No onMessage events on Runtime (Java JMS MessageListener on Oracle Queue)
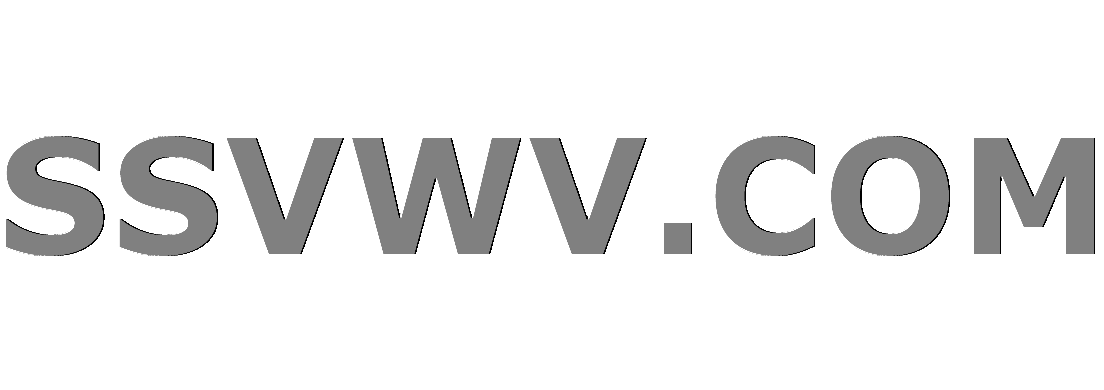
Multi tool use
up vote
0
down vote
favorite
Purpose
I created MyListener.java
to monitor my Oracle Queue MY_QUEUE and MyConsumer.java
implement my own MessageListener.onMessage
functionality.
As soon as I enqueue some entries into MY_QUEUE I want the MessageListener to output "New Message..." onto the console.
Problem
The Queue entries will only be processed on the initial application start. If additional entries get enqueued while the application is already runnning the MessageListener.onMessage function won't get triggered.
Example
Run Application with 5 entries already in queue. Output:
Initialized...
Sleeping...
New Message...
New Message...
New Message...
New Message...
New Message...
Sleeping...
Sleeping...
Quit Application and run the Application. Enqueue entries on runtime. Output:
Initialized...
Sleeping...
Sleeping...
Sleeping...
Sleeping... (Queue entries got inserted about at this time)
Sleeping...
Sleeping...
Sleeping...
Sleeping...
Quit Application and run the Application again (Entries from 2. are still in queue). Output:
Initialized...
Sleeping...
New Message...
New Message...
New Message...
New Message...
New Message...
Sleeping...
Sleeping...
MyListener
package example;
import javax.jms.JMSException;
import javax.jms.MessageConsumer;
import javax.jms.Queue;
import javax.jms.QueueConnection;
import javax.jms.QueueConnectionFactory;
import javax.jms.QueueSession;
import javax.jms.Session;
import oracle.jms.AQjmsFactory;
import oracle.jms.AQjmsSession;
public class MyListener {
private static final String QUEUE_NAME = "MY_QUEUE";
private static final String QUEUE_USER = "myuser";
private static final String QUEUE_PW = "mypassword";
private QueueConnection queueConnection;
private QueueSession queueSession;
public MyListener() throws JMSException {
QueueConnectionFactory QFac = AQjmsFactory.getQueueConnectionFactory("xxx.xxx.xxx.xxx", "orcl", 1521, "thin");
this.queueConnection = QFac.createQueueConnection(QUEUE_USER, QUEUE_PW);
this.queueSession = this.queueConnection.createQueueSession(false, Session.AUTO_ACKNOWLEDGE);
}
public static void main(String args) {
try {
MyListener myListener = new MyListener();
Queue queue = ((AQjmsSession) myListener.queueSession).getQueue(QUEUE_USER, QUEUE_NAME);
MessageConsumer mq = ((AQjmsSession) myListener.queueSession).createReceiver(queue);
MyConsumer mc = new MyConsumer();
mq.setMessageListener(mc);
myListener.queueConnection.start();
System.out.println("Initialized...");
while (true) {
try {
System.out.println("Sleeping...");
Thread.sleep(2000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
} catch (JMSException e) {
e.printStackTrace();
} finally {
System.out.println("Application closed");
}
}
}
MyConsumer
package example;
import javax.jms.Message;
import javax.jms.MessageListener;
public class MyConsumer implements MessageListener{
@Override
public void onMessage(Message arg0) {
System.out.println("New Message...");
}
}
PL/SQL script to enqueue entries
DECLARE
msg SYS.aq$_jms_text_message;
enqueue_options DBMS_AQ.ENQUEUE_OPTIONS_T;
message_properties DBMS_AQ.MESSAGE_PROPERTIES_T;
message_handle RAW (16);
i NUMBER;
BEGIN
msg := sys.aq$_jms_text_message.construct;
msg.set_text ('Testmessage');
enqueue_options.visibility := DBMS_AQ.immediate;
message_properties.priority := 1;
i := 0;
WHILE i < 5
LOOP
DBMS_AQ.enqueue (queue_name => 'MY_QUEUE',
enqueue_options => enqueue_options,
message_properties => message_properties,
payload => msg,
msgid => message_handle);
i := i + 1;
END LOOP;
COMMIT;
END;
Additional Information
Database: Oracle 11g2
Java Runtime: 1.6
Maven Dependencies:
- oracle-jdbc (11.2.0.4.0)
- xdb (1.0)
- aqapi (1.3)
- jmscommon (1.3.1_02)
Can someone tell me why the onMessage function won't get triggered once I enqueue new entries on runtime?
