How can I keep a Console App open when all processes are running on separate threads in c#?
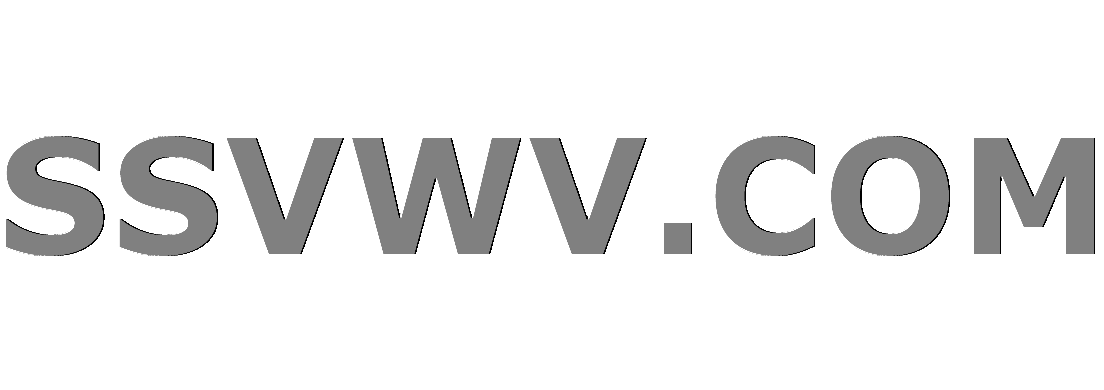
Multi tool use
I am trying to make a simple server application to learn the basics of multi threaded server programming in c#. The basic idea is simple: the client connects to the server and sends: "get time" to receive to current time of the server. All of the tcplistener threads and sockets should be running on seperate threads. I am not sure why, but when the application finishes initializing all of the threads, the console app closes.
Here is the server code:
class Program
{
static public int minPort;
static public int maxPort;
static int openPort = 0;
static byte sendData = new byte[1024];
static TcpListener listeners;
static Thread connectionThreads;
static void Main()
{
Console.Write("What do you want your minimum port to be? ");
minPort = Convert.ToInt32(Console.ReadLine());
Console.Write("What do you want your maximum port to be? ");
maxPort = Convert.ToInt32(Console.ReadLine());
//init
ThreadStart streamThreadStart = new ThreadStart(DataStream);
openPort = maxPort - minPort;
listeners = new TcpListener[maxPort - minPort];
connectionThreads = new Thread[maxPort - minPort];
for (int i = 0; i == maxPort - minPort; i++)
{
listeners[i] = new TcpListener(IPAddress.Any, minPort + i);
connectionThreads[i] = new Thread(streamThreadStart);
Thread.Sleep(10);
openPort = openPort + 1;
}
}
static void DataStream()
{
int port = openPort;
byte receiveData = new byte[1024];
listeners[openPort].Start();
Socket socket = listeners[port].AcceptSocket();
NetworkStream stream = new NetworkStream(socket);
while (true)
{
socket.Receive(receiveData);
Console.WriteLine("Received: " + BitConverter.ToString(receiveData));
socket.Send(parseCommand(receiveData));
}
}
static byte parseCommand(byte input)
{
string RCommand = BitConverter.ToString(input);
string SCommand;
if (RCommand == "get time")
{
SCommand = DateTime.Now.ToUniversalTime().ToString();
}else
{
SCommand = "Unknown Command, please try again";
}
byte output = Encoding.ASCII.GetBytes(SCommand);
return output;
}
}
c# .net multithreading sockets console-application
add a comment |
I am trying to make a simple server application to learn the basics of multi threaded server programming in c#. The basic idea is simple: the client connects to the server and sends: "get time" to receive to current time of the server. All of the tcplistener threads and sockets should be running on seperate threads. I am not sure why, but when the application finishes initializing all of the threads, the console app closes.
