JavaScript that executes after page load
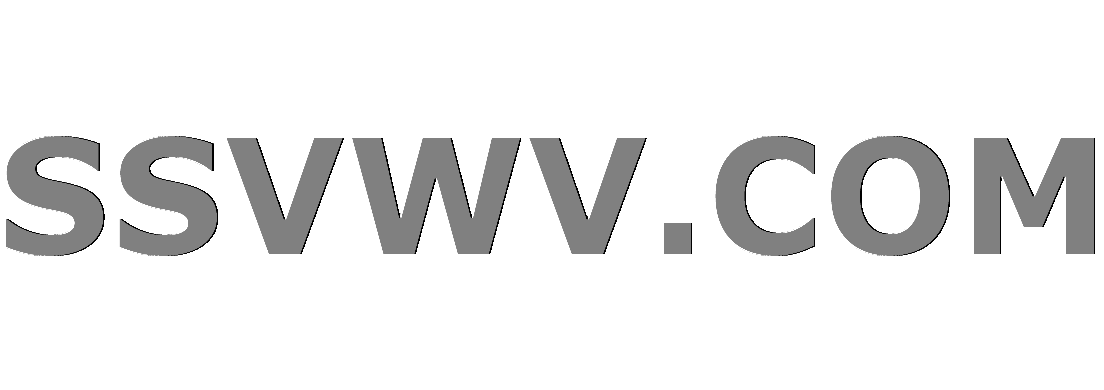
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I'm executing an external script, using a <script>
inside <head>
.
Now since the script executes before the page has loaded, I can't access the <body>
, among other things. I'd like to execute some JavaScript after the document has been "loaded" (HTML fully downloaded and in-RAM). Are there any events that I can hook onto when my script executes, that will get triggered on page load?
javascript html javascript-events pageload
add a comment |
I'm executing an external script, using a <script>
inside <head>
.
Now since the script executes before the page has loaded, I can't access the <body>
, among other things. I'd like to execute some JavaScript after the document has been "loaded" (HTML fully downloaded and in-RAM). Are there any events that I can hook onto when my script executes, that will get triggered on page load?
javascript html javascript-events pageload
add a comment |
I'm executing an external script, using a <script>
inside <head>
.
Now since the script executes before the page has loaded, I can't access the <body>
, among other things. I'd like to execute some JavaScript after the document has been "loaded" (HTML fully downloaded and in-RAM). Are there any events that I can hook onto when my script executes, that will get triggered on page load?
javascript html javascript-events pageload
I'm executing an external script, using a <script>
inside <head>
.
Now since the script executes before the page has loaded, I can't access the <body>
, among other things. I'd like to execute some JavaScript after the document has been "loaded" (HTML fully downloaded and in-RAM). Are there any events that I can hook onto when my script executes, that will get triggered on page load?
javascript html javascript-events pageload
javascript html javascript-events pageload
edited Jan 6 '18 at 21:53


Mark Amery
65.2k31258305
65.2k31258305
asked Apr 30 '09 at 16:38
RobinicksRobinicks
43.9k117341544
43.9k117341544
add a comment |
add a comment |
22 Answers
22
active
oldest
votes
These solutions will work:
<body onload="script();">
or
document.onload = function ...
or even
window.onload = function ...
Note that the last option is a better way to go since it is unobstrusive and is considered more standard.
5
What is the difference between <body onload="script();"> and document.onload=function ... ?
– Mentoliptus
Aug 16 '11 at 10:14
11
@mentoliptus:document.onload=
is non obtrusive en.wikipedia.org/wiki/Unobtrusive_JavaScript
– marcgg
Sep 5 '11 at 10:05
2
script in the html ...no no no $(function() { code here }); <- javascript.js can be placed in head and will load after DOM
– user1752532
Jan 17 '14 at 13:12
16
@gerdi OP didn't mention anything about jQuery. I also gave an unobtrusive option in my answer.
– marcgg
Jan 29 '14 at 14:48
@gerdi No problem, I edited my answer to show the difference more clearly
– marcgg
Jan 29 '14 at 14:53
|
show 6 more comments
Reasonably portable, non-framework way of having your script set a function to run at load time:
if(window.attachEvent) {
window.attachEvent('onload', yourFunctionName);
} else {
if(window.onload) {
var curronload = window.onload;
var newonload = function(evt) {
curronload(evt);
yourFunctionName(evt);
};
window.onload = newonload;
} else {
window.onload = yourFunctionName;
}
}
8
+1 for posting almost exactly what I had to come up with 6 months ago. Code like this can be necessary if other frameworks and code that you have no control over are adding onload events and you want to as well without wiping out the other onload events. I included my implementation as a function and it required var newonload = function(evt) { curronload(evt); newOnload(evt); } because for some reason the framework I am using requires an event to be passed to the onload event.
– Grant Wagner
Apr 30 '09 at 21:07
5
I just discovered in testing that the code as written results in handlers being fired in an undefined order when attached with attachEvent(). If order-of-handler-execution is important you may want to leave out the window.attachEvent branch.
– Grant Wagner
May 4 '09 at 20:00
So this will add this Function as an ADDITIONAL function to run OnLoad, correct? (rather than replacing an existing onload event)
– Clay Nichols
Jun 9 '17 at 4:47
@ClayNichols: Correct.
– chaos
Jun 9 '17 at 22:15
Good solution, but now outdated: developer.mozilla.org/en-US/docs/Web/API/EventTarget/…
– Renan
Nov 26 '17 at 5:25
add a comment |
You can put a "onload" attribute inside the body
...<body onload="myFunction()">...
Or if you are using jQuery, you can do
$(document).ready(function(){ /*code here*/ })
or
$(window).load(function(){ /*code here*/ })
I hope it answer your question.
Note that the $(window).load will execute after the document is rendered on your page.
add a comment |
Keep in mind that loading the page has more than one stage. Btw, this is pure JavaScript
"DOMContentLoaded"
This event is fired when the initial HTML document has been completely loaded and parsed, without waiting for stylesheets, images, and subframes to finish loading. At this stage you could programmatically optimize loading of images and css based on user device or bandwidth speed.
Exectues after DOM is loaded (before img and css):
document.addEventListener("DOMContentLoaded", function(){
//....
});
Note: Synchronous JavaScript pauses parsing of the DOM.
If you want the DOM to get parsed as fast as possible after the user requested the page, you could turn your JavaScript asynchronous and optimize loading of stylesheets
"load"
A very different event, load, should only be used to detect a fully-loaded page. It is an incredibly popular mistake to use load where DOMContentLoaded would be much more appropriate, so be cautious.
Exectues after everything is loaded and parsed:
window.addEventListener("load", function(){
// ....
});
MDN Resources:
https://developer.mozilla.org/en-US/docs/Web/Events/DOMContentLoaded
https://developer.mozilla.org/en-US/docs/Web/Events/load
MDN list of all events:
https://developer.mozilla.org/en-US/docs/Web/Events
Where do you place this code?
– Peter
Jul 11 '17 at 12:09
@Peter pretty sure this stands alone probably best if it in the bottom of your javascript files so it executes last
– arodebaugh
Jul 18 '17 at 14:59
Javascript pauses render of the DOM there for the best way would be to place it at the bottom of the page or to load javascript async in the header.
– DevWL
Sep 25 '17 at 22:29
1
best answer, thanks
– OZ_
Feb 27 '18 at 20:19
This is the most completed answer. onload is after, but people don't realize this at first.
– tfont
Jan 24 at 10:26
add a comment |
If the scripts are loaded within the <head>
of the document, then it's possible use the defer
attribute in script tag.
Example:
<script src="demo_defer.js" defer></script>
From https://developer.mozilla.org:
defer
This Boolean attribute is set to indicate to a browser that the script
is meant to be executed after the document has been parsed, but before
firing DOMContentLoaded.
This attribute must not be used if the src
attribute is absent (i.e. for inline scripts), in this case it would
have no effect.
To achieve a similar effect for dynamically inserted scripts use
async=false instead. Scripts with the defer attribute will execute in
the order in which they appear in the document.
2
This is definitely my favorite solution and the one I ended up going with.
– SomeoneRandom
May 3 '17 at 21:31
add a comment |
Here's a script based on deferred js loading after the page is loaded,
<script type="text/javascript">
function downloadJSAtOnload() {
var element = document.createElement("script");
element.src = "deferredfunctions.js";
document.body.appendChild(element);
}
if (window.addEventListener)
window.addEventListener("load", downloadJSAtOnload, false);
else if (window.attachEvent)
window.attachEvent("onload", downloadJSAtOnload);
else window.onload = downloadJSAtOnload;
</script>
Where do I place this?
Paste code in your HTML just before the
</body>
tag (near the bottom of your HTML file).
What does it do?
This code says wait for the entire document to load, then load the
external filedeferredfunctions.js
.
Here's an example of the above code - Defer Rendering of JS
I wrote this based on defered loading of javascript pagespeed google concept and also sourced from this article Defer loading javascript
add a comment |
Look at hooking document.onload
or in jQuery $(document).load(...)
.
Is this event reliable? (Cross browser.. IE6+ FF2+ etc)
– Robinicks
Apr 30 '09 at 16:41
1
Yes this is cross-browser, standard DOM.
– Daniel A. White
Apr 30 '09 at 16:43
13
It's actually window.onload that's more standard, not document.onload. AFAIK
– Peter Bailey
Apr 30 '09 at 16:48
1
Thanks for your correction.
