Invalid prop `x` of type `string` - expected `object` + 'y' of type `string` - expected `array`
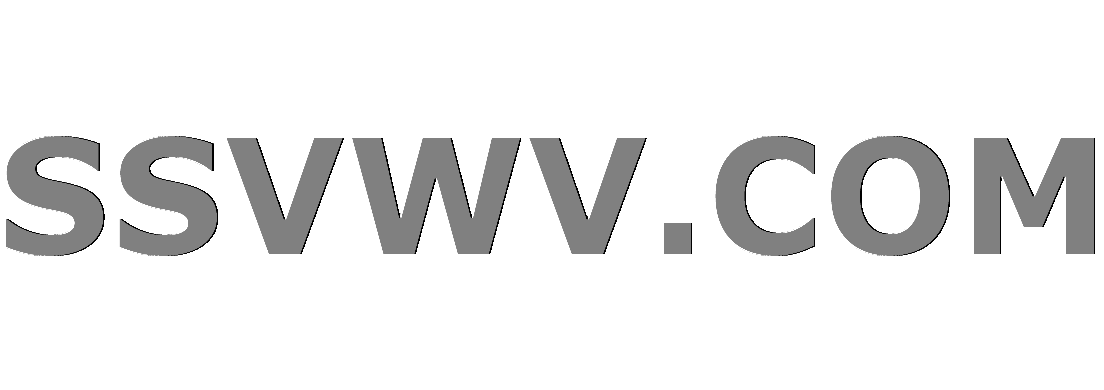
Multi tool use
Tried to search for an answer but did not found matching one. I have a problem with my simple React app (contactForm + contacts list). Locally app is not rendering and I got two alerts:
"react.js:20478 Warning: Failed prop type: Invalid prop
contact
of typestring
supplied toContactForm
, expectedobject
.
in ContactForm (created by App)
in App"
and
react.js:20478 Warning: Failed prop type: Invalid prop
items
of typestring
supplied toContacts
, expectedarray
.
in Contacts (created by App)
in App
What is interesting, alerts shows only locally (I run app with http server). When I upload code on GitHub and run via GitHub Pages (link in repository) - app is rendering and none of alerts occurrs. Can somebody explain this to me?
Files structure - script.js (only rendering App) and components: App, Contact (single contact), Contacts (list of contacts) and ContactForm.
App:
var contacts = [
{
id: 1,
firstName: 'Jan',
lastName: 'Nowak',
email: 'jan.nowak@example.com',
}
...
];
var contactForm = {
firstName: '',
lastName: '',
email: ''
};
var App = React.createClass({
render: function() {
return (
<div className='app'>
<ContactForm contact={contactForm}/>
<Contacts items={contacts}/>
</div>
);
}
});
Contact:
var Contact = React.createClass({
propTypes: {
item: React.PropTypes.object.isRequired,
},
render: function() {
return (
<div className={'contactItem col-lg-6 col-md-6 col-sm-12'}>
<div className={'contactImg col-lg-4 col-md-4 col-sm-4'}>
<img className={'contactImage'} src={'http://icons.veryicon.com/png/System/gCons/contacts.png'}/>
</div>
<div className={'contactItem col-lg-6 col-md-6 col-sm-12'}>
<p className={'contactLabel'}>Imię: {this.props.item.firstName}</p>
<p className={'contactLabel'}>Nazwisko: {this.props.item.lastName}</p>
<a className={'contactEmail'} href={'mailto:'+this.props.item.email}>{this.props.item.email}</a>
</div>
</div>
)
},
});
Contacts:
var Contacts = React.createClass({
propTypes: {
items: React.PropTypes.array.isRequired,
},
render: function() {
var contacts = this.props.items.map(function(contact) {
return <Contact item={contact} key={contact.id}/>
});
return <ul className={'contactList'}>{contacts}</ul>
}
});
ContactForm:
var ContactForm = React.createClass({
propTypes: {
contact: React.PropTypes.object.isRequired
},
render: function() {
return (
<form className={'contactForm'}>
<input type={'text'} placeholder={'Imię'} value={this.props.contact.firstName}/>
<input type={'text'} placeholder={'Nazwisko'} value={this.props.contact.lastName}/>
<input type={'email'} placeholder={'E-mail'} value={this.props.contact.email}/>
<button type={'submit'}>Dodaj kontakt</button>
</form>
)
},
})
Link to the full code:
https://github.com/galdranorn/react-exercises
javascript reactjs react-proptypes
add a comment |
Tried to search for an answer but did not found matching one. I have a problem with my simple React app (contactForm + contacts list). Locally app is not rendering and I got two alerts:
"react.js:20478 Warning: Failed prop type: Invalid prop
contact
of typestring
supplied toContactForm
, expectedobject
.
