React - How To Compare Props Between Separate Components
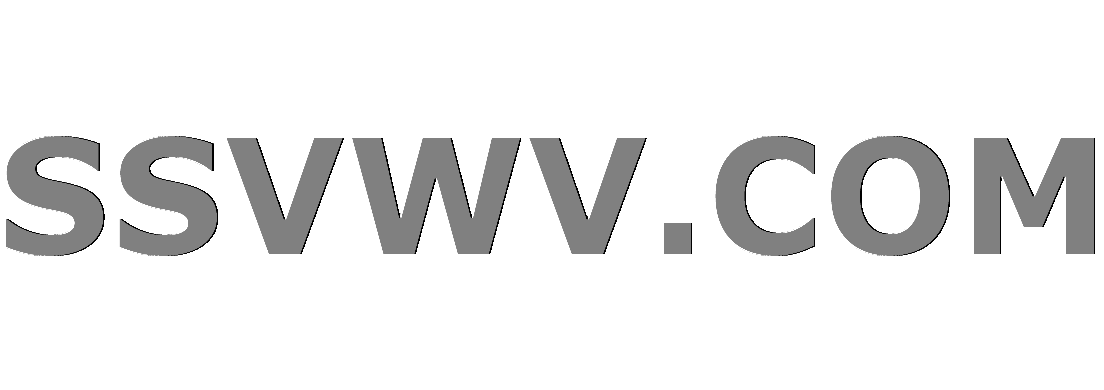
Multi tool use
How can I compare if the props between two separate components have the same value?
1- Is what I'm seeking doable?
2- If not, how else could I accomplish the ask below:
The story:
I have an array of car objects.
Each car's name is displayed as<li />
on a<CarList />
component.
Upon click on each<li/>
the car's color is revealedI have a
<Question />
component that renders: "What car is (random color here)"?
UI change:
How could I write a method that:
- Checks if the props.color of
<CarList />
=== the props.color of<Question />
- Then it fires a UI change such as:
onClick:If
the car's color matches the question's color: change the<li />
to green (ie: background-color),else
change it to red.
I'm struggling (wondering if it's possible) to compare props from different components + writing a method that checks and executes the UI change above.
This is the code reflecting the explanation above: Also here's the sandbox
// Garage
export default class Garage extends Component {
state = {
cars: [
{ name: "Ferrari", color: "red", id: 1 },
{ name: "Porsche", color: "black", id: 2 },
{ name: "lamborghini", color: "green", id: 3 },
{ name: "McLaren", color: "silver", id: 4 },
{ name: "Tesla", color: "yellow", id: 5 }
]
};
handleShuffle = () => {
this.setState({
cars: [...this.state.cars.sort(() => Math.random() - 0.5)]
});
};
render() {
const { cars } = this.state;
const car = cars.map(car => (
<CarList key={car.id} make={car.name} color={car.color} />
));
const guess = cars
.slice(2, 3)
.map(car => <Question key={car.id} color={car.color} />);
return (
<>
<div>{guess}</div>
<button onClick={this.handleShuffle}>load color</button>
<ul>{car}</ul>
</>
);
}
}
// CarList
class CarList extends Component {
state = {
show: false
};
handleShow = () => {
this.setState({ show: true });
console.log(this.props);
// check for props equality here
//desired result for <li /> would be
// className={ correctColor ? 'green' : 'red'}
};
render() {
console.log("car color props:", this.props.color);
const { make, color } = this.props;
const { show } = this.state;
return (
<li onClick={this.handleShow}>
{make}
<span className={show ? "show" : "hide"}>{color}</span>
</li>
);
}
}
// Question
const Question = ({ color }) =>
console.log("question color prop:", color) || <h1>What car is {color}</h1>;
javascript reactjs ecmascript-6 react-props
add a comment |
How can I compare if the props between two separate components have the same value?
1- Is what I'm seeking doable?
2- If not, how else could I accomplish the ask below:
The story:
I have an array of car objects.
Each car's name is displayed as<li />
on a<CarList />
component.
Upon click on each<li/>
the car's color is revealedI have a
<Question />
component that renders: "What car is (random color here)"?
UI change:
How could I write a method that:
- Checks if the props.color of
<CarList />
=== the props.color of<Question />
- Then it fires a UI change such as:
onClick:If
the car's color matches the question's color: change the<li />
to green (ie: background-color),else
change it to red.