Here is the server code:
class Program
{
static public int minPort;
static public int maxPort;
static int openPort = 0;
static byte sendData = new byte[1024];
static TcpListener listeners;
static Thread connectionThreads;
static void Main()
{
Console.Write("What do you want your minimum port to be? ");
minPort = Convert.ToInt32(Console.ReadLine());
Console.Write("What do you want your maximum port to be? ");
maxPort = Convert.ToInt32(Console.ReadLine());
//init
ThreadStart streamThreadStart = new ThreadStart(DataStream);
openPort = maxPort - minPort;
listeners = new TcpListener[maxPort - minPort];
connectionThreads = new Thread[maxPort - minPort];
for (int i = 0; i == maxPort - minPort; i++)
{
listeners[i] = new TcpListener(IPAddress.Any, minPort + i);
connectionThreads[i] = new Thread(streamThreadStart);
Thread.Sleep(10);
openPort = openPort + 1;
}
}
static void DataStream()
{
int port = openPort;
byte receiveData = new byte[1024];
listeners[openPort].Start();
Socket socket = listeners[port].AcceptSocket();
NetworkStream stream = new NetworkStream(socket);
while (true)
{
socket.Receive(receiveData);
Console.WriteLine("Received: " + BitConverter.ToString(receiveData));
socket.Send(parseCommand(receiveData));
}
}
static byte parseCommand(byte input)
{
string RCommand = BitConverter.ToString(input);
string SCommand;
if (RCommand == "get time")
{
SCommand = DateTime.Now.ToUniversalTime().ToString();
}else
{
SCommand = "Unknown Command, please try again";
}
byte output = Encoding.ASCII.GetBytes(SCommand);
return output;
}
}
c# .net multithreading sockets console-application
In a console application when main is complete, the application closes as there is no more code to execute. You will need to build a loop in main to keep the console application running.
– Ryan Wilson
Nov 21 '18 at 17:56
You know that one listener socket can service multiple clients and hand off the accepted sockets? There should never be a need to run listeners on multiple ports unless you're doing something very odd. E.g. common web servers only listen on ports 80 and/or 443. Do you think these servers dealing with thousands of clients only deal with a single client at a time? Lots of research/reading required here.
– Damien_The_Unbeliever
Nov 21 '18 at 18:06
Also, you have to pay attention to the return value fromReceive
and moral equivalents. Because all the TCP gives you is a stream of bytes, not messages. It doesn't guarantee to fill your buffer or that what you will receive matches a single buffer passed to a single call ofSend
at the other end.
– Damien_The_Unbeliever
Nov 21 '18 at 18:11
Another option would be to create a Windows Service, which is designed to run long-term without closing.
– BJ Myers
Nov 21 '18 at 19:29
add a comment |
I am trying to make a simple server application to learn the basics of multi threaded server programming in c#. The basic idea is simple: the client connects to the server and sends: "get time" to receive to current time of the server. All of the tcplistener threads and sockets should be running on seperate threads. I am not sure why, but when the application finishes initializing all of the threads, the console app closes.
Here is the server code:
class Program
{
static public int minPort;
static public int maxPort;
static int openPort = 0;
static byte sendData = new byte[1024];
static TcpListener listeners;
static Thread connectionThreads;
static void Main()
{
Console.Write("What do you want your minimum port to be? ");
minPort = Convert.ToInt32(Console.ReadLine());
Console.Write("What do you want your maximum port to be? ");
maxPort = Convert.ToInt32(Console.ReadLine());
//init
ThreadStart streamThreadStart = new ThreadStart(DataStream);
openPort = maxPort - minPort;
listeners = new TcpListener[maxPort - minPort];
connectionThreads = new Thread[maxPort - minPort];
for (int i = 0; i == maxPort - minPort; i++)
{
listeners[i] = new TcpListener(IPAddress.Any, minPort + i);
connectionThreads[i] = new Thread(streamThreadStart);
Thread.Sleep(10);
openPort = openPort + 1;
}
}
static void DataStream()
{
int port = openPort;
byte receiveData = new byte[1024];
listeners[openPort].Start();
Socket socket = listeners[port].AcceptSocket();
NetworkStream stream = new NetworkStream(socket);
while (true)
{
socket.Receive(receiveData);
Console.WriteLine("Received: " + BitConverter.ToString(receiveData));
socket.Send(parseCommand(receiveData));
}
}
static byte parseCommand(byte input)
{
string RCommand = BitConverter.ToString(input);
string SCommand;
if (RCommand == "get time")
{
SCommand = DateTime.Now.ToUniversalTime().ToString();
}else
{
SCommand = "Unknown Command, please try again";
}
byte output = Encoding.ASCII.GetBytes(SCommand);
return output;
}
}
c# .net multithreading sockets console-application
I am trying to make a simple server application to learn the basics of multi threaded server programming in c#. The basic idea is simple: the client connects to the server and sends: "get time" to receive to current time of the server. All of the tcplistener threads and sockets should be running on seperate threads. I am not sure why, but when the application finishes initializing all of the threads, the console app closes.
