Deserialize returns null key and null value (count is correct)
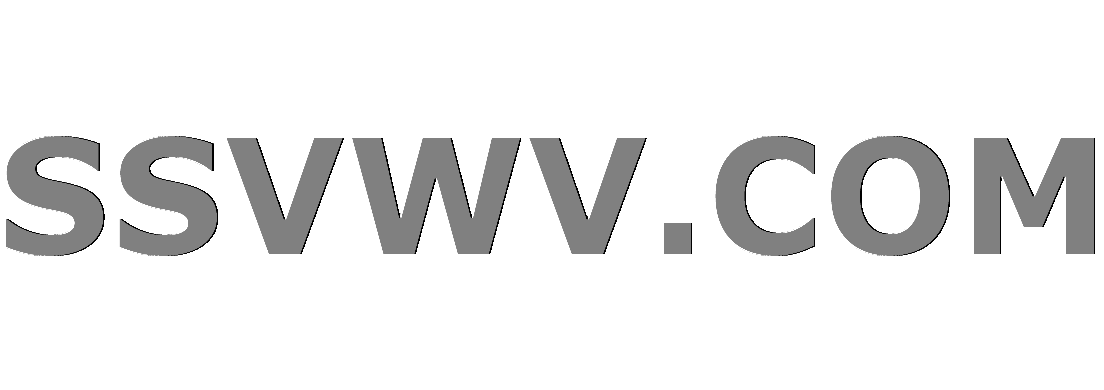
Multi tool use
I am experimenting with C# serialization, and had to serialize a Dictionary, as it isn't serializable I used an XML string, which then gets deserialized (example taken from https://blogs.msdn.microsoft.com/adam/2010/09/10/how-to-serialize-a-dictionary-or-hashtable-in-c/)
DictionaryDataItem class:
[Serializable]
public class DictionaryDataItem
{
public string Key;
public string Value;
public DictionaryDataItem(string key, string value)
{
Key = key;
Value = value;
}
public DictionaryDataItem()
{
Key = "";
Value = "";
}
}
Serialization code:
[WebMethod]
public string ListarPaises()
{
List<DictionaryDataItem> Paises = new List<DictionaryDataItem>();
XmlSerializer serializer = new XmlSerializer(typeof(List<DictionaryDataItem>));
StringWriter sw = new StringWriter();
XmlSerializerNamespaces ns = new XmlSerializerNamespaces();
ns.Add("", "");
try
{
Dictionary<string, string> diccionario = FabricaLogica.getLogicaGeneral().ListarPaises();
foreach (string key in diccionario.Keys)
{
Paises.Add(new DictionaryDataItem(key, diccionario[key]));
}
serializer.Serialize(sw, Paises, ns);
} catch (Exception ex)
{
ThrowSoapException(ex);
}
return sw.ToString();
}
The serialization works and it returns the following string as xml:
<string xmlns="http://tempuri.org/">
<?xml version="1.0" encoding="utf-16"?>
<ArrayOfDictionaryDataItem>
<DictionaryDataItem>
<Key>ARG</Key>
<Value>ARGENTINA</Value>
</DictionaryDataItem>
<DictionaryDataItem>
<Key>BRA</Key>
<Value>BRASIL</Value>
</DictionaryDataItem>
<DictionaryDataItem>
<Key>URU</Key>
<Value>URUGUAY</Value>
</DictionaryDataItem>
</ArrayOfDictionaryDataItem>
</string>
And then, my deserialization code:
Dictionary<string, string> Paises = new Dictionary<string, string>();
string RawData = new WebService.WebService().ListarPaises();
XmlSerializer xs = new XmlSerializer(typeof(List<DictionaryDataItem>));
StringReader sr = new StringReader(RawData);
List<DictionaryDataItem> templist = (List<DictionaryDataItem>)xs.Deserialize(sr);
foreach (DictionaryDataItem di in templist)
{
Paises.Add(di.Key, di.Value);
}
return Paises;
I can see that templist
has 3 items, but both key and value for all of the items is null.
