Correct approach to initialize an asynchronous JMS Listener and let it run infinitely
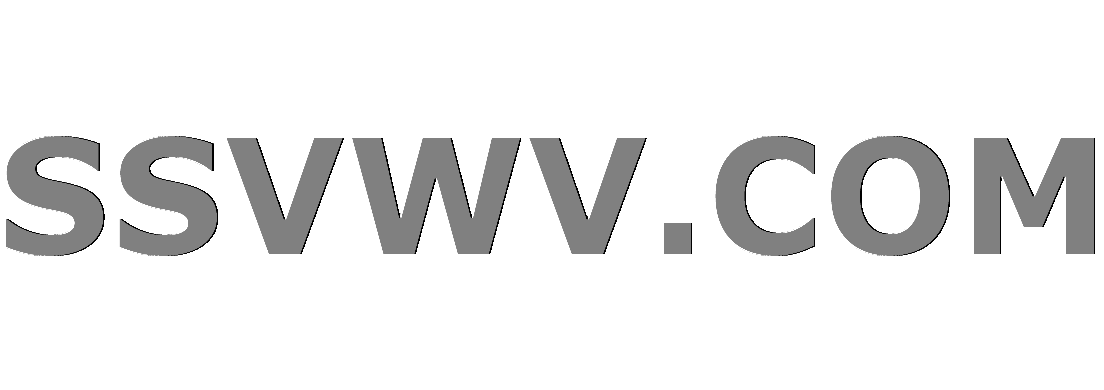
Multi tool use
up vote
0
down vote
favorite
I use the following application to connect to my oracle database, register a queue listener and wait for enqueued messages:
package sample;
import javax.jms.JMSException;
import javax.jms.MessageConsumer;
import javax.jms.Queue;
import javax.jms.QueueConnection;
import javax.jms.QueueConnectionFactory;
import javax.jms.QueueSession;
import javax.jms.Session;
import oracle.jms.AQjmsFactory;
import oracle.jms.AQjmsSession;
public class MyThread extends Thread {
private static final String QUEUE_NAME = "MY_QUEUE";
private static final String QUEUE_USER = "myuser";
private static final String QUEUE_PW = "mypassword";
private boolean running;
public MyThread() {
this.running = true;
}
public static void main(String args) {
MyThread mt = new MyThread();
mt.start();
}
private QueueConnection getQueueConnection() throws JMSException {
QueueConnectionFactory QFac = AQjmsFactory.getQueueConnectionFactory("xxx.xxx.xxx.xxx", "orcl", 1521, "thin");
QueueConnection QCon = QFac.createQueueConnection(QUEUE_USER, QUEUE_PW);
return QCon;
}
@Override
public void interrupt() {
this.running = false;
super.interrupt();
}
@Override
public void run() {
try {
QueueConnection queueConnection = getQueueConnection();
QueueSession queueSession = queueConnection.createQueueSession(false, Session.AUTO_ACKNOWLEDGE);
Queue queue = ((AQjmsSession) queueSession).getQueue(QUEUE_USER, QUEUE_NAME);
while (running) {
System.out.println("Starting...");
queueConnection.start();
MessageConsumer mq = ((AQjmsSession) queueSession).createReceiver(queue);
MyListener listener = new MyListener();
mq.setMessageListener(listener);
System.out.println("... Done, now sleep a bit and redo");
try {
Thread.sleep(5000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.println("Closing Application");
queueSession.close();
queueConnection.close();
} catch (JMSException e) {
e.printStackTrace();
}
}
}
Once a message got enqueued the onMessage function will append a message into a textfiles content:
package sample;
import java.io.BufferedWriter;
import java.io.FileWriter;
import java.io.IOException;
import java.io.PrintWriter;
import javax.jms.Message;
import javax.jms.MessageListener;
public class MyListener implements MessageListener{
@Override
public void onMessage(Message arg0) {
try {
PrintWriter out = new PrintWriter(new BufferedWriter(new FileWriter("C:/temp/output/messages.txt", true)));
out.println("New Message arrived");
out.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
On runtime my console output looks like this:
Starting...
