Transform Rows data to column from a grouped data in LINQ
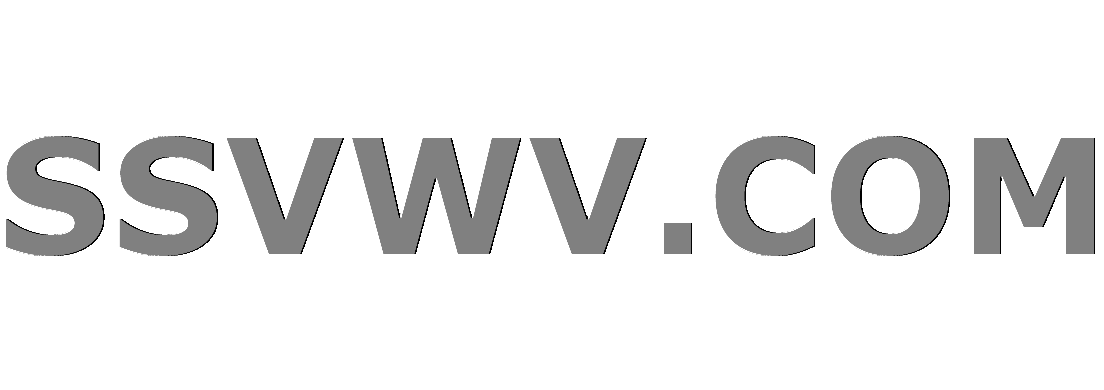
Multi tool use
I am reading a csv file.
The data is not sorted in any order. I have read the file using the below code
List<LatencyData> latencys = new List<LatencyData>();
using (var reader = new StreamReader(filePath))
{
LatencyData latencyData = null;
while (!reader.EndOfStream)
{
var line = reader.ReadLine();
var values = line.Split(';');
var row = values[0].Split(',');
latencyData = new LatencyData
{
TimeStamp = (row[0] + "000"),
Prefix = row[1],
Uplink = row[2],
Latency = row[3]
};
latencys.Add(latencyData);
}
}
The Next step was to group on the basis of Time stamp.
var grouped = latencys.GroupBy(item => item.TimeStamp);
which looks like this after grouping.
Now I want to transform the data into this format
timeStamp Latency0 Latency1 Latency2 Latency3 Latency4
1541030400 198 267.67 263 null 198
for that i used this part.
var transformed = grouped.Select(g => new
{
Hubs = g.Select(hub => new
{
stamp = g.Key,
Latency0 = g.Where(item => item.Uplink == "0").Select(data => data.Latency),
Latency1 = g.Where(item => item.Uplink == "1").Select(data => data.Latency),
Latency2 = g.Where(item => item.Uplink == "2").Select(data => data.Latency),
Latency3 = g.Where(item => item.Uplink == "3").Select(data => data.Latency),
Latency4 = g.Where(item => item.Uplink == "4").Select(data => data.Latency),
})
});
The Result is not correct and there is many rows for the same values are returned. It should have only one row per key
timeStamp Latency0 Latency1 Latency2 Latency3 Latency4
1541030400 198 267.67 263 null 198
What am I doing wrong here?
c# linq
add a comment |
I am reading a csv file.
The data is not sorted in any order. I have read the file using the below code
List<LatencyData> latencys = new List<LatencyData>();
using (var reader = new StreamReader(filePath))
{
LatencyData latencyData = null;
while (!reader.EndOfStream)
{
var line = reader.ReadLine();
var values = line.Split(';');
var row = values[0].Split(',');
latencyData = new LatencyData
{
TimeStamp = (row[0] + "000"),
Prefix = row[1],
Uplink = row[2],
Latency = row[3]
};
latencys.Add(latencyData);
}
}
The Next step was to group on the basis of Time stamp.
var grouped = latencys.GroupBy(item => item.TimeStamp);
which looks like this after grouping.
