Subscribing on topic. MQTT
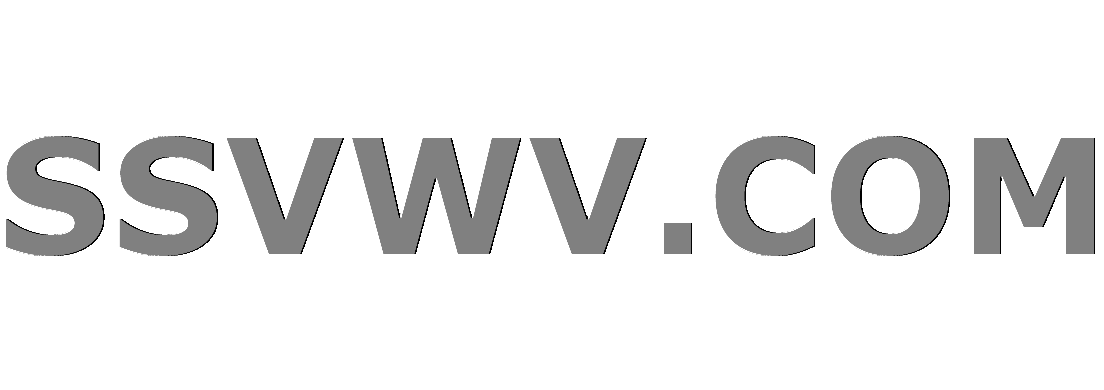
Multi tool use
I have the following part of code:
package com.company;
import org.eclipse.paho.client.mqttv3.*;
import org.eclipse.paho.client.mqttv3.persist.MemoryPersistence;
import javax.security.auth.callback.Callback;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class Main implements MqttCallback {
private static String sTopic;
private static int iQos;
private static MqttClient mqttClient;
private static String sUsername;
private static Frame frame = new Frame();
public static void main(String args) throws MqttException {
frame.getConnect().addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
int iPort;
String sIp = frame.getBrokerAddressValue();
sUsername = frame.getUsernameValue();
try {
String broker = "tcp://"; //bridge and host
iPort = frame.getPortValue();
broker+=sIp+":"+iPort;
mqttClient = new MqttClient(broker, sUsername, new MemoryPersistence()); //URI, ClientId, Persistence
MqttConnectOptions connectOptions = new MqttConnectOptions();
connectOptions.setCleanSession(true);
System.out.println("Connecting to broker: "+broker);
mqttClient.connect();
System.out.println("Connected");
}catch (NumberFormatException exc){
System.out.println("Wrong port format");
} catch (MqttException e1) {
e1.printStackTrace();
}
}
});
frame.getSubscribe().addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
sTopic = frame.getTopicValue();
try {
mqttClient.subscribe(sTopic);
} catch (MqttException e1) {
e1.printStackTrace();
}
System.out.println("Subscribed");
}
});
frame.getPublish().addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String sMessage = frame.getMessageValue();
MqttMessage message = new MqttMessage(sMessage.getBytes());
iQos = frame.getQosValue();
message.setQos(iQos);
try {
mqttClient.publish(sTopic,message);
} catch (MqttException e1) {
e1.printStackTrace();
}
System.out.println("Message published");
}
});
}
@Override
public void connectionLost(Throwable throwable) {
}
@Override
public void messageArrived(String topic, MqttMessage message) throws Exception{
frame.getTextArea().setText(String.valueOf(message));
}
@Override
public void deliveryComplete(IMqttDeliveryToken iMqttDeliveryToken) {
}
}
Here's a part of my realization of the mqtt client. Method getConnect is processing a click on button 'Connect', method getSubscribe is processesing a click on button 'Subscribe', method getPublish is processing a click on button 'Publish'.The problem is the following: when I subscribe on the topic, messages don't arrive on the clients, which are subscribed on this topic. What's the matter?
