unit testing - jest with supertest gives a timeout
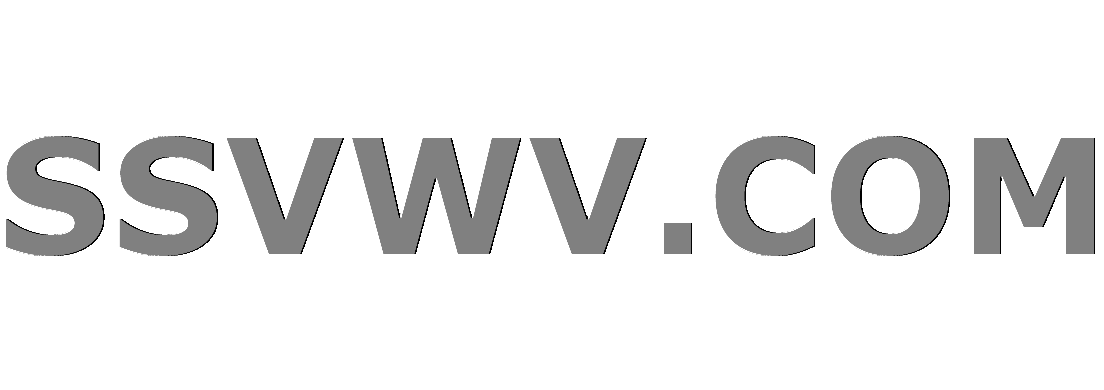
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I got an error running my unit tests. I am using a express app from NodeJS. I want to test the return message (200) and (eventually) the output of a function.
const {Router} = require('express');
const {query} = require('../dbConfig');
module.exports = (router = new Router()) => {
//get only one user from the id
router.post('/getUser', async (req,res)=>{
id = req.body.id;
sql = "SELECT lastname, firstname, email, title_id, id, state FROM users WHERE id=" + id;
let result = await query(sql);
if(result.length >= 1){
res.send(result[0]);
}
})
return router;
};
But when I try to run the following test :
const app = require('../../app')
const request = require('supertest')
jest.mock('../../dbConfig');;
describe('POST /getUser', () => {
let data = {
id:1
}
it('Response 200 + response type',(done)=>{
request.agent(app)
.post('/getUser')
.send(data)
.expect(200, done)
})
});
It returns me the following error
Timeout - Async callback was not invoked within the 5000ms timeout specified by jest.setTimeout.
I tried diffrent variations without success... I also tried the doc online which wasn't really helpful for this error... I run jest with npx jest
and the mock file looks like this :
const rep={
"lastname": "admin",
"firstname": "admin",
"email": "admin@etsmtl.ca",
"title_id": 1,
"id": 1,
"state": 1
};
const query = (sqli) =>{
return new Promise((resolve, reject) =>{
console.log("SQL request : " + sqli)
if(sqli === "SELECT lastname, firstname, email, title_id, id, state FROM users WHERE id=1"){
console.log("Passed")
resolve(rep);
}
});
}
module.exports = {query};
node.js unit-testing express jestjs supertest
add a comment |
I got an error running my unit tests. I am using a express app from NodeJS. I want to test the return message (200) and (eventually) the output of a function.
const {Router} = require('express');
const {query} = require('../dbConfig');
module.exports = (router = new Router()) => {
//get only one user from the id
router.post('/getUser', async (req,res)=>{
id = req.body.id;
sql = "SELECT lastname, firstname, email, title_id, id, state FROM users WHERE id=" + id;
let result = await query(sql);
if(result.length >= 1){
res.send(result[0]);
}
})
return router;
};
But when I try to run the following test :
const app = require('../../app')
const request = require('supertest')
jest.mock('../../dbConfig');;
describe('POST /getUser', () => {
let data = {
id:1
}
it('Response 200 + response type',(done)=>{
request.agent(app)
.post('/getUser')
.send(data)
.expect(200, done)
})
});
It returns me the following error
Timeout - Async callback was not invoked within the 5000ms timeout specified by jest.setTimeout.
