Trying to get output for ArrayList in Java
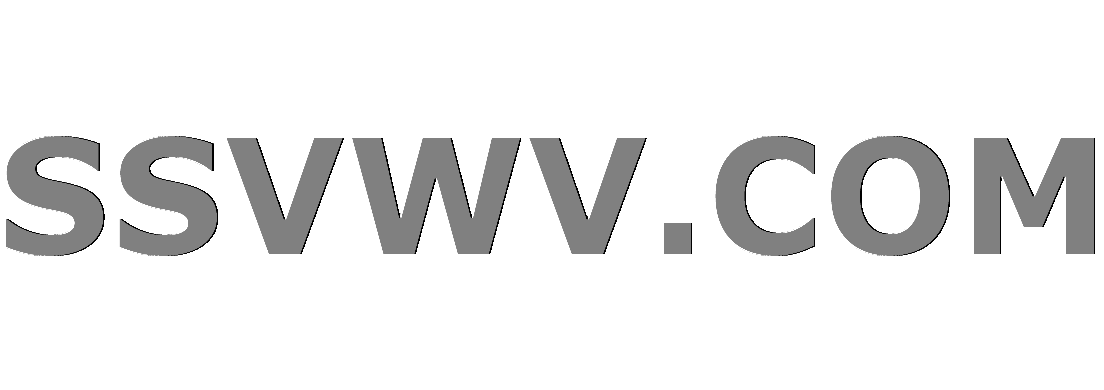
Multi tool use
I am trying to get a code that takes a csv file and puts it into an ArrayList and shows the content of the ArrayList. I believe I have the code correct for getting the data into the ArrayList but I can't get it to print. Please any advice is welcome. I am very new to Java and coding in general.
import java.io.BufferedReader;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
import java.util.ArrayList;
class Employee{
public String FirstName;
public String LastName;
public String Company;
public String Address;
public String City;
public String County;
public String State;
public String Zip;
public String Phone;
public String Fax;
public String Email;
public String Web;
}
public class JTeagueITCO321IPWeek4 {
public static void main(String args) throws FileNotFoundException, IOException {
String line = "";
ArrayList <Employee> ALEmployee = new ArrayList();
FileReader fr = new FileReader("C:\Users\User\Downloads\ITCO321_U4IP_sample_data.csv");
try (BufferedReader br = new BufferedReader(fr)) {
while ((line = br.readLine())!=null){
Employee emp = new Employee();
String empFields = line.split(",");
emp.FirstName = empFields[0];
emp.LastName = empFields[1];
emp.Company = empFields[2];
emp.Address = empFields[3];
emp.City = empFields[4];
emp.County = empFields[5];
emp.State = empFields[6];
emp.Zip = empFields[7];
emp.Phone = empFields[8];
emp.Fax = empFields[9];
emp.Email = empFields[10];
emp.Web = empFields[11];
ALEmployee.add(emp);
}
}
}
}
java arraylist
add a comment |
I am trying to get a code that takes a csv file and puts it into an ArrayList and shows the content of the ArrayList. I believe I have the code correct for getting the data into the ArrayList but I can't get it to print. Please any advice is welcome. I am very new to Java and coding in general.
import java.io.BufferedReader;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
import java.util.ArrayList;
class Employee{
public String FirstName;
public String LastName;
public String Company;
public String Address;
public String City;
public String County;
public String State;
public String Zip;
public String Phone;
public String Fax;
public String Email;
public String Web;
}
public class JTeagueITCO321IPWeek4 {
public static void main(String args) throws FileNotFoundException, IOException {
String line = "";
ArrayList <Employee> ALEmployee = new ArrayList();
FileReader fr = new FileReader("C:\Users\User\Downloads\ITCO321_U4IP_sample_data.csv");
try (BufferedReader br = new BufferedReader(fr)) {
while ((line = br.readLine())!=null){
Employee emp = new Employee();
String empFields = line.split(",");
emp.FirstName = empFields[0];
emp.LastName = empFields[1];
emp.Company = empFields[2];
emp.Address = empFields[3];
emp.City = empFields[4];
emp.County = empFields[5];
emp.State = empFields[6];
emp.Zip = empFields[7];
emp.Phone = empFields[8];
emp.Fax = empFields[9];
emp.Email = empFields[10];
emp.Web = empFields[11];
ALEmployee.add(emp);
}
}
}
}
java arraylist
You don’t appear to be printing out theArrayList
anywhere.
– Logan
Nov 20 at 23:13
That is what I am trying to figure out how to do.
– NewCoder04
Nov 20 at 23:14
UsingSystem.out.println()
?
– Logan
Nov 20 at 23:15
1
Possible duplicate of How to print out all the elements of a List in Java?