Now I want to transform the data into this format
timeStamp Latency0 Latency1 Latency2 Latency3 Latency4
1541030400 198 267.67 263 null 198
for that i used this part.
var transformed = grouped.Select(g => new
{
Hubs = g.Select(hub => new
{
stamp = g.Key,
Latency0 = g.Where(item => item.Uplink == "0").Select(data => data.Latency),
Latency1 = g.Where(item => item.Uplink == "1").Select(data => data.Latency),
Latency2 = g.Where(item => item.Uplink == "2").Select(data => data.Latency),
Latency3 = g.Where(item => item.Uplink == "3").Select(data => data.Latency),
Latency4 = g.Where(item => item.Uplink == "4").Select(data => data.Latency),
})
});
The Result is not correct and there is many rows for the same values are returned. It should have only one row per key
timeStamp Latency0 Latency1 Latency2 Latency3 Latency4
1541030400 198 267.67 263 null 198
What am I doing wrong here?
c# linq
add a comment |
I am reading a csv file.
The data is not sorted in any order. I have read the file using the below code
List<LatencyData> latencys = new List<LatencyData>();
using (var reader = new StreamReader(filePath))
{
LatencyData latencyData = null;
while (!reader.EndOfStream)
{
var line = reader.ReadLine();
var values = line.Split(';');
var row = values[0].Split(',');
latencyData = new LatencyData
{
TimeStamp = (row[0] + "000"),
Prefix = row[1],
Uplink = row[2],
Latency = row[3]
};
latencys.Add(latencyData);
}
}
The Next step was to group on the basis of Time stamp.
var grouped = latencys.GroupBy(item => item.TimeStamp);
which looks like this after grouping.
Now I want to transform the data into this format
timeStamp Latency0 Latency1 Latency2 Latency3 Latency4
1541030400 198 267.67 263 null 198
for that i used this part.
var transformed = grouped.Select(g => new
{
Hubs = g.Select(hub => new
{
stamp = g.Key,
Latency0 = g.Where(item => item.Uplink == "0").Select(data => data.Latency),
Latency1 = g.Where(item => item.Uplink == "1").Select(data => data.Latency),
Latency2 = g.Where(item => item.Uplink == "2").Select(data => data.Latency),
Latency3 = g.Where(item => item.Uplink == "3").Select(data => data.Latency),
Latency4 = g.Where(item => item.Uplink == "4").Select(data => data.Latency),
})
});
The Result is not correct and there is many rows for the same values are returned. It should have only one row per key
timeStamp Latency0 Latency1 Latency2 Latency3 Latency4
1541030400 198 267.67 263 null 198
What am I doing wrong here?
c# linq
I am reading a csv file.
The data is not sorted in any order. I have read the file using the below code
List<LatencyData> latencys = new List<LatencyData>();
using (var reader = new StreamReader(filePath))
{
LatencyData latencyData = null;
while (!reader.EndOfStream)
{
var line = reader.ReadLine();
var values = line.Split(';');
var row = values[0].Split(',');
latencyData = new LatencyData
{
TimeStamp = (row[0] + "000"),
Prefix = row[1],
Uplink = row[2],
Latency = row[3]
};
latencys.Add(latencyData);
}
}
The Next step was to group on the basis of Time stamp.
var grouped = latencys.GroupBy(item => item.TimeStamp);
which looks like this after grouping.
Now I want to transform the data into this format
timeStamp Latency0 Latency1 Latency2 Latency3 Latency4
1541030400 198 267.67 263 null 198
for that i used this part.
var transformed = grouped.Select(g => new
{
Hubs = g.Select(hub => new
{
stamp = g.Key,
Latency0 = g.Where(item => item.Uplink == "0").Select(data => data.Latency),
Latency1 = g.Where(item => item.Uplink == "1").Select(data => data.Latency),
Latency2 = g.Where(item => item.Uplink == "2").Select(data => data.Latency),
Latency3 = g.Where(item => item.Uplink == "3").Select(data => data.Latency),
Latency4 = g.Where(item => item.Uplink == "4").Select(data => data.Latency),
})
});
The Result is not correct and there is many rows for the same values are returned. It should have only one row per key
timeStamp Latency0 Latency1 Latency2 Latency3 Latency4
1541030400 198 267.67 263 null 198
What am I doing wrong here?