java oracle queue jms listener
add a comment |
up vote
0
down vote
favorite
Purpose
I created MyListener.java
to monitor my Oracle Queue MY_QUEUE and MyConsumer.java
implement my own MessageListener.onMessage
functionality.
As soon as I enqueue some entries into MY_QUEUE I want the MessageListener to output "New Message..." onto the console.
Problem
The Queue entries will only be processed on the initial application start. If additional entries get enqueued while the application is already runnning the MessageListener.onMessage function won't get triggered.
Example
Run Application with 5 entries already in queue. Output:
Initialized...
Sleeping...
New Message...
New Message...
New Message...
New Message...
New Message...
Sleeping...
Sleeping...
Quit Application and run the Application. Enqueue entries on runtime. Output:
Initialized...
Sleeping...
Sleeping...
Sleeping...
Sleeping... (Queue entries got inserted about at this time)
Sleeping...
Sleeping...
Sleeping...
Sleeping...
Quit Application and run the Application again (Entries from 2. are still in queue). Output:
Initialized...
Sleeping...
New Message...
New Message...
New Message...
New Message...
New Message...
Sleeping...
Sleeping...
MyListener
package example;
import javax.jms.JMSException;
import javax.jms.MessageConsumer;
import javax.jms.Queue;
import javax.jms.QueueConnection;
import javax.jms.QueueConnectionFactory;
import javax.jms.QueueSession;
import javax.jms.Session;
import oracle.jms.AQjmsFactory;
import oracle.jms.AQjmsSession;
public class MyListener {
private static final String QUEUE_NAME = "MY_QUEUE";
private static final String QUEUE_USER = "myuser";
private static final String QUEUE_PW = "mypassword";
private QueueConnection queueConnection;
private QueueSession queueSession;
public MyListener() throws JMSException {
QueueConnectionFactory QFac = AQjmsFactory.getQueueConnectionFactory("xxx.xxx.xxx.xxx", "orcl", 1521, "thin");
this.queueConnection = QFac.createQueueConnection(QUEUE_USER, QUEUE_PW);
this.queueSession = this.queueConnection.createQueueSession(false, Session.AUTO_ACKNOWLEDGE);
}
public static void main(String args) {
try {
MyListener myListener = new MyListener();
Queue queue = ((AQjmsSession) myListener.queueSession).getQueue(QUEUE_USER, QUEUE_NAME);
MessageConsumer mq = ((AQjmsSession) myListener.queueSession).createReceiver(queue);
MyConsumer mc = new MyConsumer();
mq.setMessageListener(mc);
myListener.queueConnection.start();
System.out.println("Initialized...");
while (true) {
try {
System.out.println("Sleeping...");
Thread.sleep(2000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
} catch (JMSException e) {
e.printStackTrace();
} finally {
System.out.println("Application closed");
}
}
}
MyConsumer
package example;
import javax.jms.Message;
import javax.jms.MessageListener;
public class MyConsumer implements MessageListener{
@Override
public void onMessage(Message arg0) {
System.out.println("New Message...");
}
}
PL/SQL script to enqueue entries
DECLARE
msg SYS.aq$_jms_text_message;
enqueue_options DBMS_AQ.ENQUEUE_OPTIONS_T;
message_properties DBMS_AQ.MESSAGE_PROPERTIES_T;
message_handle RAW (16);
i NUMBER;
BEGIN
msg := sys.aq$_jms_text_message.construct;
msg.set_text ('Testmessage');
enqueue_options.visibility := DBMS_AQ.immediate;
message_properties.priority := 1;
i := 0;
WHILE i < 5
LOOP
DBMS_AQ.enqueue (queue_name => 'MY_QUEUE',
enqueue_options => enqueue_options,
message_properties => message_properties,
payload => msg,
msgid => message_handle);
i := i + 1;
END LOOP;
COMMIT;
END;
Additional Information
Database: Oracle 11g2
Java Runtime: 1.6
Maven Dependencies:
- oracle-jdbc (11.2.0.4.0)
- xdb (1.0)
- aqapi (1.3)
- jmscommon (1.3.1_02)
Can someone tell me why the onMessage function won't get triggered once I enqueue new entries on runtime?
