Java getting the Enum name given the Enum Value
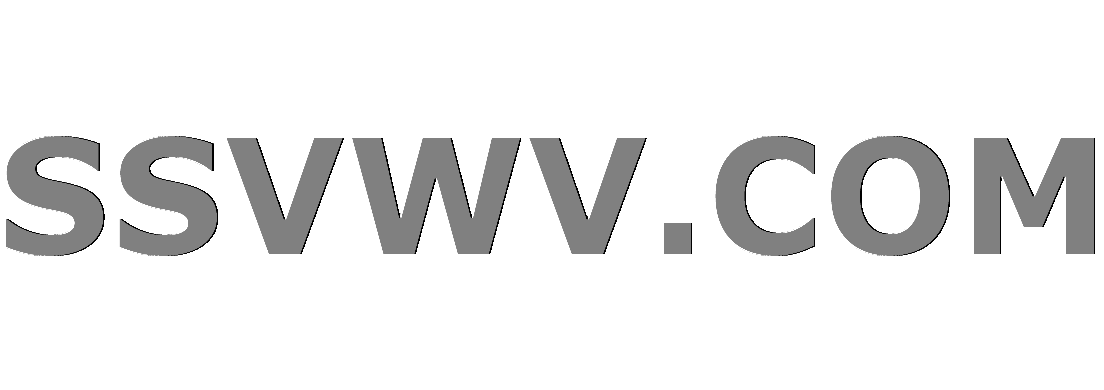
Multi tool use
How can I get the name of a Java Enum type given its value?
I have the following code which works for a particular Enum type, can I make it more generic?
public enum Category {
APPLE("3"),
ORANGE("1"),
private final String identifier;
private Category(String identifier) {
this.identifier = identifier;
}
public String toString() {
return identifier;
}
public static String getEnumNameForValue(Object value){
Category values = Category.values();
String enumValue = null;
for(Category eachValue : values) {
enumValue = eachValue.toString();
if (enumValue.equalsIgnoreCase(value)) {
return eachValue.name();
}
}
return enumValue;
}
}
java enums enumeration
add a comment |
How can I get the name of a Java Enum type given its value?
I have the following code which works for a particular Enum type, can I make it more generic?
public enum Category {
APPLE("3"),
ORANGE("1"),
private final String identifier;
private Category(String identifier) {
this.identifier = identifier;
}
public String toString() {
return identifier;
}
public static String getEnumNameForValue(Object value){
Category values = Category.values();
String enumValue = null;
for(Category eachValue : values) {
enumValue = eachValue.toString();
if (enumValue.equalsIgnoreCase(value)) {
return eachValue.name();
}
}
return enumValue;
}
}
java enums enumeration
2
Is there a special reason why you want to use something other than the name() of the Enum for looking them up? That would confuse a lot of people, and you also cannot use a simpleCategory.valueOf(name)
.
– Thilo
Oct 8 '10 at 9:36
Really can we make it more generic? I am using a lot ofnameOf(String name)
, now for each I will write a*Enum.values().stream().filter(...).findAny().get()
, which is so annoying.
– Hearen
May 18 '18 at 12:33
add a comment |
How can I get the name of a Java Enum type given its value?
I have the following code which works for a particular Enum type, can I make it more generic?
public enum Category {
APPLE("3"),
ORANGE("1"),
private final String identifier;
private Category(String identifier) {
this.identifier = identifier;
}
public String toString() {
return identifier;
}
public static String getEnumNameForValue(Object value){
Category values = Category.values();
String enumValue = null;
for(Category eachValue : values) {
enumValue = eachValue.toString();
if (enumValue.equalsIgnoreCase(value)) {
return eachValue.name();
}
}
return enumValue;
}
}
java enums enumeration
How can I get the name of a Java Enum type given its value?
I have the following code which works for a particular Enum type, can I make it more generic?
public enum Category {
APPLE("3"),
ORANGE("1"),
private final String identifier;
private Category(String identifier) {
this.identifier = identifier;
}
public String toString() {
return identifier;
}
public static String getEnumNameForValue(Object value){
Category values = Category.values();
String enumValue = null;
for(Category eachValue : values) {
enumValue = eachValue.toString();
if (enumValue.equalsIgnoreCase(value)) {
return eachValue.name();
}
}
return enumValue;
}
}
java enums enumeration
java enums enumeration
edited May 18 '18 at 13:05
Hearen
2,8051428
2,8051428
asked Oct 8 '10 at 9:23
JuliaJulia
221134
221134
2
Is there a special reason why you want to use something other than the name() of the Enum for looking them up? That would confuse a lot of people, and you also cannot use a simpleCategory.valueOf(name)
.
