DirectX11 - Geometry Shader with Stream Output
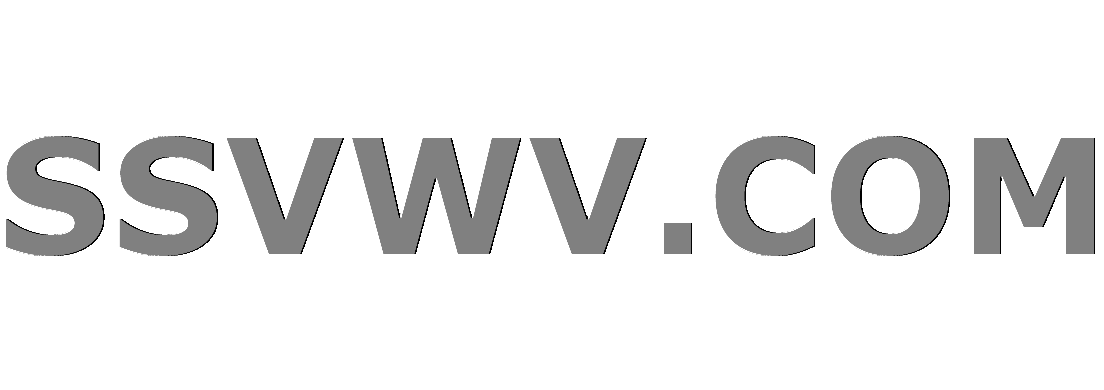
Multi tool use
I'm trying to stream out data from the geometry shader to use as a vertex buffer to be input into another shader. However, this doesn't seem to be working, and the debug layer is telling me nothing. Is there something I'm doing wrong with the buffer descriptions?
Here's how I'm creating the output buffer:
D3D11_BUFFER_DESC outputBufferDesc;
ZeroMemory(&outputBufferDesc, sizeof(outputBufferDesc));
outputBufferDesc.ByteWidth = sizeof(XMFLOAT4) + 2 * sizeof(XMFLOAT3);
outputBufferDesc.Usage = D3D11_USAGE_DEFAULT;
outputBufferDesc.BindFlags = D3D11_BIND_STREAM_OUTPUT;
outputBufferDesc.CPUAccessFlags = 0;
outputBufferDesc.MiscFlags = 0;
outputBufferDesc.StructureByteStride = 0;
result = renderer->CreateBuffer(&outputBufferDesc, NULL, &outputBuffer);
if (result != S_OK)
{
MessageBox(NULL, filename, L"Failed to create stream output buffer", MB_OK);
exit(0);
}
UINT offset[1] = { 0 };
deviceContext->SOSetTargets(1, &outputBuffer, offset);
And the draw call for the first shader:
deviceContext->VSSetShader(vertexShader, NULL, 0);
deviceContext->PSSetShader(pixelShader, NULL, 0);
deviceContext->GSSetShader(streamOutputGeometryShader, NULL, 0);
// Render the triangle.
deviceContext->DrawIndexed(indexCount, 0, 0);
UINT offset[1] = { 0 };
ID3D11Buffer* pNullBuffer = 0;
deviceContext->SOSetTargets(1, &pNullBuffer, offset);
And where I copy the information from that buffer into a second buffer:
D3D11_BUFFER_DESC vertexBufferDesc;
ZeroMemory(&vertexBufferDesc, sizeof(vertexBufferDesc));
vertexBufferDesc.ByteWidth = sizeof(XMFLOAT4) + 2 * sizeof(XMFLOAT3);
vertexBufferDesc.Usage = D3D11_USAGE_DEFAULT;
vertexBufferDesc.BindFlags = D3D11_BIND_VERTEX_BUFFER;
vertexBufferDesc.CPUAccessFlags = 0;
vertexBufferDesc.MiscFlags = 0;
vertexBufferDesc.StructureByteStride = 0;
result = renderer->CreateBuffer(&vertexBufferDesc, NULL, &vertexBuffer);
if (result != S_OK)
{
MessageBox(NULL, L"Failed to create output mesh vertex buffer", L"Failed", MB_OK);
exit(0);
}
deviceContext->CopyResource(vertexBuffer, buffer);
And where I set the input topology for the mesh using the vertex buffer:
unsigned int stride;
unsigned int offset;
// Set vertex buffer stride and offset.
