Create model method using fields from two different models?
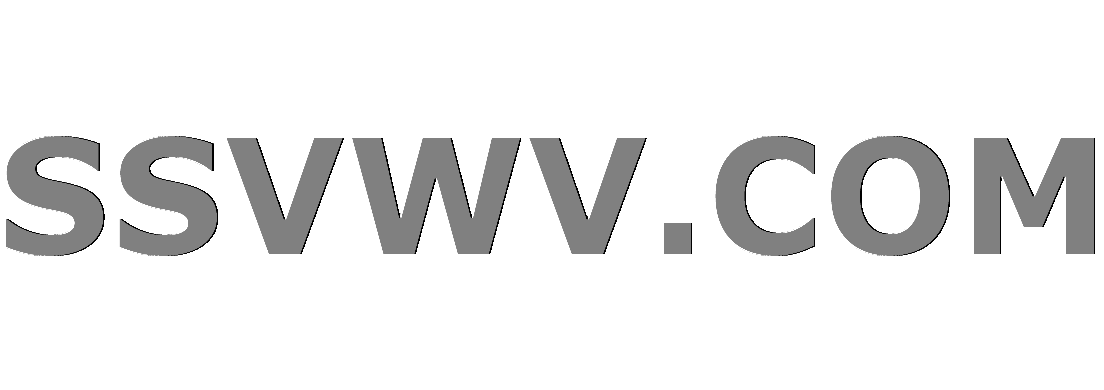
Multi tool use
I am trying to create a model method that takes data from a field in one model and performs simple arithmetic using data from a field in another model. I am not trying to create a QuerySet in views.py
, rather I am trying to create a model method in models.py
that I can easily access via a context variable in my html (e.g. obj.model.modelmethod).
Below is a very simplified version of my models (before adding the desired model method):
class Person(models.Model):
'''
This table serves as the ultimate parent.
'''
id = models.IntegerField(auto_created=False, primary_key=True)
name = models.CharField(max_length=128, null=True, blank=True)
def __str__(self):
return self.name
class SalaryYearOne(models.Model):
id = models.OneToOneField(
Person,
on_delete=models.DO_NOTHING,
db_constraint=False,
primary_key=True
)
salary1 = models.IntegerField(null=True, blank=True)
class SalaryYearTwo(models.Model):
id = models.OneToOneField(
Person,
on_delete=models.DO_NOTHING,
db_constraint=False,
primary_key=True
)
salary2 = models.IntegerField(null=True, blank=True)
The model method I want to create will be under the SalaryYearOne
class. The goal is calculate the salary percent change year-over-year (rounded to two decimal places). For example:
class SalaryYearOne(models.Model):
...
def salary_percent_change(self):
return round((self.salary1 - self.salary2) / self.salary2, 2)
The aforementioned is more for illustrative purposes. I understand that there are several issues with it as written. For example, I understand that the SalaryYearOne
table does not have a field named salary2
etc. However, I hope this clearly shows my intent. Lastly, for further background the instance of Person
will be selected from a ListView
and I want to be able to display salary_percent_change
as a context variable in my html through my DetailView
.
How would you recommend I go about this?
django python-3.x django-models django-templates django-views
add a comment |
I am trying to create a model method that takes data from a field in one model and performs simple arithmetic using data from a field in another model. I am not trying to create a QuerySet in views.py
, rather I am trying to create a model method in models.py
that I can easily access via a context variable in my html (e.g. obj.model.modelmethod).
Below is a very simplified version of my models (before adding the desired model method):
class Person(models.Model):
'''
This table serves as the ultimate parent.
'''
id = models.IntegerField(auto_created=False, primary_key=True)
name = models.CharField(max_length=128, null=True, blank=True)
def __str__(self):
return self.name
class SalaryYearOne(models.Model):
id = models.OneToOneField(
Person,
on_delete=models.DO_NOTHING,
db_constraint=False,
primary_key=True
)
salary1 = models.IntegerField(null=True, blank=True)
class SalaryYearTwo(models.Model):
id = models.OneToOneField(
Person,
on_delete=models.DO_NOTHING,
db_constraint=False,
primary_key=True
)
salary2 = models.IntegerField(null=True, blank=True)
The model method I want to create will be under the SalaryYearOne
class. The goal is calculate the salary percent change year-over-year (rounded to two decimal places). For example:
class SalaryYearOne(models.Model):
...
def salary_percent_change(self):
return round((self.salary1 - self.salary2) / self.salary2, 2)
The aforementioned is more for illustrative purposes. I understand that there are several issues with it as written. For example, I understand that the SalaryYearOne
table does not have a field named salary2
etc. However, I hope this clearly shows my intent. Lastly, for further background the instance of Person
will be selected from a ListView
and I want to be able to display salary_percent_change
as a context variable in my html through my DetailView
.