Here is the server code:
class Program
{
static public int minPort;
static public int maxPort;
static int openPort = 0;
static byte sendData = new byte[1024];
static TcpListener listeners;
static Thread connectionThreads;
static void Main()
{
Console.Write("What do you want your minimum port to be? ");
minPort = Convert.ToInt32(Console.ReadLine());
Console.Write("What do you want your maximum port to be? ");
maxPort = Convert.ToInt32(Console.ReadLine());
//init
ThreadStart streamThreadStart = new ThreadStart(DataStream);
openPort = maxPort - minPort;
listeners = new TcpListener[maxPort - minPort];
connectionThreads = new Thread[maxPort - minPort];
for (int i = 0; i == maxPort - minPort; i++)
{
listeners[i] = new TcpListener(IPAddress.Any, minPort + i);
connectionThreads[i] = new Thread(streamThreadStart);
Thread.Sleep(10);
openPort = openPort + 1;
}
}
static void DataStream()
{
int port = openPort;
byte receiveData = new byte[1024];
listeners[openPort].Start();
Socket socket = listeners[port].AcceptSocket();
NetworkStream stream = new NetworkStream(socket);
while (true)
{
socket.Receive(receiveData);
Console.WriteLine("Received: " + BitConverter.ToString(receiveData));
socket.Send(parseCommand(receiveData));
}
}
static byte parseCommand(byte input)
{
string RCommand = BitConverter.ToString(input);
string SCommand;
if (RCommand == "get time")
{
SCommand = DateTime.Now.ToUniversalTime().ToString();
}else
{
SCommand = "Unknown Command, please try again";
}
byte output = Encoding.ASCII.GetBytes(SCommand);
return output;
}
}
c# .net multithreading sockets console-application
c# .net multithreading sockets console-application
asked Nov 21 '18 at 17:53
user7323126user7323126
31
31
In a console application when main is complete, the application closes as there is no more code to execute. You will need to build a loop in main to keep the console application running.
– Ryan Wilson
Nov 21 '18 at 17:56
You know that one listener socket can service multiple clients and hand off the accepted sockets? There should never be a need to run listeners on multiple ports unless you're doing something very odd. E.g. common web servers only listen on ports 80 and/or 443. Do you think these servers dealing with thousands of clients only deal with a single client at a time? Lots of research/reading required here.
– Damien_The_Unbeliever
Nov 21 '18 at 18:06
Also, you have to pay attention to the return value fromReceive
and moral equivalents. Because all the TCP gives you is a stream of bytes, not messages. It doesn't guarantee to fill your buffer or that what you will receive matches a single buffer passed to a single call ofSend
at the other end.
– Damien_The_Unbeliever
Nov 21 '18 at 18:11
Another option would be to create a Windows Service, which is designed to run long-term without closing.
– BJ Myers
Nov 21 '18 at 19:29
add a comment |
In a console application when main is complete, the application closes as there is no more code to execute. You will need to build a loop in main to keep the console application running.
– Ryan Wilson
Nov 21 '18 at 17:56
You know that one listener socket can service multiple clients and hand off the accepted sockets? There should never be a need to run listeners on multiple ports unless you're doing something very odd. E.g. common web servers only listen on ports 80 and/or 443. Do you think these servers dealing with thousands of clients only deal with a single client at a time? Lots of research/reading required here.
