);
pthread_join(cid, NULL);
}
c pthreads conditional mutex semaphore
add a comment |
I am a beginner at C and I was just studying more in a book I picked up. I got to mutex locks and conditional variables and stuff like that. I saw an exercise in the book that said to switch a program that had mutex locks and conditional to a program that used semaphores instead to achieve the same results. I'm currently stuck because when I run the code, I believe I have the situation of being "deadlocked" and nothing happens after. I'm not sure what's wrong but any help would be appreciated. This is what I have now.
int main(){
pthread_t pid;
pthread_t cid;
sem_init(&empty, 1, BUFSIZE);
sem_init(&full, 1, BUFSIZE);
randData = (unsigned int) time(NULL);
printf("Creating threadsnn");
pthread_create(&pid, NULL, produce, ids);
pthread_create(&cid, NULL, consume, ids);
pthread_join(pid, NULL);
pthread_join(cid, NULL);
}
c pthreads conditional mutex semaphore
Just to clarify -- should there be multiple producers and consumers in the semaphore version? It looks like you had several of each in your condition variable output, but I only see you creating one of each in the new code.
– Paul
Nov 25 '18 at 8:33
There are 2 in produce. 1 for pthread_cond_wait and pthread_cond_signal There are 2 in consume, same as produce sorry about that. where should I be creating more?
– James Williams
Nov 25 '18 at 8:37
add a comment |
I am a beginner at C and I was just studying more in a book I picked up. I got to mutex locks and conditional variables and stuff like that. I saw an exercise in the book that said to switch a program that had mutex locks and conditional to a program that used semaphores instead to achieve the same results. I'm currently stuck because when I run the code, I believe I have the situation of being "deadlocked" and nothing happens after. I'm not sure what's wrong but any help would be appreciated. This is what I have now.
int main(){
pthread_t pid;
pthread_t cid;
sem_init(&empty, 1, BUFSIZE);
sem_init(&full, 1, BUFSIZE);
randData = (unsigned int) time(NULL);
printf("Creating threadsnn");
pthread_create(&pid, NULL, produce, ids);
pthread_create(&cid, NULL, consume, ids);
pthread_join(pid, NULL);
pthread_join(cid, NULL);
}
c pthreads conditional mutex semaphore
I am a beginner at C and I was just studying more in a book I picked up. I got to mutex locks and conditional variables and stuff like that. I saw an exercise in the book that said to switch a program that had mutex locks and conditional to a program that used semaphores instead to achieve the same results. I'm currently stuck because when I run the code, I believe I have the situation of being "deadlocked" and nothing happens after. I'm not sure what's wrong but any help would be appreciated. This is what I have now.
int main(){
pthread_t pid;
pthread_t cid;
sem_init(&empty, 1, BUFSIZE);
sem_init(&full, 1, BUFSIZE);
randData = (unsigned int) time(NULL);
printf("Creating threadsnn");
pthread_create(&pid, NULL, produce, ids);
pthread_create(&cid, NULL, consume, ids);
pthread_join(pid, NULL);
pthread_join(cid, NULL);
}
c pthreads conditional mutex semaphore
c pthreads conditional mutex semaphore
edited Nov 27 '18 at 10:09
James Williams
asked Nov 25 '18 at 8:16
James WilliamsJames Williams
66
66
Just to clarify -- should there be multiple producers and consumers in the semaphore version? It looks like you had several of each in your condition variable output, but I only see you creating one of each in the new code.
– Paul
Nov 25 '18 at 8:33
There are 2 in produce. 1 for pthread_cond_wait and pthread_cond_signal There are 2 in consume, same as produce sorry about that. where should I be creating more?
– James Williams
Nov 25 '18 at 8:37
add a comment |
Just to clarify -- should there be multiple producers and consumers in the semaphore version? It looks like you had several of each in your condition variable output, but I only see you creating one of each in the new code.
– Paul
Nov 25 '18 at 8:33
There are 2 in produce. 1 for pthread_cond_wait and pthread_cond_signal There are 2 in consume, same as produce sorry about that. where should I be creating more?
– James Williams
Nov 25 '18 at 8:37
Just to clarify -- should there be multiple producers and consumers in the semaphore version? It looks like you had several of each in your condition variable output, but I only see you creating one of each in the new code.
– Paul
Nov 25 '18 at 8:33
Just to clarify -- should there be multiple producers and consumers in the semaphore version? It looks like you had several of each in your condition variable output, but I only see you creating one of each in the new code.
– Paul
Nov 25 '18 at 8:33
There are 2 in produce. 1 for pthread_cond_wait and pthread_cond_signal There are 2 in consume, same as produce sorry about that. where should I be creating more?
