Re-prompt user to input a valid input in java
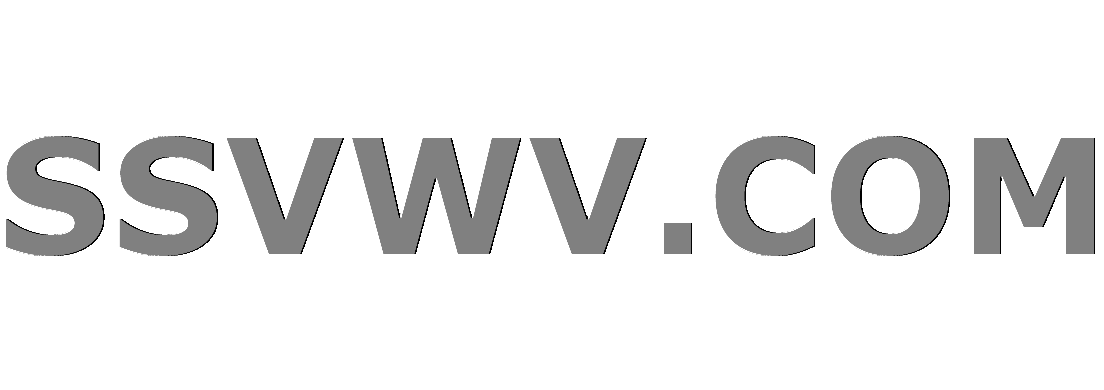
Multi tool use
I have a code that requests input from the user, it runs just fine however I want to re-prompt the user to input a valid response instead of continuing to run.
Here's the code:
import java.io.*;
public class SkiLodge {
public static void main(String args) throws Exception
{
InputStreamReader ui = new InputStreamReader (System.in);
BufferedReader inputReader = new BufferedReader(ui);
System.out.println("Select Membership Type: (Gold/Silver/Bronze)");
String membership = inputReader.readLine();
double x = 0d;
if (membership.equals("Gold"))
System.out.println("Gold Membership Selected: £" + (x = 750));
else if (membership.equals("Silver"))
System.out.println("Silver Membership Selected: £" + (x = 450));
else if (membership.equals("Bronze"))
System.out.println("Bronze Membership Selected: £" + (x = 250));
else System.out.println("Invalid Membership");
System.out.println("Do you require snow tyre rental? (yes/no)");
String tyreRental = inputReader.readLine();
if (tyreRental.equals("yes"))
System.out.println("The total so far with tyre rental: £" + (x = x * 1.1));
else if (tyreRental.equals("no"))
System.out.println("The total so far without tyre rental: £" + x);
else System.out.println("Invalid response");
System.out.println("Would you like to reserve parking? (yes/no)");
String parking = inputReader.readLine();
if (parking.equals("yes"))
System.out.println("Membership total: £" + (x = x * 1.08));
else if (parking.equals("no"))
System.out.println("Membership total: £" + x);
else System.out.println("Invalid response");
}
}
So for example if the user inputs Gold, the program prints out 750, if not then it prints out invalid membership and asks for a valid one.
Same goes for the following questions, so the program only accepts yes and no as valid responses.
Thanks in advance
java
add a comment |
I have a code that requests input from the user, it runs just fine however I want to re-prompt the user to input a valid response instead of continuing to run.