in ContactForm (created by App)
in App"
and
react.js:20478 Warning: Failed prop type: Invalid prop
items
of typestring
supplied toContacts
, expectedarray
.
in Contacts (created by App)
in App
What is interesting, alerts shows only locally (I run app with http server). When I upload code on GitHub and run via GitHub Pages (link in repository) - app is rendering and none of alerts occurrs. Can somebody explain this to me?
Files structure - script.js (only rendering App) and components: App, Contact (single contact), Contacts (list of contacts) and ContactForm.
App:
var contacts = [
{
id: 1,
firstName: 'Jan',
lastName: 'Nowak',
email: 'jan.nowak@example.com',
}
...
];
var contactForm = {
firstName: '',
lastName: '',
email: ''
};
var App = React.createClass({
render: function() {
return (
<div className='app'>
<ContactForm contact={contactForm}/>
<Contacts items={contacts}/>
</div>
);
}
});
Contact:
var Contact = React.createClass({
propTypes: {
item: React.PropTypes.object.isRequired,
},
render: function() {
return (
<div className={'contactItem col-lg-6 col-md-6 col-sm-12'}>
<div className={'contactImg col-lg-4 col-md-4 col-sm-4'}>
<img className={'contactImage'} src={'http://icons.veryicon.com/png/System/gCons/contacts.png'}/>
</div>
<div className={'contactItem col-lg-6 col-md-6 col-sm-12'}>
<p className={'contactLabel'}>Imię: {this.props.item.firstName}</p>
<p className={'contactLabel'}>Nazwisko: {this.props.item.lastName}</p>
<a className={'contactEmail'} href={'mailto:'+this.props.item.email}>{this.props.item.email}</a>
</div>
</div>
)
},
});
Contacts:
var Contacts = React.createClass({
propTypes: {
items: React.PropTypes.array.isRequired,
},
render: function() {
var contacts = this.props.items.map(function(contact) {
return <Contact item={contact} key={contact.id}/>
});
return <ul className={'contactList'}>{contacts}</ul>
}
});
ContactForm:
var ContactForm = React.createClass({
propTypes: {
contact: React.PropTypes.object.isRequired
},
render: function() {
return (
<form className={'contactForm'}>
<input type={'text'} placeholder={'Imię'} value={this.props.contact.firstName}/>
<input type={'text'} placeholder={'Nazwisko'} value={this.props.contact.lastName}/>
<input type={'email'} placeholder={'E-mail'} value={this.props.contact.email}/>
<button type={'submit'}>Dodaj kontakt</button>
</form>
)
},
})
Link to the full code:
https://github.com/galdranorn/react-exercises
javascript reactjs react-proptypes
1
Please provide the relevant code in the question itself. For further help, see this help section
– Bhojendra Rauniyar
Nov 20 at 17:51
@BhojendraRauniyar Thanks for your remarks, did as requested.
– galdranorn
Nov 20 at 18:22
add a comment |
Tried to search for an answer but did not found matching one. I have a problem with my simple React app (contactForm + contacts list). Locally app is not rendering and I got two alerts:
"react.js:20478 Warning: Failed prop type: Invalid prop
contact
of typestring
supplied toContactForm
, expectedobject
.
in ContactForm (created by App)
in App"
and
react.js:20478 Warning: Failed prop type: Invalid prop
items
of typestring
supplied toContacts
, expectedarray
.
in Contacts (created by App)
in App
What is interesting, alerts shows only locally (I run app with http server). When I upload code on GitHub and run via GitHub Pages (link in repository) - app is rendering and none of alerts occurrs. Can somebody explain this to me?
Files structure - script.js (only rendering App) and components: App, Contact (single contact), Contacts (list of contacts) and ContactForm.
