Call to undefined method “Connect”
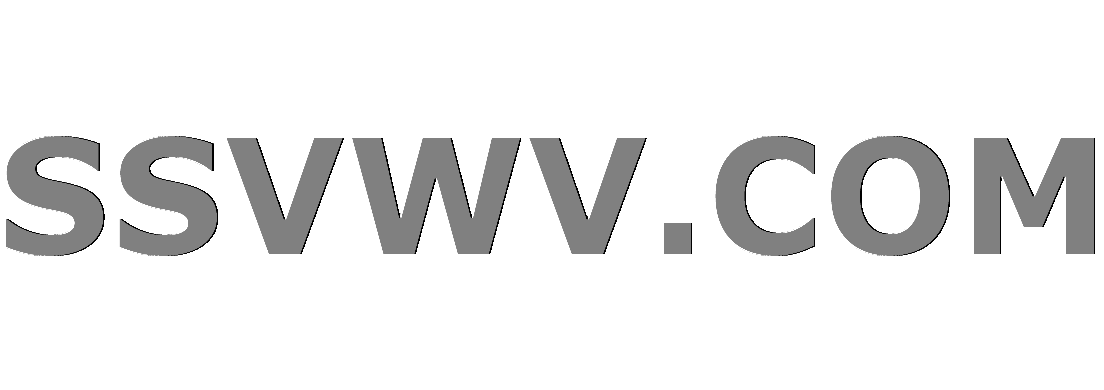
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I'm working in a multi-tenant system, I previously tried the https://github.com/hyn/multi- tenant package but in the end I decided to do this implementation with my own method. After a lot of research and testing different things I have managed to build this code, but when I execute it tells me that the connect method is not defined, here I leave what I have done, most likely this is due to some nonsense that I has overlooked, but if someone can help me solve this I would greatly appreciate it
helpers:
use IlluminateSupportFacadesConfig;
use IlluminateSupportFacadesDB;
use IlluminateSupportFacadesSchema;
if(! function_exists('conexionBD')){
/**
* Establish a tenant database connection.
*
* @param $hostname
* @param $username
* @param $password
* @param $database
*/
function conexionBD($hostname, $username, $password, $database){
DB::purge('empresa');
Config::set('database.connections.empresa.host', $hostname);
Config::set('database.connections.empresa.database', $database);
Config::set('database.connections.empresa.username', $username);
Config::set('database.connections.empresa.password', $password);
DB::reconnect('empresa');
Schema::connection('empresa')->getConnection()->reconnect();
}
}
Empresa Model:
class empresa extends Model
{
protected $fillable = [
'hostname',
'username',
'password',
'database'
];
public function ciudad() {
return $this->belongsTo('confiaciudad');
}
public function empresaUsuario() {
return $this->hasMany('confiaempresasUsuario');
}
public function connect()
{
if (! $this->connected()) {
conexionBD(
$this->hostname,
$this->username,
$this->password,
$this->database
);
}
}
/**
* Check if the current tenant connection settings matches the company's database settings.
*
* @return bool
*/
private function connected()
{
$connection = Config::get('database.connections.empresa');
return $connection['username'] == $this->username &&
$connection['password'] == $this->password &&
$connection['database'] == $this->database;
}
}
Middleware Tenant:
use Closure;
use confiaempresa;
class Tenant{
protected $company;
/**
* Tenant Conctructor
* @param empresa $empresa
*/
public function __construct(empresa $empresa)
{
$this->empresa = $empresa;
}
/**
* Handle an incoming request.
*
* @param IlluminateHttpRequest $request
* @param Closure $next
* @return mixed
*/
public function handle($request, Closure $next) {
if (($request->session()->get('empresaId')) === null)
return redirect()->route('inicio')->withErrors(['error' => __('Por favor inicie sesión en alguna empresa antes de intentar esta acción')]);
// Get the company object with the id stored in session
$empresa= $this->empresa->find($request->session()->get('empresaId'));
// Connect and place the $company object in the view
$this->connect($empresa);
return $next($request);
}
}
In my session variable empresaId I have stored the ID of my company concat to a static string, this is what gives me the name of the database associated with that company.