java mqtt
add a comment |
I have the following part of code:
package com.company;
import org.eclipse.paho.client.mqttv3.*;
import org.eclipse.paho.client.mqttv3.persist.MemoryPersistence;
import javax.security.auth.callback.Callback;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class Main implements MqttCallback {
private static String sTopic;
private static int iQos;
private static MqttClient mqttClient;
private static String sUsername;
private static Frame frame = new Frame();
public static void main(String args) throws MqttException {
frame.getConnect().addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
int iPort;
String sIp = frame.getBrokerAddressValue();
sUsername = frame.getUsernameValue();
try {
String broker = "tcp://"; //bridge and host
iPort = frame.getPortValue();
broker+=sIp+":"+iPort;
mqttClient = new MqttClient(broker, sUsername, new MemoryPersistence()); //URI, ClientId, Persistence
MqttConnectOptions connectOptions = new MqttConnectOptions();
connectOptions.setCleanSession(true);
System.out.println("Connecting to broker: "+broker);
mqttClient.connect();
System.out.println("Connected");
}catch (NumberFormatException exc){
System.out.println("Wrong port format");
} catch (MqttException e1) {
e1.printStackTrace();
}
}
});
frame.getSubscribe().addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
sTopic = frame.getTopicValue();
try {
mqttClient.subscribe(sTopic);
} catch (MqttException e1) {
e1.printStackTrace();
}
System.out.println("Subscribed");
}
});
frame.getPublish().addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String sMessage = frame.getMessageValue();
MqttMessage message = new MqttMessage(sMessage.getBytes());
iQos = frame.getQosValue();
message.setQos(iQos);
try {
mqttClient.publish(sTopic,message);
} catch (MqttException e1) {
e1.printStackTrace();
}
System.out.println("Message published");
}
});
}
@Override
public void connectionLost(Throwable throwable) {
}
@Override
public void messageArrived(String topic, MqttMessage message) throws Exception{
frame.getTextArea().setText(String.valueOf(message));
}
@Override
public void deliveryComplete(IMqttDeliveryToken iMqttDeliveryToken) {
}
}
Here's a part of my realization of the mqtt client. Method getConnect is processing a click on button 'Connect', method getSubscribe is processesing a click on button 'Subscribe', method getPublish is processing a click on button 'Publish'.The problem is the following: when I subscribe on the topic, messages don't arrive on the clients, which are subscribed on this topic. What's the matter?
java mqtt
subscribing is not the same as publishing....
– ΦXocę 웃 Пepeúpa ツ
Nov 20 at 17:50
1
@ΦXocę웃Пepeúpaツ,yes,I know. I have two methods: one for publishing and one for subscribing
– Sergei Mikhailovskii
Nov 20 at 17:55
add a comment |
I have the following part of code:
package com.company;
import org.eclipse.paho.client.mqttv3.*;
import org.eclipse.paho.client.mqttv3.persist.MemoryPersistence;
import javax.security.auth.callback.Callback;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class Main implements MqttCallback {
private static String sTopic;
private static int iQos;
private static MqttClient mqttClient;
private static String sUsername;
private static Frame frame = new Frame();
public static void main(String args) throws MqttException {
frame.getConnect().addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
int iPort;
String sIp = frame.getBrokerAddressValue();
sUsername = frame.getUsernameValue();
try {
String broker = "tcp://"; //bridge and host
iPort = frame.getPortValue();
broker+=sIp+":"+iPort;
mqttClient = new MqttClient(broker, sUsername, new MemoryPersistence()); //URI, ClientId, Persistence
MqttConnectOptions connectOptions = new MqttConnectOptions();
connectOptions.setCleanSession(true);
System.out.println("Connecting to broker: "+broker);
mqttClient.connect();
System.out.println("Connected");
}catch (NumberFormatException exc){
System.out.println("Wrong port format");
} catch (MqttException e1) {
e1.printStackTrace();
}
}
});
frame.getSubscribe().addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
sTopic = frame.getTopicValue();
try {
mqttClient.subscribe(sTopic);
} catch (MqttException e1) {
e1.printStackTrace();
}
System.out.println("Subscribed");
}
});
frame.getPublish().addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String sMessage = frame.getMessageValue();
MqttMessage message = new MqttMessage(sMessage.getBytes());
iQos = frame.getQosValue();
message.setQos(iQos);
try {
mqttClient.publish(sTopic,message);
} catch (MqttException e1) {
e1.printStackTrace();
}
System.out.println("Message published");
}
});
}
@Override
public void connectionLost(Throwable throwable) {
}
@Override
public void messageArrived(String topic, MqttMessage message) throws Exception{
frame.getTextArea().setText(String.valueOf(message));
}
@Override
public void deliveryComplete(IMqttDeliveryToken iMqttDeliveryToken) {
}
}
Here's a part of my realization of the mqtt client. Method getConnect is processing a click on button 'Connect', method getSubscribe is processesing a click on button 'Subscribe', method getPublish is processing a click on button 'Publish'.The problem is the following: when I subscribe on the topic, messages don't arrive on the clients, which are subscribed on this topic. What's the matter?
