Django - how to pass filtered querysets to Detailview
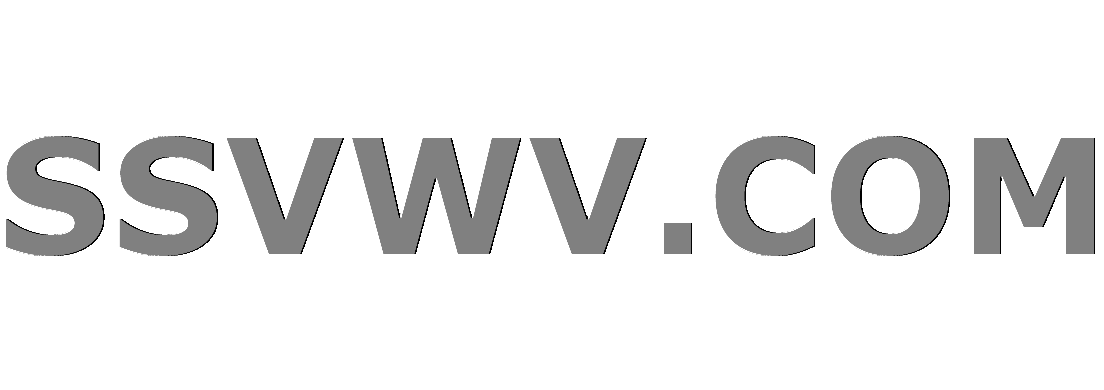
Multi tool use
I have Client, servers, users models. In the Client Detailview i would like to display the servers and users that belong to that company. How do I filter that?
models.py
class Client(models.Model):
name = models.CharField(max_length=50)
def __str__(self):
return self.name
class Server(models.Model):
company = models.ForeignKey(Client, on_delete=models.CASCADE)
name = models.CharField(max_length=50)
def __str__(self):
return self.name
class User(models.Model):
company = models.ForeignKey(Client, verbose_name="Company", on_delete=models.CASCADE)
first_name = models.CharField(max_length=50)
last_name = models.CharField(max_length=50)
def __str__(self):
return self.first_name + ' ' + self.last_name
views.py
class clientdetails(LoginRequiredMixin, DetailView):
template_name = 'myapp/clientdetails.html'
def get_queryset(self):
return Client.objects.all()
def get_context_data(self, **kwargs):
context = super(clientdetails, self).get_context_data(**kwargs)
context['servers'] = Server.objects.filter(** servers belonging to that client **)
context['users'] = User.objects.filter(** servers belonging to that client **)
return context
How do I achieve this?
python django
add a comment |
I have Client, servers, users models. In the Client Detailview i would like to display the servers and users that belong to that company. How do I filter that?
models.py
class Client(models.Model):
name = models.CharField(max_length=50)
def __str__(self):
return self.name
class Server(models.Model):
company = models.ForeignKey(Client, on_delete=models.CASCADE)
name = models.CharField(max_length=50)
def __str__(self):
return self.name
class User(models.Model):
company = models.ForeignKey(Client, verbose_name="Company", on_delete=models.CASCADE)
first_name = models.CharField(max_length=50)
last_name = models.CharField(max_length=50)
def __str__(self):
return self.first_name + ' ' + self.last_name
views.py
class clientdetails(LoginRequiredMixin, DetailView):
template_name = 'myapp/clientdetails.html'
def get_queryset(self):
return Client.objects.all()
def get_context_data(self, **kwargs):
context = super(clientdetails, self).get_context_data(**kwargs)
context['servers'] = Server.objects.filter(** servers belonging to that client **)
context['users'] = User.objects.filter(** servers belonging to that client **)
return context
How do I achieve this?
python django
add a comment |
I have Client, servers, users models. In the Client Detailview i would like to display the servers and users that belong to that company. How do I filter that?
models.py
class Client(models.Model):
name = models.CharField(max_length=50)
def __str__(self):
return self.name
class Server(models.Model):
company = models.ForeignKey(Client, on_delete=models.CASCADE)
name = models.CharField(max_length=50)
def __str__(self):
return self.name
class User(models.Model):
company = models.ForeignKey(Client, verbose_name="Company", on_delete=models.CASCADE)
first_name = models.CharField(max_length=50)
last_name = models.CharField(max_length=50)
def __str__(self):
return self.first_name + ' ' + self.last_name
views.py
class clientdetails(LoginRequiredMixin, DetailView):
template_name = 'myapp/clientdetails.html'
def get_queryset(self):
return Client.objects.all()
def get_context_data(self, **kwargs):
context = super(clientdetails, self).get_context_data(**kwargs)
context['servers'] = Server.objects.filter(** servers belonging to that client **)
context['users'] = User.objects.filter(** servers belonging to that client **)
return context
How do I achieve this?