Am I missing something?
c#
|
show 5 more comments
I am experimenting with C# serialization, and had to serialize a Dictionary, as it isn't serializable I used an XML string, which then gets deserialized (example taken from https://blogs.msdn.microsoft.com/adam/2010/09/10/how-to-serialize-a-dictionary-or-hashtable-in-c/)
DictionaryDataItem class:
[Serializable]
public class DictionaryDataItem
{
public string Key;
public string Value;
public DictionaryDataItem(string key, string value)
{
Key = key;
Value = value;
}
public DictionaryDataItem()
{
Key = "";
Value = "";
}
}
Serialization code:
[WebMethod]
public string ListarPaises()
{
List<DictionaryDataItem> Paises = new List<DictionaryDataItem>();
XmlSerializer serializer = new XmlSerializer(typeof(List<DictionaryDataItem>));
StringWriter sw = new StringWriter();
XmlSerializerNamespaces ns = new XmlSerializerNamespaces();
ns.Add("", "");
try
{
Dictionary<string, string> diccionario = FabricaLogica.getLogicaGeneral().ListarPaises();
foreach (string key in diccionario.Keys)
{
Paises.Add(new DictionaryDataItem(key, diccionario[key]));
}
serializer.Serialize(sw, Paises, ns);
} catch (Exception ex)
{
ThrowSoapException(ex);
}
return sw.ToString();
}
The serialization works and it returns the following string as xml:
<string xmlns="http://tempuri.org/">
<?xml version="1.0" encoding="utf-16"?>
<ArrayOfDictionaryDataItem>
<DictionaryDataItem>
<Key>ARG</Key>
<Value>ARGENTINA</Value>
</DictionaryDataItem>
<DictionaryDataItem>
<Key>BRA</Key>
<Value>BRASIL</Value>
</DictionaryDataItem>
<DictionaryDataItem>
<Key>URU</Key>
<Value>URUGUAY</Value>
</DictionaryDataItem>
</ArrayOfDictionaryDataItem>
</string>
And then, my deserialization code:
Dictionary<string, string> Paises = new Dictionary<string, string>();
string RawData = new WebService.WebService().ListarPaises();
XmlSerializer xs = new XmlSerializer(typeof(List<DictionaryDataItem>));
StringReader sr = new StringReader(RawData);
List<DictionaryDataItem> templist = (List<DictionaryDataItem>)xs.Deserialize(sr);
foreach (DictionaryDataItem di in templist)
{
Paises.Add(di.Key, di.Value);
}
return Paises;
I can see that templist
has 3 items, but both key and value for all of the items is null.
Am I missing something?
c#
Where do you setxmlelement
andxmlarray
?
– T.S.
Nov 26 '18 at 1:54
@T.S. hmm I have no idea... let me add the DictionaryDataItem class and the serialization code.
– Fede E.
Nov 26 '18 at 1:57
Out of curiosity, why are you learning about XML serialization? The article you're using as a guide is from 2010 and the industry has changed a lot since then: thesedays most serialization is done to JSON (which is much more space-efficient than XML) or to a binary format like protobufs.
– Dai
Nov 26 '18 at 1:57
You can serializeDictionary<TKey,TValue>
to XML usingDataContractSerializer
. The stockXmlSerializer
does not supportIDictionary
because the dev team ran out of time back in 2001 for .NET Framework 1.1. See here: theburningmonk.com/2010/05/…
– Dai
Nov 26 '18 at 1:59
@Dai it's a requirement for this a project in a class I'm taking... I saw lots of ways using json, I'm actually not a fan of xml, but had to do it with XML.
– Fede E.
Nov 26 '18 at 2:01
|
show 5 more comments
I am experimenting with C# serialization, and had to serialize a Dictionary, as it isn't serializable I used an XML string, which then gets deserialized (example taken from https://blogs.msdn.microsoft.com/adam/2010/09/10/how-to-serialize-a-dictionary-or-hashtable-in-c/)
DictionaryDataItem class:
[Serializable]
public class DictionaryDataItem
{
public string Key;
public string Value;
public DictionaryDataItem(string key, string value)
{
Key = key;
Value = value;
}
public DictionaryDataItem()
{
Key = "";
Value = "";
}
}
Serialization code:
[WebMethod]
public string ListarPaises()
{
List<DictionaryDataItem> Paises = new List<DictionaryDataItem>();
XmlSerializer serializer = new XmlSerializer(typeof(List<DictionaryDataItem>));
StringWriter sw = new StringWriter();
XmlSerializerNamespaces ns = new XmlSerializerNamespaces();
ns.Add("", "");
try
{
Dictionary<string, string> diccionario = FabricaLogica.getLogicaGeneral().ListarPaises();
foreach (string key in diccionario.Keys)
{
Paises.Add(new DictionaryDataItem(key, diccionario[key]));
}
serializer.Serialize(sw, Paises, ns);
} catch (Exception ex)
{
ThrowSoapException(ex);
}
return sw.ToString();
}
The serialization works and it returns the following string as xml:
<string xmlns="http://tempuri.org/">
<?xml version="1.0" encoding="utf-16"?>
<ArrayOfDictionaryDataItem>
<DictionaryDataItem>
<Key>ARG</Key>
<Value>ARGENTINA</Value>
</DictionaryDataItem>
<DictionaryDataItem>
<Key>BRA</Key>
<Value>BRASIL</Value>
</DictionaryDataItem>
<DictionaryDataItem>
<Key>URU</Key>
<Value>URUGUAY</Value>
</DictionaryDataItem>
</ArrayOfDictionaryDataItem>
</string>
And then, my deserialization code:
Dictionary<string, string> Paises = new Dictionary<string, string>();
string RawData = new WebService.WebService().ListarPaises();
XmlSerializer xs = new XmlSerializer(typeof(List<DictionaryDataItem>));
StringReader sr = new StringReader(RawData);
List<DictionaryDataItem> templist = (List<DictionaryDataItem>)xs.Deserialize(sr);
foreach (DictionaryDataItem di in templist)
{
Paises.Add(di.Key, di.Value);
}
return Paises;
I can see that templist
has 3 items, but both key and value for all of the items is null.
