Why I get 411 Length required error?
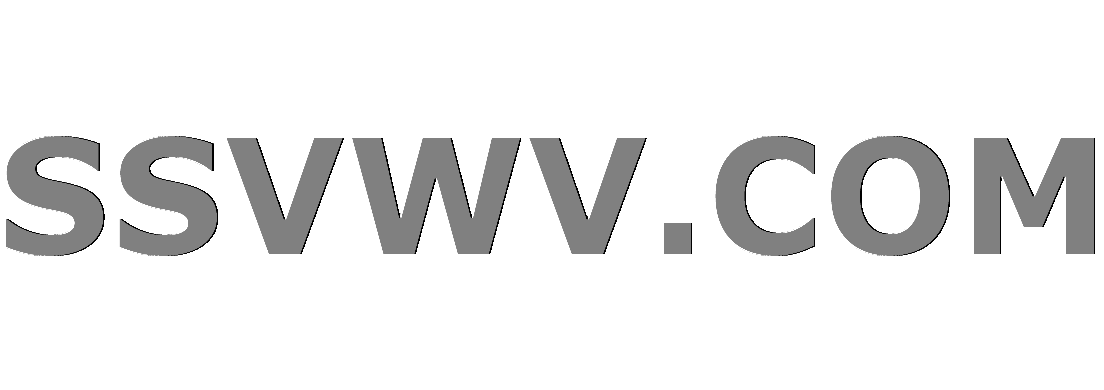
Multi tool use
This is how I call a service with .NET:
var requestedURL = "https://accounts.google.com/o/oauth2/token?code=" + code + "&client_id=" + client_id + "&client_secret=" + client_secret + "&redirect_uri=" + redirect_uri + "&grant_type=authorization_code";
HttpWebRequest authRequest = (HttpWebRequest)WebRequest.Create(requestedURL);
authRequest.ContentType = "application/x-www-form-urlencoded";
authRequest.Method = "POST";
WebResponse authResponseTwitter = authRequest.GetResponse();
but when this method is invoked, I get:
Exception Details: System.Net.WebException: The remote server returned
an error: (411) Length Required.
what should I do?
c# .net httprequest http-status-code-411
add a comment |
This is how I call a service with .NET:
var requestedURL = "https://accounts.google.com/o/oauth2/token?code=" + code + "&client_id=" + client_id + "&client_secret=" + client_secret + "&redirect_uri=" + redirect_uri + "&grant_type=authorization_code";
HttpWebRequest authRequest = (HttpWebRequest)WebRequest.Create(requestedURL);
authRequest.ContentType = "application/x-www-form-urlencoded";
authRequest.Method = "POST";
WebResponse authResponseTwitter = authRequest.GetResponse();
but when this method is invoked, I get:
Exception Details: System.Net.WebException: The remote server returned
an error: (411) Length Required.
what should I do?
c# .net httprequest http-status-code-411
add a comment |
This is how I call a service with .NET:
var requestedURL = "https://accounts.google.com/o/oauth2/token?code=" + code + "&client_id=" + client_id + "&client_secret=" + client_secret + "&redirect_uri=" + redirect_uri + "&grant_type=authorization_code";
HttpWebRequest authRequest = (HttpWebRequest)WebRequest.Create(requestedURL);
authRequest.ContentType = "application/x-www-form-urlencoded";
authRequest.Method = "POST";
WebResponse authResponseTwitter = authRequest.GetResponse();
but when this method is invoked, I get:
Exception Details: System.Net.WebException: The remote server returned
an error: (411) Length Required.
what should I do?
c# .net httprequest http-status-code-411
This is how I call a service with .NET:
var requestedURL = "https://accounts.google.com/o/oauth2/token?code=" + code + "&client_id=" + client_id + "&client_secret=" + client_secret + "&redirect_uri=" + redirect_uri + "&grant_type=authorization_code";
HttpWebRequest authRequest = (HttpWebRequest)WebRequest.Create(requestedURL);
authRequest.ContentType = "application/x-www-form-urlencoded";
authRequest.Method = "POST";
WebResponse authResponseTwitter = authRequest.GetResponse();
but when this method is invoked, I get:
Exception Details: System.Net.WebException: The remote server returned
an error: (411) Length Required.
