getLocationOnScreen of a child of GridLayout
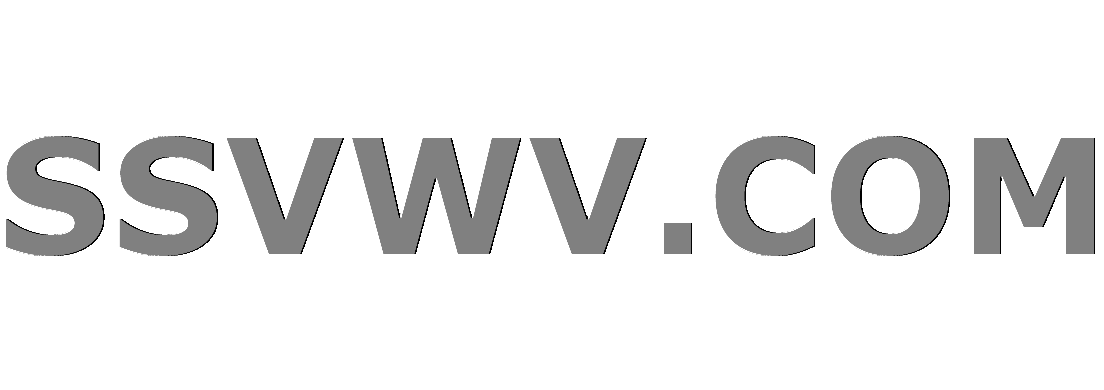
Multi tool use
up vote
2
down vote
favorite
I'm attempting to get the x/y of an imageview within a GridLayout but my log keeps showing X & Y: 0 0
any ideas?
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:id="@+id/root" >
...
<GridLayout
android:id="@+id/gridLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:columnCount="3"
android:rowCount="5" >
...
<ImageView
android:id="@+id/fivetwo"
android:layout_width="75dp"
android:layout_height="75dp"
android:contentDescription="@string/gameboard"
android:gravity="center"
android:src="@drawable/tile"
android:layout_columnSpan="1"
android:layout_rowSpan="1" />
heres the java:
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_level);
....
ImageView temp = (ImageView) findViewById(R.id.fivetwo);
int originalPos = new int[2];
temp.getLocationOnScreen( originalPos );
Log.i(TAG, "X & Y: " + originalPos[0] + " " + originalPos[1]);
...

add a comment |
up vote
2
down vote
favorite
I'm attempting to get the x/y of an imageview within a GridLayout but my log keeps showing X & Y: 0 0
any ideas?
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:id="@+id/root" >
...
<GridLayout
android:id="@+id/gridLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:columnCount="3"
android:rowCount="5" >
...
<ImageView
android:id="@+id/fivetwo"
android:layout_width="75dp"
android:layout_height="75dp"
android:contentDescription="@string/gameboard"
android:gravity="center"
android:src="@drawable/tile"
android:layout_columnSpan="1"
android:layout_rowSpan="1" />
heres the java:
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_level);
....
ImageView temp = (ImageView) findViewById(R.id.fivetwo);
int originalPos = new int[2];
temp.getLocationOnScreen( originalPos );
Log.i(TAG, "X & Y: " + originalPos[0] + " " + originalPos[1]);
...

add a comment |
up vote
2
down vote
favorite
up vote
2
down vote
favorite
I'm attempting to get the x/y of an imageview within a GridLayout but my log keeps showing X & Y: 0 0
any ideas?
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:id="@+id/root" >
...
<GridLayout
android:id="@+id/gridLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:columnCount="3"
android:rowCount="5" >
...
<ImageView
android:id="@+id/fivetwo"
android:layout_width="75dp"
android:layout_height="75dp"
android:contentDescription="@string/gameboard"
android:gravity="center"
android:src="@drawable/tile"
android:layout_columnSpan="1"
android:layout_rowSpan="1" />
heres the java:
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_level);
....
ImageView temp = (ImageView) findViewById(R.id.fivetwo);
int originalPos = new int[2];
temp.getLocationOnScreen( originalPos );
Log.i(TAG, "X & Y: " + originalPos[0] + " " + originalPos[1]);
...