When I run the system, at the time of making the change of tenant to start working with another database, I get the following error,
"Call to undefined method appHttpMiddlewareTenant::connect()"
Someone knows that my code is missing or that I am doing wrong to make the multi-tenant system work correctly?
laravel laravel-5 database-connection multi-tenant
add a comment |
I'm working in a multi-tenant system, I previously tried the https://github.com/hyn/multi- tenant package but in the end I decided to do this implementation with my own method. After a lot of research and testing different things I have managed to build this code, but when I execute it tells me that the connect method is not defined, here I leave what I have done, most likely this is due to some nonsense that I has overlooked, but if someone can help me solve this I would greatly appreciate it
helpers:
use IlluminateSupportFacadesConfig;
use IlluminateSupportFacadesDB;
use IlluminateSupportFacadesSchema;
if(! function_exists('conexionBD')){
/**
* Establish a tenant database connection.
*
* @param $hostname
* @param $username
* @param $password
* @param $database
*/
function conexionBD($hostname, $username, $password, $database){
DB::purge('empresa');
Config::set('database.connections.empresa.host', $hostname);
Config::set('database.connections.empresa.database', $database);
Config::set('database.connections.empresa.username', $username);
Config::set('database.connections.empresa.password', $password);
DB::reconnect('empresa');
Schema::connection('empresa')->getConnection()->reconnect();
}
}
Empresa Model:
class empresa extends Model
{
protected $fillable = [
'hostname',
'username',
'password',
'database'
];
public function ciudad() {
return $this->belongsTo('confiaciudad');
}
public function empresaUsuario() {
return $this->hasMany('confiaempresasUsuario');
}
public function connect()
{
if (! $this->connected()) {
conexionBD(
$this->hostname,
$this->username,
$this->password,
$this->database
);
}
}
/**
* Check if the current tenant connection settings matches the company's database settings.
*
* @return bool
*/
private function connected()
{
$connection = Config::get('database.connections.empresa');
return $connection['username'] == $this->username &&
$connection['password'] == $this->password &&
$connection['database'] == $this->database;
}
}
Middleware Tenant:
use Closure;
use confiaempresa;
class Tenant{
protected $company;
/**
* Tenant Conctructor
* @param empresa $empresa
*/
public function __construct(empresa $empresa)
{
$this->empresa = $empresa;
}
/**
* Handle an incoming request.
*
* @param IlluminateHttpRequest $request
* @param Closure $next
* @return mixed
*/
public function handle($request, Closure $next) {
if (($request->session()->get('empresaId')) === null)
return redirect()->route('inicio')->withErrors(['error' => __('Por favor inicie sesión en alguna empresa antes de intentar esta acción')]);
// Get the company object with the id stored in session
$empresa= $this->empresa->find($request->session()->get('empresaId'));
// Connect and place the $company object in the view
$this->connect($empresa);
return $next($request);
}
}
In my session variable empresaId I have stored the ID of my company concat to a static string, this is what gives me the name of the database associated with that company.
When I run the system, at the time of making the change of tenant to start working with another database, I get the following error,
"Call to undefined method appHttpMiddlewareTenant::connect()"
Someone knows that my code is missing or that I am doing wrong to make the multi-tenant system work correctly?
laravel laravel-5 database-connection multi-tenant
why are u passing $empresa to the connect method? when the connect does not require a param. and why dont u try $empresa->connect();
– Kuldeep
Nov 26 '18 at 14:51
Do you have the stack from this error? Theres a call to a static method "connect" inside Tenant class, but I can't see this method in the code you posted.
– Arthur Samarcos
Nov 26 '18 at 15:00
I changed the code of my middleware and that error no longer appears, but now it happens to me that I am not doing the configuration of the database, even though in my table of companies in the database it has all the correct values to do the configuration, the new error I'm getting is SQLSTATE [3D000]: Invalid catalog name: 1046 No database selected
– Diana Carolina Garcia Pino
Nov 26 '18 at 15:37
add a comment |
I'm working in a multi-tenant system, I previously tried the https://github.com/hyn/multi- tenant package but in the end I decided to do this implementation with my own method. After a lot of research and testing different things I have managed to build this code, but when I execute it tells me that the connect method is not defined, here I leave what I have done, most likely this is due to some nonsense that I has overlooked, but if someone can help me solve this I would greatly appreciate it
helpers:
use IlluminateSupportFacadesConfig;
use IlluminateSupportFacadesDB;
use IlluminateSupportFacadesSchema;
if(! function_exists('conexionBD')){
/**
* Establish a tenant database connection.
