Android Paho mqtt , No messages coming from MQTT
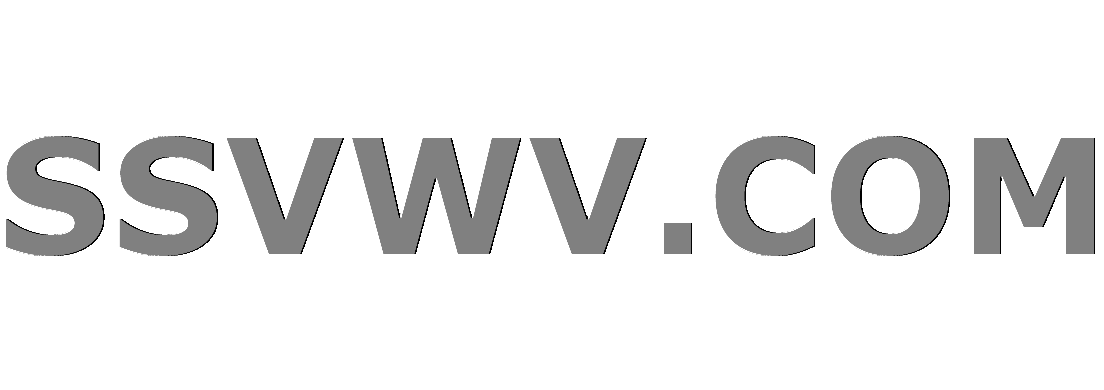
Multi tool use
Im trying to listen to MQTT network thats behind TLS(SLL), It seems Im NOT able to connect although other platforms/developers are. I dont get any authentication errors, timeout errors, or other exceptions just nothing happens.
See my Android Mqtt client code below:
package myMqttPackage;
import android.os.Bundle;
import android.os.Message;
import android.util.Log;
import org.eclipse.paho.android.service.MqttAndroidClient;
import org.eclipse.paho.client.mqttv3.DisconnectedBufferOptions;
import org.eclipse.paho.client.mqttv3.IMqttActionListener;
import org.eclipse.paho.client.mqttv3.IMqttDeliveryToken;
import org.eclipse.paho.client.mqttv3.IMqttToken;
import org.eclipse.paho.client.mqttv3.MqttCallbackExtended;
import org.eclipse.paho.client.mqttv3.MqttClientPersistence;
import org.eclipse.paho.client.mqttv3.MqttConnectOptions;
import org.eclipse.paho.client.mqttv3.MqttException;
import org.eclipse.paho.client.mqttv3.MqttMessage;
import org.eclipse.paho.client.mqttv3.MqttPersistable;
import org.eclipse.paho.client.mqttv3.MqttPersistenceException;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.ArrayList;
import java.util.Enumeration;
import java.util.List;
import java.util.Properties;
import iotrecord.IoTRecord;
import xyz.controller.core.Application.DeviceApp;
import xyz.controller.core.services.MqttBrokerService;
import xyz.controller.core.info.AlarmTopic;
import xyz.controller.core.info.AlarmTopicTool;
import xyz.controller.core.info.MasterTopicTool;
import xyz.controller.core.info.MasterTopicV2;
import xyz.controller.core.info.RawECG;
import xyz.controller.core.info.RawECGTopicTool;
import xyz.controller.core.info.StartSession;
import xyz.controller.core.info.StartSessionTool;
import xyz.controller.core.info.StopSession;
import xyz.controller.core.info.StopSessionTool;
import xyz.controller.core.info.infoRecordFactory;
public class MqttAdhocClient {
private static final String TAG = "MqttAdhocClient";
private MqttAndroidClient mqttClient;
private MqttConnectOptions connectOptions;
private static final int QOS = 0;
private IoTRecord latestIoTRecord;
private String ID;
public MqttAdhocClient(String ID) {
this.ID = ID;
}
public String getID() {
return ID;
}
public void setID(String ID) {
this.ID = ID;
}
//setup
public MqttAndroidClient setupClient(String serverPath, String serverPort, boolean authentiction, String username, String password, String clientId, boolean TLS)
{
Log.i(TAG,"setupClient()");
String mqttServerURI;
String serverURI = new String[1];
// set options for establishing a broker connection
connectOptions = new MqttConnectOptions();
Properties prop = new Properties();
if (!TLS) {
mqttServerURI = "tcp://" + serverPath + ":" + serverPort;
serverURI[0] = mqttServerURI;
}
else
{
mqttServerURI = "ssl://" + serverPath + ":" + serverPort;
serverURI[0] = mqttServerURI;
//com.ibm.ssl.protocol
prop.setProperty("com.ibm.ssl.protocol","TLS");
}
// setup the audrey broker
mqttClient = new MqttAndroidClient(DeviceApp.getInstance().getApplicationContext(), mqttServerURI, clientId);
mqttClient.setCallback(mqttClientCallback);
connectOptions.setSSLProperties(prop);
connectOptions.setServerURIs(serverURI);
connectOptions.setAutomaticReconnect(true);
connectOptions.setCleanSession(true);
if (authentiction)
{
connectOptions.setUserName(username);
if (password!=null && password.length()>0) {
connectOptions.setPassword(password.toCharArray());
}
}
try {
if (mqttClient.isConnected())
{
mqttClient.disconnect();
}
mqttClient.connect(connectOptions);
}
catch (MqttException exc)
{
exc.printStackTrace();
}
return mqttClient;
}
/**
* Callback used in creating the MQTT clients. Handles message arrival, delivery,
* and connection status callbacks. This is shared between the clients since they
* both send and receive the same messages.
