Randomly initialize BitSet with specific length
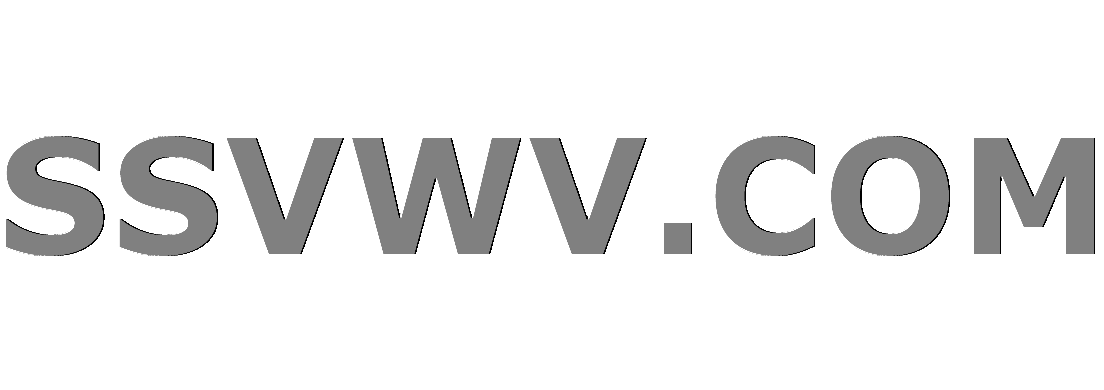
Multi tool use
up vote
1
down vote
favorite
I have two BitSets which have to be initialized randomly with the length of 20 bits.
I am trying to achieve that by initializing the BitSets with 20 bits each and within a for loop iterating through the BitSets and call nextBoolean() of the Random class. However, the length is not always 20. So, I have been playing around with it, and figured it that it might be because it does not count the false bits as part of the length. In case I understand it correctly, how do I force it to have 20 random bits always?
public static void generate() {
BitSet set1 = new BitSet(20);
BitSet set2 = new BitSet(20);
Random r = new SecureRandom();
for (int i = 0; set1.length() < 20 && set2.length() < 20; i++) {
set1.set(i, r.nextBoolean());
set2.set(i, r.nextBoolean());
}
StringBuilder s = new StringBuilder();
for (int i = 0; i < set1.length(); i++) {
s.append(temp1.get(i) == true ? 1 : 0);
}
System.out.println(s + " " + s.length() + " " + set1.length() + " "+ set2.length());
}
Thanks in advance.
java bit bitset
add a comment |
up vote
1
down vote
favorite
I have two BitSets which have to be initialized randomly with the length of 20 bits.
I am trying to achieve that by initializing the BitSets with 20 bits each and within a for loop iterating through the BitSets and call nextBoolean() of the Random class. However, the length is not always 20. So, I have been playing around with it, and figured it that it might be because it does not count the false bits as part of the length. In case I understand it correctly, how do I force it to have 20 random bits always?
public static void generate() {
BitSet set1 = new BitSet(20);
BitSet set2 = new BitSet(20);
Random r = new SecureRandom();
for (int i = 0; set1.length() < 20 && set2.length() < 20; i++) {
set1.set(i, r.nextBoolean());
set2.set(i, r.nextBoolean());
}
StringBuilder s = new StringBuilder();
for (int i = 0; i < set1.length(); i++) {
s.append(temp1.get(i) == true ? 1 : 0);
}
System.out.println(s + " " + s.length() + " " + set1.length() + " "+ set2.length());
}
Thanks in advance.
java bit bitset
if you always want 20 as your size, why not use a constant? Something likefinal int size = 20;
. Then you can replace all occurrences of20
withsize
– user3170251
Nov 20 at 14:51
Thanks for you suggestion. I have tried that, but it did not resolve the problem, unfortunately. The length is still inconsistent and now it goes above 20 sometimes
– Bab
Nov 20 at 14:59
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I have two BitSets which have to be initialized randomly with the length of 20 bits.
