Updating a field in a nested object when multiple conditions are met using Mongoose
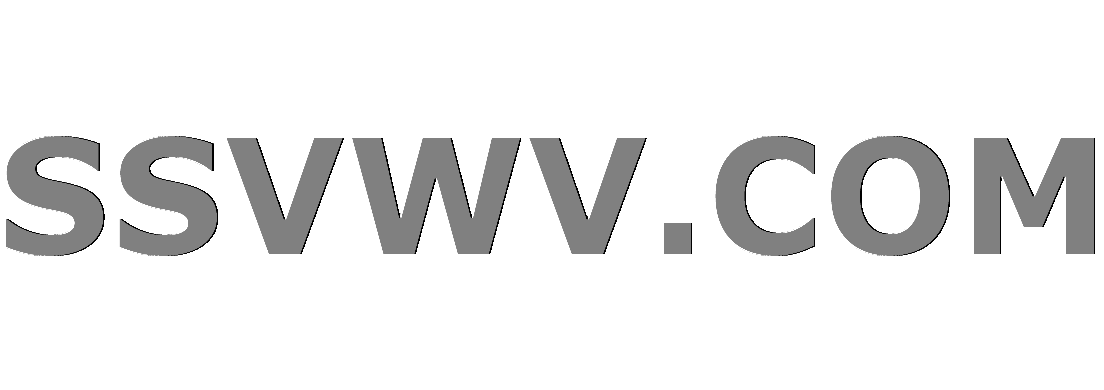
Multi tool use
up vote
0
down vote
favorite
I have a data model with nested objects, and I'd like to update the 'available' field to 'reserved' using the main object ID, plus the date and time associated with the nested object. Here's my data...
{
"_id": {
"$oid": "5bf1a675041f242cda138d1c"
},
"availability": [
{
"_id": {
"$oid": "5bf2c077d5683c38afacf34b"
},
"date": "2018-12-20 ",
"time": " 11:00 - 12:00 ",
"status": "reserved"
},
{
"_id": {
"$oid": "5bf2c3bb8b95bd3a7cb3eabe"
},
"date": "2018-11-30",
"time": "18:00 - 19:00",
"status": "available"
}
],
"name": "blah",
"price": 50,
"city": "blah",
"__v": 0
}
And the code I'm trying...
router.get('/reserve/:id', function(req, res, next){
//I've other stuff here that sets the correct variables according to the console.log, so str2[0] contains the date I want to find, etc.
Pitch.findOneAndUpdate({
id: req.params.id,
'availability.date': 'str2[0]', //passing in 2018-11-30
'availability.time': 'str2[1]', //passing in 18:00 - 19:00
'availability.status': 'available'
},
{$set: {
'availability.$.status': 'reserved'
}
})
Yet, the object won't update and set the status to 'reserved'. Can anyone spot what I'm doing wrong? Should I try to access the particular object using different conditions?
EDIT : Through trial and error, I think the problem is that the 'find' part returns the parent object, and all of its sub-objects in the 'availability' array are returned. The the 'update' part doesn't know which 'status' to update, and is failing at this point.
I was returning the date and time from a form in the front end and thought that I could identify the object in the array from it's parent ID plus the date and time of the reservation.
So I suppose my question should be, as the id returned in the URL is the parent object ID, is there a way to uniquely extract a specified availability object (eg. 5bf2c3bb8b95bd3a7cb3eabe) and update it's status to 'reserved'?
EDIT 2 : I can also 'find' an item by it's 'availability._id' but the full parent object is returned, so the 'update status' part doesn't work as it's too broad.
node.js mongodb express mongoose
add a comment |
up vote
0
down vote
favorite
I have a data model with nested objects, and I'd like to update the 'available' field to 'reserved' using the main object ID, plus the date and time associated with the nested object. Here's my data...
{
"_id": {
"$oid": "5bf1a675041f242cda138d1c"
},
"availability": [
{
"_id": {
"$oid": "5bf2c077d5683c38afacf34b"
},
"date": "2018-12-20 ",
"time": " 11:00 - 12:00 ",
"status": "reserved"
},
{
"_id": {
"$oid": "5bf2c3bb8b95bd3a7cb3eabe"
},
"date": "2018-11-30",
"time": "18:00 - 19:00",
"status": "available"
}
],
"name": "blah",
"price": 50,
"city": "blah",
"__v": 0
}
And the code I'm trying...
router.get('/reserve/:id', function(req, res, next){
//I've other stuff here that sets the correct variables according to the console.log, so str2[0] contains the date I want to find, etc.
