Character Counter using Array List
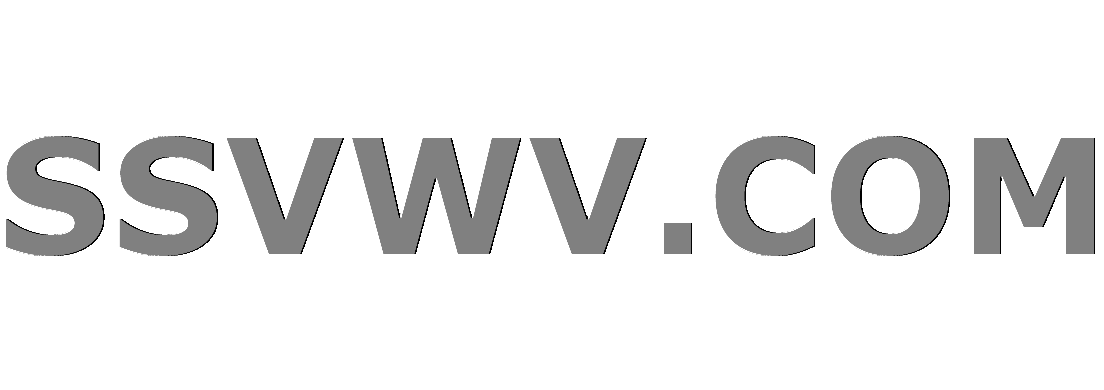
Multi tool use
I need to design a program that reads in an ASCII text file and creates an output file that contains each unique ASCII character and the number of times it appears in the file. Each unique character in the file must be represented by a character frequency class instance. The character frequency objects must be stored in an array list. My code is below:
using System.IO;
using System;
using System.Collections;
namespace ASCII
{
class CharacterFrequency
{
char ch;
int frequency;
public char getCharacter()
{
return ch;
}
public void setCharacter(char ch)
{
this.ch = ch;
}
public int getfrequency()
{
return frequency;
}
public void setfrequency(int frequency)
{
this.frequency = frequency;
}
static void Main()
{
string OutputFileName;
string InputFileName;
Console.WriteLine("Enter the file path");
InputFileName = Console.ReadLine();
Console.WriteLine("Enter the outputfile name");
OutputFileName = Console.ReadLine();
StreamWriter streamWriter = new StreamWriter(OutputFileName);
string data = File.ReadAllText(InputFileName);
ArrayList al = new ArrayList();
//create two for loops to traverse through the arraylist and compare
for (int i = 0; i < data.Length; i++)
{
int k = 0;
int f = 0;
for (int j = 0; j < data.Length; j++)
{
if (data[i].Equals(data[j]))
{
f++;
if (i > j) { k++; }
}
}
al.Add(data[i] + "(" + (int)data[i] + ")" + f + " ");
foreach (var item in al)
{
streamWriter.WriteLine(item);
}
}
streamWriter.Close();
}
}
}
When I run the program, the program does not stop running and the output file keeps getting larger until it eventually runs out memory and I get an error stating that. I am not seeing where the error is or why the loop won't terminate. It should just count the characters but it seems to keep looping and repeating counting the characters. Any help?
