Why does my function return None?
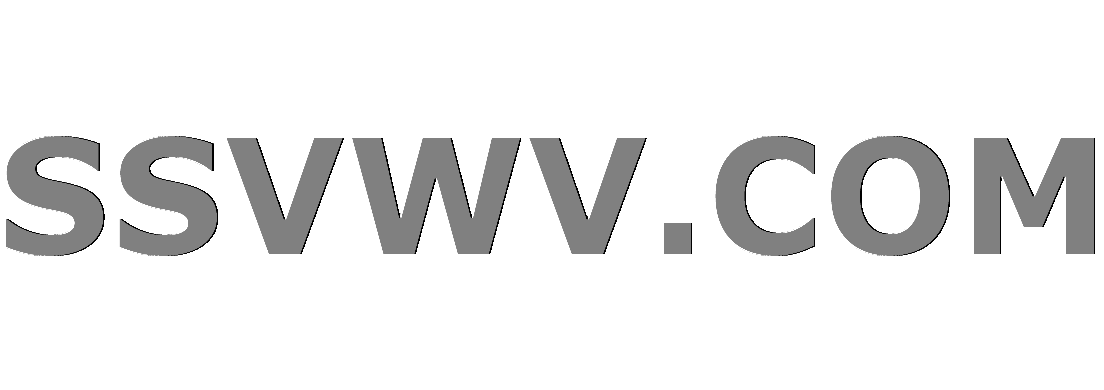
Multi tool use
This may be an easy question to answer, but I can't get this simple program to work and it's driving me crazy. I have this piece of code:
def Dat_Function():
my_var = raw_input("Type "a" or "b": ")
if my_var != "a" and my_var != "b":
print "You didn't type "a" or "b". Try again."
print " "
Dat_Function()
else:
print my_var, "-from Dat_Function"
return my_var
def main():
print Dat_Function(), "-From main()"
main()
Now, if I input just "a" or "b",everything is fine. The output is:
Type "a" or "b": a
a -from Dat_Function
a -From main()
But, if I type something else and then "a" or "b", I get this:
Type "a" or "b": purple
You didn't type "a" or "b". Try again.
Type "a" or "b": a
a -from Dat_Function
None -From main()
I don't know why Dat_Function()
is returning None
, since it should only return my_var
. The print statement shows that my_var
is the correct value, but the function doesn't return that value for some reason.
python function recursion return
add a comment |
This may be an easy question to answer, but I can't get this simple program to work and it's driving me crazy. I have this piece of code:
def Dat_Function():
my_var = raw_input("Type "a" or "b": ")
if my_var != "a" and my_var != "b":
print "You didn't type "a" or "b". Try again."
print " "
Dat_Function()
else:
print my_var, "-from Dat_Function"
return my_var
def main():
print Dat_Function(), "-From main()"
main()
Now, if I input just "a" or "b",everything is fine. The output is:
Type "a" or "b": a
a -from Dat_Function
a -From main()
But, if I type something else and then "a" or "b", I get this:
Type "a" or "b": purple
You didn't type "a" or "b". Try again.
Type "a" or "b": a
a -from Dat_Function
None -From main()
I don't know why Dat_Function()
is returning None
, since it should only return my_var
. The print statement shows that my_var
is the correct value, but the function doesn't return that value for some reason.
python function recursion return
7
You need to doreturn Dat_Function()
when calling it recursively.
– Gustav Larsson
Jul 22 '13 at 0:31
2
Just a tip: The idiomatic way of thatmy_var != "a" and my_var != "b"
condition would bemy_var not in ('a', 'b')
– gonz
May 18 '16 at 1:04
add a comment |
This may be an easy question to answer, but I can't get this simple program to work and it's driving me crazy. I have this piece of code:
def Dat_Function():
my_var = raw_input("Type "a" or "b": ")
if my_var != "a" and my_var != "b":
print "You didn't type "a" or "b". Try again."
print " "
Dat_Function()
else:
print my_var, "-from Dat_Function"
return my_var
def main():
print Dat_Function(), "-From main()"
main()
Now, if I input just "a" or "b",everything is fine. The output is:
Type "a" or "b": a
a -from Dat_Function
a -From main()
But, if I type something else and then "a" or "b", I get this:
Type "a" or "b": purple
You didn't type "a" or "b". Try again.