Here's the code:
import java.io.*;
public class SkiLodge {
public static void main(String args) throws Exception
{
InputStreamReader ui = new InputStreamReader (System.in);
BufferedReader inputReader = new BufferedReader(ui);
System.out.println("Select Membership Type: (Gold/Silver/Bronze)");
String membership = inputReader.readLine();
double x = 0d;
if (membership.equals("Gold"))
System.out.println("Gold Membership Selected: £" + (x = 750));
else if (membership.equals("Silver"))
System.out.println("Silver Membership Selected: £" + (x = 450));
else if (membership.equals("Bronze"))
System.out.println("Bronze Membership Selected: £" + (x = 250));
else System.out.println("Invalid Membership");
System.out.println("Do you require snow tyre rental? (yes/no)");
String tyreRental = inputReader.readLine();
if (tyreRental.equals("yes"))
System.out.println("The total so far with tyre rental: £" + (x = x * 1.1));
else if (tyreRental.equals("no"))
System.out.println("The total so far without tyre rental: £" + x);
else System.out.println("Invalid response");
System.out.println("Would you like to reserve parking? (yes/no)");
String parking = inputReader.readLine();
if (parking.equals("yes"))
System.out.println("Membership total: £" + (x = x * 1.08));
else if (parking.equals("no"))
System.out.println("Membership total: £" + x);
else System.out.println("Invalid response");
}
}
So for example if the user inputs Gold, the program prints out 750, if not then it prints out invalid membership and asks for a valid one.
Same goes for the following questions, so the program only accepts yes and no as valid responses.
Thanks in advance
java
add a comment |
I have a code that requests input from the user, it runs just fine however I want to re-prompt the user to input a valid response instead of continuing to run.
Here's the code:
import java.io.*;
public class SkiLodge {
public static void main(String args) throws Exception
{
InputStreamReader ui = new InputStreamReader (System.in);
BufferedReader inputReader = new BufferedReader(ui);
System.out.println("Select Membership Type: (Gold/Silver/Bronze)");
String membership = inputReader.readLine();
double x = 0d;
if (membership.equals("Gold"))
System.out.println("Gold Membership Selected: £" + (x = 750));
else if (membership.equals("Silver"))
System.out.println("Silver Membership Selected: £" + (x = 450));
else if (membership.equals("Bronze"))
System.out.println("Bronze Membership Selected: £" + (x = 250));
else System.out.println("Invalid Membership");
System.out.println("Do you require snow tyre rental? (yes/no)");
String tyreRental = inputReader.readLine();
if (tyreRental.equals("yes"))
System.out.println("The total so far with tyre rental: £" + (x = x * 1.1));
else if (tyreRental.equals("no"))
System.out.println("The total so far without tyre rental: £" + x);
else System.out.println("Invalid response");
System.out.println("Would you like to reserve parking? (yes/no)");
String parking = inputReader.readLine();
if (parking.equals("yes"))
System.out.println("Membership total: £" + (x = x * 1.08));
else if (parking.equals("no"))
System.out.println("Membership total: £" + x);
else System.out.println("Invalid response");
}
}
So for example if the user inputs Gold, the program prints out 750, if not then it prints out invalid membership and asks for a valid one.
Same goes for the following questions, so the program only accepts yes and no as valid responses.
Thanks in advance
java
I have a code that requests input from the user, it runs just fine however I want to re-prompt the user to input a valid response instead of continuing to run.
Here's the code:
import java.io.*;
public class SkiLodge {
public static void main(String args) throws Exception
{
InputStreamReader ui = new InputStreamReader (System.in);
BufferedReader inputReader = new BufferedReader(ui);
System.out.println("Select Membership Type: (Gold/Silver/Bronze)");
String membership = inputReader.readLine();
double x = 0d;
if (membership.equals("Gold"))
System.out.println("Gold Membership Selected: £" + (x = 750));
else if (membership.equals("Silver"))
System.out.println("Silver Membership Selected: £" + (x = 450));
else if (membership.equals("Bronze"))
System.out.println("Bronze Membership Selected: £" + (x = 250));
else System.out.println("Invalid Membership");
System.out.println("Do you require snow tyre rental? (yes/no)");
String tyreRental = inputReader.readLine();
if (tyreRental.equals("yes"))
System.out.println("The total so far with tyre rental: £" + (x = x * 1.1));
else if (tyreRental.equals("no"))
System.out.println("The total so far without tyre rental: £" + x);
else System.out.println("Invalid response");
System.out.println("Would you like to reserve parking? (yes/no)");
String parking = inputReader.readLine();
if (parking.equals("yes"))
System.out.println("Membership total: £" + (x = x * 1.08));
else if (parking.equals("no"))
System.out.println("Membership total: £" + x);
else System.out.println("Invalid response");
}
}
So for example if the user inputs Gold, the program prints out 750, if not then it prints out invalid membership and asks for a valid one.
