How to select following element in selenium?
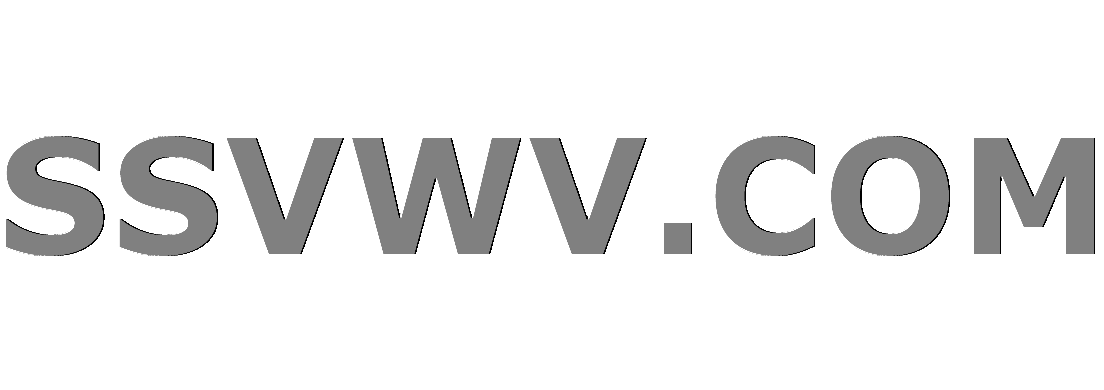
Multi tool use
I have a div which contains a input with an identifier as id and a label in it. with my selenium method i can easily select the input as a webElement but how can i get the label webElement via the Input WebElement?
<div class="item-selectable small-2 radio-item columns xsmall-12 item-selectable-stacked">
<input id="0-3" name="RatingQuestions[0].Score" type="radio" value="3" checked="">
<label for="0-3">
</label>
</div>
This is the method in selenium to select the webElement, whcih currently selects only the input and not like it should the label.
public void RateQuestion(int questionnumber, int rating)
{
string questionid = questionnumber.ToString() + "-" + rating.ToString();
IWebElement RateQuestionElement = this.Driver.FindElement(By.Id(questionid));
RateQuestionElement.Click();
}
The issue is that only the label is clickable and therefore i need to select the label from the input field, how can i do this?
c# selenium selenium-webdriver xpath
add a comment |
I have a div which contains a input with an identifier as id and a label in it. with my selenium method i can easily select the input as a webElement but how can i get the label webElement via the Input WebElement?
<div class="item-selectable small-2 radio-item columns xsmall-12 item-selectable-stacked">
<input id="0-3" name="RatingQuestions[0].Score" type="radio" value="3" checked="">
<label for="0-3">
</label>
</div>
This is the method in selenium to select the webElement, whcih currently selects only the input and not like it should the label.
public void RateQuestion(int questionnumber, int rating)
{
string questionid = questionnumber.ToString() + "-" + rating.ToString();
IWebElement RateQuestionElement = this.Driver.FindElement(By.Id(questionid));
RateQuestionElement.Click();
}
The issue is that only the label is clickable and therefore i need to select the label from the input field, how can i do this?
c# selenium selenium-webdriver xpath
Can you share your current code please? You should ask your question as minimal, complete, verifiable example
– Emre Savcı
Nov 26 '18 at 8:20
I've added my selenium selector code
– Peter K
Nov 26 '18 at 8:37
@Simon.B Why do you need to select the label from the input field? What is your usecase? Access the<input>
or the<label>
?
– DebanjanB
Nov 26 '18 at 9:21
add a comment |
I have a div which contains a input with an identifier as id and a label in it. with my selenium method i can easily select the input as a webElement but how can i get the label webElement via the Input WebElement?
<div class="item-selectable small-2 radio-item columns xsmall-12 item-selectable-stacked">
<input id="0-3" name="RatingQuestions[0].Score" type="radio" value="3" checked="">
<label for="0-3">
</label>
</div>
This is the method in selenium to select the webElement, whcih currently selects only the input and not like it should the label.
public void RateQuestion(int questionnumber, int rating)
{
string questionid = questionnumber.ToString() + "-" + rating.ToString();
IWebElement RateQuestionElement = this.Driver.FindElement(By.Id(questionid));
RateQuestionElement.Click();
}
The issue is that only the label is clickable and therefore i need to select the label from the input field, how can i do this?
c# selenium selenium-webdriver xpath
I have a div which contains a input with an identifier as id and a label in it. with my selenium method i can easily select the input as a webElement but how can i get the label webElement via the Input WebElement?