stride = sizeof(XMFLOAT4) + 2 * sizeof(XMFLOAT3);
offset = 0;
deviceContext->IASetVertexBuffers(0, 1, &vertexBuffer, &stride, &offset);
deviceContext->IASetPrimitiveTopology(D3D11_PRIMITIVE_TOPOLOGY_TRIANGLELIST);
And finally, the draw call for the second shader is simple:
deviceContext->DrawAuto();
EDIT: The geometry is being computed in the geometry shader correctly, but it's not being streamed out. I've included the geometry shader creation code below:
D3D11_SO_DECLARATION_ENTRY SODeclarationEntry[3] =
{
{ 0, "SV_POSITION", 0, 0, 4, 0 },
{ 0, "NORMAL", 0, 0, 3, 0 },
{ 0, "TEXCOORD", 0, 0, 3, 0 }
};
// Create the geometry shader from the buffer.
result = renderer->CreateGeometryShaderWithStreamOutput(geometryShaderBuffer->GetBufferPointer(), geometryShaderBuffer->GetBufferSize(), SODeclarationEntry, _countof(SODeclarationEntry),
NULL, 0, D3D11_SO_NO_RASTERIZED_STREAM, NULL, &streamOutputGeometryShader);
c++ directx hlsl
add a comment |
I'm trying to stream out data from the geometry shader to use as a vertex buffer to be input into another shader. However, this doesn't seem to be working, and the debug layer is telling me nothing. Is there something I'm doing wrong with the buffer descriptions?
Here's how I'm creating the output buffer:
D3D11_BUFFER_DESC outputBufferDesc;
ZeroMemory(&outputBufferDesc, sizeof(outputBufferDesc));
outputBufferDesc.ByteWidth = sizeof(XMFLOAT4) + 2 * sizeof(XMFLOAT3);
outputBufferDesc.Usage = D3D11_USAGE_DEFAULT;
outputBufferDesc.BindFlags = D3D11_BIND_STREAM_OUTPUT;
outputBufferDesc.CPUAccessFlags = 0;
outputBufferDesc.MiscFlags = 0;
outputBufferDesc.StructureByteStride = 0;
result = renderer->CreateBuffer(&outputBufferDesc, NULL, &outputBuffer);
if (result != S_OK)
{
MessageBox(NULL, filename, L"Failed to create stream output buffer", MB_OK);
exit(0);
}
UINT offset[1] = { 0 };
deviceContext->SOSetTargets(1, &outputBuffer, offset);
And the draw call for the first shader:
deviceContext->VSSetShader(vertexShader, NULL, 0);
deviceContext->PSSetShader(pixelShader, NULL, 0);
deviceContext->GSSetShader(streamOutputGeometryShader, NULL, 0);
// Render the triangle.
deviceContext->DrawIndexed(indexCount, 0, 0);
UINT offset[1] = { 0 };
ID3D11Buffer* pNullBuffer = 0;
deviceContext->SOSetTargets(1, &pNullBuffer, offset);
And where I copy the information from that buffer into a second buffer:
D3D11_BUFFER_DESC vertexBufferDesc;
ZeroMemory(&vertexBufferDesc, sizeof(vertexBufferDesc));
vertexBufferDesc.ByteWidth = sizeof(XMFLOAT4) + 2 * sizeof(XMFLOAT3);
vertexBufferDesc.Usage = D3D11_USAGE_DEFAULT;
vertexBufferDesc.BindFlags = D3D11_BIND_VERTEX_BUFFER;
vertexBufferDesc.CPUAccessFlags = 0;
vertexBufferDesc.MiscFlags = 0;
vertexBufferDesc.StructureByteStride = 0;
result = renderer->CreateBuffer(&vertexBufferDesc, NULL, &vertexBuffer);
if (result != S_OK)
{
MessageBox(NULL, L"Failed to create output mesh vertex buffer", L"Failed", MB_OK);
exit(0);
}
deviceContext->CopyResource(vertexBuffer, buffer);
And where I set the input topology for the mesh using the vertex buffer:
unsigned int stride;
unsigned int offset;
// Set vertex buffer stride and offset.
stride = sizeof(XMFLOAT4) + 2 * sizeof(XMFLOAT3);
offset = 0;
deviceContext->IASetVertexBuffers(0, 1, &vertexBuffer, &stride, &offset);
deviceContext->IASetPrimitiveTopology(D3D11_PRIMITIVE_TOPOLOGY_TRIANGLELIST);
And finally, the draw call for the second shader is simple:
deviceContext->DrawAuto();
EDIT: The geometry is being computed in the geometry shader correctly, but it's not being streamed out. I've included the geometry shader creation code below:
D3D11_SO_DECLARATION_ENTRY SODeclarationEntry[3] =
{
{ 0, "SV_POSITION", 0, 0, 4, 0 },
{ 0, "NORMAL", 0, 0, 3, 0 },
{ 0, "TEXCOORD", 0, 0, 3, 0 }
};
// Create the geometry shader from the buffer.
