Javascript not executing with Cordova
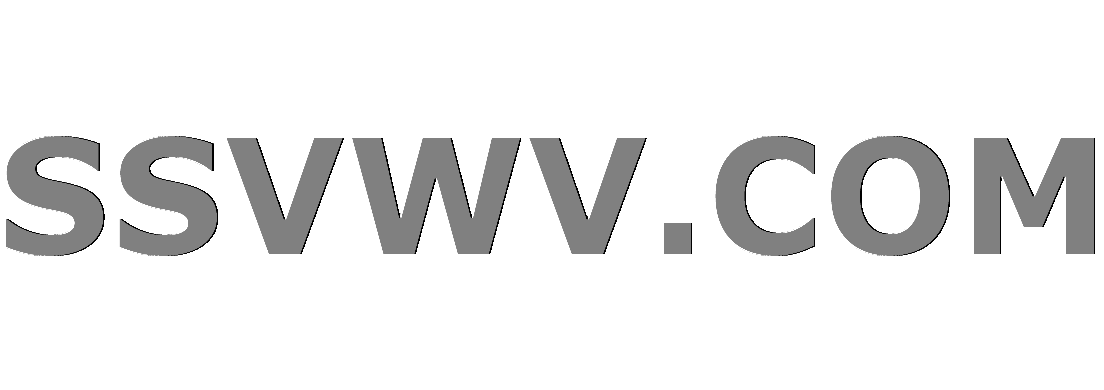
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I cant get a js
global
to work correctly with cordova
. When the device is ready, the app
receives the event, and then creates a Pool
object, which is then initialized, and should show an alert. Here is my code:
index.js
var pool = null;
var app = {
// Application Constructor
initialize: function() {
document.addEventListener('deviceready', this.onDeviceReady.bind(this), false);
},
// deviceready Event Handler
//
// Bind any cordova events here. Common events are:
// 'pause', 'resume', etc.
onDeviceReady: function() {
this.receivedEvent('deviceready');
},
// Update DOM on a Received Event
receivedEvent: function(id) {
alert('Received Event: ' + id);
pool = new Pool();
}
};
app.initialize();
In the above, I see the "Recieved event"
alert.
I have the Pool
object in another file that I am including index.html like this:
<script type="text/javascript" src="cordova.js"></script>
<script type="text/javascript" src="js/index.js"></script>
<script type="text/javascript" src="js/pool.js"></script>
pool.js looks like this:
var Pool = function() {
var pool = {
initialize: function() {
...
alert("pool initialized");
},
...
};
pool.initialize();
return pool;
};
Why don't I see the alert after the Pool
object is created?
UPDATE
My code now looks like this:
index.html
<script type="text/javascript" src="cordova.js"></script>
<script type="text/javascript" src="js/pool.js"></script>
<script type="text/javascript" src="js/index.js"></script>
index.js
var pool = null;
var app = {
// Application Constructor
initialize: function() {
document.addEventListener('deviceready', this.onDeviceReady.bind(this), false);
},
// deviceready Event Handler
//
// Bind any cordova events here. Common events are:
// 'pause', 'resume', etc.
onDeviceReady: function() {
this.receivedEvent('deviceready');
},
// Update DOM on a Received Event
receivedEvent: function(id) {
console.log('Received Event: ' + id);
pool = new Pool();
console.log(pool.fake_data);
pool.initialize();
}
};
app.initialize();
pool.js
function Pool() {
this.fake_data = [{item_name: "Hammer", pic: "", available: true}, {item_name: "Screwdriver", pic: "", available: true},
{item_name: "Iron", pic: "", available: true}, {item_name: "Pot", pic: "", available: true},
{item_name: "Stapler", pic: "", available: true}, {item_name: "Frying pan", pic: "", available: true},
{item_name: "Sugar", pic: "", available: true}, {item_name: "Tape", pic: "", available: true}],
this.initialize = function() {
console.log("pool initialized");
},
...
}
};
};
I am using safari
to debug and i get nothing in the console, not even the console.log
messages that should appear. I changed the alerts
to console.log
and I can't see either of the messages in safari
or Xcode
, is there another way to debug?
