Saving Django Model with OneToOne Relationship Field returns Object has no attribute 'id' error
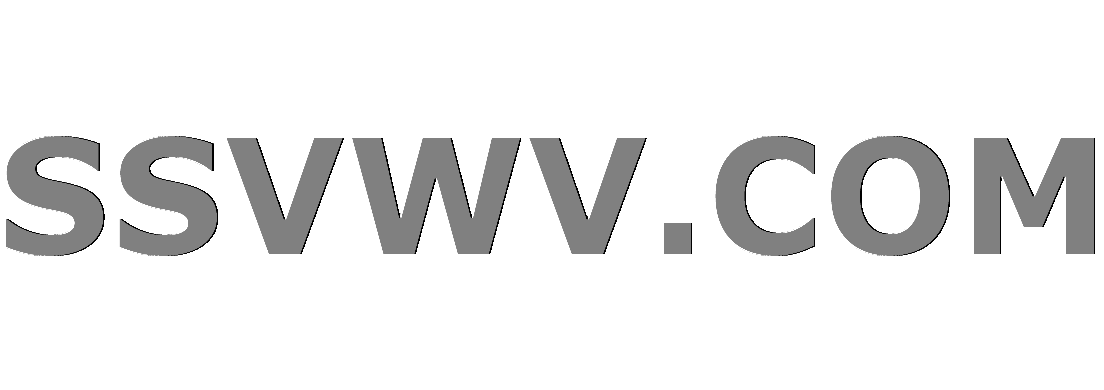
Multi tool use
up vote
0
down vote
favorite
I have defined OneToOne Relationship in model b with model a. When i try to save model b i get "Model B : Object has no attribute 'id' " error. How to resolve this issue? What am i missing here.
Models:
class ModelA:
invoicedate = models.DateTimeField(blank=True)
amount = models.DecimalField(max_digits=12, decimal_places=2)
status = models.CharField(max_length=20, default='ACTIVE')
class Meta:
db_table = "modela"
class ModelB:
rel = models.OneToOneField(ModelA, on_delete=models.CASCADE, primary_key=True)
field1 ...
class Meta:
db_table = "modelb"
Method to insert data into models:
@transaction.atomic
def methodToInsertData(args):
new_modela = ModelA(
"invoicedate" = "2018-11-05",
"amount" = 5000,
"status" = "ACTIVE"
)
new_modela.save()
new_modelb = ModelB(
rel = new_modela,
...
)
new_modelb.save() #This statement throws "object has no attribute id" error
ModelA will auto create id as primary key but model B will not have id as primary key instead rel field would be the primary key with OneToOne relationship to Model A.
Any suggestions to resolve this issue would be highly appreciated.
django django-models
add a comment |
up vote
0
down vote
favorite
I have defined OneToOne Relationship in model b with model a. When i try to save model b i get "Model B : Object has no attribute 'id' " error. How to resolve this issue? What am i missing here.
Models:
class ModelA:
invoicedate = models.DateTimeField(blank=True)
amount = models.DecimalField(max_digits=12, decimal_places=2)
status = models.CharField(max_length=20, default='ACTIVE')
class Meta:
db_table = "modela"
class ModelB:
rel = models.OneToOneField(ModelA, on_delete=models.CASCADE, primary_key=True)
field1 ...
class Meta:
db_table = "modelb"
Method to insert data into models:
@transaction.atomic
def methodToInsertData(args):
new_modela = ModelA(
"invoicedate" = "2018-11-05",
"amount" = 5000,
"status" = "ACTIVE"
)
new_modela.save()
new_modelb = ModelB(
rel = new_modela,
...
)
new_modelb.save() #This statement throws "object has no attribute id" error
ModelA will auto create id as primary key but model B will not have id as primary key instead rel field would be the primary key with OneToOne relationship to Model A.
Any suggestions to resolve this issue would be highly appreciated.
django django-models
according to your code, you should not be able to save ModelA, you should have facedSyntaxError: keyword can't be an expression
error
– ruddra
Nov 20 at 6:35
That was just a reference for a date field. I have edited it to InvoiceDate now.
– RajKrishnan
Nov 20 at 8:27
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have defined OneToOne Relationship in model b with model a. When i try to save model b i get "Model B : Object has no attribute 'id' " error. How to resolve this issue? What am i missing here.
Models:
class ModelA:
invoicedate = models.DateTimeField(blank=True)
amount = models.DecimalField(max_digits=12, decimal_places=2)
status = models.CharField(max_length=20, default='ACTIVE')
class Meta:
db_table = "modela"
class ModelB:
rel = models.OneToOneField(ModelA, on_delete=models.CASCADE, primary_key=True)
field1 ...
class Meta:
db_table = "modelb"
Method to insert data into models:
@transaction.atomic
def methodToInsertData(args):
new_modela = ModelA(
"invoicedate" = "2018-11-05",
"amount" = 5000,
"status" = "ACTIVE"
)
new_modela.save()
new_modelb = ModelB(
rel = new_modela,
...
)
new_modelb.save() #This statement throws "object has no attribute id" error
ModelA will auto create id as primary key but model B will not have id as primary key instead rel field would be the primary key with OneToOne relationship to Model A.