result = renderer->CreateGeometryShaderWithStreamOutput(geometryShaderBuffer->GetBufferPointer(), geometryShaderBuffer->GetBufferSize(), SODeclarationEntry, _countof(SODeclarationEntry),
NULL, 0, D3D11_SO_NO_RASTERIZED_STREAM, NULL, &streamOutputGeometryShader);
c++ directx hlsl
add a comment |
I'm trying to stream out data from the geometry shader to use as a vertex buffer to be input into another shader. However, this doesn't seem to be working, and the debug layer is telling me nothing. Is there something I'm doing wrong with the buffer descriptions?
Here's how I'm creating the output buffer:
D3D11_BUFFER_DESC outputBufferDesc;
ZeroMemory(&outputBufferDesc, sizeof(outputBufferDesc));
outputBufferDesc.ByteWidth = sizeof(XMFLOAT4) + 2 * sizeof(XMFLOAT3);
outputBufferDesc.Usage = D3D11_USAGE_DEFAULT;
outputBufferDesc.BindFlags = D3D11_BIND_STREAM_OUTPUT;
outputBufferDesc.CPUAccessFlags = 0;
outputBufferDesc.MiscFlags = 0;
outputBufferDesc.StructureByteStride = 0;
result = renderer->CreateBuffer(&outputBufferDesc, NULL, &outputBuffer);
if (result != S_OK)
{
MessageBox(NULL, filename, L"Failed to create stream output buffer", MB_OK);
exit(0);
}
UINT offset[1] = { 0 };
deviceContext->SOSetTargets(1, &outputBuffer, offset);
And the draw call for the first shader:
deviceContext->VSSetShader(vertexShader, NULL, 0);
deviceContext->PSSetShader(pixelShader, NULL, 0);
deviceContext->GSSetShader(streamOutputGeometryShader, NULL, 0);
// Render the triangle.
deviceContext->DrawIndexed(indexCount, 0, 0);
UINT offset[1] = { 0 };
ID3D11Buffer* pNullBuffer = 0;
deviceContext->SOSetTargets(1, &pNullBuffer, offset);
And where I copy the information from that buffer into a second buffer:
D3D11_BUFFER_DESC vertexBufferDesc;
ZeroMemory(&vertexBufferDesc, sizeof(vertexBufferDesc));
vertexBufferDesc.ByteWidth = sizeof(XMFLOAT4) + 2 * sizeof(XMFLOAT3);
vertexBufferDesc.Usage = D3D11_USAGE_DEFAULT;
vertexBufferDesc.BindFlags = D3D11_BIND_VERTEX_BUFFER;
vertexBufferDesc.CPUAccessFlags = 0;
vertexBufferDesc.MiscFlags = 0;
vertexBufferDesc.StructureByteStride = 0;
result = renderer->CreateBuffer(&vertexBufferDesc, NULL, &vertexBuffer);
if (result != S_OK)
{
MessageBox(NULL, L"Failed to create output mesh vertex buffer", L"Failed", MB_OK);
exit(0);
}
deviceContext->CopyResource(vertexBuffer, buffer);
And where I set the input topology for the mesh using the vertex buffer:
unsigned int stride;
unsigned int offset;
// Set vertex buffer stride and offset.
stride = sizeof(XMFLOAT4) + 2 * sizeof(XMFLOAT3);
offset = 0;
deviceContext->IASetVertexBuffers(0, 1, &vertexBuffer, &stride, &offset);
deviceContext->IASetPrimitiveTopology(D3D11_PRIMITIVE_TOPOLOGY_TRIANGLELIST);
And finally, the draw call for the second shader is simple:
deviceContext->DrawAuto();
EDIT: The geometry is being computed in the geometry shader correctly, but it's not being streamed out. I've included the geometry shader creation code below:
D3D11_SO_DECLARATION_ENTRY SODeclarationEntry[3] =
{
{ 0, "SV_POSITION", 0, 0, 4, 0 },
{ 0, "NORMAL", 0, 0, 3, 0 },
{ 0, "TEXCOORD", 0, 0, 3, 0 }
};
// Create the geometry shader from the buffer.
result = renderer->CreateGeometryShaderWithStreamOutput(geometryShaderBuffer->GetBufferPointer(), geometryShaderBuffer->GetBufferSize(), SODeclarationEntry, _countof(SODeclarationEntry),
NULL, 0, D3D11_SO_NO_RASTERIZED_STREAM, NULL, &streamOutputGeometryShader);
c++ directx hlsl
I'm trying to stream out data from the geometry shader to use as a vertex buffer to be input into another shader. However, this doesn't seem to be working, and the debug layer is telling me nothing. Is there something I'm doing wrong with the buffer descriptions?