Xcode console output:
2018-11-26 20:47:55.423048-0500 Pool[569:76165] Apache Cordova native platform version 4.5.5 is starting.
2018-11-26 20:47:55.423628-0500 Pool[569:76165] Multi-tasking -> Device: YES, App: YES
2018-11-26 20:47:55.443524-0500 Pool[569:76165] Using UIWebView
2018-11-26 20:47:55.445229-0500 Pool[569:76165] [CDVTimer][console] 0.065923ms
2018-11-26 20:47:55.445345-0500 Pool[569:76165] [CDVTimer][handleopenurl] 0.064969ms
2018-11-26 20:47:55.446376-0500 Pool[569:76165] [CDVTimer][intentandnavigationfilter] 0.987053ms
2018-11-26 20:47:55.446456-0500 Pool[569:76165] [CDVTimer][gesturehandler] 0.047088ms
2018-11-26 20:47:55.463569-0500 Pool[569:76165] [CDVTimer][localstorage] 17.084002ms
2018-11-26 20:47:55.463611-0500 Pool[569:76165] [CDVTimer][TotalPluginStartup] 18.489003ms
2018-11-26 20:47:55.707543-0500 Pool[569:76165] Resetting plugins due to page load.
2018-11-26 20:47:55.849767-0500 Pool[569:76165] Finished load of: file:///var/containers/Bundle/Application/6D499572-52BB-418B-8324-CEE8FD13911C/Pool.app/www/index.html
javascript cordova object initialization global-variables
|
show 3 more comments
I cant get a js
global
to work correctly with cordova
. When the device is ready, the app
receives the event, and then creates a Pool
object, which is then initialized, and should show an alert. Here is my code:
index.js
var pool = null;
var app = {
// Application Constructor
initialize: function() {
document.addEventListener('deviceready', this.onDeviceReady.bind(this), false);
},
// deviceready Event Handler
//
// Bind any cordova events here. Common events are:
// 'pause', 'resume', etc.
onDeviceReady: function() {
this.receivedEvent('deviceready');
},
// Update DOM on a Received Event
receivedEvent: function(id) {
alert('Received Event: ' + id);
pool = new Pool();
}
};
app.initialize();
In the above, I see the "Recieved event"
alert.
I have the Pool
object in another file that I am including index.html like this:
<script type="text/javascript" src="cordova.js"></script>
<script type="text/javascript" src="js/index.js"></script>
<script type="text/javascript" src="js/pool.js"></script>
pool.js looks like this:
var Pool = function() {
var pool = {
initialize: function() {
...
alert("pool initialized");
},
...
};
pool.initialize();
return pool;
};
Why don't I see the alert after the Pool
object is created?
UPDATE
My code now looks like this:
index.html
<script type="text/javascript" src="cordova.js"></script>
<script type="text/javascript" src="js/pool.js"></script>
<script type="text/javascript" src="js/index.js"></script>
index.js
var pool = null;
var app = {
// Application Constructor
initialize: function() {
document.addEventListener('deviceready', this.onDeviceReady.bind(this), false);
},
// deviceready Event Handler
//
// Bind any cordova events here. Common events are:
// 'pause', 'resume', etc.
onDeviceReady: function() {
this.receivedEvent('deviceready');
},
// Update DOM on a Received Event
receivedEvent: function(id) {
console.log('Received Event: ' + id);
pool = new Pool();
console.log(pool.fake_data);
pool.initialize();
}
};
app.initialize();
pool.js
function Pool() {
this.fake_data = [{item_name: "Hammer", pic: "", available: true}, {item_name: "Screwdriver", pic: "", available: true},
{item_name: "Iron", pic: "", available: true}, {item_name: "Pot", pic: "", available: true},
{item_name: "Stapler", pic: "", available: true}, {item_name: "Frying pan", pic: "", available: true},
{item_name: "Sugar", pic: "", available: true}, {item_name: "Tape", pic: "", available: true}],
this.initialize = function() {
console.log("pool initialized");
},
...