– Daniel A. White
Apr 30 '09 at 16:51
add a comment |
Best method, recommended by Google also. :)
<script type="text/javascript">
function downloadJSAtOnload() {
var element = document.createElement("script");
element.src = "defer.js";
document.body.appendChild(element);
}
if (window.addEventListener)
window.addEventListener("load", downloadJSAtOnload, false);
else if (window.attachEvent)
window.attachEvent("onload", downloadJSAtOnload);
else window.onload = downloadJSAtOnload;
</script>
http://www.feedthebot.com/pagespeed/defer-loading-javascript.html
load script in last order. just what i need!!! good work with asynh js
– Vladimir Ch
Jan 17 '17 at 10:46
add a comment |
Working Fiddle
<!DOCTYPE html>
<html>
<head>
<script>
function myFunction()
{
alert("Page is loaded");
}
</script>
</head>
<body onload="myFunction()">
<h1>Hello World!</h1>
</body>
</html>
add a comment |
If you are using jQuery,
$(function() {...});
is equivalent to
$(document).ready(function () { })
See What event does JQuery $function() fire on?
add a comment |
document.onreadystatechange = function(){
if(document.readyState === 'complete'){
/*code here*/
}
}
look here: http://msdn.microsoft.com/en-us/library/ie/ms536957(v=vs.85).aspx
2
one-line: document.onload = function() { ... }
– colminator
Mar 26 '15 at 17:31
best solution in my opinion. Good for Jquery not defined issue
– S.M. Nat
Dec 12 '18 at 7:20
add a comment |
<body onload="myFunction()">
This code works well.
But window.onload
method has various dependencies. So it may not work all the time.
add a comment |
I find sometimes on more complex pages that not all the elements have loaded by the time window.onload is fired. If that's the case, add setTimeout before your function to delay is a moment. It's not elegant but it's a simple hack that renders well.
window.onload = function(){ doSomethingCool(); };
becomes...
window.onload = function(){ setTimeout( function(){ doSomethingCool(); }, 1000); };
add a comment |
Just define <body onload="aFunction()">
that will be called after the page has been loaded. Your code in the script is than enclosed by aFunction() { }
.
I was too hasty and forgot to use &lt; instead of <. Sorry, but it all went soo fast
– Norbert Hartl
Apr 30 '09 at 16:46
Well is it different in comments? <bla>
– Norbert Hartl
Apr 30 '09 at 16:47
add a comment |
$(window).on("load", function(){ ... });
.ready() works best for me.
$(document).ready(function(){ ... });
.load() will work, but it won't wait till the page is loaded.
jQuery(window).load(function () { ... });
Doesn't work for me, breaks the next-to inline script. I am also using jQuery 3.2.1 along with some other jQuery forks.
To hide my websites loading overlay, I use the following:
<script>
$(window).on("load", function(){
$('.loading-page').delay(3000).fadeOut(250);
});
</script>
3
you write a answer 9 year later but with jQuery :P
– Anirudha Gupta
Oct 13 '18 at 11:51
add a comment |
Using the YUI library (I love it):
YAHOO.util.Event.onDOMReady(function(){
//your code
});
Portable and beautiful! However, if you don't use YUI for other stuff (see its doc) I would say that it's not worth to use it.
N.B. : to use this code you need to import 2 scripts
<script type="text/javascript" src="http://yui.yahooapis.com/2.7.0/build/yahoo/yahoo-min.js" ></script>
<script type="text/javascript" src="http://yui.yahooapis.com/2.7.0/build/event/event-min.js" ></script>
add a comment |
There is a very good documentation on How to detect if document has loaded using Javascript or Jquery.
Using the native Javascript this can be achieved
if (document.readyState === "complete") {
init();
}
This can also be done inside the interval
var interval = setInterval(function() {
if(document.readyState === 'complete') {
clearInterval(interval);
init();
}
}, 100);
Eg By Mozilla
switch (document.readyState) {
case "loading":
// The document is still loading.
break;
case "interactive":
// The document has finished loading. We can now access the DOM elements.
var span = document.createElement("span");
span.textContent = "A <span> element.";
document.body.appendChild(span);
break;
case "complete":
// The page is fully loaded.
console.log("Page is loaded completely");
break;
}
Using Jquery
To check only if DOM is ready
// A $( document ).ready() block.
$( document ).ready(function() {
console.log( "ready!" );
});
To check if all resources are loaded use window.load
$( window ).load(function() {
console.log( "window loaded" );
});
add a comment |
Use this code with jQuery library, this would work perfectly fine.
$(window).bind("load", function() {
// your javascript event
});
Add some description here
– Billa
Dec 21 '17 at 13:50
add a comment |
As Daniel says, you could use document.onload.
The various javascript frameworks hwoever (jQuery, Mootools, etc.) use a custom event 'domready', which I guess must be more effective. If you're developing with javascript, I'd highly recommend exploiting a framework, they massively increase your productivity.
Pity browsers didn't do what frameworks do.
– Robinicks
Apr 30 '09 at 16:54
add a comment |
My advise use asnyc
attribute for script tag thats help you to load the external scripts after page load
<script type="text/javascript" src="a.js" async></script>
<script type="text/javascript" src="b.js" async></script>
5
According to w3schools, If async is present: The script is executed asynchronously with the rest of the page (the script will be executed while the page continues the parsing). Perhaps you meantdefer
instead, like @Daniel Price mentioned?
– jk7
Apr 15 '15 at 0:06
add a comment |
<script type="text/javascript">
$(window).bind("load", function() {
// your javascript event here
});
</script>
1
This answer depends on jquery
– Chiptus
Sep 5 '18 at 11:38
add a comment |
use self execution onload function
window.onload = function (){
/* statements */
}();
You may override otheronload
handlers by using this approach. Instead, you should add listener.
– Leonid Dashko
May 29 '18 at 18:45
add a comment |
protected by K. Sopheak Dec 20 '18 at 6:49
Thank you for your interest in this question.
Because it has attracted low-quality or spam answers that had to be removed, posting an answer now requires 10 reputation on this site (the association bonus does not count).
Would you like to answer one of these unanswered questions instead?
22 Answers
22
active
oldest
votes
22 Answers
22
active
oldest
votes
active
oldest
votes
active
oldest
votes
These solutions will work:
<body onload="script();">
or
document.onload = function ...
or even
window.onload = function ...
Note that the last option is a better way to go since it is unobstrusive and is considered more standard.
5
What is the difference between <body onload="script();"> and document.onload=function ... ?
– Mentoliptus
Aug 16 '11 at 10:14
11
@mentoliptus:document.onload=
is non obtrusive en.wikipedia.org/wiki/Unobtrusive_JavaScript
– marcgg
Sep 5 '11 at 10:05
2
script in the html ...no no no $(function() { code here }); <- javascript.js can be placed in head and will load after DOM
– user1752532
Jan 17 '14 at 13:12
16
@gerdi OP didn't mention anything about jQuery. I also gave an unobtrusive option in my answer.
– marcgg
Jan 29 '14 at 14:48
@gerdi No problem, I edited my answer to show the difference more clearly
– marcgg
Jan 29 '14 at 14:53
|
show 6 more comments
These solutions will work:
<body onload="script();">
or
document.onload = function ...
or even
window.onload = function ...
Note that the last option is a better way to go since it is unobstrusive and is considered more standard.
5
What is the difference between <body onload="script();"> and document.onload=function ... ?
– Mentoliptus
Aug 16 '11 at 10:14
11
@mentoliptus:document.onload=
is non obtrusive en.wikipedia.org/wiki/Unobtrusive_JavaScript
– marcgg
Sep 5 '11 at 10:05
2
script in the html ...no no no $(function() { code here }); <- javascript.js can be placed in head and will load after DOM
– user1752532
Jan 17 '14 at 13:12
16
@gerdi OP didn't mention anything about jQuery. I also gave an unobtrusive option in my answer.
– marcgg
Jan 29 '14 at 14:48
@gerdi No problem, I edited my answer to show the difference more clearly
– marcgg
Jan 29 '14 at 14:53
|
show 6 more comments
These solutions will work:
<body onload="script();">
or
document.onload = function ...
or even
window.onload = function ...
Note that the last option is a better way to go since it is unobstrusive and is considered more standard.
These solutions will work:
<body onload="script();">
or
document.onload = function ...
or even
window.onload = function ...
Note that the last option is a better way to go since it is unobstrusive and is considered more standard.
edited May 23 '17 at 12:26
Community♦
11
11
answered Apr 30 '09 at 16:41
marcggmarcgg
42.8k46163214
42.8k46163214
5
What is the difference between <body onload="script();"> and document.onload=function ... ?
– Mentoliptus
Aug 16 '11 at 10:14
11
@mentoliptus:document.onload=
is non obtrusive en.wikipedia.org/wiki/Unobtrusive_JavaScript
– marcgg
Sep 5 '11 at 10:05
2
script in the html ...no no no $(function() { code here }); <- javascript.js can be placed in head and will load after DOM
– user1752532
Jan 17 '14 at 13:12
16
@gerdi OP didn't mention anything about jQuery. I also gave an unobtrusive option in my answer.
– marcgg
Jan 29 '14 at 14:48
@gerdi No problem, I edited my answer to show the difference more clearly
– marcgg
Jan 29 '14 at 14:53
|
show 6 more comments
5
What is the difference between <body onload="script();"> and document.onload=function ... ?