Here's how I'm creating the output buffer:
D3D11_BUFFER_DESC outputBufferDesc;
ZeroMemory(&outputBufferDesc, sizeof(outputBufferDesc));
outputBufferDesc.ByteWidth = sizeof(XMFLOAT4) + 2 * sizeof(XMFLOAT3);
outputBufferDesc.Usage = D3D11_USAGE_DEFAULT;
outputBufferDesc.BindFlags = D3D11_BIND_STREAM_OUTPUT;
outputBufferDesc.CPUAccessFlags = 0;
outputBufferDesc.MiscFlags = 0;
outputBufferDesc.StructureByteStride = 0;
result = renderer->CreateBuffer(&outputBufferDesc, NULL, &outputBuffer);
if (result != S_OK)
{
MessageBox(NULL, filename, L"Failed to create stream output buffer", MB_OK);
exit(0);
}
UINT offset[1] = { 0 };
deviceContext->SOSetTargets(1, &outputBuffer, offset);
And the draw call for the first shader:
deviceContext->VSSetShader(vertexShader, NULL, 0);
deviceContext->PSSetShader(pixelShader, NULL, 0);
deviceContext->GSSetShader(streamOutputGeometryShader, NULL, 0);
// Render the triangle.
deviceContext->DrawIndexed(indexCount, 0, 0);
UINT offset[1] = { 0 };
ID3D11Buffer* pNullBuffer = 0;
deviceContext->SOSetTargets(1, &pNullBuffer, offset);
And where I copy the information from that buffer into a second buffer:
D3D11_BUFFER_DESC vertexBufferDesc;
ZeroMemory(&vertexBufferDesc, sizeof(vertexBufferDesc));
vertexBufferDesc.ByteWidth = sizeof(XMFLOAT4) + 2 * sizeof(XMFLOAT3);
vertexBufferDesc.Usage = D3D11_USAGE_DEFAULT;
vertexBufferDesc.BindFlags = D3D11_BIND_VERTEX_BUFFER;
vertexBufferDesc.CPUAccessFlags = 0;
vertexBufferDesc.MiscFlags = 0;
vertexBufferDesc.StructureByteStride = 0;
result = renderer->CreateBuffer(&vertexBufferDesc, NULL, &vertexBuffer);
if (result != S_OK)
{
MessageBox(NULL, L"Failed to create output mesh vertex buffer", L"Failed", MB_OK);
exit(0);
}
deviceContext->CopyResource(vertexBuffer, buffer);
And where I set the input topology for the mesh using the vertex buffer:
unsigned int stride;
unsigned int offset;
// Set vertex buffer stride and offset.
stride = sizeof(XMFLOAT4) + 2 * sizeof(XMFLOAT3);
offset = 0;
deviceContext->IASetVertexBuffers(0, 1, &vertexBuffer, &stride, &offset);
deviceContext->IASetPrimitiveTopology(D3D11_PRIMITIVE_TOPOLOGY_TRIANGLELIST);
And finally, the draw call for the second shader is simple:
deviceContext->DrawAuto();
EDIT: The geometry is being computed in the geometry shader correctly, but it's not being streamed out. I've included the geometry shader creation code below:
D3D11_SO_DECLARATION_ENTRY SODeclarationEntry[3] =
{
{ 0, "SV_POSITION", 0, 0, 4, 0 },
{ 0, "NORMAL", 0, 0, 3, 0 },
{ 0, "TEXCOORD", 0, 0, 3, 0 }
};
// Create the geometry shader from the buffer.
result = renderer->CreateGeometryShaderWithStreamOutput(geometryShaderBuffer->GetBufferPointer(), geometryShaderBuffer->GetBufferSize(), SODeclarationEntry, _countof(SODeclarationEntry),
NULL, 0, D3D11_SO_NO_RASTERIZED_STREAM, NULL, &streamOutputGeometryShader);
c++ directx hlsl
c++ directx hlsl
edited Nov 22 '18 at 1:07
MattBT12
asked Nov 21 '18 at 20:24
MattBT12MattBT12
314
314
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
In addition to just the actual vertex data, buffers used as targets for the Stream Output Stage maintain some internal state to keep track of how much geometry was written out. DrawAuto()
relies on this information to know how much geometry to draw. When you copy just the vertex data out of your stream output buffer into a plain D3D11_BIND_VERTEX_BUFFER
, this information will be lost.