*/
private MqttCallbackExtended mqttClientCallback = new MqttCallbackExtended() {
@Override
public void connectComplete(boolean reconnect, String serverURI) {
Log.i(TAG, "connectComplete " + serverURI);
//it seems that we must list to both patterns alertTopicClient & alertTopicClient/
subscribe("StartSession",MqttBrokerService.Broker.info);
subscribe("Master_Topic",MqttBrokerService.Broker.info);
subscribe("Raw_ECG",MqttBrokerService.Broker.info);
subscribe("Alarm",MqttBrokerService.Broker.info);
subscribe("StopSession",MqttBrokerService.Broker.info);
subscribe("#",MqttBrokerService.Broker.info);
if (reconnect) {
Log.i(TAG, "Reconnected to broker at " + serverURI);
subscribe("StartSession",MqttBrokerService.Broker.info);
subscribe("Master_Topic",MqttBrokerService.Broker.info);
subscribe("Raw_ECG",MqttBrokerService.Broker.info);
subscribe("Alarm",MqttBrokerService.Broker.info);
subscribe("StopSession",MqttBrokerService.Broker.info);
subscribe("#",MqttBrokerService.Broker.info);
} else {
Log.d(TAG, "Connected to broker at " + serverURI);
// set options for buffering of messages when disconnected
// we only really care about the current situation, so disable buffering
DisconnectedBufferOptions bufferOptions = new DisconnectedBufferOptions();
bufferOptions.setBufferEnabled(false);
bufferOptions.setPersistBuffer(false);
bufferOptions.setDeleteOldestMessages(true);
mqttClient.setBufferOpts(bufferOptions);
}
}
@Override
public void connectionLost(Throwable cause) {
if (cause == null)
Log.d(TAG, "Connection closed");
else
Log.e(TAG, "Connection unexpectedly lost: " + cause.getMessage());
// NOTE: connectOptions.setAutomaticReconnect will automatically handle
// reconnecting if the connection is lost
}
@Override
public void messageArrived(String topic, MqttMessage message) throws Exception {
Log.d(TAG, "messageArrived(), Received message from topic " + topic);
String msg = new String(message.getPayload());
Log.d(TAG, "Msg topic: " +topic);
Log.d(TAG, "Msg content: " + msg);
infoRecordFactory infoRecordFactory = null;
if (topic.contains("StartSession"))
{
StartSession startSession = null;
infoRecordFactory = new StartSessionTool();
try {
startSession = (StartSession) infoRecordFactory.create(new JSONObject(msg));
if (startSession.getMac_bin().equals(getID()))
{
//add sensor to UI
}
}
catch (JSONException exc)
{
exc.printStackTrace();
}
}
else if (topic.contains("Master_Topic"))
{
infoRecordFactory = new MasterTopicTool();
MasterTopicV2 masterTopic;
try {
//add sensor to UI
masterTopic = (MasterTopicV2 ) infoRecordFactory.create(new JSONObject(msg));
if (masterTopic.getMac_bin().equals(getID()))
{
//add sensor to UI
}
}
catch (JSONException exc)
{
exc.printStackTrace();
}
}
else if (topic.contains("Raw_ECG"))
{
infoRecordFactory = new RawECGTopicTool();
RawECG rawECG;
try {
//add sensor to UI
rawECG = (RawECG) infoRecordFactory.create(new JSONObject(msg));
if (rawECG.getMac_bin().equals(getID()))
{
//add sensor to UI
}
}
catch (JSONException exc)
{
exc.printStackTrace();
}
}
else if (topic.contains("Alarm"))
{
//add sensor to UI
infoRecordFactory = new AlarmTopicTool();
AlarmTopic alarmTopic;
alarmTopic = (AlarmTopic) infoRecordFactory.create(new JSONObject(msg));
if (alarmTopic.getMac_bin().equals(getID()))
{
//add sensor to UI
}
}
else if (topic.contains("StopSession"))
{
//remove sensor from UI
infoRecordFactory = new StopSessionTool();
StopSession stopSession;
stopSession = (StopSession) infoRecordFactory.create(new JSONObject(msg));
if ( stopSession.getMac_bin().equals(getID()))
{
//add sensor to UI
}
}
else
{
}
}
@Override
public void deliveryComplete(IMqttDeliveryToken token) {
Log.d(TAG, "Message delivery complete for message with ID " + token.getMessageId());
}
};
/**
* Subscribes to an MQTT topic to receive data from the server.