I am trying to achieve that by initializing the BitSets with 20 bits each and within a for loop iterating through the BitSets and call nextBoolean() of the Random class. However, the length is not always 20. So, I have been playing around with it, and figured it that it might be because it does not count the false bits as part of the length. In case I understand it correctly, how do I force it to have 20 random bits always?
public static void generate() {
BitSet set1 = new BitSet(20);
BitSet set2 = new BitSet(20);
Random r = new SecureRandom();
for (int i = 0; set1.length() < 20 && set2.length() < 20; i++) {
set1.set(i, r.nextBoolean());
set2.set(i, r.nextBoolean());
}
StringBuilder s = new StringBuilder();
for (int i = 0; i < set1.length(); i++) {
s.append(temp1.get(i) == true ? 1 : 0);
}
System.out.println(s + " " + s.length() + " " + set1.length() + " "+ set2.length());
}
Thanks in advance.
java bit bitset
I have two BitSets which have to be initialized randomly with the length of 20 bits.
I am trying to achieve that by initializing the BitSets with 20 bits each and within a for loop iterating through the BitSets and call nextBoolean() of the Random class. However, the length is not always 20. So, I have been playing around with it, and figured it that it might be because it does not count the false bits as part of the length. In case I understand it correctly, how do I force it to have 20 random bits always?
public static void generate() {
BitSet set1 = new BitSet(20);
BitSet set2 = new BitSet(20);
Random r = new SecureRandom();
for (int i = 0; set1.length() < 20 && set2.length() < 20; i++) {
set1.set(i, r.nextBoolean());
set2.set(i, r.nextBoolean());
}
StringBuilder s = new StringBuilder();
for (int i = 0; i < set1.length(); i++) {
s.append(temp1.get(i) == true ? 1 : 0);
}
System.out.println(s + " " + s.length() + " " + set1.length() + " "+ set2.length());
}
Thanks in advance.
java bit bitset
java bit bitset
edited Nov 20 at 15:57


John McClane
1,0652317
1,0652317
asked Nov 20 at 14:49
Bab
12910
12910
if you always want 20 as your size, why not use a constant? Something likefinal int size = 20;
. Then you can replace all occurrences of20
withsize
– user3170251
Nov 20 at 14:51
Thanks for you suggestion. I have tried that, but it did not resolve the problem, unfortunately. The length is still inconsistent and now it goes above 20 sometimes
– Bab
Nov 20 at 14:59
add a comment |
if you always want 20 as your size, why not use a constant? Something likefinal int size = 20;
. Then you can replace all occurrences of20
withsize
– user3170251
Nov 20 at 14:51
Thanks for you suggestion. I have tried that, but it did not resolve the problem, unfortunately. The length is still inconsistent and now it goes above 20 sometimes
– Bab
Nov 20 at 14:59
if you always want 20 as your size, why not use a constant? Something like
final int size = 20;
. Then you can replace all occurrences of 20
with size
– user3170251
Nov 20 at 14:51
if you always want 20 as your size, why not use a constant? Something like
final int size = 20;
. Then you can replace all occurrences of 20
with size
– user3170251
Nov 20 at 14:51
Thanks for you suggestion. I have tried that, but it did not resolve the problem, unfortunately. The length is still inconsistent and now it goes above 20 sometimes
– Bab
Nov 20 at 14:59
Thanks for you suggestion. I have tried that, but it did not resolve the problem, unfortunately. The length is still inconsistent and now it goes above 20 sometimes
– Bab
Nov 20 at 14:59
add a comment |
3 Answers
3
active
oldest
votes
up vote
0
down vote
accepted
In case I understand it correctly, how do I force it to have 20 random bits always?
Change your for-loops to:
for (int i = 0; i < 20; i++) {
set1.set(i, r.nextBoolean());
set2.set(i, r.nextBoolean());
}
...
for (int i = 0; i < 20; i++) {
s.append(temp1.get(i) == true ? 1 : 0);
}
A BitSet
is backed by a long
and all bits are initially set to false
, so calling BitSet#length
will not return the value 20
unless the 19
th bit happens to be set, as stated by its documentation:
Returns the "logical size" of this BitSet: the index of the highest set bit in the BitSet plus one. Returns zero if the BitSet contains no set bits.
By using the value 20
as the condition in your for-loops, you're ensuring that the first 20
bits will have the opportunity of being set randomly.
