Sound programming in Linux. Capturing raw sound data
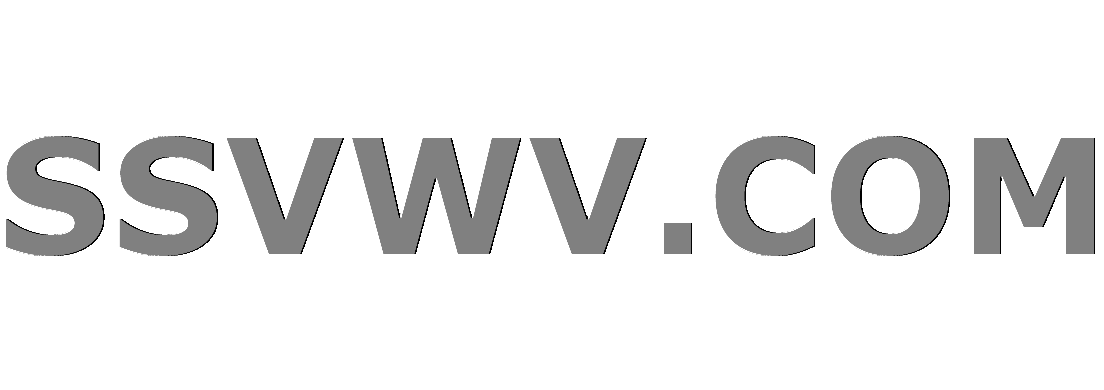
Multi tool use
I'm reading the ALSA tutorial and came up with a question about capturing PCM data. I wrote the following example (similar to one in the tutorial). Here is it (error checking and printing messages omitted):
#include <alsa/asoundlib.h>
int rate = 44100;
int periods = 2;
snd_pcm_uframes_t period_size = 8192;
int exact_rate;
int direction;
int main(int argc, char ** argv){
snd_pcm_t *pcm_handle;
snd_pcm_stream_t stream = SND_PCM_STREAM_CAPTURE; // <--- for capturin PCM data
snd_pcm_hw_params_t *hwparams;
snd_pcm_hw_params_alloca(&hwparams);
snd_pcm_open(&pcm_handle, pcm_name, stream, 0)
snd_pcm_hw_params_any(pcm_handle, hwparams)
snd_pcm_hw_params_set_access(pcm_handle, hwparams, SND_PCM_ACCESS_RW_INTERLEAVED);
snd_pcm_hw_params_set_format(pcm_handle, hwparams, SND_PCM_FORMAT_S16_LE);
snd_pcm_hw_params_set_rate_near(pcm_handle, hwparams, &exact_rate, &direction);
snd_pcm_hw_params_set_channels(pcm_handle, hwparams, 2);
snd_pcm_hw_params_set_periods(pcm_handle, hwparams, periods, direction);
snd_pcm_hw_params_set_buffer_size(pcm_handle, hwparams, (period_size * periods) >> 2);
snd_pcm_hw_params(pcm_handle, hwparams);
//reading PCM data, microphone is not plugged
char *buffer = calloc(sizeof(char), period_size * periods);
int pcm_read_return = 0;
pcm_read_return = snd_pcm_readi(pcm_handle, buffer, period_size >> 2);
printf("Read %d framesn", pcm_read_return); //always 2048
printf("Buffer: %sn", buffer); //Some data,not all bytes are 0
free(buffer);
return 0;
}
I don't really understand the behavior. The number of frames read are 2048
all the time. And they are always 0 Sometimes it produces some non-zero bytes. I expected no frames are read since microphone is unplugged and no PCM data should be available.
c linux alsa
add a comment |
I'm reading the ALSA tutorial and came up with a question about capturing PCM data. I wrote the following example (similar to one in the tutorial). Here is it (error checking and printing messages omitted):
#include <alsa/asoundlib.h>
int rate = 44100;
int periods = 2;
snd_pcm_uframes_t period_size = 8192;
int exact_rate;
int direction;
int main(int argc, char ** argv){
snd_pcm_t *pcm_handle;
snd_pcm_stream_t stream = SND_PCM_STREAM_CAPTURE; // <--- for capturin PCM data
snd_pcm_hw_params_t *hwparams;
snd_pcm_hw_params_alloca(&hwparams);
snd_pcm_open(&pcm_handle, pcm_name, stream, 0)
snd_pcm_hw_params_any(pcm_handle, hwparams)
snd_pcm_hw_params_set_access(pcm_handle, hwparams, SND_PCM_ACCESS_RW_INTERLEAVED);
snd_pcm_hw_params_set_format(pcm_handle, hwparams, SND_PCM_FORMAT_S16_LE);
snd_pcm_hw_params_set_rate_near(pcm_handle, hwparams, &exact_rate, &direction);
snd_pcm_hw_params_set_channels(pcm_handle, hwparams, 2);
snd_pcm_hw_params_set_periods(pcm_handle, hwparams, periods, direction);
snd_pcm_hw_params_set_buffer_size(pcm_handle, hwparams, (period_size * periods) >> 2);
snd_pcm_hw_params(pcm_handle, hwparams);
//reading PCM data, microphone is not plugged
char *buffer = calloc(sizeof(char), period_size * periods);
int pcm_read_return = 0;
pcm_read_return = snd_pcm_readi(pcm_handle, buffer, period_size >> 2);
printf("Read %d framesn", pcm_read_return); //always 2048
printf("Buffer: %sn", buffer); //Some data,not all bytes are 0
free(buffer);
return 0;
}
I don't really understand the behavior. The number of frames read are 2048
all the time. And they are always 0 Sometimes it produces some non-zero bytes. I expected no frames are read since microphone is unplugged and no PCM data should be available.