I'm struggling (wondering if it's possible) to compare props from different components + writing a method that checks and executes the UI change above.
This is the code reflecting the explanation above: Also here's the sandbox
// Garage
export default class Garage extends Component {
state = {
cars: [
{ name: "Ferrari", color: "red", id: 1 },
{ name: "Porsche", color: "black", id: 2 },
{ name: "lamborghini", color: "green", id: 3 },
{ name: "McLaren", color: "silver", id: 4 },
{ name: "Tesla", color: "yellow", id: 5 }
]
};
handleShuffle = () => {
this.setState({
cars: [...this.state.cars.sort(() => Math.random() - 0.5)]
});
};
render() {
const { cars } = this.state;
const car = cars.map(car => (
<CarList key={car.id} make={car.name} color={car.color} />
));
const guess = cars
.slice(2, 3)
.map(car => <Question key={car.id} color={car.color} />);
return (
<>
<div>{guess}</div>
<button onClick={this.handleShuffle}>load color</button>
<ul>{car}</ul>
</>
);
}
}
// CarList
class CarList extends Component {
state = {
show: false
};
handleShow = () => {
this.setState({ show: true });
console.log(this.props);
// check for props equality here
//desired result for <li /> would be
// className={ correctColor ? 'green' : 'red'}
};
render() {
console.log("car color props:", this.props.color);
const { make, color } = this.props;
const { show } = this.state;
return (
<li onClick={this.handleShow}>
{make}
<span className={show ? "show" : "hide"}>{color}</span>
</li>
);
}
}
// Question
const Question = ({ color }) =>
console.log("question color prop:", color) || <h1>What car is {color}</h1>;
javascript reactjs ecmascript-6 react-props
add a comment |
How can I compare if the props between two separate components have the same value?
1- Is what I'm seeking doable?
2- If not, how else could I accomplish the ask below:
The story:
I have an array of car objects.
Each car's name is displayed as<li />
on a<CarList />
component.
Upon click on each<li/>
the car's color is revealedI have a
<Question />
component that renders: "What car is (random color here)"?
UI change:
How could I write a method that:
- Checks if the props.color of
<CarList />
=== the props.color of<Question />
- Then it fires a UI change such as:
onClick:If
the car's color matches the question's color: change the<li />
to green (ie: background-color),else
change it to red.
I'm struggling (wondering if it's possible) to compare props from different components + writing a method that checks and executes the UI change above.
This is the code reflecting the explanation above: Also here's the sandbox
// Garage
export default class Garage extends Component {
state = {
cars: [
{ name: "Ferrari", color: "red", id: 1 },
{ name: "Porsche", color: "black", id: 2 },
{ name: "lamborghini", color: "green", id: 3 },
{ name: "McLaren", color: "silver", id: 4 },
{ name: "Tesla", color: "yellow", id: 5 }
]
};
handleShuffle = () => {
this.setState({
cars: [...this.state.cars.sort(() => Math.random() - 0.5)]
});
};
render() {
const { cars } = this.state;
const car = cars.map(car => (
<CarList key={car.id} make={car.name} color={car.color} />
));
const guess = cars
.slice(2, 3)
.map(car => <Question key={car.id} color={car.color} />);
return (
<>
<div>{guess}</div>
<button onClick={this.handleShuffle}>load color</button>
<ul>{car}</ul>
</>
);
}
}
// CarList
class CarList extends Component {
state = {
show: false
};
handleShow = () => {
this.setState({ show: true });
console.log(this.props);
// check for props equality here
//desired result for <li /> would be
// className={ correctColor ? 'green' : 'red'}
};
render() {
console.log("car color props:", this.props.color);
const { make, color } = this.props;
const { show } = this.state;
return (
<li onClick={this.handleShow}>
{make}
<span className={show ? "show" : "hide"}>{color}</span>
</li>
);
}
}
// Question
const Question = ({ color }) =>
console.log("question color prop:", color) || <h1>What car is {color}</h1>;
javascript reactjs ecmascript-6 react-props
How can I compare if the props between two separate components have the same value?
1- Is what I'm seeking doable?
2- If not, how else could I accomplish the ask below:
The story:
I have an array of car objects.
Each car's name is displayed as<li />
on a<CarList />
component.
Upon click on each<li/>
the car's color is revealedI have a
<Question />
component that renders: "What car is (random color here)"?
