List of checkboxes are lost when the form is submitted
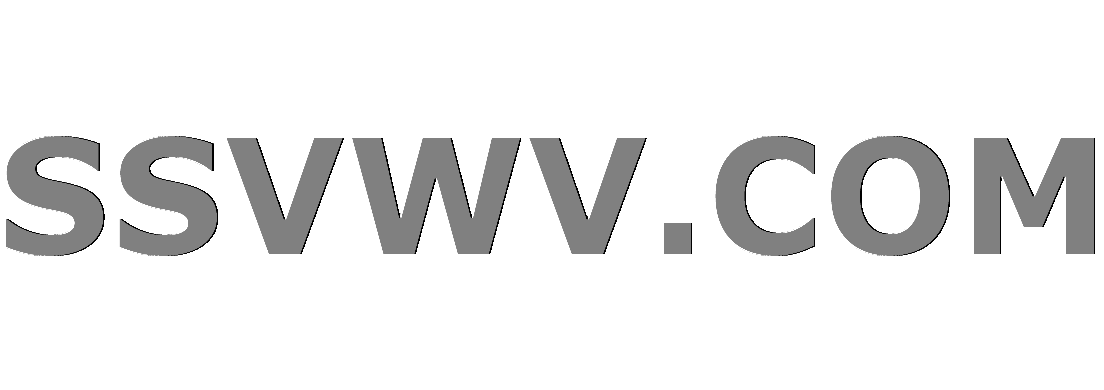
Multi tool use
I have these viewmodels for employees and their roles:
public class EmployeeViewModel
{
public int Id { get; set; }
// some more properties
public List<EmployeeRoleViewModel> EmployeeRoles { get; set; }
}
public class EmployeeRoleViewModel
{
public int Id { get; set; }
public int EmployeeId { get; set; }
public int RoleId { get; set; }
public string Title { get; set; }
public bool Selected { get; set; }
}
I am displaying the checkboxes like this in the Edit-view:
@for (int i = 0; i < Model.EmployeeRoles.Count(); i++)
{
<label>
<input type="checkbox" asp-for="@Model.EmployeeRoles[i].Selected" />
@Model.EmployeeRoles[i].Title
</label>
}
This generates this HTML for a checked checkbox:
<input type="checkbox" checked="checked"
id="EmployeeRoles_0__Selected"
name="EmployeeRoles[0].Selected" value="true" />
... and this for an unchecked one:
<input type="checkbox"
id="EmployeeRoles_2__Selected"
name="EmployeeRoles[2].Selected" value="true" />
The checkboxes are all being rendered correctly, with checkmarks on all the right boxes.
The form is posted to this controller-method (simplified for brevity):
[HttpPost]
public async Task<IActionResult> Edit(int id,[Bind("Id,EmployeeRoles")] Employee employee)
{
db.Update(employee);
await db.SaveChangesAsync();
return RedirectToAction("Details", "Branches");
}
The problem is that employee.EmployeeRoles
is a list of length 0, even though several checkboxes are checked. What am I doing wrong?
c# asp.net-core
add a comment |
I have these viewmodels for employees and their roles:
public class EmployeeViewModel
{
public int Id { get; set; }
// some more properties
public List<EmployeeRoleViewModel> EmployeeRoles { get; set; }
}
public class EmployeeRoleViewModel
{
public int Id { get; set; }
public int EmployeeId { get; set; }
public int RoleId { get; set; }
public string Title { get; set; }
public bool Selected { get; set; }
}
I am displaying the checkboxes like this in the Edit-view:
@for (int i = 0; i < Model.EmployeeRoles.Count(); i++)
{
<label>
<input type="checkbox" asp-for="@Model.EmployeeRoles[i].Selected" />
@Model.EmployeeRoles[i].Title
</label>
}
This generates this HTML for a checked checkbox:
<input type="checkbox" checked="checked"
id="EmployeeRoles_0__Selected"
name="EmployeeRoles[0].Selected" value="true" />
... and this for an unchecked one:
<input type="checkbox"
id="EmployeeRoles_2__Selected"
name="EmployeeRoles[2].Selected" value="true" />
The checkboxes are all being rendered correctly, with checkmarks on all the right boxes.