c linux alsa
A sound card may or may not know whether a microphone is plugged in. Some have hardware to determine this, some don't. If it does, it will probably disable the recording device when the microphonne is not plugged in, so the open call will fail. It doesn't make sense to enable the device and then not produce any frames.
– n.m.
Nov 21 at 6:38
@n.m. It doesn't make sense to enable the device and then not produce any frames. Why doesn't? So it produces some garbage frames?
– Some Name
Nov 21 at 6:49
1
It produces silent frames (or small noises because of interference) because it cannot tell silence from an unplugged mic. If it could, it would tell you that the mic is unplugged by refusing to open it.
– n.m.
Nov 21 at 7:09
@n.m. Thanks, understood!
– Some Name
Nov 21 at 7:13
add a comment |
I'm reading the ALSA tutorial and came up with a question about capturing PCM data. I wrote the following example (similar to one in the tutorial). Here is it (error checking and printing messages omitted):
#include <alsa/asoundlib.h>
int rate = 44100;
int periods = 2;
snd_pcm_uframes_t period_size = 8192;
int exact_rate;
int direction;
int main(int argc, char ** argv){
snd_pcm_t *pcm_handle;
snd_pcm_stream_t stream = SND_PCM_STREAM_CAPTURE; // <--- for capturin PCM data
snd_pcm_hw_params_t *hwparams;
snd_pcm_hw_params_alloca(&hwparams);
snd_pcm_open(&pcm_handle, pcm_name, stream, 0)
snd_pcm_hw_params_any(pcm_handle, hwparams)
snd_pcm_hw_params_set_access(pcm_handle, hwparams, SND_PCM_ACCESS_RW_INTERLEAVED);
snd_pcm_hw_params_set_format(pcm_handle, hwparams, SND_PCM_FORMAT_S16_LE);
snd_pcm_hw_params_set_rate_near(pcm_handle, hwparams, &exact_rate, &direction);
snd_pcm_hw_params_set_channels(pcm_handle, hwparams, 2);
snd_pcm_hw_params_set_periods(pcm_handle, hwparams, periods, direction);
snd_pcm_hw_params_set_buffer_size(pcm_handle, hwparams, (period_size * periods) >> 2);
snd_pcm_hw_params(pcm_handle, hwparams);
//reading PCM data, microphone is not plugged
char *buffer = calloc(sizeof(char), period_size * periods);
int pcm_read_return = 0;
pcm_read_return = snd_pcm_readi(pcm_handle, buffer, period_size >> 2);
printf("Read %d framesn", pcm_read_return); //always 2048
printf("Buffer: %sn", buffer); //Some data,not all bytes are 0
free(buffer);
return 0;
}
I don't really understand the behavior. The number of frames read are 2048
all the time. And they are always 0 Sometimes it produces some non-zero bytes. I expected no frames are read since microphone is unplugged and no PCM data should be available.
c linux alsa
I'm reading the ALSA tutorial and came up with a question about capturing PCM data. I wrote the following example (similar to one in the tutorial). Here is it (error checking and printing messages omitted):
#include <alsa/asoundlib.h>
int rate = 44100;
int periods = 2;
snd_pcm_uframes_t period_size = 8192;
int exact_rate;
int direction;
int main(int argc, char ** argv){
snd_pcm_t *pcm_handle;
snd_pcm_stream_t stream = SND_PCM_STREAM_CAPTURE; // <--- for capturin PCM data
snd_pcm_hw_params_t *hwparams;
snd_pcm_hw_params_alloca(&hwparams);
snd_pcm_open(&pcm_handle, pcm_name, stream, 0)
snd_pcm_hw_params_any(pcm_handle, hwparams)
snd_pcm_hw_params_set_access(pcm_handle, hwparams, SND_PCM_ACCESS_RW_INTERLEAVED);
snd_pcm_hw_params_set_format(pcm_handle, hwparams, SND_PCM_FORMAT_S16_LE);
snd_pcm_hw_params_set_rate_near(pcm_handle, hwparams, &exact_rate, &direction);
snd_pcm_hw_params_set_channels(pcm_handle, hwparams, 2);
snd_pcm_hw_params_set_periods(pcm_handle, hwparams, periods, direction);
snd_pcm_hw_params_set_buffer_size(pcm_handle, hwparams, (period_size * periods) >> 2);
snd_pcm_hw_params(pcm_handle, hwparams);
//reading PCM data, microphone is not plugged
char *buffer = calloc(sizeof(char), period_size * periods);
int pcm_read_return = 0;
pcm_read_return = snd_pcm_readi(pcm_handle, buffer, period_size >> 2);
printf("Read %d framesn", pcm_read_return); //always 2048
printf("Buffer: %sn", buffer); //Some data,not all bytes are 0
free(buffer);
return 0;
}
I don't really understand the behavior. The number of frames read are 2048
all the time. And they are always 0 Sometimes it produces some non-zero bytes. I expected no frames are read since microphone is unplugged and no PCM data should be available.