c# arrays arraylist
|
show 2 more comments
I need to design a program that reads in an ASCII text file and creates an output file that contains each unique ASCII character and the number of times it appears in the file. Each unique character in the file must be represented by a character frequency class instance. The character frequency objects must be stored in an array list. My code is below:
using System.IO;
using System;
using System.Collections;
namespace ASCII
{
class CharacterFrequency
{
char ch;
int frequency;
public char getCharacter()
{
return ch;
}
public void setCharacter(char ch)
{
this.ch = ch;
}
public int getfrequency()
{
return frequency;
}
public void setfrequency(int frequency)
{
this.frequency = frequency;
}
static void Main()
{
string OutputFileName;
string InputFileName;
Console.WriteLine("Enter the file path");
InputFileName = Console.ReadLine();
Console.WriteLine("Enter the outputfile name");
OutputFileName = Console.ReadLine();
StreamWriter streamWriter = new StreamWriter(OutputFileName);
string data = File.ReadAllText(InputFileName);
ArrayList al = new ArrayList();
//create two for loops to traverse through the arraylist and compare
for (int i = 0; i < data.Length; i++)
{
int k = 0;
int f = 0;
for (int j = 0; j < data.Length; j++)
{
if (data[i].Equals(data[j]))
{
f++;
if (i > j) { k++; }
}
}
al.Add(data[i] + "(" + (int)data[i] + ")" + f + " ");
foreach (var item in al)
{
streamWriter.WriteLine(item);
}
}
streamWriter.Close();
}
}
}
When I run the program, the program does not stop running and the output file keeps getting larger until it eventually runs out memory and I get an error stating that. I am not seeing where the error is or why the loop won't terminate. It should just count the characters but it seems to keep looping and repeating counting the characters. Any help?
c# arrays arraylist
“The character frequency objects must be stored in an array list” Why? ThatCharacterFrequency
class is rather strange as well. Is this C# or Java?
– Dour High Arch
Nov 21 at 1:25
dotnetfiddle.net/hcALTD
– Selvin
Nov 21 at 1:26
Pull that last foreach out from inside the for loop
– baileyrt
Nov 21 at 1:31
If it’s meant to be C# instead of Java use properties instead of getXXX and setXXX methods and use type-safe List<> over the obsolete ArrayList. For example your whole block before the Main method can be reduced to two lines:public char Character { get; set; }
andpublic int Frequency { get; set; }
.
– ckuri
Nov 21 at 1:54
Hmm, the ArrayList is so awkward. Can you get away with also including an object for every C0 Control and Basic Latin character, regardless if it is in the input? That way you can pre-populate it and index it directly based on Char value. When you dump to file, just skip the ones with frequency == 0.
– Tom Blodget
Nov 21 at 3:21
|
show 2 more comments
I need to design a program that reads in an ASCII text file and creates an output file that contains each unique ASCII character and the number of times it appears in the file. Each unique character in the file must be represented by a character frequency class instance. The character frequency objects must be stored in an array list. My code is below:
using System.IO;
using System;
using System.Collections;
namespace ASCII
{
class CharacterFrequency
{
char ch;
int frequency;
public char getCharacter()
{
return ch;
}
public void setCharacter(char ch)
{
this.ch = ch;
}
public int getfrequency()
{
return frequency;
}
public void setfrequency(int frequency)
{
this.frequency = frequency;
}
static void Main()
{
string OutputFileName;
string InputFileName;
Console.WriteLine("Enter the file path");
InputFileName = Console.ReadLine();
Console.WriteLine("Enter the outputfile name");
OutputFileName = Console.ReadLine();
StreamWriter streamWriter = new StreamWriter(OutputFileName);
string data = File.ReadAllText(InputFileName);
ArrayList al = new ArrayList();
//create two for loops to traverse through the arraylist and compare
for (int i = 0; i < data.Length; i++)
{
int k = 0;
int f = 0;
for (int j = 0; j < data.Length; j++)
{
if (data[i].Equals(data[j]))
{
f++;
if (i > j) { k++; }
}
}
al.Add(data[i] + "(" + (int)data[i] + ")" + f + " ");
foreach (var item in al)
{
streamWriter.WriteLine(item);
}
}
streamWriter.Close();
}
}
}
When I run the program, the program does not stop running and the output file keeps getting larger until it eventually runs out memory and I get an error stating that. I am not seeing where the error is or why the loop won't terminate. It should just count the characters but it seems to keep looping and repeating counting the characters. Any help?
