webpack-dev-server, historyApiFallback not working (webpack-4, react-router-4)
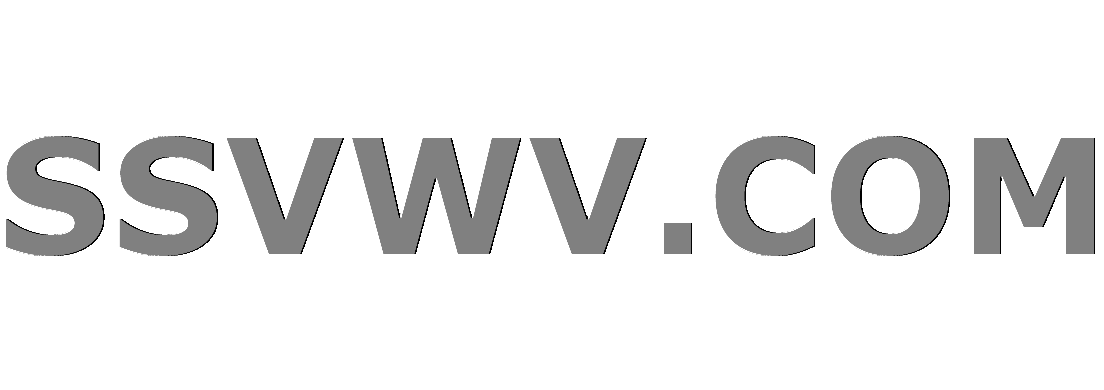
Multi tool use
up vote
0
down vote
favorite
I am testing out react-router4 with webpack4, but I can't get webpack-dev-server's setting:
{historyApiFallback: true}
to work. This use to work just fine in webpack3, so I am not sure what is wrong... Here is my webpack.config.js:
const HtmlWebpackPlugin = require('html-webpack-plugin');
module.exports = () => {
return {
mode: 'development',
devtool: 'source-map',
devServer: {
port: 8888,
historyApiFallback: true,
stats: 'minimal'
},
resolve: {
extensions: ['*', '.mjs', '.js', '.jsx']
},
module: {
rules: [
{
test: /.m?jsx?$/,
exclude: /(node_modules)/,
use: {
loader: 'babel-loader'
}
}
]
},
plugins: [
new HtmlWebpackPlugin({
title:'React Lab',
template: 'src/index.html'
})
]
}
}
and here is my simple react app with react-router4:
import React from 'react';
import ReactDOM from 'react-dom';
import {
BrowserRouter as Router,
Route,
Link,
Switch
} from 'react-router-dom';
const mountNode = document.getElementById('app');
const App = () => (
<Router>
<div>
<ul>
<li><Link to="/">Link to: /</Link></li>
<li><Link to="/page1">Link to: /page1</Link></li>
<li><Link to="/page2">Link to: /page2</Link></li>
<li><Link to="/does/not/exist">Link to: /does/not/exist</Link></li>
</ul>
<button onClick={()=>{location.reload();}}>reload page</button>
<Switch>
<Route exact path="/" component={()=>(<h2>home</h2>)} />
<Route exact path="/page1" component={()=>(<h2>page1</h2>)} />
<Route exact path="/page2" component={()=>(<h2>page2</h2>)} />
<Route component={()=>(<h2>no match</h2>)} />
</Switch>
<Route path="/" component={(props) =><div>{`props.location.pathname: ${props.location.pathname}`}</div>} />
</div>
</Router>
);
ReactDOM.render( <App/>, mountNode
After navigating to:
<Link to="/does/not/exist" />
and click
<button>reload page</button>
webpack dev server fail to redirect to main.js
Here is the complete code at github:
https://github.com/ApolloTang/webpack-dev-server-history-api-fall-back-not-working.
Any help or comment would greatly appreciated.