*
* @param topic MQTT topic to subscribe to
* @param source Broker to receive messages from
*/
private void subscribe(String topic, MqttBrokerService.Broker source) {
Log.i(TAG,"subscribed to:"+topic+",source:"+source);
// subscribe to topic on audrey broker
try {
// don't bother subscribing if not already connected
if ( mqttClient !=null && mqttClient.isConnected()) {
mqttClient.subscribe(topic, QOS, null, new IMqttActionListener() {
@Override
public void onSuccess(IMqttToken iMqttToken) {
Log.d(TAG, "yams: Subscribed to topic " + topic);
}
@Override
public void onFailure(IMqttToken iMqttToken, Throwable throwable) {
Log.e(TAG, "yams: Subscribe to topic " + topic);
if (throwable != null) {
Log.e(TAG, "yams: Subscribe to topic " + topic + " unsuccessful: "
+ throwable.getMessage());
}
}
});
}
} catch (MqttException e) {
Log.e(TAG, "yams: Exception when subscribing to topic " + topic + ": "
+ e.getMessage());
}
}
}
The code snippet that instantiates MqttAdhocClient in Service:
MqttAndroidClient client = mqttAdhocClient.setupClient(connectionPath, port, true, username, password, deviceId, true);
if (client.isConnected())
{
Log.i(TAG,"client:"+client.getServerURI()+",IS CONNECTED");
}
else
{
Log.i(TAG,"client:"+client.getServerURI()+",IS NOT CONNECTED");
}
The logs show:
2018-11-20 11:54:56.820 29016-29041/xyz.controller.core I/DpmTcmClient: RegisterTcmMonitor from: com.android.okhttp.TcmIdleTimerMonitor
2018-11-20 11:55:02.899 29016-29216/xyz.controller.core I/MqttAdhocClient: setupClient()
018-11-20 11:55:02.904 29016-29216/xyz.controller.core I/MqttBrokerService: client:ssl://someurl.com:8883,IS NOT CONNECTED

add a comment |
Im trying to listen to MQTT network thats behind TLS(SLL), It seems Im NOT able to connect although other platforms/developers are. I dont get any authentication errors, timeout errors, or other exceptions just nothing happens.
See my Android Mqtt client code below:
package myMqttPackage;
import android.os.Bundle;
import android.os.Message;
import android.util.Log;
import org.eclipse.paho.android.service.MqttAndroidClient;
import org.eclipse.paho.client.mqttv3.DisconnectedBufferOptions;
import org.eclipse.paho.client.mqttv3.IMqttActionListener;
import org.eclipse.paho.client.mqttv3.IMqttDeliveryToken;
import org.eclipse.paho.client.mqttv3.IMqttToken;
import org.eclipse.paho.client.mqttv3.MqttCallbackExtended;
import org.eclipse.paho.client.mqttv3.MqttClientPersistence;
import org.eclipse.paho.client.mqttv3.MqttConnectOptions;
import org.eclipse.paho.client.mqttv3.MqttException;
import org.eclipse.paho.client.mqttv3.MqttMessage;
import org.eclipse.paho.client.mqttv3.MqttPersistable;
import org.eclipse.paho.client.mqttv3.MqttPersistenceException;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.ArrayList;
import java.util.Enumeration;
import java.util.List;
import java.util.Properties;
import iotrecord.IoTRecord;
import xyz.controller.core.Application.DeviceApp;
import xyz.controller.core.services.MqttBrokerService;
import xyz.controller.core.info.AlarmTopic;
import xyz.controller.core.info.AlarmTopicTool;
import xyz.controller.core.info.MasterTopicTool;
import xyz.controller.core.info.MasterTopicV2;
import xyz.controller.core.info.RawECG;
import xyz.controller.core.info.RawECGTopicTool;
import xyz.controller.core.info.StartSession;
import xyz.controller.core.info.StartSessionTool;
import xyz.controller.core.info.StopSession;
import xyz.controller.core.info.StopSessionTool;
import xyz.controller.core.info.infoRecordFactory;
public class MqttAdhocClient {
private static final String TAG = "MqttAdhocClient";
private MqttAndroidClient mqttClient;
private MqttConnectOptions connectOptions;
private static final int QOS = 0;
private IoTRecord latestIoTRecord;
private String ID;
public MqttAdhocClient(String ID) {
this.ID = ID;
}
public String getID() {
return ID;
}
public void setID(String ID) {
this.ID = ID;
}
//setup
public MqttAndroidClient setupClient(String serverPath, String serverPort, boolean authentiction, String username, String password, String clientId, boolean TLS)
{
Log.i(TAG,"setupClient()");
String mqttServerURI;
String serverURI = new String[1];
// set options for establishing a broker connection
connectOptions = new MqttConnectOptions();
Properties prop = new Properties();
if (!TLS) {
mqttServerURI = "tcp://" + serverPath + ":" + serverPort;
serverURI[0] = mqttServerURI;
}
else
{
mqttServerURI = "ssl://" + serverPath + ":" + serverPort;
serverURI[0] = mqttServerURI;
//com.ibm.ssl.protocol
prop.setProperty("com.ibm.ssl.protocol","TLS");
}
// setup the audrey broker
mqttClient = new MqttAndroidClient(DeviceApp.getInstance().getApplicationContext(), mqttServerURI, clientId);
mqttClient.setCallback(mqttClientCallback);
connectOptions.setSSLProperties(prop);
connectOptions.setServerURIs(serverURI);
connectOptions.setAutomaticReconnect(true);
connectOptions.setCleanSession(true);
if (authentiction)
{
connectOptions.setUserName(username);
if (password!=null && password.length()>0) {
connectOptions.setPassword(password.toCharArray());
}
}
try {
if (mqttClient.isConnected())
{
mqttClient.disconnect();
}
mqttClient.connect(connectOptions);
}
catch (MqttException exc)
{
exc.printStackTrace();
}
return mqttClient;
}
/**
* Callback used in creating the MQTT clients. Handles message arrival, delivery,
* and connection status callbacks. This is shared between the clients since they
* both send and receive the same messages.