Same goes for the following questions, so the program only accepts yes and no as valid responses.
Thanks in advance
java
java
asked Apr 9 '16 at 11:42
Tariq Odeh
113
113
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
You can use a do-while loop.
do{
//Some code here
//if block
else { // last else block.
System.out.println("Invalid Membership");
continue; // This will skip the rest of the code and move again to the beginning of the while loop.
}
//More code
break; // If the condition was correct, then you need not worry. This statement will pull out of the loop.
}while(true);
You can modify this to put a do-while loop any where you have such requirement.
This would make your whole code to:
public static void main(String args) throws Exception
{
InputStreamReader ui = new InputStreamReader (System.in);
BufferedReader inputReader = new BufferedReader(ui);
String membership, tyreRental,parking;
double x = 0d;
do
{
System.out.println("Select Membership Type: (Gold/Silver/Bronze)");
membership = inputReader.readLine();
if (membership.equals("Gold"))
System.out.println("Gold Membership Selected: £" + (x = 750));
else if (membership.equals("Silver"))
System.out.println("Silver Membership Selected: £" + (x = 450));
else if (membership.equals("Bronze"))
System.out.println("Bronze Membership Selected: £" + (x = 250));
else{
System.out.println("Invalid Membership");
continue;
}
break;
}while(true);
do{
System.out.println("Do you require snow tyre rental? (yes/no)");
tyreRental = inputReader.readLine();
if (tyreRental.equals("yes"))
System.out.println("The total so far with tyre rental: £" + (x = x * 1.1));
else if (tyreRental.equals("no"))
System.out.println("The total so far without tyre rental: £" + x);
else{ System.out.println("Invalid response"); continue;}
break;
}while(true);
do{
System.out.println("Would you like to reserve parking? (yes/no)");
parking = inputReader.readLine();
if (parking.equals("yes"))
System.out.println("Membership total: £" + (x = x * 1.08));
else if (parking.equals("no"))
System.out.println("Membership total: £" + x);
else {System.out.println("Invalid response"); continue;}
break;
}while(true);
}
add a comment |
Unfortunately there's no really elegant way of avoiding two input statements in this situation. There are various ways of doing this but I find the neatest is the most straightforward:
System.out.println("Select Membership Type: (Gold/Silver/Bronze)");
String membership = inputReader.readLine();
while (!membership.matches("Gold|Silver|Bronze")) {
System.out.println("Illegal membership Type; please enter one of Gold, Silver or Bronze");
membership = inputReader.readLine();
}
Ideally you would encapsulate some of that logic so that you weren't repeating it in several places. That will make your code a bit neater. For example:
enum Membership {
GOLD(750),
SILVER(450),
BRONZE(250);
private int value;
Membership(int value) {
this.value = value;
}
public int getValue() {
return value;
}
public static Membership getByName(String name) {
for (Membership membership: values())
if (name.toUpperCase().equals(membership.name))
return membership;
return null;
}
}
System.out.println("Select membership type: " + Membership.values());
Membership membership = Membership.getByName(inputReader.readLine());
while (membership == null) {
System.out.println("Illegal membership Type; enter one of " + Membership.values());
membership = Membership.getByName(inputReader.readLine());
}
int x = membership.getValue();
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f36516266%2fre-prompt-user-to-input-a-valid-input-in-java%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can use a do-while loop.
do{
//Some code here
//if block
else { // last else block.
System.out.println("Invalid Membership");
continue; // This will skip the rest of the code and move again to the beginning of the while loop.
}
//More code
break; // If the condition was correct, then you need not worry. This statement will pull out of the loop.
}while(true);
You can modify this to put a do-while loop any where you have such requirement.