– James Williams
Nov 25 '18 at 8:37
There are 2 in produce. 1 for pthread_cond_wait and pthread_cond_signal There are 2 in consume, same as produce sorry about that. where should I be creating more?
– James Williams
Nov 25 '18 at 8:37
add a comment |
2 Answers
2
active
oldest
votes
In code for producer, below, mutex_wait() is for same "mutex" twice which can lock while post() is only once in consumer.
sem_wait(&empty);
sem_wait(&mutex); <---
while ( isFull() ){
printf("%*sProducer %d waitsn", id*5, "", id);
}
enter(value);
printf("%*sProducer %d stores %d ", id*5, "", id, value);
print();
printf("n");
sem_wait(&mutex); <---
sem_post(&full);
Few ideas to get it good in shape is When there is wait for empty or full, just add one check rather than loop and do mutex wait() in producer and from consumer post() it when it is empty or not full.
Can you give me an example of what you mean? Thank you.
– James Williams
Nov 25 '18 at 9:50
add a comment |
It looks like your solution is pretty close, but you still have some of the condition/wait stuff leftover that you shouldn't need. For example, once a thread take the appropriate semaphore, it knows it can proceed.
Check me on this code -- there may be some issues, but here's what I'd recommend (it's pseudo C)
void* produce(void* arg)
{
// Wait for any item to be present in queue
sem_wait(&isNotFullSem);
// Take "mutex" to protect buffer
sem_wait(&mutex);
// Enqueue new value
enter(value);
// Release mutex
sem_post(&mutex);
// Signal that buffer is not empty
// (probably not the best name, but similar to condition)
sem_post(&isNotEmptySem);
}
void* consume(void* arg)
{
// Wait for empty space to be present in queue
sem_wait(&isNotEmptySem);
// Take "mutex" to protect buffer
sem_wait(&mutex);
// Enqueue new value
value = leave();
// Release mutex
sem_post(&mutex);
// Signal that buffer is not full
sem_post(&isNotFullSem);
}
I appreciate the feedback but I keep on getting a deadlock for some reason
– James Williams
Nov 25 '18 at 9:50
@JamesWilliams Hmm, I can't see how the code I have above would result in a deadlock. Can you post your updates to your question so I can take a look? Does the deadlock occur only when the program should terminate? You need some handling for that case (i.e. set exit = 1 and post isNotFull and isNotEmpty to make threads return).
– Paul
Nov 25 '18 at 9:56
put my update using your psuedocode as an answer, thanks
– James Williams
Nov 25 '18 at 13:11
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53465763%2fusing-an-all-semaphore-program-instead-of-mutex-and-cond-t%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
In code for producer, below, mutex_wait() is for same "mutex" twice which can lock while post() is only once in consumer.
sem_wait(&empty);
sem_wait(&mutex); <---
while ( isFull() ){
printf("%*sProducer %d waitsn", id*5, "", id);
}
enter(value);
printf("%*sProducer %d stores %d ", id*5, "", id, value);
print();
printf("n");
sem_wait(&mutex); <---
sem_post(&full);
Few ideas to get it good in shape is When there is wait for empty or full, just add one check rather than loop and do mutex wait() in producer and from consumer post() it when it is empty or not full.
Can you give me an example of what you mean? Thank you.
– James Williams
Nov 25 '18 at 9:50
add a comment |
In code for producer, below, mutex_wait() is for same "mutex" twice which can lock while post() is only once in consumer.
sem_wait(&empty);
sem_wait(&mutex); <---
while ( isFull() ){
printf("%*sProducer %d waitsn", id*5, "", id);
}
enter(value);
printf("%*sProducer %d stores %d ", id*5, "", id, value);
print();
printf("n");
sem_wait(&mutex); <---
sem_post(&full);
Few ideas to get it good in shape is When there is wait for empty or full, just add one check rather than loop and do mutex wait() in producer and from consumer post() it when it is empty or not full.
Can you give me an example of what you mean? Thank you.
– James Williams
Nov 25 '18 at 9:50
add a comment |
In code for producer, below, mutex_wait() is for same "mutex" twice which can lock while post() is only once in consumer.
sem_wait(&empty);
sem_wait(&mutex); <---
while ( isFull() ){
printf("%*sProducer %d waitsn", id*5, "", id);
}
enter(value);
printf("%*sProducer %d stores %d ", id*5, "", id, value);
print();
printf("n");
sem_wait(&mutex); <---
sem_post(&full);
Few ideas to get it good in shape is When there is wait for empty or full, just add one check rather than loop and do mutex wait() in producer and from consumer post() it when it is empty or not full.