c# linq
c# linq
edited Nov 20 at 16:51


Kit
8,67423168
8,67423168
asked Nov 20 at 16:46
ankur
1,980124575
1,980124575
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
You're almost there. However you might want to return a value for each latency instead of list of objects:
var transformed = grouped.Select(g => new
{
Hubs = g.Select(hub => new
{
stamp = g.Key,
Latency0 = g.FirstOrDefault(item => item.Uplink == "0")?.Latency,
Latency1 = g.FirstOrDefault(item => item.Uplink == "1")?.Latency,
Latency2 = g.FirstOrDefault(item => item.Uplink == "2")?.Latency,
Latency3 = g.FirstOrDefault(item => item.Uplink == "3")?.Latency,
Latency4 = g.FirstOrDefault(item => item.Uplink == "4")?.Latency,
})
});
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53397709%2ftransform-rows-data-to-column-from-a-grouped-data-in-linq%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You're almost there. However you might want to return a value for each latency instead of list of objects:
var transformed = grouped.Select(g => new
{
Hubs = g.Select(hub => new
{
stamp = g.Key,
Latency0 = g.FirstOrDefault(item => item.Uplink == "0")?.Latency,
Latency1 = g.FirstOrDefault(item => item.Uplink == "1")?.Latency,
Latency2 = g.FirstOrDefault(item => item.Uplink == "2")?.Latency,
Latency3 = g.FirstOrDefault(item => item.Uplink == "3")?.Latency,
Latency4 = g.FirstOrDefault(item => item.Uplink == "4")?.Latency,
})
});
add a comment |
You're almost there. However you might want to return a value for each latency instead of list of objects:
var transformed = grouped.Select(g => new
{
Hubs = g.Select(hub => new
{
stamp = g.Key,
Latency0 = g.FirstOrDefault(item => item.Uplink == "0")?.Latency,
Latency1 = g.FirstOrDefault(item => item.Uplink == "1")?.Latency,
Latency2 = g.FirstOrDefault(item => item.Uplink == "2")?.Latency,
Latency3 = g.FirstOrDefault(item => item.Uplink == "3")?.Latency,
Latency4 = g.FirstOrDefault(item => item.Uplink == "4")?.Latency,
})
});
add a comment |
You're almost there. However you might want to return a value for each latency instead of list of objects:
var transformed = grouped.Select(g => new
{
Hubs = g.Select(hub => new
{
stamp = g.Key,
Latency0 = g.FirstOrDefault(item => item.Uplink == "0")?.Latency,
Latency1 = g.FirstOrDefault(item => item.Uplink == "1")?.Latency,
Latency2 = g.FirstOrDefault(item => item.Uplink == "2")?.Latency,
Latency3 = g.FirstOrDefault(item => item.Uplink == "3")?.Latency,
Latency4 = g.FirstOrDefault(item => item.Uplink == "4")?.Latency,
})
});
You're almost there. However you might want to return a value for each latency instead of list of objects:
var transformed = grouped.Select(g => new
{
Hubs = g.Select(hub => new
{
stamp = g.Key,
Latency0 = g.FirstOrDefault(item => item.Uplink == "0")?.Latency,
Latency1 = g.FirstOrDefault(item => item.Uplink == "1")?.Latency,
Latency2 = g.FirstOrDefault(item => item.Uplink == "2")?.Latency,
Latency3 = g.FirstOrDefault(item => item.Uplink == "3")?.Latency,
Latency4 = g.FirstOrDefault(item => item.Uplink == "4")?.Latency,
})
});
edited Nov 20 at 17:20
answered Nov 20 at 17:14
Fabjan
9,64121439
9,64121439
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53397709%2ftransform-rows-data-to-column-from-a-grouped-data-in-linq%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
WoTh3V,QyH22SqAfCPqxRf,V6DMZIb9 h6 Meuc0Hqaz6BjXl9j9V B