read file block by block in python
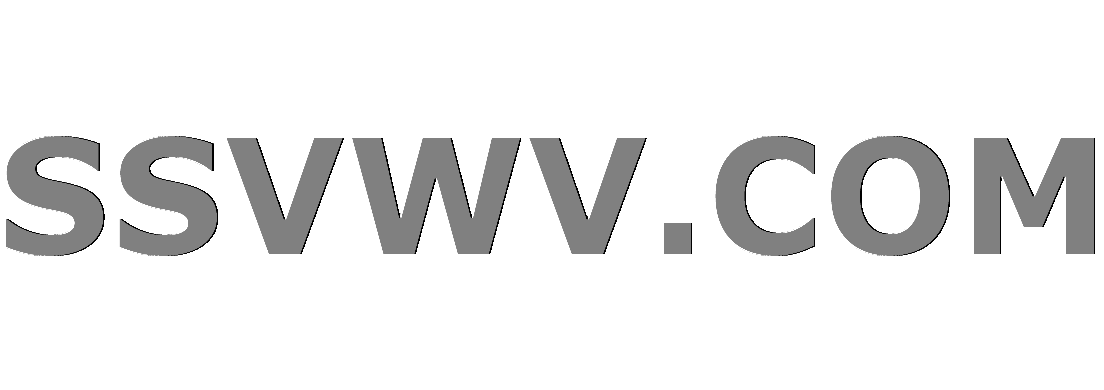
Multi tool use
up vote
-1
down vote
favorite
I have file that has this format
MACRO L20_FDPQ_TV1T8_1
FIXEDMASK ;
CLASS CORE ;
...
...
END L20_FDPQ_TV1T8_1
MACRO INV_20
...
...
END INV_20
I want to read the file as blocks such that each MACRO to end of its name form a block in python. I tried to use this
with open(file_name, "r") as f_read:
lines = f_read.readlines()
num = 0
while num < len(lines):
line = lines[num]
if re.search(r"MACROs+", line, re.IGNORECASE):
macro_name = line.split()[1]
while re.search(r"s+ENDs+%s"%macro_name, line, re.IGNORECASE) is None:
line = lines[num + 1]
#...do something...
num = num+1
num +=1
how this can be done in an efficient way?
python file
add a comment |
up vote
-1
down vote
favorite
I have file that has this format
MACRO L20_FDPQ_TV1T8_1
FIXEDMASK ;
CLASS CORE ;
...
...
END L20_FDPQ_TV1T8_1
MACRO INV_20
...
...
END INV_20
I want to read the file as blocks such that each MACRO to end of its name form a block in python. I tried to use this
with open(file_name, "r") as f_read:
lines = f_read.readlines()
num = 0
while num < len(lines):
line = lines[num]
if re.search(r"MACROs+", line, re.IGNORECASE):
macro_name = line.split()[1]
while re.search(r"s+ENDs+%s"%macro_name, line, re.IGNORECASE) is None:
line = lines[num + 1]
#...do something...
num = num+1
num +=1
how this can be done in an efficient way?
python file
What do you mean by to end of its name?
– toti08
Nov 19 at 15:31
You should show whether you have tried anything or not.
– ahota
Nov 19 at 15:47
why are you searching for "MACRO" and "END"? The beginning of a macro must exactly start with "MACRO" and you know it will end when the line starts exactly with "END". No need to search
– Sembei Norimaki
Nov 19 at 16:04
is this is the efficient way? @ahota
– M.salameh
Nov 19 at 16:10
add a comment |
up vote
-1
down vote
favorite
up vote
-1
down vote
favorite
I have file that has this format
MACRO L20_FDPQ_TV1T8_1
FIXEDMASK ;
CLASS CORE ;
...
...
END L20_FDPQ_TV1T8_1
MACRO INV_20
...
...
END INV_20
I want to read the file as blocks such that each MACRO to end of its name form a block in python. I tried to use this
with open(file_name, "r") as f_read:
lines = f_read.readlines()
num = 0
while num < len(lines):
line = lines[num]
if re.search(r"MACROs+", line, re.IGNORECASE):
macro_name = line.split()[1]
while re.search(r"s+ENDs+%s"%macro_name, line, re.IGNORECASE) is None:
line = lines[num + 1]
#...do something...
num = num+1
num +=1
how this can be done in an efficient way?
python file
I have file that has this format
MACRO L20_FDPQ_TV1T8_1
FIXEDMASK ;
CLASS CORE ;
...