– Damien_The_Unbeliever
Nov 21 '18 at 18:06
Also, you have to pay attention to the return value fromReceive
and moral equivalents. Because all the TCP gives you is a stream of bytes, not messages. It doesn't guarantee to fill your buffer or that what you will receive matches a single buffer passed to a single call ofSend
at the other end.
– Damien_The_Unbeliever
Nov 21 '18 at 18:11
Another option would be to create a Windows Service, which is designed to run long-term without closing.
– BJ Myers
Nov 21 '18 at 19:29
In a console application when main is complete, the application closes as there is no more code to execute. You will need to build a loop in main to keep the console application running.
– Ryan Wilson
Nov 21 '18 at 17:56
In a console application when main is complete, the application closes as there is no more code to execute. You will need to build a loop in main to keep the console application running.
– Ryan Wilson
Nov 21 '18 at 17:56
You know that one listener socket can service multiple clients and hand off the accepted sockets? There should never be a need to run listeners on multiple ports unless you're doing something very odd. E.g. common web servers only listen on ports 80 and/or 443. Do you think these servers dealing with thousands of clients only deal with a single client at a time? Lots of research/reading required here.
– Damien_The_Unbeliever
Nov 21 '18 at 18:06
You know that one listener socket can service multiple clients and hand off the accepted sockets? There should never be a need to run listeners on multiple ports unless you're doing something very odd. E.g. common web servers only listen on ports 80 and/or 443. Do you think these servers dealing with thousands of clients only deal with a single client at a time? Lots of research/reading required here.
– Damien_The_Unbeliever
Nov 21 '18 at 18:06
Also, you have to pay attention to the return value from
Receive
and moral equivalents. Because all the TCP gives you is a stream of bytes, not messages. It doesn't guarantee to fill your buffer or that what you will receive matches a single buffer passed to a single call of Send
at the other end.– Damien_The_Unbeliever
Nov 21 '18 at 18:11
Also, you have to pay attention to the return value from
Receive
and moral equivalents. Because all the TCP gives you is a stream of bytes, not messages. It doesn't guarantee to fill your buffer or that what you will receive matches a single buffer passed to a single call of Send
at the other end.– Damien_The_Unbeliever
Nov 21 '18 at 18:11
Another option would be to create a Windows Service, which is designed to run long-term without closing.
– BJ Myers
Nov 21 '18 at 19:29
Another option would be to create a Windows Service, which is designed to run long-term without closing.
– BJ Myers
Nov 21 '18 at 19:29
add a comment |
3 Answers
3
active
oldest
votes
You need to join your threads before exiting.
static public void Main()
{
/*
Existing code goes here
*/
//Before exiting, make sure all child threads are finished
foreach (var thread in connectionThreads) thread.Join();
}
When your program calls Thread.Join, it is telling the operating system that it needn't schedule the main thread for any timeslices until the child thread is finished. This makes it lighter weight than other techniques, such as busywaiting (i.e. running a while
loop); while the main thread will still hold onto resources, it won't consume any CPU time.
See also When would I use Thread.Join? and c# Waiting for multiple threads to finish
While I do like this answer, since it discusses the correct way to join threads back to the main thread. It does not handle the problem of having a console app that cannot be controlled. Solutions for this are, as suggested the Windows service, or changing the main thread so it also reacts to user input.
– Ben Ootjers
Nov 21 '18 at 20:58
add a comment |
In general, best practice is for a console application to offer an "Enter any key to exit" prompt when the user wants to stop the application. But you can always query for a specific key-press to quit, such as 'q'.
Here is some code to get you started:
Console.WriteLine("Press any key to exit...");
Console.ReadKey();
add a comment |
Your code doesn't start new thread!