c linux alsa
c linux alsa
asked Nov 21 at 5:58
Some Name
783214
783214
A sound card may or may not know whether a microphone is plugged in. Some have hardware to determine this, some don't. If it does, it will probably disable the recording device when the microphonne is not plugged in, so the open call will fail. It doesn't make sense to enable the device and then not produce any frames.
– n.m.
Nov 21 at 6:38
@n.m. It doesn't make sense to enable the device and then not produce any frames. Why doesn't? So it produces some garbage frames?
– Some Name
Nov 21 at 6:49
1
It produces silent frames (or small noises because of interference) because it cannot tell silence from an unplugged mic. If it could, it would tell you that the mic is unplugged by refusing to open it.
– n.m.
Nov 21 at 7:09
@n.m. Thanks, understood!
– Some Name
Nov 21 at 7:13
add a comment |
A sound card may or may not know whether a microphone is plugged in. Some have hardware to determine this, some don't. If it does, it will probably disable the recording device when the microphonne is not plugged in, so the open call will fail. It doesn't make sense to enable the device and then not produce any frames.
– n.m.
Nov 21 at 6:38
@n.m. It doesn't make sense to enable the device and then not produce any frames. Why doesn't? So it produces some garbage frames?
– Some Name
Nov 21 at 6:49
1
It produces silent frames (or small noises because of interference) because it cannot tell silence from an unplugged mic. If it could, it would tell you that the mic is unplugged by refusing to open it.
– n.m.
Nov 21 at 7:09
@n.m. Thanks, understood!
– Some Name
Nov 21 at 7:13
A sound card may or may not know whether a microphone is plugged in. Some have hardware to determine this, some don't. If it does, it will probably disable the recording device when the microphonne is not plugged in, so the open call will fail. It doesn't make sense to enable the device and then not produce any frames.
– n.m.
Nov 21 at 6:38
A sound card may or may not know whether a microphone is plugged in. Some have hardware to determine this, some don't. If it does, it will probably disable the recording device when the microphonne is not plugged in, so the open call will fail. It doesn't make sense to enable the device and then not produce any frames.
– n.m.
Nov 21 at 6:38
@n.m. It doesn't make sense to enable the device and then not produce any frames. Why doesn't? So it produces some garbage frames?
– Some Name
Nov 21 at 6:49
@n.m. It doesn't make sense to enable the device and then not produce any frames. Why doesn't? So it produces some garbage frames?
– Some Name
Nov 21 at 6:49
1
1
It produces silent frames (or small noises because of interference) because it cannot tell silence from an unplugged mic. If it could, it would tell you that the mic is unplugged by refusing to open it.
– n.m.
Nov 21 at 7:09
It produces silent frames (or small noises because of interference) because it cannot tell silence from an unplugged mic. If it could, it would tell you that the mic is unplugged by refusing to open it.
– n.m.
Nov 21 at 7:09
@n.m. Thanks, understood!
– Some Name
Nov 21 at 7:13
@n.m. Thanks, understood!
– Some Name
Nov 21 at 7:13
add a comment |
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53406050%2fsound-programming-in-linux-capturing-raw-sound-data%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53406050%2fsound-programming-in-linux-capturing-raw-sound-data%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
U7VJKze0O2yH8ofI,F
A sound card may or may not know whether a microphone is plugged in. Some have hardware to determine this, some don't. If it does, it will probably disable the recording device when the microphonne is not plugged in, so the open call will fail. It doesn't make sense to enable the device and then not produce any frames.
– n.m.
Nov 21 at 6:38
@n.m. It doesn't make sense to enable the device and then not produce any frames. Why doesn't? So it produces some garbage frames?
– Some Name
Nov 21 at 6:49
1
It produces silent frames (or small noises because of interference) because it cannot tell silence from an unplugged mic. If it could, it would tell you that the mic is unplugged by refusing to open it.
– n.m.
Nov 21 at 7:09
@n.m. Thanks, understood!
– Some Name
Nov 21 at 7:13