*
* @param $hostname
* @param $username
* @param $password
* @param $database
*/
function conexionBD($hostname, $username, $password, $database){
DB::purge('empresa');
Config::set('database.connections.empresa.host', $hostname);
Config::set('database.connections.empresa.database', $database);
Config::set('database.connections.empresa.username', $username);
Config::set('database.connections.empresa.password', $password);
DB::reconnect('empresa');
Schema::connection('empresa')->getConnection()->reconnect();
}
}
Empresa Model:
class empresa extends Model
{
protected $fillable = [
'hostname',
'username',
'password',
'database'
];
public function ciudad() {
return $this->belongsTo('confiaciudad');
}
public function empresaUsuario() {
return $this->hasMany('confiaempresasUsuario');
}
public function connect()
{
if (! $this->connected()) {
conexionBD(
$this->hostname,
$this->username,
$this->password,
$this->database
);
}
}
/**
* Check if the current tenant connection settings matches the company's database settings.
*
* @return bool
*/
private function connected()
{
$connection = Config::get('database.connections.empresa');
return $connection['username'] == $this->username &&
$connection['password'] == $this->password &&
$connection['database'] == $this->database;
}
}
Middleware Tenant:
use Closure;
use confiaempresa;
class Tenant{
protected $company;
/**
* Tenant Conctructor
* @param empresa $empresa
*/
public function __construct(empresa $empresa)
{
$this->empresa = $empresa;
}
/**
* Handle an incoming request.
*
* @param IlluminateHttpRequest $request
* @param Closure $next
* @return mixed
*/
public function handle($request, Closure $next) {
if (($request->session()->get('empresaId')) === null)
return redirect()->route('inicio')->withErrors(['error' => __('Por favor inicie sesión en alguna empresa antes de intentar esta acción')]);
// Get the company object with the id stored in session
$empresa= $this->empresa->find($request->session()->get('empresaId'));
// Connect and place the $company object in the view
$this->connect($empresa);
return $next($request);
}
}
In my session variable empresaId I have stored the ID of my company concat to a static string, this is what gives me the name of the database associated with that company.
When I run the system, at the time of making the change of tenant to start working with another database, I get the following error,
"Call to undefined method appHttpMiddlewareTenant::connect()"
Someone knows that my code is missing or that I am doing wrong to make the multi-tenant system work correctly?
laravel laravel-5 database-connection multi-tenant
I'm working in a multi-tenant system, I previously tried the https://github.com/hyn/multi- tenant package but in the end I decided to do this implementation with my own method. After a lot of research and testing different things I have managed to build this code, but when I execute it tells me that the connect method is not defined, here I leave what I have done, most likely this is due to some nonsense that I has overlooked, but if someone can help me solve this I would greatly appreciate it
helpers:
use IlluminateSupportFacadesConfig;
use IlluminateSupportFacadesDB;
use IlluminateSupportFacadesSchema;
if(! function_exists('conexionBD')){
/**
* Establish a tenant database connection.
*
* @param $hostname
* @param $username
* @param $password
* @param $database
*/
function conexionBD($hostname, $username, $password, $database){
DB::purge('empresa');
Config::set('database.connections.empresa.host', $hostname);
Config::set('database.connections.empresa.database', $database);
Config::set('database.connections.empresa.username', $username);
Config::set('database.connections.empresa.password', $password);
DB::reconnect('empresa');
Schema::connection('empresa')->getConnection()->reconnect();
}
}
Empresa Model:
class empresa extends Model
{
protected $fillable = [
'hostname',
'username',
'password',
'database'
];
public function ciudad() {
return $this->belongsTo('confiaciudad');
}
public function empresaUsuario() {
return $this->hasMany('confiaempresasUsuario');
}
public function connect()
{
if (! $this->connected()) {
conexionBD(
$this->hostname,
$this->username,
$this->password,
$this->database
);
}
}
/**
* Check if the current tenant connection settings matches the company's database settings.
*
* @return bool
*/
private function connected()
{
$connection = Config::get('database.connections.empresa');
return $connection['username'] == $this->username &&
$connection['password'] == $this->password &&
$connection['database'] == $this->database;
}
}
Middleware Tenant:
use Closure;
use confiaempresa;
class Tenant{
protected $company;
/**
* Tenant Conctructor
* @param empresa $empresa
*/
public function __construct(empresa $empresa)
{
$this->empresa = $empresa;
}
/**
* Handle an incoming request.
*
* @param IlluminateHttpRequest $request
* @param Closure $next
* @return mixed
*/
public function handle($request, Closure $next) {
if (($request->session()->get('empresaId')) === null)
return redirect()->route('inicio')->withErrors(['error' => __('Por favor inicie sesión en alguna empresa antes de intentar esta acción')]);
// Get the company object with the id stored in session
$empresa= $this->empresa->find($request->session()->get('empresaId'));
// Connect and place the $company object in the view
$this->connect($empresa);
return $next($request);
}
}
In my session variable empresaId I have stored the ID of my company concat to a static string, this is what gives me the name of the database associated with that company.