I tried diffrent variations without success... I also tried the doc online which wasn't really helpful for this error... I run jest with npx jest
and the mock file looks like this :
const rep={
"lastname": "admin",
"firstname": "admin",
"email": "admin@etsmtl.ca",
"title_id": 1,
"id": 1,
"state": 1
};
const query = (sqli) =>{
return new Promise((resolve, reject) =>{
console.log("SQL request : " + sqli)
if(sqli === "SELECT lastname, firstname, email, title_id, id, state FROM users WHERE id=1"){
console.log("Passed")
resolve(rep);
}
});
}
module.exports = {query};
node.js unit-testing express jestjs supertest
add a comment |
I got an error running my unit tests. I am using a express app from NodeJS. I want to test the return message (200) and (eventually) the output of a function.
const {Router} = require('express');
const {query} = require('../dbConfig');
module.exports = (router = new Router()) => {
//get only one user from the id
router.post('/getUser', async (req,res)=>{
id = req.body.id;
sql = "SELECT lastname, firstname, email, title_id, id, state FROM users WHERE id=" + id;
let result = await query(sql);
if(result.length >= 1){
res.send(result[0]);
}
})
return router;
};
But when I try to run the following test :
const app = require('../../app')
const request = require('supertest')
jest.mock('../../dbConfig');;
describe('POST /getUser', () => {
let data = {
id:1
}
it('Response 200 + response type',(done)=>{
request.agent(app)
.post('/getUser')
.send(data)
.expect(200, done)
})
});
It returns me the following error
Timeout - Async callback was not invoked within the 5000ms timeout specified by jest.setTimeout.
I tried diffrent variations without success... I also tried the doc online which wasn't really helpful for this error... I run jest with npx jest
and the mock file looks like this :
const rep={
"lastname": "admin",
"firstname": "admin",
"email": "admin@etsmtl.ca",
"title_id": 1,
"id": 1,
"state": 1
};
const query = (sqli) =>{
return new Promise((resolve, reject) =>{
console.log("SQL request : " + sqli)
if(sqli === "SELECT lastname, firstname, email, title_id, id, state FROM users WHERE id=1"){
console.log("Passed")
resolve(rep);
}
});
}
module.exports = {query};
node.js unit-testing express jestjs supertest
I got an error running my unit tests. I am using a express app from NodeJS. I want to test the return message (200) and (eventually) the output of a function.
const {Router} = require('express');
const {query} = require('../dbConfig');
module.exports = (router = new Router()) => {
//get only one user from the id
router.post('/getUser', async (req,res)=>{
id = req.body.id;
sql = "SELECT lastname, firstname, email, title_id, id, state FROM users WHERE id=" + id;
let result = await query(sql);
if(result.length >= 1){
res.send(result[0]);
}
})
return router;
};
But when I try to run the following test :
const app = require('../../app')
const request = require('supertest')
jest.mock('../../dbConfig');;
describe('POST /getUser', () => {
let data = {
id:1
}
it('Response 200 + response type',(done)=>{
request.agent(app)
.post('/getUser')
.send(data)
.expect(200, done)
})
});
It returns me the following error
Timeout - Async callback was not invoked within the 5000ms timeout specified by jest.setTimeout.
I tried diffrent variations without success... I also tried the doc online which wasn't really helpful for this error... I run jest with npx jest
and the mock file looks like this :
const rep={
"lastname": "admin",
"firstname": "admin",
"email": "admin@etsmtl.ca",
"title_id": 1,
"id": 1,
"state": 1
};
const query = (sqli) =>{
return new Promise((resolve, reject) =>{
console.log("SQL request : " + sqli)
if(sqli === "SELECT lastname, firstname, email, title_id, id, state FROM users WHERE id=1"){
console.log("Passed")
resolve(rep);
}
});
}
module.exports = {query};
node.js unit-testing express jestjs supertest
node.js unit-testing express jestjs supertest
edited Nov 26 '18 at 21:16
skyboyer
4,23811333
4,23811333
asked Nov 26 '18 at 15:30
etiennnretiennnr
155
155
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
Your query
method returns an object (rep
), and you check the length of it in the POST
handler. The length of an object is undefined
, so it doesn't reach the res.send
. You need to set an else
option with another res.send
so it answers the client no matter what happens. Also, you probably want to change that if
in the POST
handler, or the format of the return in the mock file.
For instance, in your Mock file:
const query = (sqli) =>{
return new Promise((resolve, reject) =>{
console.log("SQL request : " + sqli)
if(sqli === "SELECT lastname, firstname, email, title_id, id, state FROM users WHERE id=1"){
console.log("Passed")
resolve([rep]); //Now it's an array of length 1, reaches the first send
} else {
reject(); //Length is 0, so it will reach the second send
}
});
}
Controller
if(result.length >= 1){
res.send(result[0]);
} else {
res.status(500).send('Something broke!');
}
1
Thanks you! I always forget to take errors in consideration but, trust me, now I will!
– etiennnr
Nov 26 '18 at 16:41
add a comment |
Are you sure your db is initialized? Can you try this at your controller?
router.post('/getUser', async (req,res)=>{
id = req.body.id;
sql = "SELECT lastname, firstname, email, title_id, id, state FROM users WHERE id=" + id;
try {
let result = await query(sql);
if(result.length >= 1){
res.send(result[0]);
} else {
res.sendStatus(404);
}
} catch(err) {
res.sendStatus(500)
}
})
In case your db isnt correctly initialized, or if query throws an error, it will never respond
Actually, as you can see in the test, the "query" method is mocked.. So it besically returns fake data just for testing purposes. And the function also works when I try it from postman so only the unit test have someting wrong...