Type "a" or "b": a
a -from Dat_Function
None -From main()
I don't know why Dat_Function()
is returning None
, since it should only return my_var
. The print statement shows that my_var
is the correct value, but the function doesn't return that value for some reason.
python function recursion return
This may be an easy question to answer, but I can't get this simple program to work and it's driving me crazy. I have this piece of code:
def Dat_Function():
my_var = raw_input("Type "a" or "b": ")
if my_var != "a" and my_var != "b":
print "You didn't type "a" or "b". Try again."
print " "
Dat_Function()
else:
print my_var, "-from Dat_Function"
return my_var
def main():
print Dat_Function(), "-From main()"
main()
Now, if I input just "a" or "b",everything is fine. The output is:
Type "a" or "b": a
a -from Dat_Function
a -From main()
But, if I type something else and then "a" or "b", I get this:
Type "a" or "b": purple
You didn't type "a" or "b". Try again.
Type "a" or "b": a
a -from Dat_Function
None -From main()
I don't know why Dat_Function()
is returning None
, since it should only return my_var
. The print statement shows that my_var
is the correct value, but the function doesn't return that value for some reason.
python function recursion return
python function recursion return
edited Apr 11 at 0:01


Aran-Fey
20.6k53368
20.6k53368
asked Jul 22 '13 at 0:29
Cate
182127
182127
7
You need to doreturn Dat_Function()
when calling it recursively.
– Gustav Larsson
Jul 22 '13 at 0:31
2
Just a tip: The idiomatic way of thatmy_var != "a" and my_var != "b"
condition would bemy_var not in ('a', 'b')
– gonz
May 18 '16 at 1:04
add a comment |
7
You need to doreturn Dat_Function()
when calling it recursively.
– Gustav Larsson
Jul 22 '13 at 0:31
2
Just a tip: The idiomatic way of thatmy_var != "a" and my_var != "b"
condition would bemy_var not in ('a', 'b')
– gonz
May 18 '16 at 1:04
7
7
You need to do
return Dat_Function()
when calling it recursively.– Gustav Larsson
Jul 22 '13 at 0:31
You need to do
return Dat_Function()
when calling it recursively.– Gustav Larsson
Jul 22 '13 at 0:31
2
2
Just a tip: The idiomatic way of that
my_var != "a" and my_var != "b"
condition would be my_var not in ('a', 'b')
– gonz
May 18 '16 at 1:04
Just a tip: The idiomatic way of that
my_var != "a" and my_var != "b"
condition would be my_var not in ('a', 'b')
– gonz
May 18 '16 at 1:04
add a comment |
4 Answers
4
active
oldest
votes
It is returning None
because when you recursively call it:
if my_var != "a" and my_var != "b":
print "You didn't type "a" or "b". Try again."
print " "
Dat_Function()
..you don't return the value.
So while the recursion does happen, the return value gets discarded, and then you fall off the end of the function. Falling off the end of the function means that python implicitly returns None
, just like this:
>>> def f(x):
... pass
>>> print(f(20))
None
So, instead of just calling Dat Function()
in your if
statement, you need to return
it.
Shouldn't it run through the if statement again if it is called recursively? I don't understand why it wouldn't return a value.
– Cate
Jul 22 '13 at 0:35
Nope. See my edit. The recursion happens, and then you discard what the recursion returns.
– roippi
Jul 22 '13 at 0:38
So if you call a function from inside that same function the return value gets discarded, but you return the same function in that function you really just call it inmain()
?