– Thilo
Oct 8 '10 at 9:36
Really can we make it more generic? I am using a lot ofnameOf(String name)
, now for each I will write a*Enum.values().stream().filter(...).findAny().get()
, which is so annoying.
– Hearen
May 18 '18 at 12:33
add a comment |
2
Is there a special reason why you want to use something other than the name() of the Enum for looking them up? That would confuse a lot of people, and you also cannot use a simpleCategory.valueOf(name)
.
– Thilo
Oct 8 '10 at 9:36
Really can we make it more generic? I am using a lot ofnameOf(String name)
, now for each I will write a*Enum.values().stream().filter(...).findAny().get()
, which is so annoying.
– Hearen
May 18 '18 at 12:33
2
2
Is there a special reason why you want to use something other than the name() of the Enum for looking them up? That would confuse a lot of people, and you also cannot use a simple
Category.valueOf(name)
.– Thilo
Oct 8 '10 at 9:36
Is there a special reason why you want to use something other than the name() of the Enum for looking them up? That would confuse a lot of people, and you also cannot use a simple
Category.valueOf(name)
.– Thilo
Oct 8 '10 at 9:36
Really can we make it more generic? I am using a lot of
nameOf(String name)
, now for each I will write a *Enum.values().stream().filter(...).findAny().get()
, which is so annoying.– Hearen
May 18 '18 at 12:33
Really can we make it more generic? I am using a lot of
nameOf(String name)
, now for each I will write a *Enum.values().stream().filter(...).findAny().get()
, which is so annoying.– Hearen
May 18 '18 at 12:33
add a comment |
4 Answers
4
active
oldest
votes
You should replace your getEnumNameForValue
by a call to the name()
method.
1
@Jukia: Also, consider overridingtoString()
: download.oracle.com/javase/6/docs/api/java/lang/…
– trashgod
Oct 8 '10 at 11:58
5
@trashgod: She is overriding toString
– Thilo
Oct 9 '10 at 1:43
@Thilo: My error; thanks. @Julia: Sorry about misspelling your name.
– trashgod
Oct 9 '10 at 1:54
add a comment |
Try below code
public enum SalaryHeadMasterEnum {
BASIC_PAY("basic pay"),
MEDICAL_ALLOWANCE("Medical Allowance");
private String name;
private SalaryHeadMasterEnum(String stringVal) {
name=stringVal;
}
public String toString(){
return name;
}
public static String getEnumByString(String code){
for(SalaryHeadMasterEnum e : SalaryHeadMasterEnum.values()){
if(code == e.name) return e.name();
}
return null;
}
}
Now you can use below code to retrieve the Enum by Value
SalaryHeadMasterEnum.getEnumByString("Basic Pay")
Use Below code to get ENUM as String
SalaryHeadMasterEnum.BASIC_PAY.name()
Use below code to get string Value for enum
SalaryHeadMasterEnum.BASIC_PAY.toString()
3
code == e.name
is not going to work the way you meant it to, should bee.name.equals(code)
instead
– f_puras
Nov 22 '16 at 14:40
@f_puras did you test the above code??
– prashant thakre
Nov 22 '16 at 15:40
4
Well, yes:SalaryHeadMasterEnum.getEnumByString("basic pay")
does work, but relies on the JVM merging String literals. Stuff likeSalaryHeadMasterEnum.getEnumByString(new StringBuilder("basic ").append("pay").toString())
does not, but should IMHO. UsingString.equals()
would make both work ;-)
– f_puras
Nov 22 '16 at 21:46
1
- for comparing strings with == which is bad and for using a variable 'name' with a different meaning than the 'name()' method
– Hardcoded
Apr 27 '17 at 15:09
add a comment |
Try, the following code..
@Override
public String toString() {
return this.name();
}
This should work well, but why call the functiontoString()
instead ofgetName()
(Or something similar) ?
