C++/WinApi Memory Usage rising when moving window
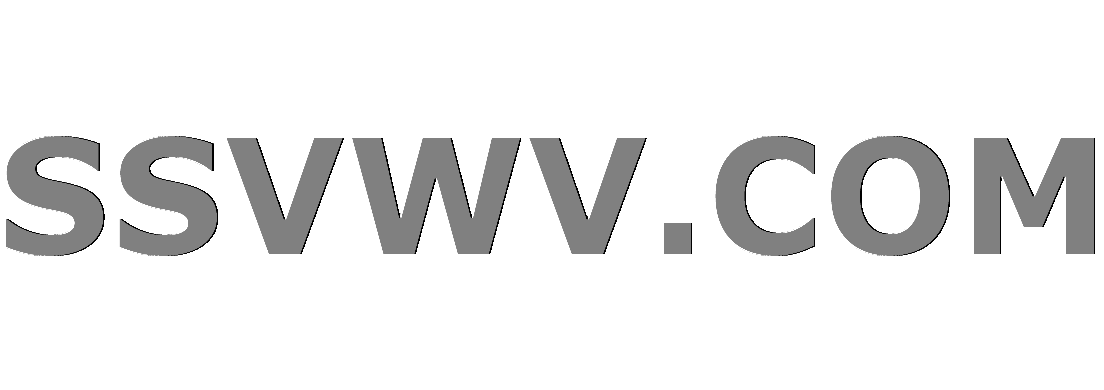
Multi tool use
So i was playing around with my test application and noticed ram usage is rising when i move my window to the edges of the screen.
Also at 19mb ram usage, the fonts disappear and the app gets buggy.
However this does not happen when the window is not touching the edges of the screen.
Here is a youtube video of me showing what i mean.
I think my code is just not very efficient.
Code for drawing text:
void drawText(LPCWSTR text, LPCWSTR fontName,int fontSize, int x, int y, HDC hdc) {
//Creat font from name and size
HFONT font = CreateFont(
fontSize,
0,
0,
0,
FW_NORMAL,
FALSE,
FALSE,
FALSE,
ANSI_CHARSET,
OUT_CHARACTER_PRECIS,
CLIP_CHARACTER_PRECIS,
CLEARTYPE_QUALITY,
DEFAULT_PITCH,
fontName);
//Change Current Font
HFONT oldFont = (HFONT) SelectObject(hdc, font);
//Draw Text
TextOut(hdc,
x,
y,
text,
wcslen(text));
//Set back old font
SelectObject(hdc, oldFont);
}
Implementation:
case WM_PAINT:
PAINTSTRUCT ps;
HDC hdc = BeginPaint(hwnd, &ps);
int y = 10;
drawText(L"Hey, im Roboto", L"Roboto", 50, 10, y, hdc);
y += 80;
drawText(L"Hey, im Arial", L"Arial",50, 10, y, hdc);
y += 80;
drawText(L"Hey, im Lucida Grande", L"Lucida Grande", 50, 10, y, hdc);
EndPaint(hwnd, &ps);
break;
c++ user-interface winapi
add a comment |
So i was playing around with my test application and noticed ram usage is rising when i move my window to the edges of the screen.
Also at 19mb ram usage, the fonts disappear and the app gets buggy.
However this does not happen when the window is not touching the edges of the screen.
Here is a youtube video of me showing what i mean.
I think my code is just not very efficient.