<div class="item-selectable small-2 radio-item columns xsmall-12 item-selectable-stacked">
<input id="0-3" name="RatingQuestions[0].Score" type="radio" value="3" checked="">
<label for="0-3">
</label>
</div>
This is the method in selenium to select the webElement, whcih currently selects only the input and not like it should the label.
public void RateQuestion(int questionnumber, int rating)
{
string questionid = questionnumber.ToString() + "-" + rating.ToString();
IWebElement RateQuestionElement = this.Driver.FindElement(By.Id(questionid));
RateQuestionElement.Click();
}
The issue is that only the label is clickable and therefore i need to select the label from the input field, how can i do this?
c# selenium selenium-webdriver xpath
c# selenium selenium-webdriver xpath
edited Nov 26 '18 at 9:30


DebanjanB
45.9k134688
45.9k134688
asked Nov 26 '18 at 8:19
Peter KPeter K
277
277
Can you share your current code please? You should ask your question as minimal, complete, verifiable example
– Emre Savcı
Nov 26 '18 at 8:20
I've added my selenium selector code
– Peter K
Nov 26 '18 at 8:37
@Simon.B Why do you need to select the label from the input field? What is your usecase? Access the<input>
or the<label>
?
– DebanjanB
Nov 26 '18 at 9:21
add a comment |
Can you share your current code please? You should ask your question as minimal, complete, verifiable example
– Emre Savcı
Nov 26 '18 at 8:20
I've added my selenium selector code
– Peter K
Nov 26 '18 at 8:37
@Simon.B Why do you need to select the label from the input field? What is your usecase? Access the<input>
or the<label>
?
– DebanjanB
Nov 26 '18 at 9:21
Can you share your current code please? You should ask your question as minimal, complete, verifiable example
– Emre Savcı
Nov 26 '18 at 8:20
Can you share your current code please? You should ask your question as minimal, complete, verifiable example
– Emre Savcı
Nov 26 '18 at 8:20
I've added my selenium selector code
– Peter K
Nov 26 '18 at 8:37
I've added my selenium selector code
– Peter K
Nov 26 '18 at 8:37
@Simon.B Why do you need to select the label from the input field? What is your usecase? Access the
<input>
or the <label>
?– DebanjanB
Nov 26 '18 at 9:21
@Simon.B Why do you need to select the label from the input field? What is your usecase? Access the
<input>
or the <label>
?– DebanjanB
Nov 26 '18 at 9:21
add a comment |
3 Answers
3
active
oldest
votes
No, you don't invoke click()
on <label>
nodes but on <input>
nodes.
As per the HTML to invoke click()
on the <input>
tag with respect to the <label>
tag you can use the following solution:
public void RateQuestion(int questionnumber, int rating)
{
string questionid = questionnumber.ToString() + "-" + rating.ToString();
IWebElement RateQuestionElement = this.Driver.FindElement(By.XPath("//label[@for='" + questionid + "']"));
RateQuestionElement.Click();
}
add a comment |
Try this:
public void RateQuestion(int questionnumber, int rating)
{
string questionid = questionnumber.ToString() + "-" + rating.ToString();
IWebElement RateQuestionElement = this.Driver.FindElement(By.Id(questionid)).FindElement(By.Xpath("./../../label"));
RateQuestionElement.Click();
}
Or this:
public void RateQuestion(int questionnumber, int rating)
{
string questionid = questionnumber.ToString() + "-" + rating.ToString();
IWebElement RateQuestionElement = this.FindElement(By.Xpath("//*[contains(local-name(), 'label') and contains(@for, questionid)]"));
RateQuestionElement.Click();
}
The latter one - I'm not that good with C#, so perhaps you'll have to put {questionid}
there, rather than just questionid
.
add a comment |
There are some options:
- After you select the element with questionId, the you can continue further to select the label.
e.g:
IWebElement RateQuestionElement = this.Driver.FindElement(By.Id(questionid));
IWebElement RateQuestionLabel = RateQuestionElement.FindElement(By.Css("label"));
RateQuestionLabel.click();
or
- You can use CssSelector
e.g:
myCssSelector = "#myQuestionId label"
IWebElement RateQuestionElement = this.Driver.FindElement(By.Css(myCssSelector));
RateQuestionElement.click();
I have not tried to execute it myself, but the concept is there
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53477055%2fhow-to-select-following-element-in-selenium%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
No, you don't invoke click()
on <label>
nodes but on <input>
nodes.