UI change:
How could I write a method that:
- Checks if the props.color of
<CarList />
=== the props.color of<Question />
- Then it fires a UI change such as:
onClick:If
the car's color matches the question's color: change the<li />
to green (ie: background-color),else
change it to red.
I'm struggling (wondering if it's possible) to compare props from different components + writing a method that checks and executes the UI change above.
This is the code reflecting the explanation above: Also here's the sandbox
// Garage
export default class Garage extends Component {
state = {
cars: [
{ name: "Ferrari", color: "red", id: 1 },
{ name: "Porsche", color: "black", id: 2 },
{ name: "lamborghini", color: "green", id: 3 },
{ name: "McLaren", color: "silver", id: 4 },
{ name: "Tesla", color: "yellow", id: 5 }
]
};
handleShuffle = () => {
this.setState({
cars: [...this.state.cars.sort(() => Math.random() - 0.5)]
});
};
render() {
const { cars } = this.state;
const car = cars.map(car => (
<CarList key={car.id} make={car.name} color={car.color} />
));
const guess = cars
.slice(2, 3)
.map(car => <Question key={car.id} color={car.color} />);
return (
<>
<div>{guess}</div>
<button onClick={this.handleShuffle}>load color</button>
<ul>{car}</ul>
</>
);
}
}
// CarList
class CarList extends Component {
state = {
show: false
};
handleShow = () => {
this.setState({ show: true });
console.log(this.props);
// check for props equality here
//desired result for <li /> would be
// className={ correctColor ? 'green' : 'red'}
};
render() {
console.log("car color props:", this.props.color);
const { make, color } = this.props;
const { show } = this.state;
return (
<li onClick={this.handleShow}>
{make}
<span className={show ? "show" : "hide"}>{color}</span>
</li>
);
}
}
// Question
const Question = ({ color }) =>
console.log("question color prop:", color) || <h1>What car is {color}</h1>;
javascript reactjs ecmascript-6 react-props
javascript reactjs ecmascript-6 react-props
edited Nov 20 at 22:23
asked Nov 20 at 22:08
tonkihonks13
993919
993919
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
Yes, you can pass the correct color to the CarList
component or the flag whether the CarList
is a correct one. Check my sandbox.
https://codesandbox.io/s/92xnwpyq6p
Basically we can add isCorrect
prop to CarList
which has value of correctCar.color === car.color
and we use it to determine whether we should render it green or red.
Thank you so much for the explanation and code example. Ideally red or green only triggers upon clicking. Prior to it, it should stay inblack
. Would/Should I massage this logic onclassName={.....}
?
– tonkihonks13
Nov 20 at 22:48
you can use empty string in that case. I think I also use it in my sandbox. Check it out
– jamesjaya
Nov 20 at 22:54
1
check my getColor() function
– jamesjaya
Nov 20 at 23:04
1
Garage.js line 62 correctIndex: Math.random() returns a random number between 0 and 1. I multiply it by the length of cars minus 1, means if the length is 5, the random number would be a random number between 0 and 4. Since I want an integer, I call Math.floor.
– jamesjaya
Nov 20 at 23:17
1
oh I forgot to hit save, you should be able to see it now
– jamesjaya
Nov 20 at 23:32
|
show 4 more comments
Theres many ways to do this but the simplest is to send the color in the question down to the car component.
https://codesandbox.io/s/my4wmn427x
Thank you! So with this approach where/how<Question/>
becomes aware of thequestionColor
sent down to<CarList />
?
– tonkihonks13
Nov 20 at 23:45
1
<Question> is not aware it's color is being sent to <CarList>. In this approach the garage is aware of both colors and chooses to additionally send down the question color to <CarList> so that each car can do a comparison against its own car color :)
– Shawn Andrews
Nov 21 at 5:25
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53402331%2freact-how-to-compare-props-between-separate-components%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Yes, you can pass the correct color to the CarList
component or the flag whether the CarList
is a correct one. Check my sandbox.
https://codesandbox.io/s/92xnwpyq6p
Basically we can add isCorrect
prop to CarList
which has value of correctCar.color === car.color
and we use it to determine whether we should render it green or red.
Thank you so much for the explanation and code example. Ideally red or green only triggers upon clicking. Prior to it, it should stay inblack
. Would/Should I massage this logic onclassName={.....}
?