Relevant quote from the documentation:
DrawAuto only works when drawing with one input buffer bound as an input to the IA stage at slot 0. Applications must create the SO buffer resource with both binding flags, D3D11_BIND_VERTEX_BUFFER and D3D11_BIND_STREAM_OUTPUT.
I'm not sure if it's supposed to be possible to copy data from one stream output buffer to another and DrawAuto()
from there. I guess that might work. What you are doing at the moment, however, is definitely not supposed to work according to the documentation. Ideally, you'd just avoid copying the data. If you really must copy the data (for whatever reason), I suggest you nevertheless first try drawing directly from the buffer you streamed into to make sure everything else is set up correctly and only once that works try to copy into another buffer that has both vertex and stream output bind flags set…
I've fixed this, but after running the shader through Visual Studio's graphics debugger it appears that nothing is being written to the stream output buffer; it remains 40 bytes in size with every element being 0. This is despite the stream output buffer being assigned and the shader outputting geometry. I've edited my post to include the shader creation, in case that might be incorrect.
– MattBT12
Nov 22 '18 at 1:10
Well, one thing that did strike me as a bit odd about your code up there is that your buffers seem tiny. I assumed you had a reason for that. But now that I notice that your strides are the same as the buffer sizes: You are aware of the fact that theByteWidth
is supposed to be the size of the entire buffer and not just the size of a single vertex?
– Michael Kenzel
Nov 22 '18 at 1:17
I figured as much given what the documentation does on MSDN; I must've forgotten to change it back to what it was after tinkering with it (that's what I get for programming at 1 in the morning). Right now I'm just setting the byte width to be a very large value that doesn't change because I don't know the number of vertices that will be in the buffer. Is this the best approach for this situation? Or is there a strategy for dynamically sizing the buffer?
– MattBT12
Nov 22 '18 at 1:57
Well, setting it to "a very large value" should be good enough to make it do something (as long as that very large value is not so large that the allocation fails, of course). Obviously, it's not the best approach in general to just blindly allocate some arbitrary, huge amount of memory. In the case of stream output, you should have at least some idea how much geometry your application is going to process and be able to derive some worst-case size that the buffer needs to be!?
– Michael Kenzel
Nov 22 '18 at 10:43
I do know roughly how much data will be output, but sizing the buffer for a worst case scenario seems somewhat inefficient. I was just curious to see if there's a better way of doing it that I hadn't thought of.
– MattBT12
Nov 22 '18 at 21:39
|
show 1 more comment
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53419965%2fdirectx11-geometry-shader-with-stream-output%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
In addition to just the actual vertex data, buffers used as targets for the Stream Output Stage maintain some internal state to keep track of how much geometry was written out. DrawAuto()
relies on this information to know how much geometry to draw. When you copy just the vertex data out of your stream output buffer into a plain D3D11_BIND_VERTEX_BUFFER
, this information will be lost.
Relevant quote from the documentation:
DrawAuto only works when drawing with one input buffer bound as an input to the IA stage at slot 0. Applications must create the SO buffer resource with both binding flags, D3D11_BIND_VERTEX_BUFFER and D3D11_BIND_STREAM_OUTPUT.
I'm not sure if it's supposed to be possible to copy data from one stream output buffer to another and DrawAuto()
from there. I guess that might work. What you are doing at the moment, however, is definitely not supposed to work according to the documentation. Ideally, you'd just avoid copying the data. If you really must copy the data (for whatever reason), I suggest you nevertheless first try drawing directly from the buffer you streamed into to make sure everything else is set up correctly and only once that works try to copy into another buffer that has both vertex and stream output bind flags set…
I've fixed this, but after running the shader through Visual Studio's graphics debugger it appears that nothing is being written to the stream output buffer; it remains 40 bytes in size with every element being 0. This is despite the stream output buffer being assigned and the shader outputting geometry. I've edited my post to include the shader creation, in case that might be incorrect.
– MattBT12
Nov 22 '18 at 1:10
Well, one thing that did strike me as a bit odd about your code up there is that your buffers seem tiny. I assumed you had a reason for that. But now that I notice that your strides are the same as the buffer sizes: You are aware of the fact that theByteWidth
is supposed to be the size of the entire buffer and not just the size of a single vertex?