java mqtt
I have the following part of code:
package com.company;
import org.eclipse.paho.client.mqttv3.*;
import org.eclipse.paho.client.mqttv3.persist.MemoryPersistence;
import javax.security.auth.callback.Callback;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class Main implements MqttCallback {
private static String sTopic;
private static int iQos;
private static MqttClient mqttClient;
private static String sUsername;
private static Frame frame = new Frame();
public static void main(String args) throws MqttException {
frame.getConnect().addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
int iPort;
String sIp = frame.getBrokerAddressValue();
sUsername = frame.getUsernameValue();
try {
String broker = "tcp://"; //bridge and host
iPort = frame.getPortValue();
broker+=sIp+":"+iPort;
mqttClient = new MqttClient(broker, sUsername, new MemoryPersistence()); //URI, ClientId, Persistence
MqttConnectOptions connectOptions = new MqttConnectOptions();
connectOptions.setCleanSession(true);
System.out.println("Connecting to broker: "+broker);
mqttClient.connect();
System.out.println("Connected");
}catch (NumberFormatException exc){
System.out.println("Wrong port format");
} catch (MqttException e1) {
e1.printStackTrace();
}
}
});
frame.getSubscribe().addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
sTopic = frame.getTopicValue();
try {
mqttClient.subscribe(sTopic);
} catch (MqttException e1) {
e1.printStackTrace();
}
System.out.println("Subscribed");
}
});
frame.getPublish().addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String sMessage = frame.getMessageValue();
MqttMessage message = new MqttMessage(sMessage.getBytes());
iQos = frame.getQosValue();
message.setQos(iQos);
try {
mqttClient.publish(sTopic,message);
} catch (MqttException e1) {
e1.printStackTrace();
}
System.out.println("Message published");
}
});
}
@Override
public void connectionLost(Throwable throwable) {
}
@Override
public void messageArrived(String topic, MqttMessage message) throws Exception{
frame.getTextArea().setText(String.valueOf(message));
}
@Override
public void deliveryComplete(IMqttDeliveryToken iMqttDeliveryToken) {
}
}
Here's a part of my realization of the mqtt client. Method getConnect is processing a click on button 'Connect', method getSubscribe is processesing a click on button 'Subscribe', method getPublish is processing a click on button 'Publish'.The problem is the following: when I subscribe on the topic, messages don't arrive on the clients, which are subscribed on this topic. What's the matter?
java mqtt
java mqtt
asked Nov 20 at 17:46


Sergei Mikhailovskii
847
847
subscribing is not the same as publishing....
– ΦXocę 웃 Пepeúpa ツ
Nov 20 at 17:50
1
@ΦXocę웃Пepeúpaツ,yes,I know. I have two methods: one for publishing and one for subscribing
– Sergei Mikhailovskii
Nov 20 at 17:55
add a comment |
subscribing is not the same as publishing....
– ΦXocę 웃 Пepeúpa ツ
Nov 20 at 17:50
1
@ΦXocę웃Пepeúpaツ,yes,I know. I have two methods: one for publishing and one for subscribing
– Sergei Mikhailovskii
Nov 20 at 17:55
subscribing is not the same as publishing....
– ΦXocę 웃 Пepeúpa ツ
Nov 20 at 17:50
subscribing is not the same as publishing....
– ΦXocę 웃 Пepeúpa ツ
Nov 20 at 17:50
1
1
@ΦXocę웃Пepeúpaツ,yes,I know. I have two methods: one for publishing and one for subscribing
– Sergei Mikhailovskii
Nov 20 at 17:55
@ΦXocę웃Пepeúpaツ,yes,I know. I have two methods: one for publishing and one for subscribing
– Sergei Mikhailovskii
Nov 20 at 17:55
add a comment |
1 Answer
1
active
oldest
votes
If I understand your question correctly,You are subscribed to a topic 'sTopic' but you are not receiving messages when some one publish message to the topic 'sTopic'.
Are you sure Mqtt client is connected successfully to broker ? Make mqtt client is connected before making subscribe call.
if( mqttClient.isConnected()) {
mqttClient.subscribe(sTopic);
}
and same applies for publish also.
if( mqttClient.isConnected()) {
mqttClient.publish(sTopic,message);
}
Once if these call goes, you should see published messages in messageArrived call back method.
You need to set the callback method to mqttClient
clientCallback = new MqttCallback() {
@Override
public void connectionLost(Throwable cause) {
}
@Override
public void messageArrived(String topic, MqttMessage message) throws Exception {
}
@Override
public void deliveryComplete(IMqttDeliveryToken token) {
}
};
mqttClient.setCallback(clientCallback);
1
Yes, you understood me right. I used in debugger and yes, client is connected in all cases. I probably think that there's the problem in publish method. I saw some examples of clients and there is the same line in all of them: client.setCallback(this); But I don't know how to add it to my project, as arguement 'this' is always underlined and writes that setCallback (org.eclipse.paho.client.mqttv3.MqttCallback) in MqttClient cannot be applied to (anonymous java.awt.event.ActionListener)
– Sergei Mikhailovskii
Nov 20 at 20:06
Try that mqttCallback.. It should work.
