ASP.NET COR 2 handler to handle HTTP 4xx errors for WEB API request
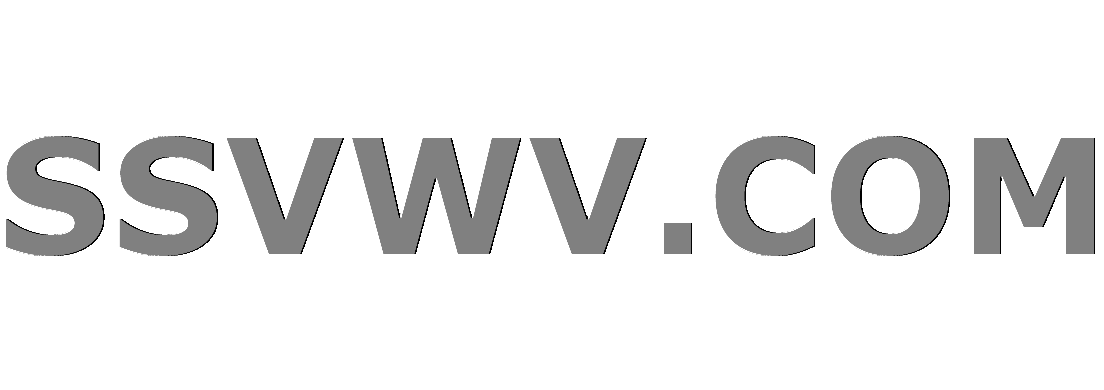
Multi tool use
Similar to UseExceptionHandler which handles the HTTP 5xx errors, is there any handler provided by ASP.NET CORE 2 to handle the HTTP 4xx errors.
Here I am trying to catch any HTTP 4xx errors produced during the request processing pipeline and process it with send it back to the consumer.
c# asp.net-core-2.1
add a comment |
Similar to UseExceptionHandler which handles the HTTP 5xx errors, is there any handler provided by ASP.NET CORE 2 to handle the HTTP 4xx errors.
Here I am trying to catch any HTTP 4xx errors produced during the request processing pipeline and process it with send it back to the consumer.
c# asp.net-core-2.1
add a comment |
Similar to UseExceptionHandler which handles the HTTP 5xx errors, is there any handler provided by ASP.NET CORE 2 to handle the HTTP 4xx errors.
Here I am trying to catch any HTTP 4xx errors produced during the request processing pipeline and process it with send it back to the consumer.
c# asp.net-core-2.1
Similar to UseExceptionHandler which handles the HTTP 5xx errors, is there any handler provided by ASP.NET CORE 2 to handle the HTTP 4xx errors.
Here I am trying to catch any HTTP 4xx errors produced during the request processing pipeline and process it with send it back to the consumer.
c# asp.net-core-2.1
c# asp.net-core-2.1
asked Nov 20 at 20:03


santosh kumar patro
1,81482657
1,81482657
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
You can create a new middleware to handle your exceptions:
public class ErrorHandlingMiddleware
{
private readonly RequestDelegate _next;
/// <summary>
/// Default constructor
/// </summary>
/// <param name="next">Next request in the pipeline</param>
public ErrorHandlingMiddleware(RequestDelegate next)
{
_next = next;
}
/// <summary>
/// Entry point into middleware logic
/// </summary>
/// <param name="context">Current http context</param>
/// <returns></returns>
public async Task Invoke(HttpContext context)
{
try
{
await _next(context);
}
catch (HttpException httpException)
{
context.Response.StatusCode = httpException.StatusCode;
}
catch (Exception ex)
{
await HandleExceptionAsync(context, ex);
}
}
private static Task HandleExceptionAsync(HttpContext context, Exception exception)
{
var code = HttpStatusCode.InternalServerError; // 500 if unexpected
var result = JsonConvert.SerializeObject(new { Error = "Internal Server error" });
context.Response.ContentType = "application/json";
context.Response.StatusCode = (int)code;
return context.Response.WriteAsync(result);
}
}
Use it like this in your Startup.cs
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
app.UseMiddleware(typeof(ErrorHandlingMiddleware));
app.UseMvc();
}
Thanks for the quick response. I am already using the UseExceptionHandler to catch the HTTP 5xx errors. I tried to debug and see if it handle the 4xx errors but found it is not catching those errors.