How would you recommend I go about this?
django python-3.x django-models django-templates django-views
add a comment |
I am trying to create a model method that takes data from a field in one model and performs simple arithmetic using data from a field in another model. I am not trying to create a QuerySet in views.py
, rather I am trying to create a model method in models.py
that I can easily access via a context variable in my html (e.g. obj.model.modelmethod).
Below is a very simplified version of my models (before adding the desired model method):
class Person(models.Model):
'''
This table serves as the ultimate parent.
'''
id = models.IntegerField(auto_created=False, primary_key=True)
name = models.CharField(max_length=128, null=True, blank=True)
def __str__(self):
return self.name
class SalaryYearOne(models.Model):
id = models.OneToOneField(
Person,
on_delete=models.DO_NOTHING,
db_constraint=False,
primary_key=True
)
salary1 = models.IntegerField(null=True, blank=True)
class SalaryYearTwo(models.Model):
id = models.OneToOneField(
Person,
on_delete=models.DO_NOTHING,
db_constraint=False,
primary_key=True
)
salary2 = models.IntegerField(null=True, blank=True)
The model method I want to create will be under the SalaryYearOne
class. The goal is calculate the salary percent change year-over-year (rounded to two decimal places). For example:
class SalaryYearOne(models.Model):
...
def salary_percent_change(self):
return round((self.salary1 - self.salary2) / self.salary2, 2)
The aforementioned is more for illustrative purposes. I understand that there are several issues with it as written. For example, I understand that the SalaryYearOne
table does not have a field named salary2
etc. However, I hope this clearly shows my intent. Lastly, for further background the instance of Person
will be selected from a ListView
and I want to be able to display salary_percent_change
as a context variable in my html through my DetailView
.
How would you recommend I go about this?
django python-3.x django-models django-templates django-views
I am trying to create a model method that takes data from a field in one model and performs simple arithmetic using data from a field in another model. I am not trying to create a QuerySet in views.py
, rather I am trying to create a model method in models.py
that I can easily access via a context variable in my html (e.g. obj.model.modelmethod).
Below is a very simplified version of my models (before adding the desired model method):
class Person(models.Model):
'''
This table serves as the ultimate parent.
'''
id = models.IntegerField(auto_created=False, primary_key=True)
name = models.CharField(max_length=128, null=True, blank=True)
def __str__(self):
return self.name
class SalaryYearOne(models.Model):
id = models.OneToOneField(
Person,
on_delete=models.DO_NOTHING,
db_constraint=False,
primary_key=True
)
salary1 = models.IntegerField(null=True, blank=True)
class SalaryYearTwo(models.Model):
id = models.OneToOneField(
Person,
on_delete=models.DO_NOTHING,
db_constraint=False,
primary_key=True
)
salary2 = models.IntegerField(null=True, blank=True)
The model method I want to create will be under the SalaryYearOne
class. The goal is calculate the salary percent change year-over-year (rounded to two decimal places). For example:
class SalaryYearOne(models.Model):
...
def salary_percent_change(self):
return round((self.salary1 - self.salary2) / self.salary2, 2)
The aforementioned is more for illustrative purposes. I understand that there are several issues with it as written. For example, I understand that the SalaryYearOne
table does not have a field named salary2
etc. However, I hope this clearly shows my intent. Lastly, for further background the instance of Person
will be selected from a ListView
and I want to be able to display salary_percent_change
as a context variable in my html through my DetailView
.
How would you recommend I go about this?
django python-3.x django-models django-templates django-views
django python-3.x django-models django-templates django-views
asked Nov 26 '18 at 0:08
jc4mjc4m
62
62
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53473271%2fcreate-model-method-using-fields-from-two-different-models%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53473271%2fcreate-model-method-using-fields-from-two-different-models%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
JUebU J S7sFETG7GMe95QSxAo 05cE5P8O Kx,B8z0LB,4DUw3OJqdg7XWdJ KKI5Ux9P KwQfmDCHAhtmr2,o8pgO qxYkt7pLF