webpack react-router webpack-dev-server
add a comment |
up vote
0
down vote
favorite
I am testing out react-router4 with webpack4, but I can't get webpack-dev-server's setting:
{historyApiFallback: true}
to work. This use to work just fine in webpack3, so I am not sure what is wrong... Here is my webpack.config.js:
const HtmlWebpackPlugin = require('html-webpack-plugin');
module.exports = () => {
return {
mode: 'development',
devtool: 'source-map',
devServer: {
port: 8888,
historyApiFallback: true,
stats: 'minimal'
},
resolve: {
extensions: ['*', '.mjs', '.js', '.jsx']
},
module: {
rules: [
{
test: /.m?jsx?$/,
exclude: /(node_modules)/,
use: {
loader: 'babel-loader'
}
}
]
},
plugins: [
new HtmlWebpackPlugin({
title:'React Lab',
template: 'src/index.html'
})
]
}
}
and here is my simple react app with react-router4:
import React from 'react';
import ReactDOM from 'react-dom';
import {
BrowserRouter as Router,
Route,
Link,
Switch
} from 'react-router-dom';
const mountNode = document.getElementById('app');
const App = () => (
<Router>
<div>
<ul>
<li><Link to="/">Link to: /</Link></li>
<li><Link to="/page1">Link to: /page1</Link></li>
<li><Link to="/page2">Link to: /page2</Link></li>
<li><Link to="/does/not/exist">Link to: /does/not/exist</Link></li>
</ul>
<button onClick={()=>{location.reload();}}>reload page</button>
<Switch>
<Route exact path="/" component={()=>(<h2>home</h2>)} />
<Route exact path="/page1" component={()=>(<h2>page1</h2>)} />
<Route exact path="/page2" component={()=>(<h2>page2</h2>)} />
<Route component={()=>(<h2>no match</h2>)} />
</Switch>
<Route path="/" component={(props) =><div>{`props.location.pathname: ${props.location.pathname}`}</div>} />
</div>
</Router>
);
ReactDOM.render( <App/>, mountNode
After navigating to:
<Link to="/does/not/exist" />
and click
<button>reload page</button>
webpack dev server fail to redirect to main.js
Here is the complete code at github:
https://github.com/ApolloTang/webpack-dev-server-history-api-fall-back-not-working.
Any help or comment would greatly appreciated.
webpack react-router webpack-dev-server
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am testing out react-router4 with webpack4, but I can't get webpack-dev-server's setting:
{historyApiFallback: true}
to work. This use to work just fine in webpack3, so I am not sure what is wrong... Here is my webpack.config.js:
const HtmlWebpackPlugin = require('html-webpack-plugin');
module.exports = () => {
return {
mode: 'development',
devtool: 'source-map',
devServer: {
port: 8888,
historyApiFallback: true,
stats: 'minimal'
},
resolve: {
extensions: ['*', '.mjs', '.js', '.jsx']
},
module: {
rules: [
{
test: /.m?jsx?$/,
exclude: /(node_modules)/,
use: {
loader: 'babel-loader'
}
}
]
},
plugins: [
new HtmlWebpackPlugin({
title:'React Lab',
template: 'src/index.html'
})
]
}
}
and here is my simple react app with react-router4:
import React from 'react';
import ReactDOM from 'react-dom';
import {
BrowserRouter as Router,
Route,
Link,
Switch
} from 'react-router-dom';
const mountNode = document.getElementById('app');
const App = () => (
<Router>
<div>
<ul>
<li><Link to="/">Link to: /</Link></li>
<li><Link to="/page1">Link to: /page1</Link></li>
<li><Link to="/page2">Link to: /page2</Link></li>
<li><Link to="/does/not/exist">Link to: /does/not/exist</Link></li>
</ul>
<button onClick={()=>{location.reload();}}>reload page</button>
<Switch>
<Route exact path="/" component={()=>(<h2>home</h2>)} />
<Route exact path="/page1" component={()=>(<h2>page1</h2>)} />
<Route exact path="/page2" component={()=>(<h2>page2</h2>)} />
<Route component={()=>(<h2>no match</h2>)} />
</Switch>
<Route path="/" component={(props) =><div>{`props.location.pathname: ${props.location.pathname}`}</div>} />
</div>
</Router>
);
ReactDOM.render( <App/>, mountNode
After navigating to:
<Link to="/does/not/exist" />
and click
<button>reload page</button>
webpack dev server fail to redirect to main.js
Here is the complete code at github:
https://github.com/ApolloTang/webpack-dev-server-history-api-fall-back-not-working.
Any help or comment would greatly appreciated.
