Unity 2D rotate the AI enemy to look at player
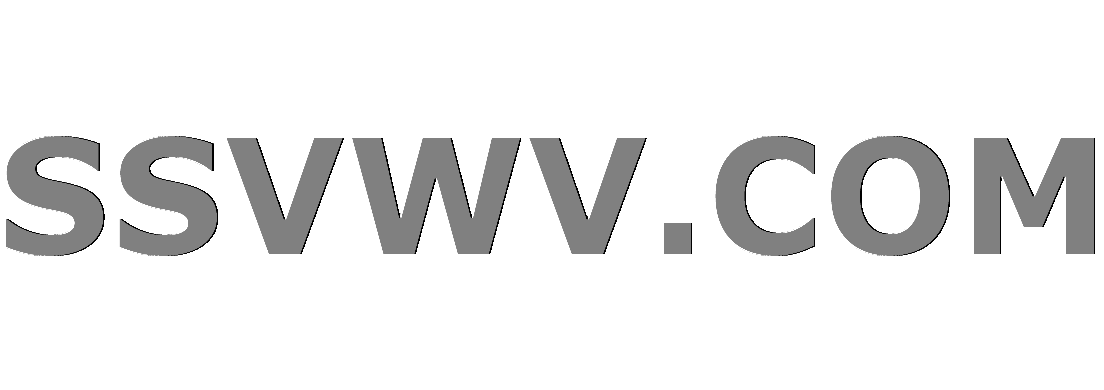
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I'm making a 2D game with two sides, left and right, so the player can only move to the left or right or jump. I made an AI as the enemy. The AI is working fine. He can go to the player to kill him.
The problem is the enemy can't rotate or face the player when he goes to kill him. I want the AI to look at the player. When the player is to the left of the enemy, the enemy should rotate to the left to look at the player. I searched many websites and I didn't get any correct solution. This is my enemy script:
public class enemy : MonoBehaviour
{
public float speed;
private Animator animvar;
private Transform target;
public GameObject effect;
public float distance;
private bool movingRight = true;
public Transform groundedDetection;
public float moveInput;
public bool facingRight = true;
void Start()
{
animvar = GetComponent<Animator>();
target = GameObject.FindGameObjectWithTag("Player").transform;
}
// Update is called once per frame
void Update()
{
if (Vector3.Distance(target.position, transform.position) < 20)
{
transform.position = Vector2.MoveTowards(transform.position, target.position, speed * Time.deltaTime);
}
else
{
transform.Translate(Vector2.right * speed * Time.deltaTime);
}
RaycastHit2D groundInfo = Physics2D.Raycast(groundedDetection.position, Vector2.down, distance);
}
void OnCollisionEnter2D(Collision2D col)
{
if (col.gameObject.tag.Equals("danger"))
{
Instantiate(effect, transform.position, Quaternion.identity);
Destroy(gameObject);
}
if (col.gameObject.tag.Equals("Player"))
{
Instantiate(effect, transform.position, Quaternion.identity);
Destroy(gameObject);
}
}
}
The game is 2D, left, right, with jump using Unity3D.
c# unity3d 2d
add a comment |
I'm making a 2D game with two sides, left and right, so the player can only move to the left or right or jump. I made an AI as the enemy. The AI is working fine. He can go to the player to kill him.
The problem is the enemy can't rotate or face the player when he goes to kill him. I want the AI to look at the player. When the player is to the left of the enemy, the enemy should rotate to the left to look at the player. I searched many websites and I didn't get any correct solution. This is my enemy script:
public class enemy : MonoBehaviour
{
public float speed;
private Animator animvar;
private Transform target;
public GameObject effect;
public float distance;
private bool movingRight = true;
public Transform groundedDetection;
public float moveInput;
public bool facingRight = true;
void Start()
{
animvar = GetComponent<Animator>();
target = GameObject.FindGameObjectWithTag("Player").transform;
}
// Update is called once per frame
void Update()
{
if (Vector3.Distance(target.position, transform.position) < 20)
{
transform.position = Vector2.MoveTowards(transform.position, target.position, speed * Time.deltaTime);
}
else
{
transform.Translate(Vector2.right * speed * Time.deltaTime);
}
RaycastHit2D groundInfo = Physics2D.Raycast(groundedDetection.position, Vector2.down, distance);
}
void OnCollisionEnter2D(Collision2D col)
{
if (col.gameObject.tag.Equals("danger"))
{
Instantiate(effect, transform.position, Quaternion.identity);
Destroy(gameObject);
}
if (col.gameObject.tag.Equals("Player"))
{
Instantiate(effect, transform.position, Quaternion.identity);
Destroy(gameObject);
}
}
}
The game is 2D, left, right, with jump using Unity3D.
c# unity3d 2d
a clear and defined question would help!