– santosh kumar patro
Nov 20 at 20:20
By default, an app doesn't provide a rich status code page for HTTP status codes, such as 404 Not Found. To provide status code pages, use Status Code Pages Middleware.
According to this: docs.microsoft.com/en-us/aspnet/core/fundamentals/… I think you should be using StatusCodePages. But in WEB API you shouldn't return HTML. You should return some type of serializable data which is what my middleware does by returning JSON. Look at their example, they return a plain text result.
– Hubert Jarema
Nov 20 at 20:28
I tried the code that you mentioned but I see any HTTP 4xx errors are not getting trapped by the ErrorHandlingMiddleware
– santosh kumar patro
Nov 21 at 8:04
Yes, that's because 4xx is not an exception. If you controller action returns 4xx that means the controller action is handling the resulting value. This will only work for exception that you didn't handle in your code.
– Hubert Jarema
Nov 21 at 15:09
Thanks much for the details
– santosh kumar patro
Nov 21 at 15:36
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53400715%2fasp-net-cor-2-handler-to-handle-http-4xx-errors-for-web-api-request%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can create a new middleware to handle your exceptions:
public class ErrorHandlingMiddleware
{
private readonly RequestDelegate _next;
/// <summary>
/// Default constructor
/// </summary>
/// <param name="next">Next request in the pipeline</param>
public ErrorHandlingMiddleware(RequestDelegate next)
{
_next = next;
}
/// <summary>
/// Entry point into middleware logic
/// </summary>
/// <param name="context">Current http context</param>
/// <returns></returns>
public async Task Invoke(HttpContext context)
{
try
{
await _next(context);
}
catch (HttpException httpException)
{
context.Response.StatusCode = httpException.StatusCode;
}
catch (Exception ex)
{
await HandleExceptionAsync(context, ex);
}
}
private static Task HandleExceptionAsync(HttpContext context, Exception exception)
{
var code = HttpStatusCode.InternalServerError; // 500 if unexpected
var result = JsonConvert.SerializeObject(new { Error = "Internal Server error" });
context.Response.ContentType = "application/json";
context.Response.StatusCode = (int)code;
return context.Response.WriteAsync(result);
}
}
Use it like this in your Startup.cs
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
app.UseMiddleware(typeof(ErrorHandlingMiddleware));
app.UseMvc();
}
Thanks for the quick response. I am already using the UseExceptionHandler to catch the HTTP 5xx errors. I tried to debug and see if it handle the 4xx errors but found it is not catching those errors.
– santosh kumar patro
Nov 20 at 20:20
By default, an app doesn't provide a rich status code page for HTTP status codes, such as 404 Not Found. To provide status code pages, use Status Code Pages Middleware.
According to this: docs.microsoft.com/en-us/aspnet/core/fundamentals/… I think you should be using StatusCodePages. But in WEB API you shouldn't return HTML. You should return some type of serializable data which is what my middleware does by returning JSON. Look at their example, they return a plain text result.
– Hubert Jarema
Nov 20 at 20:28
I tried the code that you mentioned but I see any HTTP 4xx errors are not getting trapped by the ErrorHandlingMiddleware
– santosh kumar patro
Nov 21 at 8:04
Yes, that's because 4xx is not an exception. If you controller action returns 4xx that means the controller action is handling the resulting value. This will only work for exception that you didn't handle in your code.
– Hubert Jarema
Nov 21 at 15:09
Thanks much for the details
– santosh kumar patro
Nov 21 at 15:36
add a comment |
You can create a new middleware to handle your exceptions:
public class ErrorHandlingMiddleware
{
private readonly RequestDelegate _next;
/// <summary>
/// Default constructor
/// </summary>
/// <param name="next">Next request in the pipeline</param>
public ErrorHandlingMiddleware(RequestDelegate next)
{
_next = next;
}
/// <summary>
/// Entry point into middleware logic
/// </summary>
/// <param name="context">Current http context</param>
/// <returns></returns>
public async Task Invoke(HttpContext context)
{
try
{
await _next(context);
}
catch (HttpException httpException)
{
context.Response.StatusCode = httpException.StatusCode;
}
catch (Exception ex)
{
await HandleExceptionAsync(context, ex);
}
}
private static Task HandleExceptionAsync(HttpContext context, Exception exception)
{
var code = HttpStatusCode.InternalServerError; // 500 if unexpected
var result = JsonConvert.SerializeObject(new { Error = "Internal Server error" });
context.Response.ContentType = "application/json";
context.Response.StatusCode = (int)code;
return context.Response.WriteAsync(result);
}
}
Use it like this in your Startup.cs
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
app.UseMiddleware(typeof(ErrorHandlingMiddleware));
app.UseMvc();
}
Thanks for the quick response. I am already using the UseExceptionHandler to catch the HTTP 5xx errors. I tried to debug and see if it handle the 4xx errors but found it is not catching those errors.