So even if I change my for loops and print the BitSet length, I won't always get 20 bits? An example of my print:10101001110111111110 20 19 20
. 20 is the length of the StringBuilder, 19 is the length of the first BitSet and 20 is the length of the second BitSet
– Bab
Nov 20 at 16:12
There's a 50% chance you'll get a length of20
, as it depends on the last bit set by the for-loop. But if you use20
as the condition in the for-loop, you can be sure that the first20
bits will be set randomly, even ifBitSet#length
doesn't return20
.
– Jacob G.
Nov 20 at 16:14
I see. What must I do in order to ensure that there are exactly 20 random bits then? i.e. 20 in length
– Bab
Nov 20 at 16:41
By just changing the for-loop condition.BitSet#length
depends on the last bit set, so don't rely on its value, as you're setting bits randomly.
– Jacob G.
Nov 20 at 16:44
Maybe I am misunderstanding you; I understand that I have to change the for loops, but what confuses me then is that when I print the length of the BitSet after the for loops, it is inconsistent. I do understand that if I prints
I will get 20, but that is not the real BitSet's length. Would an if statement, which always sets the 20th bit to true help?
– Bab
Nov 20 at 16:52
|
show 2 more comments
up vote
0
down vote
Why not use Bitset.valueOf(byte array)
to initialize the bit set from a random byte array?
Something like:
public BitSet getBits(SecureRandom sr, int size) {
byte ar = new byte[(int) Math.ceil(size / 8F)];
sr.nextBytes(ar);
return BitSet.valueOf(ar).get(0, size);
}
add a comment |
up vote
0
down vote
If you are using Java 7, you can initialize a random byte array with Random.nextBytes(byte) then use the static BitSet.valueOf(byte) method to create a BitSet from the same byte array.
Random rnd = new Random();
// ...
byte randomBytes = new byte[NUM_BYTES];
rnd.nextBytes(randomBytes);
return BitSet.valueOf(randomBytes);
Credits for: https://stackoverflow.com/a/8566871/5133329
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53395590%2frandomly-initialize-bitset-with-specific-length%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
In case I understand it correctly, how do I force it to have 20 random bits always?
Change your for-loops to:
for (int i = 0; i < 20; i++) {
set1.set(i, r.nextBoolean());
set2.set(i, r.nextBoolean());
}
...
for (int i = 0; i < 20; i++) {
s.append(temp1.get(i) == true ? 1 : 0);
}
A BitSet
is backed by a long
and all bits are initially set to false
, so calling BitSet#length
will not return the value 20
unless the 19
th bit happens to be set, as stated by its documentation:
Returns the "logical size" of this BitSet: the index of the highest set bit in the BitSet plus one. Returns zero if the BitSet contains no set bits.
By using the value 20
as the condition in your for-loops, you're ensuring that the first 20
bits will have the opportunity of being set randomly.
So even if I change my for loops and print the BitSet length, I won't always get 20 bits? An example of my print:10101001110111111110 20 19 20
. 20 is the length of the StringBuilder, 19 is the length of the first BitSet and 20 is the length of the second BitSet
– Bab
Nov 20 at 16:12
There's a 50% chance you'll get a length of20
, as it depends on the last bit set by the for-loop. But if you use20
as the condition in the for-loop, you can be sure that the first20
bits will be set randomly, even ifBitSet#length
doesn't return20
.
– Jacob G.
Nov 20 at 16:14
I see. What must I do in order to ensure that there are exactly 20 random bits then? i.e. 20 in length
– Bab
Nov 20 at 16:41
By just changing the for-loop condition.BitSet#length
depends on the last bit set, so don't rely on its value, as you're setting bits randomly.
– Jacob G.
Nov 20 at 16:44
Maybe I am misunderstanding you; I understand that I have to change the for loops, but what confuses me then is that when I print the length of the BitSet after the for loops, it is inconsistent. I do understand that if I prints
I will get 20, but that is not the real BitSet's length. Would an if statement, which always sets the 20th bit to true help?
– Bab
Nov 20 at 16:52
|
show 2 more comments
up vote
0
down vote
accepted
In case I understand it correctly, how do I force it to have 20 random bits always?