This would make your whole code to:
public static void main(String args) throws Exception
{
InputStreamReader ui = new InputStreamReader (System.in);
BufferedReader inputReader = new BufferedReader(ui);
String membership, tyreRental,parking;
double x = 0d;
do
{
System.out.println("Select Membership Type: (Gold/Silver/Bronze)");
membership = inputReader.readLine();
if (membership.equals("Gold"))
System.out.println("Gold Membership Selected: £" + (x = 750));
else if (membership.equals("Silver"))
System.out.println("Silver Membership Selected: £" + (x = 450));
else if (membership.equals("Bronze"))
System.out.println("Bronze Membership Selected: £" + (x = 250));
else{
System.out.println("Invalid Membership");
continue;
}
break;
}while(true);
do{
System.out.println("Do you require snow tyre rental? (yes/no)");
tyreRental = inputReader.readLine();
if (tyreRental.equals("yes"))
System.out.println("The total so far with tyre rental: £" + (x = x * 1.1));
else if (tyreRental.equals("no"))
System.out.println("The total so far without tyre rental: £" + x);
else{ System.out.println("Invalid response"); continue;}
break;
}while(true);
do{
System.out.println("Would you like to reserve parking? (yes/no)");
parking = inputReader.readLine();
if (parking.equals("yes"))
System.out.println("Membership total: £" + (x = x * 1.08));
else if (parking.equals("no"))
System.out.println("Membership total: £" + x);
else {System.out.println("Invalid response"); continue;}
break;
}while(true);
}
add a comment |
You can use a do-while loop.
do{
//Some code here
//if block
else { // last else block.
System.out.println("Invalid Membership");
continue; // This will skip the rest of the code and move again to the beginning of the while loop.
}
//More code
break; // If the condition was correct, then you need not worry. This statement will pull out of the loop.
}while(true);
You can modify this to put a do-while loop any where you have such requirement.
This would make your whole code to:
public static void main(String args) throws Exception
{
InputStreamReader ui = new InputStreamReader (System.in);
BufferedReader inputReader = new BufferedReader(ui);
String membership, tyreRental,parking;
double x = 0d;
do
{
System.out.println("Select Membership Type: (Gold/Silver/Bronze)");
membership = inputReader.readLine();
if (membership.equals("Gold"))
System.out.println("Gold Membership Selected: £" + (x = 750));
else if (membership.equals("Silver"))
System.out.println("Silver Membership Selected: £" + (x = 450));
else if (membership.equals("Bronze"))
System.out.println("Bronze Membership Selected: £" + (x = 250));
else{
System.out.println("Invalid Membership");
continue;
}
break;
}while(true);
do{
System.out.println("Do you require snow tyre rental? (yes/no)");
tyreRental = inputReader.readLine();
if (tyreRental.equals("yes"))
System.out.println("The total so far with tyre rental: £" + (x = x * 1.1));
else if (tyreRental.equals("no"))
System.out.println("The total so far without tyre rental: £" + x);
else{ System.out.println("Invalid response"); continue;}
break;
}while(true);
do{
System.out.println("Would you like to reserve parking? (yes/no)");
parking = inputReader.readLine();
if (parking.equals("yes"))
System.out.println("Membership total: £" + (x = x * 1.08));
else if (parking.equals("no"))
System.out.println("Membership total: £" + x);
else {System.out.println("Invalid response"); continue;}
break;
}while(true);
}
add a comment |
You can use a do-while loop.
do{
//Some code here
//if block
else { // last else block.
System.out.println("Invalid Membership");
continue; // This will skip the rest of the code and move again to the beginning of the while loop.
}
//More code
break; // If the condition was correct, then you need not worry. This statement will pull out of the loop.
}while(true);
You can modify this to put a do-while loop any where you have such requirement.