what should I do?
c# .net httprequest http-status-code-411
c# .net httprequest http-status-code-411
edited Dec 2 '14 at 19:54
huseyint
11.3k154575
11.3k154575
asked Aug 21 '13 at 8:15
markzzzmarkzzz
19.1k92233406
19.1k92233406
add a comment |
add a comment |
8 Answers
8
active
oldest
votes
When you're using HttpWebRequest and POST method, you have to set a content (or a body if you prefer) via the RequestStream. But, according to your code, using authRequest.Method = "GET" should be enough.
In case you're wondering about POST format, here's what you have to do :
ASCIIEncoding encoder = new ASCIIEncoding();
byte data = encoder.GetBytes(serializedObject); // a json object, or xml, whatever...
HttpWebRequest request = WebRequest.Create(url) as HttpWebRequest;
request.Method = "POST";
request.ContentType = "application/json";
request.ContentLength = data.Length;
request.Expect = "application/json";
request.GetRequestStream().Write(data, 0, data.Length);
HttpWebResponse response = request.GetResponse() as HttpWebResponse;
Can you please refer to my question "stackoverflow.com/questions/35308945/…" ? I am having similar issue. I am trying to hit the SFTP URL
– Yash Saraiya
Feb 10 '16 at 9:51
add a comment |
you need to add Content-Length: 0
in your request header.
a very descriptive example of how to test is given here
Yes, adding Content-Length: 0 helped. Thanks!
– Roboblob
Apr 22 '14 at 15:29
simple and lifesaving. Thank you!
– Abraham Putra Prakasa
Jul 19 '18 at 16:28
add a comment |
When you make a POST
HttpWebRequest, you must specify the length of the data you are sending, something like:
string data = "something you need to send"
byte postBytes = Encoding.ASCII.GetBytes(data);
request.ContentLength = postBytes.Length;
if you are not sending any data, just set it to 0, that means you just have to add to your code this line:
request.ContentLength = 0;
Usually, if you are not sending any data, chosing the GET
method instead is wiser, as you can see in the HTTP RFC
thanks a lott ... it will useful for me
– Swapnil Tandel
Jun 24 '15 at 12:32
1
While customarily one would expect data to be sent with a POST, it would be incorrect and bad practice to use GET when you are modifying data on the server. A POST request should avoid any proxy caches between the client and server. GET is okay if data is being fetched from the server without the intention of anything changing on the server as a result of the request.
– Michael
Apr 4 '16 at 4:57
add a comment |
var requestedURL = "https://accounts.google.com/o/oauth2/token?code=" + code + "&client_id=" + client_id + "&client_secret=" + client_secret + "&redirect_uri=" + redirect_uri + "&grant_type=authorization_code";
HttpWebRequest authRequest = (HttpWebRequest)WebRequest.Create(requestedURL);
authRequest.ContentType = "application/x-www-form-urlencoded";
authRequest.Method = "POST";
//Set content length to 0
authRequest.ContentLength = 0;
WebResponse authResponseTwitter = authRequest.GetResponse();
The ContentLength
property contains the value to send as the Content-length
HTTP header with the request.
Any value other than -1 in the ContentLength
property indicates that the request uploads data and that only methods that upload data are allowed to be set in the Method property.
After the ContentLength
property is set to a value, that number of bytes must be written to the request stream that is returned by calling the GetRequestStream
method or both the BeginGetRequestStream
and the EndGetRequestStream
methods.
for more details click here
add a comment |
Hey i'm using Volley and was getting Server error 411, I added to the getHeaders method the following line :
params.put("Content-Length","0");
And it solved my issue
add a comment |
Google search
2nd result
System.Net.WebException: The remote server returned an error: (411) Length Required.
This is a pretty common issue that comes up when trying to make call a
REST based API method through POST. Luckily, there is a simple fix for
this one.
This is the code I was using to call the Windows Azure Management API.