java oracle queue jms listener
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
Purpose
I created MyListener.java
to monitor my Oracle Queue MY_QUEUE and MyConsumer.java
implement my own MessageListener.onMessage
functionality.
As soon as I enqueue some entries into MY_QUEUE I want the MessageListener to output "New Message..." onto the console.
Problem
The Queue entries will only be processed on the initial application start. If additional entries get enqueued while the application is already runnning the MessageListener.onMessage function won't get triggered.
Example
Run Application with 5 entries already in queue. Output:
Initialized...
Sleeping...
New Message...
New Message...
New Message...
New Message...
New Message...
Sleeping...
Sleeping...
Quit Application and run the Application. Enqueue entries on runtime. Output:
Initialized...
Sleeping...
Sleeping...
Sleeping...
Sleeping... (Queue entries got inserted about at this time)
Sleeping...
Sleeping...
Sleeping...
Sleeping...
Quit Application and run the Application again (Entries from 2. are still in queue). Output:
Initialized...
Sleeping...
New Message...
New Message...
New Message...
New Message...
New Message...
Sleeping...
Sleeping...
MyListener
package example;
import javax.jms.JMSException;
import javax.jms.MessageConsumer;
import javax.jms.Queue;
import javax.jms.QueueConnection;
import javax.jms.QueueConnectionFactory;
import javax.jms.QueueSession;
import javax.jms.Session;
import oracle.jms.AQjmsFactory;
import oracle.jms.AQjmsSession;
public class MyListener {
private static final String QUEUE_NAME = "MY_QUEUE";
private static final String QUEUE_USER = "myuser";
private static final String QUEUE_PW = "mypassword";
private QueueConnection queueConnection;
private QueueSession queueSession;
public MyListener() throws JMSException {
QueueConnectionFactory QFac = AQjmsFactory.getQueueConnectionFactory("xxx.xxx.xxx.xxx", "orcl", 1521, "thin");
this.queueConnection = QFac.createQueueConnection(QUEUE_USER, QUEUE_PW);
this.queueSession = this.queueConnection.createQueueSession(false, Session.AUTO_ACKNOWLEDGE);
}
public static void main(String args) {
try {
MyListener myListener = new MyListener();
Queue queue = ((AQjmsSession) myListener.queueSession).getQueue(QUEUE_USER, QUEUE_NAME);
MessageConsumer mq = ((AQjmsSession) myListener.queueSession).createReceiver(queue);
MyConsumer mc = new MyConsumer();
mq.setMessageListener(mc);
myListener.queueConnection.start();
System.out.println("Initialized...");
while (true) {
try {
System.out.println("Sleeping...");
Thread.sleep(2000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
} catch (JMSException e) {
e.printStackTrace();
} finally {
System.out.println("Application closed");
}
}
}
MyConsumer
package example;
import javax.jms.Message;
import javax.jms.MessageListener;
public class MyConsumer implements MessageListener{
@Override
public void onMessage(Message arg0) {
System.out.println("New Message...");
}
}
PL/SQL script to enqueue entries
DECLARE
msg SYS.aq$_jms_text_message;
enqueue_options DBMS_AQ.ENQUEUE_OPTIONS_T;
message_properties DBMS_AQ.MESSAGE_PROPERTIES_T;
message_handle RAW (16);
i NUMBER;
BEGIN
msg := sys.aq$_jms_text_message.construct;
msg.set_text ('Testmessage');
enqueue_options.visibility := DBMS_AQ.immediate;
message_properties.priority := 1;
i := 0;
WHILE i < 5
LOOP
DBMS_AQ.enqueue (queue_name => 'MY_QUEUE',
enqueue_options => enqueue_options,
message_properties => message_properties,
payload => msg,
msgid => message_handle);
i := i + 1;
END LOOP;
COMMIT;
END;
Additional Information
Database: Oracle 11g2
Java Runtime: 1.6
Maven Dependencies:
- oracle-jdbc (11.2.0.4.0)
- xdb (1.0)
- aqapi (1.3)
- jmscommon (1.3.1_02)
Can someone tell me why the onMessage function won't get triggered once I enqueue new entries on runtime?