... Done, now sleep a bit an redo
Starting...
... Done, now sleep a bit an redo
Starting...
... Done, now sleep a bit an redo
Starting...
... Done, now sleep a bit an redo
If there where any enqueues the file will contain the new messages.
So: I can dequeue Events and the onMessage event will be triggered with this code.
Now to my question: I'm pretty sure waiting 5 seconds to register the Listener again (and call queueConnection.start() to receive the onMessage calls) is not the correct approach. But if I don't do this there won't be any onMessage events (File stays empty).
What is the correct approach to start listening to a queue infinitely without a fixed Thread.sleep() call and without the need to register the listener again even if there weren't any events?
Additional Information
Database: Oracle 11g2
Java Runtime: 1.6
Maven Dependencies:
- oracle-jdbc (11.2.0.4.0)
- xdb (1.0)
- aqapi (1.3)
- jmscommon (1.3.1_02)
oracle asynchronous queue jms listener
add a comment |
up vote
0
down vote
favorite
I use the following application to connect to my oracle database, register a queue listener and wait for enqueued messages:
package sample;
import javax.jms.JMSException;
import javax.jms.MessageConsumer;
import javax.jms.Queue;
import javax.jms.QueueConnection;
import javax.jms.QueueConnectionFactory;
import javax.jms.QueueSession;
import javax.jms.Session;
import oracle.jms.AQjmsFactory;
import oracle.jms.AQjmsSession;
public class MyThread extends Thread {
private static final String QUEUE_NAME = "MY_QUEUE";
private static final String QUEUE_USER = "myuser";
private static final String QUEUE_PW = "mypassword";
private boolean running;
public MyThread() {
this.running = true;
}
public static void main(String args) {
MyThread mt = new MyThread();
mt.start();
}
private QueueConnection getQueueConnection() throws JMSException {
QueueConnectionFactory QFac = AQjmsFactory.getQueueConnectionFactory("xxx.xxx.xxx.xxx", "orcl", 1521, "thin");
QueueConnection QCon = QFac.createQueueConnection(QUEUE_USER, QUEUE_PW);
return QCon;
}
@Override
public void interrupt() {
this.running = false;
super.interrupt();
}
@Override
public void run() {
try {
QueueConnection queueConnection = getQueueConnection();
QueueSession queueSession = queueConnection.createQueueSession(false, Session.AUTO_ACKNOWLEDGE);
Queue queue = ((AQjmsSession) queueSession).getQueue(QUEUE_USER, QUEUE_NAME);
while (running) {
System.out.println("Starting...");
queueConnection.start();
MessageConsumer mq = ((AQjmsSession) queueSession).createReceiver(queue);
MyListener listener = new MyListener();
mq.setMessageListener(listener);
System.out.println("... Done, now sleep a bit and redo");
try {
Thread.sleep(5000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.println("Closing Application");
queueSession.close();
queueConnection.close();
} catch (JMSException e) {
e.printStackTrace();
}
}
}
Once a message got enqueued the onMessage function will append a message into a textfiles content:
package sample;
import java.io.BufferedWriter;
import java.io.FileWriter;
import java.io.IOException;
import java.io.PrintWriter;
import javax.jms.Message;
import javax.jms.MessageListener;
public class MyListener implements MessageListener{
@Override
public void onMessage(Message arg0) {
try {
PrintWriter out = new PrintWriter(new BufferedWriter(new FileWriter("C:/temp/output/messages.txt", true)));
out.println("New Message arrived");
out.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
On runtime my console output looks like this:
Starting...
... Done, now sleep a bit an redo
Starting...
... Done, now sleep a bit an redo
Starting...
... Done, now sleep a bit an redo
Starting...
... Done, now sleep a bit an redo
If there where any enqueues the file will contain the new messages.
So: I can dequeue Events and the onMessage event will be triggered with this code.
Now to my question: I'm pretty sure waiting 5 seconds to register the Listener again (and call queueConnection.start() to receive the onMessage calls) is not the correct approach. But if I don't do this there won't be any onMessage events (File stays empty).