In code for producer, below, mutex_wait() is for same "mutex" twice which can lock while post() is only once in consumer.
sem_wait(&empty);
sem_wait(&mutex); <---
while ( isFull() ){
printf("%*sProducer %d waitsn", id*5, "", id);
}
enter(value);
printf("%*sProducer %d stores %d ", id*5, "", id, value);
print();
printf("n");
sem_wait(&mutex); <---
sem_post(&full);
Few ideas to get it good in shape is When there is wait for empty or full, just add one check rather than loop and do mutex wait() in producer and from consumer post() it when it is empty or not full.
answered Nov 25 '18 at 8:38
anandanand
1377
1377
Can you give me an example of what you mean? Thank you.
– James Williams
Nov 25 '18 at 9:50
add a comment |
Can you give me an example of what you mean? Thank you.
– James Williams
Nov 25 '18 at 9:50
Can you give me an example of what you mean? Thank you.
– James Williams
Nov 25 '18 at 9:50
Can you give me an example of what you mean? Thank you.
– James Williams
Nov 25 '18 at 9:50
add a comment |
It looks like your solution is pretty close, but you still have some of the condition/wait stuff leftover that you shouldn't need. For example, once a thread take the appropriate semaphore, it knows it can proceed.
Check me on this code -- there may be some issues, but here's what I'd recommend (it's pseudo C)
void* produce(void* arg)
{
// Wait for any item to be present in queue
sem_wait(&isNotFullSem);
// Take "mutex" to protect buffer
sem_wait(&mutex);
// Enqueue new value
enter(value);
// Release mutex
sem_post(&mutex);
// Signal that buffer is not empty
// (probably not the best name, but similar to condition)
sem_post(&isNotEmptySem);
}
void* consume(void* arg)
{
// Wait for empty space to be present in queue
sem_wait(&isNotEmptySem);
// Take "mutex" to protect buffer
sem_wait(&mutex);
// Enqueue new value
value = leave();
// Release mutex
sem_post(&mutex);
// Signal that buffer is not full
sem_post(&isNotFullSem);
}
I appreciate the feedback but I keep on getting a deadlock for some reason
– James Williams
Nov 25 '18 at 9:50
@JamesWilliams Hmm, I can't see how the code I have above would result in a deadlock. Can you post your updates to your question so I can take a look? Does the deadlock occur only when the program should terminate? You need some handling for that case (i.e. set exit = 1 and post isNotFull and isNotEmpty to make threads return).
– Paul
Nov 25 '18 at 9:56
put my update using your psuedocode as an answer, thanks
– James Williams
Nov 25 '18 at 13:11
add a comment |
It looks like your solution is pretty close, but you still have some of the condition/wait stuff leftover that you shouldn't need. For example, once a thread take the appropriate semaphore, it knows it can proceed.
Check me on this code -- there may be some issues, but here's what I'd recommend (it's pseudo C)
void* produce(void* arg)
{
// Wait for any item to be present in queue
sem_wait(&isNotFullSem);
// Take "mutex" to protect buffer
sem_wait(&mutex);
// Enqueue new value
enter(value);
// Release mutex
sem_post(&mutex);
// Signal that buffer is not empty
// (probably not the best name, but similar to condition)
sem_post(&isNotEmptySem);
}
void* consume(void* arg)
{
// Wait for empty space to be present in queue
sem_wait(&isNotEmptySem);
// Take "mutex" to protect buffer
sem_wait(&mutex);
// Enqueue new value
value = leave();
// Release mutex
sem_post(&mutex);
// Signal that buffer is not full
sem_post(&isNotFullSem);
}
I appreciate the feedback but I keep on getting a deadlock for some reason
– James Williams
Nov 25 '18 at 9:50
@JamesWilliams Hmm, I can't see how the code I have above would result in a deadlock. Can you post your updates to your question so I can take a look? Does the deadlock occur only when the program should terminate? You need some handling for that case (i.e. set exit = 1 and post isNotFull and isNotEmpty to make threads return).
– Paul
Nov 25 '18 at 9:56
put my update using your psuedocode as an answer, thanks
– James Williams
Nov 25 '18 at 13:11
add a comment |
It looks like your solution is pretty close, but you still have some of the condition/wait stuff leftover that you shouldn't need. For example, once a thread take the appropriate semaphore, it knows it can proceed.
