How do I apply XSL with Javascript when the XSL uses xsl:include?
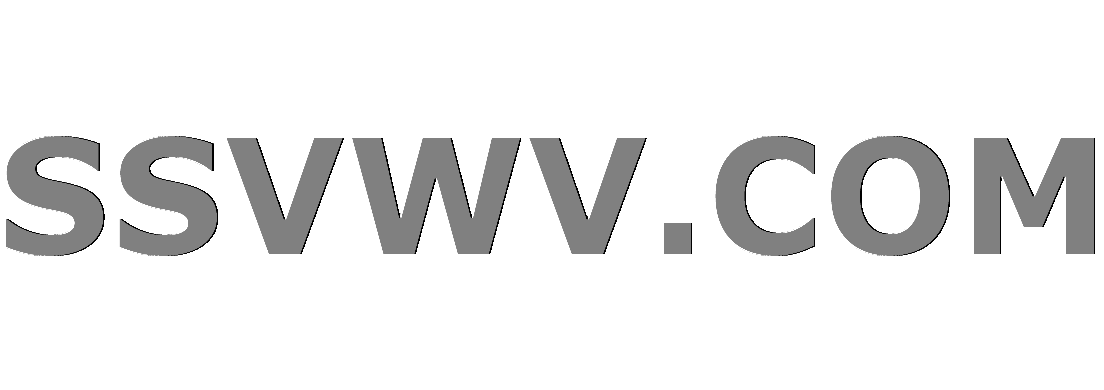
Multi tool use
I'm working with the XSLT code sample on W3Schools: https://www.w3schools.com/xml/xsl_client.asp
I have this section of code working in IE with basic xslt processing...
<!DOCTYPE html>
<html>
<head>
<script>
function loadXMLDoc(filename)
{
if (window.ActiveXObject)
{
xhttp = new ActiveXObject("Msxml2.XMLHTTP");
}
else
{
xhttp = new XMLHttpRequest();
}
xhttp.open("GET", filename, false);
try {xhttp.responseType = "msxml-document"} catch(err) {} // Helping IE11
xhttp.send("");
return xhttp.responseXML;
}
function displayResult()
{
xml = loadXMLDoc("cdcatalog.xml");
xsl = loadXMLDoc("cdcatalog.xsl");
// code for IE
if (window.ActiveXObject || xhttp.responseType == "msxml-document")
{
ex = xml.transformNode(xsl);
document.getElementById("example").innerHTML = ex;
}
}
</script>
</head>
<body onload="displayResult()">
<div id="example" />
</body>
</html>
The problem is the XSL I'm scaling this solution for has a complex structure, so there are several xsl:include statements to pull in other xsl templates (https://www.w3schools.com/xml/ref_xsl_el_include.asp). Javascript isn't processing the xsl:include imports and the XSLT processor keeps complaining about missing templates.
How do I get Javascript to process xsl:include?
javascript xml xslt
add a comment |
I'm working with the XSLT code sample on W3Schools: https://www.w3schools.com/xml/xsl_client.asp
I have this section of code working in IE with basic xslt processing...
<!DOCTYPE html>
<html>
<head>
<script>
function loadXMLDoc(filename)
{
if (window.ActiveXObject)
{
xhttp = new ActiveXObject("Msxml2.XMLHTTP");
}
else
{
xhttp = new XMLHttpRequest();
}
xhttp.open("GET", filename, false);
try {xhttp.responseType = "msxml-document"} catch(err) {} // Helping IE11
xhttp.send("");
return xhttp.responseXML;
}
function displayResult()
{
xml = loadXMLDoc("cdcatalog.xml");
xsl = loadXMLDoc("cdcatalog.xsl");
// code for IE
if (window.ActiveXObject || xhttp.responseType == "msxml-document")
{
ex = xml.transformNode(xsl);
document.getElementById("example").innerHTML = ex;
}
}
</script>
</head>
<body onload="displayResult()">
<div id="example" />
</body>
</html>
The problem is the XSL I'm scaling this solution for has a complex structure, so there are several xsl:include statements to pull in other xsl templates (https://www.w3schools.com/xml/ref_xsl_el_include.asp). Javascript isn't processing the xsl:include imports and the XSLT processor keeps complaining about missing templates.