App:
var contacts = [
{
id: 1,
firstName: 'Jan',
lastName: 'Nowak',
email: 'jan.nowak@example.com',
}
...
];
var contactForm = {
firstName: '',
lastName: '',
email: ''
};
var App = React.createClass({
render: function() {
return (
<div className='app'>
<ContactForm contact={contactForm}/>
<Contacts items={contacts}/>
</div>
);
}
});
Contact:
var Contact = React.createClass({
propTypes: {
item: React.PropTypes.object.isRequired,
},
render: function() {
return (
<div className={'contactItem col-lg-6 col-md-6 col-sm-12'}>
<div className={'contactImg col-lg-4 col-md-4 col-sm-4'}>
<img className={'contactImage'} src={'http://icons.veryicon.com/png/System/gCons/contacts.png'}/>
</div>
<div className={'contactItem col-lg-6 col-md-6 col-sm-12'}>
<p className={'contactLabel'}>Imię: {this.props.item.firstName}</p>
<p className={'contactLabel'}>Nazwisko: {this.props.item.lastName}</p>
<a className={'contactEmail'} href={'mailto:'+this.props.item.email}>{this.props.item.email}</a>
</div>
</div>
)
},
});
Contacts:
var Contacts = React.createClass({
propTypes: {
items: React.PropTypes.array.isRequired,
},
render: function() {
var contacts = this.props.items.map(function(contact) {
return <Contact item={contact} key={contact.id}/>
});
return <ul className={'contactList'}>{contacts}</ul>
}
});
ContactForm:
var ContactForm = React.createClass({
propTypes: {
contact: React.PropTypes.object.isRequired
},
render: function() {
return (
<form className={'contactForm'}>
<input type={'text'} placeholder={'Imię'} value={this.props.contact.firstName}/>
<input type={'text'} placeholder={'Nazwisko'} value={this.props.contact.lastName}/>
<input type={'email'} placeholder={'E-mail'} value={this.props.contact.email}/>
<button type={'submit'}>Dodaj kontakt</button>
</form>
)
},
})
Link to the full code:
https://github.com/galdranorn/react-exercises
javascript reactjs react-proptypes
Tried to search for an answer but did not found matching one. I have a problem with my simple React app (contactForm + contacts list). Locally app is not rendering and I got two alerts:
"react.js:20478 Warning: Failed prop type: Invalid prop
contact
of typestring
supplied toContactForm
, expectedobject
.
in ContactForm (created by App)
in App"
and
react.js:20478 Warning: Failed prop type: Invalid prop
items
of typestring
supplied toContacts
, expectedarray
.
in Contacts (created by App)
in App
What is interesting, alerts shows only locally (I run app with http server). When I upload code on GitHub and run via GitHub Pages (link in repository) - app is rendering and none of alerts occurrs. Can somebody explain this to me?
Files structure - script.js (only rendering App) and components: App, Contact (single contact), Contacts (list of contacts) and ContactForm.
App:
var contacts = [
{
id: 1,
firstName: 'Jan',
lastName: 'Nowak',
email: 'jan.nowak@example.com',
}
...
];
var contactForm = {
firstName: '',
lastName: '',
email: ''
};
var App = React.createClass({
render: function() {
return (
<div className='app'>
<ContactForm contact={contactForm}/>
<Contacts items={contacts}/>
</div>
);
}
});
Contact:
var Contact = React.createClass({
propTypes: {
item: React.PropTypes.object.isRequired,
},
render: function() {
return (
<div className={'contactItem col-lg-6 col-md-6 col-sm-12'}>
<div className={'contactImg col-lg-4 col-md-4 col-sm-4'}>
<img className={'contactImage'} src={'http://icons.veryicon.com/png/System/gCons/contacts.png'}/>
</div>
<div className={'contactItem col-lg-6 col-md-6 col-sm-12'}>
<p className={'contactLabel'}>Imię: {this.props.item.firstName}</p>
<p className={'contactLabel'}>Nazwisko: {this.props.item.lastName}</p>
<a className={'contactEmail'} href={'mailto:'+this.props.item.email}>{this.props.item.email}</a>
</div>
</div>
)
},
});
Contacts:
var Contacts = React.createClass({
propTypes: {
items: React.PropTypes.array.isRequired,
},
render: function() {
var contacts = this.props.items.map(function(contact) {
return <Contact item={contact} key={contact.id}/>
});
return <ul className={'contactList'}>{contacts}</ul>
}
});
ContactForm:
var ContactForm = React.createClass({
propTypes: {
contact: React.PropTypes.object.isRequired
},
render: function() {
return (
<form className={'contactForm'}>
<input type={'text'} placeholder={'Imię'} value={this.props.contact.firstName}/>
<input type={'text'} placeholder={'Nazwisko'} value={this.props.contact.lastName}/>
<input type={'email'} placeholder={'E-mail'} value={this.props.contact.email}/>
<button type={'submit'}>Dodaj kontakt</button>
</form>
)
},
})
Link to the full code:
https://github.com/galdranorn/react-exercises
javascript reactjs react-proptypes
javascript reactjs react-proptypes
edited Nov 20 at 18:21
asked Nov 20 at 17:48
galdranorn
13
13
1
Please provide the relevant code in the question itself. For further help, see this help section
– Bhojendra Rauniyar
Nov 20 at 17:51
@BhojendraRauniyar Thanks for your remarks, did as requested.
