How to schedule python script to exit at given time
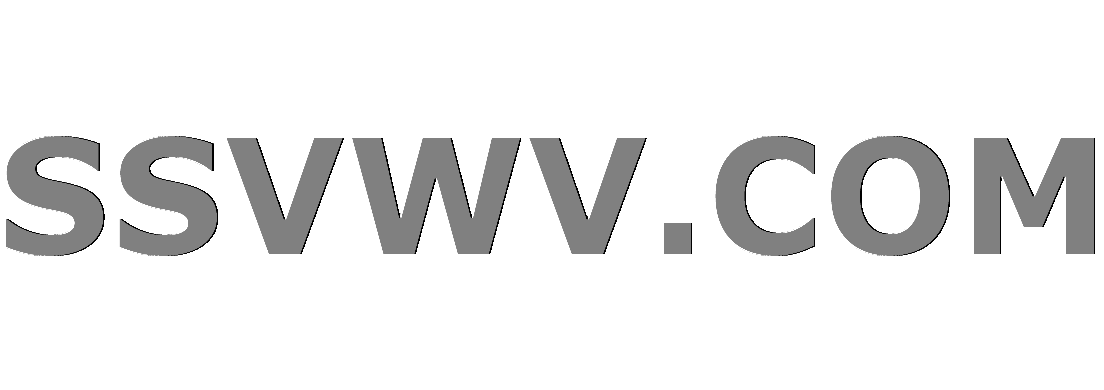
Multi tool use
I need to schedule a python script which can exit and kill it self at a given time. For scheduling, I am using python schedule
and below is the code:
import schedule
from threading import Thread
import time
import sys
def exit_data():
print("Exiting")
sys.exit()
def exit_data_thread():
schedule.every().day.at('13:20').do(exit_data)
while True:
schedule.run_pending()
time.sleep(1)
def main():
Thread(target=exit_data_thread).start()
while True:
time.sleep(1)
main()
Function exit_data()
runs at given time and it prints Exiting
but do not exit. It only prints Exiting
and then it keeps running. I have also used quit
instead of sys.exit()
. Please help. Thanks
python exit schedule
add a comment |
I need to schedule a python script which can exit and kill it self at a given time. For scheduling, I am using python schedule
and below is the code:
import schedule
from threading import Thread
import time
import sys
def exit_data():
print("Exiting")
sys.exit()
def exit_data_thread():
schedule.every().day.at('13:20').do(exit_data)
while True:
schedule.run_pending()
time.sleep(1)
def main():
Thread(target=exit_data_thread).start()
while True:
time.sleep(1)
main()
Function exit_data()
runs at given time and it prints Exiting
but do not exit. It only prints Exiting
and then it keeps running. I have also used quit
instead of sys.exit()
. Please help. Thanks
python exit schedule
How can you tell that the script is not exiting? Can you provide a bit more information on what's going on?
– Unsolved Cypher
Nov 22 '18 at 5:45
add a comment |
I need to schedule a python script which can exit and kill it self at a given time. For scheduling, I am using python schedule
and below is the code:
import schedule
from threading import Thread
import time
import sys
def exit_data():
print("Exiting")
sys.exit()
def exit_data_thread():
schedule.every().day.at('13:20').do(exit_data)
while True:
schedule.run_pending()
time.sleep(1)
def main():
Thread(target=exit_data_thread).start()
while True:
time.sleep(1)
main()
Function exit_data()
runs at given time and it prints Exiting
but do not exit. It only prints Exiting
and then it keeps running. I have also used quit
instead of sys.exit()
. Please help. Thanks
python exit schedule
I need to schedule a python script which can exit and kill it self at a given time. For scheduling, I am using python schedule
and below is the code:
import schedule
from threading import Thread
import time
import sys
def exit_data():
print("Exiting")
sys.exit()
def exit_data_thread():
schedule.every().day.at('13:20').do(exit_data)
while True:
schedule.run_pending()
time.sleep(1)
def main():
Thread(target=exit_data_thread).start()
while True:
time.sleep(1)
main()
Function exit_data()
runs at given time and it prints Exiting
but do not exit. It only prints Exiting
and then it keeps running. I have also used quit
instead of sys.exit()
. Please help. Thanks
python exit schedule
python exit schedule
edited Nov 22 '18 at 5:50
S Andrew
asked Nov 22 '18 at 5:41
S AndrewS Andrew
704937
704937
How can you tell that the script is not exiting? Can you provide a bit more information on what's going on?
