.NET get full property list for AD Computers (to match Powershell Get-ADComputer API)
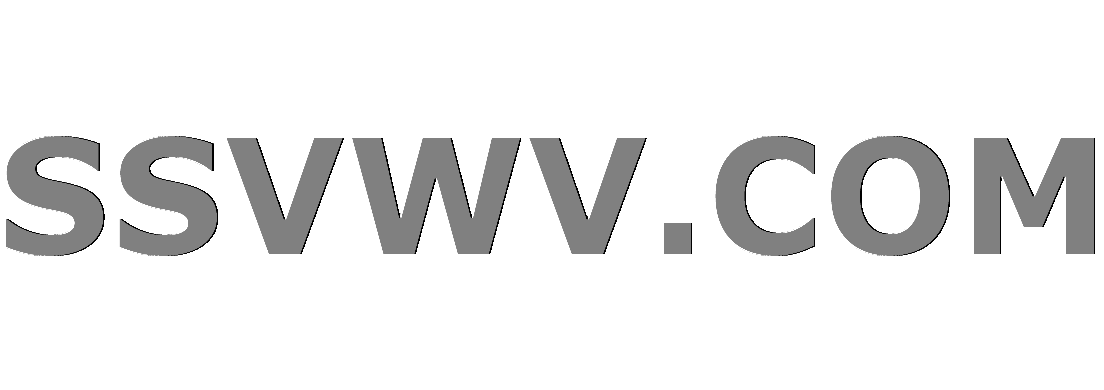
Multi tool use
up vote
1
down vote
favorite
I'm converting Powershell code to C# and in Powershell I call this API "Get-ADComputer" https://docs.microsoft.com/en-us/powershell/module/activedirectory/get-adcomputer?view=winserver2012-ps. When you look at the examples in the link, it shows an exhaustive list of properties that can be returned.
I'm trying to replicate this in C#, but I get a subset of what the Powershell call returns. For instance, I'm looking for "PrimaryGroup", which is only coming back in the Powershell call.
Here are the code snippets:
POWERSHELL
Get-ADComputer -Filter "*" -Properties "*"
C#
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.DirectoryServices;
using System.DirectoryServices.ActiveDirectory;
using System.IO;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Test
{
class Program
{
static void Main(string args)
{
foreach (var p in GetProperties())
Console.WriteLine(p);
}
private static IEnumerable<string> GetDomainNames()
{
ICollection<string> domains = new List<string>();
foreach (Domain domain in Forest.GetCurrentForest().Domains)
domains.Add(domain.Name);
return domains;
}
public static List<string> GetProperties()
{
List<string> properties = new List<string>();
foreach (var domainName in GetDomainNames())
{
using (DirectoryEntry directoryEntry = new DirectoryEntry(@"LDAP://" + domainName))
{
using (DirectorySearcher mySearcher = new DirectorySearcher(directoryEntry))
{
mySearcher.Filter = ("(objectClass=computer)");
mySearcher.SizeLimit = 0; // no size limit
mySearcher.PageSize = 250; // paging
mySearcher.PropertiesToLoad.Add("PrimaryGroup"); // only want this property, for testing, comment out this line to get all properties returned
foreach (SearchResult resEnt in mySearcher.FindAll())
{
foreach (var p in resEnt.Properties.PropertyNames)
properties.Add(p.ToString());
}
}
}
}
properties.Sort();
return properties;
}
}
}
I'm guessing I just haven't quite setup everything correctly in the C# code. I get many of the same properties back, but not all. I'm hoping for guidance.
thanks.
c# .net powershell active-directory
add a comment |
up vote
1
down vote
favorite
I'm converting Powershell code to C# and in Powershell I call this API "Get-ADComputer" https://docs.microsoft.com/en-us/powershell/module/activedirectory/get-adcomputer?view=winserver2012-ps. When you look at the examples in the link, it shows an exhaustive list of properties that can be returned.
I'm trying to replicate this in C#, but I get a subset of what the Powershell call returns. For instance, I'm looking for "PrimaryGroup", which is only coming back in the Powershell call.