What is the correct approach to start listening to a queue infinitely without a fixed Thread.sleep() call and without the need to register the listener again even if there weren't any events?
Additional Information
Database: Oracle 11g2
Java Runtime: 1.6
Maven Dependencies:
- oracle-jdbc (11.2.0.4.0)
- xdb (1.0)
- aqapi (1.3)
- jmscommon (1.3.1_02)
oracle asynchronous queue jms listener
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I use the following application to connect to my oracle database, register a queue listener and wait for enqueued messages:
package sample;
import javax.jms.JMSException;
import javax.jms.MessageConsumer;
import javax.jms.Queue;
import javax.jms.QueueConnection;
import javax.jms.QueueConnectionFactory;
import javax.jms.QueueSession;
import javax.jms.Session;
import oracle.jms.AQjmsFactory;
import oracle.jms.AQjmsSession;
public class MyThread extends Thread {
private static final String QUEUE_NAME = "MY_QUEUE";
private static final String QUEUE_USER = "myuser";
private static final String QUEUE_PW = "mypassword";
private boolean running;
public MyThread() {
this.running = true;
}
public static void main(String args) {
MyThread mt = new MyThread();
mt.start();
}
private QueueConnection getQueueConnection() throws JMSException {
QueueConnectionFactory QFac = AQjmsFactory.getQueueConnectionFactory("xxx.xxx.xxx.xxx", "orcl", 1521, "thin");
QueueConnection QCon = QFac.createQueueConnection(QUEUE_USER, QUEUE_PW);
return QCon;
}
@Override
public void interrupt() {
this.running = false;
super.interrupt();
}
@Override
public void run() {
try {
QueueConnection queueConnection = getQueueConnection();
QueueSession queueSession = queueConnection.createQueueSession(false, Session.AUTO_ACKNOWLEDGE);
Queue queue = ((AQjmsSession) queueSession).getQueue(QUEUE_USER, QUEUE_NAME);
while (running) {
System.out.println("Starting...");
queueConnection.start();
MessageConsumer mq = ((AQjmsSession) queueSession).createReceiver(queue);
MyListener listener = new MyListener();
mq.setMessageListener(listener);
System.out.println("... Done, now sleep a bit and redo");
try {
Thread.sleep(5000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.println("Closing Application");
queueSession.close();
queueConnection.close();
} catch (JMSException e) {
e.printStackTrace();
}
}
}
Once a message got enqueued the onMessage function will append a message into a textfiles content:
package sample;
import java.io.BufferedWriter;
import java.io.FileWriter;
import java.io.IOException;
import java.io.PrintWriter;
import javax.jms.Message;
import javax.jms.MessageListener;
public class MyListener implements MessageListener{
@Override
public void onMessage(Message arg0) {
try {
PrintWriter out = new PrintWriter(new BufferedWriter(new FileWriter("C:/temp/output/messages.txt", true)));
out.println("New Message arrived");
out.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
On runtime my console output looks like this:
Starting...
... Done, now sleep a bit an redo
Starting...
... Done, now sleep a bit an redo
Starting...
... Done, now sleep a bit an redo
Starting...
... Done, now sleep a bit an redo
If there where any enqueues the file will contain the new messages.
So: I can dequeue Events and the onMessage event will be triggered with this code.
Now to my question: I'm pretty sure waiting 5 seconds to register the Listener again (and call queueConnection.start() to receive the onMessage calls) is not the correct approach. But if I don't do this there won't be any onMessage events (File stays empty).
What is the correct approach to start listening to a queue infinitely without a fixed Thread.sleep() call and without the need to register the listener again even if there weren't any events?