python django
I have Client, servers, users models. In the Client Detailview i would like to display the servers and users that belong to that company. How do I filter that?
models.py
class Client(models.Model):
name = models.CharField(max_length=50)
def __str__(self):
return self.name
class Server(models.Model):
company = models.ForeignKey(Client, on_delete=models.CASCADE)
name = models.CharField(max_length=50)
def __str__(self):
return self.name
class User(models.Model):
company = models.ForeignKey(Client, verbose_name="Company", on_delete=models.CASCADE)
first_name = models.CharField(max_length=50)
last_name = models.CharField(max_length=50)
def __str__(self):
return self.first_name + ' ' + self.last_name
views.py
class clientdetails(LoginRequiredMixin, DetailView):
template_name = 'myapp/clientdetails.html'
def get_queryset(self):
return Client.objects.all()
def get_context_data(self, **kwargs):
context = super(clientdetails, self).get_context_data(**kwargs)
context['servers'] = Server.objects.filter(** servers belonging to that client **)
context['users'] = User.objects.filter(** servers belonging to that client **)
return context
How do I achieve this?
python django
python django
asked Nov 21 '18 at 19:55
kfkenshinkfkenshin
132
132
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
If you want this to be a general use case i.e all objects retrieved from the server need to be for a specific client. You can take a look at using limit-choices-to
class ClientDetails(LoginRequiredMixin, DetailView):
template_name = 'myapp/clientdetails.html'
model = Client
def get_context_data(self, **kwargs):
client = self.object
context = super(ClientDetails, self).get_context_data(**kwargs)
context['servers'] = Server.objects.filter(company=client)
context['users'] = User.objects.filter(company=client)
return context
OR using the related names
def get_context_data(self, **kwargs):
context = super(ClientDetails, self).get_context_data(**kwargs)
context['servers'] = self.object.server_set.all()
context['users'] = self.object.user_set.all()
return context
ah.. client = self.object, that's what I missed. thx.
– kfkenshin
Nov 22 '18 at 14:35
add a comment |
You don't really need to do this in the view at all; you can follow the relationships in the template.
{{ object.name }}
{% for server in object.server_set.all %}
{{ server.name }}
{% endfor %}
{% for user in object.user_set.all %}
{{ user.first_name }}
{% endfor %}
Now you can remove your get_context_data method altogether.
thanks you. I haven't tested this but this is a good idea. but I prefer to do it in the view and keep the templates "simple".
– kfkenshin
Nov 22 '18 at 14:37
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53419608%2fdjango-how-to-pass-filtered-querysets-to-detailview%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
If you want this to be a general use case i.e all objects retrieved from the server need to be for a specific client. You can take a look at using limit-choices-to
class ClientDetails(LoginRequiredMixin, DetailView):
template_name = 'myapp/clientdetails.html'
model = Client
def get_context_data(self, **kwargs):
client = self.object
context = super(ClientDetails, self).get_context_data(**kwargs)
context['servers'] = Server.objects.filter(company=client)
context['users'] = User.objects.filter(company=client)
return context
OR using the related names
def get_context_data(self, **kwargs):
context = super(ClientDetails, self).get_context_data(**kwargs)
context['servers'] = self.object.server_set.all()
context['users'] = self.object.user_set.all()
return context
ah.. client = self.object, that's what I missed. thx.
– kfkenshin
Nov 22 '18 at 14:35
add a comment |
If you want this to be a general use case i.e all objects retrieved from the server need to be for a specific client. You can take a look at using limit-choices-to
class ClientDetails(LoginRequiredMixin, DetailView):
template_name = 'myapp/clientdetails.html'
model = Client
def get_context_data(self, **kwargs):
client = self.object
context = super(ClientDetails, self).get_context_data(**kwargs)
context['servers'] = Server.objects.filter(company=client)
context['users'] = User.objects.filter(company=client)
return context
OR using the related names
def get_context_data(self, **kwargs):
context = super(ClientDetails, self).get_context_data(**kwargs)
context['servers'] = self.object.server_set.all()
context['users'] = self.object.user_set.all()
return context
ah.. client = self.object, that's what I missed. thx.