– Mentoliptus
Aug 16 '11 at 10:14
11
@mentoliptus:document.onload=
is non obtrusive en.wikipedia.org/wiki/Unobtrusive_JavaScript
– marcgg
Sep 5 '11 at 10:05
2
script in the html ...no no no $(function() { code here }); <- javascript.js can be placed in head and will load after DOM
– user1752532
Jan 17 '14 at 13:12
16
@gerdi OP didn't mention anything about jQuery. I also gave an unobtrusive option in my answer.
– marcgg
Jan 29 '14 at 14:48
@gerdi No problem, I edited my answer to show the difference more clearly
– marcgg
Jan 29 '14 at 14:53
5
5
What is the difference between <body onload="script();"> and document.onload=function ... ?
– Mentoliptus
Aug 16 '11 at 10:14
What is the difference between <body onload="script();"> and document.onload=function ... ?
– Mentoliptus
Aug 16 '11 at 10:14
11
11
@mentoliptus:
document.onload=
is non obtrusive en.wikipedia.org/wiki/Unobtrusive_JavaScript– marcgg
Sep 5 '11 at 10:05
@mentoliptus:
document.onload=
is non obtrusive en.wikipedia.org/wiki/Unobtrusive_JavaScript– marcgg
Sep 5 '11 at 10:05
2
2
script in the html ...no no no $(function() { code here }); <- javascript.js can be placed in head and will load after DOM
– user1752532
Jan 17 '14 at 13:12
script in the html ...no no no $(function() { code here }); <- javascript.js can be placed in head and will load after DOM
– user1752532
Jan 17 '14 at 13:12
16
16
@gerdi OP didn't mention anything about jQuery. I also gave an unobtrusive option in my answer.
– marcgg
Jan 29 '14 at 14:48
@gerdi OP didn't mention anything about jQuery. I also gave an unobtrusive option in my answer.
– marcgg
Jan 29 '14 at 14:48
@gerdi No problem, I edited my answer to show the difference more clearly
– marcgg
Jan 29 '14 at 14:53
@gerdi No problem, I edited my answer to show the difference more clearly
– marcgg
Jan 29 '14 at 14:53
|
show 6 more comments
Reasonably portable, non-framework way of having your script set a function to run at load time:
if(window.attachEvent) {
window.attachEvent('onload', yourFunctionName);
} else {
if(window.onload) {
var curronload = window.onload;
var newonload = function(evt) {
curronload(evt);
yourFunctionName(evt);
};
window.onload = newonload;
} else {
window.onload = yourFunctionName;
}
}
8
+1 for posting almost exactly what I had to come up with 6 months ago. Code like this can be necessary if other frameworks and code that you have no control over are adding onload events and you want to as well without wiping out the other onload events. I included my implementation as a function and it required var newonload = function(evt) { curronload(evt); newOnload(evt); } because for some reason the framework I am using requires an event to be passed to the onload event.
– Grant Wagner
Apr 30 '09 at 21:07
5
I just discovered in testing that the code as written results in handlers being fired in an undefined order when attached with attachEvent(). If order-of-handler-execution is important you may want to leave out the window.attachEvent branch.
– Grant Wagner
May 4 '09 at 20:00
So this will add this Function as an ADDITIONAL function to run OnLoad, correct? (rather than replacing an existing onload event)
– Clay Nichols
Jun 9 '17 at 4:47
@ClayNichols: Correct.
– chaos
Jun 9 '17 at 22:15
Good solution, but now outdated: developer.mozilla.org/en-US/docs/Web/API/EventTarget/…
– Renan
Nov 26 '17 at 5:25
add a comment |
Reasonably portable, non-framework way of having your script set a function to run at load time:
if(window.attachEvent) {
window.attachEvent('onload', yourFunctionName);
} else {
if(window.onload) {
var curronload = window.onload;
var newonload = function(evt) {
curronload(evt);
yourFunctionName(evt);
};
window.onload = newonload;
} else {
window.onload = yourFunctionName;
}
}
8
+1 for posting almost exactly what I had to come up with 6 months ago. Code like this can be necessary if other frameworks and code that you have no control over are adding onload events and you want to as well without wiping out the other onload events. I included my implementation as a function and it required var newonload = function(evt) { curronload(evt); newOnload(evt); } because for some reason the framework I am using requires an event to be passed to the onload event.
– Grant Wagner
Apr 30 '09 at 21:07
5
I just discovered in testing that the code as written results in handlers being fired in an undefined order when attached with attachEvent(). If order-of-handler-execution is important you may want to leave out the window.attachEvent branch.
– Grant Wagner
May 4 '09 at 20:00
So this will add this Function as an ADDITIONAL function to run OnLoad, correct? (rather than replacing an existing onload event)
– Clay Nichols
Jun 9 '17 at 4:47
@ClayNichols: Correct.
– chaos
Jun 9 '17 at 22:15
Good solution, but now outdated: developer.mozilla.org/en-US/docs/Web/API/EventTarget/…
– Renan
Nov 26 '17 at 5:25
add a comment |
Reasonably portable, non-framework way of having your script set a function to run at load time:
if(window.attachEvent) {
window.attachEvent('onload', yourFunctionName);
} else {
if(window.onload) {
var curronload = window.onload;
var newonload = function(evt) {
curronload(evt);
yourFunctionName(evt);
};
window.onload = newonload;
} else {
window.onload = yourFunctionName;
}
}
Reasonably portable, non-framework way of having your script set a function to run at load time:
if(window.attachEvent) {
window.attachEvent('onload', yourFunctionName);
} else {
if(window.onload) {
var curronload = window.onload;
var newonload = function(evt) {
curronload(evt);
yourFunctionName(evt);
};
window.onload = newonload;
} else {
window.onload = yourFunctionName;
}
}
edited Oct 8 '15 at 13:45
answered Apr 30 '09 at 16:59
chaoschaos
105k26273289
105k26273289
8
+1 for posting almost exactly what I had to come up with 6 months ago. Code like this can be necessary if other frameworks and code that you have no control over are adding onload events and you want to as well without wiping out the other onload events. I included my implementation as a function and it required var newonload = function(evt) { curronload(evt); newOnload(evt); } because for some reason the framework I am using requires an event to be passed to the onload event.
– Grant Wagner
Apr 30 '09 at 21:07
5
I just discovered in testing that the code as written results in handlers being fired in an undefined order when attached with attachEvent(). If order-of-handler-execution is important you may want to leave out the window.attachEvent branch.
– Grant Wagner
May 4 '09 at 20:00
So this will add this Function as an ADDITIONAL function to run OnLoad, correct? (rather than replacing an existing onload event)
– Clay Nichols
Jun 9 '17 at 4:47
@ClayNichols: Correct.
– chaos
Jun 9 '17 at 22:15
Good solution, but now outdated: developer.mozilla.org/en-US/docs/Web/API/EventTarget/…
– Renan
Nov 26 '17 at 5:25
add a comment |
8
+1 for posting almost exactly what I had to come up with 6 months ago. Code like this can be necessary if other frameworks and code that you have no control over are adding onload events and you want to as well without wiping out the other onload events. I included my implementation as a function and it required var newonload = function(evt) { curronload(evt); newOnload(evt); } because for some reason the framework I am using requires an event to be passed to the onload event.
– Grant Wagner
Apr 30 '09 at 21:07
5
I just discovered in testing that the code as written results in handlers being fired in an undefined order when attached with attachEvent(). If order-of-handler-execution is important you may want to leave out the window.attachEvent branch.
– Grant Wagner
May 4 '09 at 20:00
So this will add this Function as an ADDITIONAL function to run OnLoad, correct? (rather than replacing an existing onload event)
– Clay Nichols
Jun 9 '17 at 4:47
@ClayNichols: Correct.
– chaos
Jun 9 '17 at 22:15
Good solution, but now outdated: developer.mozilla.org/en-US/docs/Web/API/EventTarget/…
– Renan
Nov 26 '17 at 5:25
8
8
+1 for posting almost exactly what I had to come up with 6 months ago. Code like this can be necessary if other frameworks and code that you have no control over are adding onload events and you want to as well without wiping out the other onload events. I included my implementation as a function and it required var newonload = function(evt) { curronload(evt); newOnload(evt); } because for some reason the framework I am using requires an event to be passed to the onload event.
– Grant Wagner
Apr 30 '09 at 21:07
+1 for posting almost exactly what I had to come up with 6 months ago. Code like this can be necessary if other frameworks and code that you have no control over are adding onload events and you want to as well without wiping out the other onload events. I included my implementation as a function and it required var newonload = function(evt) { curronload(evt); newOnload(evt); } because for some reason the framework I am using requires an event to be passed to the onload event.
– Grant Wagner
Apr 30 '09 at 21:07
5
5
I just discovered in testing that the code as written results in handlers being fired in an undefined order when attached with attachEvent(). If order-of-handler-execution is important you may want to leave out the window.attachEvent branch.
– Grant Wagner
May 4 '09 at 20:00
I just discovered in testing that the code as written results in handlers being fired in an undefined order when attached with attachEvent(). If order-of-handler-execution is important you may want to leave out the window.attachEvent branch.
– Grant Wagner
May 4 '09 at 20:00
So this will add this Function as an ADDITIONAL function to run OnLoad, correct? (rather than replacing an existing onload event)
– Clay Nichols
Jun 9 '17 at 4:47
So this will add this Function as an ADDITIONAL function to run OnLoad, correct? (rather than replacing an existing onload event)
– Clay Nichols
Jun 9 '17 at 4:47
@ClayNichols: Correct.
– chaos
Jun 9 '17 at 22:15
@ClayNichols: Correct.