Here are the code snippets:
POWERSHELL
Get-ADComputer -Filter "*" -Properties "*"
C#
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.DirectoryServices;
using System.DirectoryServices.ActiveDirectory;
using System.IO;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Test
{
class Program
{
static void Main(string args)
{
foreach (var p in GetProperties())
Console.WriteLine(p);
}
private static IEnumerable<string> GetDomainNames()
{
ICollection<string> domains = new List<string>();
foreach (Domain domain in Forest.GetCurrentForest().Domains)
domains.Add(domain.Name);
return domains;
}
public static List<string> GetProperties()
{
List<string> properties = new List<string>();
foreach (var domainName in GetDomainNames())
{
using (DirectoryEntry directoryEntry = new DirectoryEntry(@"LDAP://" + domainName))
{
using (DirectorySearcher mySearcher = new DirectorySearcher(directoryEntry))
{
mySearcher.Filter = ("(objectClass=computer)");
mySearcher.SizeLimit = 0; // no size limit
mySearcher.PageSize = 250; // paging
mySearcher.PropertiesToLoad.Add("PrimaryGroup"); // only want this property, for testing, comment out this line to get all properties returned
foreach (SearchResult resEnt in mySearcher.FindAll())
{
foreach (var p in resEnt.Properties.PropertyNames)
properties.Add(p.ToString());
}
}
}
}
properties.Sort();
return properties;
}
}
}
I'm guessing I just haven't quite setup everything correctly in the C# code. I get many of the same properties back, but not all. I'm hoping for guidance.
thanks.
c# .net powershell active-directory
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I'm converting Powershell code to C# and in Powershell I call this API "Get-ADComputer" https://docs.microsoft.com/en-us/powershell/module/activedirectory/get-adcomputer?view=winserver2012-ps. When you look at the examples in the link, it shows an exhaustive list of properties that can be returned.
I'm trying to replicate this in C#, but I get a subset of what the Powershell call returns. For instance, I'm looking for "PrimaryGroup", which is only coming back in the Powershell call.
Here are the code snippets:
POWERSHELL
Get-ADComputer -Filter "*" -Properties "*"
C#
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.DirectoryServices;
using System.DirectoryServices.ActiveDirectory;
using System.IO;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Test
{
class Program
{
static void Main(string args)
{
foreach (var p in GetProperties())
Console.WriteLine(p);
}
private static IEnumerable<string> GetDomainNames()
{
ICollection<string> domains = new List<string>();
foreach (Domain domain in Forest.GetCurrentForest().Domains)
domains.Add(domain.Name);
return domains;
}
public static List<string> GetProperties()
{
List<string> properties = new List<string>();
foreach (var domainName in GetDomainNames())
{
using (DirectoryEntry directoryEntry = new DirectoryEntry(@"LDAP://" + domainName))
{
using (DirectorySearcher mySearcher = new DirectorySearcher(directoryEntry))
{
mySearcher.Filter = ("(objectClass=computer)");
mySearcher.SizeLimit = 0; // no size limit
mySearcher.PageSize = 250; // paging
mySearcher.PropertiesToLoad.Add("PrimaryGroup"); // only want this property, for testing, comment out this line to get all properties returned
foreach (SearchResult resEnt in mySearcher.FindAll())
{
foreach (var p in resEnt.Properties.PropertyNames)
properties.Add(p.ToString());
}
}
}
}
properties.Sort();
return properties;
}
}
}
I'm guessing I just haven't quite setup everything correctly in the C# code. I get many of the same properties back, but not all. I'm hoping for guidance.
thanks.
c# .net powershell active-directory
I'm converting Powershell code to C# and in Powershell I call this API "Get-ADComputer" https://docs.microsoft.com/en-us/powershell/module/activedirectory/get-adcomputer?view=winserver2012-ps. When you look at the examples in the link, it shows an exhaustive list of properties that can be returned.
I'm trying to replicate this in C#, but I get a subset of what the Powershell call returns. For instance, I'm looking for "PrimaryGroup", which is only coming back in the Powershell call.