As per the HTML to invoke click()
on the <input>
tag with respect to the <label>
tag you can use the following solution:
public void RateQuestion(int questionnumber, int rating)
{
string questionid = questionnumber.ToString() + "-" + rating.ToString();
IWebElement RateQuestionElement = this.Driver.FindElement(By.XPath("//label[@for='" + questionid + "']"));
RateQuestionElement.Click();
}
add a comment |
No, you don't invoke click()
on <label>
nodes but on <input>
nodes.
As per the HTML to invoke click()
on the <input>
tag with respect to the <label>
tag you can use the following solution:
public void RateQuestion(int questionnumber, int rating)
{
string questionid = questionnumber.ToString() + "-" + rating.ToString();
IWebElement RateQuestionElement = this.Driver.FindElement(By.XPath("//label[@for='" + questionid + "']"));
RateQuestionElement.Click();
}
add a comment |
No, you don't invoke click()
on <label>
nodes but on <input>
nodes.
As per the HTML to invoke click()
on the <input>
tag with respect to the <label>
tag you can use the following solution:
public void RateQuestion(int questionnumber, int rating)
{
string questionid = questionnumber.ToString() + "-" + rating.ToString();
IWebElement RateQuestionElement = this.Driver.FindElement(By.XPath("//label[@for='" + questionid + "']"));
RateQuestionElement.Click();
}
No, you don't invoke click()
on <label>
nodes but on <input>
nodes.
As per the HTML to invoke click()
on the <input>
tag with respect to the <label>
tag you can use the following solution:
public void RateQuestion(int questionnumber, int rating)
{
string questionid = questionnumber.ToString() + "-" + rating.ToString();
IWebElement RateQuestionElement = this.Driver.FindElement(By.XPath("//label[@for='" + questionid + "']"));
RateQuestionElement.Click();
}
answered Nov 26 '18 at 9:31


DebanjanBDebanjanB
45.9k134688
45.9k134688
add a comment |
add a comment |
Try this:
public void RateQuestion(int questionnumber, int rating)
{
string questionid = questionnumber.ToString() + "-" + rating.ToString();
IWebElement RateQuestionElement = this.Driver.FindElement(By.Id(questionid)).FindElement(By.Xpath("./../../label"));
RateQuestionElement.Click();
}
Or this:
public void RateQuestion(int questionnumber, int rating)
{
string questionid = questionnumber.ToString() + "-" + rating.ToString();
IWebElement RateQuestionElement = this.FindElement(By.Xpath("//*[contains(local-name(), 'label') and contains(@for, questionid)]"));
RateQuestionElement.Click();
}
The latter one - I'm not that good with C#, so perhaps you'll have to put {questionid}
there, rather than just questionid
.
add a comment |
Try this:
public void RateQuestion(int questionnumber, int rating)
{
string questionid = questionnumber.ToString() + "-" + rating.ToString();
IWebElement RateQuestionElement = this.Driver.FindElement(By.Id(questionid)).FindElement(By.Xpath("./../../label"));
RateQuestionElement.Click();
}
Or this:
public void RateQuestion(int questionnumber, int rating)
{
string questionid = questionnumber.ToString() + "-" + rating.ToString();
IWebElement RateQuestionElement = this.FindElement(By.Xpath("//*[contains(local-name(), 'label') and contains(@for, questionid)]"));
RateQuestionElement.Click();
}
The latter one - I'm not that good with C#, so perhaps you'll have to put {questionid}
there, rather than just questionid
.
add a comment |
Try this:
public void RateQuestion(int questionnumber, int rating)
{
string questionid = questionnumber.ToString() + "-" + rating.ToString();
IWebElement RateQuestionElement = this.Driver.FindElement(By.Id(questionid)).FindElement(By.Xpath("./../../label"));
RateQuestionElement.Click();
}
Or this:
public void RateQuestion(int questionnumber, int rating)
{
string questionid = questionnumber.ToString() + "-" + rating.ToString();
IWebElement RateQuestionElement = this.FindElement(By.Xpath("//*[contains(local-name(), 'label') and contains(@for, questionid)]"));
RateQuestionElement.Click();
}
The latter one - I'm not that good with C#, so perhaps you'll have to put {questionid}
there, rather than just questionid
.