– galdranorn
Nov 20 at 18:22
add a comment |
1
Please provide the relevant code in the question itself. For further help, see this help section
– Bhojendra Rauniyar
Nov 20 at 17:51
@BhojendraRauniyar Thanks for your remarks, did as requested.
– galdranorn
Nov 20 at 18:22
1
1
Please provide the relevant code in the question itself. For further help, see this help section
– Bhojendra Rauniyar
Nov 20 at 17:51
Please provide the relevant code in the question itself. For further help, see this help section
– Bhojendra Rauniyar
Nov 20 at 17:51
@BhojendraRauniyar Thanks for your remarks, did as requested.
– galdranorn
Nov 20 at 18:22
@BhojendraRauniyar Thanks for your remarks, did as requested.
– galdranorn
Nov 20 at 18:22
add a comment |
2 Answers
2
active
oldest
votes
@galdranon: I checked out your code and am not getting any errors.
Hm.. interesting. Thanks for your feedback.
– galdranorn
Nov 21 at 9:27
add a comment |
Problem solved: code was OK, but I had cashe issue. Cleaning the browser/ctrl+F5 habit solves the problem.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53398713%2finvalid-prop-x-of-type-string-expected-object-y-of-type-string-e%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
@galdranon: I checked out your code and am not getting any errors.
Hm.. interesting. Thanks for your feedback.
– galdranorn
Nov 21 at 9:27
add a comment |
@galdranon: I checked out your code and am not getting any errors.
Hm.. interesting. Thanks for your feedback.
– galdranorn
Nov 21 at 9:27
add a comment |
@galdranon: I checked out your code and am not getting any errors.
@galdranon: I checked out your code and am not getting any errors.
answered Nov 20 at 19:10
shmit
44239
44239
Hm.. interesting. Thanks for your feedback.
– galdranorn
Nov 21 at 9:27
add a comment |
Hm.. interesting. Thanks for your feedback.
– galdranorn
Nov 21 at 9:27
Hm.. interesting. Thanks for your feedback.
– galdranorn
Nov 21 at 9:27
Hm.. interesting. Thanks for your feedback.
– galdranorn
Nov 21 at 9:27
add a comment |
Problem solved: code was OK, but I had cashe issue. Cleaning the browser/ctrl+F5 habit solves the problem.
add a comment |
Problem solved: code was OK, but I had cashe issue. Cleaning the browser/ctrl+F5 habit solves the problem.
add a comment |
Problem solved: code was OK, but I had cashe issue. Cleaning the browser/ctrl+F5 habit solves the problem.
Problem solved: code was OK, but I had cashe issue. Cleaning the browser/ctrl+F5 habit solves the problem.
answered Nov 29 at 18:28
galdranorn
13
13
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53398713%2finvalid-prop-x-of-type-string-expected-object-y-of-type-string-e%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
W3dW4R6iTX5JpHs fx7uoTaLtSA2H
1
Please provide the relevant code in the question itself. For further help, see this help section
– Bhojendra Rauniyar
Nov 20 at 17:51
@BhojendraRauniyar Thanks for your remarks, did as requested.
– galdranorn
Nov 20 at 18:22