Here are the code snippets:
POWERSHELL
Get-ADComputer -Filter "*" -Properties "*"
C#
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.DirectoryServices;
using System.DirectoryServices.ActiveDirectory;
using System.IO;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Test
{
class Program
{
static void Main(string args)
{
foreach (var p in GetProperties())
Console.WriteLine(p);
}
private static IEnumerable<string> GetDomainNames()
{
ICollection<string> domains = new List<string>();
foreach (Domain domain in Forest.GetCurrentForest().Domains)
domains.Add(domain.Name);
return domains;
}
public static List<string> GetProperties()
{
List<string> properties = new List<string>();
foreach (var domainName in GetDomainNames())
{
using (DirectoryEntry directoryEntry = new DirectoryEntry(@"LDAP://" + domainName))
{
using (DirectorySearcher mySearcher = new DirectorySearcher(directoryEntry))
{
mySearcher.Filter = ("(objectClass=computer)");
mySearcher.SizeLimit = 0; // no size limit
mySearcher.PageSize = 250; // paging
mySearcher.PropertiesToLoad.Add("PrimaryGroup"); // only want this property, for testing, comment out this line to get all properties returned
foreach (SearchResult resEnt in mySearcher.FindAll())
{
foreach (var p in resEnt.Properties.PropertyNames)
properties.Add(p.ToString());
}
}
}
}
properties.Sort();
return properties;
}
}
}
I'm guessing I just haven't quite setup everything correctly in the C# code. I get many of the same properties back, but not all. I'm hoping for guidance.
thanks.
c# .net powershell active-directory
c# .net powershell active-directory
edited Nov 20 at 15:02
asked Nov 20 at 14:50
GreekFire
119210
119210
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
2
down vote
accepted
PowerShell sometimes uses "display names" for the attributes, where the actual name of the attributes in AD are slightly different. PowerShell also has some added properties that translates some of the data in AD to something useful. This is an example of that.
The PrimaryGroup
property returns the distinguished name of the primary group. However, there is no attribute in AD that stores that. The primary group is determined from the primaryGroupId
attribute, which is the RID (Relative Identifier) of the group.
So you have to ask for the primaryGroupId
attribute and translate that to find the actual group. I wrote an article about Finding all of a user’s groups where I shared a method that will do that. This accepts a DirectoryEntry
, but it really just needs to know the primaryGroupId
and the objectSid
(since it uses the user's SID to construct the SID of the group):
private static string GetUserPrimaryGroup(DirectoryEntry de) {
de.RefreshCache(new {"primaryGroupID", "objectSid"});
//Get the user's SID as a string
var sid = new SecurityIdentifier((byte)de.Properties["objectSid"].Value, 0).ToString();
//Replace the RID portion of the user's SID with the primaryGroupId
//so we're left with the group's SID
sid = sid.Remove(sid.LastIndexOf("-", StringComparison.Ordinal) + 1);
sid = sid + de.Properties["primaryGroupId"].Value;
//Find the group by its SID
var group = new DirectoryEntry($"LDAP://<SID={sid}>");
group.RefreshCache(new {"cn"});
return group.Properties["cn"].Value as string;
}
You should be able to adapt that to pull the values from the DirectorySearcher
.
As a side note, if you don't touch the PropertiesToLoad
collection, it will return every attribute with a value (excluding constructed attributes). That said, it is good practice to use the PropertiesToLoad
if you don't legitimately need to see every attribute.
Thanks for the response Gabriel. However, in the return values for the Powershell call I see 'PrimaryGroup' and 'primaryGroupID', both with different values. The C# code will return 'primaryGroupID' if I comment out 'mySearcher.PropertiesToLoad.Add("PrimaryGrou")', but not 'PrimaryGroup'
– GreekFire
Nov 20 at 15:02
Yes, that is expected.PrimaryGroup
doesn't actually exist in AD. It's a PowerShell property that finds the primary group based on theprimaryGroupId
attribute in AD.