Additional Information
Database: Oracle 11g2
Java Runtime: 1.6
Maven Dependencies:
- oracle-jdbc (11.2.0.4.0)
- xdb (1.0)
- aqapi (1.3)
- jmscommon (1.3.1_02)
oracle asynchronous queue jms listener
I use the following application to connect to my oracle database, register a queue listener and wait for enqueued messages:
package sample;
import javax.jms.JMSException;
import javax.jms.MessageConsumer;
import javax.jms.Queue;
import javax.jms.QueueConnection;
import javax.jms.QueueConnectionFactory;
import javax.jms.QueueSession;
import javax.jms.Session;
import oracle.jms.AQjmsFactory;
import oracle.jms.AQjmsSession;
public class MyThread extends Thread {
private static final String QUEUE_NAME = "MY_QUEUE";
private static final String QUEUE_USER = "myuser";
private static final String QUEUE_PW = "mypassword";
private boolean running;
public MyThread() {
this.running = true;
}
public static void main(String args) {
MyThread mt = new MyThread();
mt.start();
}
private QueueConnection getQueueConnection() throws JMSException {
QueueConnectionFactory QFac = AQjmsFactory.getQueueConnectionFactory("xxx.xxx.xxx.xxx", "orcl", 1521, "thin");
QueueConnection QCon = QFac.createQueueConnection(QUEUE_USER, QUEUE_PW);
return QCon;
}
@Override
public void interrupt() {
this.running = false;
super.interrupt();
}
@Override
public void run() {
try {
QueueConnection queueConnection = getQueueConnection();
QueueSession queueSession = queueConnection.createQueueSession(false, Session.AUTO_ACKNOWLEDGE);
Queue queue = ((AQjmsSession) queueSession).getQueue(QUEUE_USER, QUEUE_NAME);
while (running) {
System.out.println("Starting...");
queueConnection.start();
MessageConsumer mq = ((AQjmsSession) queueSession).createReceiver(queue);
MyListener listener = new MyListener();
mq.setMessageListener(listener);
System.out.println("... Done, now sleep a bit and redo");
try {
Thread.sleep(5000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.println("Closing Application");
queueSession.close();
queueConnection.close();
} catch (JMSException e) {
e.printStackTrace();
}
}
}
Once a message got enqueued the onMessage function will append a message into a textfiles content:
package sample;
import java.io.BufferedWriter;
import java.io.FileWriter;
import java.io.IOException;
import java.io.PrintWriter;
import javax.jms.Message;
import javax.jms.MessageListener;
public class MyListener implements MessageListener{
@Override
public void onMessage(Message arg0) {
try {
PrintWriter out = new PrintWriter(new BufferedWriter(new FileWriter("C:/temp/output/messages.txt", true)));
out.println("New Message arrived");
out.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
On runtime my console output looks like this:
Starting...
... Done, now sleep a bit an redo
Starting...
... Done, now sleep a bit an redo
Starting...
... Done, now sleep a bit an redo
Starting...
... Done, now sleep a bit an redo
If there where any enqueues the file will contain the new messages.
So: I can dequeue Events and the onMessage event will be triggered with this code.
Now to my question: I'm pretty sure waiting 5 seconds to register the Listener again (and call queueConnection.start() to receive the onMessage calls) is not the correct approach. But if I don't do this there won't be any onMessage events (File stays empty).
What is the correct approach to start listening to a queue infinitely without a fixed Thread.sleep() call and without the need to register the listener again even if there weren't any events?
Additional Information
Database: Oracle 11g2
Java Runtime: 1.6
Maven Dependencies:
- oracle-jdbc (11.2.0.4.0)
- xdb (1.0)
- aqapi (1.3)
- jmscommon (1.3.1_02)
oracle asynchronous queue jms listener
oracle asynchronous queue jms listener
asked Nov 19 at 16:01
Lolan
34
34
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
There's no reason to run a thread to create a JMS consumer and set its message listener. The whole point of a JMS message listener is to receive message asynchronously (functionality which you appear to be trying to duplicate for some reason).
You simply need to create the JMS consumer and set the message listener and then ensure the consumer isn't closed. Depending on how the application is written it is sometimes necessary to have a while
loop to make sure the program doesn't terminate and therefore close the consumer. Your thread isn't doing that. It's letting the consumer fall out of scope after waiting for messages for 5 seconds which means that it will be garbage collected and I expect for most JMS implementations that means it will be closed. It could be worse than that, though. By not explicitly closing the consumer and just letting it fall out of scope you could be leaking consumers which would eventually bog down your message broker. This is not only sloppy programming, but potentially problematic for other users trying to consume messages.