*/
private MqttCallbackExtended mqttClientCallback = new MqttCallbackExtended() {
@Override
public void connectComplete(boolean reconnect, String serverURI) {
Log.i(TAG, "connectComplete " + serverURI);
//it seems that we must list to both patterns alertTopicClient & alertTopicClient/
subscribe("StartSession",MqttBrokerService.Broker.info);
subscribe("Master_Topic",MqttBrokerService.Broker.info);
subscribe("Raw_ECG",MqttBrokerService.Broker.info);
subscribe("Alarm",MqttBrokerService.Broker.info);
subscribe("StopSession",MqttBrokerService.Broker.info);
subscribe("#",MqttBrokerService.Broker.info);
if (reconnect) {
Log.i(TAG, "Reconnected to broker at " + serverURI);
subscribe("StartSession",MqttBrokerService.Broker.info);
subscribe("Master_Topic",MqttBrokerService.Broker.info);
subscribe("Raw_ECG",MqttBrokerService.Broker.info);
subscribe("Alarm",MqttBrokerService.Broker.info);
subscribe("StopSession",MqttBrokerService.Broker.info);
subscribe("#",MqttBrokerService.Broker.info);
} else {
Log.d(TAG, "Connected to broker at " + serverURI);
// set options for buffering of messages when disconnected
// we only really care about the current situation, so disable buffering
DisconnectedBufferOptions bufferOptions = new DisconnectedBufferOptions();
bufferOptions.setBufferEnabled(false);
bufferOptions.setPersistBuffer(false);
bufferOptions.setDeleteOldestMessages(true);
mqttClient.setBufferOpts(bufferOptions);
}
}
@Override
public void connectionLost(Throwable cause) {
if (cause == null)
Log.d(TAG, "Connection closed");
else
Log.e(TAG, "Connection unexpectedly lost: " + cause.getMessage());
// NOTE: connectOptions.setAutomaticReconnect will automatically handle
// reconnecting if the connection is lost
}
@Override
public void messageArrived(String topic, MqttMessage message) throws Exception {
Log.d(TAG, "messageArrived(), Received message from topic " + topic);
String msg = new String(message.getPayload());
Log.d(TAG, "Msg topic: " +topic);
Log.d(TAG, "Msg content: " + msg);
infoRecordFactory infoRecordFactory = null;
if (topic.contains("StartSession"))
{
StartSession startSession = null;
infoRecordFactory = new StartSessionTool();
try {
startSession = (StartSession) infoRecordFactory.create(new JSONObject(msg));
if (startSession.getMac_bin().equals(getID()))
{
//add sensor to UI
}
}
catch (JSONException exc)
{
exc.printStackTrace();
}
}
else if (topic.contains("Master_Topic"))
{
infoRecordFactory = new MasterTopicTool();
MasterTopicV2 masterTopic;
try {
//add sensor to UI
masterTopic = (MasterTopicV2 ) infoRecordFactory.create(new JSONObject(msg));
if (masterTopic.getMac_bin().equals(getID()))
{
//add sensor to UI
}
}
catch (JSONException exc)
{
exc.printStackTrace();
}
}
else if (topic.contains("Raw_ECG"))
{
infoRecordFactory = new RawECGTopicTool();
RawECG rawECG;
try {
//add sensor to UI
rawECG = (RawECG) infoRecordFactory.create(new JSONObject(msg));
if (rawECG.getMac_bin().equals(getID()))
{
//add sensor to UI
}
}
catch (JSONException exc)
{
exc.printStackTrace();
}
}
else if (topic.contains("Alarm"))
{
//add sensor to UI
infoRecordFactory = new AlarmTopicTool();
AlarmTopic alarmTopic;
alarmTopic = (AlarmTopic) infoRecordFactory.create(new JSONObject(msg));
if (alarmTopic.getMac_bin().equals(getID()))
{
//add sensor to UI
}
}
else if (topic.contains("StopSession"))
{
//remove sensor from UI
infoRecordFactory = new StopSessionTool();
StopSession stopSession;
stopSession = (StopSession) infoRecordFactory.create(new JSONObject(msg));
if ( stopSession.getMac_bin().equals(getID()))
{
//add sensor to UI
}
}
else
{
}
}
@Override
public void deliveryComplete(IMqttDeliveryToken token) {
Log.d(TAG, "Message delivery complete for message with ID " + token.getMessageId());
}
};
/**
* Subscribes to an MQTT topic to receive data from the server.
*
* @param topic MQTT topic to subscribe to
* @param source Broker to receive messages from
*/
private void subscribe(String topic, MqttBrokerService.Broker source) {
Log.i(TAG,"subscribed to:"+topic+",source:"+source);
// subscribe to topic on audrey broker
try {
// don't bother subscribing if not already connected
if ( mqttClient !=null && mqttClient.isConnected()) {
mqttClient.subscribe(topic, QOS, null, new IMqttActionListener() {
@Override
public void onSuccess(IMqttToken iMqttToken) {
Log.d(TAG, "yams: Subscribed to topic " + topic);
}
@Override
public void onFailure(IMqttToken iMqttToken, Throwable throwable) {
Log.e(TAG, "yams: Subscribe to topic " + topic);
if (throwable != null) {
Log.e(TAG, "yams: Subscribe to topic " + topic + " unsuccessful: "
+ throwable.getMessage());
}
}
});
}
} catch (MqttException e) {
Log.e(TAG, "yams: Exception when subscribing to topic " + topic + ": "
+ e.getMessage());
}
}
}
The code snippet that instantiates MqttAdhocClient in Service:
MqttAndroidClient client = mqttAdhocClient.setupClient(connectionPath, port, true, username, password, deviceId, true);
if (client.isConnected())
{
Log.i(TAG,"client:"+client.getServerURI()+",IS CONNECTED");
}
else
{
Log.i(TAG,"client:"+client.getServerURI()+",IS NOT CONNECTED");
}
The logs show:
2018-11-20 11:54:56.820 29016-29041/xyz.controller.core I/DpmTcmClient: RegisterTcmMonitor from: com.android.okhttp.TcmIdleTimerMonitor
2018-11-20 11:55:02.899 29016-29216/xyz.controller.core I/MqttAdhocClient: setupClient()
018-11-20 11:55:02.904 29016-29216/xyz.controller.core I/MqttBrokerService: client:ssl://someurl.com:8883,IS NOT CONNECTED

add a comment |
Im trying to listen to MQTT network thats behind TLS(SLL), It seems Im NOT able to connect although other platforms/developers are. I dont get any authentication errors, timeout errors, or other exceptions just nothing happens.