This would make your whole code to:
public static void main(String args) throws Exception
{
InputStreamReader ui = new InputStreamReader (System.in);
BufferedReader inputReader = new BufferedReader(ui);
String membership, tyreRental,parking;
double x = 0d;
do
{
System.out.println("Select Membership Type: (Gold/Silver/Bronze)");
membership = inputReader.readLine();
if (membership.equals("Gold"))
System.out.println("Gold Membership Selected: £" + (x = 750));
else if (membership.equals("Silver"))
System.out.println("Silver Membership Selected: £" + (x = 450));
else if (membership.equals("Bronze"))
System.out.println("Bronze Membership Selected: £" + (x = 250));
else{
System.out.println("Invalid Membership");
continue;
}
break;
}while(true);
do{
System.out.println("Do you require snow tyre rental? (yes/no)");
tyreRental = inputReader.readLine();
if (tyreRental.equals("yes"))
System.out.println("The total so far with tyre rental: £" + (x = x * 1.1));
else if (tyreRental.equals("no"))
System.out.println("The total so far without tyre rental: £" + x);
else{ System.out.println("Invalid response"); continue;}
break;
}while(true);
do{
System.out.println("Would you like to reserve parking? (yes/no)");
parking = inputReader.readLine();
if (parking.equals("yes"))
System.out.println("Membership total: £" + (x = x * 1.08));
else if (parking.equals("no"))
System.out.println("Membership total: £" + x);
else {System.out.println("Invalid response"); continue;}
break;
}while(true);
}
You can use a do-while loop.
do{
//Some code here
//if block
else { // last else block.
System.out.println("Invalid Membership");
continue; // This will skip the rest of the code and move again to the beginning of the while loop.
}
//More code
break; // If the condition was correct, then you need not worry. This statement will pull out of the loop.
}while(true);
You can modify this to put a do-while loop any where you have such requirement.
This would make your whole code to:
public static void main(String args) throws Exception
{
InputStreamReader ui = new InputStreamReader (System.in);
BufferedReader inputReader = new BufferedReader(ui);
String membership, tyreRental,parking;
double x = 0d;
do
{
System.out.println("Select Membership Type: (Gold/Silver/Bronze)");
membership = inputReader.readLine();
if (membership.equals("Gold"))
System.out.println("Gold Membership Selected: £" + (x = 750));
else if (membership.equals("Silver"))
System.out.println("Silver Membership Selected: £" + (x = 450));
else if (membership.equals("Bronze"))
System.out.println("Bronze Membership Selected: £" + (x = 250));
else{
System.out.println("Invalid Membership");
continue;
}
break;
}while(true);
do{
System.out.println("Do you require snow tyre rental? (yes/no)");
tyreRental = inputReader.readLine();
if (tyreRental.equals("yes"))
System.out.println("The total so far with tyre rental: £" + (x = x * 1.1));
else if (tyreRental.equals("no"))
System.out.println("The total so far without tyre rental: £" + x);
else{ System.out.println("Invalid response"); continue;}
break;
}while(true);
do{
System.out.println("Would you like to reserve parking? (yes/no)");
parking = inputReader.readLine();
if (parking.equals("yes"))
System.out.println("Membership total: £" + (x = x * 1.08));
else if (parking.equals("no"))
System.out.println("Membership total: £" + x);
else {System.out.println("Invalid response"); continue;}
break;
}while(true);
}
edited Apr 9 '16 at 12:01
answered Apr 9 '16 at 11:44


dryairship
4,59721844
4,59721844
add a comment |
add a comment |
Unfortunately there's no really elegant way of avoiding two input statements in this situation. There are various ways of doing this but I find the neatest is the most straightforward:
System.out.println("Select Membership Type: (Gold/Silver/Bronze)");
String membership = inputReader.readLine();
while (!membership.matches("Gold|Silver|Bronze")) {
System.out.println("Illegal membership Type; please enter one of Gold, Silver or Bronze");
membership = inputReader.readLine();
}
Ideally you would encapsulate some of that logic so that you weren't repeating it in several places. That will make your code a bit neater. For example:
enum Membership {
GOLD(750),
SILVER(450),
BRONZE(250);
private int value;
Membership(int value) {
this.value = value;
}
public int getValue() {
return value;
}
public static Membership getByName(String name) {
for (Membership membership: values())
if (name.toUpperCase().equals(membership.name))
return membership;
return null;
}
}
System.out.println("Select membership type: " + Membership.values());