}
};
};
I am using safari
to debug and i get nothing in the console, not even the console.log
messages that should appear. I changed the alerts
to console.log
and I can't see either of the messages in safari
or Xcode
, is there another way to debug?
Xcode console output:
2018-11-26 20:47:55.423048-0500 Pool[569:76165] Apache Cordova native platform version 4.5.5 is starting.
2018-11-26 20:47:55.423628-0500 Pool[569:76165] Multi-tasking -> Device: YES, App: YES
2018-11-26 20:47:55.443524-0500 Pool[569:76165] Using UIWebView
2018-11-26 20:47:55.445229-0500 Pool[569:76165] [CDVTimer][console] 0.065923ms
2018-11-26 20:47:55.445345-0500 Pool[569:76165] [CDVTimer][handleopenurl] 0.064969ms
2018-11-26 20:47:55.446376-0500 Pool[569:76165] [CDVTimer][intentandnavigationfilter] 0.987053ms
2018-11-26 20:47:55.446456-0500 Pool[569:76165] [CDVTimer][gesturehandler] 0.047088ms
2018-11-26 20:47:55.463569-0500 Pool[569:76165] [CDVTimer][localstorage] 17.084002ms
2018-11-26 20:47:55.463611-0500 Pool[569:76165] [CDVTimer][TotalPluginStartup] 18.489003ms
2018-11-26 20:47:55.707543-0500 Pool[569:76165] Resetting plugins due to page load.
2018-11-26 20:47:55.849767-0500 Pool[569:76165] Finished load of: file:///var/containers/Bundle/Application/6D499572-52BB-418B-8324-CEE8FD13911C/Pool.app/www/index.html
javascript cordova object initialization global-variables
To usenew
, you need to change (and simplify) things a bit: jsfiddle.net/khrismuc/jxoa29pb So the problem isn't that your JS code isn't executing, it's that the syntax was bad, and the console should have told you so.
– Chris G
Nov 27 '18 at 1:01
thanks, so you are saying I cant have two separate files?
– ewizard
Nov 27 '18 at 1:02
Not at all. But you probably need to includepool.js
beforeindex.js
. Still: if you usenew Pool()
thenPool()
will be called as constructor function, which means it mustn't return some arbitrary var. In other words, your problem isn't cordova-related.
– Chris G
Nov 27 '18 at 1:03
ok thanks ill rearrange things and give it a shot
– ewizard
Nov 27 '18 at 1:11
@ChrisG I updated my question, I am still having trouble, check out my changes, thanks
– ewizard
Nov 27 '18 at 1:46
|
show 3 more comments
I cant get a js
global
to work correctly with cordova
. When the device is ready, the app
receives the event, and then creates a Pool
object, which is then initialized, and should show an alert. Here is my code:
index.js
var pool = null;
var app = {
// Application Constructor
initialize: function() {
document.addEventListener('deviceready', this.onDeviceReady.bind(this), false);
},
// deviceready Event Handler
//
// Bind any cordova events here. Common events are:
// 'pause', 'resume', etc.
onDeviceReady: function() {
this.receivedEvent('deviceready');
},
// Update DOM on a Received Event
receivedEvent: function(id) {
alert('Received Event: ' + id);
pool = new Pool();
}
};
app.initialize();
In the above, I see the "Recieved event"
alert.
I have the Pool
object in another file that I am including index.html like this:
<script type="text/javascript" src="cordova.js"></script>
<script type="text/javascript" src="js/index.js"></script>
<script type="text/javascript" src="js/pool.js"></script>
pool.js looks like this:
var Pool = function() {
var pool = {
initialize: function() {
...
alert("pool initialized");
},
...
};
pool.initialize();
return pool;
};
Why don't I see the alert after the Pool
object is created?