Am I missing something?
c#
I am experimenting with C# serialization, and had to serialize a Dictionary, as it isn't serializable I used an XML string, which then gets deserialized (example taken from https://blogs.msdn.microsoft.com/adam/2010/09/10/how-to-serialize-a-dictionary-or-hashtable-in-c/)
DictionaryDataItem class:
[Serializable]
public class DictionaryDataItem
{
public string Key;
public string Value;
public DictionaryDataItem(string key, string value)
{
Key = key;
Value = value;
}
public DictionaryDataItem()
{
Key = "";
Value = "";
}
}
Serialization code:
[WebMethod]
public string ListarPaises()
{
List<DictionaryDataItem> Paises = new List<DictionaryDataItem>();
XmlSerializer serializer = new XmlSerializer(typeof(List<DictionaryDataItem>));
StringWriter sw = new StringWriter();
XmlSerializerNamespaces ns = new XmlSerializerNamespaces();
ns.Add("", "");
try
{
Dictionary<string, string> diccionario = FabricaLogica.getLogicaGeneral().ListarPaises();
foreach (string key in diccionario.Keys)
{
Paises.Add(new DictionaryDataItem(key, diccionario[key]));
}
serializer.Serialize(sw, Paises, ns);
} catch (Exception ex)
{
ThrowSoapException(ex);
}
return sw.ToString();
}
The serialization works and it returns the following string as xml:
<string xmlns="http://tempuri.org/">
<?xml version="1.0" encoding="utf-16"?>
<ArrayOfDictionaryDataItem>
<DictionaryDataItem>
<Key>ARG</Key>
<Value>ARGENTINA</Value>
</DictionaryDataItem>
<DictionaryDataItem>
<Key>BRA</Key>
<Value>BRASIL</Value>
</DictionaryDataItem>
<DictionaryDataItem>
<Key>URU</Key>
<Value>URUGUAY</Value>
</DictionaryDataItem>
</ArrayOfDictionaryDataItem>
</string>
And then, my deserialization code:
Dictionary<string, string> Paises = new Dictionary<string, string>();
string RawData = new WebService.WebService().ListarPaises();
XmlSerializer xs = new XmlSerializer(typeof(List<DictionaryDataItem>));
StringReader sr = new StringReader(RawData);
List<DictionaryDataItem> templist = (List<DictionaryDataItem>)xs.Deserialize(sr);
foreach (DictionaryDataItem di in templist)
{
Paises.Add(di.Key, di.Value);
}
return Paises;
I can see that templist
has 3 items, but both key and value for all of the items is null.
Am I missing something?
c#
c#
edited Nov 26 '18 at 1:59
Fede E.
asked Nov 26 '18 at 1:47
Fede E.Fede E.
4721615
4721615
Where do you setxmlelement
andxmlarray
?
– T.S.
Nov 26 '18 at 1:54
@T.S. hmm I have no idea... let me add the DictionaryDataItem class and the serialization code.
– Fede E.
Nov 26 '18 at 1:57
Out of curiosity, why are you learning about XML serialization? The article you're using as a guide is from 2010 and the industry has changed a lot since then: thesedays most serialization is done to JSON (which is much more space-efficient than XML) or to a binary format like protobufs.
– Dai
Nov 26 '18 at 1:57
You can serializeDictionary<TKey,TValue>
to XML usingDataContractSerializer
. The stockXmlSerializer
does not supportIDictionary
because the dev team ran out of time back in 2001 for .NET Framework 1.1. See here: theburningmonk.com/2010/05/…
– Dai
Nov 26 '18 at 1:59
@Dai it's a requirement for this a project in a class I'm taking... I saw lots of ways using json, I'm actually not a fan of xml, but had to do it with XML.