c# arrays arraylist
I need to design a program that reads in an ASCII text file and creates an output file that contains each unique ASCII character and the number of times it appears in the file. Each unique character in the file must be represented by a character frequency class instance. The character frequency objects must be stored in an array list. My code is below:
using System.IO;
using System;
using System.Collections;
namespace ASCII
{
class CharacterFrequency
{
char ch;
int frequency;
public char getCharacter()
{
return ch;
}
public void setCharacter(char ch)
{
this.ch = ch;
}
public int getfrequency()
{
return frequency;
}
public void setfrequency(int frequency)
{
this.frequency = frequency;
}
static void Main()
{
string OutputFileName;
string InputFileName;
Console.WriteLine("Enter the file path");
InputFileName = Console.ReadLine();
Console.WriteLine("Enter the outputfile name");
OutputFileName = Console.ReadLine();
StreamWriter streamWriter = new StreamWriter(OutputFileName);
string data = File.ReadAllText(InputFileName);
ArrayList al = new ArrayList();
//create two for loops to traverse through the arraylist and compare
for (int i = 0; i < data.Length; i++)
{
int k = 0;
int f = 0;
for (int j = 0; j < data.Length; j++)
{
if (data[i].Equals(data[j]))
{
f++;
if (i > j) { k++; }
}
}
al.Add(data[i] + "(" + (int)data[i] + ")" + f + " ");
foreach (var item in al)
{
streamWriter.WriteLine(item);
}
}
streamWriter.Close();
}
}
}
When I run the program, the program does not stop running and the output file keeps getting larger until it eventually runs out memory and I get an error stating that. I am not seeing where the error is or why the loop won't terminate. It should just count the characters but it seems to keep looping and repeating counting the characters. Any help?
c# arrays arraylist
c# arrays arraylist
asked Nov 21 at 1:18
Zeze
82
82
“The character frequency objects must be stored in an array list” Why? ThatCharacterFrequency
class is rather strange as well. Is this C# or Java?
– Dour High Arch
Nov 21 at 1:25
dotnetfiddle.net/hcALTD
– Selvin
Nov 21 at 1:26
Pull that last foreach out from inside the for loop
– baileyrt
Nov 21 at 1:31
If it’s meant to be C# instead of Java use properties instead of getXXX and setXXX methods and use type-safe List<> over the obsolete ArrayList. For example your whole block before the Main method can be reduced to two lines:public char Character { get; set; }
andpublic int Frequency { get; set; }
.
– ckuri
Nov 21 at 1:54
Hmm, the ArrayList is so awkward. Can you get away with also including an object for every C0 Control and Basic Latin character, regardless if it is in the input? That way you can pre-populate it and index it directly based on Char value. When you dump to file, just skip the ones with frequency == 0.
– Tom Blodget
Nov 21 at 3:21
|
show 2 more comments
“The character frequency objects must be stored in an array list” Why? ThatCharacterFrequency
class is rather strange as well. Is this C# or Java?
– Dour High Arch
Nov 21 at 1:25
dotnetfiddle.net/hcALTD
– Selvin
Nov 21 at 1:26
Pull that last foreach out from inside the for loop
– baileyrt
Nov 21 at 1:31
If it’s meant to be C# instead of Java use properties instead of getXXX and setXXX methods and use type-safe List<> over the obsolete ArrayList. For example your whole block before the Main method can be reduced to two lines:public char Character { get; set; }
andpublic int Frequency { get; set; }
.
– ckuri
Nov 21 at 1:54
Hmm, the ArrayList is so awkward. Can you get away with also including an object for every C0 Control and Basic Latin character, regardless if it is in the input? That way you can pre-populate it and index it directly based on Char value. When you dump to file, just skip the ones with frequency == 0.