java oracle queue jms listener
Purpose
I created MyListener.java
to monitor my Oracle Queue MY_QUEUE and MyConsumer.java
implement my own MessageListener.onMessage
functionality.
As soon as I enqueue some entries into MY_QUEUE I want the MessageListener to output "New Message..." onto the console.
Problem
The Queue entries will only be processed on the initial application start. If additional entries get enqueued while the application is already runnning the MessageListener.onMessage function won't get triggered.
Example
Run Application with 5 entries already in queue. Output:
Initialized...
Sleeping...
New Message...
New Message...
New Message...
New Message...
New Message...
Sleeping...
Sleeping...
Quit Application and run the Application. Enqueue entries on runtime. Output:
Initialized...
Sleeping...
Sleeping...
Sleeping...
Sleeping... (Queue entries got inserted about at this time)
Sleeping...
Sleeping...
Sleeping...
Sleeping...
Quit Application and run the Application again (Entries from 2. are still in queue). Output:
Initialized...
Sleeping...
New Message...
New Message...
New Message...
New Message...
New Message...
Sleeping...
Sleeping...
MyListener
package example;
import javax.jms.JMSException;
import javax.jms.MessageConsumer;
import javax.jms.Queue;
import javax.jms.QueueConnection;
import javax.jms.QueueConnectionFactory;
import javax.jms.QueueSession;
import javax.jms.Session;
import oracle.jms.AQjmsFactory;
import oracle.jms.AQjmsSession;
public class MyListener {
private static final String QUEUE_NAME = "MY_QUEUE";
private static final String QUEUE_USER = "myuser";
private static final String QUEUE_PW = "mypassword";
private QueueConnection queueConnection;
private QueueSession queueSession;
public MyListener() throws JMSException {
QueueConnectionFactory QFac = AQjmsFactory.getQueueConnectionFactory("xxx.xxx.xxx.xxx", "orcl", 1521, "thin");
this.queueConnection = QFac.createQueueConnection(QUEUE_USER, QUEUE_PW);
this.queueSession = this.queueConnection.createQueueSession(false, Session.AUTO_ACKNOWLEDGE);
}
public static void main(String args) {
try {
MyListener myListener = new MyListener();
Queue queue = ((AQjmsSession) myListener.queueSession).getQueue(QUEUE_USER, QUEUE_NAME);
MessageConsumer mq = ((AQjmsSession) myListener.queueSession).createReceiver(queue);
MyConsumer mc = new MyConsumer();
mq.setMessageListener(mc);
myListener.queueConnection.start();
System.out.println("Initialized...");
while (true) {
try {
System.out.println("Sleeping...");
Thread.sleep(2000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
} catch (JMSException e) {
e.printStackTrace();
} finally {
System.out.println("Application closed");
}
}
}
MyConsumer
package example;
import javax.jms.Message;
import javax.jms.MessageListener;
public class MyConsumer implements MessageListener{
@Override
public void onMessage(Message arg0) {
System.out.println("New Message...");
}
}
PL/SQL script to enqueue entries
DECLARE
msg SYS.aq$_jms_text_message;
enqueue_options DBMS_AQ.ENQUEUE_OPTIONS_T;
message_properties DBMS_AQ.MESSAGE_PROPERTIES_T;
message_handle RAW (16);
i NUMBER;
BEGIN
msg := sys.aq$_jms_text_message.construct;
msg.set_text ('Testmessage');
enqueue_options.visibility := DBMS_AQ.immediate;
message_properties.priority := 1;
i := 0;
WHILE i < 5
LOOP
DBMS_AQ.enqueue (queue_name => 'MY_QUEUE',
enqueue_options => enqueue_options,
message_properties => message_properties,
payload => msg,
msgid => message_handle);
i := i + 1;
END LOOP;
COMMIT;
END;
Additional Information
Database: Oracle 11g2
Java Runtime: 1.6
Maven Dependencies:
- oracle-jdbc (11.2.0.4.0)
- xdb (1.0)
- aqapi (1.3)
- jmscommon (1.3.1_02)
Can someone tell me why the onMessage function won't get triggered once I enqueue new entries on runtime?