How do I get Javascript to process xsl:include?
javascript xml xslt
Is that problem specific to IE? Which versions do you target?
– Martin Honnen
Nov 20 at 18:08
I'm on IE 11, but I suspect this is more of an issue with xsl transformations where the xsl references other xsl files, along with how javascript loads them. I took all of the references out and put all the xsl in one giant file and it loaded fine. It works for a POC demo but I'd like to retain the original format if possible, but I'd have to get the files to load properly.
– Joshua Hedges
Nov 21 at 18:20
add a comment |
I'm working with the XSLT code sample on W3Schools: https://www.w3schools.com/xml/xsl_client.asp
I have this section of code working in IE with basic xslt processing...
<!DOCTYPE html>
<html>
<head>
<script>
function loadXMLDoc(filename)
{
if (window.ActiveXObject)
{
xhttp = new ActiveXObject("Msxml2.XMLHTTP");
}
else
{
xhttp = new XMLHttpRequest();
}
xhttp.open("GET", filename, false);
try {xhttp.responseType = "msxml-document"} catch(err) {} // Helping IE11
xhttp.send("");
return xhttp.responseXML;
}
function displayResult()
{
xml = loadXMLDoc("cdcatalog.xml");
xsl = loadXMLDoc("cdcatalog.xsl");
// code for IE
if (window.ActiveXObject || xhttp.responseType == "msxml-document")
{
ex = xml.transformNode(xsl);
document.getElementById("example").innerHTML = ex;
}
}
</script>
</head>
<body onload="displayResult()">
<div id="example" />
</body>
</html>
The problem is the XSL I'm scaling this solution for has a complex structure, so there are several xsl:include statements to pull in other xsl templates (https://www.w3schools.com/xml/ref_xsl_el_include.asp). Javascript isn't processing the xsl:include imports and the XSLT processor keeps complaining about missing templates.
How do I get Javascript to process xsl:include?
javascript xml xslt
I'm working with the XSLT code sample on W3Schools: https://www.w3schools.com/xml/xsl_client.asp
I have this section of code working in IE with basic xslt processing...
<!DOCTYPE html>
<html>
<head>
<script>
function loadXMLDoc(filename)
{
if (window.ActiveXObject)
{
xhttp = new ActiveXObject("Msxml2.XMLHTTP");
}
else
{
xhttp = new XMLHttpRequest();
}
xhttp.open("GET", filename, false);
try {xhttp.responseType = "msxml-document"} catch(err) {} // Helping IE11
xhttp.send("");
return xhttp.responseXML;
}
function displayResult()
{
xml = loadXMLDoc("cdcatalog.xml");
xsl = loadXMLDoc("cdcatalog.xsl");
// code for IE
if (window.ActiveXObject || xhttp.responseType == "msxml-document")
{
ex = xml.transformNode(xsl);
document.getElementById("example").innerHTML = ex;
}
}
</script>
</head>
<body onload="displayResult()">
<div id="example" />
</body>
</html>
The problem is the XSL I'm scaling this solution for has a complex structure, so there are several xsl:include statements to pull in other xsl templates (https://www.w3schools.com/xml/ref_xsl_el_include.asp). Javascript isn't processing the xsl:include imports and the XSLT processor keeps complaining about missing templates.
How do I get Javascript to process xsl:include?
javascript xml xslt
javascript xml xslt
asked Nov 20 at 17:49
Joshua Hedges
478
478
Is that problem specific to IE? Which versions do you target?
– Martin Honnen
Nov 20 at 18:08
I'm on IE 11, but I suspect this is more of an issue with xsl transformations where the xsl references other xsl files, along with how javascript loads them. I took all of the references out and put all the xsl in one giant file and it loaded fine. It works for a POC demo but I'd like to retain the original format if possible, but I'd have to get the files to load properly.
