Nodejs request : REST API call inside REST API
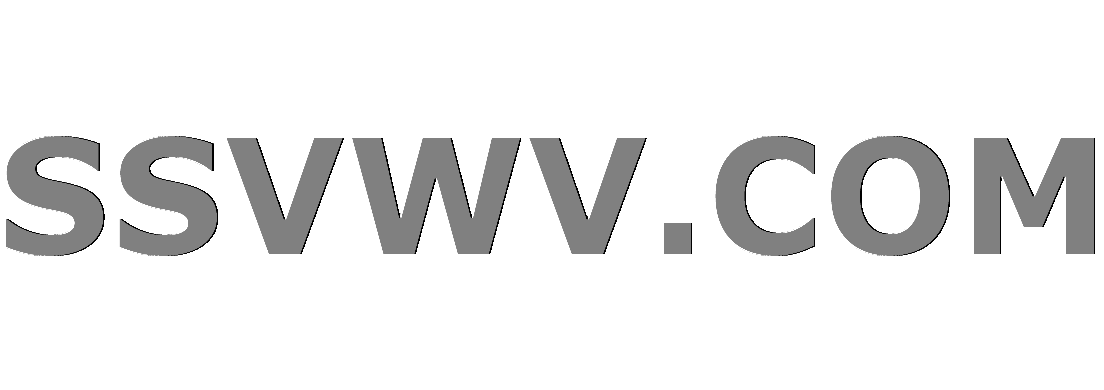
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I am developing a REST API Framework. The client will call a my end point using POST. My code then will make an additional call to an external system using POST. But, I am running into issues with the request library. Look at my code snippet below.
var express = require("express");
var app = express();
var cfenv = require("cfenv");
var bodyParser = require('body-parser');
var request = require('request');
app.use(bodyParser.urlencoded({
extended: false
}))
app.use(bodyParser.json())
var mydb;
app.post("/token", function (req, response) {
console.log("Call to /token");
var token = "a"
var headers = {
'Content-Type': 'application/json'
}
var options = {
url: 'https://xyz/oauth2//token',
method: 'POST',
headers: headers,
json: {
'key1': 'xxx',
'key2': 'yyy'
}
}
request(options, function (error, response, body) {
if (!error && response.statusCode == 200) {
console.log(body)
} else {
console.log(body)
}
})
return response.send(token)
The return will always be 'a' because of the async nature of the request method.
How to return the right value everytime?
node.js node-modules node-request
add a comment |
I am developing a REST API Framework. The client will call a my end point using POST. My code then will make an additional call to an external system using POST. But, I am running into issues with the request library. Look at my code snippet below.
var express = require("express");
var app = express();
var cfenv = require("cfenv");
var bodyParser = require('body-parser');
var request = require('request');
app.use(bodyParser.urlencoded({
extended: false
}))
app.use(bodyParser.json())
var mydb;
app.post("/token", function (req, response) {
console.log("Call to /token");
var token = "a"
var headers = {
'Content-Type': 'application/json'
}
var options = {
url: 'https://xyz/oauth2//token',
method: 'POST',
headers: headers,
json: {
'key1': 'xxx',
'key2': 'yyy'
}
}
request(options, function (error, response, body) {
if (!error && response.statusCode == 200) {
console.log(body)
} else {
console.log(body)
}
})
return response.send(token)
The return will always be 'a' because of the async nature of the request method.
How to return the right value everytime?
node.js node-modules node-request
Can you please edit your code to fix the indentation and to remove the double spacing so we can read it better here on stack overflow.
– jfriend00
Nov 27 '18 at 6:07
token is never reassigned in your code. Therefor it will always be "a" as declared at the top: var token = "a"
– Luke Walker
Nov 27 '18 at 10:52
add a comment |
I am developing a REST API Framework. The client will call a my end point using POST. My code then will make an additional call to an external system using POST. But, I am running into issues with the request library. Look at my code snippet below.
var express = require("express");
var app = express();
var cfenv = require("cfenv");
var bodyParser = require('body-parser');
var request = require('request');
app.use(bodyParser.urlencoded({
extended: false
}))
app.use(bodyParser.json())
var mydb;
app.post("/token", function (req, response) {
console.log("Call to /token");
var token = "a"
var headers = {
'Content-Type': 'application/json'
}
var options = {
url: 'https://xyz/oauth2//token',
method: 'POST',
headers: headers,
json: {
'key1': 'xxx',
'key2': 'yyy'
}
}
request(options, function (error, response, body) {
if (!error && response.statusCode == 200) {
console.log(body)
} else {
console.log(body)
}
})
return response.send(token)
The return will always be 'a' because of the async nature of the request method.
How to return the right value everytime?
node.js node-modules node-request
I am developing a REST API Framework. The client will call a my end point using POST. My code then will make an additional call to an external system using POST. But, I am running into issues with the request library. Look at my code snippet below.
var express = require("express");
var app = express();
var cfenv = require("cfenv");
var bodyParser = require('body-parser');
var request = require('request');
app.use(bodyParser.urlencoded({
extended: false
}))
app.use(bodyParser.json())
var mydb;
app.post("/token", function (req, response) {
console.log("Call to /token");
var token = "a"
var headers = {
'Content-Type': 'application/json'
}
var options = {
url: 'https://xyz/oauth2//token',
method: 'POST',
headers: headers,
json: {
'key1': 'xxx',
'key2': 'yyy'
}
}
request(options, function (error, response, body) {
if (!error && response.statusCode == 200) {
console.log(body)
} else {
console.log(body)
}
})
return response.send(token)
The return will always be 'a' because of the async nature of the request method.