See my Android Mqtt client code below:
package myMqttPackage;
import android.os.Bundle;
import android.os.Message;
import android.util.Log;
import org.eclipse.paho.android.service.MqttAndroidClient;
import org.eclipse.paho.client.mqttv3.DisconnectedBufferOptions;
import org.eclipse.paho.client.mqttv3.IMqttActionListener;
import org.eclipse.paho.client.mqttv3.IMqttDeliveryToken;
import org.eclipse.paho.client.mqttv3.IMqttToken;
import org.eclipse.paho.client.mqttv3.MqttCallbackExtended;
import org.eclipse.paho.client.mqttv3.MqttClientPersistence;
import org.eclipse.paho.client.mqttv3.MqttConnectOptions;
import org.eclipse.paho.client.mqttv3.MqttException;
import org.eclipse.paho.client.mqttv3.MqttMessage;
import org.eclipse.paho.client.mqttv3.MqttPersistable;
import org.eclipse.paho.client.mqttv3.MqttPersistenceException;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.ArrayList;
import java.util.Enumeration;
import java.util.List;
import java.util.Properties;
import iotrecord.IoTRecord;
import xyz.controller.core.Application.DeviceApp;
import xyz.controller.core.services.MqttBrokerService;
import xyz.controller.core.info.AlarmTopic;
import xyz.controller.core.info.AlarmTopicTool;
import xyz.controller.core.info.MasterTopicTool;
import xyz.controller.core.info.MasterTopicV2;
import xyz.controller.core.info.RawECG;
import xyz.controller.core.info.RawECGTopicTool;
import xyz.controller.core.info.StartSession;
import xyz.controller.core.info.StartSessionTool;
import xyz.controller.core.info.StopSession;
import xyz.controller.core.info.StopSessionTool;
import xyz.controller.core.info.infoRecordFactory;
public class MqttAdhocClient {
private static final String TAG = "MqttAdhocClient";
private MqttAndroidClient mqttClient;
private MqttConnectOptions connectOptions;
private static final int QOS = 0;
private IoTRecord latestIoTRecord;
private String ID;
public MqttAdhocClient(String ID) {
this.ID = ID;
}
public String getID() {
return ID;
}
public void setID(String ID) {
this.ID = ID;
}
//setup
public MqttAndroidClient setupClient(String serverPath, String serverPort, boolean authentiction, String username, String password, String clientId, boolean TLS)
{
Log.i(TAG,"setupClient()");
String mqttServerURI;
String serverURI = new String[1];
// set options for establishing a broker connection
connectOptions = new MqttConnectOptions();
Properties prop = new Properties();
if (!TLS) {
mqttServerURI = "tcp://" + serverPath + ":" + serverPort;
serverURI[0] = mqttServerURI;
}
else
{
mqttServerURI = "ssl://" + serverPath + ":" + serverPort;
serverURI[0] = mqttServerURI;
//com.ibm.ssl.protocol
prop.setProperty("com.ibm.ssl.protocol","TLS");
}
// setup the audrey broker
mqttClient = new MqttAndroidClient(DeviceApp.getInstance().getApplicationContext(), mqttServerURI, clientId);
mqttClient.setCallback(mqttClientCallback);
connectOptions.setSSLProperties(prop);
connectOptions.setServerURIs(serverURI);
connectOptions.setAutomaticReconnect(true);
connectOptions.setCleanSession(true);
if (authentiction)
{
connectOptions.setUserName(username);
if (password!=null && password.length()>0) {
connectOptions.setPassword(password.toCharArray());
}
}
try {
if (mqttClient.isConnected())
{
mqttClient.disconnect();
}
mqttClient.connect(connectOptions);
}
catch (MqttException exc)
{
exc.printStackTrace();
}
return mqttClient;
}
/**
* Callback used in creating the MQTT clients. Handles message arrival, delivery,
* and connection status callbacks. This is shared between the clients since they
* both send and receive the same messages.