– Joshua Hedges
Nov 21 at 18:20
add a comment |
Is that problem specific to IE? Which versions do you target?
– Martin Honnen
Nov 20 at 18:08
I'm on IE 11, but I suspect this is more of an issue with xsl transformations where the xsl references other xsl files, along with how javascript loads them. I took all of the references out and put all the xsl in one giant file and it loaded fine. It works for a POC demo but I'd like to retain the original format if possible, but I'd have to get the files to load properly.
– Joshua Hedges
Nov 21 at 18:20
Is that problem specific to IE? Which versions do you target?
– Martin Honnen
Nov 20 at 18:08
Is that problem specific to IE? Which versions do you target?
– Martin Honnen
Nov 20 at 18:08
I'm on IE 11, but I suspect this is more of an issue with xsl transformations where the xsl references other xsl files, along with how javascript loads them. I took all of the references out and put all the xsl in one giant file and it loaded fine. It works for a POC demo but I'd like to retain the original format if possible, but I'd have to get the files to load properly.
– Joshua Hedges
Nov 21 at 18:20
I'm on IE 11, but I suspect this is more of an issue with xsl transformations where the xsl references other xsl files, along with how javascript loads them. I took all of the references out and put all the xsl in one giant file and it loaded fine. It works for a POC demo but I'd like to retain the original format if possible, but I'd have to get the files to load properly.
– Joshua Hedges
Nov 21 at 18:20
add a comment |
1 Answer
1
active
oldest
votes
Using XSLT in IE and Edge through Javascript is a bit of a struggle as initially you would do that by using the various MSXML (Microsoft's COM based XML, XPath and XSLT 1.0 software package) components directly with the Microsoft specific new ActiveXObject
. However, to be more compliant with other browsers they have, at least on the surface, moved to support XMLHttpRequest
(in IE and Edge) and XSLTProcessor
(in Edge), but as far as I understand under the hood it is still MSXML 6 that is used, in particular for XSLT.
As MSXML 6 by default sets the property resolveExternals
to false the use of xsl:import
and xsl:include
fails, so to use MSXML 6 programmatically (in the browser you do that with Javascript) you first need to set that property to true to have MSXML then resolve and load external components like imported or included stylesheet modules. Only these properties are not well exposed when you load XML through XMLHttpRequest.
I have unsuccessfully tried to do that without resorting to too much IE specific code with the use of new ActiveXObject
in https://martin-honnen.github.io/xslt/2018/test2018112004.html (code visible at https://github.com/martin-honnen/martin-honnen.github.io/blob/master/xslt/2018/test2018112004.html), however, when I abandon using XMLHttpRequest for IE (respectively where XSLTProcessor is not supported) and directly use all MSXML 6 components with new ActiveXObject
and set the above named property, I get IE 11 on Windows 10 to resolve and use an imported stylesheet through Javascript in https://martin-honnen.github.io/xslt/2018/test2018112005.html (code visible at https://github.com/martin-honnen/martin-honnen.github.io/blob/master/xslt/2018/test2018112005.html). I haven't tried with earlier IE versions, other than trying to use the emulation mode in the IE 11 developer tools, based on that it also works with IE 10 and 9.
So what I do in
function loadDocForXslt(url) {
return new Promise(function(resolve) {
if (typeof XSLTProcessor === 'undefined') {
var doc = new ActiveXObject('Msxml2.DOMDocument.6.0');
doc.setProperty('ResolveExternals', true);
doc.onreadystatechange = function() {
if (doc.readyState === 4) {
resolve(doc);
}
};
doc.load(url);
}
else {
var req = new XMLHttpRequest();
req.open("GET", url);
if (typeof XSLTProcessor === 'undefined') {
try {
req.responseType = 'msxml-document';
}
catch (e) {}
}
req.onload = function() {
resolve(this.responseXML)
}
req.send();
}
});
}
is creating an MSXML 6 XML DOM document with new ActiveXObject('Msxml2.DOMDocument.6.0')
directly and set is property doc.setProperty('ResolveExternals', true);
so enable xsl:import/xsl:include
.