– santosh kumar patro
Nov 20 at 20:20
By default, an app doesn't provide a rich status code page for HTTP status codes, such as 404 Not Found. To provide status code pages, use Status Code Pages Middleware.
According to this: docs.microsoft.com/en-us/aspnet/core/fundamentals/… I think you should be using StatusCodePages. But in WEB API you shouldn't return HTML. You should return some type of serializable data which is what my middleware does by returning JSON. Look at their example, they return a plain text result.
– Hubert Jarema
Nov 20 at 20:28
I tried the code that you mentioned but I see any HTTP 4xx errors are not getting trapped by the ErrorHandlingMiddleware
– santosh kumar patro
Nov 21 at 8:04
Yes, that's because 4xx is not an exception. If you controller action returns 4xx that means the controller action is handling the resulting value. This will only work for exception that you didn't handle in your code.
– Hubert Jarema
Nov 21 at 15:09
Thanks much for the details
– santosh kumar patro
Nov 21 at 15:36
add a comment |
You can create a new middleware to handle your exceptions:
public class ErrorHandlingMiddleware
{
private readonly RequestDelegate _next;
/// <summary>
/// Default constructor
/// </summary>
/// <param name="next">Next request in the pipeline</param>
public ErrorHandlingMiddleware(RequestDelegate next)
{
_next = next;
}
/// <summary>
/// Entry point into middleware logic
/// </summary>
/// <param name="context">Current http context</param>
/// <returns></returns>
public async Task Invoke(HttpContext context)
{
try
{
await _next(context);
}
catch (HttpException httpException)
{
context.Response.StatusCode = httpException.StatusCode;
}
catch (Exception ex)
{
await HandleExceptionAsync(context, ex);
}
}
private static Task HandleExceptionAsync(HttpContext context, Exception exception)
{
var code = HttpStatusCode.InternalServerError; // 500 if unexpected
var result = JsonConvert.SerializeObject(new { Error = "Internal Server error" });
context.Response.ContentType = "application/json";
context.Response.StatusCode = (int)code;
return context.Response.WriteAsync(result);
}
}
Use it like this in your Startup.cs
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
app.UseMiddleware(typeof(ErrorHandlingMiddleware));
app.UseMvc();
}
You can create a new middleware to handle your exceptions:
public class ErrorHandlingMiddleware
{
private readonly RequestDelegate _next;
/// <summary>
/// Default constructor
/// </summary>
/// <param name="next">Next request in the pipeline</param>
public ErrorHandlingMiddleware(RequestDelegate next)
{
_next = next;
}
/// <summary>
/// Entry point into middleware logic
/// </summary>
/// <param name="context">Current http context</param>
/// <returns></returns>
public async Task Invoke(HttpContext context)
{
try
{
await _next(context);
}
catch (HttpException httpException)
{
context.Response.StatusCode = httpException.StatusCode;
}
catch (Exception ex)
{
await HandleExceptionAsync(context, ex);
}
}
private static Task HandleExceptionAsync(HttpContext context, Exception exception)
{
var code = HttpStatusCode.InternalServerError; // 500 if unexpected
var result = JsonConvert.SerializeObject(new { Error = "Internal Server error" });
context.Response.ContentType = "application/json";
context.Response.StatusCode = (int)code;
return context.Response.WriteAsync(result);
}
}
Use it like this in your Startup.cs
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
app.UseMiddleware(typeof(ErrorHandlingMiddleware));
app.UseMvc();
}
answered Nov 20 at 20:07
Hubert Jarema
6,21972865
6,21972865
Thanks for the quick response. I am already using the UseExceptionHandler to catch the HTTP 5xx errors. I tried to debug and see if it handle the 4xx errors but found it is not catching those errors.
– santosh kumar patro
Nov 20 at 20:20
By default, an app doesn't provide a rich status code page for HTTP status codes, such as 404 Not Found. To provide status code pages, use Status Code Pages Middleware.