When I run the system, at the time of making the change of tenant to start working with another database, I get the following error,
"Call to undefined method appHttpMiddlewareTenant::connect()"
Someone knows that my code is missing or that I am doing wrong to make the multi-tenant system work correctly?
laravel laravel-5 database-connection multi-tenant
laravel laravel-5 database-connection multi-tenant
asked Nov 26 '18 at 13:53


Diana Carolina Garcia PinoDiana Carolina Garcia Pino
31
31
why are u passing $empresa to the connect method? when the connect does not require a param. and why dont u try $empresa->connect();
– Kuldeep
Nov 26 '18 at 14:51
Do you have the stack from this error? Theres a call to a static method "connect" inside Tenant class, but I can't see this method in the code you posted.
– Arthur Samarcos
Nov 26 '18 at 15:00
I changed the code of my middleware and that error no longer appears, but now it happens to me that I am not doing the configuration of the database, even though in my table of companies in the database it has all the correct values to do the configuration, the new error I'm getting is SQLSTATE [3D000]: Invalid catalog name: 1046 No database selected
– Diana Carolina Garcia Pino
Nov 26 '18 at 15:37
add a comment |
why are u passing $empresa to the connect method? when the connect does not require a param. and why dont u try $empresa->connect();
– Kuldeep
Nov 26 '18 at 14:51
Do you have the stack from this error? Theres a call to a static method "connect" inside Tenant class, but I can't see this method in the code you posted.
– Arthur Samarcos
Nov 26 '18 at 15:00
I changed the code of my middleware and that error no longer appears, but now it happens to me that I am not doing the configuration of the database, even though in my table of companies in the database it has all the correct values to do the configuration, the new error I'm getting is SQLSTATE [3D000]: Invalid catalog name: 1046 No database selected
– Diana Carolina Garcia Pino
Nov 26 '18 at 15:37
why are u passing $empresa to the connect method? when the connect does not require a param. and why dont u try $empresa->connect();
– Kuldeep
Nov 26 '18 at 14:51
why are u passing $empresa to the connect method? when the connect does not require a param. and why dont u try $empresa->connect();
– Kuldeep
Nov 26 '18 at 14:51
Do you have the stack from this error? Theres a call to a static method "connect" inside Tenant class, but I can't see this method in the code you posted.
– Arthur Samarcos
Nov 26 '18 at 15:00
Do you have the stack from this error? Theres a call to a static method "connect" inside Tenant class, but I can't see this method in the code you posted.
– Arthur Samarcos
Nov 26 '18 at 15:00
I changed the code of my middleware and that error no longer appears, but now it happens to me that I am not doing the configuration of the database, even though in my table of companies in the database it has all the correct values to do the configuration, the new error I'm getting is SQLSTATE [3D000]: Invalid catalog name: 1046 No database selected
– Diana Carolina Garcia Pino
Nov 26 '18 at 15:37
I changed the code of my middleware and that error no longer appears, but now it happens to me that I am not doing the configuration of the database, even though in my table of companies in the database it has all the correct values to do the configuration, the new error I'm getting is SQLSTATE [3D000]: Invalid catalog name: 1046 No database selected
– Diana Carolina Garcia Pino
Nov 26 '18 at 15:37
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53482624%2fcall-to-undefined-method-connect%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53482624%2fcall-to-undefined-method-connect%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
0UZ,Y56i,kZDtP Byary9f96dnzgsnS 4,8a1C7,CzofZ1,5vSLGkG7bSqdLIPeEGDTolWAkV5IcEoqbPlVjP,pUvD,5,GSn
why are u passing $empresa to the connect method? when the connect does not require a param. and why dont u try $empresa->connect();
– Kuldeep
Nov 26 '18 at 14:51
Do you have the stack from this error? Theres a call to a static method "connect" inside Tenant class, but I can't see this method in the code you posted.
– Arthur Samarcos
Nov 26 '18 at 15:00
I changed the code of my middleware and that error no longer appears, but now it happens to me that I am not doing the configuration of the database, even though in my table of companies in the database it has all the correct values to do the configuration, the new error I'm getting is SQLSTATE [3D000]: Invalid catalog name: 1046 No database selected
– Diana Carolina Garcia Pino
Nov 26 '18 at 15:37