– Mayuso
Oct 20 '16 at 11:15
add a comment |
Here is the below code, it will return the Enum name from Enum value.
public enum Test {
PLUS("Plus One"), MINUS("MinusTwo"), TIMES("MultiplyByFour"), DIVIDE(
"DivideByZero");
private String operationName;
private Test(final String operationName) {
setOperationName(operationName);
}
public String getOperationName() {
return operationName;
}
public void setOperationName(final String operationName) {
this.operationName = operationName;
}
public static Test getOperationName(final String operationName) {
for (Test oprname : Test.values()) {
if (operationName.equals(oprname.toString())) {
return oprname;
}
}
return null;
}
@Override
public String toString() {
return operationName;
}
}
public class Main {
public static void main(String args) {
Test test = Test.getOperationName("Plus One");
switch (test) {
case PLUS:
System.out.println("Plus.....");
break;
case MINUS:
System.out.println("Minus.....");
break;
default:
System.out.println("Nothing..");
break;
}
}
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f3889248%2fjava-getting-the-enum-name-given-the-enum-value%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
You should replace your getEnumNameForValue
by a call to the name()
method.
1
@Jukia: Also, consider overridingtoString()
: download.oracle.com/javase/6/docs/api/java/lang/…
– trashgod
Oct 8 '10 at 11:58
5
@trashgod: She is overriding toString
– Thilo
Oct 9 '10 at 1:43
@Thilo: My error; thanks. @Julia: Sorry about misspelling your name.
– trashgod
Oct 9 '10 at 1:54
add a comment |
You should replace your getEnumNameForValue
by a call to the name()
method.
1
@Jukia: Also, consider overridingtoString()
: download.oracle.com/javase/6/docs/api/java/lang/…
– trashgod
Oct 8 '10 at 11:58
5
@trashgod: She is overriding toString
– Thilo
Oct 9 '10 at 1:43
@Thilo: My error; thanks. @Julia: Sorry about misspelling your name.
– trashgod
Oct 9 '10 at 1:54
add a comment |
You should replace your getEnumNameForValue
by a call to the name()
method.
You should replace your getEnumNameForValue
by a call to the name()
method.
edited Oct 15 '13 at 15:47
thSoft
15.6k57593
15.6k57593
answered Oct 8 '10 at 9:26
RiduidelRiduidel
18k1160134
18k1160134
1
@Jukia: Also, consider overridingtoString()
: download.oracle.com/javase/6/docs/api/java/lang/…
– trashgod
Oct 8 '10 at 11:58
5
@trashgod: She is overriding toString
– Thilo
Oct 9 '10 at 1:43
@Thilo: My error; thanks. @Julia: Sorry about misspelling your name.
– trashgod
Oct 9 '10 at 1:54
add a comment |
1
@Jukia: Also, consider overridingtoString()
: download.oracle.com/javase/6/docs/api/java/lang/…
– trashgod
Oct 8 '10 at 11:58
5
@trashgod: She is overriding toString
– Thilo
Oct 9 '10 at 1:43
@Thilo: My error; thanks. @Julia: Sorry about misspelling your name.
– trashgod
Oct 9 '10 at 1:54
1
1
@Jukia: Also, consider overriding
toString()
: download.oracle.com/javase/6/docs/api/java/lang/…– trashgod
Oct 8 '10 at 11:58
@Jukia: Also, consider overriding
toString()
: download.oracle.com/javase/6/docs/api/java/lang/…– trashgod
Oct 8 '10 at 11:58
5
5
@trashgod: She is overriding toString
– Thilo
Oct 9 '10 at 1:43
@trashgod: She is overriding toString
– Thilo
Oct 9 '10 at 1:43
@Thilo: My error; thanks. @Julia: Sorry about misspelling your name.
– trashgod
Oct 9 '10 at 1:54
@Thilo: My error; thanks. @Julia: Sorry about misspelling your name.
– trashgod
Oct 9 '10 at 1:54
add a comment |
Try below code
public enum SalaryHeadMasterEnum {
BASIC_PAY("basic pay"),
MEDICAL_ALLOWANCE("Medical Allowance");
private String name;
private SalaryHeadMasterEnum(String stringVal) {
name=stringVal;
}
public String toString(){
return name;
}
public static String getEnumByString(String code){
for(SalaryHeadMasterEnum e : SalaryHeadMasterEnum.values()){
if(code == e.name) return e.name();
}
return null;
}
}
Now you can use below code to retrieve the Enum by Value
SalaryHeadMasterEnum.getEnumByString("Basic Pay")
Use Below code to get ENUM as String
SalaryHeadMasterEnum.BASIC_PAY.name()
Use below code to get string Value for enum
SalaryHeadMasterEnum.BASIC_PAY.toString()
3
code == e.name
is not going to work the way you meant it to, should bee.name.equals(code)
instead
– f_puras
Nov 22 '16 at 14:40
@f_puras did you test the above code??