So, i made some changes in your code:
class Program
{
static public int minPort;
static public int maxPort;
//static int openPort = 0;
static byte sendData = new byte[1024];
static TcpListener listeners;
static Thread connectionThreads;
static void Main()
{
Console.Write("What do you want your minimum port to be? ");
minPort = Convert.ToInt32(Console.ReadLine());
Console.Write("What do you want your maximum port to be? ");
maxPort = Convert.ToInt32(Console.ReadLine());
//init
// ThreadStart streamThreadStart = new ThreadStart(DataStream(0));
//openPort = minPort;
listeners = new TcpListener[maxPort - minPort];
connectionThreads = new Thread[maxPort - minPort];
//for (int i = 0; i == maxPort - minPort; i++) it can't work
for (int i = 0; i < maxPort - minPort; i++)
{
listeners[i] = new TcpListener(IPAddress.Any, minPort + i);
connectionThreads[i] = new Thread(new ParameterizedThreadStart(DataStream)); //thread with start parameter
connectionThreads[i].Start(i); // start thread with index
Thread.Sleep(10);
}
}
static void DataStream(object o)
{
int index = (int)o; //get index
byte receiveData = new byte[1024];
listeners[index].Start(); // start listener with right index
Socket socket = listeners[index].AcceptSocket();
NetworkStream stream = new NetworkStream(socket);
while (true)
{
socket.Receive(receiveData);
Console.WriteLine("Received: " + BitConverter.ToString(receiveData));
socket.Send(parseCommand(receiveData));
}
}
static byte parseCommand(byte input)
{
string RCommand = BitConverter.ToString(input);
string SCommand;
if (RCommand == "get time")
{
SCommand = DateTime.Now.ToUniversalTime().ToString();
}
else
{
SCommand = "Unknown Command, please try again";
}
byte output = Encoding.ASCII.GetBytes(SCommand);
return output;
}
}
2
Please give a detailed description of what you have changed and why this makes it an answer.
– Poul Bak
Nov 21 '18 at 19:16
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53417950%2fhow-can-i-keep-a-console-app-open-when-all-processes-are-running-on-separate-thr%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
You need to join your threads before exiting.
static public void Main()
{
/*
Existing code goes here
*/
//Before exiting, make sure all child threads are finished
foreach (var thread in connectionThreads) thread.Join();
}
When your program calls Thread.Join, it is telling the operating system that it needn't schedule the main thread for any timeslices until the child thread is finished. This makes it lighter weight than other techniques, such as busywaiting (i.e. running a while
loop); while the main thread will still hold onto resources, it won't consume any CPU time.
See also When would I use Thread.Join? and c# Waiting for multiple threads to finish
While I do like this answer, since it discusses the correct way to join threads back to the main thread. It does not handle the problem of having a console app that cannot be controlled. Solutions for this are, as suggested the Windows service, or changing the main thread so it also reacts to user input.
– Ben Ootjers
Nov 21 '18 at 20:58
add a comment |
You need to join your threads before exiting.
static public void Main()
{
/*
Existing code goes here
*/
//Before exiting, make sure all child threads are finished
foreach (var thread in connectionThreads) thread.Join();
}
When your program calls Thread.Join, it is telling the operating system that it needn't schedule the main thread for any timeslices until the child thread is finished. This makes it lighter weight than other techniques, such as busywaiting (i.e. running a while
loop); while the main thread will still hold onto resources, it won't consume any CPU time.
See also When would I use Thread.Join? and c# Waiting for multiple threads to finish
While I do like this answer, since it discusses the correct way to join threads back to the main thread. It does not handle the problem of having a console app that cannot be controlled. Solutions for this are, as suggested the Windows service, or changing the main thread so it also reacts to user input.
– Ben Ootjers
Nov 21 '18 at 20:58
add a comment |
You need to join your threads before exiting.
static public void Main()
{
/*
Existing code goes here
*/
//Before exiting, make sure all child threads are finished
foreach (var thread in connectionThreads) thread.Join();
}
When your program calls Thread.Join, it is telling the operating system that it needn't schedule the main thread for any timeslices until the child thread is finished. This makes it lighter weight than other techniques, such as busywaiting (i.e. running a while
loop); while the main thread will still hold onto resources, it won't consume any CPU time.
See also When would I use Thread.Join? and c# Waiting for multiple threads to finish
You need to join your threads before exiting.
static public void Main()
{
/*
Existing code goes here
*/
//Before exiting, make sure all child threads are finished
foreach (var thread in connectionThreads) thread.Join();
}
When your program calls Thread.Join, it is telling the operating system that it needn't schedule the main thread for any timeslices until the child thread is finished. This makes it lighter weight than other techniques, such as busywaiting (i.e. running a while
loop); while the main thread will still hold onto resources, it won't consume any CPU time.