Change your for-loops to:
for (int i = 0; i < 20; i++) {
set1.set(i, r.nextBoolean());
set2.set(i, r.nextBoolean());
}
...
for (int i = 0; i < 20; i++) {
s.append(temp1.get(i) == true ? 1 : 0);
}
A BitSet
is backed by a long
and all bits are initially set to false
, so calling BitSet#length
will not return the value 20
unless the 19
th bit happens to be set, as stated by its documentation:
Returns the "logical size" of this BitSet: the index of the highest set bit in the BitSet plus one. Returns zero if the BitSet contains no set bits.
By using the value 20
as the condition in your for-loops, you're ensuring that the first 20
bits will have the opportunity of being set randomly.
So even if I change my for loops and print the BitSet length, I won't always get 20 bits? An example of my print:10101001110111111110 20 19 20
. 20 is the length of the StringBuilder, 19 is the length of the first BitSet and 20 is the length of the second BitSet
– Bab
Nov 20 at 16:12
There's a 50% chance you'll get a length of20
, as it depends on the last bit set by the for-loop. But if you use20
as the condition in the for-loop, you can be sure that the first20
bits will be set randomly, even ifBitSet#length
doesn't return20
.
– Jacob G.
Nov 20 at 16:14
I see. What must I do in order to ensure that there are exactly 20 random bits then? i.e. 20 in length
– Bab
Nov 20 at 16:41
By just changing the for-loop condition.BitSet#length
depends on the last bit set, so don't rely on its value, as you're setting bits randomly.
– Jacob G.
Nov 20 at 16:44
Maybe I am misunderstanding you; I understand that I have to change the for loops, but what confuses me then is that when I print the length of the BitSet after the for loops, it is inconsistent. I do understand that if I prints
I will get 20, but that is not the real BitSet's length. Would an if statement, which always sets the 20th bit to true help?
– Bab
Nov 20 at 16:52
|
show 2 more comments
up vote
0
down vote
accepted
up vote
0
down vote
accepted
In case I understand it correctly, how do I force it to have 20 random bits always?
Change your for-loops to:
for (int i = 0; i < 20; i++) {
set1.set(i, r.nextBoolean());
set2.set(i, r.nextBoolean());
}
...
for (int i = 0; i < 20; i++) {
s.append(temp1.get(i) == true ? 1 : 0);
}
A BitSet
is backed by a long
and all bits are initially set to false
, so calling BitSet#length
will not return the value 20
unless the 19
th bit happens to be set, as stated by its documentation:
Returns the "logical size" of this BitSet: the index of the highest set bit in the BitSet plus one. Returns zero if the BitSet contains no set bits.
By using the value 20
as the condition in your for-loops, you're ensuring that the first 20
bits will have the opportunity of being set randomly.
In case I understand it correctly, how do I force it to have 20 random bits always?
Change your for-loops to:
for (int i = 0; i < 20; i++) {
set1.set(i, r.nextBoolean());
set2.set(i, r.nextBoolean());
}
...
for (int i = 0; i < 20; i++) {
s.append(temp1.get(i) == true ? 1 : 0);
}
A BitSet
is backed by a long
and all bits are initially set to false
, so calling BitSet#length
will not return the value 20
unless the 19
th bit happens to be set, as stated by its documentation:
Returns the "logical size" of this BitSet: the index of the highest set bit in the BitSet plus one. Returns zero if the BitSet contains no set bits.
By using the value 20
as the condition in your for-loops, you're ensuring that the first 20
bits will have the opportunity of being set randomly.
answered Nov 20 at 15:08


Jacob G.
15.2k52162
15.2k52162
So even if I change my for loops and print the BitSet length, I won't always get 20 bits? An example of my print:10101001110111111110 20 19 20
. 20 is the length of the StringBuilder, 19 is the length of the first BitSet and 20 is the length of the second BitSet
– Bab
Nov 20 at 16:12
There's a 50% chance you'll get a length of20
, as it depends on the last bit set by the for-loop. But if you use20
as the condition in the for-loop, you can be sure that the first20
bits will be set randomly, even ifBitSet#length
doesn't return20
.