– Tom Blodget
Nov 21 at 3:21
“The character frequency objects must be stored in an array list” Why? That
CharacterFrequency
class is rather strange as well. Is this C# or Java?– Dour High Arch
Nov 21 at 1:25
“The character frequency objects must be stored in an array list” Why? That
CharacterFrequency
class is rather strange as well. Is this C# or Java?– Dour High Arch
Nov 21 at 1:25
dotnetfiddle.net/hcALTD
– Selvin
Nov 21 at 1:26
dotnetfiddle.net/hcALTD
– Selvin
Nov 21 at 1:26
Pull that last foreach out from inside the for loop
– baileyrt
Nov 21 at 1:31
Pull that last foreach out from inside the for loop
– baileyrt
Nov 21 at 1:31
If it’s meant to be C# instead of Java use properties instead of getXXX and setXXX methods and use type-safe List<> over the obsolete ArrayList. For example your whole block before the Main method can be reduced to two lines:
public char Character { get; set; }
and public int Frequency { get; set; }
.– ckuri
Nov 21 at 1:54
If it’s meant to be C# instead of Java use properties instead of getXXX and setXXX methods and use type-safe List<> over the obsolete ArrayList. For example your whole block before the Main method can be reduced to two lines:
public char Character { get; set; }
and public int Frequency { get; set; }
.– ckuri
Nov 21 at 1:54
Hmm, the ArrayList is so awkward. Can you get away with also including an object for every C0 Control and Basic Latin character, regardless if it is in the input? That way you can pre-populate it and index it directly based on Char value. When you dump to file, just skip the ones with frequency == 0.
– Tom Blodget
Nov 21 at 3:21
Hmm, the ArrayList is so awkward. Can you get away with also including an object for every C0 Control and Basic Latin character, regardless if it is in the input? That way you can pre-populate it and index it directly based on Char value. When you dump to file, just skip the ones with frequency == 0.
– Tom Blodget
Nov 21 at 3:21
|
show 2 more comments
1 Answer
1
active
oldest
votes
Try this approach :
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace yourNamespace
{
class Char_frrequency
{
Dictionary<Char, int> countMap = new Dictionary<char, int>();
public String getStringWithUniqueCharacters(String input)
{
List<Char> uniqueList = new List<Char>();
foreach (Char x in input)
{
if (countMap.ContainsKey(x))
{
countMap[x]++;
}
else
{
countMap.Add(x, 1);
}
if (!uniqueList.Contains(x))
{
uniqueList.Add(x);
}
}
Char uniqueArray = uniqueList.ToArray();
return new String(uniqueArray);
}
public int getFrequency(Char x)
{
return countMap[x];
}
}
}
This might not be the ideal solution. But you can use these methods
Ah, I see you are reading "unique character" as "character occurring exactly once." I think it means to make a different count for each different character value.
– Tom Blodget
Nov 21 at 3:39
1
Since mycountMap
contains only the characters that occurred in the input string, you can get all the unique characters by iterating through the countMap key List
– Gihan Saranga Siriwardhana
Nov 21 at 3:44
1
You should modify your answer and delete theuniqueList
because as you point out it is not necessary. Leaving it in only confuses the issue. The key insight is how aDictionary
naturally captures all the unique characters. DO NOT allow client code to iteratecountMap
. Client code should only be calling methods and not manipulating internal state or have access to any class properties/fields. Add aOccursOnce
method toChar_frrequency
.
– radarbob
Nov 21 at 4:25
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53403982%2fcharacter-counter-using-array-list%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Try this approach :
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace yourNamespace
{
class Char_frrequency
{
Dictionary<Char, int> countMap = new Dictionary<char, int>();
public String getStringWithUniqueCharacters(String input)
{
List<Char> uniqueList = new List<Char>();
foreach (Char x in input)
{
if (countMap.ContainsKey(x))
{
countMap[x]++;
}
else
{
countMap.Add(x, 1);
}
if (!uniqueList.Contains(x))
{
uniqueList.Add(x);
}
}
Char uniqueArray = uniqueList.ToArray();
return new String(uniqueArray);
}
public int getFrequency(Char x)
{
return countMap[x];
}
}
}
This might not be the ideal solution. But you can use these methods
Ah, I see you are reading "unique character" as "character occurring exactly once." I think it means to make a different count for each different character value.