UPDATE
My code now looks like this:
index.html
<script type="text/javascript" src="cordova.js"></script>
<script type="text/javascript" src="js/pool.js"></script>
<script type="text/javascript" src="js/index.js"></script>
index.js
var pool = null;
var app = {
// Application Constructor
initialize: function() {
document.addEventListener('deviceready', this.onDeviceReady.bind(this), false);
},
// deviceready Event Handler
//
// Bind any cordova events here. Common events are:
// 'pause', 'resume', etc.
onDeviceReady: function() {
this.receivedEvent('deviceready');
},
// Update DOM on a Received Event
receivedEvent: function(id) {
console.log('Received Event: ' + id);
pool = new Pool();
console.log(pool.fake_data);
pool.initialize();
}
};
app.initialize();
pool.js
function Pool() {
this.fake_data = [{item_name: "Hammer", pic: "", available: true}, {item_name: "Screwdriver", pic: "", available: true},
{item_name: "Iron", pic: "", available: true}, {item_name: "Pot", pic: "", available: true},
{item_name: "Stapler", pic: "", available: true}, {item_name: "Frying pan", pic: "", available: true},
{item_name: "Sugar", pic: "", available: true}, {item_name: "Tape", pic: "", available: true}],
this.initialize = function() {
console.log("pool initialized");
},
...
}
};
};
I am using safari
to debug and i get nothing in the console, not even the console.log
messages that should appear. I changed the alerts
to console.log
and I can't see either of the messages in safari
or Xcode
, is there another way to debug?
Xcode console output:
2018-11-26 20:47:55.423048-0500 Pool[569:76165] Apache Cordova native platform version 4.5.5 is starting.
2018-11-26 20:47:55.423628-0500 Pool[569:76165] Multi-tasking -> Device: YES, App: YES
2018-11-26 20:47:55.443524-0500 Pool[569:76165] Using UIWebView
2018-11-26 20:47:55.445229-0500 Pool[569:76165] [CDVTimer][console] 0.065923ms
2018-11-26 20:47:55.445345-0500 Pool[569:76165] [CDVTimer][handleopenurl] 0.064969ms
2018-11-26 20:47:55.446376-0500 Pool[569:76165] [CDVTimer][intentandnavigationfilter] 0.987053ms
2018-11-26 20:47:55.446456-0500 Pool[569:76165] [CDVTimer][gesturehandler] 0.047088ms
2018-11-26 20:47:55.463569-0500 Pool[569:76165] [CDVTimer][localstorage] 17.084002ms
2018-11-26 20:47:55.463611-0500 Pool[569:76165] [CDVTimer][TotalPluginStartup] 18.489003ms
2018-11-26 20:47:55.707543-0500 Pool[569:76165] Resetting plugins due to page load.
2018-11-26 20:47:55.849767-0500 Pool[569:76165] Finished load of: file:///var/containers/Bundle/Application/6D499572-52BB-418B-8324-CEE8FD13911C/Pool.app/www/index.html
javascript cordova object initialization global-variables
I cant get a js
global
to work correctly with cordova
. When the device is ready, the app
receives the event, and then creates a Pool
object, which is then initialized, and should show an alert. Here is my code:
index.js
var pool = null;
var app = {
// Application Constructor
initialize: function() {
document.addEventListener('deviceready', this.onDeviceReady.bind(this), false);
},
// deviceready Event Handler
//
// Bind any cordova events here. Common events are:
// 'pause', 'resume', etc.
onDeviceReady: function() {
this.receivedEvent('deviceready');
},
// Update DOM on a Received Event
receivedEvent: function(id) {
alert('Received Event: ' + id);
pool = new Pool();
}
};
app.initialize();
In the above, I see the "Recieved event"
alert.
I have the Pool
object in another file that I am including index.html like this:
<script type="text/javascript" src="cordova.js"></script>
<script type="text/javascript" src="js/index.js"></script>
<script type="text/javascript" src="js/pool.js"></script>
pool.js looks like this:
var Pool = function() {
var pool = {
initialize: function() {
...
alert("pool initialized");
},
...
};
pool.initialize();
return pool;
};
Why don't I see the alert after the Pool
object is created?