*/
private MqttCallbackExtended mqttClientCallback = new MqttCallbackExtended() {
@Override
public void connectComplete(boolean reconnect, String serverURI) {
Log.i(TAG, "connectComplete " + serverURI);
//it seems that we must list to both patterns alertTopicClient & alertTopicClient/
subscribe("StartSession",MqttBrokerService.Broker.info);
subscribe("Master_Topic",MqttBrokerService.Broker.info);
subscribe("Raw_ECG",MqttBrokerService.Broker.info);
subscribe("Alarm",MqttBrokerService.Broker.info);
subscribe("StopSession",MqttBrokerService.Broker.info);
subscribe("#",MqttBrokerService.Broker.info);
if (reconnect) {
Log.i(TAG, "Reconnected to broker at " + serverURI);
subscribe("StartSession",MqttBrokerService.Broker.info);
subscribe("Master_Topic",MqttBrokerService.Broker.info);
subscribe("Raw_ECG",MqttBrokerService.Broker.info);
subscribe("Alarm",MqttBrokerService.Broker.info);
subscribe("StopSession",MqttBrokerService.Broker.info);
subscribe("#",MqttBrokerService.Broker.info);
} else {
Log.d(TAG, "Connected to broker at " + serverURI);
// set options for buffering of messages when disconnected
// we only really care about the current situation, so disable buffering
DisconnectedBufferOptions bufferOptions = new DisconnectedBufferOptions();
bufferOptions.setBufferEnabled(false);
bufferOptions.setPersistBuffer(false);
bufferOptions.setDeleteOldestMessages(true);
mqttClient.setBufferOpts(bufferOptions);
}
}
@Override
public void connectionLost(Throwable cause) {
if (cause == null)
Log.d(TAG, "Connection closed");
else
Log.e(TAG, "Connection unexpectedly lost: " + cause.getMessage());
// NOTE: connectOptions.setAutomaticReconnect will automatically handle
// reconnecting if the connection is lost
}
@Override
public void messageArrived(String topic, MqttMessage message) throws Exception {
Log.d(TAG, "messageArrived(), Received message from topic " + topic);
String msg = new String(message.getPayload());
Log.d(TAG, "Msg topic: " +topic);
Log.d(TAG, "Msg content: " + msg);
infoRecordFactory infoRecordFactory = null;
if (topic.contains("StartSession"))
{
StartSession startSession = null;
infoRecordFactory = new StartSessionTool();
try {
startSession = (StartSession) infoRecordFactory.create(new JSONObject(msg));
if (startSession.getMac_bin().equals(getID()))
{
//add sensor to UI
}
}
catch (JSONException exc)
{
exc.printStackTrace();
}
}
else if (topic.contains("Master_Topic"))
{
infoRecordFactory = new MasterTopicTool();
MasterTopicV2 masterTopic;
try {
//add sensor to UI
masterTopic = (MasterTopicV2 ) infoRecordFactory.create(new JSONObject(msg));
if (masterTopic.getMac_bin().equals(getID()))
{
//add sensor to UI
}
}
catch (JSONException exc)
{
exc.printStackTrace();
}
}
else if (topic.contains("Raw_ECG"))
{
infoRecordFactory = new RawECGTopicTool();
RawECG rawECG;
try {
//add sensor to UI
rawECG = (RawECG) infoRecordFactory.create(new JSONObject(msg));
if (rawECG.getMac_bin().equals(getID()))
{
//add sensor to UI
}
}
catch (JSONException exc)
{
exc.printStackTrace();
}
}
else if (topic.contains("Alarm"))
{
//add sensor to UI
infoRecordFactory = new AlarmTopicTool();
AlarmTopic alarmTopic;
alarmTopic = (AlarmTopic) infoRecordFactory.create(new JSONObject(msg));
if (alarmTopic.getMac_bin().equals(getID()))
{
//add sensor to UI
}
}
else if (topic.contains("StopSession"))
{
//remove sensor from UI
infoRecordFactory = new StopSessionTool();
StopSession stopSession;
stopSession = (StopSession) infoRecordFactory.create(new JSONObject(msg));
if ( stopSession.getMac_bin().equals(getID()))
{
//add sensor to UI
}
}
else
{
}
}
@Override
public void deliveryComplete(IMqttDeliveryToken token) {
Log.d(TAG, "Message delivery complete for message with ID " + token.getMessageId());
}
};
/**
* Subscribes to an MQTT topic to receive data from the server.
*
* @param topic MQTT topic to subscribe to
* @param source Broker to receive messages from
*/
private void subscribe(String topic, MqttBrokerService.Broker source) {
Log.i(TAG,"subscribed to:"+topic+",source:"+source);
// subscribe to topic on audrey broker
try {
// don't bother subscribing if not already connected
if ( mqttClient !=null && mqttClient.isConnected()) {
mqttClient.subscribe(topic, QOS, null, new IMqttActionListener() {
@Override
public void onSuccess(IMqttToken iMqttToken) {
Log.d(TAG, "yams: Subscribed to topic " + topic);
}
@Override
public void onFailure(IMqttToken iMqttToken, Throwable throwable) {
Log.e(TAG, "yams: Subscribe to topic " + topic);
if (throwable != null) {
Log.e(TAG, "yams: Subscribe to topic " + topic + " unsuccessful: "
+ throwable.getMessage());
}
}
});
}
} catch (MqttException e) {
Log.e(TAG, "yams: Exception when subscribing to topic " + topic + ": "
+ e.getMessage());
}
}
}
The code snippet that instantiates MqttAdhocClient in Service:
MqttAndroidClient client = mqttAdhocClient.setupClient(connectionPath, port, true, username, password, deviceId, true);
if (client.isConnected())
{
Log.i(TAG,"client:"+client.getServerURI()+",IS CONNECTED");
}
else
{
Log.i(TAG,"client:"+client.getServerURI()+",IS NOT CONNECTED");
}
The logs show:
2018-11-20 11:54:56.820 29016-29041/xyz.controller.core I/DpmTcmClient: RegisterTcmMonitor from: com.android.okhttp.TcmIdleTimerMonitor
2018-11-20 11:55:02.899 29016-29216/xyz.controller.core I/MqttAdhocClient: setupClient()
018-11-20 11:55:02.904 29016-29216/xyz.controller.core I/MqttBrokerService: client:ssl://someurl.com:8883,IS NOT CONNECTED

Im trying to listen to MQTT network thats behind TLS(SLL), It seems Im NOT able to connect although other platforms/developers are. I dont get any authentication errors, timeout errors, or other exceptions just nothing happens.