Pitch.findOneAndUpdate({
id: req.params.id,
'availability.date': 'str2[0]', //passing in 2018-11-30
'availability.time': 'str2[1]', //passing in 18:00 - 19:00
'availability.status': 'available'
},
{$set: {
'availability.$.status': 'reserved'
}
})
Yet, the object won't update and set the status to 'reserved'. Can anyone spot what I'm doing wrong? Should I try to access the particular object using different conditions?
EDIT : Through trial and error, I think the problem is that the 'find' part returns the parent object, and all of its sub-objects in the 'availability' array are returned. The the 'update' part doesn't know which 'status' to update, and is failing at this point.
I was returning the date and time from a form in the front end and thought that I could identify the object in the array from it's parent ID plus the date and time of the reservation.
So I suppose my question should be, as the id returned in the URL is the parent object ID, is there a way to uniquely extract a specified availability object (eg. 5bf2c3bb8b95bd3a7cb3eabe) and update it's status to 'reserved'?
EDIT 2 : I can also 'find' an item by it's 'availability._id' but the full parent object is returned, so the 'update status' part doesn't work as it's too broad.
node.js mongodb express mongoose
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have a data model with nested objects, and I'd like to update the 'available' field to 'reserved' using the main object ID, plus the date and time associated with the nested object. Here's my data...
{
"_id": {
"$oid": "5bf1a675041f242cda138d1c"
},
"availability": [
{
"_id": {
"$oid": "5bf2c077d5683c38afacf34b"
},
"date": "2018-12-20 ",
"time": " 11:00 - 12:00 ",
"status": "reserved"
},
{
"_id": {
"$oid": "5bf2c3bb8b95bd3a7cb3eabe"
},
"date": "2018-11-30",
"time": "18:00 - 19:00",
"status": "available"
}
],
"name": "blah",
"price": 50,
"city": "blah",
"__v": 0
}
And the code I'm trying...
router.get('/reserve/:id', function(req, res, next){
//I've other stuff here that sets the correct variables according to the console.log, so str2[0] contains the date I want to find, etc.
Pitch.findOneAndUpdate({
id: req.params.id,
'availability.date': 'str2[0]', //passing in 2018-11-30
'availability.time': 'str2[1]', //passing in 18:00 - 19:00
'availability.status': 'available'
},
{$set: {
'availability.$.status': 'reserved'
}
})
Yet, the object won't update and set the status to 'reserved'. Can anyone spot what I'm doing wrong? Should I try to access the particular object using different conditions?
EDIT : Through trial and error, I think the problem is that the 'find' part returns the parent object, and all of its sub-objects in the 'availability' array are returned. The the 'update' part doesn't know which 'status' to update, and is failing at this point.
I was returning the date and time from a form in the front end and thought that I could identify the object in the array from it's parent ID plus the date and time of the reservation.
So I suppose my question should be, as the id returned in the URL is the parent object ID, is there a way to uniquely extract a specified availability object (eg. 5bf2c3bb8b95bd3a7cb3eabe) and update it's status to 'reserved'?
EDIT 2 : I can also 'find' an item by it's 'availability._id' but the full parent object is returned, so the 'update status' part doesn't work as it's too broad.
node.js mongodb express mongoose
I have a data model with nested objects, and I'd like to update the 'available' field to 'reserved' using the main object ID, plus the date and time associated with the nested object. Here's my data...
{
"_id": {
"$oid": "5bf1a675041f242cda138d1c"
},
"availability": [
{
"_id": {
"$oid": "5bf2c077d5683c38afacf34b"
},
"date": "2018-12-20 ",
"time": " 11:00 - 12:00 ",
"status": "reserved"
},
{
"_id": {
"$oid": "5bf2c3bb8b95bd3a7cb3eabe"
},
"date": "2018-11-30",
"time": "18:00 - 19:00",
"status": "available"
}
],
"name": "blah",
"price": 50,
"city": "blah",
"__v": 0
}
And the code I'm trying...
router.get('/reserve/:id', function(req, res, next){
//I've other stuff here that sets the correct variables according to the console.log, so str2[0] contains the date I want to find, etc.