– Unsolved Cypher
Nov 22 '18 at 5:45
add a comment |
How can you tell that the script is not exiting? Can you provide a bit more information on what's going on?
– Unsolved Cypher
Nov 22 '18 at 5:45
How can you tell that the script is not exiting? Can you provide a bit more information on what's going on?
– Unsolved Cypher
Nov 22 '18 at 5:45
How can you tell that the script is not exiting? Can you provide a bit more information on what's going on?
– Unsolved Cypher
Nov 22 '18 at 5:45
add a comment |
2 Answers
2
active
oldest
votes
Try to send signal to yourself :p
import schedule
from threading import Thread
import time
import sys
import os
import signal
def exit_data():
print("Exiting")
# sys.exit()
os.kill(os.getpid(), signal.SIGTERM)
def exit_data_thread():
schedule.every(3).seconds.do(exit_data)
while True:
schedule.run_pending()
time.sleep(1)
def main():
Thread(target=exit_data_thread).start()
while True:
time.sleep(1)
main()
add a comment |
To close the entire program within a thread, you can use os._exit()
. Calling sys.exit()
will only exit the thread, not the entire program.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53424532%2fhow-to-schedule-python-script-to-exit-at-given-time%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Try to send signal to yourself :p
import schedule
from threading import Thread
import time
import sys
import os
import signal
def exit_data():
print("Exiting")
# sys.exit()
os.kill(os.getpid(), signal.SIGTERM)
def exit_data_thread():
schedule.every(3).seconds.do(exit_data)
while True:
schedule.run_pending()
time.sleep(1)
def main():
Thread(target=exit_data_thread).start()
while True:
time.sleep(1)
main()
add a comment |
Try to send signal to yourself :p
import schedule
from threading import Thread
import time
import sys
import os
import signal
def exit_data():
print("Exiting")
# sys.exit()
os.kill(os.getpid(), signal.SIGTERM)
def exit_data_thread():
schedule.every(3).seconds.do(exit_data)
while True:
schedule.run_pending()
time.sleep(1)
def main():
Thread(target=exit_data_thread).start()
while True:
time.sleep(1)
main()
add a comment |
Try to send signal to yourself :p
import schedule
from threading import Thread
import time
import sys
import os
import signal
def exit_data():
print("Exiting")
# sys.exit()
os.kill(os.getpid(), signal.SIGTERM)
def exit_data_thread():
schedule.every(3).seconds.do(exit_data)
while True:
schedule.run_pending()
time.sleep(1)
def main():
Thread(target=exit_data_thread).start()
while True:
time.sleep(1)
main()
Try to send signal to yourself :p
import schedule
from threading import Thread
import time
import sys
import os
import signal
def exit_data():
print("Exiting")
# sys.exit()
os.kill(os.getpid(), signal.SIGTERM)
def exit_data_thread():
schedule.every(3).seconds.do(exit_data)
while True:
schedule.run_pending()
time.sleep(1)
def main():
Thread(target=exit_data_thread).start()
while True:
time.sleep(1)
main()
answered Nov 22 '18 at 5:52
suplsupl
895
895
add a comment |
add a comment |
To close the entire program within a thread, you can use os._exit()
. Calling sys.exit()
will only exit the thread, not the entire program.
add a comment |
To close the entire program within a thread, you can use os._exit()
. Calling sys.exit()
will only exit the thread, not the entire program.
add a comment |
To close the entire program within a thread, you can use os._exit()
. Calling sys.exit()
will only exit the thread, not the entire program.
To close the entire program within a thread, you can use os._exit()
. Calling sys.exit()
will only exit the thread, not the entire program.
answered Nov 22 '18 at 5:57
Unsolved CypherUnsolved Cypher
495314
495314
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53424532%2fhow-to-schedule-python-script-to-exit-at-given-time%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
FAlsYEkmJg PxBh R2RzFXnNV5kK4Q MN,PsotCZ5yDGOdq,siN7,w3ga3Fr
How can you tell that the script is not exiting? Can you provide a bit more information on what's going on?
– Unsolved Cypher
Nov 22 '18 at 5:45