– prashant thakre
Nov 22 '16 at 15:40
4
Well, yes:SalaryHeadMasterEnum.getEnumByString("basic pay")
does work, but relies on the JVM merging String literals. Stuff likeSalaryHeadMasterEnum.getEnumByString(new StringBuilder("basic ").append("pay").toString())
does not, but should IMHO. UsingString.equals()
would make both work ;-)
– f_puras
Nov 22 '16 at 21:46
1
- for comparing strings with == which is bad and for using a variable 'name' with a different meaning than the 'name()' method
– Hardcoded
Apr 27 '17 at 15:09
add a comment |
Try below code
public enum SalaryHeadMasterEnum {
BASIC_PAY("basic pay"),
MEDICAL_ALLOWANCE("Medical Allowance");
private String name;
private SalaryHeadMasterEnum(String stringVal) {
name=stringVal;
}
public String toString(){
return name;
}
public static String getEnumByString(String code){
for(SalaryHeadMasterEnum e : SalaryHeadMasterEnum.values()){
if(code == e.name) return e.name();
}
return null;
}
}
Now you can use below code to retrieve the Enum by Value
SalaryHeadMasterEnum.getEnumByString("Basic Pay")
Use Below code to get ENUM as String
SalaryHeadMasterEnum.BASIC_PAY.name()
Use below code to get string Value for enum
SalaryHeadMasterEnum.BASIC_PAY.toString()
3
code == e.name
is not going to work the way you meant it to, should bee.name.equals(code)
instead
– f_puras
Nov 22 '16 at 14:40
@f_puras did you test the above code??
– prashant thakre
Nov 22 '16 at 15:40
4
Well, yes:SalaryHeadMasterEnum.getEnumByString("basic pay")
does work, but relies on the JVM merging String literals. Stuff likeSalaryHeadMasterEnum.getEnumByString(new StringBuilder("basic ").append("pay").toString())
does not, but should IMHO. UsingString.equals()
would make both work ;-)
– f_puras
Nov 22 '16 at 21:46
1
- for comparing strings with == which is bad and for using a variable 'name' with a different meaning than the 'name()' method
– Hardcoded
Apr 27 '17 at 15:09
add a comment |
Try below code
public enum SalaryHeadMasterEnum {
BASIC_PAY("basic pay"),
MEDICAL_ALLOWANCE("Medical Allowance");
private String name;
private SalaryHeadMasterEnum(String stringVal) {
name=stringVal;
}
public String toString(){
return name;
}
public static String getEnumByString(String code){
for(SalaryHeadMasterEnum e : SalaryHeadMasterEnum.values()){
if(code == e.name) return e.name();
}
return null;
}
}
Now you can use below code to retrieve the Enum by Value
SalaryHeadMasterEnum.getEnumByString("Basic Pay")
Use Below code to get ENUM as String
SalaryHeadMasterEnum.BASIC_PAY.name()
Use below code to get string Value for enum
SalaryHeadMasterEnum.BASIC_PAY.toString()
Try below code
public enum SalaryHeadMasterEnum {
BASIC_PAY("basic pay"),
MEDICAL_ALLOWANCE("Medical Allowance");
private String name;
private SalaryHeadMasterEnum(String stringVal) {
name=stringVal;
}
public String toString(){
return name;
}
public static String getEnumByString(String code){
for(SalaryHeadMasterEnum e : SalaryHeadMasterEnum.values()){
if(code == e.name) return e.name();
}
return null;
}
}
Now you can use below code to retrieve the Enum by Value
SalaryHeadMasterEnum.getEnumByString("Basic Pay")
Use Below code to get ENUM as String
SalaryHeadMasterEnum.BASIC_PAY.name()
Use below code to get string Value for enum
SalaryHeadMasterEnum.BASIC_PAY.toString()
edited Jun 30 '16 at 13:47
Riduidel
18k1160134
18k1160134
answered Jun 15 '13 at 9:43
prashant thakreprashant thakre
3,50911934
3,50911934
3
code == e.name
is not going to work the way you meant it to, should bee.name.equals(code)
instead
– f_puras
Nov 22 '16 at 14:40
@f_puras did you test the above code??