webpack react-router webpack-dev-server
I am testing out react-router4 with webpack4, but I can't get webpack-dev-server's setting:
{historyApiFallback: true}
to work. This use to work just fine in webpack3, so I am not sure what is wrong... Here is my webpack.config.js:
const HtmlWebpackPlugin = require('html-webpack-plugin');
module.exports = () => {
return {
mode: 'development',
devtool: 'source-map',
devServer: {
port: 8888,
historyApiFallback: true,
stats: 'minimal'
},
resolve: {
extensions: ['*', '.mjs', '.js', '.jsx']
},
module: {
rules: [
{
test: /.m?jsx?$/,
exclude: /(node_modules)/,
use: {
loader: 'babel-loader'
}
}
]
},
plugins: [
new HtmlWebpackPlugin({
title:'React Lab',
template: 'src/index.html'
})
]
}
}
and here is my simple react app with react-router4:
import React from 'react';
import ReactDOM from 'react-dom';
import {
BrowserRouter as Router,
Route,
Link,
Switch
} from 'react-router-dom';
const mountNode = document.getElementById('app');
const App = () => (
<Router>
<div>
<ul>
<li><Link to="/">Link to: /</Link></li>
<li><Link to="/page1">Link to: /page1</Link></li>
<li><Link to="/page2">Link to: /page2</Link></li>
<li><Link to="/does/not/exist">Link to: /does/not/exist</Link></li>
</ul>
<button onClick={()=>{location.reload();}}>reload page</button>
<Switch>
<Route exact path="/" component={()=>(<h2>home</h2>)} />
<Route exact path="/page1" component={()=>(<h2>page1</h2>)} />
<Route exact path="/page2" component={()=>(<h2>page2</h2>)} />
<Route component={()=>(<h2>no match</h2>)} />
</Switch>
<Route path="/" component={(props) =><div>{`props.location.pathname: ${props.location.pathname}`}</div>} />
</div>
</Router>
);
ReactDOM.render( <App/>, mountNode
After navigating to:
<Link to="/does/not/exist" />
and click
<button>reload page</button>
webpack dev server fail to redirect to main.js
Here is the complete code at github:
https://github.com/ApolloTang/webpack-dev-server-history-api-fall-back-not-working.
Any help or comment would greatly appreciated.
webpack react-router webpack-dev-server
webpack react-router webpack-dev-server
asked Nov 20 at 6:58
apollo
12519
12519
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
It turns out that I am missing output.publicPath in webpack.config.js:
output: {
// must specified output.publicPath otherwise
// devServer.historyApiFallback will not work
publicPath: '/'
},
with output.publicPath specified as above, historyApiFallback works.
I can't remember where I have read that said output.publicPath is an optional in webpack4's configuration, but it does required to work with webpack-dev-server.
The documentation at https://webpack.js.org/configuration/output/#output-publicpath said:
The webpack-dev-server also takes a hint from publicPath, using it to
determine where to serve the output files from.
But I don't understand how is this to do with hisitoryApiFallback.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
It turns out that I am missing output.publicPath in webpack.config.js:
output: {
// must specified output.publicPath otherwise
// devServer.historyApiFallback will not work
publicPath: '/'
},
with output.publicPath specified as above, historyApiFallback works.
I can't remember where I have read that said output.publicPath is an optional in webpack4's configuration, but it does required to work with webpack-dev-server.
The documentation at https://webpack.js.org/configuration/output/#output-publicpath said:
The webpack-dev-server also takes a hint from publicPath, using it to
determine where to serve the output files from.
But I don't understand how is this to do with hisitoryApiFallback.
add a comment |
up vote
0
down vote
It turns out that I am missing output.publicPath in webpack.config.js:
output: {
// must specified output.publicPath otherwise
// devServer.historyApiFallback will not work
publicPath: '/'
},
with output.publicPath specified as above, historyApiFallback works.
I can't remember where I have read that said output.publicPath is an optional in webpack4's configuration, but it does required to work with webpack-dev-server.
The documentation at https://webpack.js.org/configuration/output/#output-publicpath said:
The webpack-dev-server also takes a hint from publicPath, using it to
determine where to serve the output files from.
But I don't understand how is this to do with hisitoryApiFallback.
add a comment |
up vote
0
down vote
up vote
0
down vote
It turns out that I am missing output.publicPath in webpack.config.js:
output: {
// must specified output.publicPath otherwise
// devServer.historyApiFallback will not work
publicPath: '/'
},
with output.publicPath specified as above, historyApiFallback works.
I can't remember where I have read that said output.publicPath is an optional in webpack4's configuration, but it does required to work with webpack-dev-server.
The documentation at https://webpack.js.org/configuration/output/#output-publicpath said:
The webpack-dev-server also takes a hint from publicPath, using it to
determine where to serve the output files from.
But I don't understand how is this to do with hisitoryApiFallback.
It turns out that I am missing output.publicPath in webpack.config.js:
output: {
// must specified output.publicPath otherwise
// devServer.historyApiFallback will not work
publicPath: '/'
},
with output.publicPath specified as above, historyApiFallback works.
I can't remember where I have read that said output.publicPath is an optional in webpack4's configuration, but it does required to work with webpack-dev-server.
The documentation at https://webpack.js.org/configuration/output/#output-publicpath said:
The webpack-dev-server also takes a hint from publicPath, using it to
determine where to serve the output files from.
But I don't understand how is this to do with hisitoryApiFallback.
answered Nov 21 at 7:09
apollo
12519
12519
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53387756%2fwebpack-dev-server-historyapifallback-not-working-webpack-4-react-router-4%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ir8bHKuZVXKSTaO8Il7wK ZdatzmUNPqxc3