UPDATE
My code now looks like this:
index.html
<script type="text/javascript" src="cordova.js"></script>
<script type="text/javascript" src="js/pool.js"></script>
<script type="text/javascript" src="js/index.js"></script>
index.js
var pool = null;
var app = {
// Application Constructor
initialize: function() {
document.addEventListener('deviceready', this.onDeviceReady.bind(this), false);
},
// deviceready Event Handler
//
// Bind any cordova events here. Common events are:
// 'pause', 'resume', etc.
onDeviceReady: function() {
this.receivedEvent('deviceready');
},
// Update DOM on a Received Event
receivedEvent: function(id) {
console.log('Received Event: ' + id);
pool = new Pool();
console.log(pool.fake_data);
pool.initialize();
}
};
app.initialize();
pool.js
function Pool() {
this.fake_data = [{item_name: "Hammer", pic: "", available: true}, {item_name: "Screwdriver", pic: "", available: true},
{item_name: "Iron", pic: "", available: true}, {item_name: "Pot", pic: "", available: true},
{item_name: "Stapler", pic: "", available: true}, {item_name: "Frying pan", pic: "", available: true},
{item_name: "Sugar", pic: "", available: true}, {item_name: "Tape", pic: "", available: true}],
this.initialize = function() {
console.log("pool initialized");
},
...
}
};
};
I am using safari
to debug and i get nothing in the console, not even the console.log
messages that should appear. I changed the alerts
to console.log
and I can't see either of the messages in safari
or Xcode
, is there another way to debug?
Xcode console output:
2018-11-26 20:47:55.423048-0500 Pool[569:76165] Apache Cordova native platform version 4.5.5 is starting.
2018-11-26 20:47:55.423628-0500 Pool[569:76165] Multi-tasking -> Device: YES, App: YES
2018-11-26 20:47:55.443524-0500 Pool[569:76165] Using UIWebView
2018-11-26 20:47:55.445229-0500 Pool[569:76165] [CDVTimer][console] 0.065923ms
2018-11-26 20:47:55.445345-0500 Pool[569:76165] [CDVTimer][handleopenurl] 0.064969ms
2018-11-26 20:47:55.446376-0500 Pool[569:76165] [CDVTimer][intentandnavigationfilter] 0.987053ms
2018-11-26 20:47:55.446456-0500 Pool[569:76165] [CDVTimer][gesturehandler] 0.047088ms
2018-11-26 20:47:55.463569-0500 Pool[569:76165] [CDVTimer][localstorage] 17.084002ms
2018-11-26 20:47:55.463611-0500 Pool[569:76165] [CDVTimer][TotalPluginStartup] 18.489003ms
2018-11-26 20:47:55.707543-0500 Pool[569:76165] Resetting plugins due to page load.
2018-11-26 20:47:55.849767-0500 Pool[569:76165] Finished load of: file:///var/containers/Bundle/Application/6D499572-52BB-418B-8324-CEE8FD13911C/Pool.app/www/index.html
javascript cordova object initialization global-variables
javascript cordova object initialization global-variables
edited Nov 27 '18 at 1:45
ewizard
asked Nov 27 '18 at 0:55


ewizardewizard
1,12832772
1,12832772
To usenew
, you need to change (and simplify) things a bit: jsfiddle.net/khrismuc/jxoa29pb So the problem isn't that your JS code isn't executing, it's that the syntax was bad, and the console should have told you so.
– Chris G
Nov 27 '18 at 1:01
thanks, so you are saying I cant have two separate files?
– ewizard
Nov 27 '18 at 1:02
Not at all. But you probably need to includepool.js
beforeindex.js
. Still: if you usenew Pool()
thenPool()
will be called as constructor function, which means it mustn't return some arbitrary var. In other words, your problem isn't cordova-related.
– Chris G
Nov 27 '18 at 1:03
ok thanks ill rearrange things and give it a shot
– ewizard
Nov 27 '18 at 1:11
@ChrisG I updated my question, I am still having trouble, check out my changes, thanks
– ewizard
Nov 27 '18 at 1:46
|
show 3 more comments
To usenew
, you need to change (and simplify) things a bit: jsfiddle.net/khrismuc/jxoa29pb So the problem isn't that your JS code isn't executing, it's that the syntax was bad, and the console should have told you so.