– chaos
Jun 9 '17 at 22:15
Good solution, but now outdated: developer.mozilla.org/en-US/docs/Web/API/EventTarget/…
– Renan
Nov 26 '17 at 5:25
Good solution, but now outdated: developer.mozilla.org/en-US/docs/Web/API/EventTarget/…
– Renan
Nov 26 '17 at 5:25
add a comment |
You can put a "onload" attribute inside the body
...<body onload="myFunction()">...
Or if you are using jQuery, you can do
$(document).ready(function(){ /*code here*/ })
or
$(window).load(function(){ /*code here*/ })
I hope it answer your question.
Note that the $(window).load will execute after the document is rendered on your page.
add a comment |
You can put a "onload" attribute inside the body
...<body onload="myFunction()">...
Or if you are using jQuery, you can do
$(document).ready(function(){ /*code here*/ })
or
$(window).load(function(){ /*code here*/ })
I hope it answer your question.
Note that the $(window).load will execute after the document is rendered on your page.
add a comment |
You can put a "onload" attribute inside the body
...<body onload="myFunction()">...
Or if you are using jQuery, you can do
$(document).ready(function(){ /*code here*/ })
or
$(window).load(function(){ /*code here*/ })
I hope it answer your question.
Note that the $(window).load will execute after the document is rendered on your page.
You can put a "onload" attribute inside the body
...<body onload="myFunction()">...
Or if you are using jQuery, you can do
$(document).ready(function(){ /*code here*/ })
or
$(window).load(function(){ /*code here*/ })
I hope it answer your question.
Note that the $(window).load will execute after the document is rendered on your page.
answered Apr 30 '09 at 16:42


NordesNordes
1,80521625
1,80521625
add a comment |
add a comment |
Keep in mind that loading the page has more than one stage. Btw, this is pure JavaScript
"DOMContentLoaded"
This event is fired when the initial HTML document has been completely loaded and parsed, without waiting for stylesheets, images, and subframes to finish loading. At this stage you could programmatically optimize loading of images and css based on user device or bandwidth speed.
Exectues after DOM is loaded (before img and css):
document.addEventListener("DOMContentLoaded", function(){
//....
});
Note: Synchronous JavaScript pauses parsing of the DOM.
If you want the DOM to get parsed as fast as possible after the user requested the page, you could turn your JavaScript asynchronous and optimize loading of stylesheets
"load"
A very different event, load, should only be used to detect a fully-loaded page. It is an incredibly popular mistake to use load where DOMContentLoaded would be much more appropriate, so be cautious.
Exectues after everything is loaded and parsed:
window.addEventListener("load", function(){
// ....
});
MDN Resources:
https://developer.mozilla.org/en-US/docs/Web/Events/DOMContentLoaded
https://developer.mozilla.org/en-US/docs/Web/Events/load
MDN list of all events:
https://developer.mozilla.org/en-US/docs/Web/Events
Where do you place this code?
– Peter
Jul 11 '17 at 12:09
@Peter pretty sure this stands alone probably best if it in the bottom of your javascript files so it executes last
– arodebaugh
Jul 18 '17 at 14:59
Javascript pauses render of the DOM there for the best way would be to place it at the bottom of the page or to load javascript async in the header.
– DevWL
Sep 25 '17 at 22:29
1
best answer, thanks
– OZ_
Feb 27 '18 at 20:19
This is the most completed answer. onload is after, but people don't realize this at first.
– tfont
Jan 24 at 10:26
add a comment |
Keep in mind that loading the page has more than one stage. Btw, this is pure JavaScript
"DOMContentLoaded"
This event is fired when the initial HTML document has been completely loaded and parsed, without waiting for stylesheets, images, and subframes to finish loading. At this stage you could programmatically optimize loading of images and css based on user device or bandwidth speed.
Exectues after DOM is loaded (before img and css):
document.addEventListener("DOMContentLoaded", function(){
//....
});
Note: Synchronous JavaScript pauses parsing of the DOM.
If you want the DOM to get parsed as fast as possible after the user requested the page, you could turn your JavaScript asynchronous and optimize loading of stylesheets
"load"
A very different event, load, should only be used to detect a fully-loaded page. It is an incredibly popular mistake to use load where DOMContentLoaded would be much more appropriate, so be cautious.
Exectues after everything is loaded and parsed:
window.addEventListener("load", function(){
// ....
});
MDN Resources:
https://developer.mozilla.org/en-US/docs/Web/Events/DOMContentLoaded
https://developer.mozilla.org/en-US/docs/Web/Events/load
MDN list of all events:
https://developer.mozilla.org/en-US/docs/Web/Events
Where do you place this code?
– Peter
Jul 11 '17 at 12:09
@Peter pretty sure this stands alone probably best if it in the bottom of your javascript files so it executes last
– arodebaugh
Jul 18 '17 at 14:59
Javascript pauses render of the DOM there for the best way would be to place it at the bottom of the page or to load javascript async in the header.
– DevWL
Sep 25 '17 at 22:29
1
best answer, thanks
– OZ_
Feb 27 '18 at 20:19
This is the most completed answer. onload is after, but people don't realize this at first.
– tfont
Jan 24 at 10:26
add a comment |
Keep in mind that loading the page has more than one stage. Btw, this is pure JavaScript
"DOMContentLoaded"
This event is fired when the initial HTML document has been completely loaded and parsed, without waiting for stylesheets, images, and subframes to finish loading. At this stage you could programmatically optimize loading of images and css based on user device or bandwidth speed.
Exectues after DOM is loaded (before img and css):
document.addEventListener("DOMContentLoaded", function(){
//....
});
Note: Synchronous JavaScript pauses parsing of the DOM.
If you want the DOM to get parsed as fast as possible after the user requested the page, you could turn your JavaScript asynchronous and optimize loading of stylesheets
"load"
A very different event, load, should only be used to detect a fully-loaded page. It is an incredibly popular mistake to use load where DOMContentLoaded would be much more appropriate, so be cautious.
Exectues after everything is loaded and parsed:
window.addEventListener("load", function(){
// ....
});
MDN Resources:
https://developer.mozilla.org/en-US/docs/Web/Events/DOMContentLoaded
https://developer.mozilla.org/en-US/docs/Web/Events/load
MDN list of all events:
https://developer.mozilla.org/en-US/docs/Web/Events
Keep in mind that loading the page has more than one stage. Btw, this is pure JavaScript
"DOMContentLoaded"
This event is fired when the initial HTML document has been completely loaded and parsed, without waiting for stylesheets, images, and subframes to finish loading. At this stage you could programmatically optimize loading of images and css based on user device or bandwidth speed.
Exectues after DOM is loaded (before img and css):
document.addEventListener("DOMContentLoaded", function(){
//....
});
Note: Synchronous JavaScript pauses parsing of the DOM.
If you want the DOM to get parsed as fast as possible after the user requested the page, you could turn your JavaScript asynchronous and optimize loading of stylesheets
"load"
A very different event, load, should only be used to detect a fully-loaded page. It is an incredibly popular mistake to use load where DOMContentLoaded would be much more appropriate, so be cautious.
Exectues after everything is loaded and parsed:
window.addEventListener("load", function(){
// ....
});
MDN Resources:
https://developer.mozilla.org/en-US/docs/Web/Events/DOMContentLoaded
https://developer.mozilla.org/en-US/docs/Web/Events/load
MDN list of all events:
https://developer.mozilla.org/en-US/docs/Web/Events
edited Nov 14 '17 at 15:05


RageFoxx
94
94
answered Mar 19 '16 at 0:50


DevWLDevWL
6,89035354
6,89035354
Where do you place this code?
– Peter
Jul 11 '17 at 12:09
@Peter pretty sure this stands alone probably best if it in the bottom of your javascript files so it executes last
– arodebaugh
Jul 18 '17 at 14:59
Javascript pauses render of the DOM there for the best way would be to place it at the bottom of the page or to load javascript async in the header.
– DevWL
Sep 25 '17 at 22:29
1
best answer, thanks
– OZ_
Feb 27 '18 at 20:19
This is the most completed answer. onload is after, but people don't realize this at first.
– tfont
Jan 24 at 10:26
add a comment |
Where do you place this code?
– Peter
Jul 11 '17 at 12:09
@Peter pretty sure this stands alone probably best if it in the bottom of your javascript files so it executes last
– arodebaugh
Jul 18 '17 at 14:59
Javascript pauses render of the DOM there for the best way would be to place it at the bottom of the page or to load javascript async in the header.
– DevWL
Sep 25 '17 at 22:29
1
best answer, thanks
– OZ_
Feb 27 '18 at 20:19
This is the most completed answer. onload is after, but people don't realize this at first.
– tfont
Jan 24 at 10:26
Where do you place this code?
– Peter
Jul 11 '17 at 12:09
Where do you place this code?
– Peter
Jul 11 '17 at 12:09
@Peter pretty sure this stands alone probably best if it in the bottom of your javascript files so it executes last
– arodebaugh
Jul 18 '17 at 14:59
@Peter pretty sure this stands alone probably best if it in the bottom of your javascript files so it executes last
– arodebaugh
Jul 18 '17 at 14:59
Javascript pauses render of the DOM there for the best way would be to place it at the bottom of the page or to load javascript async in the header.
– DevWL
Sep 25 '17 at 22:29
Javascript pauses render of the DOM there for the best way would be to place it at the bottom of the page or to load javascript async in the header.
– DevWL
Sep 25 '17 at 22:29
1
1
best answer, thanks
– OZ_
Feb 27 '18 at 20:19
best answer, thanks
– OZ_
Feb 27 '18 at 20:19
This is the most completed answer. onload is after, but people don't realize this at first.