– prashant thakre
Nov 22 '16 at 15:40
4
Well, yes:SalaryHeadMasterEnum.getEnumByString("basic pay")
does work, but relies on the JVM merging String literals. Stuff likeSalaryHeadMasterEnum.getEnumByString(new StringBuilder("basic ").append("pay").toString())
does not, but should IMHO. UsingString.equals()
would make both work ;-)
– f_puras
Nov 22 '16 at 21:46
1
- for comparing strings with == which is bad and for using a variable 'name' with a different meaning than the 'name()' method
– Hardcoded
Apr 27 '17 at 15:09
add a comment |
3
code == e.name
is not going to work the way you meant it to, should bee.name.equals(code)
instead
– f_puras
Nov 22 '16 at 14:40
@f_puras did you test the above code??
– prashant thakre
Nov 22 '16 at 15:40
4
Well, yes:SalaryHeadMasterEnum.getEnumByString("basic pay")
does work, but relies on the JVM merging String literals. Stuff likeSalaryHeadMasterEnum.getEnumByString(new StringBuilder("basic ").append("pay").toString())
does not, but should IMHO. UsingString.equals()
would make both work ;-)
– f_puras
Nov 22 '16 at 21:46
1
- for comparing strings with == which is bad and for using a variable 'name' with a different meaning than the 'name()' method
– Hardcoded
Apr 27 '17 at 15:09
3
3
code == e.name
is not going to work the way you meant it to, should be e.name.equals(code)
instead– f_puras
Nov 22 '16 at 14:40
code == e.name
is not going to work the way you meant it to, should be e.name.equals(code)
instead– f_puras
Nov 22 '16 at 14:40
@f_puras did you test the above code??
– prashant thakre
Nov 22 '16 at 15:40
@f_puras did you test the above code??
– prashant thakre
Nov 22 '16 at 15:40
4
4
Well, yes:
SalaryHeadMasterEnum.getEnumByString("basic pay")
does work, but relies on the JVM merging String literals. Stuff like SalaryHeadMasterEnum.getEnumByString(new StringBuilder("basic ").append("pay").toString())
does not, but should IMHO. Using String.equals()
would make both work ;-)– f_puras
Nov 22 '16 at 21:46
Well, yes:
SalaryHeadMasterEnum.getEnumByString("basic pay")
does work, but relies on the JVM merging String literals. Stuff like SalaryHeadMasterEnum.getEnumByString(new StringBuilder("basic ").append("pay").toString())
does not, but should IMHO. Using String.equals()
would make both work ;-)– f_puras
Nov 22 '16 at 21:46
1
1
- for comparing strings with == which is bad and for using a variable 'name' with a different meaning than the 'name()' method
– Hardcoded
Apr 27 '17 at 15:09
- for comparing strings with == which is bad and for using a variable 'name' with a different meaning than the 'name()' method
– Hardcoded
Apr 27 '17 at 15:09
add a comment |
Try, the following code..
@Override
public String toString() {
return this.name();
}
This should work well, but why call the functiontoString()
instead ofgetName()
(Or something similar) ?
– Mayuso
Oct 20 '16 at 11:15
add a comment |
Try, the following code..
@Override
public String toString() {
return this.name();
}
This should work well, but why call the functiontoString()
instead ofgetName()
(Or something similar) ?
– Mayuso
Oct 20 '16 at 11:15
add a comment |
Try, the following code..
@Override
public String toString() {
return this.name();
}
Try, the following code..
@Override
public String toString() {
return this.name();
}
answered Oct 20 '16 at 11:07


JavaciJavaci
6113
6113
This should work well, but why call the functiontoString()
instead ofgetName()
(Or something similar) ?
– Mayuso
Oct 20 '16 at 11:15
add a comment |
This should work well, but why call the functiontoString()
instead ofgetName()
(Or something similar) ?