See also When would I use Thread.Join? and c# Waiting for multiple threads to finish
edited Nov 21 '18 at 19:31
answered Nov 21 '18 at 18:21
John WuJohn Wu
29.6k42652
29.6k42652
While I do like this answer, since it discusses the correct way to join threads back to the main thread. It does not handle the problem of having a console app that cannot be controlled. Solutions for this are, as suggested the Windows service, or changing the main thread so it also reacts to user input.
– Ben Ootjers
Nov 21 '18 at 20:58
add a comment |
While I do like this answer, since it discusses the correct way to join threads back to the main thread. It does not handle the problem of having a console app that cannot be controlled. Solutions for this are, as suggested the Windows service, or changing the main thread so it also reacts to user input.
– Ben Ootjers
Nov 21 '18 at 20:58
While I do like this answer, since it discusses the correct way to join threads back to the main thread. It does not handle the problem of having a console app that cannot be controlled. Solutions for this are, as suggested the Windows service, or changing the main thread so it also reacts to user input.
– Ben Ootjers
Nov 21 '18 at 20:58
While I do like this answer, since it discusses the correct way to join threads back to the main thread. It does not handle the problem of having a console app that cannot be controlled. Solutions for this are, as suggested the Windows service, or changing the main thread so it also reacts to user input.
– Ben Ootjers
Nov 21 '18 at 20:58
add a comment |
In general, best practice is for a console application to offer an "Enter any key to exit" prompt when the user wants to stop the application. But you can always query for a specific key-press to quit, such as 'q'.
Here is some code to get you started:
Console.WriteLine("Press any key to exit...");
Console.ReadKey();
add a comment |
In general, best practice is for a console application to offer an "Enter any key to exit" prompt when the user wants to stop the application. But you can always query for a specific key-press to quit, such as 'q'.
Here is some code to get you started:
Console.WriteLine("Press any key to exit...");
Console.ReadKey();
add a comment |
In general, best practice is for a console application to offer an "Enter any key to exit" prompt when the user wants to stop the application. But you can always query for a specific key-press to quit, such as 'q'.
Here is some code to get you started:
Console.WriteLine("Press any key to exit...");
Console.ReadKey();
In general, best practice is for a console application to offer an "Enter any key to exit" prompt when the user wants to stop the application. But you can always query for a specific key-press to quit, such as 'q'.
Here is some code to get you started:
Console.WriteLine("Press any key to exit...");
Console.ReadKey();
answered Nov 21 '18 at 18:02
benhorgenbenhorgen
1,1021725
1,1021725
add a comment |
add a comment |
Your code doesn't start new thread!
So, i made some changes in your code:
class Program
{
static public int minPort;
static public int maxPort;
//static int openPort = 0;
static byte sendData = new byte[1024];
static TcpListener listeners;
static Thread connectionThreads;
static void Main()
{
Console.Write("What do you want your minimum port to be? ");
minPort = Convert.ToInt32(Console.ReadLine());
Console.Write("What do you want your maximum port to be? ");
maxPort = Convert.ToInt32(Console.ReadLine());
//init
// ThreadStart streamThreadStart = new ThreadStart(DataStream(0));
//openPort = minPort;
listeners = new TcpListener[maxPort - minPort];
connectionThreads = new Thread[maxPort - minPort];
//for (int i = 0; i == maxPort - minPort; i++) it can't work
for (int i = 0; i < maxPort - minPort; i++)
{
listeners[i] = new TcpListener(IPAddress.Any, minPort + i);
connectionThreads[i] = new Thread(new ParameterizedThreadStart(DataStream)); //thread with start parameter
connectionThreads[i].Start(i); // start thread with index
Thread.Sleep(10);
}
}
static void DataStream(object o)
{
int index = (int)o; //get index
byte receiveData = new byte[1024];
listeners[index].Start(); // start listener with right index
Socket socket = listeners[index].AcceptSocket();
NetworkStream stream = new NetworkStream(socket);
while (true)
{
socket.Receive(receiveData);
Console.WriteLine("Received: " + BitConverter.ToString(receiveData));
socket.Send(parseCommand(receiveData));
}
}
static byte parseCommand(byte input)
{
string RCommand = BitConverter.ToString(input);
string SCommand;
if (RCommand == "get time")
{
SCommand = DateTime.Now.ToUniversalTime().ToString();
}
else
{
SCommand = "Unknown Command, please try again";
}
byte output = Encoding.ASCII.GetBytes(SCommand);
return output;
}
}
2
Please give a detailed description of what you have changed and why this makes it an answer.