– Chris G
Nov 27 '18 at 1:01
thanks, so you are saying I cant have two separate files?
– ewizard
Nov 27 '18 at 1:02
Not at all. But you probably need to includepool.js
beforeindex.js
. Still: if you usenew Pool()
thenPool()
will be called as constructor function, which means it mustn't return some arbitrary var. In other words, your problem isn't cordova-related.
– Chris G
Nov 27 '18 at 1:03
ok thanks ill rearrange things and give it a shot
– ewizard
Nov 27 '18 at 1:11
@ChrisG I updated my question, I am still having trouble, check out my changes, thanks
– ewizard
Nov 27 '18 at 1:46
To use
new
, you need to change (and simplify) things a bit: jsfiddle.net/khrismuc/jxoa29pb So the problem isn't that your JS code isn't executing, it's that the syntax was bad, and the console should have told you so.– Chris G
Nov 27 '18 at 1:01
To use
new
, you need to change (and simplify) things a bit: jsfiddle.net/khrismuc/jxoa29pb So the problem isn't that your JS code isn't executing, it's that the syntax was bad, and the console should have told you so.– Chris G
Nov 27 '18 at 1:01
thanks, so you are saying I cant have two separate files?
– ewizard
Nov 27 '18 at 1:02
thanks, so you are saying I cant have two separate files?
– ewizard
Nov 27 '18 at 1:02
Not at all. But you probably need to include
pool.js
before index.js
. Still: if you use new Pool()
then Pool()
will be called as constructor function, which means it mustn't return some arbitrary var. In other words, your problem isn't cordova-related.– Chris G
Nov 27 '18 at 1:03
Not at all. But you probably need to include
pool.js
before index.js
. Still: if you use new Pool()
then Pool()
will be called as constructor function, which means it mustn't return some arbitrary var. In other words, your problem isn't cordova-related.– Chris G
Nov 27 '18 at 1:03
ok thanks ill rearrange things and give it a shot
– ewizard
Nov 27 '18 at 1:11
ok thanks ill rearrange things and give it a shot
– ewizard
Nov 27 '18 at 1:11
@ChrisG I updated my question, I am still having trouble, check out my changes, thanks
– ewizard
Nov 27 '18 at 1:46
@ChrisG I updated my question, I am still having trouble, check out my changes, thanks
– ewizard
Nov 27 '18 at 1:46
|
show 3 more comments
1 Answer
1
active
oldest
votes
Caused by javascript code errors, just had to fix those (I used https://jshint.com/ to help sort things out faster.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53491281%2fjavascript-not-executing-with-cordova%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Caused by javascript code errors, just had to fix those (I used https://jshint.com/ to help sort things out faster.
add a comment |
Caused by javascript code errors, just had to fix those (I used https://jshint.com/ to help sort things out faster.
add a comment |
Caused by javascript code errors, just had to fix those (I used https://jshint.com/ to help sort things out faster.
Caused by javascript code errors, just had to fix those (I used https://jshint.com/ to help sort things out faster.
answered Nov 27 '18 at 2:06


ewizardewizard
1,12832772
1,12832772
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53491281%2fjavascript-not-executing-with-cordova%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
6Y,FAUYzhVt49OYoKP
To use
new
, you need to change (and simplify) things a bit: jsfiddle.net/khrismuc/jxoa29pb So the problem isn't that your JS code isn't executing, it's that the syntax was bad, and the console should have told you so.– Chris G
Nov 27 '18 at 1:01
thanks, so you are saying I cant have two separate files?
– ewizard
Nov 27 '18 at 1:02
Not at all. But you probably need to include
pool.js
beforeindex.js
. Still: if you usenew Pool()
thenPool()
will be called as constructor function, which means it mustn't return some arbitrary var. In other words, your problem isn't cordova-related.– Chris G
Nov 27 '18 at 1:03
ok thanks ill rearrange things and give it a shot
– ewizard
Nov 27 '18 at 1:11
@ChrisG I updated my question, I am still having trouble, check out my changes, thanks
– ewizard
Nov 27 '18 at 1:46