– Gino Mempin
Nov 20 at 23:48
Yes, I was planning on using the System.out.println(). I was just running into issues on what to put in the ().
– NewCoder04
Nov 20 at 23:51
add a comment |
I am trying to get a code that takes a csv file and puts it into an ArrayList and shows the content of the ArrayList. I believe I have the code correct for getting the data into the ArrayList but I can't get it to print. Please any advice is welcome. I am very new to Java and coding in general.
import java.io.BufferedReader;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
import java.util.ArrayList;
class Employee{
public String FirstName;
public String LastName;
public String Company;
public String Address;
public String City;
public String County;
public String State;
public String Zip;
public String Phone;
public String Fax;
public String Email;
public String Web;
}
public class JTeagueITCO321IPWeek4 {
public static void main(String args) throws FileNotFoundException, IOException {
String line = "";
ArrayList <Employee> ALEmployee = new ArrayList();
FileReader fr = new FileReader("C:\Users\User\Downloads\ITCO321_U4IP_sample_data.csv");
try (BufferedReader br = new BufferedReader(fr)) {
while ((line = br.readLine())!=null){
Employee emp = new Employee();
String empFields = line.split(",");
emp.FirstName = empFields[0];
emp.LastName = empFields[1];
emp.Company = empFields[2];
emp.Address = empFields[3];
emp.City = empFields[4];
emp.County = empFields[5];
emp.State = empFields[6];
emp.Zip = empFields[7];
emp.Phone = empFields[8];
emp.Fax = empFields[9];
emp.Email = empFields[10];
emp.Web = empFields[11];
ALEmployee.add(emp);
}
}
}
}
java arraylist
I am trying to get a code that takes a csv file and puts it into an ArrayList and shows the content of the ArrayList. I believe I have the code correct for getting the data into the ArrayList but I can't get it to print. Please any advice is welcome. I am very new to Java and coding in general.
import java.io.BufferedReader;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
import java.util.ArrayList;
class Employee{
public String FirstName;
public String LastName;
public String Company;
public String Address;
public String City;
public String County;
public String State;
public String Zip;
public String Phone;
public String Fax;
public String Email;
public String Web;
}
public class JTeagueITCO321IPWeek4 {
public static void main(String args) throws FileNotFoundException, IOException {
String line = "";
ArrayList <Employee> ALEmployee = new ArrayList();
FileReader fr = new FileReader("C:\Users\User\Downloads\ITCO321_U4IP_sample_data.csv");
try (BufferedReader br = new BufferedReader(fr)) {
while ((line = br.readLine())!=null){
Employee emp = new Employee();
String empFields = line.split(",");
emp.FirstName = empFields[0];
emp.LastName = empFields[1];
emp.Company = empFields[2];
emp.Address = empFields[3];
emp.City = empFields[4];
emp.County = empFields[5];
emp.State = empFields[6];
emp.Zip = empFields[7];
emp.Phone = empFields[8];
emp.Fax = empFields[9];
emp.Email = empFields[10];
emp.Web = empFields[11];
ALEmployee.add(emp);
}
}
}
}
java arraylist
java arraylist
edited Nov 20 at 23:19


azro
10.3k41438
10.3k41438
asked Nov 20 at 23:12
NewCoder04
84
84
You don’t appear to be printing out theArrayList
anywhere.
– Logan
Nov 20 at 23:13
That is what I am trying to figure out how to do.
– NewCoder04
Nov 20 at 23:14
UsingSystem.out.println()
?
– Logan
Nov 20 at 23:15
1
Possible duplicate of How to print out all the elements of a List in Java?
– Gino Mempin
Nov 20 at 23:48
Yes, I was planning on using the System.out.println(). I was just running into issues on what to put in the ().
– NewCoder04
Nov 20 at 23:51
add a comment |
You don’t appear to be printing out theArrayList
anywhere.
– Logan
Nov 20 at 23:13
That is what I am trying to figure out how to do.
– NewCoder04
Nov 20 at 23:14
UsingSystem.out.println()
?
– Logan
Nov 20 at 23:15
1
Possible duplicate of How to print out all the elements of a List in Java?
– Gino Mempin
Nov 20 at 23:48
Yes, I was planning on using the System.out.println(). I was just running into issues on what to put in the ().
– NewCoder04
Nov 20 at 23:51
You don’t appear to be printing out the
ArrayList
anywhere.– Logan
Nov 20 at 23:13
You don’t appear to be printing out the
ArrayList
anywhere.– Logan
Nov 20 at 23:13
That is what I am trying to figure out how to do.
– NewCoder04
Nov 20 at 23:14
That is what I am trying to figure out how to do.
– NewCoder04
Nov 20 at 23:14
Using
System.out.println()
?– Logan
Nov 20 at 23:15
Using
System.out.println()
?– Logan
Nov 20 at 23:15
1
1
Possible duplicate of How to print out all the elements of a List in Java?