– Poul Bak
Nov 21 '18 at 19:16
add a comment |
Your code doesn't start new thread!
So, i made some changes in your code:
class Program
{
static public int minPort;
static public int maxPort;
//static int openPort = 0;
static byte sendData = new byte[1024];
static TcpListener listeners;
static Thread connectionThreads;
static void Main()
{
Console.Write("What do you want your minimum port to be? ");
minPort = Convert.ToInt32(Console.ReadLine());
Console.Write("What do you want your maximum port to be? ");
maxPort = Convert.ToInt32(Console.ReadLine());
//init
// ThreadStart streamThreadStart = new ThreadStart(DataStream(0));
//openPort = minPort;
listeners = new TcpListener[maxPort - minPort];
connectionThreads = new Thread[maxPort - minPort];
//for (int i = 0; i == maxPort - minPort; i++) it can't work
for (int i = 0; i < maxPort - minPort; i++)
{
listeners[i] = new TcpListener(IPAddress.Any, minPort + i);
connectionThreads[i] = new Thread(new ParameterizedThreadStart(DataStream)); //thread with start parameter
connectionThreads[i].Start(i); // start thread with index
Thread.Sleep(10);
}
}
static void DataStream(object o)
{
int index = (int)o; //get index
byte receiveData = new byte[1024];
listeners[index].Start(); // start listener with right index
Socket socket = listeners[index].AcceptSocket();
NetworkStream stream = new NetworkStream(socket);
while (true)
{
socket.Receive(receiveData);
Console.WriteLine("Received: " + BitConverter.ToString(receiveData));
socket.Send(parseCommand(receiveData));
}
}
static byte parseCommand(byte input)
{
string RCommand = BitConverter.ToString(input);
string SCommand;
if (RCommand == "get time")
{
SCommand = DateTime.Now.ToUniversalTime().ToString();
}
else
{
SCommand = "Unknown Command, please try again";
}
byte output = Encoding.ASCII.GetBytes(SCommand);
return output;
}
}
2
Please give a detailed description of what you have changed and why this makes it an answer.
– Poul Bak
Nov 21 '18 at 19:16
add a comment |
Your code doesn't start new thread!
So, i made some changes in your code:
class Program
{
static public int minPort;
static public int maxPort;
//static int openPort = 0;
static byte sendData = new byte[1024];
static TcpListener listeners;
static Thread connectionThreads;
static void Main()
{
Console.Write("What do you want your minimum port to be? ");
minPort = Convert.ToInt32(Console.ReadLine());
Console.Write("What do you want your maximum port to be? ");
maxPort = Convert.ToInt32(Console.ReadLine());
//init
// ThreadStart streamThreadStart = new ThreadStart(DataStream(0));
//openPort = minPort;
listeners = new TcpListener[maxPort - minPort];
connectionThreads = new Thread[maxPort - minPort];
//for (int i = 0; i == maxPort - minPort; i++) it can't work
for (int i = 0; i < maxPort - minPort; i++)
{
listeners[i] = new TcpListener(IPAddress.Any, minPort + i);
connectionThreads[i] = new Thread(new ParameterizedThreadStart(DataStream)); //thread with start parameter
connectionThreads[i].Start(i); // start thread with index
Thread.Sleep(10);
}
}
static void DataStream(object o)
{
int index = (int)o; //get index
byte receiveData = new byte[1024];
listeners[index].Start(); // start listener with right index
Socket socket = listeners[index].AcceptSocket();
NetworkStream stream = new NetworkStream(socket);
while (true)
{
socket.Receive(receiveData);
Console.WriteLine("Received: " + BitConverter.ToString(receiveData));
socket.Send(parseCommand(receiveData));
}
}
static byte parseCommand(byte input)
{
string RCommand = BitConverter.ToString(input);
string SCommand;
if (RCommand == "get time")
{
SCommand = DateTime.Now.ToUniversalTime().ToString();
}
else
{
SCommand = "Unknown Command, please try again";
}
byte output = Encoding.ASCII.GetBytes(SCommand);
return output;
}
}
Your code doesn't start new thread!