– Tom Blodget
Nov 21 at 3:39
1
Since mycountMap
contains only the characters that occurred in the input string, you can get all the unique characters by iterating through the countMap key List
– Gihan Saranga Siriwardhana
Nov 21 at 3:44
1
You should modify your answer and delete theuniqueList
because as you point out it is not necessary. Leaving it in only confuses the issue. The key insight is how aDictionary
naturally captures all the unique characters. DO NOT allow client code to iteratecountMap
. Client code should only be calling methods and not manipulating internal state or have access to any class properties/fields. Add aOccursOnce
method toChar_frrequency
.
– radarbob
Nov 21 at 4:25
add a comment |
Try this approach :
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace yourNamespace
{
class Char_frrequency
{
Dictionary<Char, int> countMap = new Dictionary<char, int>();
public String getStringWithUniqueCharacters(String input)
{
List<Char> uniqueList = new List<Char>();
foreach (Char x in input)
{
if (countMap.ContainsKey(x))
{
countMap[x]++;
}
else
{
countMap.Add(x, 1);
}
if (!uniqueList.Contains(x))
{
uniqueList.Add(x);
}
}
Char uniqueArray = uniqueList.ToArray();
return new String(uniqueArray);
}
public int getFrequency(Char x)
{
return countMap[x];
}
}
}
This might not be the ideal solution. But you can use these methods
Ah, I see you are reading "unique character" as "character occurring exactly once." I think it means to make a different count for each different character value.
– Tom Blodget
Nov 21 at 3:39
1
Since mycountMap
contains only the characters that occurred in the input string, you can get all the unique characters by iterating through the countMap key List
– Gihan Saranga Siriwardhana
Nov 21 at 3:44
1
You should modify your answer and delete theuniqueList
because as you point out it is not necessary. Leaving it in only confuses the issue. The key insight is how aDictionary
naturally captures all the unique characters. DO NOT allow client code to iteratecountMap
. Client code should only be calling methods and not manipulating internal state or have access to any class properties/fields. Add aOccursOnce
method toChar_frrequency
.
– radarbob
Nov 21 at 4:25
add a comment |
Try this approach :
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace yourNamespace
{
class Char_frrequency
{
Dictionary<Char, int> countMap = new Dictionary<char, int>();
public String getStringWithUniqueCharacters(String input)
{
List<Char> uniqueList = new List<Char>();
foreach (Char x in input)
{
if (countMap.ContainsKey(x))
{
countMap[x]++;
}
else
{
countMap.Add(x, 1);
}
if (!uniqueList.Contains(x))
{
uniqueList.Add(x);
}
}
Char uniqueArray = uniqueList.ToArray();
return new String(uniqueArray);
}
public int getFrequency(Char x)
{
return countMap[x];
}
}
}
This might not be the ideal solution. But you can use these methods
Try this approach :
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace yourNamespace
{
class Char_frrequency
{
Dictionary<Char, int> countMap = new Dictionary<char, int>();
public String getStringWithUniqueCharacters(String input)
{
List<Char> uniqueList = new List<Char>();
foreach (Char x in input)
{
if (countMap.ContainsKey(x))
{
countMap[x]++;
}
else
{
countMap.Add(x, 1);
}
if (!uniqueList.Contains(x))
{
uniqueList.Add(x);
}
}
Char uniqueArray = uniqueList.ToArray();
return new String(uniqueArray);
}
public int getFrequency(Char x)
{
return countMap[x];
}
}
}
This might not be the ideal solution. But you can use these methods
edited Nov 21 at 3:30
answered Nov 21 at 3:14


Gihan Saranga Siriwardhana
625420
625420
Ah, I see you are reading "unique character" as "character occurring exactly once." I think it means to make a different count for each different character value.