I'm attempting to get the x/y of an imageview within a GridLayout but my log keeps showing X & Y: 0 0
any ideas?
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:id="@+id/root" >
...
<GridLayout
android:id="@+id/gridLayout1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:columnCount="3"
android:rowCount="5" >
...
<ImageView
android:id="@+id/fivetwo"
android:layout_width="75dp"
android:layout_height="75dp"
android:contentDescription="@string/gameboard"
android:gravity="center"
android:src="@drawable/tile"
android:layout_columnSpan="1"
android:layout_rowSpan="1" />
heres the java:
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_level);
....
ImageView temp = (ImageView) findViewById(R.id.fivetwo);
int originalPos = new int[2];
temp.getLocationOnScreen( originalPos );
Log.i(TAG, "X & Y: " + originalPos[0] + " " + originalPos[1]);
...


asked Oct 22 '13 at 18:50
jcaruso
79312051
79312051
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
up vote
8
down vote
accepted
Remember:
getX()
andgetY()
return0
if components are not drawn yet (in onCreate(){} ).
To find out the position of a view
as soon as it is ready:
Add a tree observer to the layout. This should return the correct position.
onCreate is called before the layout of the child views are done. So the width and height is not calculated yet. To get the height and width. Put this on the onCreate method
final ImageView temp = (ImageView) findViewById(R.id.fivetwo);
ViewTreeObserver vto = temp.getViewTreeObserver();
vto.addOnGlobalLayoutListener(new OnGlobalLayoutListener() {
@Override
public void onGlobalLayout() {
temp.getViewTreeObserver().removeGlobalOnLayoutListener(this);
int x = temp.getX();
int y = temp.getY();
Log.v(TAG, String.format("X:%d Y:%d",x,y);
}
});
add a comment |
up vote
5
down vote
You need to wait for the callback when the layout has placed the children views. The code you are using returns 0 because the position is returned before the layout is placed. Use this code:
temp.getViewTreeObserver().addOnGlobalLayoutListener(new OnGlobalLayoutListener() {
@Override
public void onGlobalLayout() {
getViewTreeObserver().removeGlobalOnLayoutListener(this);
int locations = new int[2];
temp.getLocationOnScreen(locations);
int x = locations[0];
int y = locations[1];
}
});
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f19525945%2fgetlocationonscreen-of-a-child-of-gridlayout%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
8
down vote
accepted
Remember:
getX()
andgetY()
return0
if components are not drawn yet (in onCreate(){} ).
To find out the position of a view
as soon as it is ready:
Add a tree observer to the layout. This should return the correct position.
onCreate is called before the layout of the child views are done. So the width and height is not calculated yet. To get the height and width. Put this on the onCreate method
final ImageView temp = (ImageView) findViewById(R.id.fivetwo);
ViewTreeObserver vto = temp.getViewTreeObserver();
vto.addOnGlobalLayoutListener(new OnGlobalLayoutListener() {
@Override
public void onGlobalLayout() {
temp.getViewTreeObserver().removeGlobalOnLayoutListener(this);
int x = temp.getX();
int y = temp.getY();
Log.v(TAG, String.format("X:%d Y:%d",x,y);
}
});
add a comment |
up vote
8
down vote
accepted
Remember:
getX()
andgetY()
return0
if components are not drawn yet (in onCreate(){} ).
To find out the position of a view
as soon as it is ready:
Add a tree observer to the layout. This should return the correct position.
onCreate is called before the layout of the child views are done. So the width and height is not calculated yet. To get the height and width. Put this on the onCreate method
final ImageView temp = (ImageView) findViewById(R.id.fivetwo);
ViewTreeObserver vto = temp.getViewTreeObserver();
vto.addOnGlobalLayoutListener(new OnGlobalLayoutListener() {
@Override
public void onGlobalLayout() {
temp.getViewTreeObserver().removeGlobalOnLayoutListener(this);
int x = temp.getX();
int y = temp.getY();
Log.v(TAG, String.format("X:%d Y:%d",x,y);
}
});
add a comment |
up vote
8
down vote
accepted
up vote
8
down vote
accepted
Remember:
getX()
andgetY()
return0
if components are not drawn yet (in onCreate(){} ).
To find out the position of a view
as soon as it is ready:
Add a tree observer to the layout. This should return the correct position.
onCreate is called before the layout of the child views are done. So the width and height is not calculated yet. To get the height and width. Put this on the onCreate method
final ImageView temp = (ImageView) findViewById(R.id.fivetwo);
ViewTreeObserver vto = temp.getViewTreeObserver();
vto.addOnGlobalLayoutListener(new OnGlobalLayoutListener() {
@Override
public void onGlobalLayout() {
temp.getViewTreeObserver().removeGlobalOnLayoutListener(this);
int x = temp.getX();
int y = temp.getY();
Log.v(TAG, String.format("X:%d Y:%d",x,y);
}
});
Remember:
getX()
andgetY()
return0
if components are not drawn yet (in onCreate(){} ).
To find out the position of a view
as soon as it is ready:
Add a tree observer to the layout. This should return the correct position.
onCreate is called before the layout of the child views are done. So the width and height is not calculated yet. To get the height and width. Put this on the onCreate method
final ImageView temp = (ImageView) findViewById(R.id.fivetwo);
ViewTreeObserver vto = temp.getViewTreeObserver();
vto.addOnGlobalLayoutListener(new OnGlobalLayoutListener() {
@Override
public void onGlobalLayout() {
temp.getViewTreeObserver().removeGlobalOnLayoutListener(this);
int x = temp.getX();
int y = temp.getY();
Log.v(TAG, String.format("X:%d Y:%d",x,y);
}
});
edited Oct 22 '13 at 20:04
answered Oct 22 '13 at 19:04