So, i made some changes in your code:
class Program
{
static public int minPort;
static public int maxPort;
//static int openPort = 0;
static byte sendData = new byte[1024];
static TcpListener listeners;
static Thread connectionThreads;
static void Main()
{
Console.Write("What do you want your minimum port to be? ");
minPort = Convert.ToInt32(Console.ReadLine());
Console.Write("What do you want your maximum port to be? ");
maxPort = Convert.ToInt32(Console.ReadLine());
//init
// ThreadStart streamThreadStart = new ThreadStart(DataStream(0));
//openPort = minPort;
listeners = new TcpListener[maxPort - minPort];
connectionThreads = new Thread[maxPort - minPort];
//for (int i = 0; i == maxPort - minPort; i++) it can't work
for (int i = 0; i < maxPort - minPort; i++)
{
listeners[i] = new TcpListener(IPAddress.Any, minPort + i);
connectionThreads[i] = new Thread(new ParameterizedThreadStart(DataStream)); //thread with start parameter
connectionThreads[i].Start(i); // start thread with index
Thread.Sleep(10);
}
}
static void DataStream(object o)
{
int index = (int)o; //get index
byte receiveData = new byte[1024];
listeners[index].Start(); // start listener with right index
Socket socket = listeners[index].AcceptSocket();
NetworkStream stream = new NetworkStream(socket);
while (true)
{
socket.Receive(receiveData);
Console.WriteLine("Received: " + BitConverter.ToString(receiveData));
socket.Send(parseCommand(receiveData));
}
}
static byte parseCommand(byte input)
{
string RCommand = BitConverter.ToString(input);
string SCommand;
if (RCommand == "get time")
{
SCommand = DateTime.Now.ToUniversalTime().ToString();
}
else
{
SCommand = "Unknown Command, please try again";
}
byte output = Encoding.ASCII.GetBytes(SCommand);
return output;
}
}
answered Nov 21 '18 at 18:57


Michael HakenMichael Haken
1
1
2
Please give a detailed description of what you have changed and why this makes it an answer.
– Poul Bak
Nov 21 '18 at 19:16
add a comment |
2
Please give a detailed description of what you have changed and why this makes it an answer.
– Poul Bak
Nov 21 '18 at 19:16
2
2
Please give a detailed description of what you have changed and why this makes it an answer.
– Poul Bak
Nov 21 '18 at 19:16
Please give a detailed description of what you have changed and why this makes it an answer.
– Poul Bak
Nov 21 '18 at 19:16
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53417950%2fhow-can-i-keep-a-console-app-open-when-all-processes-are-running-on-separate-thr%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
fr9D rWlbZsq0PL
In a console application when main is complete, the application closes as there is no more code to execute. You will need to build a loop in main to keep the console application running.
– Ryan Wilson
Nov 21 '18 at 17:56
You know that one listener socket can service multiple clients and hand off the accepted sockets? There should never be a need to run listeners on multiple ports unless you're doing something very odd. E.g. common web servers only listen on ports 80 and/or 443. Do you think these servers dealing with thousands of clients only deal with a single client at a time? Lots of research/reading required here.
– Damien_The_Unbeliever
Nov 21 '18 at 18:06
Also, you have to pay attention to the return value from
Receive
and moral equivalents. Because all the TCP gives you is a stream of bytes, not messages. It doesn't guarantee to fill your buffer or that what you will receive matches a single buffer passed to a single call ofSend
at the other end.– Damien_The_Unbeliever
Nov 21 '18 at 18:11
Another option would be to create a Windows Service, which is designed to run long-term without closing.
– BJ Myers
Nov 21 '18 at 19:29