– Cate
Jul 22 '13 at 0:49
1
You lost me with thatmain()
bit... You can fail as many times as you want to, the one that "succeeds" will returnmy_var
, which will get passed down (return
ed) through all of the recursive calls all the way down to the original caller. Which, yes, ismain()
.
– roippi
Jul 22 '13 at 0:54
I was thinking that when youreturn Dat_Function()
you're really just callingDat_Function()
again inmain()
.Dat_Function()
now returns a function andmain()
has go call it.
– Cate
Jul 22 '13 at 0:58
|
show 1 more comment
To return a value other than None, you need to use a return statement.
In your case, the if block only executes a return when executing one branch. Either move the return outside of the if/else block, or have returns in both options.
I've tried moving it out of the block, but to no avail. Instead of returning the correct value, it returns the first incorrect value. Also, I don't want a return statement for the if part of the if/else statement because I want the function to only return a correct value.
– Cate
Jul 22 '13 at 0:39
add a comment |
def Dat_Function():
my_var = raw_input("Type "a" or "b": ")
if my_var != "a" and my_var != "b":
print "You didn't type "a" or "b". Try again."
print " "
return Dat_Function()
else:
print my_var, "-from Dat_Function"
return my_var
def main():
print Dat_Function(), "-From main()"
main()
add a comment |
I think that you should use while loops.
if my_var != "a" and my_var != "b":
print "You didn't type "a" or "b". Try again."
print " "
return Dat_Function()
Consider that you type something different than "a" and "b", of course, it will call Dat_Function
but then it is skipping the next part. Which is:
else:
print my_var, "-from Dat_Function"
return my_var
And will go directly into:
def main():
print Dat_Function(), "-From main()"
So, if you use while loop as:
while my_var!="a" and my_var!="b":
print ('you didn't type a or b')
return Dat_Function()
This way I think that you can handle it.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f17778372%2fwhy-does-my-function-return-none%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
It is returning None
because when you recursively call it:
if my_var != "a" and my_var != "b":
print "You didn't type "a" or "b". Try again."
print " "
Dat_Function()
..you don't return the value.
So while the recursion does happen, the return value gets discarded, and then you fall off the end of the function. Falling off the end of the function means that python implicitly returns None
, just like this:
>>> def f(x):
... pass
>>> print(f(20))
None
So, instead of just calling Dat Function()
in your if
statement, you need to return
it.
Shouldn't it run through the if statement again if it is called recursively? I don't understand why it wouldn't return a value.
– Cate
Jul 22 '13 at 0:35
Nope. See my edit. The recursion happens, and then you discard what the recursion returns.
– roippi
Jul 22 '13 at 0:38
So if you call a function from inside that same function the return value gets discarded, but you return the same function in that function you really just call it inmain()
?
– Cate
Jul 22 '13 at 0:49
1
You lost me with thatmain()
bit... You can fail as many times as you want to, the one that "succeeds" will returnmy_var
, which will get passed down (return
ed) through all of the recursive calls all the way down to the original caller. Which, yes, ismain()
.
– roippi
Jul 22 '13 at 0:54
I was thinking that when youreturn Dat_Function()
you're really just callingDat_Function()
again inmain()
.Dat_Function()
now returns a function andmain()
has go call it.
– Cate
Jul 22 '13 at 0:58
|
show 1 more comment
It is returning None
because when you recursively call it:
if my_var != "a" and my_var != "b":
print "You didn't type "a" or "b". Try again."
print " "
Dat_Function()
..you don't return the value.
So while the recursion does happen, the return value gets discarded, and then you fall off the end of the function. Falling off the end of the function means that python implicitly returns None
, just like this:
>>> def f(x):
... pass
>>> print(f(20))
None
So, instead of just calling Dat Function()
in your if
statement, you need to return
it.
Shouldn't it run through the if statement again if it is called recursively? I don't understand why it wouldn't return a value.
– Cate
Jul 22 '13 at 0:35
Nope. See my edit. The recursion happens, and then you discard what the recursion returns.