This particular API call requires the request method to be set as
POST, however there is no information that needs to be sent to the
server.
var request = (HttpWebRequest) HttpWebRequest.Create(requestUri);
request.Headers.Add("x-ms-version", "2012-08-01"); request.Method =
"POST"; request.ContentType = "application/xml";
To fix this error, add an explicit content length to your request
before making the API call.
request.ContentLength = 0;
add a comment |
I had the same error when I imported web requests from fiddler captured sessions to Visual Studio webtests. Some POST requests did not have a StringHttpBody tag. I added an empty one to them and the error was gone. Add this after the Headers tag:
<StringHttpBody ContentType="" InsertByteOrderMark="False">
</StringHttpBody>
add a comment |
Change the way you requested the method from POST to GET ..
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f18352190%2fwhy-i-get-411-length-required-error%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
8 Answers
8
active
oldest
votes
8 Answers
8
active
oldest
votes
active
oldest
votes
active
oldest
votes
When you're using HttpWebRequest and POST method, you have to set a content (or a body if you prefer) via the RequestStream. But, according to your code, using authRequest.Method = "GET" should be enough.
In case you're wondering about POST format, here's what you have to do :
ASCIIEncoding encoder = new ASCIIEncoding();
byte data = encoder.GetBytes(serializedObject); // a json object, or xml, whatever...
HttpWebRequest request = WebRequest.Create(url) as HttpWebRequest;
request.Method = "POST";
request.ContentType = "application/json";
request.ContentLength = data.Length;
request.Expect = "application/json";
request.GetRequestStream().Write(data, 0, data.Length);
HttpWebResponse response = request.GetResponse() as HttpWebResponse;
Can you please refer to my question "stackoverflow.com/questions/35308945/…" ? I am having similar issue. I am trying to hit the SFTP URL
– Yash Saraiya
Feb 10 '16 at 9:51
add a comment |
When you're using HttpWebRequest and POST method, you have to set a content (or a body if you prefer) via the RequestStream. But, according to your code, using authRequest.Method = "GET" should be enough.
In case you're wondering about POST format, here's what you have to do :
ASCIIEncoding encoder = new ASCIIEncoding();
byte data = encoder.GetBytes(serializedObject); // a json object, or xml, whatever...
HttpWebRequest request = WebRequest.Create(url) as HttpWebRequest;
request.Method = "POST";
request.ContentType = "application/json";
request.ContentLength = data.Length;
request.Expect = "application/json";
request.GetRequestStream().Write(data, 0, data.Length);
HttpWebResponse response = request.GetResponse() as HttpWebResponse;
Can you please refer to my question "stackoverflow.com/questions/35308945/…" ? I am having similar issue. I am trying to hit the SFTP URL
– Yash Saraiya
Feb 10 '16 at 9:51
add a comment |
When you're using HttpWebRequest and POST method, you have to set a content (or a body if you prefer) via the RequestStream. But, according to your code, using authRequest.Method = "GET" should be enough.
In case you're wondering about POST format, here's what you have to do :
ASCIIEncoding encoder = new ASCIIEncoding();
byte data = encoder.GetBytes(serializedObject); // a json object, or xml, whatever...
HttpWebRequest request = WebRequest.Create(url) as HttpWebRequest;
request.Method = "POST";
request.ContentType = "application/json";
request.ContentLength = data.Length;
request.Expect = "application/json";
request.GetRequestStream().Write(data, 0, data.Length);
HttpWebResponse response = request.GetResponse() as HttpWebResponse;
When you're using HttpWebRequest and POST method, you have to set a content (or a body if you prefer) via the RequestStream. But, according to your code, using authRequest.Method = "GET" should be enough.
In case you're wondering about POST format, here's what you have to do :
ASCIIEncoding encoder = new ASCIIEncoding();
byte data = encoder.GetBytes(serializedObject); // a json object, or xml, whatever...