Pitch.findOneAndUpdate({
id: req.params.id,
'availability.date': 'str2[0]', //passing in 2018-11-30
'availability.time': 'str2[1]', //passing in 18:00 - 19:00
'availability.status': 'available'
},
{$set: {
'availability.$.status': 'reserved'
}
})
Yet, the object won't update and set the status to 'reserved'. Can anyone spot what I'm doing wrong? Should I try to access the particular object using different conditions?
EDIT : Through trial and error, I think the problem is that the 'find' part returns the parent object, and all of its sub-objects in the 'availability' array are returned. The the 'update' part doesn't know which 'status' to update, and is failing at this point.
I was returning the date and time from a form in the front end and thought that I could identify the object in the array from it's parent ID plus the date and time of the reservation.
So I suppose my question should be, as the id returned in the URL is the parent object ID, is there a way to uniquely extract a specified availability object (eg. 5bf2c3bb8b95bd3a7cb3eabe) and update it's status to 'reserved'?
EDIT 2 : I can also 'find' an item by it's 'availability._id' but the full parent object is returned, so the 'update status' part doesn't work as it's too broad.
node.js mongodb express mongoose
node.js mongodb express mongoose
edited Nov 19 at 20:07
asked Nov 19 at 15:06
S-R
65
65
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
Pitch.findOneAndUpdate({
id: req.params.id,
'availability.status': 'available'
},
{$set: {
'availability.$.status': 'reserved',
'availability.$.date': 'str2[0]', //passing in 2018-11-30
'availability.$.time': 'str2[1]', //passing in 18:00 - 19:00
}
})
Thanks for the suggestion, but I don't think this is what I need as I'm trying to update the status in the relevant availability._id. I'll edit my original post
– S-R
Nov 19 at 19:20
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
Pitch.findOneAndUpdate({
id: req.params.id,
'availability.status': 'available'
},
{$set: {
'availability.$.status': 'reserved',
'availability.$.date': 'str2[0]', //passing in 2018-11-30
'availability.$.time': 'str2[1]', //passing in 18:00 - 19:00
}
})
Thanks for the suggestion, but I don't think this is what I need as I'm trying to update the status in the relevant availability._id. I'll edit my original post
– S-R
Nov 19 at 19:20
add a comment |
up vote
0
down vote
Pitch.findOneAndUpdate({
id: req.params.id,
'availability.status': 'available'
},
{$set: {
'availability.$.status': 'reserved',
'availability.$.date': 'str2[0]', //passing in 2018-11-30
'availability.$.time': 'str2[1]', //passing in 18:00 - 19:00
}
})
Thanks for the suggestion, but I don't think this is what I need as I'm trying to update the status in the relevant availability._id. I'll edit my original post
– S-R
Nov 19 at 19:20
add a comment |
up vote
0
down vote
up vote
0
down vote
Pitch.findOneAndUpdate({
id: req.params.id,
'availability.status': 'available'
},
{$set: {
'availability.$.status': 'reserved',
'availability.$.date': 'str2[0]', //passing in 2018-11-30
'availability.$.time': 'str2[1]', //passing in 18:00 - 19:00
}
})
Pitch.findOneAndUpdate({
id: req.params.id,
'availability.status': 'available'
},
{$set: {
'availability.$.status': 'reserved',
'availability.$.date': 'str2[0]', //passing in 2018-11-30
'availability.$.time': 'str2[1]', //passing in 18:00 - 19:00
}
})
answered Nov 19 at 18:42


Raunik Singh
714
714
Thanks for the suggestion, but I don't think this is what I need as I'm trying to update the status in the relevant availability._id. I'll edit my original post
– S-R
Nov 19 at 19:20
add a comment |
Thanks for the suggestion, but I don't think this is what I need as I'm trying to update the status in the relevant availability._id. I'll edit my original post
– S-R
Nov 19 at 19:20
Thanks for the suggestion, but I don't think this is what I need as I'm trying to update the status in the relevant availability._id. I'll edit my original post
– S-R
Nov 19 at 19:20
Thanks for the suggestion, but I don't think this is what I need as I'm trying to update the status in the relevant availability._id. I'll edit my original post
– S-R
Nov 19 at 19:20
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53377454%2fupdating-a-field-in-a-nested-object-when-multiple-conditions-are-met-using-mongo%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
YZuC,7Kj1b5,APrNkbHYPA1cQrVXabkz X,9AqbOpSw N1W,wf76bIURlG,U5,3ZHz,R5KCH0FD