Code for drawing text:
void drawText(LPCWSTR text, LPCWSTR fontName,int fontSize, int x, int y, HDC hdc) {
//Creat font from name and size
HFONT font = CreateFont(
fontSize,
0,
0,
0,
FW_NORMAL,
FALSE,
FALSE,
FALSE,
ANSI_CHARSET,
OUT_CHARACTER_PRECIS,
CLIP_CHARACTER_PRECIS,
CLEARTYPE_QUALITY,
DEFAULT_PITCH,
fontName);
//Change Current Font
HFONT oldFont = (HFONT) SelectObject(hdc, font);
//Draw Text
TextOut(hdc,
x,
y,
text,
wcslen(text));
//Set back old font
SelectObject(hdc, oldFont);
}
Implementation:
case WM_PAINT:
PAINTSTRUCT ps;
HDC hdc = BeginPaint(hwnd, &ps);
int y = 10;
drawText(L"Hey, im Roboto", L"Roboto", 50, 10, y, hdc);
y += 80;
drawText(L"Hey, im Arial", L"Arial",50, 10, y, hdc);
y += 80;
drawText(L"Hey, im Lucida Grande", L"Lucida Grande", 50, 10, y, hdc);
EndPaint(hwnd, &ps);
break;
c++ user-interface winapi
7
It looks like you forgot to delete the font.
– Raymond Chen
Nov 24 '18 at 15:43
Thanks DeleteObject(font); did solve the Problem. But i would like to know the reason of the memory rising.
– Brian Engel
Nov 24 '18 at 15:53
1
You aren't releasing resources after use. Why would you expect memory usage to not increase?
– IInspectable
Nov 24 '18 at 16:14
add a comment |
So i was playing around with my test application and noticed ram usage is rising when i move my window to the edges of the screen.
Also at 19mb ram usage, the fonts disappear and the app gets buggy.
However this does not happen when the window is not touching the edges of the screen.
Here is a youtube video of me showing what i mean.
I think my code is just not very efficient.
Code for drawing text:
void drawText(LPCWSTR text, LPCWSTR fontName,int fontSize, int x, int y, HDC hdc) {
//Creat font from name and size
HFONT font = CreateFont(
fontSize,
0,
0,
0,
FW_NORMAL,
FALSE,
FALSE,
FALSE,
ANSI_CHARSET,
OUT_CHARACTER_PRECIS,
CLIP_CHARACTER_PRECIS,
CLEARTYPE_QUALITY,
DEFAULT_PITCH,
fontName);
//Change Current Font
HFONT oldFont = (HFONT) SelectObject(hdc, font);
//Draw Text
TextOut(hdc,
x,
y,
text,
wcslen(text));
//Set back old font
SelectObject(hdc, oldFont);
}
Implementation:
case WM_PAINT:
PAINTSTRUCT ps;
HDC hdc = BeginPaint(hwnd, &ps);
int y = 10;
drawText(L"Hey, im Roboto", L"Roboto", 50, 10, y, hdc);
y += 80;
drawText(L"Hey, im Arial", L"Arial",50, 10, y, hdc);
y += 80;
drawText(L"Hey, im Lucida Grande", L"Lucida Grande", 50, 10, y, hdc);
EndPaint(hwnd, &ps);
break;
c++ user-interface winapi
So i was playing around with my test application and noticed ram usage is rising when i move my window to the edges of the screen.
Also at 19mb ram usage, the fonts disappear and the app gets buggy.
However this does not happen when the window is not touching the edges of the screen.
Here is a youtube video of me showing what i mean.
I think my code is just not very efficient.
Code for drawing text:
void drawText(LPCWSTR text, LPCWSTR fontName,int fontSize, int x, int y, HDC hdc) {
//Creat font from name and size
HFONT font = CreateFont(
fontSize,
0,
0,
0,
FW_NORMAL,
FALSE,
FALSE,
FALSE,
ANSI_CHARSET,
OUT_CHARACTER_PRECIS,
CLIP_CHARACTER_PRECIS,
CLEARTYPE_QUALITY,
DEFAULT_PITCH,
fontName);
//Change Current Font
HFONT oldFont = (HFONT) SelectObject(hdc, font);
//Draw Text
TextOut(hdc,
x,
y,
text,
wcslen(text));
//Set back old font
SelectObject(hdc, oldFont);
}
Implementation:
case WM_PAINT:
PAINTSTRUCT ps;
HDC hdc = BeginPaint(hwnd, &ps);
int y = 10;
drawText(L"Hey, im Roboto", L"Roboto", 50, 10, y, hdc);
y += 80;
drawText(L"Hey, im Arial", L"Arial",50, 10, y, hdc);
y += 80;
drawText(L"Hey, im Lucida Grande", L"Lucida Grande", 50, 10, y, hdc);
EndPaint(hwnd, &ps);
break;
c++ user-interface winapi
c++ user-interface winapi
asked Nov 24 '18 at 15:41


Brian EngelBrian Engel
11
11
7
It looks like you forgot to delete the font.