How to return the right value everytime?
node.js node-modules node-request
node.js node-modules node-request
edited Nov 27 '18 at 10:08


Anuj raval
85
85
asked Nov 27 '18 at 1:55


Pranam CodurPranam Codur
82
82
Can you please edit your code to fix the indentation and to remove the double spacing so we can read it better here on stack overflow.
– jfriend00
Nov 27 '18 at 6:07
token is never reassigned in your code. Therefor it will always be "a" as declared at the top: var token = "a"
– Luke Walker
Nov 27 '18 at 10:52
add a comment |
Can you please edit your code to fix the indentation and to remove the double spacing so we can read it better here on stack overflow.
– jfriend00
Nov 27 '18 at 6:07
token is never reassigned in your code. Therefor it will always be "a" as declared at the top: var token = "a"
– Luke Walker
Nov 27 '18 at 10:52
Can you please edit your code to fix the indentation and to remove the double spacing so we can read it better here on stack overflow.
– jfriend00
Nov 27 '18 at 6:07
Can you please edit your code to fix the indentation and to remove the double spacing so we can read it better here on stack overflow.
– jfriend00
Nov 27 '18 at 6:07
token is never reassigned in your code. Therefor it will always be "a" as declared at the top: var token = "a"
– Luke Walker
Nov 27 '18 at 10:52
token is never reassigned in your code. Therefor it will always be "a" as declared at the top: var token = "a"
– Luke Walker
Nov 27 '18 at 10:52
add a comment |
2 Answers
2
active
oldest
votes
You understand the problem, the next step is easy. call res.send
inside your callback, like this below:
request(options, function (error, response, body) {
if (!error && response.statusCode == 200) {
// Print out the response body
console.log(body)
res.send(body)
} else {
console.log(body)
}
})
And remove the return from the end of the function
add a comment |
From what I see you do not reassign value of token
variable in the code sample you've posted, so it will always be a
. Please elaborate on what you want to achieve, what you want to have in token
variable and what do you want to do with it? I'll be glad to help.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53491667%2fnodejs-request-rest-api-call-inside-rest-api%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You understand the problem, the next step is easy. call res.send
inside your callback, like this below:
request(options, function (error, response, body) {
if (!error && response.statusCode == 200) {
// Print out the response body
console.log(body)
res.send(body)
} else {
console.log(body)
}
})
And remove the return from the end of the function
add a comment |
You understand the problem, the next step is easy. call res.send
inside your callback, like this below:
request(options, function (error, response, body) {
if (!error && response.statusCode == 200) {
// Print out the response body
console.log(body)
res.send(body)
} else {
console.log(body)
}
})
And remove the return from the end of the function
add a comment |
You understand the problem, the next step is easy. call res.send
inside your callback, like this below:
request(options, function (error, response, body) {
if (!error && response.statusCode == 200) {
// Print out the response body
console.log(body)
res.send(body)
} else {
console.log(body)
}
})
And remove the return from the end of the function
You understand the problem, the next step is easy. call res.send
inside your callback, like this below:
request(options, function (error, response, body) {
if (!error && response.statusCode == 200) {
// Print out the response body
console.log(body)
res.send(body)
} else {
console.log(body)
}
})
And remove the return from the end of the function
edited Nov 27 '18 at 13:20
Luke Walker
279115
279115
answered Nov 27 '18 at 2:23
iagowpiagowp
1,42411326
1,42411326
add a comment |
add a comment |
From what I see you do not reassign value of token
variable in the code sample you've posted, so it will always be a
. Please elaborate on what you want to achieve, what you want to have in token
variable and what do you want to do with it? I'll be glad to help.
add a comment |
From what I see you do not reassign value of token
variable in the code sample you've posted, so it will always be a
. Please elaborate on what you want to achieve, what you want to have in token
variable and what do you want to do with it? I'll be glad to help.
add a comment |
From what I see you do not reassign value of token
variable in the code sample you've posted, so it will always be a
. Please elaborate on what you want to achieve, what you want to have in token
variable and what do you want to do with it? I'll be glad to help.
From what I see you do not reassign value of token
variable in the code sample you've posted, so it will always be a
. Please elaborate on what you want to achieve, what you want to have in token
variable and what do you want to do with it? I'll be glad to help.
answered Nov 27 '18 at 10:49
mindrivenmindriven
12818
12818
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53491667%2fnodejs-request-rest-api-call-inside-rest-api%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
OJ,BuF3Ob8efDVl4C Mcr ydGQ l0IuDP3YiJt D8
Can you please edit your code to fix the indentation and to remove the double spacing so we can read it better here on stack overflow.
– jfriend00
Nov 27 '18 at 6:07
token is never reassigned in your code. Therefor it will always be "a" as declared at the top: var token = "a"
– Luke Walker
Nov 27 '18 at 10:52