– Falco Alexander
Nov 26 '18 at 22:31
add a comment |
I'm making a 2D game with two sides, left and right, so the player can only move to the left or right or jump. I made an AI as the enemy. The AI is working fine. He can go to the player to kill him.
The problem is the enemy can't rotate or face the player when he goes to kill him. I want the AI to look at the player. When the player is to the left of the enemy, the enemy should rotate to the left to look at the player. I searched many websites and I didn't get any correct solution. This is my enemy script:
public class enemy : MonoBehaviour
{
public float speed;
private Animator animvar;
private Transform target;
public GameObject effect;
public float distance;
private bool movingRight = true;
public Transform groundedDetection;
public float moveInput;
public bool facingRight = true;
void Start()
{
animvar = GetComponent<Animator>();
target = GameObject.FindGameObjectWithTag("Player").transform;
}
// Update is called once per frame
void Update()
{
if (Vector3.Distance(target.position, transform.position) < 20)
{
transform.position = Vector2.MoveTowards(transform.position, target.position, speed * Time.deltaTime);
}
else
{
transform.Translate(Vector2.right * speed * Time.deltaTime);
}
RaycastHit2D groundInfo = Physics2D.Raycast(groundedDetection.position, Vector2.down, distance);
}
void OnCollisionEnter2D(Collision2D col)
{
if (col.gameObject.tag.Equals("danger"))
{
Instantiate(effect, transform.position, Quaternion.identity);
Destroy(gameObject);
}
if (col.gameObject.tag.Equals("Player"))
{
Instantiate(effect, transform.position, Quaternion.identity);
Destroy(gameObject);
}
}
}
The game is 2D, left, right, with jump using Unity3D.
c# unity3d 2d
I'm making a 2D game with two sides, left and right, so the player can only move to the left or right or jump. I made an AI as the enemy. The AI is working fine. He can go to the player to kill him.
The problem is the enemy can't rotate or face the player when he goes to kill him. I want the AI to look at the player. When the player is to the left of the enemy, the enemy should rotate to the left to look at the player. I searched many websites and I didn't get any correct solution. This is my enemy script:
public class enemy : MonoBehaviour
{
public float speed;
private Animator animvar;
private Transform target;
public GameObject effect;
public float distance;
private bool movingRight = true;
public Transform groundedDetection;
public float moveInput;
public bool facingRight = true;
void Start()
{
animvar = GetComponent<Animator>();
target = GameObject.FindGameObjectWithTag("Player").transform;
}
// Update is called once per frame
void Update()
{
if (Vector3.Distance(target.position, transform.position) < 20)
{
transform.position = Vector2.MoveTowards(transform.position, target.position, speed * Time.deltaTime);
}
else
{
transform.Translate(Vector2.right * speed * Time.deltaTime);
}
RaycastHit2D groundInfo = Physics2D.Raycast(groundedDetection.position, Vector2.down, distance);
}
void OnCollisionEnter2D(Collision2D col)
{
if (col.gameObject.tag.Equals("danger"))
{
Instantiate(effect, transform.position, Quaternion.identity);
Destroy(gameObject);
}
if (col.gameObject.tag.Equals("Player"))
{
Instantiate(effect, transform.position, Quaternion.identity);
Destroy(gameObject);
}
}
}
The game is 2D, left, right, with jump using Unity3D.
c# unity3d 2d
c# unity3d 2d
edited Nov 27 '18 at 0:49


Mihai Chelaru
2,485101424
2,485101424
asked Nov 26 '18 at 20:25


SHOW CASESHOW CASE
144
144
a clear and defined question would help!
– Falco Alexander
Nov 26 '18 at 22:31
add a comment |
a clear and defined question would help!
– Falco Alexander
Nov 26 '18 at 22:31
a clear and defined question would help!