– Gabriel Luci
Nov 20 at 15:08
Sorry, I re-read my answer and realized why I confused you :) I updated my answer to make it more clear what's going on, and how to get the primary group.
– Gabriel Luci
Nov 20 at 15:28
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53395613%2fnet-get-full-property-list-for-ad-computers-to-match-powershell-get-adcomputer%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
PowerShell sometimes uses "display names" for the attributes, where the actual name of the attributes in AD are slightly different. PowerShell also has some added properties that translates some of the data in AD to something useful. This is an example of that.
The PrimaryGroup
property returns the distinguished name of the primary group. However, there is no attribute in AD that stores that. The primary group is determined from the primaryGroupId
attribute, which is the RID (Relative Identifier) of the group.
So you have to ask for the primaryGroupId
attribute and translate that to find the actual group. I wrote an article about Finding all of a user’s groups where I shared a method that will do that. This accepts a DirectoryEntry
, but it really just needs to know the primaryGroupId
and the objectSid
(since it uses the user's SID to construct the SID of the group):
private static string GetUserPrimaryGroup(DirectoryEntry de) {
de.RefreshCache(new {"primaryGroupID", "objectSid"});
//Get the user's SID as a string
var sid = new SecurityIdentifier((byte)de.Properties["objectSid"].Value, 0).ToString();
//Replace the RID portion of the user's SID with the primaryGroupId
//so we're left with the group's SID
sid = sid.Remove(sid.LastIndexOf("-", StringComparison.Ordinal) + 1);
sid = sid + de.Properties["primaryGroupId"].Value;
//Find the group by its SID
var group = new DirectoryEntry($"LDAP://<SID={sid}>");
group.RefreshCache(new {"cn"});
return group.Properties["cn"].Value as string;
}
You should be able to adapt that to pull the values from the DirectorySearcher
.
As a side note, if you don't touch the PropertiesToLoad
collection, it will return every attribute with a value (excluding constructed attributes). That said, it is good practice to use the PropertiesToLoad
if you don't legitimately need to see every attribute.
Thanks for the response Gabriel. However, in the return values for the Powershell call I see 'PrimaryGroup' and 'primaryGroupID', both with different values. The C# code will return 'primaryGroupID' if I comment out 'mySearcher.PropertiesToLoad.Add("PrimaryGrou")', but not 'PrimaryGroup'
– GreekFire
Nov 20 at 15:02
Yes, that is expected.PrimaryGroup
doesn't actually exist in AD. It's a PowerShell property that finds the primary group based on theprimaryGroupId
attribute in AD.
– Gabriel Luci
Nov 20 at 15:08
Sorry, I re-read my answer and realized why I confused you :) I updated my answer to make it more clear what's going on, and how to get the primary group.
– Gabriel Luci
Nov 20 at 15:28
add a comment |
up vote
2
down vote
accepted
PowerShell sometimes uses "display names" for the attributes, where the actual name of the attributes in AD are slightly different. PowerShell also has some added properties that translates some of the data in AD to something useful. This is an example of that.
The PrimaryGroup
property returns the distinguished name of the primary group. However, there is no attribute in AD that stores that. The primary group is determined from the primaryGroupId
attribute, which is the RID (Relative Identifier) of the group.
So you have to ask for the primaryGroupId
attribute and translate that to find the actual group. I wrote an article about Finding all of a user’s groups where I shared a method that will do that. This accepts a DirectoryEntry
, but it really just needs to know the primaryGroupId
and the objectSid
(since it uses the user's SID to construct the SID of the group):
private static string GetUserPrimaryGroup(DirectoryEntry de) {
de.RefreshCache(new {"primaryGroupID", "objectSid"});
//Get the user's SID as a string
var sid = new SecurityIdentifier((byte)de.Properties["objectSid"].Value, 0).ToString();
//Replace the RID portion of the user's SID with the primaryGroupId
//so we're left with the group's SID
sid = sid.Remove(sid.LastIndexOf("-", StringComparison.Ordinal) + 1);
sid = sid + de.Properties["primaryGroupId"].Value;
//Find the group by its SID
var group = new DirectoryEntry($"LDAP://<SID={sid}>");
group.RefreshCache(new {"cn"});
return group.Properties["cn"].Value as string;
}
You should be able to adapt that to pull the values from the DirectorySearcher
.