– Michael Kenzel
Nov 22 '18 at 1:17
I figured as much given what the documentation does on MSDN; I must've forgotten to change it back to what it was after tinkering with it (that's what I get for programming at 1 in the morning). Right now I'm just setting the byte width to be a very large value that doesn't change because I don't know the number of vertices that will be in the buffer. Is this the best approach for this situation? Or is there a strategy for dynamically sizing the buffer?
– MattBT12
Nov 22 '18 at 1:57
Well, setting it to "a very large value" should be good enough to make it do something (as long as that very large value is not so large that the allocation fails, of course). Obviously, it's not the best approach in general to just blindly allocate some arbitrary, huge amount of memory. In the case of stream output, you should have at least some idea how much geometry your application is going to process and be able to derive some worst-case size that the buffer needs to be!?
– Michael Kenzel
Nov 22 '18 at 10:43
I do know roughly how much data will be output, but sizing the buffer for a worst case scenario seems somewhat inefficient. I was just curious to see if there's a better way of doing it that I hadn't thought of.
– MattBT12
Nov 22 '18 at 21:39
|
show 1 more comment
In addition to just the actual vertex data, buffers used as targets for the Stream Output Stage maintain some internal state to keep track of how much geometry was written out. DrawAuto()
relies on this information to know how much geometry to draw. When you copy just the vertex data out of your stream output buffer into a plain D3D11_BIND_VERTEX_BUFFER
, this information will be lost.
Relevant quote from the documentation:
DrawAuto only works when drawing with one input buffer bound as an input to the IA stage at slot 0. Applications must create the SO buffer resource with both binding flags, D3D11_BIND_VERTEX_BUFFER and D3D11_BIND_STREAM_OUTPUT.
I'm not sure if it's supposed to be possible to copy data from one stream output buffer to another and DrawAuto()
from there. I guess that might work. What you are doing at the moment, however, is definitely not supposed to work according to the documentation. Ideally, you'd just avoid copying the data. If you really must copy the data (for whatever reason), I suggest you nevertheless first try drawing directly from the buffer you streamed into to make sure everything else is set up correctly and only once that works try to copy into another buffer that has both vertex and stream output bind flags set…
I've fixed this, but after running the shader through Visual Studio's graphics debugger it appears that nothing is being written to the stream output buffer; it remains 40 bytes in size with every element being 0. This is despite the stream output buffer being assigned and the shader outputting geometry. I've edited my post to include the shader creation, in case that might be incorrect.
– MattBT12
Nov 22 '18 at 1:10
Well, one thing that did strike me as a bit odd about your code up there is that your buffers seem tiny. I assumed you had a reason for that. But now that I notice that your strides are the same as the buffer sizes: You are aware of the fact that theByteWidth
is supposed to be the size of the entire buffer and not just the size of a single vertex?
– Michael Kenzel
Nov 22 '18 at 1:17
I figured as much given what the documentation does on MSDN; I must've forgotten to change it back to what it was after tinkering with it (that's what I get for programming at 1 in the morning). Right now I'm just setting the byte width to be a very large value that doesn't change because I don't know the number of vertices that will be in the buffer. Is this the best approach for this situation? Or is there a strategy for dynamically sizing the buffer?
– MattBT12
Nov 22 '18 at 1:57
Well, setting it to "a very large value" should be good enough to make it do something (as long as that very large value is not so large that the allocation fails, of course). Obviously, it's not the best approach in general to just blindly allocate some arbitrary, huge amount of memory. In the case of stream output, you should have at least some idea how much geometry your application is going to process and be able to derive some worst-case size that the buffer needs to be!?
– Michael Kenzel
Nov 22 '18 at 10:43
I do know roughly how much data will be output, but sizing the buffer for a worst case scenario seems somewhat inefficient. I was just curious to see if there's a better way of doing it that I hadn't thought of.
– MattBT12
Nov 22 '18 at 21:39
|
show 1 more comment
In addition to just the actual vertex data, buffers used as targets for the Stream Output Stage maintain some internal state to keep track of how much geometry was written out. DrawAuto()
relies on this information to know how much geometry to draw. When you copy just the vertex data out of your stream output buffer into a plain D3D11_BIND_VERTEX_BUFFER
, this information will be lost.
