I can not open the movie details when I click on the movie card
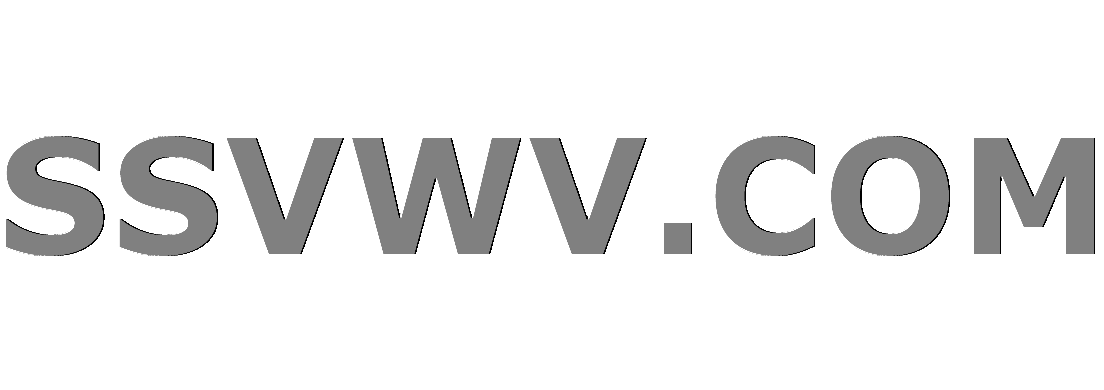
Multi tool use
This is my code that shows popular movies from a TMDB API key. I tried different solutions but always returns the same error, that is, I do not open the details of the film when I click on the card.
import React, { Component } from 'react';
import axios from 'axios';
import { createStackNavigator } from 'react-navigation';
import { Platform, StyleSheet, View, Text, ScrollView, Image } from 'react-native';
import { Card, CardItem, Icon, List } from 'native-base';
class Popular extends Component {
constructor(props){
super(props);
this.state= {
moviesList:
}
}
componentWillMount(){
axios.get('https://api.themoviedb.org/3/movie/popular?api_key=MY_API_KEY&language=it-IT&page=1').then(response => {
this.setState({ moviesList: response.data.results });
});
}
// This is a function that open movie details
viewDetails() {
const url = "https://www.themoviedb.org/movie/"
window.location.href = url
}
render () {
const ImgURL = 'http://image.tmdb.org/t/p/original';
const movies = this.state.moviesList.map(movie => {
return (
<Card key={ movie } style={{width: 135, marginRight: 15}}>
<CardItem cardBody button onPress={this.viewDetails}> // <-- here gives me the error by not opening the window with the details of the film
<Image source={{uri: ImgURL + movie.poster_path}} style={{width: 135, height: 185}}/>
</CardItem>
<CardItem style={{flex: 1, justifyContent: 'center'}}>
<Text>{movie.title}</Text>
</CardItem>
</Card>
)
});
return(
<ScrollView horizontal={true} showsHorizontalScrollIndicator={false}>
{ movies }
</ScrollView>
)
}
}
export default Popular;
javascript reactjs api react-native
add a comment |
This is my code that shows popular movies from a TMDB API key. I tried different solutions but always returns the same error, that is, I do not open the details of the film when I click on the card.
import React, { Component } from 'react';
import axios from 'axios';
import { createStackNavigator } from 'react-navigation';
import { Platform, StyleSheet, View, Text, ScrollView, Image } from 'react-native';
import { Card, CardItem, Icon, List } from 'native-base';
class Popular extends Component {
constructor(props){
super(props);
this.state= {
moviesList:
}
}
componentWillMount(){
axios.get('https://api.themoviedb.org/3/movie/popular?api_key=MY_API_KEY&language=it-IT&page=1').then(response => {
this.setState({ moviesList: response.data.results });
});
}
// This is a function that open movie details
viewDetails() {
const url = "https://www.themoviedb.org/movie/"
window.location.href = url
}
render () {
const ImgURL = 'http://image.tmdb.org/t/p/original';
const movies = this.state.moviesList.map(movie => {
return (
<Card key={ movie } style={{width: 135, marginRight: 15}}>
<CardItem cardBody button onPress={this.viewDetails}> // <-- here gives me the error by not opening the window with the details of the film
<Image source={{uri: ImgURL + movie.poster_path}} style={{width: 135, height: 185}}/>
</CardItem>
<CardItem style={{flex: 1, justifyContent: 'center'}}>
<Text>{movie.title}</Text>
</CardItem>
</Card>
)
});
return(
<ScrollView horizontal={true} showsHorizontalScrollIndicator={false}>
{ movies }
</ScrollView>
)
}
}
export default Popular;
javascript reactjs api react-native
Hi, welcome to Stack Overflow! I've removed the snippet since your code is not executable in the browser. I also removed a tag from the title, since there's no need for redundancy :)
– Federico Grandi
Nov 24 '18 at 14:08
add a comment |
This is my code that shows popular movies from a TMDB API key. I tried different solutions but always returns the same error, that is, I do not open the details of the film when I click on the card.