– Falco Alexander
Nov 26 '18 at 22:31
a clear and defined question would help!
– Falco Alexander
Nov 26 '18 at 22:31
add a comment |
1 Answer
1
active
oldest
votes
You need a function that would flip your sprite, you can do that by changing the scale of the transform, and keep a boolean to check where it's facing
bool facingRight;
, so something like this
void Flip(){
Vector3 scale = transform.localScale;
scale.x *= -1;
transform.localScale = scale;
facingRight = !facingRight;
}
and in your Update
check if it needs to be flipped or not
if (Vector3.Distance(target.position,transform.position)<20)
{
transform.position=Vector2.MoveTowards(transform.position, target.position,speed*Time.deltaTime);
if(target.position.x > transform.position.x && !facingRight) //if the target is to the right of enemy and the enemy is not facing right
Flip();
if(target.position.x < transform.position.x && facingRight)
Flip();
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53488507%2funity-2d-rotate-the-ai-enemy-to-look-at-player%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You need a function that would flip your sprite, you can do that by changing the scale of the transform, and keep a boolean to check where it's facing
bool facingRight;
, so something like this
void Flip(){
Vector3 scale = transform.localScale;
scale.x *= -1;
transform.localScale = scale;
facingRight = !facingRight;
}
and in your Update
check if it needs to be flipped or not
if (Vector3.Distance(target.position,transform.position)<20)
{
transform.position=Vector2.MoveTowards(transform.position, target.position,speed*Time.deltaTime);
if(target.position.x > transform.position.x && !facingRight) //if the target is to the right of enemy and the enemy is not facing right
Flip();
if(target.position.x < transform.position.x && facingRight)
Flip();
}
add a comment |
You need a function that would flip your sprite, you can do that by changing the scale of the transform, and keep a boolean to check where it's facing
bool facingRight;
, so something like this
void Flip(){
Vector3 scale = transform.localScale;
scale.x *= -1;
transform.localScale = scale;
facingRight = !facingRight;
}
and in your Update
check if it needs to be flipped or not
if (Vector3.Distance(target.position,transform.position)<20)
{
transform.position=Vector2.MoveTowards(transform.position, target.position,speed*Time.deltaTime);
if(target.position.x > transform.position.x && !facingRight) //if the target is to the right of enemy and the enemy is not facing right
Flip();
if(target.position.x < transform.position.x && facingRight)
Flip();
}
add a comment |
You need a function that would flip your sprite, you can do that by changing the scale of the transform, and keep a boolean to check where it's facing
bool facingRight;
, so something like this
void Flip(){
Vector3 scale = transform.localScale;
scale.x *= -1;
transform.localScale = scale;
facingRight = !facingRight;
}
and in your Update
check if it needs to be flipped or not
if (Vector3.Distance(target.position,transform.position)<20)
{
transform.position=Vector2.MoveTowards(transform.position, target.position,speed*Time.deltaTime);
if(target.position.x > transform.position.x && !facingRight) //if the target is to the right of enemy and the enemy is not facing right
Flip();
if(target.position.x < transform.position.x && facingRight)
Flip();
}
You need a function that would flip your sprite, you can do that by changing the scale of the transform, and keep a boolean to check where it's facing
bool facingRight;
, so something like this
void Flip(){
Vector3 scale = transform.localScale;
scale.x *= -1;
transform.localScale = scale;
facingRight = !facingRight;
}
and in your Update
check if it needs to be flipped or not
if (Vector3.Distance(target.position,transform.position)<20)
{
transform.position=Vector2.MoveTowards(transform.position, target.position,speed*Time.deltaTime);
if(target.position.x > transform.position.x && !facingRight) //if the target is to the right of enemy and the enemy is not facing right
Flip();
if(target.position.x < transform.position.x && facingRight)
Flip();
}
answered Nov 27 '18 at 0:48
JimmarJimmar
1,63711530
1,63711530
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53488507%2funity-2d-rotate-the-ai-enemy-to-look-at-player%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
eqN GWu,Xp,joU,7ddn8Y7KIMRmH ojquMwoHfc J dYkEwAh9QP5Z835BwZoBq,Ykfidtaw,kC 9c
a clear and defined question would help!
– Falco Alexander
Nov 26 '18 at 22:31