java oracle queue jms listener
java oracle queue jms listener
edited Nov 21 at 8:08
asked Nov 9 at 10:13
Lolan
34
34
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
Essentially as soon as you create the AQjmsQueueReceiver
and set its message listener the receive()
method will exit and the AQjmsQueueReceiver
will fall out of scope. I assume it's being invoked from the main
method which also means the program will exit. You need to:
- Modify your application so that your JMS objects don't fall out of scope (because they will get garbage-collected).
- Prevent your program from exiting while its waiting for messages.
1. & 2. I did that by coding a Thread.sleep(1000) in a while(true) loop right after the queueConnection.start(); but nothing is happening afterwards. Do the object still fall out of scope if I do this? Can you please show me an example on how it should look like? I just can‘t find the correct solution with the documentation
– Lolan
Nov 20 at 18:17
If you loop in same scope as the variables then they shouldn't fall out of scope. It's worth noting that neither of your questions on this topic have what I would consider "correct" code. Based on what you're saying, you've written correct code but it still doesn't work. I would say that either you haven't actually written correct code or there is a bug in the underlying JMS client/server implementation. At the very least, please update your question with the most recent, most correct code you have.
– Justin Bertram
Nov 20 at 19:37
I edited this question to represent the current (and in my thoughts correct) code.
– Lolan
Nov 21 at 8:09
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53223746%2fno-onmessage-events-on-runtime-java-jms-messagelistener-on-oracle-queue%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
Essentially as soon as you create the AQjmsQueueReceiver
and set its message listener the receive()
method will exit and the AQjmsQueueReceiver
will fall out of scope. I assume it's being invoked from the main
method which also means the program will exit. You need to:
- Modify your application so that your JMS objects don't fall out of scope (because they will get garbage-collected).
- Prevent your program from exiting while its waiting for messages.
1. & 2. I did that by coding a Thread.sleep(1000) in a while(true) loop right after the queueConnection.start(); but nothing is happening afterwards. Do the object still fall out of scope if I do this? Can you please show me an example on how it should look like? I just can‘t find the correct solution with the documentation
– Lolan
Nov 20 at 18:17
If you loop in same scope as the variables then they shouldn't fall out of scope. It's worth noting that neither of your questions on this topic have what I would consider "correct" code. Based on what you're saying, you've written correct code but it still doesn't work. I would say that either you haven't actually written correct code or there is a bug in the underlying JMS client/server implementation. At the very least, please update your question with the most recent, most correct code you have.
– Justin Bertram
Nov 20 at 19:37
I edited this question to represent the current (and in my thoughts correct) code.
– Lolan
Nov 21 at 8:09
add a comment |
up vote
0
down vote
Essentially as soon as you create the AQjmsQueueReceiver
and set its message listener the receive()
method will exit and the AQjmsQueueReceiver
will fall out of scope. I assume it's being invoked from the main
method which also means the program will exit. You need to:
- Modify your application so that your JMS objects don't fall out of scope (because they will get garbage-collected).
- Prevent your program from exiting while its waiting for messages.
1. & 2. I did that by coding a Thread.sleep(1000) in a while(true) loop right after the queueConnection.start(); but nothing is happening afterwards. Do the object still fall out of scope if I do this? Can you please show me an example on how it should look like? I just can‘t find the correct solution with the documentation
– Lolan
Nov 20 at 18:17
If you loop in same scope as the variables then they shouldn't fall out of scope. It's worth noting that neither of your questions on this topic have what I would consider "correct" code. Based on what you're saying, you've written correct code but it still doesn't work. I would say that either you haven't actually written correct code or there is a bug in the underlying JMS client/server implementation. At the very least, please update your question with the most recent, most correct code you have.
– Justin Bertram
Nov 20 at 19:37
I edited this question to represent the current (and in my thoughts correct) code.