– tonkihonks13
Nov 20 at 22:48
you can use empty string in that case. I think I also use it in my sandbox. Check it out
– jamesjaya
Nov 20 at 22:54
1
check my getColor() function
– jamesjaya
Nov 20 at 23:04
1
Garage.js line 62 correctIndex: Math.random() returns a random number between 0 and 1. I multiply it by the length of cars minus 1, means if the length is 5, the random number would be a random number between 0 and 4. Since I want an integer, I call Math.floor.
– jamesjaya
Nov 20 at 23:17
1
oh I forgot to hit save, you should be able to see it now
– jamesjaya
Nov 20 at 23:32
|
show 4 more comments
Yes, you can pass the correct color to the CarList
component or the flag whether the CarList
is a correct one. Check my sandbox.
https://codesandbox.io/s/92xnwpyq6p
Basically we can add isCorrect
prop to CarList
which has value of correctCar.color === car.color
and we use it to determine whether we should render it green or red.
Thank you so much for the explanation and code example. Ideally red or green only triggers upon clicking. Prior to it, it should stay inblack
. Would/Should I massage this logic onclassName={.....}
?
– tonkihonks13
Nov 20 at 22:48
you can use empty string in that case. I think I also use it in my sandbox. Check it out
– jamesjaya
Nov 20 at 22:54
1
check my getColor() function
– jamesjaya
Nov 20 at 23:04
1
Garage.js line 62 correctIndex: Math.random() returns a random number between 0 and 1. I multiply it by the length of cars minus 1, means if the length is 5, the random number would be a random number between 0 and 4. Since I want an integer, I call Math.floor.
– jamesjaya
Nov 20 at 23:17
1
oh I forgot to hit save, you should be able to see it now
– jamesjaya
Nov 20 at 23:32
|
show 4 more comments
Yes, you can pass the correct color to the CarList
component or the flag whether the CarList
is a correct one. Check my sandbox.
https://codesandbox.io/s/92xnwpyq6p
Basically we can add isCorrect
prop to CarList
which has value of correctCar.color === car.color
and we use it to determine whether we should render it green or red.
Yes, you can pass the correct color to the CarList
component or the flag whether the CarList
is a correct one. Check my sandbox.
https://codesandbox.io/s/92xnwpyq6p
Basically we can add isCorrect
prop to CarList
which has value of correctCar.color === car.color
and we use it to determine whether we should render it green or red.
answered Nov 20 at 22:38
jamesjaya
657412
657412
Thank you so much for the explanation and code example. Ideally red or green only triggers upon clicking. Prior to it, it should stay inblack
. Would/Should I massage this logic onclassName={.....}
?
– tonkihonks13
Nov 20 at 22:48
you can use empty string in that case. I think I also use it in my sandbox. Check it out
– jamesjaya
Nov 20 at 22:54
1
check my getColor() function
– jamesjaya
Nov 20 at 23:04
1
Garage.js line 62 correctIndex: Math.random() returns a random number between 0 and 1. I multiply it by the length of cars minus 1, means if the length is 5, the random number would be a random number between 0 and 4. Since I want an integer, I call Math.floor.
– jamesjaya
Nov 20 at 23:17
1
oh I forgot to hit save, you should be able to see it now
– jamesjaya
Nov 20 at 23:32
|
show 4 more comments
Thank you so much for the explanation and code example. Ideally red or green only triggers upon clicking. Prior to it, it should stay inblack
. Would/Should I massage this logic onclassName={.....}
?
– tonkihonks13
Nov 20 at 22:48
you can use empty string in that case. I think I also use it in my sandbox. Check it out
– jamesjaya
Nov 20 at 22:54
1
check my getColor() function
– jamesjaya
Nov 20 at 23:04
1
Garage.js line 62 correctIndex: Math.random() returns a random number between 0 and 1. I multiply it by the length of cars minus 1, means if the length is 5, the random number would be a random number between 0 and 4. Since I want an integer, I call Math.floor.
– jamesjaya
Nov 20 at 23:17
1
oh I forgot to hit save, you should be able to see it now
– jamesjaya
Nov 20 at 23:32
Thank you so much for the explanation and code example. Ideally red or green only triggers upon clicking. Prior to it, it should stay in
black
. Would/Should I massage this logic on className={.....}
?– tonkihonks13
Nov 20 at 22:48
Thank you so much for the explanation and code example. Ideally red or green only triggers upon clicking. Prior to it, it should stay in
black
. Would/Should I massage this logic on className={.....}
?– tonkihonks13
Nov 20 at 22:48
you can use empty string in that case. I think I also use it in my sandbox. Check it out
– jamesjaya
Nov 20 at 22:54
you can use empty string in that case. I think I also use it in my sandbox. Check it out
– jamesjaya
Nov 20 at 22:54
1
1
check my getColor() function
– jamesjaya
Nov 20 at 23:04
check my getColor() function
– jamesjaya
Nov 20 at 23:04
1
1
Garage.js line 62 correctIndex: Math.random() returns a random number between 0 and 1. I multiply it by the length of cars minus 1, means if the length is 5, the random number would be a random number between 0 and 4. Since I want an integer, I call Math.floor.