Of course, as with most stuff you do in the browser, the same origin policy by default doesn't allow you to load XML from anything but the same origin your HTML with the script comes from.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53398718%2fhow-do-i-apply-xsl-with-javascript-when-the-xsl-uses-xslinclude%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Using XSLT in IE and Edge through Javascript is a bit of a struggle as initially you would do that by using the various MSXML (Microsoft's COM based XML, XPath and XSLT 1.0 software package) components directly with the Microsoft specific new ActiveXObject
. However, to be more compliant with other browsers they have, at least on the surface, moved to support XMLHttpRequest
(in IE and Edge) and XSLTProcessor
(in Edge), but as far as I understand under the hood it is still MSXML 6 that is used, in particular for XSLT.
As MSXML 6 by default sets the property resolveExternals
to false the use of xsl:import
and xsl:include
fails, so to use MSXML 6 programmatically (in the browser you do that with Javascript) you first need to set that property to true to have MSXML then resolve and load external components like imported or included stylesheet modules. Only these properties are not well exposed when you load XML through XMLHttpRequest.
I have unsuccessfully tried to do that without resorting to too much IE specific code with the use of new ActiveXObject
in https://martin-honnen.github.io/xslt/2018/test2018112004.html (code visible at https://github.com/martin-honnen/martin-honnen.github.io/blob/master/xslt/2018/test2018112004.html), however, when I abandon using XMLHttpRequest for IE (respectively where XSLTProcessor is not supported) and directly use all MSXML 6 components with new ActiveXObject
and set the above named property, I get IE 11 on Windows 10 to resolve and use an imported stylesheet through Javascript in https://martin-honnen.github.io/xslt/2018/test2018112005.html (code visible at https://github.com/martin-honnen/martin-honnen.github.io/blob/master/xslt/2018/test2018112005.html). I haven't tried with earlier IE versions, other than trying to use the emulation mode in the IE 11 developer tools, based on that it also works with IE 10 and 9.
So what I do in
function loadDocForXslt(url) {
return new Promise(function(resolve) {
if (typeof XSLTProcessor === 'undefined') {
var doc = new ActiveXObject('Msxml2.DOMDocument.6.0');
doc.setProperty('ResolveExternals', true);
doc.onreadystatechange = function() {
if (doc.readyState === 4) {
resolve(doc);
}
};
doc.load(url);
}
else {
var req = new XMLHttpRequest();
req.open("GET", url);
if (typeof XSLTProcessor === 'undefined') {
try {
req.responseType = 'msxml-document';
}
catch (e) {}
}
req.onload = function() {
resolve(this.responseXML)
}
req.send();
}
});
}
is creating an MSXML 6 XML DOM document with new ActiveXObject('Msxml2.DOMDocument.6.0')
directly and set is property doc.setProperty('ResolveExternals', true);
so enable xsl:import/xsl:include
.
Of course, as with most stuff you do in the browser, the same origin policy by default doesn't allow you to load XML from anything but the same origin your HTML with the script comes from.
add a comment |
Using XSLT in IE and Edge through Javascript is a bit of a struggle as initially you would do that by using the various MSXML (Microsoft's COM based XML, XPath and XSLT 1.0 software package) components directly with the Microsoft specific new ActiveXObject
. However, to be more compliant with other browsers they have, at least on the surface, moved to support XMLHttpRequest
(in IE and Edge) and XSLTProcessor
(in Edge), but as far as I understand under the hood it is still MSXML 6 that is used, in particular for XSLT.
As MSXML 6 by default sets the property resolveExternals
to false the use of xsl:import
and xsl:include
fails, so to use MSXML 6 programmatically (in the browser you do that with Javascript) you first need to set that property to true to have MSXML then resolve and load external components like imported or included stylesheet modules. Only these properties are not well exposed when you load XML through XMLHttpRequest.