nana
3,47812745
3,47812745
add a comment |
add a comment |
up vote
5
down vote
You need to wait for the callback when the layout has placed the children views. The code you are using returns 0 because the position is returned before the layout is placed. Use this code:
temp.getViewTreeObserver().addOnGlobalLayoutListener(new OnGlobalLayoutListener() {
@Override
public void onGlobalLayout() {
getViewTreeObserver().removeGlobalOnLayoutListener(this);
int locations = new int[2];
temp.getLocationOnScreen(locations);
int x = locations[0];
int y = locations[1];
}
});
add a comment |
up vote
5
down vote
You need to wait for the callback when the layout has placed the children views. The code you are using returns 0 because the position is returned before the layout is placed. Use this code:
temp.getViewTreeObserver().addOnGlobalLayoutListener(new OnGlobalLayoutListener() {
@Override
public void onGlobalLayout() {
getViewTreeObserver().removeGlobalOnLayoutListener(this);
int locations = new int[2];
temp.getLocationOnScreen(locations);
int x = locations[0];
int y = locations[1];
}
});
add a comment |
up vote
5
down vote
up vote
5
down vote
You need to wait for the callback when the layout has placed the children views. The code you are using returns 0 because the position is returned before the layout is placed. Use this code:
temp.getViewTreeObserver().addOnGlobalLayoutListener(new OnGlobalLayoutListener() {
@Override
public void onGlobalLayout() {
getViewTreeObserver().removeGlobalOnLayoutListener(this);
int locations = new int[2];
temp.getLocationOnScreen(locations);
int x = locations[0];
int y = locations[1];
}
});
You need to wait for the callback when the layout has placed the children views. The code you are using returns 0 because the position is returned before the layout is placed. Use this code:
temp.getViewTreeObserver().addOnGlobalLayoutListener(new OnGlobalLayoutListener() {
@Override
public void onGlobalLayout() {
getViewTreeObserver().removeGlobalOnLayoutListener(this);
int locations = new int[2];
temp.getLocationOnScreen(locations);
int x = locations[0];
int y = locations[1];
}
});
edited Nov 20 at 9:37


Aayush Goyal
1391315
1391315
answered Oct 22 '13 at 19:05
Naddy
1,82761837
1,82761837
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f19525945%2fgetlocationonscreen-of-a-child-of-gridlayout%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Y,mKc6Sm0E1iNcQCn8BADmQNbb00vvBvtDsYCIFU