– kfkenshin
Nov 22 '18 at 14:35
add a comment |
If you want this to be a general use case i.e all objects retrieved from the server need to be for a specific client. You can take a look at using limit-choices-to
class ClientDetails(LoginRequiredMixin, DetailView):
template_name = 'myapp/clientdetails.html'
model = Client
def get_context_data(self, **kwargs):
client = self.object
context = super(ClientDetails, self).get_context_data(**kwargs)
context['servers'] = Server.objects.filter(company=client)
context['users'] = User.objects.filter(company=client)
return context
OR using the related names
def get_context_data(self, **kwargs):
context = super(ClientDetails, self).get_context_data(**kwargs)
context['servers'] = self.object.server_set.all()
context['users'] = self.object.user_set.all()
return context
If you want this to be a general use case i.e all objects retrieved from the server need to be for a specific client. You can take a look at using limit-choices-to
class ClientDetails(LoginRequiredMixin, DetailView):
template_name = 'myapp/clientdetails.html'
model = Client
def get_context_data(self, **kwargs):
client = self.object
context = super(ClientDetails, self).get_context_data(**kwargs)
context['servers'] = Server.objects.filter(company=client)
context['users'] = User.objects.filter(company=client)
return context
OR using the related names
def get_context_data(self, **kwargs):
context = super(ClientDetails, self).get_context_data(**kwargs)
context['servers'] = self.object.server_set.all()
context['users'] = self.object.user_set.all()
return context
edited Nov 22 '18 at 16:35
answered Nov 21 '18 at 20:06
jackotonyejackotonye
1,3901121
1,3901121
ah.. client = self.object, that's what I missed. thx.
– kfkenshin
Nov 22 '18 at 14:35
add a comment |
ah.. client = self.object, that's what I missed. thx.
– kfkenshin
Nov 22 '18 at 14:35
ah.. client = self.object, that's what I missed. thx.
– kfkenshin
Nov 22 '18 at 14:35
ah.. client = self.object, that's what I missed. thx.
– kfkenshin
Nov 22 '18 at 14:35
add a comment |
You don't really need to do this in the view at all; you can follow the relationships in the template.
{{ object.name }}
{% for server in object.server_set.all %}
{{ server.name }}
{% endfor %}
{% for user in object.user_set.all %}
{{ user.first_name }}
{% endfor %}
Now you can remove your get_context_data method altogether.
thanks you. I haven't tested this but this is a good idea. but I prefer to do it in the view and keep the templates "simple".
– kfkenshin
Nov 22 '18 at 14:37
add a comment |
You don't really need to do this in the view at all; you can follow the relationships in the template.
{{ object.name }}
{% for server in object.server_set.all %}
{{ server.name }}
{% endfor %}
{% for user in object.user_set.all %}
{{ user.first_name }}
{% endfor %}
Now you can remove your get_context_data method altogether.
thanks you. I haven't tested this but this is a good idea. but I prefer to do it in the view and keep the templates "simple".
– kfkenshin
Nov 22 '18 at 14:37
add a comment |
You don't really need to do this in the view at all; you can follow the relationships in the template.
{{ object.name }}
{% for server in object.server_set.all %}
{{ server.name }}
{% endfor %}
{% for user in object.user_set.all %}
{{ user.first_name }}
{% endfor %}
Now you can remove your get_context_data method altogether.
You don't really need to do this in the view at all; you can follow the relationships in the template.
{{ object.name }}
{% for server in object.server_set.all %}
{{ server.name }}
{% endfor %}
{% for user in object.user_set.all %}
{{ user.first_name }}
{% endfor %}
Now you can remove your get_context_data method altogether.
answered Nov 21 '18 at 21:01
Daniel RosemanDaniel Roseman
445k41576632
445k41576632
thanks you. I haven't tested this but this is a good idea. but I prefer to do it in the view and keep the templates "simple".
– kfkenshin
Nov 22 '18 at 14:37
add a comment |
thanks you. I haven't tested this but this is a good idea. but I prefer to do it in the view and keep the templates "simple".
– kfkenshin
Nov 22 '18 at 14:37
thanks you. I haven't tested this but this is a good idea. but I prefer to do it in the view and keep the templates "simple".
– kfkenshin
Nov 22 '18 at 14:37
thanks you. I haven't tested this but this is a good idea. but I prefer to do it in the view and keep the templates "simple".
– kfkenshin
Nov 22 '18 at 14:37
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53419608%2fdjango-how-to-pass-filtered-querysets-to-detailview%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
LUigI137erSk bEz