– tfont
Jan 24 at 10:26
This is the most completed answer. onload is after, but people don't realize this at first.
– tfont
Jan 24 at 10:26
add a comment |
If the scripts are loaded within the <head>
of the document, then it's possible use the defer
attribute in script tag.
Example:
<script src="demo_defer.js" defer></script>
From https://developer.mozilla.org:
defer
This Boolean attribute is set to indicate to a browser that the script
is meant to be executed after the document has been parsed, but before
firing DOMContentLoaded.
This attribute must not be used if the src
attribute is absent (i.e. for inline scripts), in this case it would
have no effect.
To achieve a similar effect for dynamically inserted scripts use
async=false instead. Scripts with the defer attribute will execute in
the order in which they appear in the document.
2
This is definitely my favorite solution and the one I ended up going with.
– SomeoneRandom
May 3 '17 at 21:31
add a comment |
If the scripts are loaded within the <head>
of the document, then it's possible use the defer
attribute in script tag.
Example:
<script src="demo_defer.js" defer></script>
From https://developer.mozilla.org:
defer
This Boolean attribute is set to indicate to a browser that the script
is meant to be executed after the document has been parsed, but before
firing DOMContentLoaded.
This attribute must not be used if the src
attribute is absent (i.e. for inline scripts), in this case it would
have no effect.
To achieve a similar effect for dynamically inserted scripts use
async=false instead. Scripts with the defer attribute will execute in
the order in which they appear in the document.
2
This is definitely my favorite solution and the one I ended up going with.
– SomeoneRandom
May 3 '17 at 21:31
add a comment |
If the scripts are loaded within the <head>
of the document, then it's possible use the defer
attribute in script tag.
Example:
<script src="demo_defer.js" defer></script>
From https://developer.mozilla.org:
defer
This Boolean attribute is set to indicate to a browser that the script
is meant to be executed after the document has been parsed, but before
firing DOMContentLoaded.
This attribute must not be used if the src
attribute is absent (i.e. for inline scripts), in this case it would
have no effect.
To achieve a similar effect for dynamically inserted scripts use
async=false instead. Scripts with the defer attribute will execute in
the order in which they appear in the document.
If the scripts are loaded within the <head>
of the document, then it's possible use the defer
attribute in script tag.
Example:
<script src="demo_defer.js" defer></script>
From https://developer.mozilla.org:
defer
This Boolean attribute is set to indicate to a browser that the script
is meant to be executed after the document has been parsed, but before
firing DOMContentLoaded.
This attribute must not be used if the src
attribute is absent (i.e. for inline scripts), in this case it would
have no effect.
To achieve a similar effect for dynamically inserted scripts use
async=false instead. Scripts with the defer attribute will execute in
the order in which they appear in the document.
edited Feb 21 '18 at 13:48
answered Jan 10 '14 at 8:52


Daniel PriceDaniel Price
707516
707516
2
This is definitely my favorite solution and the one I ended up going with.
– SomeoneRandom
May 3 '17 at 21:31
add a comment |
2
This is definitely my favorite solution and the one I ended up going with.
– SomeoneRandom
May 3 '17 at 21:31
2
2
This is definitely my favorite solution and the one I ended up going with.
– SomeoneRandom
May 3 '17 at 21:31
This is definitely my favorite solution and the one I ended up going with.
– SomeoneRandom
May 3 '17 at 21:31
add a comment |
Here's a script based on deferred js loading after the page is loaded,
<script type="text/javascript">
function downloadJSAtOnload() {
var element = document.createElement("script");
element.src = "deferredfunctions.js";
document.body.appendChild(element);
}
if (window.addEventListener)
window.addEventListener("load", downloadJSAtOnload, false);
else if (window.attachEvent)
window.attachEvent("onload", downloadJSAtOnload);
else window.onload = downloadJSAtOnload;
</script>
Where do I place this?
Paste code in your HTML just before the
</body>
tag (near the bottom of your HTML file).
What does it do?
This code says wait for the entire document to load, then load the
external filedeferredfunctions.js
.
Here's an example of the above code - Defer Rendering of JS
I wrote this based on defered loading of javascript pagespeed google concept and also sourced from this article Defer loading javascript
add a comment |
Here's a script based on deferred js loading after the page is loaded,
<script type="text/javascript">
function downloadJSAtOnload() {
var element = document.createElement("script");
element.src = "deferredfunctions.js";
document.body.appendChild(element);
}
if (window.addEventListener)
window.addEventListener("load", downloadJSAtOnload, false);
else if (window.attachEvent)
window.attachEvent("onload", downloadJSAtOnload);
else window.onload = downloadJSAtOnload;
</script>
Where do I place this?
Paste code in your HTML just before the
</body>
tag (near the bottom of your HTML file).
What does it do?
This code says wait for the entire document to load, then load the
external filedeferredfunctions.js
.
Here's an example of the above code - Defer Rendering of JS
I wrote this based on defered loading of javascript pagespeed google concept and also sourced from this article Defer loading javascript
add a comment |
Here's a script based on deferred js loading after the page is loaded,
<script type="text/javascript">
function downloadJSAtOnload() {
var element = document.createElement("script");
element.src = "deferredfunctions.js";
document.body.appendChild(element);
}
if (window.addEventListener)
window.addEventListener("load", downloadJSAtOnload, false);
else if (window.attachEvent)
window.attachEvent("onload", downloadJSAtOnload);
else window.onload = downloadJSAtOnload;
</script>
Where do I place this?
Paste code in your HTML just before the
</body>
tag (near the bottom of your HTML file).
What does it do?
This code says wait for the entire document to load, then load the
external filedeferredfunctions.js
.
Here's an example of the above code - Defer Rendering of JS
I wrote this based on defered loading of javascript pagespeed google concept and also sourced from this article Defer loading javascript
Here's a script based on deferred js loading after the page is loaded,
<script type="text/javascript">
function downloadJSAtOnload() {
var element = document.createElement("script");
element.src = "deferredfunctions.js";
document.body.appendChild(element);
}
if (window.addEventListener)
window.addEventListener("load", downloadJSAtOnload, false);
else if (window.attachEvent)
window.attachEvent("onload", downloadJSAtOnload);
else window.onload = downloadJSAtOnload;
</script>
Where do I place this?
Paste code in your HTML just before the
</body>
tag (near the bottom of your HTML file).
What does it do?
This code says wait for the entire document to load, then load the
external filedeferredfunctions.js
.
Here's an example of the above code - Defer Rendering of JS
I wrote this based on defered loading of javascript pagespeed google concept and also sourced from this article Defer loading javascript
edited Mar 22 '17 at 10:37
answered Feb 5 '13 at 12:40


LuckyLucky
11.1k1182120
11.1k1182120
add a comment |
add a comment |
Look at hooking document.onload
or in jQuery $(document).load(...)
.
Is this event reliable? (Cross browser.. IE6+ FF2+ etc)
– Robinicks
Apr 30 '09 at 16:41
1
Yes this is cross-browser, standard DOM.
– Daniel A. White
Apr 30 '09 at 16:43
13
It's actually window.onload that's more standard, not document.onload. AFAIK
– Peter Bailey
Apr 30 '09 at 16:48
1
Thanks for your correction.
– Daniel A. White
Apr 30 '09 at 16:51
add a comment |
Look at hooking document.onload
or in jQuery $(document).load(...)
.
Is this event reliable? (Cross browser.. IE6+ FF2+ etc)
– Robinicks
Apr 30 '09 at 16:41
1
Yes this is cross-browser, standard DOM.
– Daniel A. White
Apr 30 '09 at 16:43
13
It's actually window.onload that's more standard, not document.onload. AFAIK
– Peter Bailey
Apr 30 '09 at 16:48
1
Thanks for your correction.
– Daniel A. White
Apr 30 '09 at 16:51
add a comment |
Look at hooking document.onload
or in jQuery $(document).load(...)
.
Look at hooking document.onload
or in jQuery $(document).load(...)
.
answered Apr 30 '09 at 16:40
Daniel A. WhiteDaniel A. White
151k37297377
151k37297377
Is this event reliable? (Cross browser.. IE6+ FF2+ etc)
– Robinicks
Apr 30 '09 at 16:41
1
Yes this is cross-browser, standard DOM.
– Daniel A. White
Apr 30 '09 at 16:43
13
It's actually window.onload that's more standard, not document.onload. AFAIK
– Peter Bailey
Apr 30 '09 at 16:48
1
Thanks for your correction.
– Daniel A. White
Apr 30 '09 at 16:51
add a comment |
Is this event reliable? (Cross browser.. IE6+ FF2+ etc)
– Robinicks
Apr 30 '09 at 16:41
1
Yes this is cross-browser, standard DOM.
– Daniel A. White
Apr 30 '09 at 16:43
13
It's actually window.onload that's more standard, not document.onload. AFAIK
– Peter Bailey
Apr 30 '09 at 16:48
1
Thanks for your correction.
– Daniel A. White
Apr 30 '09 at 16:51
Is this event reliable? (Cross browser.. IE6+ FF2+ etc)
– Robinicks
Apr 30 '09 at 16:41
Is this event reliable? (Cross browser.. IE6+ FF2+ etc)
– Robinicks
Apr 30 '09 at 16:41
1
1
Yes this is cross-browser, standard DOM.
– Daniel A. White
Apr 30 '09 at 16:43
Yes this is cross-browser, standard DOM.