– Raymond Chen
Nov 24 '18 at 15:43
Thanks DeleteObject(font); did solve the Problem. But i would like to know the reason of the memory rising.
– Brian Engel
Nov 24 '18 at 15:53
1
You aren't releasing resources after use. Why would you expect memory usage to not increase?
– IInspectable
Nov 24 '18 at 16:14
add a comment |
7
It looks like you forgot to delete the font.
– Raymond Chen
Nov 24 '18 at 15:43
Thanks DeleteObject(font); did solve the Problem. But i would like to know the reason of the memory rising.
– Brian Engel
Nov 24 '18 at 15:53
1
You aren't releasing resources after use. Why would you expect memory usage to not increase?
– IInspectable
Nov 24 '18 at 16:14
7
7
It looks like you forgot to delete the font.
– Raymond Chen
Nov 24 '18 at 15:43
It looks like you forgot to delete the font.
– Raymond Chen
Nov 24 '18 at 15:43
Thanks DeleteObject(font); did solve the Problem. But i would like to know the reason of the memory rising.
– Brian Engel
Nov 24 '18 at 15:53
Thanks DeleteObject(font); did solve the Problem. But i would like to know the reason of the memory rising.
– Brian Engel
Nov 24 '18 at 15:53
1
1
You aren't releasing resources after use. Why would you expect memory usage to not increase?
– IInspectable
Nov 24 '18 at 16:14
You aren't releasing resources after use. Why would you expect memory usage to not increase?
– IInspectable
Nov 24 '18 at 16:14
add a comment |
1 Answer
1
active
oldest
votes
This seems to be a usual case of a memory leak. According to MSDN:
The WM_PAINT message is sent when the system or another application makes a request to paint a portion of an application's window.
This means that the WM_PAINT message will be sent to your WindowProc callback function every time the system wants to request the program to redraw the window. And if you're moving the window using the mouse, it gets redrawn every time the window changes position at all! You can play around with Control Spy v2.0 to see that this is indeed the case.
Either way, you're creating a new font every time the window needs to be redrawn for any reason. Such as when it gets moved even a little bit. This way, the memory usage will increase fast. Whereas if you delete the font using DeleteObject after applying it to the text, it deallocates the font from memory, so no real memory increase is noticeable.
This is not just a memory leak. It is a resource leak. Different objects are allocated from different memory. While the process heap is usually available in exuberant amounts, GDI objects (like fonts) are allocated from a heap far more limited in size.
– IInspectable
Nov 24 '18 at 18:43
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53459743%2fc-winapi-memory-usage-rising-when-moving-window%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
This seems to be a usual case of a memory leak. According to MSDN:
The WM_PAINT message is sent when the system or another application makes a request to paint a portion of an application's window.
This means that the WM_PAINT message will be sent to your WindowProc callback function every time the system wants to request the program to redraw the window. And if you're moving the window using the mouse, it gets redrawn every time the window changes position at all! You can play around with Control Spy v2.0 to see that this is indeed the case.
Either way, you're creating a new font every time the window needs to be redrawn for any reason. Such as when it gets moved even a little bit. This way, the memory usage will increase fast. Whereas if you delete the font using DeleteObject after applying it to the text, it deallocates the font from memory, so no real memory increase is noticeable.
This is not just a memory leak. It is a resource leak. Different objects are allocated from different memory. While the process heap is usually available in exuberant amounts, GDI objects (like fonts) are allocated from a heap far more limited in size.
