How to mock a MEF ExportFactory using Moq instances?
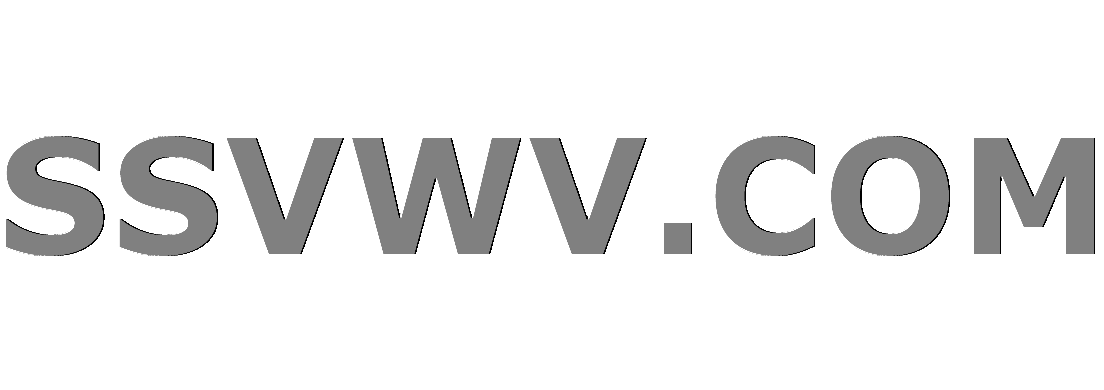
Multi tool use
up vote
2
down vote
favorite
How can I mock a Managed Extensibility Framework (MEF) import into an ExportFactory[IMyType[T]], using mocks of IMyType[T] (being itself a generic interface)? Or, in general, specify instances to be returned by the ExportFactory, which can't be created with a constructor alone?
Moq can't create a mock instance directly, as far as I know. I want an alternative to implementing the whole interface, which may be much larger and change, while I don't want to change the test.
I once found a very complex ExportProvider code, with key-value strings, which didn't work if the generic type of the ExportFactory[T] was itself generic.
// actual generic type is meaningless here, but it is a generic interface
public interface IMyType<T>
{
string GetMessage();
}
public class FactoryImporter<T>
{
[Import]
ExportFactory<IMyType<T>> MyTypeFactory {get;set;}
}
public class Tester
{
public void TestFactoryImporter()
{
Func<IMyType<string>> createMockFunc = () =>
{
var mock = new Mock<IMyType<string>>();
mock.Setup(m => m.GetMessage()).Returns("I'm a mocked IMyType<string>");
return mock.Object;
}
var regBuilder = new RegistrationBuilder();
// pseudo-code, how to do this in reality?
regBuilder.ForType<IMyType<string>>().CreateInstance(createMockFunc);
var catalog = new AggregateCatalog();
var appCatalog = new ApplicationCatalog(regBuilder);
catalog.Catalogs.Add(appCatalog);
var container = new CompositionContainer(catalog);
var factoryImporter = new FactoryImporter<string>();
container.ComposeParts(factoryImporter);
Assert.AreEqual(
"I'm a mocked IMyType<string>",
factoryImporter.MyTypeFactory.GetExport().Value.GetMessage());
}
}
c# .net moq mef
add a comment |
up vote
2
down vote
favorite
How can I mock a Managed Extensibility Framework (MEF) import into an ExportFactory[IMyType[T]], using mocks of IMyType[T] (being itself a generic interface)? Or, in general, specify instances to be returned by the ExportFactory, which can't be created with a constructor alone?
Moq can't create a mock instance directly, as far as I know. I want an alternative to implementing the whole interface, which may be much larger and change, while I don't want to change the test.
I once found a very complex ExportProvider code, with key-value strings, which didn't work if the generic type of the ExportFactory[T] was itself generic.
// actual generic type is meaningless here, but it is a generic interface
public interface IMyType<T>
{
string GetMessage();
}
public class FactoryImporter<T>
{
[Import]
ExportFactory<IMyType<T>> MyTypeFactory {get;set;}
}
public class Tester
{
public void TestFactoryImporter()
{
Func<IMyType<string>> createMockFunc = () =>
{
var mock = new Mock<IMyType<string>>();
mock.Setup(m => m.GetMessage()).Returns("I'm a mocked IMyType<string>");
return mock.Object;
}
var regBuilder = new RegistrationBuilder();
// pseudo-code, how to do this in reality?