Any suggestions to resolve this issue would be highly appreciated.
django django-models
I have defined OneToOne Relationship in model b with model a. When i try to save model b i get "Model B : Object has no attribute 'id' " error. How to resolve this issue? What am i missing here.
Models:
class ModelA:
invoicedate = models.DateTimeField(blank=True)
amount = models.DecimalField(max_digits=12, decimal_places=2)
status = models.CharField(max_length=20, default='ACTIVE')
class Meta:
db_table = "modela"
class ModelB:
rel = models.OneToOneField(ModelA, on_delete=models.CASCADE, primary_key=True)
field1 ...
class Meta:
db_table = "modelb"
Method to insert data into models:
@transaction.atomic
def methodToInsertData(args):
new_modela = ModelA(
"invoicedate" = "2018-11-05",
"amount" = 5000,
"status" = "ACTIVE"
)
new_modela.save()
new_modelb = ModelB(
rel = new_modela,
...
)
new_modelb.save() #This statement throws "object has no attribute id" error
ModelA will auto create id as primary key but model B will not have id as primary key instead rel field would be the primary key with OneToOne relationship to Model A.
Any suggestions to resolve this issue would be highly appreciated.
django django-models
django django-models
edited Nov 20 at 8:26
asked Nov 20 at 6:30
RajKrishnan
77
77
according to your code, you should not be able to save ModelA, you should have facedSyntaxError: keyword can't be an expression
error
– ruddra
Nov 20 at 6:35
That was just a reference for a date field. I have edited it to InvoiceDate now.
– RajKrishnan
Nov 20 at 8:27
add a comment |
according to your code, you should not be able to save ModelA, you should have facedSyntaxError: keyword can't be an expression
error
– ruddra
Nov 20 at 6:35
That was just a reference for a date field. I have edited it to InvoiceDate now.
– RajKrishnan
Nov 20 at 8:27
according to your code, you should not be able to save ModelA, you should have faced
SyntaxError: keyword can't be an expression
error– ruddra
Nov 20 at 6:35
according to your code, you should not be able to save ModelA, you should have faced
SyntaxError: keyword can't be an expression
error– ruddra
Nov 20 at 6:35
That was just a reference for a date field. I have edited it to InvoiceDate now.
– RajKrishnan
Nov 20 at 8:27
That was just a reference for a date field. I have edited it to InvoiceDate now.
– RajKrishnan
Nov 20 at 8:27
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
It may be that you set the OneToOneField as the model's primary key. Try removing primary_key=True and either explicitly setting another field (uuid or integer) as pk, or simply allowing the model to set its own id.
rel = models.OneToOneField(ModelA, on_delete=models.CASCADE)
Model B's rel is an extension of Model A and rel key must be a primary key.
– RajKrishnan
Nov 21 at 12:00
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
It may be that you set the OneToOneField as the model's primary key. Try removing primary_key=True and either explicitly setting another field (uuid or integer) as pk, or simply allowing the model to set its own id.
rel = models.OneToOneField(ModelA, on_delete=models.CASCADE)
Model B's rel is an extension of Model A and rel key must be a primary key.
– RajKrishnan
Nov 21 at 12:00
add a comment |
up vote
0
down vote
It may be that you set the OneToOneField as the model's primary key. Try removing primary_key=True and either explicitly setting another field (uuid or integer) as pk, or simply allowing the model to set its own id.
rel = models.OneToOneField(ModelA, on_delete=models.CASCADE)
Model B's rel is an extension of Model A and rel key must be a primary key.
– RajKrishnan
Nov 21 at 12:00
add a comment |
up vote
0
down vote
up vote
0
down vote
It may be that you set the OneToOneField as the model's primary key. Try removing primary_key=True and either explicitly setting another field (uuid or integer) as pk, or simply allowing the model to set its own id.
rel = models.OneToOneField(ModelA, on_delete=models.CASCADE)
It may be that you set the OneToOneField as the model's primary key. Try removing primary_key=True and either explicitly setting another field (uuid or integer) as pk, or simply allowing the model to set its own id.
rel = models.OneToOneField(ModelA, on_delete=models.CASCADE)
answered Nov 20 at 6:46
Whodini
37713
37713
Model B's rel is an extension of Model A and rel key must be a primary key.
– RajKrishnan
Nov 21 at 12:00
add a comment |
Model B's rel is an extension of Model A and rel key must be a primary key.
– RajKrishnan
Nov 21 at 12:00
Model B's rel is an extension of Model A and rel key must be a primary key.
– RajKrishnan
Nov 21 at 12:00
Model B's rel is an extension of Model A and rel key must be a primary key.
– RajKrishnan
Nov 21 at 12:00
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53387443%2fsaving-django-model-with-onetoone-relationship-field-returns-object-has-no-attri%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
SVcwEnucTaKS,VROByX4t w OltQ9s,18VKZeRh5HIJfK,OUNzuq504I4VOn x EKPz7qjJ szJ7bc,K37Bkmy6B5 Y
according to your code, you should not be able to save ModelA, you should have faced
SyntaxError: keyword can't be an expression
error– ruddra
Nov 20 at 6:35
That was just a reference for a date field. I have edited it to InvoiceDate now.
– RajKrishnan
Nov 20 at 8:27