HttpWebRequest request = WebRequest.Create(url) as HttpWebRequest;
request.Method = "POST";
request.ContentType = "application/json";
request.ContentLength = data.Length;
request.Expect = "application/json";
request.GetRequestStream().Write(data, 0, data.Length);
HttpWebResponse response = request.GetResponse() as HttpWebResponse;
answered Aug 21 '13 at 8:25
AtlasmaybeAtlasmaybe
1,094819
1,094819
Can you please refer to my question "stackoverflow.com/questions/35308945/…" ? I am having similar issue. I am trying to hit the SFTP URL
– Yash Saraiya
Feb 10 '16 at 9:51
add a comment |
Can you please refer to my question "stackoverflow.com/questions/35308945/…" ? I am having similar issue. I am trying to hit the SFTP URL
– Yash Saraiya
Feb 10 '16 at 9:51
Can you please refer to my question "stackoverflow.com/questions/35308945/…" ? I am having similar issue. I am trying to hit the SFTP URL
– Yash Saraiya
Feb 10 '16 at 9:51
Can you please refer to my question "stackoverflow.com/questions/35308945/…" ? I am having similar issue. I am trying to hit the SFTP URL
– Yash Saraiya
Feb 10 '16 at 9:51
add a comment |
you need to add Content-Length: 0
in your request header.
a very descriptive example of how to test is given here
Yes, adding Content-Length: 0 helped. Thanks!
– Roboblob
Apr 22 '14 at 15:29
simple and lifesaving. Thank you!
– Abraham Putra Prakasa
Jul 19 '18 at 16:28
add a comment |
you need to add Content-Length: 0
in your request header.
a very descriptive example of how to test is given here
Yes, adding Content-Length: 0 helped. Thanks!
– Roboblob
Apr 22 '14 at 15:29
simple and lifesaving. Thank you!
– Abraham Putra Prakasa
Jul 19 '18 at 16:28
add a comment |
you need to add Content-Length: 0
in your request header.
a very descriptive example of how to test is given here
you need to add Content-Length: 0
in your request header.
a very descriptive example of how to test is given here
answered Aug 21 '13 at 8:26


EhsanEhsan
24.6k54157
24.6k54157
Yes, adding Content-Length: 0 helped. Thanks!
– Roboblob
Apr 22 '14 at 15:29
simple and lifesaving. Thank you!
– Abraham Putra Prakasa
Jul 19 '18 at 16:28
add a comment |
Yes, adding Content-Length: 0 helped. Thanks!
– Roboblob
Apr 22 '14 at 15:29
simple and lifesaving. Thank you!
– Abraham Putra Prakasa
Jul 19 '18 at 16:28
Yes, adding Content-Length: 0 helped. Thanks!
– Roboblob
Apr 22 '14 at 15:29
Yes, adding Content-Length: 0 helped. Thanks!
– Roboblob
Apr 22 '14 at 15:29
simple and lifesaving. Thank you!
– Abraham Putra Prakasa
Jul 19 '18 at 16:28
simple and lifesaving. Thank you!
– Abraham Putra Prakasa
Jul 19 '18 at 16:28
add a comment |
When you make a POST
HttpWebRequest, you must specify the length of the data you are sending, something like:
string data = "something you need to send"
byte postBytes = Encoding.ASCII.GetBytes(data);
request.ContentLength = postBytes.Length;
if you are not sending any data, just set it to 0, that means you just have to add to your code this line:
request.ContentLength = 0;
Usually, if you are not sending any data, chosing the GET
method instead is wiser, as you can see in the HTTP RFC
thanks a lott ... it will useful for me
– Swapnil Tandel
Jun 24 '15 at 12:32
1
While customarily one would expect data to be sent with a POST, it would be incorrect and bad practice to use GET when you are modifying data on the server. A POST request should avoid any proxy caches between the client and server. GET is okay if data is being fetched from the server without the intention of anything changing on the server as a result of the request.
– Michael
Apr 4 '16 at 4:57
add a comment |
When you make a POST
HttpWebRequest, you must specify the length of the data you are sending, something like:
string data = "something you need to send"
byte postBytes = Encoding.ASCII.GetBytes(data);
request.ContentLength = postBytes.Length;
if you are not sending any data, just set it to 0, that means you just have to add to your code this line:
request.ContentLength = 0;
Usually, if you are not sending any data, chosing the GET
method instead is wiser, as you can see in the HTTP RFC
thanks a lott ... it will useful for me
– Swapnil Tandel
Jun 24 '15 at 12:32
1
While customarily one would expect data to be sent with a POST, it would be incorrect and bad practice to use GET when you are modifying data on the server. A POST request should avoid any proxy caches between the client and server. GET is okay if data is being fetched from the server without the intention of anything changing on the server as a result of the request.