See my Android Mqtt client code below:
package myMqttPackage;
import android.os.Bundle;
import android.os.Message;
import android.util.Log;
import org.eclipse.paho.android.service.MqttAndroidClient;
import org.eclipse.paho.client.mqttv3.DisconnectedBufferOptions;
import org.eclipse.paho.client.mqttv3.IMqttActionListener;
import org.eclipse.paho.client.mqttv3.IMqttDeliveryToken;
import org.eclipse.paho.client.mqttv3.IMqttToken;
import org.eclipse.paho.client.mqttv3.MqttCallbackExtended;
import org.eclipse.paho.client.mqttv3.MqttClientPersistence;
import org.eclipse.paho.client.mqttv3.MqttConnectOptions;
import org.eclipse.paho.client.mqttv3.MqttException;
import org.eclipse.paho.client.mqttv3.MqttMessage;
import org.eclipse.paho.client.mqttv3.MqttPersistable;
import org.eclipse.paho.client.mqttv3.MqttPersistenceException;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.ArrayList;
import java.util.Enumeration;
import java.util.List;
import java.util.Properties;
import iotrecord.IoTRecord;
import xyz.controller.core.Application.DeviceApp;
import xyz.controller.core.services.MqttBrokerService;
import xyz.controller.core.info.AlarmTopic;
import xyz.controller.core.info.AlarmTopicTool;
import xyz.controller.core.info.MasterTopicTool;
import xyz.controller.core.info.MasterTopicV2;
import xyz.controller.core.info.RawECG;
import xyz.controller.core.info.RawECGTopicTool;
import xyz.controller.core.info.StartSession;
import xyz.controller.core.info.StartSessionTool;
import xyz.controller.core.info.StopSession;
import xyz.controller.core.info.StopSessionTool;
import xyz.controller.core.info.infoRecordFactory;
public class MqttAdhocClient {
private static final String TAG = "MqttAdhocClient";
private MqttAndroidClient mqttClient;
private MqttConnectOptions connectOptions;
private static final int QOS = 0;
private IoTRecord latestIoTRecord;
private String ID;
public MqttAdhocClient(String ID) {
this.ID = ID;
}
public String getID() {
return ID;
}
public void setID(String ID) {
this.ID = ID;
}
//setup
public MqttAndroidClient setupClient(String serverPath, String serverPort, boolean authentiction, String username, String password, String clientId, boolean TLS)
{
Log.i(TAG,"setupClient()");
String mqttServerURI;
String serverURI = new String[1];
// set options for establishing a broker connection
connectOptions = new MqttConnectOptions();
Properties prop = new Properties();
if (!TLS) {
mqttServerURI = "tcp://" + serverPath + ":" + serverPort;
serverURI[0] = mqttServerURI;
}
else
{
mqttServerURI = "ssl://" + serverPath + ":" + serverPort;
serverURI[0] = mqttServerURI;
//com.ibm.ssl.protocol
prop.setProperty("com.ibm.ssl.protocol","TLS");
}
// setup the audrey broker
mqttClient = new MqttAndroidClient(DeviceApp.getInstance().getApplicationContext(), mqttServerURI, clientId);
mqttClient.setCallback(mqttClientCallback);
connectOptions.setSSLProperties(prop);
connectOptions.setServerURIs(serverURI);
connectOptions.setAutomaticReconnect(true);
connectOptions.setCleanSession(true);
if (authentiction)
{
connectOptions.setUserName(username);
if (password!=null && password.length()>0) {
connectOptions.setPassword(password.toCharArray());
}
}
try {
if (mqttClient.isConnected())
{
mqttClient.disconnect();
}
mqttClient.connect(connectOptions);
}
catch (MqttException exc)
{
exc.printStackTrace();
}
return mqttClient;
}
/**
* Callback used in creating the MQTT clients. Handles message arrival, delivery,
* and connection status callbacks. This is shared between the clients since they
* both send and receive the same messages.