import React, { Component } from 'react';
import axios from 'axios';
import { createStackNavigator } from 'react-navigation';
import { Platform, StyleSheet, View, Text, ScrollView, Image } from 'react-native';
import { Card, CardItem, Icon, List } from 'native-base';
class Popular extends Component {
constructor(props){
super(props);
this.state= {
moviesList:
}
}
componentWillMount(){
axios.get('https://api.themoviedb.org/3/movie/popular?api_key=MY_API_KEY&language=it-IT&page=1').then(response => {
this.setState({ moviesList: response.data.results });
});
}
// This is a function that open movie details
viewDetails() {
const url = "https://www.themoviedb.org/movie/"
window.location.href = url
}
render () {
const ImgURL = 'http://image.tmdb.org/t/p/original';
const movies = this.state.moviesList.map(movie => {
return (
<Card key={ movie } style={{width: 135, marginRight: 15}}>
<CardItem cardBody button onPress={this.viewDetails}> // <-- here gives me the error by not opening the window with the details of the film
<Image source={{uri: ImgURL + movie.poster_path}} style={{width: 135, height: 185}}/>
</CardItem>
<CardItem style={{flex: 1, justifyContent: 'center'}}>
<Text>{movie.title}</Text>
</CardItem>
</Card>
)
});
return(
<ScrollView horizontal={true} showsHorizontalScrollIndicator={false}>
{ movies }
</ScrollView>
)
}
}
export default Popular;
javascript reactjs api react-native
This is my code that shows popular movies from a TMDB API key. I tried different solutions but always returns the same error, that is, I do not open the details of the film when I click on the card.
import React, { Component } from 'react';
import axios from 'axios';
import { createStackNavigator } from 'react-navigation';
import { Platform, StyleSheet, View, Text, ScrollView, Image } from 'react-native';
import { Card, CardItem, Icon, List } from 'native-base';
class Popular extends Component {
constructor(props){
super(props);
this.state= {
moviesList:
}
}
componentWillMount(){
axios.get('https://api.themoviedb.org/3/movie/popular?api_key=MY_API_KEY&language=it-IT&page=1').then(response => {
this.setState({ moviesList: response.data.results });
});
}
// This is a function that open movie details
viewDetails() {
const url = "https://www.themoviedb.org/movie/"
window.location.href = url
}
render () {
const ImgURL = 'http://image.tmdb.org/t/p/original';
const movies = this.state.moviesList.map(movie => {
return (
<Card key={ movie } style={{width: 135, marginRight: 15}}>
<CardItem cardBody button onPress={this.viewDetails}> // <-- here gives me the error by not opening the window with the details of the film
<Image source={{uri: ImgURL + movie.poster_path}} style={{width: 135, height: 185}}/>
</CardItem>
<CardItem style={{flex: 1, justifyContent: 'center'}}>
<Text>{movie.title}</Text>
</CardItem>
</Card>
)
});
return(
<ScrollView horizontal={true} showsHorizontalScrollIndicator={false}>
{ movies }
</ScrollView>
)
}
}
export default Popular;
javascript reactjs api react-native
javascript reactjs api react-native
edited Nov 24 '18 at 14:08


Federico Grandi
3,08821229
3,08821229
asked Nov 23 '18 at 19:11


Antonio FiorilloAntonio Fiorillo
11
11
Hi, welcome to Stack Overflow! I've removed the snippet since your code is not executable in the browser. I also removed a tag from the title, since there's no need for redundancy :)
– Federico Grandi
Nov 24 '18 at 14:08
add a comment |
Hi, welcome to Stack Overflow! I've removed the snippet since your code is not executable in the browser. I also removed a tag from the title, since there's no need for redundancy :)
– Federico Grandi
Nov 24 '18 at 14:08
Hi, welcome to Stack Overflow! I've removed the snippet since your code is not executable in the browser. I also removed a tag from the title, since there's no need for redundancy :)
– Federico Grandi
Nov 24 '18 at 14:08
Hi, welcome to Stack Overflow! I've removed the snippet since your code is not executable in the browser. I also removed a tag from the title, since there's no need for redundancy :)
– Federico Grandi
Nov 24 '18 at 14:08
add a comment |
1 Answer
1
active
oldest
votes
React Native has no window object so the following wont work
const url = "https://www.themoviedb.org/movie/"
window.location.href = url
If all you need is to open a url you can use WebView https://facebook.github.io/react-native/docs/webview. You can either navigate to a new screen or just open a modal for it.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53451888%2fi-can-not-open-the-movie-details-when-i-click-on-the-movie-card%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
React Native has no window object so the following wont work
const url = "https://www.themoviedb.org/movie/"
window.location.href = url
If all you need is to open a url you can use WebView https://facebook.github.io/react-native/docs/webview. You can either navigate to a new screen or just open a modal for it.
add a comment |
React Native has no window object so the following wont work
const url = "https://www.themoviedb.org/movie/"
window.location.href = url
If all you need is to open a url you can use WebView https://facebook.github.io/react-native/docs/webview. You can either navigate to a new screen or just open a modal for it.
add a comment |
React Native has no window object so the following wont work
const url = "https://www.themoviedb.org/movie/"
window.location.href = url
If all you need is to open a url you can use WebView https://facebook.github.io/react-native/docs/webview. You can either navigate to a new screen or just open a modal for it.
React Native has no window object so the following wont work
const url = "https://www.themoviedb.org/movie/"
window.location.href = url
If all you need is to open a url you can use WebView https://facebook.github.io/react-native/docs/webview. You can either navigate to a new screen or just open a modal for it.
answered Nov 23 '18 at 20:03
YongzhiYongzhi
6881411
6881411
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53451888%2fi-can-not-open-the-movie-details-when-i-click-on-the-movie-card%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
VOnJqea0r 1wxls3zbkbDJ,f0D7h31,JyaccH0Jd kU,tQSiD8kvvG96V89CzaD4XD R7hb BX9IncS78JW5f6XjiajPyKn6Jg3,CnCG
Hi, welcome to Stack Overflow! I've removed the snippet since your code is not executable in the browser. I also removed a tag from the title, since there's no need for redundancy :)
– Federico Grandi
Nov 24 '18 at 14:08