– Fede E.
Nov 26 '18 at 2:01
|
show 5 more comments
Where do you setxmlelement
andxmlarray
?
– T.S.
Nov 26 '18 at 1:54
@T.S. hmm I have no idea... let me add the DictionaryDataItem class and the serialization code.
– Fede E.
Nov 26 '18 at 1:57
Out of curiosity, why are you learning about XML serialization? The article you're using as a guide is from 2010 and the industry has changed a lot since then: thesedays most serialization is done to JSON (which is much more space-efficient than XML) or to a binary format like protobufs.
– Dai
Nov 26 '18 at 1:57
You can serializeDictionary<TKey,TValue>
to XML usingDataContractSerializer
. The stockXmlSerializer
does not supportIDictionary
because the dev team ran out of time back in 2001 for .NET Framework 1.1. See here: theburningmonk.com/2010/05/…
– Dai
Nov 26 '18 at 1:59
@Dai it's a requirement for this a project in a class I'm taking... I saw lots of ways using json, I'm actually not a fan of xml, but had to do it with XML.
– Fede E.
Nov 26 '18 at 2:01
Where do you set
xmlelement
and xmlarray
?– T.S.
Nov 26 '18 at 1:54
Where do you set
xmlelement
and xmlarray
?– T.S.
Nov 26 '18 at 1:54
@T.S. hmm I have no idea... let me add the DictionaryDataItem class and the serialization code.
– Fede E.
Nov 26 '18 at 1:57
@T.S. hmm I have no idea... let me add the DictionaryDataItem class and the serialization code.
– Fede E.
Nov 26 '18 at 1:57
Out of curiosity, why are you learning about XML serialization? The article you're using as a guide is from 2010 and the industry has changed a lot since then: thesedays most serialization is done to JSON (which is much more space-efficient than XML) or to a binary format like protobufs.
– Dai
Nov 26 '18 at 1:57
Out of curiosity, why are you learning about XML serialization? The article you're using as a guide is from 2010 and the industry has changed a lot since then: thesedays most serialization is done to JSON (which is much more space-efficient than XML) or to a binary format like protobufs.
– Dai
Nov 26 '18 at 1:57
You can serialize
Dictionary<TKey,TValue>
to XML using DataContractSerializer
. The stock XmlSerializer
does not support IDictionary
because the dev team ran out of time back in 2001 for .NET Framework 1.1. See here: theburningmonk.com/2010/05/…– Dai
Nov 26 '18 at 1:59
You can serialize
Dictionary<TKey,TValue>
to XML using DataContractSerializer
. The stock XmlSerializer
does not support IDictionary
because the dev team ran out of time back in 2001 for .NET Framework 1.1. See here: theburningmonk.com/2010/05/…– Dai
Nov 26 '18 at 1:59
@Dai it's a requirement for this a project in a class I'm taking... I saw lots of ways using json, I'm actually not a fan of xml, but had to do it with XML.
– Fede E.
Nov 26 '18 at 2:01
@Dai it's a requirement for this a project in a class I'm taking... I saw lots of ways using json, I'm actually not a fan of xml, but had to do it with XML.
– Fede E.
Nov 26 '18 at 2:01
|
show 5 more comments
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53473798%2fdeserialize-returns-null-key-and-null-value-count-is-correct%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53473798%2fdeserialize-returns-null-key-and-null-value-count-is-correct%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
9,0 oeXW
Where do you set
xmlelement
andxmlarray
?– T.S.
Nov 26 '18 at 1:54
@T.S. hmm I have no idea... let me add the DictionaryDataItem class and the serialization code.
– Fede E.
Nov 26 '18 at 1:57
Out of curiosity, why are you learning about XML serialization? The article you're using as a guide is from 2010 and the industry has changed a lot since then: thesedays most serialization is done to JSON (which is much more space-efficient than XML) or to a binary format like protobufs.
– Dai
Nov 26 '18 at 1:57
You can serialize
Dictionary<TKey,TValue>
to XML usingDataContractSerializer
. The stockXmlSerializer
does not supportIDictionary
because the dev team ran out of time back in 2001 for .NET Framework 1.1. See here: theburningmonk.com/2010/05/…– Dai
Nov 26 '18 at 1:59
@Dai it's a requirement for this a project in a class I'm taking... I saw lots of ways using json, I'm actually not a fan of xml, but had to do it with XML.
– Fede E.
Nov 26 '18 at 2:01