According to this: docs.microsoft.com/en-us/aspnet/core/fundamentals/… I think you should be using StatusCodePages. But in WEB API you shouldn't return HTML. You should return some type of serializable data which is what my middleware does by returning JSON. Look at their example, they return a plain text result.
– Hubert Jarema
Nov 20 at 20:28
I tried the code that you mentioned but I see any HTTP 4xx errors are not getting trapped by the ErrorHandlingMiddleware
– santosh kumar patro
Nov 21 at 8:04
Yes, that's because 4xx is not an exception. If you controller action returns 4xx that means the controller action is handling the resulting value. This will only work for exception that you didn't handle in your code.
– Hubert Jarema
Nov 21 at 15:09
Thanks much for the details
– santosh kumar patro
Nov 21 at 15:36
add a comment |
Thanks for the quick response. I am already using the UseExceptionHandler to catch the HTTP 5xx errors. I tried to debug and see if it handle the 4xx errors but found it is not catching those errors.
– santosh kumar patro
Nov 20 at 20:20
By default, an app doesn't provide a rich status code page for HTTP status codes, such as 404 Not Found. To provide status code pages, use Status Code Pages Middleware.
According to this: docs.microsoft.com/en-us/aspnet/core/fundamentals/… I think you should be using StatusCodePages. But in WEB API you shouldn't return HTML. You should return some type of serializable data which is what my middleware does by returning JSON. Look at their example, they return a plain text result.
– Hubert Jarema
Nov 20 at 20:28
I tried the code that you mentioned but I see any HTTP 4xx errors are not getting trapped by the ErrorHandlingMiddleware
– santosh kumar patro
Nov 21 at 8:04
Yes, that's because 4xx is not an exception. If you controller action returns 4xx that means the controller action is handling the resulting value. This will only work for exception that you didn't handle in your code.
– Hubert Jarema
Nov 21 at 15:09
Thanks much for the details
– santosh kumar patro
Nov 21 at 15:36
Thanks for the quick response. I am already using the UseExceptionHandler to catch the HTTP 5xx errors. I tried to debug and see if it handle the 4xx errors but found it is not catching those errors.
– santosh kumar patro
Nov 20 at 20:20
Thanks for the quick response. I am already using the UseExceptionHandler to catch the HTTP 5xx errors. I tried to debug and see if it handle the 4xx errors but found it is not catching those errors.
– santosh kumar patro
Nov 20 at 20:20
By default, an app doesn't provide a rich status code page for HTTP status codes, such as 404 Not Found. To provide status code pages, use Status Code Pages Middleware.
According to this: docs.microsoft.com/en-us/aspnet/core/fundamentals/… I think you should be using StatusCodePages. But in WEB API you shouldn't return HTML. You should return some type of serializable data which is what my middleware does by returning JSON. Look at their example, they return a plain text result.– Hubert Jarema
Nov 20 at 20:28
By default, an app doesn't provide a rich status code page for HTTP status codes, such as 404 Not Found. To provide status code pages, use Status Code Pages Middleware.
According to this: docs.microsoft.com/en-us/aspnet/core/fundamentals/… I think you should be using StatusCodePages. But in WEB API you shouldn't return HTML. You should return some type of serializable data which is what my middleware does by returning JSON. Look at their example, they return a plain text result.– Hubert Jarema
Nov 20 at 20:28
I tried the code that you mentioned but I see any HTTP 4xx errors are not getting trapped by the ErrorHandlingMiddleware
– santosh kumar patro
Nov 21 at 8:04
I tried the code that you mentioned but I see any HTTP 4xx errors are not getting trapped by the ErrorHandlingMiddleware
– santosh kumar patro
Nov 21 at 8:04
Yes, that's because 4xx is not an exception. If you controller action returns 4xx that means the controller action is handling the resulting value. This will only work for exception that you didn't handle in your code.
– Hubert Jarema
Nov 21 at 15:09
Yes, that's because 4xx is not an exception. If you controller action returns 4xx that means the controller action is handling the resulting value. This will only work for exception that you didn't handle in your code.
– Hubert Jarema
Nov 21 at 15:09
Thanks much for the details
– santosh kumar patro
Nov 21 at 15:36
Thanks much for the details
– santosh kumar patro
Nov 21 at 15:36
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53400715%2fasp-net-cor-2-handler-to-handle-http-4xx-errors-for-web-api-request%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
dubJ,zs998BnrcS SKn8YE jwazKVfcbG,PfME49z