– Daniel A. White
Apr 30 '09 at 16:43
13
13
It's actually window.onload that's more standard, not document.onload. AFAIK
– Peter Bailey
Apr 30 '09 at 16:48
It's actually window.onload that's more standard, not document.onload. AFAIK
– Peter Bailey
Apr 30 '09 at 16:48
1
1
Thanks for your correction.
– Daniel A. White
Apr 30 '09 at 16:51
Thanks for your correction.
– Daniel A. White
Apr 30 '09 at 16:51
add a comment |
Best method, recommended by Google also. :)
<script type="text/javascript">
function downloadJSAtOnload() {
var element = document.createElement("script");
element.src = "defer.js";
document.body.appendChild(element);
}
if (window.addEventListener)
window.addEventListener("load", downloadJSAtOnload, false);
else if (window.attachEvent)
window.attachEvent("onload", downloadJSAtOnload);
else window.onload = downloadJSAtOnload;
</script>
http://www.feedthebot.com/pagespeed/defer-loading-javascript.html
load script in last order. just what i need!!! good work with asynh js
– Vladimir Ch
Jan 17 '17 at 10:46
add a comment |
Best method, recommended by Google also. :)
<script type="text/javascript">
function downloadJSAtOnload() {
var element = document.createElement("script");
element.src = "defer.js";
document.body.appendChild(element);
}
if (window.addEventListener)
window.addEventListener("load", downloadJSAtOnload, false);
else if (window.attachEvent)
window.attachEvent("onload", downloadJSAtOnload);
else window.onload = downloadJSAtOnload;
</script>
http://www.feedthebot.com/pagespeed/defer-loading-javascript.html
load script in last order. just what i need!!! good work with asynh js
– Vladimir Ch
Jan 17 '17 at 10:46
add a comment |
Best method, recommended by Google also. :)
<script type="text/javascript">
function downloadJSAtOnload() {
var element = document.createElement("script");
element.src = "defer.js";
document.body.appendChild(element);
}
if (window.addEventListener)
window.addEventListener("load", downloadJSAtOnload, false);
else if (window.attachEvent)
window.attachEvent("onload", downloadJSAtOnload);
else window.onload = downloadJSAtOnload;
</script>
http://www.feedthebot.com/pagespeed/defer-loading-javascript.html
Best method, recommended by Google also. :)
<script type="text/javascript">
function downloadJSAtOnload() {
var element = document.createElement("script");
element.src = "defer.js";
document.body.appendChild(element);
}
if (window.addEventListener)
window.addEventListener("load", downloadJSAtOnload, false);
else if (window.attachEvent)
window.attachEvent("onload", downloadJSAtOnload);
else window.onload = downloadJSAtOnload;
</script>
http://www.feedthebot.com/pagespeed/defer-loading-javascript.html
answered Sep 11 '14 at 19:23
FrankeyFrankey
3,0982022
3,0982022
load script in last order. just what i need!!! good work with asynh js
– Vladimir Ch
Jan 17 '17 at 10:46
add a comment |
load script in last order. just what i need!!! good work with asynh js
– Vladimir Ch
Jan 17 '17 at 10:46
load script in last order. just what i need!!! good work with asynh js
– Vladimir Ch
Jan 17 '17 at 10:46
load script in last order. just what i need!!! good work with asynh js
– Vladimir Ch
Jan 17 '17 at 10:46
add a comment |
Working Fiddle
<!DOCTYPE html>
<html>
<head>
<script>
function myFunction()
{
alert("Page is loaded");
}
</script>
</head>
<body onload="myFunction()">
<h1>Hello World!</h1>
</body>
</html>
add a comment |
Working Fiddle
<!DOCTYPE html>
<html>
<head>
<script>
function myFunction()
{
alert("Page is loaded");
}
</script>
</head>
<body onload="myFunction()">
<h1>Hello World!</h1>
</body>
</html>
add a comment |
Working Fiddle
<!DOCTYPE html>
<html>
<head>
<script>
function myFunction()
{
alert("Page is loaded");
}
</script>
</head>
<body onload="myFunction()">
<h1>Hello World!</h1>
</body>
</html>
Working Fiddle
<!DOCTYPE html>
<html>
<head>
<script>
function myFunction()
{
alert("Page is loaded");
}
</script>
</head>
<body onload="myFunction()">
<h1>Hello World!</h1>
</body>
</html>
edited Jan 8 '15 at 13:23
answered Mar 26 '13 at 9:39
Aamir ShahzadAamir Shahzad
4,51063953
4,51063953
add a comment |
add a comment |
If you are using jQuery,
$(function() {...});
is equivalent to
$(document).ready(function () { })
See What event does JQuery $function() fire on?
add a comment |
If you are using jQuery,
$(function() {...});
is equivalent to
$(document).ready(function () { })
See What event does JQuery $function() fire on?
add a comment |
If you are using jQuery,
$(function() {...});
is equivalent to
$(document).ready(function () { })
See What event does JQuery $function() fire on?
If you are using jQuery,
$(function() {...});
is equivalent to
$(document).ready(function () { })
See What event does JQuery $function() fire on?
edited May 23 '17 at 11:47
Community♦
11
11
answered Mar 6 '13 at 20:39


Benjamin CrouzierBenjamin Crouzier
23.8k31125188
23.8k31125188
add a comment |
add a comment |
document.onreadystatechange = function(){
if(document.readyState === 'complete'){
/*code here*/
}
}
look here: http://msdn.microsoft.com/en-us/library/ie/ms536957(v=vs.85).aspx
2
one-line: document.onload = function() { ... }
– colminator
Mar 26 '15 at 17:31
best solution in my opinion. Good for Jquery not defined issue
– S.M. Nat
Dec 12 '18 at 7:20
add a comment |
document.onreadystatechange = function(){
if(document.readyState === 'complete'){
/*code here*/
}
}
look here: http://msdn.microsoft.com/en-us/library/ie/ms536957(v=vs.85).aspx
2
one-line: document.onload = function() { ... }
– colminator
Mar 26 '15 at 17:31
best solution in my opinion. Good for Jquery not defined issue
– S.M. Nat
Dec 12 '18 at 7:20
add a comment |
document.onreadystatechange = function(){
if(document.readyState === 'complete'){
/*code here*/
}
}
look here: http://msdn.microsoft.com/en-us/library/ie/ms536957(v=vs.85).aspx
document.onreadystatechange = function(){
if(document.readyState === 'complete'){
/*code here*/
}
}
look here: http://msdn.microsoft.com/en-us/library/ie/ms536957(v=vs.85).aspx
answered Sep 11 '14 at 11:41


ArthurArthur
3,91321214
3,91321214
2
one-line: document.onload = function() { ... }
– colminator
Mar 26 '15 at 17:31
best solution in my opinion. Good for Jquery not defined issue
– S.M. Nat
Dec 12 '18 at 7:20
add a comment |
2
one-line: document.onload = function() { ... }
– colminator
Mar 26 '15 at 17:31
best solution in my opinion. Good for Jquery not defined issue
– S.M. Nat
Dec 12 '18 at 7:20
2
2
one-line: document.onload = function() { ... }
– colminator
Mar 26 '15 at 17:31
one-line: document.onload = function() { ... }
– colminator
Mar 26 '15 at 17:31
best solution in my opinion. Good for Jquery not defined issue
– S.M. Nat
Dec 12 '18 at 7:20
best solution in my opinion. Good for Jquery not defined issue
– S.M. Nat
Dec 12 '18 at 7:20
add a comment |
<body onload="myFunction()">
This code works well.
But window.onload
method has various dependencies. So it may not work all the time.
add a comment |
<body onload="myFunction()">
This code works well.
But window.onload
method has various dependencies. So it may not work all the time.
add a comment |
<body onload="myFunction()">
This code works well.
But window.onload
method has various dependencies. So it may not work all the time.
<body onload="myFunction()">
This code works well.
But window.onload
method has various dependencies. So it may not work all the time.
edited Apr 29 '13 at 11:58
Hakan KOSE
661915
661915
answered Apr 29 '13 at 11:32
Akarsh SatijaAkarsh Satija
1,2861828
1,2861828
add a comment |
add a comment |
I find sometimes on more complex pages that not all the elements have loaded by the time window.onload is fired. If that's the case, add setTimeout before your function to delay is a moment. It's not elegant but it's a simple hack that renders well.
window.onload = function(){ doSomethingCool(); };
becomes...
window.onload = function(){ setTimeout( function(){ doSomethingCool(); }, 1000); };
add a comment |
I find sometimes on more complex pages that not all the elements have loaded by the time window.onload is fired. If that's the case, add setTimeout before your function to delay is a moment. It's not elegant but it's a simple hack that renders well.
window.onload = function(){ doSomethingCool(); };
becomes...
window.onload = function(){ setTimeout( function(){ doSomethingCool(); }, 1000); };
add a comment |
I find sometimes on more complex pages that not all the elements have loaded by the time window.onload is fired. If that's the case, add setTimeout before your function to delay is a moment. It's not elegant but it's a simple hack that renders well.
window.onload = function(){ doSomethingCool(); };
becomes...
window.onload = function(){ setTimeout( function(){ doSomethingCool(); }, 1000); };
I find sometimes on more complex pages that not all the elements have loaded by the time window.onload is fired. If that's the case, add setTimeout before your function to delay is a moment. It's not elegant but it's a simple hack that renders well.
window.onload = function(){ doSomethingCool(); };
becomes...
window.onload = function(){ setTimeout( function(){ doSomethingCool(); }, 1000); };
edited Jun 11 '15 at 10:45


ekostadinov
5,72121941
5,72121941
answered Jul 20 '13 at 21:13


Charles JaimetCharles Jaimet
47649
47649
add a comment |
add a comment |
Just define <body onload="aFunction()">
that will be called after the page has been loaded. Your code in the script is than enclosed by aFunction() { }
.