– roippi
Jul 22 '13 at 0:38
So if you call a function from inside that same function the return value gets discarded, but you return the same function in that function you really just call it inmain()
?
– Cate
Jul 22 '13 at 0:49
1
You lost me with thatmain()
bit... You can fail as many times as you want to, the one that "succeeds" will returnmy_var
, which will get passed down (return
ed) through all of the recursive calls all the way down to the original caller. Which, yes, ismain()
.
– roippi
Jul 22 '13 at 0:54
I was thinking that when youreturn Dat_Function()
you're really just callingDat_Function()
again inmain()
.Dat_Function()
now returns a function andmain()
has go call it.
– Cate
Jul 22 '13 at 0:58
|
show 1 more comment
It is returning None
because when you recursively call it:
if my_var != "a" and my_var != "b":
print "You didn't type "a" or "b". Try again."
print " "
Dat_Function()
..you don't return the value.
So while the recursion does happen, the return value gets discarded, and then you fall off the end of the function. Falling off the end of the function means that python implicitly returns None
, just like this:
>>> def f(x):
... pass
>>> print(f(20))
None
So, instead of just calling Dat Function()
in your if
statement, you need to return
it.
It is returning None
because when you recursively call it:
if my_var != "a" and my_var != "b":
print "You didn't type "a" or "b". Try again."
print " "
Dat_Function()
..you don't return the value.
So while the recursion does happen, the return value gets discarded, and then you fall off the end of the function. Falling off the end of the function means that python implicitly returns None
, just like this:
>>> def f(x):
... pass
>>> print(f(20))
None
So, instead of just calling Dat Function()
in your if
statement, you need to return
it.
edited Jul 22 '13 at 0:38
answered Jul 22 '13 at 0:31


roippi
19.8k33153
19.8k33153
Shouldn't it run through the if statement again if it is called recursively? I don't understand why it wouldn't return a value.
– Cate
Jul 22 '13 at 0:35
Nope. See my edit. The recursion happens, and then you discard what the recursion returns.
– roippi
Jul 22 '13 at 0:38
So if you call a function from inside that same function the return value gets discarded, but you return the same function in that function you really just call it inmain()
?
– Cate
Jul 22 '13 at 0:49
1
You lost me with thatmain()
bit... You can fail as many times as you want to, the one that "succeeds" will returnmy_var
, which will get passed down (return
ed) through all of the recursive calls all the way down to the original caller. Which, yes, ismain()
.
– roippi
Jul 22 '13 at 0:54
I was thinking that when youreturn Dat_Function()
you're really just callingDat_Function()
again inmain()
.Dat_Function()
now returns a function andmain()
has go call it.
– Cate
Jul 22 '13 at 0:58
|
show 1 more comment
Shouldn't it run through the if statement again if it is called recursively? I don't understand why it wouldn't return a value.
– Cate
Jul 22 '13 at 0:35
Nope. See my edit. The recursion happens, and then you discard what the recursion returns.
– roippi
Jul 22 '13 at 0:38
So if you call a function from inside that same function the return value gets discarded, but you return the same function in that function you really just call it inmain()
?
– Cate
Jul 22 '13 at 0:49
1
You lost me with thatmain()
bit... You can fail as many times as you want to, the one that "succeeds" will returnmy_var
, which will get passed down (return
ed) through all of the recursive calls all the way down to the original caller. Which, yes, ismain()
.
– roippi
Jul 22 '13 at 0:54
I was thinking that when youreturn Dat_Function()
you're really just callingDat_Function()
again inmain()
.Dat_Function()
now returns a function andmain()
has go call it.
– Cate
Jul 22 '13 at 0:58
Shouldn't it run through the if statement again if it is called recursively? I don't understand why it wouldn't return a value.
– Cate
Jul 22 '13 at 0:35
Shouldn't it run through the if statement again if it is called recursively? I don't understand why it wouldn't return a value.