As a side note, if you don't touch the PropertiesToLoad
collection, it will return every attribute with a value (excluding constructed attributes). That said, it is good practice to use the PropertiesToLoad
if you don't legitimately need to see every attribute.
Thanks for the response Gabriel. However, in the return values for the Powershell call I see 'PrimaryGroup' and 'primaryGroupID', both with different values. The C# code will return 'primaryGroupID' if I comment out 'mySearcher.PropertiesToLoad.Add("PrimaryGrou")', but not 'PrimaryGroup'
– GreekFire
Nov 20 at 15:02
Yes, that is expected.PrimaryGroup
doesn't actually exist in AD. It's a PowerShell property that finds the primary group based on theprimaryGroupId
attribute in AD.
– Gabriel Luci
Nov 20 at 15:08
Sorry, I re-read my answer and realized why I confused you :) I updated my answer to make it more clear what's going on, and how to get the primary group.
– Gabriel Luci
Nov 20 at 15:28
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
PowerShell sometimes uses "display names" for the attributes, where the actual name of the attributes in AD are slightly different. PowerShell also has some added properties that translates some of the data in AD to something useful. This is an example of that.
The PrimaryGroup
property returns the distinguished name of the primary group. However, there is no attribute in AD that stores that. The primary group is determined from the primaryGroupId
attribute, which is the RID (Relative Identifier) of the group.
So you have to ask for the primaryGroupId
attribute and translate that to find the actual group. I wrote an article about Finding all of a user’s groups where I shared a method that will do that. This accepts a DirectoryEntry
, but it really just needs to know the primaryGroupId
and the objectSid
(since it uses the user's SID to construct the SID of the group):
private static string GetUserPrimaryGroup(DirectoryEntry de) {
de.RefreshCache(new {"primaryGroupID", "objectSid"});
//Get the user's SID as a string
var sid = new SecurityIdentifier((byte)de.Properties["objectSid"].Value, 0).ToString();
//Replace the RID portion of the user's SID with the primaryGroupId
//so we're left with the group's SID
sid = sid.Remove(sid.LastIndexOf("-", StringComparison.Ordinal) + 1);
sid = sid + de.Properties["primaryGroupId"].Value;
//Find the group by its SID
var group = new DirectoryEntry($"LDAP://<SID={sid}>");
group.RefreshCache(new {"cn"});
return group.Properties["cn"].Value as string;
}
You should be able to adapt that to pull the values from the DirectorySearcher
.
As a side note, if you don't touch the PropertiesToLoad
collection, it will return every attribute with a value (excluding constructed attributes). That said, it is good practice to use the PropertiesToLoad
if you don't legitimately need to see every attribute.
PowerShell sometimes uses "display names" for the attributes, where the actual name of the attributes in AD are slightly different. PowerShell also has some added properties that translates some of the data in AD to something useful. This is an example of that.
The PrimaryGroup
property returns the distinguished name of the primary group. However, there is no attribute in AD that stores that. The primary group is determined from the primaryGroupId
attribute, which is the RID (Relative Identifier) of the group.
So you have to ask for the primaryGroupId
attribute and translate that to find the actual group. I wrote an article about Finding all of a user’s groups where I shared a method that will do that. This accepts a DirectoryEntry
, but it really just needs to know the primaryGroupId
and the objectSid
(since it uses the user's SID to construct the SID of the group):
private static string GetUserPrimaryGroup(DirectoryEntry de) {
de.RefreshCache(new {"primaryGroupID", "objectSid"});
//Get the user's SID as a string
var sid = new SecurityIdentifier((byte)de.Properties["objectSid"].Value, 0).ToString();
//Replace the RID portion of the user's SID with the primaryGroupId
//so we're left with the group's SID
sid = sid.Remove(sid.LastIndexOf("-", StringComparison.Ordinal) + 1);
sid = sid + de.Properties["primaryGroupId"].Value;
//Find the group by its SID
var group = new DirectoryEntry($"LDAP://<SID={sid}>");
group.RefreshCache(new {"cn"});
return group.Properties["cn"].Value as string;
}
You should be able to adapt that to pull the values from the DirectorySearcher
.