– jamesjaya
Nov 20 at 23:17
Garage.js line 62 correctIndex: Math.random() returns a random number between 0 and 1. I multiply it by the length of cars minus 1, means if the length is 5, the random number would be a random number between 0 and 4. Since I want an integer, I call Math.floor.
– jamesjaya
Nov 20 at 23:17
1
1
oh I forgot to hit save, you should be able to see it now
– jamesjaya
Nov 20 at 23:32
oh I forgot to hit save, you should be able to see it now
– jamesjaya
Nov 20 at 23:32
|
show 4 more comments
Theres many ways to do this but the simplest is to send the color in the question down to the car component.
https://codesandbox.io/s/my4wmn427x
Thank you! So with this approach where/how<Question/>
becomes aware of thequestionColor
sent down to<CarList />
?
– tonkihonks13
Nov 20 at 23:45
1
<Question> is not aware it's color is being sent to <CarList>. In this approach the garage is aware of both colors and chooses to additionally send down the question color to <CarList> so that each car can do a comparison against its own car color :)
– Shawn Andrews
Nov 21 at 5:25
add a comment |
Theres many ways to do this but the simplest is to send the color in the question down to the car component.
https://codesandbox.io/s/my4wmn427x
Thank you! So with this approach where/how<Question/>
becomes aware of thequestionColor
sent down to<CarList />
?
– tonkihonks13
Nov 20 at 23:45
1
<Question> is not aware it's color is being sent to <CarList>. In this approach the garage is aware of both colors and chooses to additionally send down the question color to <CarList> so that each car can do a comparison against its own car color :)
– Shawn Andrews
Nov 21 at 5:25
add a comment |
Theres many ways to do this but the simplest is to send the color in the question down to the car component.
https://codesandbox.io/s/my4wmn427x
Theres many ways to do this but the simplest is to send the color in the question down to the car component.
https://codesandbox.io/s/my4wmn427x
answered Nov 20 at 22:42


Shawn Andrews
945616
945616
Thank you! So with this approach where/how<Question/>
becomes aware of thequestionColor
sent down to<CarList />
?
– tonkihonks13
Nov 20 at 23:45
1
<Question> is not aware it's color is being sent to <CarList>. In this approach the garage is aware of both colors and chooses to additionally send down the question color to <CarList> so that each car can do a comparison against its own car color :)
– Shawn Andrews
Nov 21 at 5:25
add a comment |
Thank you! So with this approach where/how<Question/>
becomes aware of thequestionColor
sent down to<CarList />
?
– tonkihonks13
Nov 20 at 23:45
1
<Question> is not aware it's color is being sent to <CarList>. In this approach the garage is aware of both colors and chooses to additionally send down the question color to <CarList> so that each car can do a comparison against its own car color :)
– Shawn Andrews
Nov 21 at 5:25
Thank you! So with this approach where/how
<Question/>
becomes aware of the questionColor
sent down to <CarList />
?– tonkihonks13
Nov 20 at 23:45
Thank you! So with this approach where/how
<Question/>
becomes aware of the questionColor
sent down to <CarList />
?– tonkihonks13
Nov 20 at 23:45
1
1
<Question> is not aware it's color is being sent to <CarList>. In this approach the garage is aware of both colors and chooses to additionally send down the question color to <CarList> so that each car can do a comparison against its own car color :)
– Shawn Andrews
Nov 21 at 5:25
<Question> is not aware it's color is being sent to <CarList>. In this approach the garage is aware of both colors and chooses to additionally send down the question color to <CarList> so that each car can do a comparison against its own car color :)
– Shawn Andrews
Nov 21 at 5:25
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53402331%2freact-how-to-compare-props-between-separate-components%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ItdKIoxOuIp xn,1w0ZVYA W80vw,RcuR XULm2t,L5r,huiU1pEL5Bk7Yq,P