The form is posted to this controller-method (simplified for brevity):
[HttpPost]
public async Task<IActionResult> Edit(int id,[Bind("Id,EmployeeRoles")] Employee employee)
{
db.Update(employee);
await db.SaveChangesAsync();
return RedirectToAction("Details", "Branches");
}
The problem is that employee.EmployeeRoles
is a list of length 0, even though several checkboxes are checked. What am I doing wrong?
c# asp.net-core
add a comment |
I have these viewmodels for employees and their roles:
public class EmployeeViewModel
{
public int Id { get; set; }
// some more properties
public List<EmployeeRoleViewModel> EmployeeRoles { get; set; }
}
public class EmployeeRoleViewModel
{
public int Id { get; set; }
public int EmployeeId { get; set; }
public int RoleId { get; set; }
public string Title { get; set; }
public bool Selected { get; set; }
}
I am displaying the checkboxes like this in the Edit-view:
@for (int i = 0; i < Model.EmployeeRoles.Count(); i++)
{
<label>
<input type="checkbox" asp-for="@Model.EmployeeRoles[i].Selected" />
@Model.EmployeeRoles[i].Title
</label>
}
This generates this HTML for a checked checkbox:
<input type="checkbox" checked="checked"
id="EmployeeRoles_0__Selected"
name="EmployeeRoles[0].Selected" value="true" />
... and this for an unchecked one:
<input type="checkbox"
id="EmployeeRoles_2__Selected"
name="EmployeeRoles[2].Selected" value="true" />
The checkboxes are all being rendered correctly, with checkmarks on all the right boxes.
The form is posted to this controller-method (simplified for brevity):
[HttpPost]
public async Task<IActionResult> Edit(int id,[Bind("Id,EmployeeRoles")] Employee employee)
{
db.Update(employee);
await db.SaveChangesAsync();
return RedirectToAction("Details", "Branches");
}
The problem is that employee.EmployeeRoles
is a list of length 0, even though several checkboxes are checked. What am I doing wrong?
c# asp.net-core
I have these viewmodels for employees and their roles:
public class EmployeeViewModel
{
public int Id { get; set; }
// some more properties
public List<EmployeeRoleViewModel> EmployeeRoles { get; set; }
}
public class EmployeeRoleViewModel
{
public int Id { get; set; }
public int EmployeeId { get; set; }
public int RoleId { get; set; }
public string Title { get; set; }
public bool Selected { get; set; }
}
I am displaying the checkboxes like this in the Edit-view:
@for (int i = 0; i < Model.EmployeeRoles.Count(); i++)
{
<label>
<input type="checkbox" asp-for="@Model.EmployeeRoles[i].Selected" />
@Model.EmployeeRoles[i].Title
</label>
}
This generates this HTML for a checked checkbox:
<input type="checkbox" checked="checked"
id="EmployeeRoles_0__Selected"
name="EmployeeRoles[0].Selected" value="true" />
... and this for an unchecked one:
<input type="checkbox"
id="EmployeeRoles_2__Selected"
name="EmployeeRoles[2].Selected" value="true" />
The checkboxes are all being rendered correctly, with checkmarks on all the right boxes.
The form is posted to this controller-method (simplified for brevity):
[HttpPost]
public async Task<IActionResult> Edit(int id,[Bind("Id,EmployeeRoles")] Employee employee)
{
db.Update(employee);
await db.SaveChangesAsync();
return RedirectToAction("Details", "Branches");
}
The problem is that employee.EmployeeRoles
is a list of length 0, even though several checkboxes are checked. What am I doing wrong?
c# asp.net-core
c# asp.net-core
edited Nov 25 '18 at 20:19
Stian
asked Nov 25 '18 at 20:14


StianStian
364418
364418
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
The name
of the input
field will have to be
name="Employee.EmployeeRoles[index].Selected"
in order for EmployeeRoles[index]
to be bound to the EmployeeRoles
list inside the Employee
object.
add a comment |
Please firstly confirm that you are using same view model both in view and in action function . You can add hidden input field to help you bind the properties :
@for (int i = 0; i < Model.EmployeeRoles.Count(); i++)
{
<label>
<input type="checkbox" asp-for="@Model.EmployeeRoles[i].Selected" />
@Model.EmployeeRoles[i].Title
</label>
<input type="hidden" asp-for="@Model.EmployeeRoles[i].Id" />
<input type="hidden" asp-for="@Model.EmployeeRoles[i].Title" />
}
Then on controller , you can get the lists :
Then check the Selected
properties to confirm whether current role is selected .