– Jacob G.
Nov 20 at 16:14
I see. What must I do in order to ensure that there are exactly 20 random bits then? i.e. 20 in length
– Bab
Nov 20 at 16:41
By just changing the for-loop condition.BitSet#length
depends on the last bit set, so don't rely on its value, as you're setting bits randomly.
– Jacob G.
Nov 20 at 16:44
Maybe I am misunderstanding you; I understand that I have to change the for loops, but what confuses me then is that when I print the length of the BitSet after the for loops, it is inconsistent. I do understand that if I prints
I will get 20, but that is not the real BitSet's length. Would an if statement, which always sets the 20th bit to true help?
– Bab
Nov 20 at 16:52
|
show 2 more comments
So even if I change my for loops and print the BitSet length, I won't always get 20 bits? An example of my print:10101001110111111110 20 19 20
. 20 is the length of the StringBuilder, 19 is the length of the first BitSet and 20 is the length of the second BitSet
– Bab
Nov 20 at 16:12
There's a 50% chance you'll get a length of20
, as it depends on the last bit set by the for-loop. But if you use20
as the condition in the for-loop, you can be sure that the first20
bits will be set randomly, even ifBitSet#length
doesn't return20
.
– Jacob G.
Nov 20 at 16:14
I see. What must I do in order to ensure that there are exactly 20 random bits then? i.e. 20 in length
– Bab
Nov 20 at 16:41
By just changing the for-loop condition.BitSet#length
depends on the last bit set, so don't rely on its value, as you're setting bits randomly.
– Jacob G.
Nov 20 at 16:44
Maybe I am misunderstanding you; I understand that I have to change the for loops, but what confuses me then is that when I print the length of the BitSet after the for loops, it is inconsistent. I do understand that if I prints
I will get 20, but that is not the real BitSet's length. Would an if statement, which always sets the 20th bit to true help?
– Bab
Nov 20 at 16:52
So even if I change my for loops and print the BitSet length, I won't always get 20 bits? An example of my print:
10101001110111111110 20 19 20
. 20 is the length of the StringBuilder, 19 is the length of the first BitSet and 20 is the length of the second BitSet– Bab
Nov 20 at 16:12
So even if I change my for loops and print the BitSet length, I won't always get 20 bits? An example of my print:
10101001110111111110 20 19 20
. 20 is the length of the StringBuilder, 19 is the length of the first BitSet and 20 is the length of the second BitSet– Bab
Nov 20 at 16:12
There's a 50% chance you'll get a length of
20
, as it depends on the last bit set by the for-loop. But if you use 20
as the condition in the for-loop, you can be sure that the first 20
bits will be set randomly, even if BitSet#length
doesn't return 20
.– Jacob G.
Nov 20 at 16:14
There's a 50% chance you'll get a length of
20
, as it depends on the last bit set by the for-loop. But if you use 20
as the condition in the for-loop, you can be sure that the first 20
bits will be set randomly, even if BitSet#length
doesn't return 20
.– Jacob G.
Nov 20 at 16:14
I see. What must I do in order to ensure that there are exactly 20 random bits then? i.e. 20 in length
– Bab
Nov 20 at 16:41
I see. What must I do in order to ensure that there are exactly 20 random bits then? i.e. 20 in length
– Bab
Nov 20 at 16:41
By just changing the for-loop condition.
BitSet#length
depends on the last bit set, so don't rely on its value, as you're setting bits randomly.– Jacob G.
Nov 20 at 16:44
By just changing the for-loop condition.
BitSet#length
depends on the last bit set, so don't rely on its value, as you're setting bits randomly.– Jacob G.
Nov 20 at 16:44
Maybe I am misunderstanding you; I understand that I have to change the for loops, but what confuses me then is that when I print the length of the BitSet after the for loops, it is inconsistent. I do understand that if I print
s
I will get 20, but that is not the real BitSet's length. Would an if statement, which always sets the 20th bit to true help?– Bab
Nov 20 at 16:52
Maybe I am misunderstanding you; I understand that I have to change the for loops, but what confuses me then is that when I print the length of the BitSet after the for loops, it is inconsistent. I do understand that if I print
s
I will get 20, but that is not the real BitSet's length. Would an if statement, which always sets the 20th bit to true help?– Bab
Nov 20 at 16:52
|
show 2 more comments
up vote
0
down vote
Why not use Bitset.valueOf(byte array)
to initialize the bit set from a random byte array?