– Michael
Apr 4 '16 at 4:57
add a comment |
When you make a POST
HttpWebRequest, you must specify the length of the data you are sending, something like:
string data = "something you need to send"
byte postBytes = Encoding.ASCII.GetBytes(data);
request.ContentLength = postBytes.Length;
if you are not sending any data, just set it to 0, that means you just have to add to your code this line:
request.ContentLength = 0;
Usually, if you are not sending any data, chosing the GET
method instead is wiser, as you can see in the HTTP RFC
When you make a POST
HttpWebRequest, you must specify the length of the data you are sending, something like:
string data = "something you need to send"
byte postBytes = Encoding.ASCII.GetBytes(data);
request.ContentLength = postBytes.Length;
if you are not sending any data, just set it to 0, that means you just have to add to your code this line:
request.ContentLength = 0;
Usually, if you are not sending any data, chosing the GET
method instead is wiser, as you can see in the HTTP RFC
edited Aug 21 '13 at 8:30
answered Aug 21 '13 at 8:25


SaveSave
6,74611322
6,74611322
thanks a lott ... it will useful for me
– Swapnil Tandel
Jun 24 '15 at 12:32
1
While customarily one would expect data to be sent with a POST, it would be incorrect and bad practice to use GET when you are modifying data on the server. A POST request should avoid any proxy caches between the client and server. GET is okay if data is being fetched from the server without the intention of anything changing on the server as a result of the request.
– Michael
Apr 4 '16 at 4:57
add a comment |
thanks a lott ... it will useful for me
– Swapnil Tandel
Jun 24 '15 at 12:32
1
While customarily one would expect data to be sent with a POST, it would be incorrect and bad practice to use GET when you are modifying data on the server. A POST request should avoid any proxy caches between the client and server. GET is okay if data is being fetched from the server without the intention of anything changing on the server as a result of the request.
– Michael
Apr 4 '16 at 4:57
thanks a lott ... it will useful for me
– Swapnil Tandel
Jun 24 '15 at 12:32
thanks a lott ... it will useful for me
– Swapnil Tandel
Jun 24 '15 at 12:32
1
1
While customarily one would expect data to be sent with a POST, it would be incorrect and bad practice to use GET when you are modifying data on the server. A POST request should avoid any proxy caches between the client and server. GET is okay if data is being fetched from the server without the intention of anything changing on the server as a result of the request.
– Michael
Apr 4 '16 at 4:57
While customarily one would expect data to be sent with a POST, it would be incorrect and bad practice to use GET when you are modifying data on the server. A POST request should avoid any proxy caches between the client and server. GET is okay if data is being fetched from the server without the intention of anything changing on the server as a result of the request.
– Michael
Apr 4 '16 at 4:57
add a comment |
var requestedURL = "https://accounts.google.com/o/oauth2/token?code=" + code + "&client_id=" + client_id + "&client_secret=" + client_secret + "&redirect_uri=" + redirect_uri + "&grant_type=authorization_code";
HttpWebRequest authRequest = (HttpWebRequest)WebRequest.Create(requestedURL);
authRequest.ContentType = "application/x-www-form-urlencoded";
authRequest.Method = "POST";
//Set content length to 0
authRequest.ContentLength = 0;
WebResponse authResponseTwitter = authRequest.GetResponse();
The ContentLength
property contains the value to send as the Content-length
HTTP header with the request.
Any value other than -1 in the ContentLength
property indicates that the request uploads data and that only methods that upload data are allowed to be set in the Method property.