I have unsuccessfully tried to do that without resorting to too much IE specific code with the use of new ActiveXObject
in https://martin-honnen.github.io/xslt/2018/test2018112004.html (code visible at https://github.com/martin-honnen/martin-honnen.github.io/blob/master/xslt/2018/test2018112004.html), however, when I abandon using XMLHttpRequest for IE (respectively where XSLTProcessor is not supported) and directly use all MSXML 6 components with new ActiveXObject
and set the above named property, I get IE 11 on Windows 10 to resolve and use an imported stylesheet through Javascript in https://martin-honnen.github.io/xslt/2018/test2018112005.html (code visible at https://github.com/martin-honnen/martin-honnen.github.io/blob/master/xslt/2018/test2018112005.html). I haven't tried with earlier IE versions, other than trying to use the emulation mode in the IE 11 developer tools, based on that it also works with IE 10 and 9.
So what I do in
function loadDocForXslt(url) {
return new Promise(function(resolve) {
if (typeof XSLTProcessor === 'undefined') {
var doc = new ActiveXObject('Msxml2.DOMDocument.6.0');
doc.setProperty('ResolveExternals', true);
doc.onreadystatechange = function() {
if (doc.readyState === 4) {
resolve(doc);
}
};
doc.load(url);
}
else {
var req = new XMLHttpRequest();
req.open("GET", url);
if (typeof XSLTProcessor === 'undefined') {
try {
req.responseType = 'msxml-document';
}
catch (e) {}
}
req.onload = function() {
resolve(this.responseXML)
}
req.send();
}
});
}
is creating an MSXML 6 XML DOM document with new ActiveXObject('Msxml2.DOMDocument.6.0')
directly and set is property doc.setProperty('ResolveExternals', true);
so enable xsl:import/xsl:include
.
Of course, as with most stuff you do in the browser, the same origin policy by default doesn't allow you to load XML from anything but the same origin your HTML with the script comes from.
add a comment |
Using XSLT in IE and Edge through Javascript is a bit of a struggle as initially you would do that by using the various MSXML (Microsoft's COM based XML, XPath and XSLT 1.0 software package) components directly with the Microsoft specific new ActiveXObject
. However, to be more compliant with other browsers they have, at least on the surface, moved to support XMLHttpRequest
(in IE and Edge) and XSLTProcessor
(in Edge), but as far as I understand under the hood it is still MSXML 6 that is used, in particular for XSLT.
As MSXML 6 by default sets the property resolveExternals
to false the use of xsl:import
and xsl:include
fails, so to use MSXML 6 programmatically (in the browser you do that with Javascript) you first need to set that property to true to have MSXML then resolve and load external components like imported or included stylesheet modules. Only these properties are not well exposed when you load XML through XMLHttpRequest.
I have unsuccessfully tried to do that without resorting to too much IE specific code with the use of new ActiveXObject
in https://martin-honnen.github.io/xslt/2018/test2018112004.html (code visible at https://github.com/martin-honnen/martin-honnen.github.io/blob/master/xslt/2018/test2018112004.html), however, when I abandon using XMLHttpRequest for IE (respectively where XSLTProcessor is not supported) and directly use all MSXML 6 components with new ActiveXObject
and set the above named property, I get IE 11 on Windows 10 to resolve and use an imported stylesheet through Javascript in https://martin-honnen.github.io/xslt/2018/test2018112005.html (code visible at https://github.com/martin-honnen/martin-honnen.github.io/blob/master/xslt/2018/test2018112005.html). I haven't tried with earlier IE versions, other than trying to use the emulation mode in the IE 11 developer tools, based on that it also works with IE 10 and 9.
So what I do in
function loadDocForXslt(url) {
return new Promise(function(resolve) {
if (typeof XSLTProcessor === 'undefined') {
var doc = new ActiveXObject('Msxml2.DOMDocument.6.0');
doc.setProperty('ResolveExternals', true);
doc.onreadystatechange = function() {
if (doc.readyState === 4) {
resolve(doc);
}
};
doc.load(url);
}
else {
var req = new XMLHttpRequest();
req.open("GET", url);
if (typeof XSLTProcessor === 'undefined') {
try {
req.responseType = 'msxml-document';
}
catch (e) {}
}
req.onload = function() {
resolve(this.responseXML)
}
req.send();
}
});
}
is creating an MSXML 6 XML DOM document with new ActiveXObject('Msxml2.DOMDocument.6.0')
directly and set is property doc.setProperty('ResolveExternals', true);
so enable xsl:import/xsl:include
.