– etiennnr
Nov 26 '18 at 16:36
@etiennnr Well, the reason you had a timeout still was the lack of an else/catch
– iagowp
Nov 26 '18 at 19:12
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53484391%2funit-testing-jest-with-supertest-gives-a-timeout%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Your query
method returns an object (rep
), and you check the length of it in the POST
handler. The length of an object is undefined
, so it doesn't reach the res.send
. You need to set an else
option with another res.send
so it answers the client no matter what happens. Also, you probably want to change that if
in the POST
handler, or the format of the return in the mock file.
For instance, in your Mock file:
const query = (sqli) =>{
return new Promise((resolve, reject) =>{
console.log("SQL request : " + sqli)
if(sqli === "SELECT lastname, firstname, email, title_id, id, state FROM users WHERE id=1"){
console.log("Passed")
resolve([rep]); //Now it's an array of length 1, reaches the first send
} else {
reject(); //Length is 0, so it will reach the second send
}
});
}
Controller
if(result.length >= 1){
res.send(result[0]);
} else {
res.status(500).send('Something broke!');
}
1
Thanks you! I always forget to take errors in consideration but, trust me, now I will!
– etiennnr
Nov 26 '18 at 16:41
add a comment |
Your query
method returns an object (rep
), and you check the length of it in the POST
handler. The length of an object is undefined
, so it doesn't reach the res.send
. You need to set an else
option with another res.send
so it answers the client no matter what happens. Also, you probably want to change that if
in the POST
handler, or the format of the return in the mock file.
For instance, in your Mock file:
const query = (sqli) =>{
return new Promise((resolve, reject) =>{
console.log("SQL request : " + sqli)
if(sqli === "SELECT lastname, firstname, email, title_id, id, state FROM users WHERE id=1"){
console.log("Passed")
resolve([rep]); //Now it's an array of length 1, reaches the first send
} else {
reject(); //Length is 0, so it will reach the second send
}
});
}
Controller
if(result.length >= 1){
res.send(result[0]);
} else {
res.status(500).send('Something broke!');
}
1
Thanks you! I always forget to take errors in consideration but, trust me, now I will!
– etiennnr
Nov 26 '18 at 16:41
add a comment |
Your query
method returns an object (rep
), and you check the length of it in the POST
handler. The length of an object is undefined
, so it doesn't reach the res.send
. You need to set an else
option with another res.send
so it answers the client no matter what happens. Also, you probably want to change that if
in the POST
handler, or the format of the return in the mock file.
For instance, in your Mock file:
const query = (sqli) =>{
return new Promise((resolve, reject) =>{
console.log("SQL request : " + sqli)
if(sqli === "SELECT lastname, firstname, email, title_id, id, state FROM users WHERE id=1"){
console.log("Passed")
resolve([rep]); //Now it's an array of length 1, reaches the first send
} else {
reject(); //Length is 0, so it will reach the second send
}
});
}
Controller
if(result.length >= 1){
res.send(result[0]);
} else {
res.status(500).send('Something broke!');
}
Your query
method returns an object (rep
), and you check the length of it in the POST
handler. The length of an object is undefined
, so it doesn't reach the res.send
. You need to set an else
option with another res.send
so it answers the client no matter what happens. Also, you probably want to change that if
in the POST
handler, or the format of the return in the mock file.
For instance, in your Mock file:
const query = (sqli) =>{
return new Promise((resolve, reject) =>{
console.log("SQL request : " + sqli)
if(sqli === "SELECT lastname, firstname, email, title_id, id, state FROM users WHERE id=1"){
console.log("Passed")
resolve([rep]); //Now it's an array of length 1, reaches the first send
} else {
reject(); //Length is 0, so it will reach the second send
}
});
}
Controller
if(result.length >= 1){
res.send(result[0]);
} else {
res.status(500).send('Something broke!');
}
edited Nov 26 '18 at 15:57
answered Nov 26 '18 at 15:50


J. MariaJ. Maria
152111
152111
1
Thanks you! I always forget to take errors in consideration but, trust me, now I will!
– etiennnr
Nov 26 '18 at 16:41
add a comment |
1
Thanks you! I always forget to take errors in consideration but, trust me, now I will!
– etiennnr
Nov 26 '18 at 16:41
1
1
Thanks you! I always forget to take errors in consideration but, trust me, now I will!