Which command closes my consumer after 5 seconds? Is it because I create a new one after 5 seconds? How do I avoid closing my consumer? Do you have any other hints on how I should change my application to work how I want it to work? Thank you for your time!
– Lolan
Nov 19 at 20:01
I updated my answer to clarify what happens after the 5 secondsleep()
. In my opinion your "application" needs to be completely rewritten to use the JMS API as it was designed to be used (e.g. eliminate theThread
implementation, only create a single consumer, etc.).
– Justin Bertram
Nov 19 at 20:33
Thank you. I tried to do just that in the past and faced the problem that I did not receive any onMessage notification while the application ran. See "Main Application: Listener" in this question. I also put a while loop right after the queueConnection.start(); to check if the consumer was still available and it was. I just didn't get any notification.
– Lolan
Nov 20 at 7:32
I just provided an answer on that question. That code suffered from a similar problem as the code from this question.
– Justin Bertram
Nov 20 at 16:26
You provided the details on why my code is not working as intended in this case (Consumer gets closed + garbage collected) so I will accept your answer as the solution. I updated the question mentioned in my previous comment to represent the current state of my code.
– Lolan
Nov 21 at 8:16
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
There's no reason to run a thread to create a JMS consumer and set its message listener. The whole point of a JMS message listener is to receive message asynchronously (functionality which you appear to be trying to duplicate for some reason).
You simply need to create the JMS consumer and set the message listener and then ensure the consumer isn't closed. Depending on how the application is written it is sometimes necessary to have a while
loop to make sure the program doesn't terminate and therefore close the consumer. Your thread isn't doing that. It's letting the consumer fall out of scope after waiting for messages for 5 seconds which means that it will be garbage collected and I expect for most JMS implementations that means it will be closed. It could be worse than that, though. By not explicitly closing the consumer and just letting it fall out of scope you could be leaking consumers which would eventually bog down your message broker. This is not only sloppy programming, but potentially problematic for other users trying to consume messages.
Which command closes my consumer after 5 seconds? Is it because I create a new one after 5 seconds? How do I avoid closing my consumer? Do you have any other hints on how I should change my application to work how I want it to work? Thank you for your time!
– Lolan
Nov 19 at 20:01
I updated my answer to clarify what happens after the 5 secondsleep()
. In my opinion your "application" needs to be completely rewritten to use the JMS API as it was designed to be used (e.g. eliminate theThread
implementation, only create a single consumer, etc.).
– Justin Bertram
Nov 19 at 20:33
Thank you. I tried to do just that in the past and faced the problem that I did not receive any onMessage notification while the application ran. See "Main Application: Listener" in this question. I also put a while loop right after the queueConnection.start(); to check if the consumer was still available and it was. I just didn't get any notification.
– Lolan
Nov 20 at 7:32
I just provided an answer on that question. That code suffered from a similar problem as the code from this question.
– Justin Bertram
Nov 20 at 16:26
You provided the details on why my code is not working as intended in this case (Consumer gets closed + garbage collected) so I will accept your answer as the solution. I updated the question mentioned in my previous comment to represent the current state of my code.
– Lolan
Nov 21 at 8:16
add a comment |
up vote
1
down vote
accepted
There's no reason to run a thread to create a JMS consumer and set its message listener. The whole point of a JMS message listener is to receive message asynchronously (functionality which you appear to be trying to duplicate for some reason).
You simply need to create the JMS consumer and set the message listener and then ensure the consumer isn't closed. Depending on how the application is written it is sometimes necessary to have a while
loop to make sure the program doesn't terminate and therefore close the consumer. Your thread isn't doing that. It's letting the consumer fall out of scope after waiting for messages for 5 seconds which means that it will be garbage collected and I expect for most JMS implementations that means it will be closed. It could be worse than that, though. By not explicitly closing the consumer and just letting it fall out of scope you could be leaking consumers which would eventually bog down your message broker. This is not only sloppy programming, but potentially problematic for other users trying to consume messages.