I was too hasty and forgot to use &lt; instead of <. Sorry, but it all went soo fast
– Norbert Hartl
Apr 30 '09 at 16:46
Well is it different in comments? <bla>
– Norbert Hartl
Apr 30 '09 at 16:47
add a comment |
Just define <body onload="aFunction()">
that will be called after the page has been loaded. Your code in the script is than enclosed by aFunction() { }
.
I was too hasty and forgot to use &lt; instead of <. Sorry, but it all went soo fast
– Norbert Hartl
Apr 30 '09 at 16:46
Well is it different in comments? <bla>
– Norbert Hartl
Apr 30 '09 at 16:47
add a comment |
Just define <body onload="aFunction()">
that will be called after the page has been loaded. Your code in the script is than enclosed by aFunction() { }
.
Just define <body onload="aFunction()">
that will be called after the page has been loaded. Your code in the script is than enclosed by aFunction() { }
.
edited Nov 8 '16 at 2:40
fragilewindows
1,1981921
1,1981921
answered Apr 30 '09 at 16:41
Norbert HartlNorbert Hartl
7,30042644
7,30042644
I was too hasty and forgot to use &lt; instead of <. Sorry, but it all went soo fast
– Norbert Hartl
Apr 30 '09 at 16:46
Well is it different in comments? <bla>
– Norbert Hartl
Apr 30 '09 at 16:47
add a comment |
I was too hasty and forgot to use &lt; instead of <. Sorry, but it all went soo fast
– Norbert Hartl
Apr 30 '09 at 16:46
Well is it different in comments? <bla>
– Norbert Hartl
Apr 30 '09 at 16:47
I was too hasty and forgot to use &lt; instead of <. Sorry, but it all went soo fast
– Norbert Hartl
Apr 30 '09 at 16:46
I was too hasty and forgot to use &lt; instead of <. Sorry, but it all went soo fast
– Norbert Hartl
Apr 30 '09 at 16:46
Well is it different in comments? <bla>
– Norbert Hartl
Apr 30 '09 at 16:47
Well is it different in comments? <bla>
– Norbert Hartl
Apr 30 '09 at 16:47
add a comment |
$(window).on("load", function(){ ... });
.ready() works best for me.
$(document).ready(function(){ ... });
.load() will work, but it won't wait till the page is loaded.
jQuery(window).load(function () { ... });
Doesn't work for me, breaks the next-to inline script. I am also using jQuery 3.2.1 along with some other jQuery forks.
To hide my websites loading overlay, I use the following:
<script>
$(window).on("load", function(){
$('.loading-page').delay(3000).fadeOut(250);
});
</script>
3
you write a answer 9 year later but with jQuery :P
– Anirudha Gupta
Oct 13 '18 at 11:51
add a comment |
$(window).on("load", function(){ ... });
.ready() works best for me.
$(document).ready(function(){ ... });
.load() will work, but it won't wait till the page is loaded.
jQuery(window).load(function () { ... });
Doesn't work for me, breaks the next-to inline script. I am also using jQuery 3.2.1 along with some other jQuery forks.
To hide my websites loading overlay, I use the following:
<script>
$(window).on("load", function(){
$('.loading-page').delay(3000).fadeOut(250);
});
</script>
3
you write a answer 9 year later but with jQuery :P
– Anirudha Gupta
Oct 13 '18 at 11:51
add a comment |
$(window).on("load", function(){ ... });
.ready() works best for me.
$(document).ready(function(){ ... });
.load() will work, but it won't wait till the page is loaded.
jQuery(window).load(function () { ... });
Doesn't work for me, breaks the next-to inline script. I am also using jQuery 3.2.1 along with some other jQuery forks.
To hide my websites loading overlay, I use the following:
<script>
$(window).on("load", function(){
$('.loading-page').delay(3000).fadeOut(250);
});
</script>
$(window).on("load", function(){ ... });
.ready() works best for me.
$(document).ready(function(){ ... });
.load() will work, but it won't wait till the page is loaded.
jQuery(window).load(function () { ... });
Doesn't work for me, breaks the next-to inline script. I am also using jQuery 3.2.1 along with some other jQuery forks.
To hide my websites loading overlay, I use the following:
<script>
$(window).on("load", function(){
$('.loading-page').delay(3000).fadeOut(250);
});
</script>
edited Nov 16 '18 at 16:51


eddy white
188120
188120
answered Mar 8 '18 at 2:42


Zach ReynoldsZach Reynolds
689
689
3
you write a answer 9 year later but with jQuery :P
– Anirudha Gupta
Oct 13 '18 at 11:51
add a comment |
3
you write a answer 9 year later but with jQuery :P
– Anirudha Gupta
Oct 13 '18 at 11:51
3
3
you write a answer 9 year later but with jQuery :P
– Anirudha Gupta
Oct 13 '18 at 11:51
you write a answer 9 year later but with jQuery :P
– Anirudha Gupta
Oct 13 '18 at 11:51
add a comment |
Using the YUI library (I love it):
YAHOO.util.Event.onDOMReady(function(){
//your code
});
Portable and beautiful! However, if you don't use YUI for other stuff (see its doc) I would say that it's not worth to use it.
N.B. : to use this code you need to import 2 scripts
<script type="text/javascript" src="http://yui.yahooapis.com/2.7.0/build/yahoo/yahoo-min.js" ></script>
<script type="text/javascript" src="http://yui.yahooapis.com/2.7.0/build/event/event-min.js" ></script>
add a comment |
Using the YUI library (I love it):
YAHOO.util.Event.onDOMReady(function(){
//your code
});
Portable and beautiful! However, if you don't use YUI for other stuff (see its doc) I would say that it's not worth to use it.
N.B. : to use this code you need to import 2 scripts
<script type="text/javascript" src="http://yui.yahooapis.com/2.7.0/build/yahoo/yahoo-min.js" ></script>
<script type="text/javascript" src="http://yui.yahooapis.com/2.7.0/build/event/event-min.js" ></script>
add a comment |
Using the YUI library (I love it):
YAHOO.util.Event.onDOMReady(function(){
//your code
});
Portable and beautiful! However, if you don't use YUI for other stuff (see its doc) I would say that it's not worth to use it.
N.B. : to use this code you need to import 2 scripts
<script type="text/javascript" src="http://yui.yahooapis.com/2.7.0/build/yahoo/yahoo-min.js" ></script>
<script type="text/javascript" src="http://yui.yahooapis.com/2.7.0/build/event/event-min.js" ></script>
Using the YUI library (I love it):
YAHOO.util.Event.onDOMReady(function(){
//your code
});
Portable and beautiful! However, if you don't use YUI for other stuff (see its doc) I would say that it's not worth to use it.
N.B. : to use this code you need to import 2 scripts
<script type="text/javascript" src="http://yui.yahooapis.com/2.7.0/build/yahoo/yahoo-min.js" ></script>
<script type="text/javascript" src="http://yui.yahooapis.com/2.7.0/build/event/event-min.js" ></script>
answered Apr 30 '09 at 17:36
Valentin JacqueminValentin Jacquemin
1,55511532
1,55511532
add a comment |
add a comment |
There is a very good documentation on How to detect if document has loaded using Javascript or Jquery.
Using the native Javascript this can be achieved
if (document.readyState === "complete") {
init();
}
This can also be done inside the interval
var interval = setInterval(function() {
if(document.readyState === 'complete') {
clearInterval(interval);
init();
}
}, 100);
Eg By Mozilla
switch (document.readyState) {
case "loading":
// The document is still loading.
break;
case "interactive":
// The document has finished loading. We can now access the DOM elements.
var span = document.createElement("span");
span.textContent = "A <span> element.";
document.body.appendChild(span);
break;
case "complete":
// The page is fully loaded.
console.log("Page is loaded completely");
break;
}
Using Jquery
To check only if DOM is ready
// A $( document ).ready() block.
$( document ).ready(function() {
console.log( "ready!" );
});
To check if all resources are loaded use window.load
$( window ).load(function() {
console.log( "window loaded" );
});
add a comment |
There is a very good documentation on How to detect if document has loaded using Javascript or Jquery.
Using the native Javascript this can be achieved
if (document.readyState === "complete") {
init();
}
This can also be done inside the interval
var interval = setInterval(function() {
if(document.readyState === 'complete') {
clearInterval(interval);
init();
}
}, 100);
Eg By Mozilla
switch (document.readyState) {
case "loading":
// The document is still loading.
break;
case "interactive":
// The document has finished loading. We can now access the DOM elements.
var span = document.createElement("span");
span.textContent = "A <span> element.";
document.body.appendChild(span);
break;
case "complete":
// The page is fully loaded.
console.log("Page is loaded completely");
break;
}
Using Jquery
To check only if DOM is ready
// A $( document ).ready() block.
$( document ).ready(function() {
console.log( "ready!" );
});
To check if all resources are loaded use window.load
$( window ).load(function() {
console.log( "window loaded" );
});
add a comment |
There is a very good documentation on How to detect if document has loaded using Javascript or Jquery.