regBuilder.ForType<IMyType<string>>().CreateInstance(createMockFunc);
var catalog = new AggregateCatalog();
var appCatalog = new ApplicationCatalog(regBuilder);
catalog.Catalogs.Add(appCatalog);
var container = new CompositionContainer(catalog);
var factoryImporter = new FactoryImporter<string>();
container.ComposeParts(factoryImporter);
Assert.AreEqual(
"I'm a mocked IMyType<string>",
factoryImporter.MyTypeFactory.GetExport().Value.GetMessage());
}
}
c# .net moq mef
add a comment |
up vote
2
down vote
favorite
up vote
2
down vote
favorite
How can I mock a Managed Extensibility Framework (MEF) import into an ExportFactory[IMyType[T]], using mocks of IMyType[T] (being itself a generic interface)? Or, in general, specify instances to be returned by the ExportFactory, which can't be created with a constructor alone?
Moq can't create a mock instance directly, as far as I know. I want an alternative to implementing the whole interface, which may be much larger and change, while I don't want to change the test.
I once found a very complex ExportProvider code, with key-value strings, which didn't work if the generic type of the ExportFactory[T] was itself generic.
// actual generic type is meaningless here, but it is a generic interface
public interface IMyType<T>
{
string GetMessage();
}
public class FactoryImporter<T>
{
[Import]
ExportFactory<IMyType<T>> MyTypeFactory {get;set;}
}
public class Tester
{
public void TestFactoryImporter()
{
Func<IMyType<string>> createMockFunc = () =>
{
var mock = new Mock<IMyType<string>>();
mock.Setup(m => m.GetMessage()).Returns("I'm a mocked IMyType<string>");
return mock.Object;
}
var regBuilder = new RegistrationBuilder();
// pseudo-code, how to do this in reality?
regBuilder.ForType<IMyType<string>>().CreateInstance(createMockFunc);
var catalog = new AggregateCatalog();
var appCatalog = new ApplicationCatalog(regBuilder);
catalog.Catalogs.Add(appCatalog);
var container = new CompositionContainer(catalog);
var factoryImporter = new FactoryImporter<string>();
container.ComposeParts(factoryImporter);
Assert.AreEqual(
"I'm a mocked IMyType<string>",
factoryImporter.MyTypeFactory.GetExport().Value.GetMessage());
}
}
c# .net moq mef
How can I mock a Managed Extensibility Framework (MEF) import into an ExportFactory[IMyType[T]], using mocks of IMyType[T] (being itself a generic interface)? Or, in general, specify instances to be returned by the ExportFactory, which can't be created with a constructor alone?
Moq can't create a mock instance directly, as far as I know. I want an alternative to implementing the whole interface, which may be much larger and change, while I don't want to change the test.
I once found a very complex ExportProvider code, with key-value strings, which didn't work if the generic type of the ExportFactory[T] was itself generic.
// actual generic type is meaningless here, but it is a generic interface
public interface IMyType<T>
{
string GetMessage();
}
public class FactoryImporter<T>
{
[Import]
ExportFactory<IMyType<T>> MyTypeFactory {get;set;}
}
public class Tester
{
public void TestFactoryImporter()
{
Func<IMyType<string>> createMockFunc = () =>
{
var mock = new Mock<IMyType<string>>();
mock.Setup(m => m.GetMessage()).Returns("I'm a mocked IMyType<string>");
return mock.Object;
}
var regBuilder = new RegistrationBuilder();
// pseudo-code, how to do this in reality?
regBuilder.ForType<IMyType<string>>().CreateInstance(createMockFunc);
var catalog = new AggregateCatalog();
var appCatalog = new ApplicationCatalog(regBuilder);
catalog.Catalogs.Add(appCatalog);
var container = new CompositionContainer(catalog);
var factoryImporter = new FactoryImporter<string>();
container.ComposeParts(factoryImporter);
Assert.AreEqual(
"I'm a mocked IMyType<string>",
factoryImporter.MyTypeFactory.GetExport().Value.GetMessage());
}
}
c# .net moq mef
c# .net moq mef
edited Nov 19 at 15:56
asked Nov 19 at 15:50
Erik Hart
567518
567518
add a comment |
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53378255%2fhow-to-mock-a-mef-exportfactorytgeneric-using-moq-instances%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
pvxc92kmXL fIr8JHCBgFt,AcrBc0Kz6AAIUbvYcvlSt,XAN9Jzb4ES5D,NrA dor,W6 9ec5ls iXS,Ioe 9,SDxW43