Relevant quote from the documentation:
DrawAuto only works when drawing with one input buffer bound as an input to the IA stage at slot 0. Applications must create the SO buffer resource with both binding flags, D3D11_BIND_VERTEX_BUFFER and D3D11_BIND_STREAM_OUTPUT.
I'm not sure if it's supposed to be possible to copy data from one stream output buffer to another and DrawAuto()
from there. I guess that might work. What you are doing at the moment, however, is definitely not supposed to work according to the documentation. Ideally, you'd just avoid copying the data. If you really must copy the data (for whatever reason), I suggest you nevertheless first try drawing directly from the buffer you streamed into to make sure everything else is set up correctly and only once that works try to copy into another buffer that has both vertex and stream output bind flags set…
In addition to just the actual vertex data, buffers used as targets for the Stream Output Stage maintain some internal state to keep track of how much geometry was written out. DrawAuto()
relies on this information to know how much geometry to draw. When you copy just the vertex data out of your stream output buffer into a plain D3D11_BIND_VERTEX_BUFFER
, this information will be lost.
Relevant quote from the documentation:
DrawAuto only works when drawing with one input buffer bound as an input to the IA stage at slot 0. Applications must create the SO buffer resource with both binding flags, D3D11_BIND_VERTEX_BUFFER and D3D11_BIND_STREAM_OUTPUT.
I'm not sure if it's supposed to be possible to copy data from one stream output buffer to another and DrawAuto()
from there. I guess that might work. What you are doing at the moment, however, is definitely not supposed to work according to the documentation. Ideally, you'd just avoid copying the data. If you really must copy the data (for whatever reason), I suggest you nevertheless first try drawing directly from the buffer you streamed into to make sure everything else is set up correctly and only once that works try to copy into another buffer that has both vertex and stream output bind flags set…
edited Nov 22 '18 at 0:55
answered Nov 22 '18 at 0:39


Michael KenzelMichael Kenzel
4,043719
4,043719
I've fixed this, but after running the shader through Visual Studio's graphics debugger it appears that nothing is being written to the stream output buffer; it remains 40 bytes in size with every element being 0. This is despite the stream output buffer being assigned and the shader outputting geometry. I've edited my post to include the shader creation, in case that might be incorrect.
– MattBT12
Nov 22 '18 at 1:10
Well, one thing that did strike me as a bit odd about your code up there is that your buffers seem tiny. I assumed you had a reason for that. But now that I notice that your strides are the same as the buffer sizes: You are aware of the fact that theByteWidth
is supposed to be the size of the entire buffer and not just the size of a single vertex?
– Michael Kenzel
Nov 22 '18 at 1:17
I figured as much given what the documentation does on MSDN; I must've forgotten to change it back to what it was after tinkering with it (that's what I get for programming at 1 in the morning). Right now I'm just setting the byte width to be a very large value that doesn't change because I don't know the number of vertices that will be in the buffer. Is this the best approach for this situation? Or is there a strategy for dynamically sizing the buffer?
– MattBT12
Nov 22 '18 at 1:57
Well, setting it to "a very large value" should be good enough to make it do something (as long as that very large value is not so large that the allocation fails, of course). Obviously, it's not the best approach in general to just blindly allocate some arbitrary, huge amount of memory. In the case of stream output, you should have at least some idea how much geometry your application is going to process and be able to derive some worst-case size that the buffer needs to be!?
– Michael Kenzel
Nov 22 '18 at 10:43
I do know roughly how much data will be output, but sizing the buffer for a worst case scenario seems somewhat inefficient. I was just curious to see if there's a better way of doing it that I hadn't thought of.
– MattBT12
Nov 22 '18 at 21:39
|
show 1 more comment
I've fixed this, but after running the shader through Visual Studio's graphics debugger it appears that nothing is being written to the stream output buffer; it remains 40 bytes in size with every element being 0. This is despite the stream output buffer being assigned and the shader outputting geometry. I've edited my post to include the shader creation, in case that might be incorrect.
– MattBT12
Nov 22 '18 at 1:10
Well, one thing that did strike me as a bit odd about your code up there is that your buffers seem tiny. I assumed you had a reason for that. But now that I notice that your strides are the same as the buffer sizes: You are aware of the fact that theByteWidth
is supposed to be the size of the entire buffer and not just the size of a single vertex?
– Michael Kenzel
Nov 22 '18 at 1:17
I figured as much given what the documentation does on MSDN; I must've forgotten to change it back to what it was after tinkering with it (that's what I get for programming at 1 in the morning). Right now I'm just setting the byte width to be a very large value that doesn't change because I don't know the number of vertices that will be in the buffer. Is this the best approach for this situation? Or is there a strategy for dynamically sizing the buffer?