– etiennnr
Nov 26 '18 at 16:41
Thanks you! I always forget to take errors in consideration but, trust me, now I will!
– etiennnr
Nov 26 '18 at 16:41
add a comment |
Are you sure your db is initialized? Can you try this at your controller?
router.post('/getUser', async (req,res)=>{
id = req.body.id;
sql = "SELECT lastname, firstname, email, title_id, id, state FROM users WHERE id=" + id;
try {
let result = await query(sql);
if(result.length >= 1){
res.send(result[0]);
} else {
res.sendStatus(404);
}
} catch(err) {
res.sendStatus(500)
}
})
In case your db isnt correctly initialized, or if query throws an error, it will never respond
Actually, as you can see in the test, the "query" method is mocked.. So it besically returns fake data just for testing purposes. And the function also works when I try it from postman so only the unit test have someting wrong...
– etiennnr
Nov 26 '18 at 16:36
@etiennnr Well, the reason you had a timeout still was the lack of an else/catch
– iagowp
Nov 26 '18 at 19:12
add a comment |
Are you sure your db is initialized? Can you try this at your controller?
router.post('/getUser', async (req,res)=>{
id = req.body.id;
sql = "SELECT lastname, firstname, email, title_id, id, state FROM users WHERE id=" + id;
try {
let result = await query(sql);
if(result.length >= 1){
res.send(result[0]);
} else {
res.sendStatus(404);
}
} catch(err) {
res.sendStatus(500)
}
})
In case your db isnt correctly initialized, or if query throws an error, it will never respond
Actually, as you can see in the test, the "query" method is mocked.. So it besically returns fake data just for testing purposes. And the function also works when I try it from postman so only the unit test have someting wrong...
– etiennnr
Nov 26 '18 at 16:36
@etiennnr Well, the reason you had a timeout still was the lack of an else/catch
– iagowp
Nov 26 '18 at 19:12
add a comment |
Are you sure your db is initialized? Can you try this at your controller?
router.post('/getUser', async (req,res)=>{
id = req.body.id;
sql = "SELECT lastname, firstname, email, title_id, id, state FROM users WHERE id=" + id;
try {
let result = await query(sql);
if(result.length >= 1){
res.send(result[0]);
} else {
res.sendStatus(404);
}
} catch(err) {
res.sendStatus(500)
}
})
In case your db isnt correctly initialized, or if query throws an error, it will never respond
Are you sure your db is initialized? Can you try this at your controller?
router.post('/getUser', async (req,res)=>{
id = req.body.id;
sql = "SELECT lastname, firstname, email, title_id, id, state FROM users WHERE id=" + id;
try {
let result = await query(sql);
if(result.length >= 1){
res.send(result[0]);
} else {
res.sendStatus(404);
}
} catch(err) {
res.sendStatus(500)
}
})
In case your db isnt correctly initialized, or if query throws an error, it will never respond
answered Nov 26 '18 at 15:48
iagowpiagowp
1,40211326
1,40211326
Actually, as you can see in the test, the "query" method is mocked.. So it besically returns fake data just for testing purposes. And the function also works when I try it from postman so only the unit test have someting wrong...
– etiennnr
Nov 26 '18 at 16:36
@etiennnr Well, the reason you had a timeout still was the lack of an else/catch
– iagowp
Nov 26 '18 at 19:12
add a comment |
Actually, as you can see in the test, the "query" method is mocked.. So it besically returns fake data just for testing purposes. And the function also works when I try it from postman so only the unit test have someting wrong...
– etiennnr
Nov 26 '18 at 16:36
@etiennnr Well, the reason you had a timeout still was the lack of an else/catch
– iagowp
Nov 26 '18 at 19:12
Actually, as you can see in the test, the "query" method is mocked.. So it besically returns fake data just for testing purposes. And the function also works when I try it from postman so only the unit test have someting wrong...
– etiennnr
Nov 26 '18 at 16:36
Actually, as you can see in the test, the "query" method is mocked.. So it besically returns fake data just for testing purposes. And the function also works when I try it from postman so only the unit test have someting wrong...
– etiennnr
Nov 26 '18 at 16:36
@etiennnr Well, the reason you had a timeout still was the lack of an else/catch
– iagowp
Nov 26 '18 at 19:12
@etiennnr Well, the reason you had a timeout still was the lack of an else/catch
– iagowp
Nov 26 '18 at 19:12
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53484391%2funit-testing-jest-with-supertest-gives-a-timeout%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
q8iB9ZWlF6e7bHuLiLaTh3ep17THgXMfFOZb 2LRiv,rRk3sdeIMl28axJrVHpTbVqfMA34F3e12,L7SIs ML1appsFfA5ikwGo