– Ramesh Yankati
Nov 20 at 20:29
2
yes, now it works, thanks. So, as I understand, there's no need in connectionLost, messageArrived and deliveryComplete methods in the end of the class?
– Sergei Mikhailovskii
Nov 20 at 20:47
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53398683%2fsubscribing-on-topic-mqtt%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
If I understand your question correctly,You are subscribed to a topic 'sTopic' but you are not receiving messages when some one publish message to the topic 'sTopic'.
Are you sure Mqtt client is connected successfully to broker ? Make mqtt client is connected before making subscribe call.
if( mqttClient.isConnected()) {
mqttClient.subscribe(sTopic);
}
and same applies for publish also.
if( mqttClient.isConnected()) {
mqttClient.publish(sTopic,message);
}
Once if these call goes, you should see published messages in messageArrived call back method.
You need to set the callback method to mqttClient
clientCallback = new MqttCallback() {
@Override
public void connectionLost(Throwable cause) {
}
@Override
public void messageArrived(String topic, MqttMessage message) throws Exception {
}
@Override
public void deliveryComplete(IMqttDeliveryToken token) {
}
};
mqttClient.setCallback(clientCallback);
1
Yes, you understood me right. I used in debugger and yes, client is connected in all cases. I probably think that there's the problem in publish method. I saw some examples of clients and there is the same line in all of them: client.setCallback(this); But I don't know how to add it to my project, as arguement 'this' is always underlined and writes that setCallback (org.eclipse.paho.client.mqttv3.MqttCallback) in MqttClient cannot be applied to (anonymous java.awt.event.ActionListener)
– Sergei Mikhailovskii
Nov 20 at 20:06
Try that mqttCallback.. It should work.
– Ramesh Yankati
Nov 20 at 20:29
2
yes, now it works, thanks. So, as I understand, there's no need in connectionLost, messageArrived and deliveryComplete methods in the end of the class?
– Sergei Mikhailovskii
Nov 20 at 20:47
add a comment |
If I understand your question correctly,You are subscribed to a topic 'sTopic' but you are not receiving messages when some one publish message to the topic 'sTopic'.
Are you sure Mqtt client is connected successfully to broker ? Make mqtt client is connected before making subscribe call.
if( mqttClient.isConnected()) {
mqttClient.subscribe(sTopic);
}
and same applies for publish also.
if( mqttClient.isConnected()) {
mqttClient.publish(sTopic,message);
}
Once if these call goes, you should see published messages in messageArrived call back method.
You need to set the callback method to mqttClient
clientCallback = new MqttCallback() {
@Override
public void connectionLost(Throwable cause) {
}
@Override
public void messageArrived(String topic, MqttMessage message) throws Exception {
}
@Override
public void deliveryComplete(IMqttDeliveryToken token) {
}
};
mqttClient.setCallback(clientCallback);
1
Yes, you understood me right. I used in debugger and yes, client is connected in all cases. I probably think that there's the problem in publish method. I saw some examples of clients and there is the same line in all of them: client.setCallback(this); But I don't know how to add it to my project, as arguement 'this' is always underlined and writes that setCallback (org.eclipse.paho.client.mqttv3.MqttCallback) in MqttClient cannot be applied to (anonymous java.awt.event.ActionListener)
– Sergei Mikhailovskii
Nov 20 at 20:06
Try that mqttCallback.. It should work.
– Ramesh Yankati
Nov 20 at 20:29
2
yes, now it works, thanks. So, as I understand, there's no need in connectionLost, messageArrived and deliveryComplete methods in the end of the class?
– Sergei Mikhailovskii
Nov 20 at 20:47
add a comment |
If I understand your question correctly,You are subscribed to a topic 'sTopic' but you are not receiving messages when some one publish message to the topic 'sTopic'.
Are you sure Mqtt client is connected successfully to broker ? Make mqtt client is connected before making subscribe call.
if( mqttClient.isConnected()) {
mqttClient.subscribe(sTopic);
}
and same applies for publish also.
if( mqttClient.isConnected()) {
mqttClient.publish(sTopic,message);
}
Once if these call goes, you should see published messages in messageArrived call back method.
You need to set the callback method to mqttClient
clientCallback = new MqttCallback() {
@Override
public void connectionLost(Throwable cause) {
}
@Override
public void messageArrived(String topic, MqttMessage message) throws Exception {
}
@Override
public void deliveryComplete(IMqttDeliveryToken token) {
}
};
mqttClient.setCallback(clientCallback);
If I understand your question correctly,You are subscribed to a topic 'sTopic' but you are not receiving messages when some one publish message to the topic 'sTopic'.