– IInspectable
Nov 24 '18 at 18:43
add a comment |
This seems to be a usual case of a memory leak. According to MSDN:
The WM_PAINT message is sent when the system or another application makes a request to paint a portion of an application's window.
This means that the WM_PAINT message will be sent to your WindowProc callback function every time the system wants to request the program to redraw the window. And if you're moving the window using the mouse, it gets redrawn every time the window changes position at all! You can play around with Control Spy v2.0 to see that this is indeed the case.
Either way, you're creating a new font every time the window needs to be redrawn for any reason. Such as when it gets moved even a little bit. This way, the memory usage will increase fast. Whereas if you delete the font using DeleteObject after applying it to the text, it deallocates the font from memory, so no real memory increase is noticeable.
This is not just a memory leak. It is a resource leak. Different objects are allocated from different memory. While the process heap is usually available in exuberant amounts, GDI objects (like fonts) are allocated from a heap far more limited in size.
– IInspectable
Nov 24 '18 at 18:43
add a comment |
This seems to be a usual case of a memory leak. According to MSDN:
The WM_PAINT message is sent when the system or another application makes a request to paint a portion of an application's window.
This means that the WM_PAINT message will be sent to your WindowProc callback function every time the system wants to request the program to redraw the window. And if you're moving the window using the mouse, it gets redrawn every time the window changes position at all! You can play around with Control Spy v2.0 to see that this is indeed the case.
Either way, you're creating a new font every time the window needs to be redrawn for any reason. Such as when it gets moved even a little bit. This way, the memory usage will increase fast. Whereas if you delete the font using DeleteObject after applying it to the text, it deallocates the font from memory, so no real memory increase is noticeable.
This seems to be a usual case of a memory leak. According to MSDN:
The WM_PAINT message is sent when the system or another application makes a request to paint a portion of an application's window.
This means that the WM_PAINT message will be sent to your WindowProc callback function every time the system wants to request the program to redraw the window. And if you're moving the window using the mouse, it gets redrawn every time the window changes position at all! You can play around with Control Spy v2.0 to see that this is indeed the case.
Either way, you're creating a new font every time the window needs to be redrawn for any reason. Such as when it gets moved even a little bit. This way, the memory usage will increase fast. Whereas if you delete the font using DeleteObject after applying it to the text, it deallocates the font from memory, so no real memory increase is noticeable.
edited Nov 24 '18 at 16:57
Remy Lebeau
339k19262456
339k19262456
answered Nov 24 '18 at 16:19
KirkoKirko
343
343
This is not just a memory leak. It is a resource leak. Different objects are allocated from different memory. While the process heap is usually available in exuberant amounts, GDI objects (like fonts) are allocated from a heap far more limited in size.
– IInspectable
Nov 24 '18 at 18:43
add a comment |
This is not just a memory leak. It is a resource leak. Different objects are allocated from different memory. While the process heap is usually available in exuberant amounts, GDI objects (like fonts) are allocated from a heap far more limited in size.
– IInspectable
Nov 24 '18 at 18:43
This is not just a memory leak. It is a resource leak. Different objects are allocated from different memory. While the process heap is usually available in exuberant amounts, GDI objects (like fonts) are allocated from a heap far more limited in size.
– IInspectable
Nov 24 '18 at 18:43
This is not just a memory leak. It is a resource leak. Different objects are allocated from different memory. While the process heap is usually available in exuberant amounts, GDI objects (like fonts) are allocated from a heap far more limited in size.
– IInspectable
Nov 24 '18 at 18:43
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53459743%2fc-winapi-memory-usage-rising-when-moving-window%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
cnv Y,ZNga,d
7
It looks like you forgot to delete the font.
– Raymond Chen
Nov 24 '18 at 15:43
Thanks DeleteObject(font); did solve the Problem. But i would like to know the reason of the memory rising.
– Brian Engel
Nov 24 '18 at 15:53
1
You aren't releasing resources after use. Why would you expect memory usage to not increase?
– IInspectable
Nov 24 '18 at 16:14