As a side note, if you don't touch the PropertiesToLoad
collection, it will return every attribute with a value (excluding constructed attributes). That said, it is good practice to use the PropertiesToLoad
if you don't legitimately need to see every attribute.
edited Nov 20 at 15:28
answered Nov 20 at 14:58
Gabriel Luci
9,72511324
9,72511324
Thanks for the response Gabriel. However, in the return values for the Powershell call I see 'PrimaryGroup' and 'primaryGroupID', both with different values. The C# code will return 'primaryGroupID' if I comment out 'mySearcher.PropertiesToLoad.Add("PrimaryGrou")', but not 'PrimaryGroup'
– GreekFire
Nov 20 at 15:02
Yes, that is expected.PrimaryGroup
doesn't actually exist in AD. It's a PowerShell property that finds the primary group based on theprimaryGroupId
attribute in AD.
– Gabriel Luci
Nov 20 at 15:08
Sorry, I re-read my answer and realized why I confused you :) I updated my answer to make it more clear what's going on, and how to get the primary group.
– Gabriel Luci
Nov 20 at 15:28
add a comment |
Thanks for the response Gabriel. However, in the return values for the Powershell call I see 'PrimaryGroup' and 'primaryGroupID', both with different values. The C# code will return 'primaryGroupID' if I comment out 'mySearcher.PropertiesToLoad.Add("PrimaryGrou")', but not 'PrimaryGroup'
– GreekFire
Nov 20 at 15:02
Yes, that is expected.PrimaryGroup
doesn't actually exist in AD. It's a PowerShell property that finds the primary group based on theprimaryGroupId
attribute in AD.
– Gabriel Luci
Nov 20 at 15:08
Sorry, I re-read my answer and realized why I confused you :) I updated my answer to make it more clear what's going on, and how to get the primary group.
– Gabriel Luci
Nov 20 at 15:28
Thanks for the response Gabriel. However, in the return values for the Powershell call I see 'PrimaryGroup' and 'primaryGroupID', both with different values. The C# code will return 'primaryGroupID' if I comment out 'mySearcher.PropertiesToLoad.Add("PrimaryGrou")', but not 'PrimaryGroup'
– GreekFire
Nov 20 at 15:02
Thanks for the response Gabriel. However, in the return values for the Powershell call I see 'PrimaryGroup' and 'primaryGroupID', both with different values. The C# code will return 'primaryGroupID' if I comment out 'mySearcher.PropertiesToLoad.Add("PrimaryGrou")', but not 'PrimaryGroup'
– GreekFire
Nov 20 at 15:02
Yes, that is expected.
PrimaryGroup
doesn't actually exist in AD. It's a PowerShell property that finds the primary group based on the primaryGroupId
attribute in AD.– Gabriel Luci
Nov 20 at 15:08
Yes, that is expected.
PrimaryGroup
doesn't actually exist in AD. It's a PowerShell property that finds the primary group based on the primaryGroupId
attribute in AD.– Gabriel Luci
Nov 20 at 15:08
Sorry, I re-read my answer and realized why I confused you :) I updated my answer to make it more clear what's going on, and how to get the primary group.
– Gabriel Luci
Nov 20 at 15:28
Sorry, I re-read my answer and realized why I confused you :) I updated my answer to make it more clear what's going on, and how to get the primary group.
– Gabriel Luci
Nov 20 at 15:28
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53395613%2fnet-get-full-property-list-for-ad-computers-to-match-powershell-get-adcomputer%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
qF0 Ju,0HgppR17X 8 2OC f0,qUuR5XGmpWi15bPc6RW,zD