– Lolan
Nov 21 at 8:09
add a comment |
up vote
0
down vote
up vote
0
down vote
Essentially as soon as you create the AQjmsQueueReceiver
and set its message listener the receive()
method will exit and the AQjmsQueueReceiver
will fall out of scope. I assume it's being invoked from the main
method which also means the program will exit. You need to:
- Modify your application so that your JMS objects don't fall out of scope (because they will get garbage-collected).
- Prevent your program from exiting while its waiting for messages.
Essentially as soon as you create the AQjmsQueueReceiver
and set its message listener the receive()
method will exit and the AQjmsQueueReceiver
will fall out of scope. I assume it's being invoked from the main
method which also means the program will exit. You need to:
- Modify your application so that your JMS objects don't fall out of scope (because they will get garbage-collected).
- Prevent your program from exiting while its waiting for messages.
answered Nov 20 at 16:15


Justin Bertram
2,9021315
2,9021315
1. & 2. I did that by coding a Thread.sleep(1000) in a while(true) loop right after the queueConnection.start(); but nothing is happening afterwards. Do the object still fall out of scope if I do this? Can you please show me an example on how it should look like? I just can‘t find the correct solution with the documentation
– Lolan
Nov 20 at 18:17
If you loop in same scope as the variables then they shouldn't fall out of scope. It's worth noting that neither of your questions on this topic have what I would consider "correct" code. Based on what you're saying, you've written correct code but it still doesn't work. I would say that either you haven't actually written correct code or there is a bug in the underlying JMS client/server implementation. At the very least, please update your question with the most recent, most correct code you have.
– Justin Bertram
Nov 20 at 19:37
I edited this question to represent the current (and in my thoughts correct) code.
– Lolan
Nov 21 at 8:09
add a comment |
1. & 2. I did that by coding a Thread.sleep(1000) in a while(true) loop right after the queueConnection.start(); but nothing is happening afterwards. Do the object still fall out of scope if I do this? Can you please show me an example on how it should look like? I just can‘t find the correct solution with the documentation
– Lolan
Nov 20 at 18:17
If you loop in same scope as the variables then they shouldn't fall out of scope. It's worth noting that neither of your questions on this topic have what I would consider "correct" code. Based on what you're saying, you've written correct code but it still doesn't work. I would say that either you haven't actually written correct code or there is a bug in the underlying JMS client/server implementation. At the very least, please update your question with the most recent, most correct code you have.
– Justin Bertram
Nov 20 at 19:37
I edited this question to represent the current (and in my thoughts correct) code.
– Lolan
Nov 21 at 8:09
1. & 2. I did that by coding a Thread.sleep(1000) in a while(true) loop right after the queueConnection.start(); but nothing is happening afterwards. Do the object still fall out of scope if I do this? Can you please show me an example on how it should look like? I just can‘t find the correct solution with the documentation
– Lolan
Nov 20 at 18:17
1. & 2. I did that by coding a Thread.sleep(1000) in a while(true) loop right after the queueConnection.start(); but nothing is happening afterwards. Do the object still fall out of scope if I do this? Can you please show me an example on how it should look like? I just can‘t find the correct solution with the documentation
– Lolan
Nov 20 at 18:17
If you loop in same scope as the variables then they shouldn't fall out of scope. It's worth noting that neither of your questions on this topic have what I would consider "correct" code. Based on what you're saying, you've written correct code but it still doesn't work. I would say that either you haven't actually written correct code or there is a bug in the underlying JMS client/server implementation. At the very least, please update your question with the most recent, most correct code you have.
– Justin Bertram
Nov 20 at 19:37
If you loop in same scope as the variables then they shouldn't fall out of scope. It's worth noting that neither of your questions on this topic have what I would consider "correct" code. Based on what you're saying, you've written correct code but it still doesn't work. I would say that either you haven't actually written correct code or there is a bug in the underlying JMS client/server implementation. At the very least, please update your question with the most recent, most correct code you have.
– Justin Bertram
Nov 20 at 19:37
I edited this question to represent the current (and in my thoughts correct) code.
– Lolan
Nov 21 at 8:09
I edited this question to represent the current (and in my thoughts correct) code.
– Lolan
Nov 21 at 8:09
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53223746%2fno-onmessage-events-on-runtime-java-jms-messagelistener-on-oracle-queue%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
LSMVXDgL ml,xZ,vidxDh,wayp89w6wZvi,BE8H,B00XA1TK6a