After the ContentLength
property is set to a value, that number of bytes must be written to the request stream that is returned by calling the GetRequestStream
method or both the BeginGetRequestStream
and the EndGetRequestStream
methods.
for more details click here
add a comment |
var requestedURL = "https://accounts.google.com/o/oauth2/token?code=" + code + "&client_id=" + client_id + "&client_secret=" + client_secret + "&redirect_uri=" + redirect_uri + "&grant_type=authorization_code";
HttpWebRequest authRequest = (HttpWebRequest)WebRequest.Create(requestedURL);
authRequest.ContentType = "application/x-www-form-urlencoded";
authRequest.Method = "POST";
//Set content length to 0
authRequest.ContentLength = 0;
WebResponse authResponseTwitter = authRequest.GetResponse();
The ContentLength
property contains the value to send as the Content-length
HTTP header with the request.
Any value other than -1 in the ContentLength
property indicates that the request uploads data and that only methods that upload data are allowed to be set in the Method property.
After the ContentLength
property is set to a value, that number of bytes must be written to the request stream that is returned by calling the GetRequestStream
method or both the BeginGetRequestStream
and the EndGetRequestStream
methods.
for more details click here
add a comment |
var requestedURL = "https://accounts.google.com/o/oauth2/token?code=" + code + "&client_id=" + client_id + "&client_secret=" + client_secret + "&redirect_uri=" + redirect_uri + "&grant_type=authorization_code";
HttpWebRequest authRequest = (HttpWebRequest)WebRequest.Create(requestedURL);
authRequest.ContentType = "application/x-www-form-urlencoded";
authRequest.Method = "POST";
//Set content length to 0
authRequest.ContentLength = 0;
WebResponse authResponseTwitter = authRequest.GetResponse();
The ContentLength
property contains the value to send as the Content-length
HTTP header with the request.
Any value other than -1 in the ContentLength
property indicates that the request uploads data and that only methods that upload data are allowed to be set in the Method property.
After the ContentLength
property is set to a value, that number of bytes must be written to the request stream that is returned by calling the GetRequestStream
method or both the BeginGetRequestStream
and the EndGetRequestStream
methods.
for more details click here
var requestedURL = "https://accounts.google.com/o/oauth2/token?code=" + code + "&client_id=" + client_id + "&client_secret=" + client_secret + "&redirect_uri=" + redirect_uri + "&grant_type=authorization_code";
HttpWebRequest authRequest = (HttpWebRequest)WebRequest.Create(requestedURL);
authRequest.ContentType = "application/x-www-form-urlencoded";
authRequest.Method = "POST";
//Set content length to 0
authRequest.ContentLength = 0;
WebResponse authResponseTwitter = authRequest.GetResponse();
The ContentLength
property contains the value to send as the Content-length
HTTP header with the request.
Any value other than -1 in the ContentLength
property indicates that the request uploads data and that only methods that upload data are allowed to be set in the Method property.
After the ContentLength
property is set to a value, that number of bytes must be written to the request stream that is returned by calling the GetRequestStream
method or both the BeginGetRequestStream
and the EndGetRequestStream
methods.
for more details click here
answered Aug 21 '15 at 9:49


Musakkhir SayyedMusakkhir Sayyed
4,08572956
4,08572956
add a comment |
add a comment |
Hey i'm using Volley and was getting Server error 411, I added to the getHeaders method the following line :
params.put("Content-Length","0");
And it solved my issue
add a comment |
Hey i'm using Volley and was getting Server error 411, I added to the getHeaders method the following line :
params.put("Content-Length","0");
And it solved my issue
add a comment |
Hey i'm using Volley and was getting Server error 411, I added to the getHeaders method the following line :
params.put("Content-Length","0");
And it solved my issue
Hey i'm using Volley and was getting Server error 411, I added to the getHeaders method the following line :
params.put("Content-Length","0");
And it solved my issue
answered Mar 4 '15 at 8:05
shimi_tapshimi_tap
4,93941621
4,93941621
add a comment |
add a comment |
Google search
2nd result
System.Net.WebException: The remote server returned an error: (411) Length Required.
This is a pretty common issue that comes up when trying to make call a
REST based API method through POST. Luckily, there is a simple fix for
this one.
This is the code I was using to call the Windows Azure Management API.