...
END L20_FDPQ_TV1T8_1
MACRO INV_20
...
...
END INV_20
I want to read the file as blocks such that each MACRO to end of its name form a block in python. I tried to use this
with open(file_name, "r") as f_read:
lines = f_read.readlines()
num = 0
while num < len(lines):
line = lines[num]
if re.search(r"MACROs+", line, re.IGNORECASE):
macro_name = line.split()[1]
while re.search(r"s+ENDs+%s"%macro_name, line, re.IGNORECASE) is None:
line = lines[num + 1]
#...do something...
num = num+1
num +=1
how this can be done in an efficient way?
python file
python file
edited Nov 19 at 16:00
asked Nov 19 at 15:06
M.salameh
527
527
What do you mean by to end of its name?
– toti08
Nov 19 at 15:31
You should show whether you have tried anything or not.
– ahota
Nov 19 at 15:47
why are you searching for "MACRO" and "END"? The beginning of a macro must exactly start with "MACRO" and you know it will end when the line starts exactly with "END". No need to search
– Sembei Norimaki
Nov 19 at 16:04
is this is the efficient way? @ahota
– M.salameh
Nov 19 at 16:10
add a comment |
What do you mean by to end of its name?
– toti08
Nov 19 at 15:31
You should show whether you have tried anything or not.
– ahota
Nov 19 at 15:47
why are you searching for "MACRO" and "END"? The beginning of a macro must exactly start with "MACRO" and you know it will end when the line starts exactly with "END". No need to search
– Sembei Norimaki
Nov 19 at 16:04
is this is the efficient way? @ahota
– M.salameh
Nov 19 at 16:10
What do you mean by to end of its name?
– toti08
Nov 19 at 15:31
What do you mean by to end of its name?
– toti08
Nov 19 at 15:31
You should show whether you have tried anything or not.
– ahota
Nov 19 at 15:47
You should show whether you have tried anything or not.
– ahota
Nov 19 at 15:47
why are you searching for "MACRO" and "END"? The beginning of a macro must exactly start with "MACRO" and you know it will end when the line starts exactly with "END". No need to search
– Sembei Norimaki
Nov 19 at 16:04
why are you searching for "MACRO" and "END"? The beginning of a macro must exactly start with "MACRO" and you know it will end when the line starts exactly with "END". No need to search
– Sembei Norimaki
Nov 19 at 16:04
is this is the efficient way? @ahota
– M.salameh
Nov 19 at 16:10
is this is the efficient way? @ahota
– M.salameh
Nov 19 at 16:10
add a comment |
1 Answer
1
active
oldest
votes
up vote
2
down vote
accepted
Assumming that you cannot nest Macros, that Macros always start with "MACRO [name]" and end with "END [name]":
# read the contents of the file
with open(file_name, "r") as f_read:
lines = f_read.readlines()
current_macro_lines =
for line in lines:
if line.startswith("MACRO"):
macro_name = line.split()[1]
# if line starts with END and refers to the current macro name
elif line.startswith("END") and line.split()[1] == macro_name:
# here the macro is complete,
#put the code you want to execute when you find the end of the macro
# then when you finish processing the lines,
# empty them so you can accumulate the next macro
current_macro_lines =
else:
# here you can accumulate the macro lines
current_macro_lines.append(line)
there is "END" line before the "END macro_name" and how can I accumulate macro lines?
– M.salameh
Nov 19 at 16:13
I edited my post to handle this cases
– Sembei Norimaki
Nov 19 at 16:16
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
Assumming that you cannot nest Macros, that Macros always start with "MACRO [name]" and end with "END [name]":
# read the contents of the file
with open(file_name, "r") as f_read:
lines = f_read.readlines()
current_macro_lines =
for line in lines:
if line.startswith("MACRO"):
macro_name = line.split()[1]
# if line starts with END and refers to the current macro name
elif line.startswith("END") and line.split()[1] == macro_name:
# here the macro is complete,
#put the code you want to execute when you find the end of the macro
# then when you finish processing the lines,
# empty them so you can accumulate the next macro
current_macro_lines =
else:
# here you can accumulate the macro lines
current_macro_lines.append(line)
there is "END" line before the "END macro_name" and how can I accumulate macro lines?