Are you sure Mqtt client is connected successfully to broker ? Make mqtt client is connected before making subscribe call.
if( mqttClient.isConnected()) {
mqttClient.subscribe(sTopic);
}
and same applies for publish also.
if( mqttClient.isConnected()) {
mqttClient.publish(sTopic,message);
}
Once if these call goes, you should see published messages in messageArrived call back method.
You need to set the callback method to mqttClient
clientCallback = new MqttCallback() {
@Override
public void connectionLost(Throwable cause) {
}
@Override
public void messageArrived(String topic, MqttMessage message) throws Exception {
}
@Override
public void deliveryComplete(IMqttDeliveryToken token) {
}
};
mqttClient.setCallback(clientCallback);
edited Nov 20 at 20:28
answered Nov 20 at 18:37
Ramesh Yankati
63848
63848
1
Yes, you understood me right. I used in debugger and yes, client is connected in all cases. I probably think that there's the problem in publish method. I saw some examples of clients and there is the same line in all of them: client.setCallback(this); But I don't know how to add it to my project, as arguement 'this' is always underlined and writes that setCallback (org.eclipse.paho.client.mqttv3.MqttCallback) in MqttClient cannot be applied to (anonymous java.awt.event.ActionListener)
– Sergei Mikhailovskii
Nov 20 at 20:06
Try that mqttCallback.. It should work.
– Ramesh Yankati
Nov 20 at 20:29
2
yes, now it works, thanks. So, as I understand, there's no need in connectionLost, messageArrived and deliveryComplete methods in the end of the class?
– Sergei Mikhailovskii
Nov 20 at 20:47
add a comment |
1
Yes, you understood me right. I used in debugger and yes, client is connected in all cases. I probably think that there's the problem in publish method. I saw some examples of clients and there is the same line in all of them: client.setCallback(this); But I don't know how to add it to my project, as arguement 'this' is always underlined and writes that setCallback (org.eclipse.paho.client.mqttv3.MqttCallback) in MqttClient cannot be applied to (anonymous java.awt.event.ActionListener)
– Sergei Mikhailovskii
Nov 20 at 20:06
Try that mqttCallback.. It should work.
– Ramesh Yankati
Nov 20 at 20:29
2
yes, now it works, thanks. So, as I understand, there's no need in connectionLost, messageArrived and deliveryComplete methods in the end of the class?
– Sergei Mikhailovskii
Nov 20 at 20:47
1
1
Yes, you understood me right. I used in debugger and yes, client is connected in all cases. I probably think that there's the problem in publish method. I saw some examples of clients and there is the same line in all of them: client.setCallback(this); But I don't know how to add it to my project, as arguement 'this' is always underlined and writes that setCallback (org.eclipse.paho.client.mqttv3.MqttCallback) in MqttClient cannot be applied to (anonymous java.awt.event.ActionListener)
– Sergei Mikhailovskii
Nov 20 at 20:06
Yes, you understood me right. I used in debugger and yes, client is connected in all cases. I probably think that there's the problem in publish method. I saw some examples of clients and there is the same line in all of them: client.setCallback(this); But I don't know how to add it to my project, as arguement 'this' is always underlined and writes that setCallback (org.eclipse.paho.client.mqttv3.MqttCallback) in MqttClient cannot be applied to (anonymous java.awt.event.ActionListener)
– Sergei Mikhailovskii
Nov 20 at 20:06
Try that mqttCallback.. It should work.
– Ramesh Yankati
Nov 20 at 20:29
Try that mqttCallback.. It should work.
– Ramesh Yankati
Nov 20 at 20:29
2
2
yes, now it works, thanks. So, as I understand, there's no need in connectionLost, messageArrived and deliveryComplete methods in the end of the class?
– Sergei Mikhailovskii
Nov 20 at 20:47
yes, now it works, thanks. So, as I understand, there's no need in connectionLost, messageArrived and deliveryComplete methods in the end of the class?
– Sergei Mikhailovskii
Nov 20 at 20:47
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53398683%2fsubscribing-on-topic-mqtt%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
3Q12Y2c3w0yUFBQ,ZmymzT
subscribing is not the same as publishing....
– ΦXocę 웃 Пepeúpa ツ
Nov 20 at 17:50
1
@ΦXocę웃Пepeúpaツ,yes,I know. I have two methods: one for publishing and one for subscribing
– Sergei Mikhailovskii
Nov 20 at 17:55