– Tom Blodget
Nov 21 at 3:39
1
Since mycountMap
contains only the characters that occurred in the input string, you can get all the unique characters by iterating through the countMap key List
– Gihan Saranga Siriwardhana
Nov 21 at 3:44
1
You should modify your answer and delete theuniqueList
because as you point out it is not necessary. Leaving it in only confuses the issue. The key insight is how aDictionary
naturally captures all the unique characters. DO NOT allow client code to iteratecountMap
. Client code should only be calling methods and not manipulating internal state or have access to any class properties/fields. Add aOccursOnce
method toChar_frrequency
.
– radarbob
Nov 21 at 4:25
add a comment |
Ah, I see you are reading "unique character" as "character occurring exactly once." I think it means to make a different count for each different character value.
– Tom Blodget
Nov 21 at 3:39
1
Since mycountMap
contains only the characters that occurred in the input string, you can get all the unique characters by iterating through the countMap key List
– Gihan Saranga Siriwardhana
Nov 21 at 3:44
1
You should modify your answer and delete theuniqueList
because as you point out it is not necessary. Leaving it in only confuses the issue. The key insight is how aDictionary
naturally captures all the unique characters. DO NOT allow client code to iteratecountMap
. Client code should only be calling methods and not manipulating internal state or have access to any class properties/fields. Add aOccursOnce
method toChar_frrequency
.
– radarbob
Nov 21 at 4:25
Ah, I see you are reading "unique character" as "character occurring exactly once." I think it means to make a different count for each different character value.
– Tom Blodget
Nov 21 at 3:39
Ah, I see you are reading "unique character" as "character occurring exactly once." I think it means to make a different count for each different character value.
– Tom Blodget
Nov 21 at 3:39
1
1
Since my
countMap
contains only the characters that occurred in the input string, you can get all the unique characters by iterating through the countMap key List– Gihan Saranga Siriwardhana
Nov 21 at 3:44
Since my
countMap
contains only the characters that occurred in the input string, you can get all the unique characters by iterating through the countMap key List– Gihan Saranga Siriwardhana
Nov 21 at 3:44
1
1
You should modify your answer and delete the
uniqueList
because as you point out it is not necessary. Leaving it in only confuses the issue. The key insight is how a Dictionary
naturally captures all the unique characters. DO NOT allow client code to iterate countMap
. Client code should only be calling methods and not manipulating internal state or have access to any class properties/fields. Add a OccursOnce
method to Char_frrequency
.– radarbob
Nov 21 at 4:25
You should modify your answer and delete the
uniqueList
because as you point out it is not necessary. Leaving it in only confuses the issue. The key insight is how a Dictionary
naturally captures all the unique characters. DO NOT allow client code to iterate countMap
. Client code should only be calling methods and not manipulating internal state or have access to any class properties/fields. Add a OccursOnce
method to Char_frrequency
.– radarbob
Nov 21 at 4:25
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53403982%2fcharacter-counter-using-array-list%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ffz,ISpQb FAzrtUXYxSMZD2d,m6aQQApdqoVvS,q2sPwLdqMuDaWd
“The character frequency objects must be stored in an array list” Why? That
CharacterFrequency
class is rather strange as well. Is this C# or Java?– Dour High Arch
Nov 21 at 1:25
dotnetfiddle.net/hcALTD
– Selvin
Nov 21 at 1:26
Pull that last foreach out from inside the for loop
– baileyrt
Nov 21 at 1:31
If it’s meant to be C# instead of Java use properties instead of getXXX and setXXX methods and use type-safe List<> over the obsolete ArrayList. For example your whole block before the Main method can be reduced to two lines:
public char Character { get; set; }
andpublic int Frequency { get; set; }
.– ckuri
Nov 21 at 1:54
Hmm, the ArrayList is so awkward. Can you get away with also including an object for every C0 Control and Basic Latin character, regardless if it is in the input? That way you can pre-populate it and index it directly based on Char value. When you dump to file, just skip the ones with frequency == 0.
– Tom Blodget
Nov 21 at 3:21