Of course, as with most stuff you do in the browser, the same origin policy by default doesn't allow you to load XML from anything but the same origin your HTML with the script comes from.
Using XSLT in IE and Edge through Javascript is a bit of a struggle as initially you would do that by using the various MSXML (Microsoft's COM based XML, XPath and XSLT 1.0 software package) components directly with the Microsoft specific new ActiveXObject
. However, to be more compliant with other browsers they have, at least on the surface, moved to support XMLHttpRequest
(in IE and Edge) and XSLTProcessor
(in Edge), but as far as I understand under the hood it is still MSXML 6 that is used, in particular for XSLT.
As MSXML 6 by default sets the property resolveExternals
to false the use of xsl:import
and xsl:include
fails, so to use MSXML 6 programmatically (in the browser you do that with Javascript) you first need to set that property to true to have MSXML then resolve and load external components like imported or included stylesheet modules. Only these properties are not well exposed when you load XML through XMLHttpRequest.
I have unsuccessfully tried to do that without resorting to too much IE specific code with the use of new ActiveXObject
in https://martin-honnen.github.io/xslt/2018/test2018112004.html (code visible at https://github.com/martin-honnen/martin-honnen.github.io/blob/master/xslt/2018/test2018112004.html), however, when I abandon using XMLHttpRequest for IE (respectively where XSLTProcessor is not supported) and directly use all MSXML 6 components with new ActiveXObject
and set the above named property, I get IE 11 on Windows 10 to resolve and use an imported stylesheet through Javascript in https://martin-honnen.github.io/xslt/2018/test2018112005.html (code visible at https://github.com/martin-honnen/martin-honnen.github.io/blob/master/xslt/2018/test2018112005.html). I haven't tried with earlier IE versions, other than trying to use the emulation mode in the IE 11 developer tools, based on that it also works with IE 10 and 9.
So what I do in
function loadDocForXslt(url) {
return new Promise(function(resolve) {
if (typeof XSLTProcessor === 'undefined') {
var doc = new ActiveXObject('Msxml2.DOMDocument.6.0');
doc.setProperty('ResolveExternals', true);
doc.onreadystatechange = function() {
if (doc.readyState === 4) {
resolve(doc);
}
};
doc.load(url);
}
else {
var req = new XMLHttpRequest();
req.open("GET", url);
if (typeof XSLTProcessor === 'undefined') {
try {
req.responseType = 'msxml-document';
}
catch (e) {}
}
req.onload = function() {
resolve(this.responseXML)
}
req.send();
}
});
}
is creating an MSXML 6 XML DOM document with new ActiveXObject('Msxml2.DOMDocument.6.0')
directly and set is property doc.setProperty('ResolveExternals', true);
so enable xsl:import/xsl:include
.
Of course, as with most stuff you do in the browser, the same origin policy by default doesn't allow you to load XML from anything but the same origin your HTML with the script comes from.
answered Nov 22 at 6:08
Martin Honnen
110k65876
110k65876
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53398718%2fhow-do-i-apply-xsl-with-javascript-when-the-xsl-uses-xslinclude%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
QRWPxw4w8,fO4Zl,chljY1cc26
Is that problem specific to IE? Which versions do you target?
– Martin Honnen
Nov 20 at 18:08
I'm on IE 11, but I suspect this is more of an issue with xsl transformations where the xsl references other xsl files, along with how javascript loads them. I took all of the references out and put all the xsl in one giant file and it loaded fine. It works for a POC demo but I'd like to retain the original format if possible, but I'd have to get the files to load properly.
– Joshua Hedges
Nov 21 at 18:20