– Cate
Jul 22 '13 at 0:35
Nope. See my edit. The recursion happens, and then you discard what the recursion returns.
– roippi
Jul 22 '13 at 0:38
Nope. See my edit. The recursion happens, and then you discard what the recursion returns.
– roippi
Jul 22 '13 at 0:38
So if you call a function from inside that same function the return value gets discarded, but you return the same function in that function you really just call it in
main()
?– Cate
Jul 22 '13 at 0:49
So if you call a function from inside that same function the return value gets discarded, but you return the same function in that function you really just call it in
main()
?– Cate
Jul 22 '13 at 0:49
1
1
You lost me with that
main()
bit... You can fail as many times as you want to, the one that "succeeds" will return my_var
, which will get passed down (return
ed) through all of the recursive calls all the way down to the original caller. Which, yes, is main()
.– roippi
Jul 22 '13 at 0:54
You lost me with that
main()
bit... You can fail as many times as you want to, the one that "succeeds" will return my_var
, which will get passed down (return
ed) through all of the recursive calls all the way down to the original caller. Which, yes, is main()
.– roippi
Jul 22 '13 at 0:54
I was thinking that when you
return Dat_Function()
you're really just calling Dat_Function()
again in main()
. Dat_Function()
now returns a function and main()
has go call it.– Cate
Jul 22 '13 at 0:58
I was thinking that when you
return Dat_Function()
you're really just calling Dat_Function()
again in main()
. Dat_Function()
now returns a function and main()
has go call it.– Cate
Jul 22 '13 at 0:58
|
show 1 more comment
To return a value other than None, you need to use a return statement.
In your case, the if block only executes a return when executing one branch. Either move the return outside of the if/else block, or have returns in both options.
I've tried moving it out of the block, but to no avail. Instead of returning the correct value, it returns the first incorrect value. Also, I don't want a return statement for the if part of the if/else statement because I want the function to only return a correct value.
– Cate
Jul 22 '13 at 0:39
add a comment |
To return a value other than None, you need to use a return statement.
In your case, the if block only executes a return when executing one branch. Either move the return outside of the if/else block, or have returns in both options.
I've tried moving it out of the block, but to no avail. Instead of returning the correct value, it returns the first incorrect value. Also, I don't want a return statement for the if part of the if/else statement because I want the function to only return a correct value.
– Cate
Jul 22 '13 at 0:39
add a comment |
To return a value other than None, you need to use a return statement.
In your case, the if block only executes a return when executing one branch. Either move the return outside of the if/else block, or have returns in both options.
To return a value other than None, you need to use a return statement.
In your case, the if block only executes a return when executing one branch. Either move the return outside of the if/else block, or have returns in both options.
answered Jul 22 '13 at 0:32
Simon
611
611
I've tried moving it out of the block, but to no avail. Instead of returning the correct value, it returns the first incorrect value. Also, I don't want a return statement for the if part of the if/else statement because I want the function to only return a correct value.
– Cate
Jul 22 '13 at 0:39
add a comment |
I've tried moving it out of the block, but to no avail. Instead of returning the correct value, it returns the first incorrect value. Also, I don't want a return statement for the if part of the if/else statement because I want the function to only return a correct value.
– Cate
Jul 22 '13 at 0:39
I've tried moving it out of the block, but to no avail. Instead of returning the correct value, it returns the first incorrect value. Also, I don't want a return statement for the if part of the if/else statement because I want the function to only return a correct value.
– Cate
Jul 22 '13 at 0:39
I've tried moving it out of the block, but to no avail. Instead of returning the correct value, it returns the first incorrect value. Also, I don't want a return statement for the if part of the if/else statement because I want the function to only return a correct value.