Membership membership = Membership.getByName(inputReader.readLine());
while (membership == null) {
System.out.println("Illegal membership Type; enter one of " + Membership.values());
membership = Membership.getByName(inputReader.readLine());
}
int x = membership.getValue();
add a comment |
Unfortunately there's no really elegant way of avoiding two input statements in this situation. There are various ways of doing this but I find the neatest is the most straightforward:
System.out.println("Select Membership Type: (Gold/Silver/Bronze)");
String membership = inputReader.readLine();
while (!membership.matches("Gold|Silver|Bronze")) {
System.out.println("Illegal membership Type; please enter one of Gold, Silver or Bronze");
membership = inputReader.readLine();
}
Ideally you would encapsulate some of that logic so that you weren't repeating it in several places. That will make your code a bit neater. For example:
enum Membership {
GOLD(750),
SILVER(450),
BRONZE(250);
private int value;
Membership(int value) {
this.value = value;
}
public int getValue() {
return value;
}
public static Membership getByName(String name) {
for (Membership membership: values())
if (name.toUpperCase().equals(membership.name))
return membership;
return null;
}
}
System.out.println("Select membership type: " + Membership.values());
Membership membership = Membership.getByName(inputReader.readLine());
while (membership == null) {
System.out.println("Illegal membership Type; enter one of " + Membership.values());
membership = Membership.getByName(inputReader.readLine());
}
int x = membership.getValue();
add a comment |
Unfortunately there's no really elegant way of avoiding two input statements in this situation. There are various ways of doing this but I find the neatest is the most straightforward:
System.out.println("Select Membership Type: (Gold/Silver/Bronze)");
String membership = inputReader.readLine();
while (!membership.matches("Gold|Silver|Bronze")) {
System.out.println("Illegal membership Type; please enter one of Gold, Silver or Bronze");
membership = inputReader.readLine();
}
Ideally you would encapsulate some of that logic so that you weren't repeating it in several places. That will make your code a bit neater. For example:
enum Membership {
GOLD(750),
SILVER(450),
BRONZE(250);
private int value;
Membership(int value) {
this.value = value;
}
public int getValue() {
return value;
}
public static Membership getByName(String name) {
for (Membership membership: values())
if (name.toUpperCase().equals(membership.name))
return membership;
return null;
}
}
System.out.println("Select membership type: " + Membership.values());
Membership membership = Membership.getByName(inputReader.readLine());
while (membership == null) {
System.out.println("Illegal membership Type; enter one of " + Membership.values());
membership = Membership.getByName(inputReader.readLine());
}
int x = membership.getValue();
Unfortunately there's no really elegant way of avoiding two input statements in this situation. There are various ways of doing this but I find the neatest is the most straightforward:
System.out.println("Select Membership Type: (Gold/Silver/Bronze)");
String membership = inputReader.readLine();
while (!membership.matches("Gold|Silver|Bronze")) {
System.out.println("Illegal membership Type; please enter one of Gold, Silver or Bronze");
membership = inputReader.readLine();
}
Ideally you would encapsulate some of that logic so that you weren't repeating it in several places. That will make your code a bit neater. For example:
enum Membership {
GOLD(750),
SILVER(450),
BRONZE(250);
private int value;
Membership(int value) {
this.value = value;
}
public int getValue() {
return value;
}
public static Membership getByName(String name) {
for (Membership membership: values())
if (name.toUpperCase().equals(membership.name))
return membership;
return null;
}
}
System.out.println("Select membership type: " + Membership.values());
Membership membership = Membership.getByName(inputReader.readLine());
while (membership == null) {
System.out.println("Illegal membership Type; enter one of " + Membership.values());
membership = Membership.getByName(inputReader.readLine());
}
int x = membership.getValue();
edited Apr 9 '16 at 12:44
answered Apr 9 '16 at 12:32
sprinter
16.3k32760
16.3k32760
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f36516266%2fre-prompt-user-to-input-a-valid-input-in-java%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
JSU,i1Xo9,lEd,f0n5nGibRe,ao0