Using the native Javascript this can be achieved
if (document.readyState === "complete") {
init();
}
This can also be done inside the interval
var interval = setInterval(function() {
if(document.readyState === 'complete') {
clearInterval(interval);
init();
}
}, 100);
Eg By Mozilla
switch (document.readyState) {
case "loading":
// The document is still loading.
break;
case "interactive":
// The document has finished loading. We can now access the DOM elements.
var span = document.createElement("span");
span.textContent = "A <span> element.";
document.body.appendChild(span);
break;
case "complete":
// The page is fully loaded.
console.log("Page is loaded completely");
break;
}
Using Jquery
To check only if DOM is ready
// A $( document ).ready() block.
$( document ).ready(function() {
console.log( "ready!" );
});
To check if all resources are loaded use window.load
$( window ).load(function() {
console.log( "window loaded" );
});
There is a very good documentation on How to detect if document has loaded using Javascript or Jquery.
Using the native Javascript this can be achieved
if (document.readyState === "complete") {
init();
}
This can also be done inside the interval
var interval = setInterval(function() {
if(document.readyState === 'complete') {
clearInterval(interval);
init();
}
}, 100);
Eg By Mozilla
switch (document.readyState) {
case "loading":
// The document is still loading.
break;
case "interactive":
// The document has finished loading. We can now access the DOM elements.
var span = document.createElement("span");
span.textContent = "A <span> element.";
document.body.appendChild(span);
break;
case "complete":
// The page is fully loaded.
console.log("Page is loaded completely");
break;
}
Using Jquery
To check only if DOM is ready
// A $( document ).ready() block.
$( document ).ready(function() {
console.log( "ready!" );
});
To check if all resources are loaded use window.load
$( window ).load(function() {
console.log( "window loaded" );
});
answered Dec 12 '15 at 8:42
user5642061
add a comment |
add a comment |
Use this code with jQuery library, this would work perfectly fine.
$(window).bind("load", function() {
// your javascript event
});
Add some description here
– Billa
Dec 21 '17 at 13:50
add a comment |
Use this code with jQuery library, this would work perfectly fine.
$(window).bind("load", function() {
// your javascript event
});
Add some description here
– Billa
Dec 21 '17 at 13:50
add a comment |
Use this code with jQuery library, this would work perfectly fine.
$(window).bind("load", function() {
// your javascript event
});
Use this code with jQuery library, this would work perfectly fine.
$(window).bind("load", function() {
// your javascript event
});
edited Dec 25 '17 at 19:53
answered Dec 21 '17 at 13:32
Harish KumarHarish Kumar
5419
5419
Add some description here
– Billa
Dec 21 '17 at 13:50
add a comment |
Add some description here
– Billa
Dec 21 '17 at 13:50
Add some description here
– Billa
Dec 21 '17 at 13:50
Add some description here
– Billa
Dec 21 '17 at 13:50
add a comment |
As Daniel says, you could use document.onload.
The various javascript frameworks hwoever (jQuery, Mootools, etc.) use a custom event 'domready', which I guess must be more effective. If you're developing with javascript, I'd highly recommend exploiting a framework, they massively increase your productivity.
Pity browsers didn't do what frameworks do.
– Robinicks
Apr 30 '09 at 16:54
add a comment |
As Daniel says, you could use document.onload.
The various javascript frameworks hwoever (jQuery, Mootools, etc.) use a custom event 'domready', which I guess must be more effective. If you're developing with javascript, I'd highly recommend exploiting a framework, they massively increase your productivity.
Pity browsers didn't do what frameworks do.
– Robinicks
Apr 30 '09 at 16:54
add a comment |
As Daniel says, you could use document.onload.
The various javascript frameworks hwoever (jQuery, Mootools, etc.) use a custom event 'domready', which I guess must be more effective. If you're developing with javascript, I'd highly recommend exploiting a framework, they massively increase your productivity.
As Daniel says, you could use document.onload.
The various javascript frameworks hwoever (jQuery, Mootools, etc.) use a custom event 'domready', which I guess must be more effective. If you're developing with javascript, I'd highly recommend exploiting a framework, they massively increase your productivity.
answered Apr 30 '09 at 16:47
IainIain
3001310
3001310
Pity browsers didn't do what frameworks do.
– Robinicks
Apr 30 '09 at 16:54
add a comment |
Pity browsers didn't do what frameworks do.
– Robinicks
Apr 30 '09 at 16:54
Pity browsers didn't do what frameworks do.
– Robinicks
Apr 30 '09 at 16:54
Pity browsers didn't do what frameworks do.
– Robinicks
Apr 30 '09 at 16:54
add a comment |
My advise use asnyc
attribute for script tag thats help you to load the external scripts after page load
<script type="text/javascript" src="a.js" async></script>
<script type="text/javascript" src="b.js" async></script>
5
According to w3schools, If async is present: The script is executed asynchronously with the rest of the page (the script will be executed while the page continues the parsing). Perhaps you meantdefer
instead, like @Daniel Price mentioned?
– jk7
Apr 15 '15 at 0:06
add a comment |
My advise use asnyc
attribute for script tag thats help you to load the external scripts after page load
<script type="text/javascript" src="a.js" async></script>
<script type="text/javascript" src="b.js" async></script>
5
According to w3schools, If async is present: The script is executed asynchronously with the rest of the page (the script will be executed while the page continues the parsing). Perhaps you meantdefer
instead, like @Daniel Price mentioned?
– jk7
Apr 15 '15 at 0:06
add a comment |
My advise use asnyc
attribute for script tag thats help you to load the external scripts after page load
<script type="text/javascript" src="a.js" async></script>
<script type="text/javascript" src="b.js" async></script>
My advise use asnyc
attribute for script tag thats help you to load the external scripts after page load
<script type="text/javascript" src="a.js" async></script>
<script type="text/javascript" src="b.js" async></script>
answered Mar 30 '15 at 5:43


KalidassKalidass
181115
181115
5
According to w3schools, If async is present: The script is executed asynchronously with the rest of the page (the script will be executed while the page continues the parsing). Perhaps you meantdefer
instead, like @Daniel Price mentioned?
– jk7
Apr 15 '15 at 0:06
add a comment |
5
According to w3schools, If async is present: The script is executed asynchronously with the rest of the page (the script will be executed while the page continues the parsing). Perhaps you meantdefer
instead, like @Daniel Price mentioned?
– jk7
Apr 15 '15 at 0:06
5
5
According to w3schools, If async is present: The script is executed asynchronously with the rest of the page (the script will be executed while the page continues the parsing). Perhaps you meant
defer
instead, like @Daniel Price mentioned?– jk7
Apr 15 '15 at 0:06
According to w3schools, If async is present: The script is executed asynchronously with the rest of the page (the script will be executed while the page continues the parsing). Perhaps you meant
defer
instead, like @Daniel Price mentioned?– jk7
Apr 15 '15 at 0:06
add a comment |
<script type="text/javascript">
$(window).bind("load", function() {
// your javascript event here
});
</script>
1
This answer depends on jquery
– Chiptus
Sep 5 '18 at 11:38
add a comment |
<script type="text/javascript">
$(window).bind("load", function() {
// your javascript event here
});
</script>
1
This answer depends on jquery
– Chiptus
Sep 5 '18 at 11:38
add a comment |
<script type="text/javascript">
$(window).bind("load", function() {
// your javascript event here
});
</script>
<script type="text/javascript">
$(window).bind("load", function() {
// your javascript event here
});
</script>
<script type="text/javascript">
$(window).bind("load", function() {
// your javascript event here
});
</script>
<script type="text/javascript">
$(window).bind("load", function() {
// your javascript event here
});
</script>
edited Jun 8 '18 at 14:02


Jared Lovin
536923
536923
answered Sep 29 '15 at 8:43
Vô VịVô Vị
15712
15712
1
This answer depends on jquery
– Chiptus
Sep 5 '18 at 11:38
add a comment |
1
This answer depends on jquery
– Chiptus
Sep 5 '18 at 11:38
1
1
This answer depends on jquery
– Chiptus
Sep 5 '18 at 11:38
This answer depends on jquery
– Chiptus
Sep 5 '18 at 11:38
add a comment |
use self execution onload function
window.onload = function (){
/* statements */
}();
You may override otheronload
handlers by using this approach. Instead, you should add listener.
– Leonid Dashko
May 29 '18 at 18:45
add a comment |
use self execution onload function
window.onload = function (){
/* statements */
}();
You may override otheronload
handlers by using this approach. Instead, you should add listener.
– Leonid Dashko
May 29 '18 at 18:45
add a comment |
use self execution onload function
window.onload = function (){
/* statements */
}();
use self execution onload function
window.onload = function (){
/* statements */
}();
answered Feb 19 '18 at 15:59
user7087840user7087840
191
191
You may override otheronload
handlers by using this approach. Instead, you should add listener.
– Leonid Dashko
May 29 '18 at 18:45
add a comment |
You may override otheronload
handlers by using this approach. Instead, you should add listener.
– Leonid Dashko
May 29 '18 at 18:45
You may override other
onload
handlers by using this approach. Instead, you should add listener.– Leonid Dashko
May 29 '18 at 18:45
You may override other
onload
handlers by using this approach. Instead, you should add listener.– Leonid Dashko
May 29 '18 at 18:45
add a comment |
protected by K. Sopheak Dec 20 '18 at 6:49
Thank you for your interest in this question.
Because it has attracted low-quality or spam answers that had to be removed, posting an answer now requires 10 reputation on this site (the association bonus does not count).
Would you like to answer one of these unanswered questions instead?
pLkDHKKktAiYbMpgVZLGxRKCAoN3nG61UWhoQyME3QZRfv,FSeA,ekwRt7gGPPfN4wGR,9Latlxae6,El3Sdj7