– Gino Mempin
Nov 20 at 23:48
Possible duplicate of How to print out all the elements of a List in Java?
– Gino Mempin
Nov 20 at 23:48
Yes, I was planning on using the System.out.println(). I was just running into issues on what to put in the ().
– NewCoder04
Nov 20 at 23:51
Yes, I was planning on using the System.out.println(). I was just running into issues on what to put in the ().
– NewCoder04
Nov 20 at 23:51
add a comment |
1 Answer
1
active
oldest
votes
To print an ArrayList
you can just
Use
System.out.println(ALEmployee);
or be more precise and print each element one by one
for(Employee e : ALEmployee){
System.out.println(e);
}
In both case you'll need to implement the toString()
method in Employee
class as :
public String toString(){
return FirstName+" "+LastName+" "+Company; // do whatever you want here
}
Also I would suggest you, to follow Java conventions and coding conventions to:
- use
lowerCamelCase
for naming the attributs/variables - set the attributs as
private
and usesetters
or a constructor to instanciate anEmployee
with its attributs
Thank you! This was very helpful!
– NewCoder04
Nov 20 at 23:53
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53403010%2ftrying-to-get-output-for-arraylist-in-java%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
To print an ArrayList
you can just
Use
System.out.println(ALEmployee);
or be more precise and print each element one by one
for(Employee e : ALEmployee){
System.out.println(e);
}
In both case you'll need to implement the toString()
method in Employee
class as :
public String toString(){
return FirstName+" "+LastName+" "+Company; // do whatever you want here
}
Also I would suggest you, to follow Java conventions and coding conventions to:
- use
lowerCamelCase
for naming the attributs/variables - set the attributs as
private
and usesetters
or a constructor to instanciate anEmployee
with its attributs
Thank you! This was very helpful!
– NewCoder04
Nov 20 at 23:53
add a comment |
To print an ArrayList
you can just
Use
System.out.println(ALEmployee);
or be more precise and print each element one by one
for(Employee e : ALEmployee){
System.out.println(e);
}
In both case you'll need to implement the toString()
method in Employee
class as :
public String toString(){
return FirstName+" "+LastName+" "+Company; // do whatever you want here
}
Also I would suggest you, to follow Java conventions and coding conventions to:
- use
lowerCamelCase
for naming the attributs/variables - set the attributs as
private
and usesetters
or a constructor to instanciate anEmployee
with its attributs
Thank you! This was very helpful!
– NewCoder04
Nov 20 at 23:53
add a comment |
To print an ArrayList
you can just
Use
System.out.println(ALEmployee);
or be more precise and print each element one by one
for(Employee e : ALEmployee){
System.out.println(e);
}
In both case you'll need to implement the toString()
method in Employee
class as :
public String toString(){
return FirstName+" "+LastName+" "+Company; // do whatever you want here
}
Also I would suggest you, to follow Java conventions and coding conventions to:
- use
lowerCamelCase
for naming the attributs/variables - set the attributs as
private
and usesetters
or a constructor to instanciate anEmployee
with its attributs
To print an ArrayList
you can just
Use
System.out.println(ALEmployee);
or be more precise and print each element one by one
for(Employee e : ALEmployee){
System.out.println(e);
}
In both case you'll need to implement the toString()
method in Employee
class as :
public String toString(){
return FirstName+" "+LastName+" "+Company; // do whatever you want here
}
Also I would suggest you, to follow Java conventions and coding conventions to:
- use
lowerCamelCase
for naming the attributs/variables - set the attributs as
private
and usesetters
or a constructor to instanciate anEmployee
with its attributs
answered Nov 20 at 23:16


azro
10.3k41438
10.3k41438
Thank you! This was very helpful!
– NewCoder04
Nov 20 at 23:53
add a comment |
Thank you! This was very helpful!
– NewCoder04
Nov 20 at 23:53
Thank you! This was very helpful!
– NewCoder04
Nov 20 at 23:53
Thank you! This was very helpful!
– NewCoder04
Nov 20 at 23:53
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53403010%2ftrying-to-get-output-for-arraylist-in-java%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
BOp7rygkWuYr y,Sf,MZZ5
You don’t appear to be printing out the
ArrayList
anywhere.– Logan
Nov 20 at 23:13
That is what I am trying to figure out how to do.
– NewCoder04
Nov 20 at 23:14
Using
System.out.println()
?– Logan
Nov 20 at 23:15
1
Possible duplicate of How to print out all the elements of a List in Java?
– Gino Mempin
Nov 20 at 23:48
Yes, I was planning on using the System.out.println(). I was just running into issues on what to put in the ().
– NewCoder04
Nov 20 at 23:51