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53471487%2flist-of-checkboxes-are-lost-when-the-form-is-submitted%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
The name
of the input
field will have to be
name="Employee.EmployeeRoles[index].Selected"
in order for EmployeeRoles[index]
to be bound to the EmployeeRoles
list inside the Employee
object.
add a comment |
The name
of the input
field will have to be
name="Employee.EmployeeRoles[index].Selected"
in order for EmployeeRoles[index]
to be bound to the EmployeeRoles
list inside the Employee
object.
add a comment |
The name
of the input
field will have to be
name="Employee.EmployeeRoles[index].Selected"
in order for EmployeeRoles[index]
to be bound to the EmployeeRoles
list inside the Employee
object.
The name
of the input
field will have to be
name="Employee.EmployeeRoles[index].Selected"
in order for EmployeeRoles[index]
to be bound to the EmployeeRoles
list inside the Employee
object.
answered Nov 25 '18 at 23:06
Anton ToshikAnton Toshik
949921
949921
add a comment |
add a comment |
Please firstly confirm that you are using same view model both in view and in action function . You can add hidden input field to help you bind the properties :
@for (int i = 0; i < Model.EmployeeRoles.Count(); i++)
{
<label>
<input type="checkbox" asp-for="@Model.EmployeeRoles[i].Selected" />
@Model.EmployeeRoles[i].Title
</label>
<input type="hidden" asp-for="@Model.EmployeeRoles[i].Id" />
<input type="hidden" asp-for="@Model.EmployeeRoles[i].Title" />
}
Then on controller , you can get the lists :
Then check the Selected
properties to confirm whether current role is selected .
add a comment |
Please firstly confirm that you are using same view model both in view and in action function . You can add hidden input field to help you bind the properties :
@for (int i = 0; i < Model.EmployeeRoles.Count(); i++)
{
<label>
<input type="checkbox" asp-for="@Model.EmployeeRoles[i].Selected" />
@Model.EmployeeRoles[i].Title
</label>
<input type="hidden" asp-for="@Model.EmployeeRoles[i].Id" />
<input type="hidden" asp-for="@Model.EmployeeRoles[i].Title" />
}
Then on controller , you can get the lists :
Then check the Selected
properties to confirm whether current role is selected .
add a comment |
Please firstly confirm that you are using same view model both in view and in action function . You can add hidden input field to help you bind the properties :
@for (int i = 0; i < Model.EmployeeRoles.Count(); i++)
{
<label>
<input type="checkbox" asp-for="@Model.EmployeeRoles[i].Selected" />
@Model.EmployeeRoles[i].Title
</label>
<input type="hidden" asp-for="@Model.EmployeeRoles[i].Id" />
<input type="hidden" asp-for="@Model.EmployeeRoles[i].Title" />
}
Then on controller , you can get the lists :
Then check the Selected
properties to confirm whether current role is selected .
Please firstly confirm that you are using same view model both in view and in action function . You can add hidden input field to help you bind the properties :
@for (int i = 0; i < Model.EmployeeRoles.Count(); i++)
{
<label>
<input type="checkbox" asp-for="@Model.EmployeeRoles[i].Selected" />
@Model.EmployeeRoles[i].Title
</label>
<input type="hidden" asp-for="@Model.EmployeeRoles[i].Id" />
<input type="hidden" asp-for="@Model.EmployeeRoles[i].Title" />
}
Then on controller , you can get the lists :
Then check the Selected
properties to confirm whether current role is selected .
edited Nov 26 '18 at 8:19
answered Nov 26 '18 at 8:00


Nan YuNan Yu
7,3052761
7,3052761
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53471487%2flist-of-checkboxes-are-lost-when-the-form-is-submitted%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
PC,0133A7CbQUNxJaR88JpoQSRj BkE32BSFG2j8nGNIV