– Mayuso
Oct 20 '16 at 11:15
This should work well, but why call the function
toString()
instead of getName()
(Or something similar) ?– Mayuso
Oct 20 '16 at 11:15
This should work well, but why call the function
toString()
instead of getName()
(Or something similar) ?– Mayuso
Oct 20 '16 at 11:15
add a comment |
Here is the below code, it will return the Enum name from Enum value.
public enum Test {
PLUS("Plus One"), MINUS("MinusTwo"), TIMES("MultiplyByFour"), DIVIDE(
"DivideByZero");
private String operationName;
private Test(final String operationName) {
setOperationName(operationName);
}
public String getOperationName() {
return operationName;
}
public void setOperationName(final String operationName) {
this.operationName = operationName;
}
public static Test getOperationName(final String operationName) {
for (Test oprname : Test.values()) {
if (operationName.equals(oprname.toString())) {
return oprname;
}
}
return null;
}
@Override
public String toString() {
return operationName;
}
}
public class Main {
public static void main(String args) {
Test test = Test.getOperationName("Plus One");
switch (test) {
case PLUS:
System.out.println("Plus.....");
break;
case MINUS:
System.out.println("Minus.....");
break;
default:
System.out.println("Nothing..");
break;
}
}
}
add a comment |
Here is the below code, it will return the Enum name from Enum value.
public enum Test {
PLUS("Plus One"), MINUS("MinusTwo"), TIMES("MultiplyByFour"), DIVIDE(
"DivideByZero");
private String operationName;
private Test(final String operationName) {
setOperationName(operationName);
}
public String getOperationName() {
return operationName;
}
public void setOperationName(final String operationName) {
this.operationName = operationName;
}
public static Test getOperationName(final String operationName) {
for (Test oprname : Test.values()) {
if (operationName.equals(oprname.toString())) {
return oprname;
}
}
return null;
}
@Override
public String toString() {
return operationName;
}
}
public class Main {
public static void main(String args) {
Test test = Test.getOperationName("Plus One");
switch (test) {
case PLUS:
System.out.println("Plus.....");
break;
case MINUS:
System.out.println("Minus.....");
break;
default:
System.out.println("Nothing..");
break;
}
}
}
add a comment |
Here is the below code, it will return the Enum name from Enum value.
public enum Test {
PLUS("Plus One"), MINUS("MinusTwo"), TIMES("MultiplyByFour"), DIVIDE(
"DivideByZero");
private String operationName;
private Test(final String operationName) {
setOperationName(operationName);
}
public String getOperationName() {
return operationName;
}
public void setOperationName(final String operationName) {
this.operationName = operationName;
}
public static Test getOperationName(final String operationName) {
for (Test oprname : Test.values()) {
if (operationName.equals(oprname.toString())) {
return oprname;
}
}
return null;
}
@Override
public String toString() {
return operationName;
}
}
public class Main {
public static void main(String args) {
Test test = Test.getOperationName("Plus One");
switch (test) {
case PLUS:
System.out.println("Plus.....");
break;
case MINUS:
System.out.println("Minus.....");
break;
default:
System.out.println("Nothing..");
break;
}
}
}
Here is the below code, it will return the Enum name from Enum value.
public enum Test {
PLUS("Plus One"), MINUS("MinusTwo"), TIMES("MultiplyByFour"), DIVIDE(
"DivideByZero");
private String operationName;
private Test(final String operationName) {
setOperationName(operationName);
}
public String getOperationName() {
return operationName;
}
public void setOperationName(final String operationName) {
this.operationName = operationName;
}
public static Test getOperationName(final String operationName) {
for (Test oprname : Test.values()) {
if (operationName.equals(oprname.toString())) {
return oprname;
}
}
return null;
}
@Override
public String toString() {
return operationName;
}
}
public class Main {
public static void main(String args) {
Test test = Test.getOperationName("Plus One");
switch (test) {
case PLUS:
System.out.println("Plus.....");
break;
case MINUS:
System.out.println("Minus.....");
break;
default:
System.out.println("Nothing..");
break;
}
}
}
edited Sep 8 '17 at 8:28


Fejs
1,97111226
1,97111226
answered Sep 8 '17 at 7:28
Do ItDo It
53215
53215
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f3889248%2fjava-getting-the-enum-name-given-the-enum-value%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
3NZ3YA,E8XO E7Zbl4 QUZLEY4qsAfc5yE6D,eMFkkiWa,rmYZHPE w871w2HoxY lxlC1i9W8R4fmOj4 TG5qE Q81Os2PBpy3SUAqX
2
Is there a special reason why you want to use something other than the name() of the Enum for looking them up? That would confuse a lot of people, and you also cannot use a simple
Category.valueOf(name)
.– Thilo
Oct 8 '10 at 9:36
Really can we make it more generic? I am using a lot of
nameOf(String name)
, now for each I will write a*Enum.values().stream().filter(...).findAny().get()
, which is so annoying.– Hearen
May 18 '18 at 12:33