– Cate
Jul 22 '13 at 0:39
add a comment |
def Dat_Function():
my_var = raw_input("Type "a" or "b": ")
if my_var != "a" and my_var != "b":
print "You didn't type "a" or "b". Try again."
print " "
return Dat_Function()
else:
print my_var, "-from Dat_Function"
return my_var
def main():
print Dat_Function(), "-From main()"
main()
add a comment |
def Dat_Function():
my_var = raw_input("Type "a" or "b": ")
if my_var != "a" and my_var != "b":
print "You didn't type "a" or "b". Try again."
print " "
return Dat_Function()
else:
print my_var, "-from Dat_Function"
return my_var
def main():
print Dat_Function(), "-From main()"
main()
add a comment |
def Dat_Function():
my_var = raw_input("Type "a" or "b": ")
if my_var != "a" and my_var != "b":
print "You didn't type "a" or "b". Try again."
print " "
return Dat_Function()
else:
print my_var, "-from Dat_Function"
return my_var
def main():
print Dat_Function(), "-From main()"
main()
def Dat_Function():
my_var = raw_input("Type "a" or "b": ")
if my_var != "a" and my_var != "b":
print "You didn't type "a" or "b". Try again."
print " "
return Dat_Function()
else:
print my_var, "-from Dat_Function"
return my_var
def main():
print Dat_Function(), "-From main()"
main()
edited May 18 '16 at 0:59
gonz
3,48423147
3,48423147
answered May 17 '16 at 23:34
user6348168
391
391
add a comment |
add a comment |
I think that you should use while loops.
if my_var != "a" and my_var != "b":
print "You didn't type "a" or "b". Try again."
print " "
return Dat_Function()
Consider that you type something different than "a" and "b", of course, it will call Dat_Function
but then it is skipping the next part. Which is:
else:
print my_var, "-from Dat_Function"
return my_var
And will go directly into:
def main():
print Dat_Function(), "-From main()"
So, if you use while loop as:
while my_var!="a" and my_var!="b":
print ('you didn't type a or b')
return Dat_Function()
This way I think that you can handle it.
add a comment |
I think that you should use while loops.
if my_var != "a" and my_var != "b":
print "You didn't type "a" or "b". Try again."
print " "
return Dat_Function()
Consider that you type something different than "a" and "b", of course, it will call Dat_Function
but then it is skipping the next part. Which is:
else:
print my_var, "-from Dat_Function"
return my_var
And will go directly into:
def main():
print Dat_Function(), "-From main()"
So, if you use while loop as:
while my_var!="a" and my_var!="b":
print ('you didn't type a or b')
return Dat_Function()
This way I think that you can handle it.
add a comment |
I think that you should use while loops.
if my_var != "a" and my_var != "b":
print "You didn't type "a" or "b". Try again."
print " "
return Dat_Function()
Consider that you type something different than "a" and "b", of course, it will call Dat_Function
but then it is skipping the next part. Which is:
else:
print my_var, "-from Dat_Function"
return my_var
And will go directly into:
def main():
print Dat_Function(), "-From main()"
So, if you use while loop as:
while my_var!="a" and my_var!="b":
print ('you didn't type a or b')
return Dat_Function()
This way I think that you can handle it.
I think that you should use while loops.
if my_var != "a" and my_var != "b":
print "You didn't type "a" or "b". Try again."
print " "
return Dat_Function()
Consider that you type something different than "a" and "b", of course, it will call Dat_Function
but then it is skipping the next part. Which is:
else:
print my_var, "-from Dat_Function"
return my_var
And will go directly into:
def main():
print Dat_Function(), "-From main()"
So, if you use while loop as:
while my_var!="a" and my_var!="b":
print ('you didn't type a or b')
return Dat_Function()
This way I think that you can handle it.
answered Feb 14 at 16:37


asylturatbek dooranov
1
1
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f17778372%2fwhy-does-my-function-return-none%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
6XOpAQ
7
You need to do
return Dat_Function()
when calling it recursively.– Gustav Larsson
Jul 22 '13 at 0:31
2
Just a tip: The idiomatic way of that
my_var != "a" and my_var != "b"
condition would bemy_var not in ('a', 'b')
– gonz
May 18 '16 at 1:04