Which command closes my consumer after 5 seconds? Is it because I create a new one after 5 seconds? How do I avoid closing my consumer? Do you have any other hints on how I should change my application to work how I want it to work? Thank you for your time!
– Lolan
Nov 19 at 20:01
I updated my answer to clarify what happens after the 5 secondsleep()
. In my opinion your "application" needs to be completely rewritten to use the JMS API as it was designed to be used (e.g. eliminate theThread
implementation, only create a single consumer, etc.).
– Justin Bertram
Nov 19 at 20:33
Thank you. I tried to do just that in the past and faced the problem that I did not receive any onMessage notification while the application ran. See "Main Application: Listener" in this question. I also put a while loop right after the queueConnection.start(); to check if the consumer was still available and it was. I just didn't get any notification.
– Lolan
Nov 20 at 7:32
I just provided an answer on that question. That code suffered from a similar problem as the code from this question.
– Justin Bertram
Nov 20 at 16:26
You provided the details on why my code is not working as intended in this case (Consumer gets closed + garbage collected) so I will accept your answer as the solution. I updated the question mentioned in my previous comment to represent the current state of my code.
– Lolan
Nov 21 at 8:16
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
There's no reason to run a thread to create a JMS consumer and set its message listener. The whole point of a JMS message listener is to receive message asynchronously (functionality which you appear to be trying to duplicate for some reason).
You simply need to create the JMS consumer and set the message listener and then ensure the consumer isn't closed. Depending on how the application is written it is sometimes necessary to have a while
loop to make sure the program doesn't terminate and therefore close the consumer. Your thread isn't doing that. It's letting the consumer fall out of scope after waiting for messages for 5 seconds which means that it will be garbage collected and I expect for most JMS implementations that means it will be closed. It could be worse than that, though. By not explicitly closing the consumer and just letting it fall out of scope you could be leaking consumers which would eventually bog down your message broker. This is not only sloppy programming, but potentially problematic for other users trying to consume messages.
There's no reason to run a thread to create a JMS consumer and set its message listener. The whole point of a JMS message listener is to receive message asynchronously (functionality which you appear to be trying to duplicate for some reason).
You simply need to create the JMS consumer and set the message listener and then ensure the consumer isn't closed. Depending on how the application is written it is sometimes necessary to have a while
loop to make sure the program doesn't terminate and therefore close the consumer. Your thread isn't doing that. It's letting the consumer fall out of scope after waiting for messages for 5 seconds which means that it will be garbage collected and I expect for most JMS implementations that means it will be closed. It could be worse than that, though. By not explicitly closing the consumer and just letting it fall out of scope you could be leaking consumers which would eventually bog down your message broker. This is not only sloppy programming, but potentially problematic for other users trying to consume messages.
edited Nov 19 at 20:30
answered Nov 19 at 18:20


Justin Bertram
2,4731315
2,4731315
Which command closes my consumer after 5 seconds? Is it because I create a new one after 5 seconds? How do I avoid closing my consumer? Do you have any other hints on how I should change my application to work how I want it to work? Thank you for your time!
– Lolan
Nov 19 at 20:01
I updated my answer to clarify what happens after the 5 secondsleep()
. In my opinion your "application" needs to be completely rewritten to use the JMS API as it was designed to be used (e.g. eliminate theThread
implementation, only create a single consumer, etc.).
– Justin Bertram
Nov 19 at 20:33
Thank you. I tried to do just that in the past and faced the problem that I did not receive any onMessage notification while the application ran. See "Main Application: Listener" in this question. I also put a while loop right after the queueConnection.start(); to check if the consumer was still available and it was. I just didn't get any notification.
– Lolan
Nov 20 at 7:32
I just provided an answer on that question. That code suffered from a similar problem as the code from this question.