– MattBT12
Nov 22 '18 at 1:57
Well, setting it to "a very large value" should be good enough to make it do something (as long as that very large value is not so large that the allocation fails, of course). Obviously, it's not the best approach in general to just blindly allocate some arbitrary, huge amount of memory. In the case of stream output, you should have at least some idea how much geometry your application is going to process and be able to derive some worst-case size that the buffer needs to be!?
– Michael Kenzel
Nov 22 '18 at 10:43
I do know roughly how much data will be output, but sizing the buffer for a worst case scenario seems somewhat inefficient. I was just curious to see if there's a better way of doing it that I hadn't thought of.
– MattBT12
Nov 22 '18 at 21:39
I've fixed this, but after running the shader through Visual Studio's graphics debugger it appears that nothing is being written to the stream output buffer; it remains 40 bytes in size with every element being 0. This is despite the stream output buffer being assigned and the shader outputting geometry. I've edited my post to include the shader creation, in case that might be incorrect.
– MattBT12
Nov 22 '18 at 1:10
I've fixed this, but after running the shader through Visual Studio's graphics debugger it appears that nothing is being written to the stream output buffer; it remains 40 bytes in size with every element being 0. This is despite the stream output buffer being assigned and the shader outputting geometry. I've edited my post to include the shader creation, in case that might be incorrect.
– MattBT12
Nov 22 '18 at 1:10
Well, one thing that did strike me as a bit odd about your code up there is that your buffers seem tiny. I assumed you had a reason for that. But now that I notice that your strides are the same as the buffer sizes: You are aware of the fact that the
ByteWidth
is supposed to be the size of the entire buffer and not just the size of a single vertex?– Michael Kenzel
Nov 22 '18 at 1:17
Well, one thing that did strike me as a bit odd about your code up there is that your buffers seem tiny. I assumed you had a reason for that. But now that I notice that your strides are the same as the buffer sizes: You are aware of the fact that the
ByteWidth
is supposed to be the size of the entire buffer and not just the size of a single vertex?– Michael Kenzel
Nov 22 '18 at 1:17
I figured as much given what the documentation does on MSDN; I must've forgotten to change it back to what it was after tinkering with it (that's what I get for programming at 1 in the morning). Right now I'm just setting the byte width to be a very large value that doesn't change because I don't know the number of vertices that will be in the buffer. Is this the best approach for this situation? Or is there a strategy for dynamically sizing the buffer?
– MattBT12
Nov 22 '18 at 1:57
I figured as much given what the documentation does on MSDN; I must've forgotten to change it back to what it was after tinkering with it (that's what I get for programming at 1 in the morning). Right now I'm just setting the byte width to be a very large value that doesn't change because I don't know the number of vertices that will be in the buffer. Is this the best approach for this situation? Or is there a strategy for dynamically sizing the buffer?
– MattBT12
Nov 22 '18 at 1:57
Well, setting it to "a very large value" should be good enough to make it do something (as long as that very large value is not so large that the allocation fails, of course). Obviously, it's not the best approach in general to just blindly allocate some arbitrary, huge amount of memory. In the case of stream output, you should have at least some idea how much geometry your application is going to process and be able to derive some worst-case size that the buffer needs to be!?
– Michael Kenzel
Nov 22 '18 at 10:43
Well, setting it to "a very large value" should be good enough to make it do something (as long as that very large value is not so large that the allocation fails, of course). Obviously, it's not the best approach in general to just blindly allocate some arbitrary, huge amount of memory. In the case of stream output, you should have at least some idea how much geometry your application is going to process and be able to derive some worst-case size that the buffer needs to be!?
– Michael Kenzel
Nov 22 '18 at 10:43
I do know roughly how much data will be output, but sizing the buffer for a worst case scenario seems somewhat inefficient. I was just curious to see if there's a better way of doing it that I hadn't thought of.
– MattBT12
Nov 22 '18 at 21:39
I do know roughly how much data will be output, but sizing the buffer for a worst case scenario seems somewhat inefficient. I was just curious to see if there's a better way of doing it that I hadn't thought of.
– MattBT12
Nov 22 '18 at 21:39
|
show 1 more comment
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53419965%2fdirectx11-geometry-shader-with-stream-output%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
7,lkc4og2,twVSifnNUzkS 27aFGsKoQmPaE3v,wOUFB3Zhw7 lNm9STb,daN90M hfHY QG5