Something like:
public BitSet getBits(SecureRandom sr, int size) {
byte ar = new byte[(int) Math.ceil(size / 8F)];
sr.nextBytes(ar);
return BitSet.valueOf(ar).get(0, size);
}
add a comment |
up vote
0
down vote
Why not use Bitset.valueOf(byte array)
to initialize the bit set from a random byte array?
Something like:
public BitSet getBits(SecureRandom sr, int size) {
byte ar = new byte[(int) Math.ceil(size / 8F)];
sr.nextBytes(ar);
return BitSet.valueOf(ar).get(0, size);
}
add a comment |
up vote
0
down vote
up vote
0
down vote
Why not use Bitset.valueOf(byte array)
to initialize the bit set from a random byte array?
Something like:
public BitSet getBits(SecureRandom sr, int size) {
byte ar = new byte[(int) Math.ceil(size / 8F)];
sr.nextBytes(ar);
return BitSet.valueOf(ar).get(0, size);
}
Why not use Bitset.valueOf(byte array)
to initialize the bit set from a random byte array?
Something like:
public BitSet getBits(SecureRandom sr, int size) {
byte ar = new byte[(int) Math.ceil(size / 8F)];
sr.nextBytes(ar);
return BitSet.valueOf(ar).get(0, size);
}
answered Nov 20 at 15:04
Guss
13.3k116090
13.3k116090
add a comment |
add a comment |
up vote
0
down vote
If you are using Java 7, you can initialize a random byte array with Random.nextBytes(byte) then use the static BitSet.valueOf(byte) method to create a BitSet from the same byte array.
Random rnd = new Random();
// ...
byte randomBytes = new byte[NUM_BYTES];
rnd.nextBytes(randomBytes);
return BitSet.valueOf(randomBytes);
Credits for: https://stackoverflow.com/a/8566871/5133329
add a comment |
up vote
0
down vote
If you are using Java 7, you can initialize a random byte array with Random.nextBytes(byte) then use the static BitSet.valueOf(byte) method to create a BitSet from the same byte array.
Random rnd = new Random();
// ...
byte randomBytes = new byte[NUM_BYTES];
rnd.nextBytes(randomBytes);
return BitSet.valueOf(randomBytes);
Credits for: https://stackoverflow.com/a/8566871/5133329
add a comment |
up vote
0
down vote
up vote
0
down vote
If you are using Java 7, you can initialize a random byte array with Random.nextBytes(byte) then use the static BitSet.valueOf(byte) method to create a BitSet from the same byte array.
Random rnd = new Random();
// ...
byte randomBytes = new byte[NUM_BYTES];
rnd.nextBytes(randomBytes);
return BitSet.valueOf(randomBytes);
Credits for: https://stackoverflow.com/a/8566871/5133329
If you are using Java 7, you can initialize a random byte array with Random.nextBytes(byte) then use the static BitSet.valueOf(byte) method to create a BitSet from the same byte array.
Random rnd = new Random();
// ...
byte randomBytes = new byte[NUM_BYTES];
rnd.nextBytes(randomBytes);
return BitSet.valueOf(randomBytes);
Credits for: https://stackoverflow.com/a/8566871/5133329
answered Nov 20 at 15:32
Josemy
485618
485618
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53395590%2frandomly-initialize-bitset-with-specific-length%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
lXvi4t5mgUsPNpkRNdan4rT3c gUTe6Kt fYTl 5r,Rpqw6uqOaZp4 dtom4lU2,5c1 twn7 ae VQFJ,1q zKN3e
if you always want 20 as your size, why not use a constant? Something like
final int size = 20;
. Then you can replace all occurrences of20
withsize
– user3170251
Nov 20 at 14:51
Thanks for you suggestion. I have tried that, but it did not resolve the problem, unfortunately. The length is still inconsistent and now it goes above 20 sometimes
– Bab
Nov 20 at 14:59