– Justin Bertram
Nov 20 at 16:26
You provided the details on why my code is not working as intended in this case (Consumer gets closed + garbage collected) so I will accept your answer as the solution. I updated the question mentioned in my previous comment to represent the current state of my code.
– Lolan
Nov 21 at 8:16
add a comment |
Which command closes my consumer after 5 seconds? Is it because I create a new one after 5 seconds? How do I avoid closing my consumer? Do you have any other hints on how I should change my application to work how I want it to work? Thank you for your time!
– Lolan
Nov 19 at 20:01
I updated my answer to clarify what happens after the 5 secondsleep()
. In my opinion your "application" needs to be completely rewritten to use the JMS API as it was designed to be used (e.g. eliminate theThread
implementation, only create a single consumer, etc.).
– Justin Bertram
Nov 19 at 20:33
Thank you. I tried to do just that in the past and faced the problem that I did not receive any onMessage notification while the application ran. See "Main Application: Listener" in this question. I also put a while loop right after the queueConnection.start(); to check if the consumer was still available and it was. I just didn't get any notification.
– Lolan
Nov 20 at 7:32
I just provided an answer on that question. That code suffered from a similar problem as the code from this question.
– Justin Bertram
Nov 20 at 16:26
You provided the details on why my code is not working as intended in this case (Consumer gets closed + garbage collected) so I will accept your answer as the solution. I updated the question mentioned in my previous comment to represent the current state of my code.
– Lolan
Nov 21 at 8:16
Which command closes my consumer after 5 seconds? Is it because I create a new one after 5 seconds? How do I avoid closing my consumer? Do you have any other hints on how I should change my application to work how I want it to work? Thank you for your time!
– Lolan
Nov 19 at 20:01
Which command closes my consumer after 5 seconds? Is it because I create a new one after 5 seconds? How do I avoid closing my consumer? Do you have any other hints on how I should change my application to work how I want it to work? Thank you for your time!
– Lolan
Nov 19 at 20:01
I updated my answer to clarify what happens after the 5 second
sleep()
. In my opinion your "application" needs to be completely rewritten to use the JMS API as it was designed to be used (e.g. eliminate the Thread
implementation, only create a single consumer, etc.).– Justin Bertram
Nov 19 at 20:33
I updated my answer to clarify what happens after the 5 second
sleep()
. In my opinion your "application" needs to be completely rewritten to use the JMS API as it was designed to be used (e.g. eliminate the Thread
implementation, only create a single consumer, etc.).– Justin Bertram
Nov 19 at 20:33
Thank you. I tried to do just that in the past and faced the problem that I did not receive any onMessage notification while the application ran. See "Main Application: Listener" in this question. I also put a while loop right after the queueConnection.start(); to check if the consumer was still available and it was. I just didn't get any notification.
– Lolan
Nov 20 at 7:32
Thank you. I tried to do just that in the past and faced the problem that I did not receive any onMessage notification while the application ran. See "Main Application: Listener" in this question. I also put a while loop right after the queueConnection.start(); to check if the consumer was still available and it was. I just didn't get any notification.
– Lolan
Nov 20 at 7:32
I just provided an answer on that question. That code suffered from a similar problem as the code from this question.
– Justin Bertram
Nov 20 at 16:26
I just provided an answer on that question. That code suffered from a similar problem as the code from this question.
– Justin Bertram
Nov 20 at 16:26
You provided the details on why my code is not working as intended in this case (Consumer gets closed + garbage collected) so I will accept your answer as the solution. I updated the question mentioned in my previous comment to represent the current state of my code.
– Lolan
Nov 21 at 8:16
You provided the details on why my code is not working as intended in this case (Consumer gets closed + garbage collected) so I will accept your answer as the solution. I updated the question mentioned in my previous comment to represent the current state of my code.
– Lolan
Nov 21 at 8:16
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53378450%2fcorrect-approach-to-initialize-an-asynchronous-jms-listener-and-let-it-run-infin%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
lIKlN4AvZGw Mlun1XLEp,drcO9QMOSnTEBEyG14k,4Vo1l5c6p4sXb,QD549m j1y,Eh5eKz,1q SbuyNJz