*/
private MqttCallbackExtended mqttClientCallback = new MqttCallbackExtended() {
@Override
public void connectComplete(boolean reconnect, String serverURI) {
Log.i(TAG, "connectComplete " + serverURI);
//it seems that we must list to both patterns alertTopicClient & alertTopicClient/
subscribe("StartSession",MqttBrokerService.Broker.info);
subscribe("Master_Topic",MqttBrokerService.Broker.info);
subscribe("Raw_ECG",MqttBrokerService.Broker.info);
subscribe("Alarm",MqttBrokerService.Broker.info);
subscribe("StopSession",MqttBrokerService.Broker.info);
subscribe("#",MqttBrokerService.Broker.info);
if (reconnect) {
Log.i(TAG, "Reconnected to broker at " + serverURI);
subscribe("StartSession",MqttBrokerService.Broker.info);
subscribe("Master_Topic",MqttBrokerService.Broker.info);
subscribe("Raw_ECG",MqttBrokerService.Broker.info);
subscribe("Alarm",MqttBrokerService.Broker.info);
subscribe("StopSession",MqttBrokerService.Broker.info);
subscribe("#",MqttBrokerService.Broker.info);
} else {
Log.d(TAG, "Connected to broker at " + serverURI);
// set options for buffering of messages when disconnected
// we only really care about the current situation, so disable buffering
DisconnectedBufferOptions bufferOptions = new DisconnectedBufferOptions();
bufferOptions.setBufferEnabled(false);
bufferOptions.setPersistBuffer(false);
bufferOptions.setDeleteOldestMessages(true);
mqttClient.setBufferOpts(bufferOptions);
}
}
@Override
public void connectionLost(Throwable cause) {
if (cause == null)
Log.d(TAG, "Connection closed");
else
Log.e(TAG, "Connection unexpectedly lost: " + cause.getMessage());
// NOTE: connectOptions.setAutomaticReconnect will automatically handle
// reconnecting if the connection is lost
}
@Override
public void messageArrived(String topic, MqttMessage message) throws Exception {
Log.d(TAG, "messageArrived(), Received message from topic " + topic);
String msg = new String(message.getPayload());
Log.d(TAG, "Msg topic: " +topic);
Log.d(TAG, "Msg content: " + msg);
infoRecordFactory infoRecordFactory = null;
if (topic.contains("StartSession"))
{
StartSession startSession = null;
infoRecordFactory = new StartSessionTool();
try {
startSession = (StartSession) infoRecordFactory.create(new JSONObject(msg));
if (startSession.getMac_bin().equals(getID()))
{
//add sensor to UI
}
}
catch (JSONException exc)
{
exc.printStackTrace();
}
}
else if (topic.contains("Master_Topic"))
{
infoRecordFactory = new MasterTopicTool();
MasterTopicV2 masterTopic;
try {
//add sensor to UI
masterTopic = (MasterTopicV2 ) infoRecordFactory.create(new JSONObject(msg));
if (masterTopic.getMac_bin().equals(getID()))
{
//add sensor to UI
}
}
catch (JSONException exc)
{
exc.printStackTrace();
}
}
else if (topic.contains("Raw_ECG"))
{
infoRecordFactory = new RawECGTopicTool();
RawECG rawECG;
try {
//add sensor to UI
rawECG = (RawECG) infoRecordFactory.create(new JSONObject(msg));
if (rawECG.getMac_bin().equals(getID()))
{
//add sensor to UI
}
}
catch (JSONException exc)
{
exc.printStackTrace();
}
}
else if (topic.contains("Alarm"))
{
//add sensor to UI
infoRecordFactory = new AlarmTopicTool();
AlarmTopic alarmTopic;
alarmTopic = (AlarmTopic) infoRecordFactory.create(new JSONObject(msg));
if (alarmTopic.getMac_bin().equals(getID()))
{
//add sensor to UI
}
}
else if (topic.contains("StopSession"))
{
//remove sensor from UI
infoRecordFactory = new StopSessionTool();
StopSession stopSession;
stopSession = (StopSession) infoRecordFactory.create(new JSONObject(msg));
if ( stopSession.getMac_bin().equals(getID()))
{
//add sensor to UI
}
}
else
{
}
}
@Override
public void deliveryComplete(IMqttDeliveryToken token) {
Log.d(TAG, "Message delivery complete for message with ID " + token.getMessageId());
}
};
/**
* Subscribes to an MQTT topic to receive data from the server.
*
* @param topic MQTT topic to subscribe to
* @param source Broker to receive messages from
*/
private void subscribe(String topic, MqttBrokerService.Broker source) {
Log.i(TAG,"subscribed to:"+topic+",source:"+source);
// subscribe to topic on audrey broker
try {
// don't bother subscribing if not already connected
if ( mqttClient !=null && mqttClient.isConnected()) {
mqttClient.subscribe(topic, QOS, null, new IMqttActionListener() {
@Override
public void onSuccess(IMqttToken iMqttToken) {
Log.d(TAG, "yams: Subscribed to topic " + topic);
}
@Override
public void onFailure(IMqttToken iMqttToken, Throwable throwable) {
Log.e(TAG, "yams: Subscribe to topic " + topic);
if (throwable != null) {
Log.e(TAG, "yams: Subscribe to topic " + topic + " unsuccessful: "
+ throwable.getMessage());
}
}
});
}
} catch (MqttException e) {
Log.e(TAG, "yams: Exception when subscribing to topic " + topic + ": "
+ e.getMessage());
}
}
}
The code snippet that instantiates MqttAdhocClient in Service:
MqttAndroidClient client = mqttAdhocClient.setupClient(connectionPath, port, true, username, password, deviceId, true);
if (client.isConnected())
{
Log.i(TAG,"client:"+client.getServerURI()+",IS CONNECTED");
}
else
{
Log.i(TAG,"client:"+client.getServerURI()+",IS NOT CONNECTED");
}
The logs show:
2018-11-20 11:54:56.820 29016-29041/xyz.controller.core I/DpmTcmClient: RegisterTcmMonitor from: com.android.okhttp.TcmIdleTimerMonitor
2018-11-20 11:55:02.899 29016-29216/xyz.controller.core I/MqttAdhocClient: setupClient()
018-11-20 11:55:02.904 29016-29216/xyz.controller.core I/MqttBrokerService: client:ssl://someurl.com:8883,IS NOT CONNECTED


asked Nov 20 at 20:02
cyber101
1,04083067
1,04083067
add a comment |
add a comment |
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53400692%2fandroid-paho-mqtt-no-messages-coming-from-mqtt%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53400692%2fandroid-paho-mqtt-no-messages-coming-from-mqtt%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
qHlYsOOgWw2CWHm5 vyJxWi3eTP BH8WzfIjZpyFmCg1,MYfPPJ31KqUAgntbPjEf K4Sw8QpoIeb8ck,gq