Check me on this code -- there may be some issues, but here's what I'd recommend (it's pseudo C)
void* produce(void* arg)
{
// Wait for any item to be present in queue
sem_wait(&isNotFullSem);
// Take "mutex" to protect buffer
sem_wait(&mutex);
// Enqueue new value
enter(value);
// Release mutex
sem_post(&mutex);
// Signal that buffer is not empty
// (probably not the best name, but similar to condition)
sem_post(&isNotEmptySem);
}
void* consume(void* arg)
{
// Wait for empty space to be present in queue
sem_wait(&isNotEmptySem);
// Take "mutex" to protect buffer
sem_wait(&mutex);
// Enqueue new value
value = leave();
// Release mutex
sem_post(&mutex);
// Signal that buffer is not full
sem_post(&isNotFullSem);
}
It looks like your solution is pretty close, but you still have some of the condition/wait stuff leftover that you shouldn't need. For example, once a thread take the appropriate semaphore, it knows it can proceed.
Check me on this code -- there may be some issues, but here's what I'd recommend (it's pseudo C)
void* produce(void* arg)
{
// Wait for any item to be present in queue
sem_wait(&isNotFullSem);
// Take "mutex" to protect buffer
sem_wait(&mutex);
// Enqueue new value
enter(value);
// Release mutex
sem_post(&mutex);
// Signal that buffer is not empty
// (probably not the best name, but similar to condition)
sem_post(&isNotEmptySem);
}
void* consume(void* arg)
{
// Wait for empty space to be present in queue
sem_wait(&isNotEmptySem);
// Take "mutex" to protect buffer
sem_wait(&mutex);
// Enqueue new value
value = leave();
// Release mutex
sem_post(&mutex);
// Signal that buffer is not full
sem_post(&isNotFullSem);
}
answered Nov 25 '18 at 8:40


PaulPaul
3606
3606
I appreciate the feedback but I keep on getting a deadlock for some reason
– James Williams
Nov 25 '18 at 9:50
@JamesWilliams Hmm, I can't see how the code I have above would result in a deadlock. Can you post your updates to your question so I can take a look? Does the deadlock occur only when the program should terminate? You need some handling for that case (i.e. set exit = 1 and post isNotFull and isNotEmpty to make threads return).
– Paul
Nov 25 '18 at 9:56
put my update using your psuedocode as an answer, thanks
– James Williams
Nov 25 '18 at 13:11
add a comment |
I appreciate the feedback but I keep on getting a deadlock for some reason
– James Williams
Nov 25 '18 at 9:50
@JamesWilliams Hmm, I can't see how the code I have above would result in a deadlock. Can you post your updates to your question so I can take a look? Does the deadlock occur only when the program should terminate? You need some handling for that case (i.e. set exit = 1 and post isNotFull and isNotEmpty to make threads return).
– Paul
Nov 25 '18 at 9:56
put my update using your psuedocode as an answer, thanks
– James Williams
Nov 25 '18 at 13:11
I appreciate the feedback but I keep on getting a deadlock for some reason
– James Williams
Nov 25 '18 at 9:50
I appreciate the feedback but I keep on getting a deadlock for some reason
– James Williams
Nov 25 '18 at 9:50
@JamesWilliams Hmm, I can't see how the code I have above would result in a deadlock. Can you post your updates to your question so I can take a look? Does the deadlock occur only when the program should terminate? You need some handling for that case (i.e. set exit = 1 and post isNotFull and isNotEmpty to make threads return).
– Paul
Nov 25 '18 at 9:56
@JamesWilliams Hmm, I can't see how the code I have above would result in a deadlock. Can you post your updates to your question so I can take a look? Does the deadlock occur only when the program should terminate? You need some handling for that case (i.e. set exit = 1 and post isNotFull and isNotEmpty to make threads return).
– Paul
Nov 25 '18 at 9:56
put my update using your psuedocode as an answer, thanks
– James Williams
Nov 25 '18 at 13:11
put my update using your psuedocode as an answer, thanks
– James Williams
Nov 25 '18 at 13:11
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53465763%2fusing-an-all-semaphore-program-instead-of-mutex-and-cond-t%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Pf64Ll03 PlLpPdHq,hTe5T2 rA4L7xnAkl uCBVlhiGkix KzwiWcpN0WsIbZ L8CFU e1GOq mV4W1
Just to clarify -- should there be multiple producers and consumers in the semaphore version? It looks like you had several of each in your condition variable output, but I only see you creating one of each in the new code.
– Paul
Nov 25 '18 at 8:33
There are 2 in produce. 1 for pthread_cond_wait and pthread_cond_signal There are 2 in consume, same as produce sorry about that. where should I be creating more?
– James Williams
Nov 25 '18 at 8:37