Try this:
public void RateQuestion(int questionnumber, int rating)
{
string questionid = questionnumber.ToString() + "-" + rating.ToString();
IWebElement RateQuestionElement = this.Driver.FindElement(By.Id(questionid)).FindElement(By.Xpath("./../../label"));
RateQuestionElement.Click();
}
Or this:
public void RateQuestion(int questionnumber, int rating)
{
string questionid = questionnumber.ToString() + "-" + rating.ToString();
IWebElement RateQuestionElement = this.FindElement(By.Xpath("//*[contains(local-name(), 'label') and contains(@for, questionid)]"));
RateQuestionElement.Click();
}
The latter one - I'm not that good with C#, so perhaps you'll have to put {questionid}
there, rather than just questionid
.
edited Nov 28 '18 at 11:46
answered Nov 28 '18 at 11:32


AlichinoAlichino
8131818
8131818
add a comment |
add a comment |
There are some options:
- After you select the element with questionId, the you can continue further to select the label.
e.g:
IWebElement RateQuestionElement = this.Driver.FindElement(By.Id(questionid));
IWebElement RateQuestionLabel = RateQuestionElement.FindElement(By.Css("label"));
RateQuestionLabel.click();
or
- You can use CssSelector
e.g:
myCssSelector = "#myQuestionId label"
IWebElement RateQuestionElement = this.Driver.FindElement(By.Css(myCssSelector));
RateQuestionElement.click();
I have not tried to execute it myself, but the concept is there
add a comment |
There are some options:
- After you select the element with questionId, the you can continue further to select the label.
e.g:
IWebElement RateQuestionElement = this.Driver.FindElement(By.Id(questionid));
IWebElement RateQuestionLabel = RateQuestionElement.FindElement(By.Css("label"));
RateQuestionLabel.click();
or
- You can use CssSelector
e.g:
myCssSelector = "#myQuestionId label"
IWebElement RateQuestionElement = this.Driver.FindElement(By.Css(myCssSelector));
RateQuestionElement.click();
I have not tried to execute it myself, but the concept is there
add a comment |
There are some options:
- After you select the element with questionId, the you can continue further to select the label.
e.g:
IWebElement RateQuestionElement = this.Driver.FindElement(By.Id(questionid));
IWebElement RateQuestionLabel = RateQuestionElement.FindElement(By.Css("label"));
RateQuestionLabel.click();
or
- You can use CssSelector
e.g:
myCssSelector = "#myQuestionId label"
IWebElement RateQuestionElement = this.Driver.FindElement(By.Css(myCssSelector));
RateQuestionElement.click();
I have not tried to execute it myself, but the concept is there
There are some options:
- After you select the element with questionId, the you can continue further to select the label.
e.g:
IWebElement RateQuestionElement = this.Driver.FindElement(By.Id(questionid));
IWebElement RateQuestionLabel = RateQuestionElement.FindElement(By.Css("label"));
RateQuestionLabel.click();
or
- You can use CssSelector
e.g:
myCssSelector = "#myQuestionId label"
IWebElement RateQuestionElement = this.Driver.FindElement(By.Css(myCssSelector));
RateQuestionElement.click();
I have not tried to execute it myself, but the concept is there
edited Mar 13 at 23:28
answered Nov 26 '18 at 9:43
andyssundaypinkandyssundaypink
30136
30136
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53477055%2fhow-to-select-following-element-in-selenium%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
gg6CyeaVkS9i h6 WCx60zS04w0NA 06i,EtcYaX92L0H2QNk ubBy1JmTw
Can you share your current code please? You should ask your question as minimal, complete, verifiable example
– Emre Savcı
Nov 26 '18 at 8:20
I've added my selenium selector code
– Peter K
Nov 26 '18 at 8:37
@Simon.B Why do you need to select the label from the input field? What is your usecase? Access the
<input>
or the<label>
?– DebanjanB
Nov 26 '18 at 9:21