This particular API call requires the request method to be set as
POST, however there is no information that needs to be sent to the
server.
var request = (HttpWebRequest) HttpWebRequest.Create(requestUri);
request.Headers.Add("x-ms-version", "2012-08-01"); request.Method =
"POST"; request.ContentType = "application/xml";
To fix this error, add an explicit content length to your request
before making the API call.
request.ContentLength = 0;
add a comment |
Google search
2nd result
System.Net.WebException: The remote server returned an error: (411) Length Required.
This is a pretty common issue that comes up when trying to make call a
REST based API method through POST. Luckily, there is a simple fix for
this one.
This is the code I was using to call the Windows Azure Management API.
This particular API call requires the request method to be set as
POST, however there is no information that needs to be sent to the
server.
var request = (HttpWebRequest) HttpWebRequest.Create(requestUri);
request.Headers.Add("x-ms-version", "2012-08-01"); request.Method =
"POST"; request.ContentType = "application/xml";
To fix this error, add an explicit content length to your request
before making the API call.
request.ContentLength = 0;
add a comment |
Google search
2nd result
System.Net.WebException: The remote server returned an error: (411) Length Required.
This is a pretty common issue that comes up when trying to make call a
REST based API method through POST. Luckily, there is a simple fix for
this one.
This is the code I was using to call the Windows Azure Management API.
This particular API call requires the request method to be set as
POST, however there is no information that needs to be sent to the
server.
var request = (HttpWebRequest) HttpWebRequest.Create(requestUri);
request.Headers.Add("x-ms-version", "2012-08-01"); request.Method =
"POST"; request.ContentType = "application/xml";
To fix this error, add an explicit content length to your request
before making the API call.
request.ContentLength = 0;
Google search
2nd result
System.Net.WebException: The remote server returned an error: (411) Length Required.
This is a pretty common issue that comes up when trying to make call a
REST based API method through POST. Luckily, there is a simple fix for
this one.
This is the code I was using to call the Windows Azure Management API.
This particular API call requires the request method to be set as
POST, however there is no information that needs to be sent to the
server.
var request = (HttpWebRequest) HttpWebRequest.Create(requestUri);
request.Headers.Add("x-ms-version", "2012-08-01"); request.Method =
"POST"; request.ContentType = "application/xml";
To fix this error, add an explicit content length to your request
before making the API call.
request.ContentLength = 0;
answered Aug 21 '13 at 8:28
iabbottiabbott
7651720
7651720
add a comment |
add a comment |
I had the same error when I imported web requests from fiddler captured sessions to Visual Studio webtests. Some POST requests did not have a StringHttpBody tag. I added an empty one to them and the error was gone. Add this after the Headers tag:
<StringHttpBody ContentType="" InsertByteOrderMark="False">
</StringHttpBody>
add a comment |
I had the same error when I imported web requests from fiddler captured sessions to Visual Studio webtests. Some POST requests did not have a StringHttpBody tag. I added an empty one to them and the error was gone. Add this after the Headers tag:
<StringHttpBody ContentType="" InsertByteOrderMark="False">
</StringHttpBody>
add a comment |
I had the same error when I imported web requests from fiddler captured sessions to Visual Studio webtests. Some POST requests did not have a StringHttpBody tag. I added an empty one to them and the error was gone. Add this after the Headers tag:
<StringHttpBody ContentType="" InsertByteOrderMark="False">
</StringHttpBody>
I had the same error when I imported web requests from fiddler captured sessions to Visual Studio webtests. Some POST requests did not have a StringHttpBody tag. I added an empty one to them and the error was gone. Add this after the Headers tag:
<StringHttpBody ContentType="" InsertByteOrderMark="False">
</StringHttpBody>
answered Apr 17 '18 at 12:48


Youcef KelanemerYoucef Kelanemer
479
479
add a comment |
add a comment |
Change the way you requested the method from POST to GET ..
add a comment |
Change the way you requested the method from POST to GET ..
add a comment |
Change the way you requested the method from POST to GET ..
Change the way you requested the method from POST to GET ..
edited Nov 24 '18 at 16:11
Dipak Rathod
10712
10712
answered Nov 24 '18 at 10:30


Solomon ThomasSolomon Thomas
11
11
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f18352190%2fwhy-i-get-411-length-required-error%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
wM1cdICsZwYl XRB