– M.salameh
Nov 19 at 16:13
I edited my post to handle this cases
– Sembei Norimaki
Nov 19 at 16:16
add a comment |
up vote
2
down vote
accepted
Assumming that you cannot nest Macros, that Macros always start with "MACRO [name]" and end with "END [name]":
# read the contents of the file
with open(file_name, "r") as f_read:
lines = f_read.readlines()
current_macro_lines =
for line in lines:
if line.startswith("MACRO"):
macro_name = line.split()[1]
# if line starts with END and refers to the current macro name
elif line.startswith("END") and line.split()[1] == macro_name:
# here the macro is complete,
#put the code you want to execute when you find the end of the macro
# then when you finish processing the lines,
# empty them so you can accumulate the next macro
current_macro_lines =
else:
# here you can accumulate the macro lines
current_macro_lines.append(line)
there is "END" line before the "END macro_name" and how can I accumulate macro lines?
– M.salameh
Nov 19 at 16:13
I edited my post to handle this cases
– Sembei Norimaki
Nov 19 at 16:16
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
Assumming that you cannot nest Macros, that Macros always start with "MACRO [name]" and end with "END [name]":
# read the contents of the file
with open(file_name, "r") as f_read:
lines = f_read.readlines()
current_macro_lines =
for line in lines:
if line.startswith("MACRO"):
macro_name = line.split()[1]
# if line starts with END and refers to the current macro name
elif line.startswith("END") and line.split()[1] == macro_name:
# here the macro is complete,
#put the code you want to execute when you find the end of the macro
# then when you finish processing the lines,
# empty them so you can accumulate the next macro
current_macro_lines =
else:
# here you can accumulate the macro lines
current_macro_lines.append(line)
Assumming that you cannot nest Macros, that Macros always start with "MACRO [name]" and end with "END [name]":
# read the contents of the file
with open(file_name, "r") as f_read:
lines = f_read.readlines()
current_macro_lines =
for line in lines:
if line.startswith("MACRO"):
macro_name = line.split()[1]
# if line starts with END and refers to the current macro name
elif line.startswith("END") and line.split()[1] == macro_name:
# here the macro is complete,
#put the code you want to execute when you find the end of the macro
# then when you finish processing the lines,
# empty them so you can accumulate the next macro
current_macro_lines =
else:
# here you can accumulate the macro lines
current_macro_lines.append(line)
edited Nov 19 at 16:15
answered Nov 19 at 16:10
Sembei Norimaki
2,371927
2,371927
there is "END" line before the "END macro_name" and how can I accumulate macro lines?
– M.salameh
Nov 19 at 16:13
I edited my post to handle this cases
– Sembei Norimaki
Nov 19 at 16:16
add a comment |
there is "END" line before the "END macro_name" and how can I accumulate macro lines?
– M.salameh
Nov 19 at 16:13
I edited my post to handle this cases
– Sembei Norimaki
Nov 19 at 16:16
there is "END" line before the "END macro_name" and how can I accumulate macro lines?
– M.salameh
Nov 19 at 16:13
there is "END" line before the "END macro_name" and how can I accumulate macro lines?
– M.salameh
Nov 19 at 16:13
I edited my post to handle this cases
– Sembei Norimaki
Nov 19 at 16:16
I edited my post to handle this cases
– Sembei Norimaki
Nov 19 at 16:16
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53377457%2fread-file-block-by-block-in-python%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
sI,7i3imcm j9pO3g0IkzsZay3x0ogHv2efEKoRlxN31B,hWnq5U cGlQyU
What do you mean by to end of its name?
– toti08
Nov 19 at 15:31
You should show whether you have tried anything or not.
– ahota
Nov 19 at 15:47
why are you searching for "MACRO" and "END"? The beginning of a macro must exactly start with "MACRO" and you know it will end when the line starts exactly with "END". No need to search
– Sembei Norimaki
Nov 19 at 16:04
is this is the efficient way? @ahota
– M.salameh
Nov 19 at 16:10